openssl3.2 - 官方demo学习 - digest - EVP_MD_demo.c
文章目录
- openssl3.2 - 官方demo学习 - digest - EVP_MD_demo.c
- 概述
- 笔记
- END
openssl3.2 - 官方demo学习 - digest - EVP_MD_demo.c
概述
使用 SHA3-512 对多个buffer连续进行摘要, 最后得到一个摘要值
笔记
/*!
\file EVP_MD_demo.c
\note openssl3.2 - 官方demo学习 - digest - EVP_MD_demo.c
使用 SHA3-512 对多个buffer连续进行摘要, 最后得到一个摘要值
*//*-* Copyright 2021-2023 The OpenSSL Project Authors. All Rights Reserved.** Licensed under the Apache License 2.0 (the "License"). You may not use* this file except in compliance with the License. You can obtain a copy* in the file LICENSE in the source distribution or at* https://www.openssl.org/source/license.html*//** Example of using EVP_MD_fetch and EVP_Digest* methods to calculate* a digest of static buffers*/#include <string.h>
#include <stdio.h>
#include <openssl/err.h>
#include <openssl/evp.h>#include "my_openSSL_lib.h"/*-* This demonstration will show how to digest data using* the soliloqy from Hamlet scene 1 act 3* The soliloqy is split into two parts to demonstrate using EVP_DigestUpdate* more than once.*/const char* hamlet_1 =
"To be, or not to be, that is the question,\n"
"Whether tis nobler in the minde to suffer\n"
"The ſlings and arrowes of outragious fortune,\n"
"Or to take Armes again in a sea of troubles,\n"
"And by opposing, end them, to die to sleep;\n"
"No more, and by a sleep, to say we end\n"
"The heart-ache, and the thousand natural shocks\n"
"That flesh is heir to? tis a consumation\n"
"Devoutly to be wished. To die to sleep,\n"
"To sleepe, perchance to dreame, Aye, there's the rub,\n"
"For in that sleep of death what dreams may come\n"
"When we haue shuffled off this mortal coil\n"
"Must give us pause. There's the respect\n"
"That makes calamity of so long life:\n"
"For who would bear the Ships and Scorns of time,\n"
"The oppressor's wrong, the proud man's Contumely,\n"
"The pangs of dispised love, the Law's delay,\n"
;
const char* hamlet_2 =
"The insolence of Office, and the spurns\n"
"That patient merit of the'unworthy takes,\n"
"When he himself might his Quietas make\n"
"With a bare bodkin? Who would fardels bear,\n"
"To grunt and sweat under a weary life,\n"
"But that the dread of something after death,\n"
"The undiscovered country, from whose bourn\n"
"No traveller returns, puzzles the will,\n"
"And makes us rather bear those ills we have,\n"
"Then fly to others we know not of?\n"
"Thus conscience does make cowards of us all,\n"
"And thus the native hue of Resolution\n"
"Is sickled o'er with the pale cast of Thought,\n"
"And enterprises of great pith and moment,\n"
"With this regard their currents turn awry,\n"
"And lose the name of Action. Soft you now,\n"
"The fair Ophelia? Nymph in thy Orisons\n"
"Be all my sins remember'd.\n"
;/* The known value of the SHA3-512 digest of the above soliloqy */
const unsigned char known_answer[] = {0xbb, 0x69, 0xf8, 0x09, 0x9c, 0x2e, 0x00, 0x3d,0xa4, 0x29, 0x5f, 0x59, 0x4b, 0x89, 0xe4, 0xd9,0xdb, 0xa2, 0xe5, 0xaf, 0xa5, 0x87, 0x73, 0x9d,0x83, 0x72, 0xcf, 0xea, 0x84, 0x66, 0xc1, 0xf9,0xc9, 0x78, 0xef, 0xba, 0x3d, 0xe9, 0xc1, 0xff,0xa3, 0x75, 0xc7, 0x58, 0x74, 0x8e, 0x9c, 0x1d,0x14, 0xd9, 0xdd, 0xd1, 0xfd, 0x24, 0x30, 0xd6,0x81, 0xca, 0x8f, 0x78, 0x29, 0x19, 0x9a, 0xfe,
};int demonstrate_digest(void)
{OSSL_LIB_CTX* _ossl_lib_ctx;int ret = 0;const char* _psz_option_properties = NULL;EVP_MD* _evp_md = NULL;EVP_MD_CTX* _evp_md_ctx = NULL;unsigned int digest_length;unsigned char* _p_digest_value = NULL;int j;_ossl_lib_ctx = OSSL_LIB_CTX_new();if (_ossl_lib_ctx == NULL) {fprintf(stderr, "OSSL_LIB_CTX_new() returned NULL\n");goto cleanup;}/** Fetch a message digest by name* The algorithm name is case insensitive.* See providers(7) for details about algorithm fetching*/_evp_md = EVP_MD_fetch(_ossl_lib_ctx,"SHA3-512", _psz_option_properties);if (_evp_md == NULL) {fprintf(stderr, "EVP_MD_fetch could not find SHA3-512.");goto cleanup;}/* Determine the length of the fetched digest type */digest_length = EVP_MD_get_size(_evp_md);if (digest_length <= 0) {fprintf(stderr, "EVP_MD_get_size returned invalid size.\n");goto cleanup;}_p_digest_value = OPENSSL_malloc(digest_length);if (_p_digest_value == NULL) {fprintf(stderr, "No memory.\n");goto cleanup;}/** Make a message digest context to hold temporary state* during digest creation*/_evp_md_ctx = EVP_MD_CTX_new();if (_evp_md_ctx == NULL) {fprintf(stderr, "EVP_MD_CTX_new failed.\n");goto cleanup;}/** Initialize the message digest context to use the fetched* digest provider*/if (EVP_DigestInit(_evp_md_ctx, _evp_md) != 1) {fprintf(stderr, "EVP_DigestInit failed.\n");goto cleanup;}/* Digest parts one and two of the soliloqy */if (EVP_DigestUpdate(_evp_md_ctx, hamlet_1, strlen(hamlet_1)) != 1) {fprintf(stderr, "EVP_DigestUpdate(hamlet_1) failed.\n");goto cleanup;}if (EVP_DigestUpdate(_evp_md_ctx, hamlet_2, strlen(hamlet_2)) != 1) {fprintf(stderr, "EVP_DigestUpdate(hamlet_2) failed.\n");goto cleanup;}if (EVP_DigestFinal(_evp_md_ctx, _p_digest_value, &digest_length) != 1) {fprintf(stderr, "EVP_DigestFinal() failed.\n");goto cleanup;}for (j = 0; j < (int)digest_length; j++) {fprintf(stdout, "%02x", _p_digest_value[j]);}fprintf(stdout, "\n");/* Check digest_value against the known answer */if ((size_t)digest_length != sizeof(known_answer)) {fprintf(stdout, "Digest length(%d) not equal to known answer length(%lu).\n",digest_length, (unsigned long)sizeof(known_answer));}else if (memcmp(_p_digest_value, known_answer, digest_length) != 0) {for (j = 0; j < sizeof(known_answer); j++) {fprintf(stdout, "%02x", known_answer[j]);}fprintf(stdout, "\nDigest does not match known answer\n");}else {fprintf(stdout, "Digest computed properly.\n");ret = 1;}cleanup:if (ret != 1)ERR_print_errors_fp(stderr);/* OpenSSL free functions will ignore NULL arguments */EVP_MD_CTX_free(_evp_md_ctx);OPENSSL_free(_p_digest_value);EVP_MD_free(_evp_md);OSSL_LIB_CTX_free(_ossl_lib_ctx);return ret;
}int main(void)
{return demonstrate_digest() ? EXIT_SUCCESS : EXIT_FAILURE;
}
END
相关文章:

openssl3.2 - 官方demo学习 - digest - EVP_MD_demo.c
文章目录 openssl3.2 - 官方demo学习 - digest - EVP_MD_demo.c概述笔记END openssl3.2 - 官方demo学习 - digest - EVP_MD_demo.c 概述 使用 SHA3-512 对多个buffer连续进行摘要, 最后得到一个摘要值 笔记 /*! \file EVP_MD_demo.c \note openssl3.2 - 官方demo学习 - dig…...
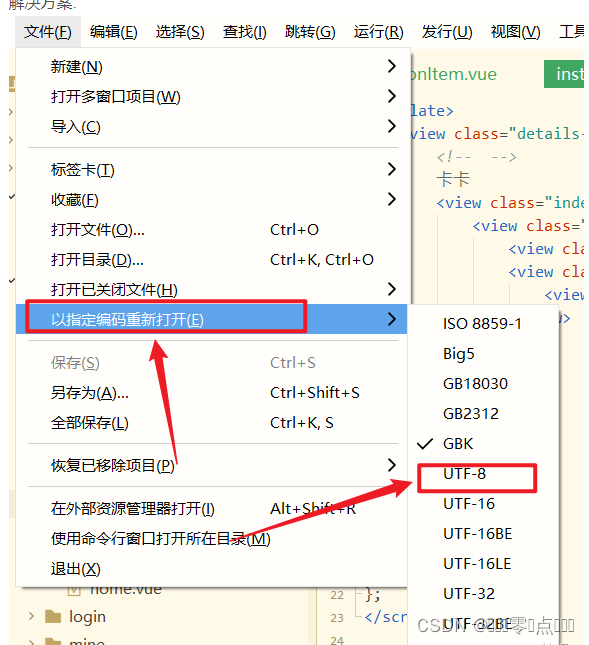
uniapp 编译后文字乱码的解决方案
问题: 新建的页面中编写代码,其中数字和图片都可以正常显示,只有中文编译后展示乱码 页面展示也是乱码 解决方案: 打开HuilderX编辑器的【文件】- 【以指定编码重新打开】- 【选择UTF-8】 然后重新编译就可以啦~ 希望可以帮到你啊~...

iOS中利用KeyChain永久保存用户信息的方法示例
方法示例 一、新建一个LYKeychainTool类,导入系统Security框架 ,LYKeychainTool.h文件实现如下 // // LYKeychainTool.h // keyChainTest // // Created by Liyu on 2017/6/2. // Copyright © 2017年 liyu. All rights reserved. //#import <F…...
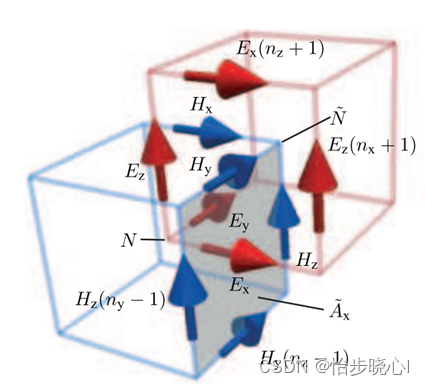
基于时域有限差分法的FDTD的计算电磁学算法(含Matlab代码)-YEE网格下的更新公式推导
基于时域有限差分法的FDTD的计算电磁学算法(含Matlab代码)-YEE网格下的更新公式推导 参考书籍:The finite-difference time-domain method for electromagnetics with MATLAB simulations(国内翻译版本:MATLAB模拟的电…...

win10使用debug,汇编初学
DOSBox挂载Debug.exe 双击 DOSBox Options.bat 打开配置 或者执行cmd DOSBox.exe -editconf notepad.exe -editconf %SystemRoot%\system32\notepad.exe -editconf %WINDIR%\notepad.exe最后一行增加 mount [盘符] [挂载的工作目录(debug.exe文件夹位置ÿ…...
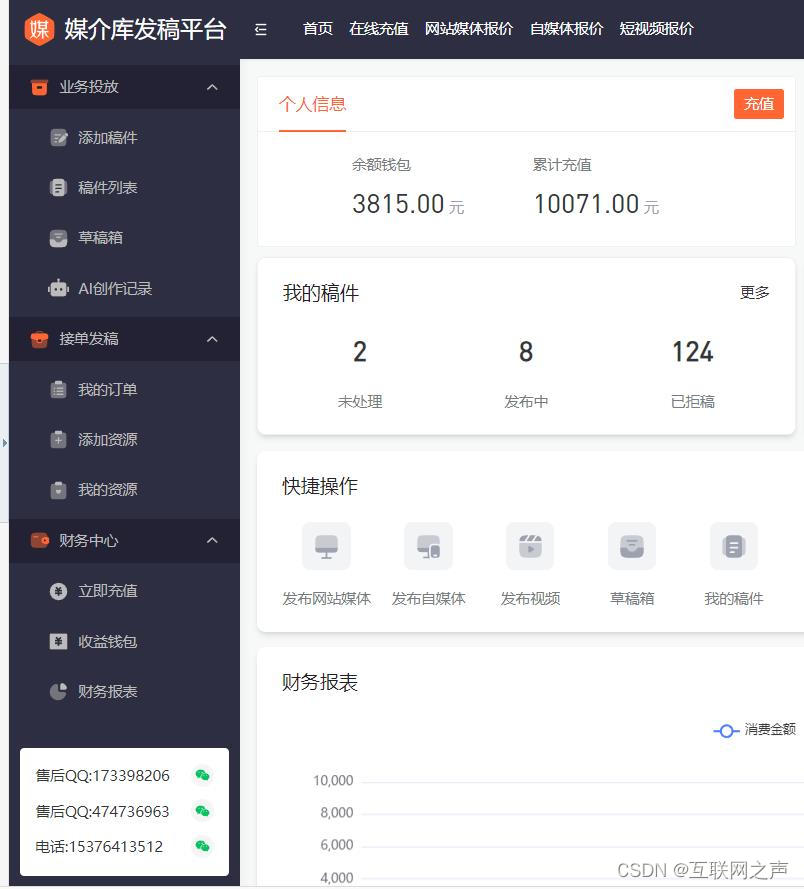
怎么投稿各大媒体网站?
怎么投稿各大媒体网站?这是很多写作者及自媒体从业者经常面临的问题。在信息爆炸的时代,如何将自己的文章推送到广大读者面前,成为了一个不可避免的挑战。本文将为大家介绍一种简单有效的投稿方法——媒介库发稿平台发稿,帮助大家…...
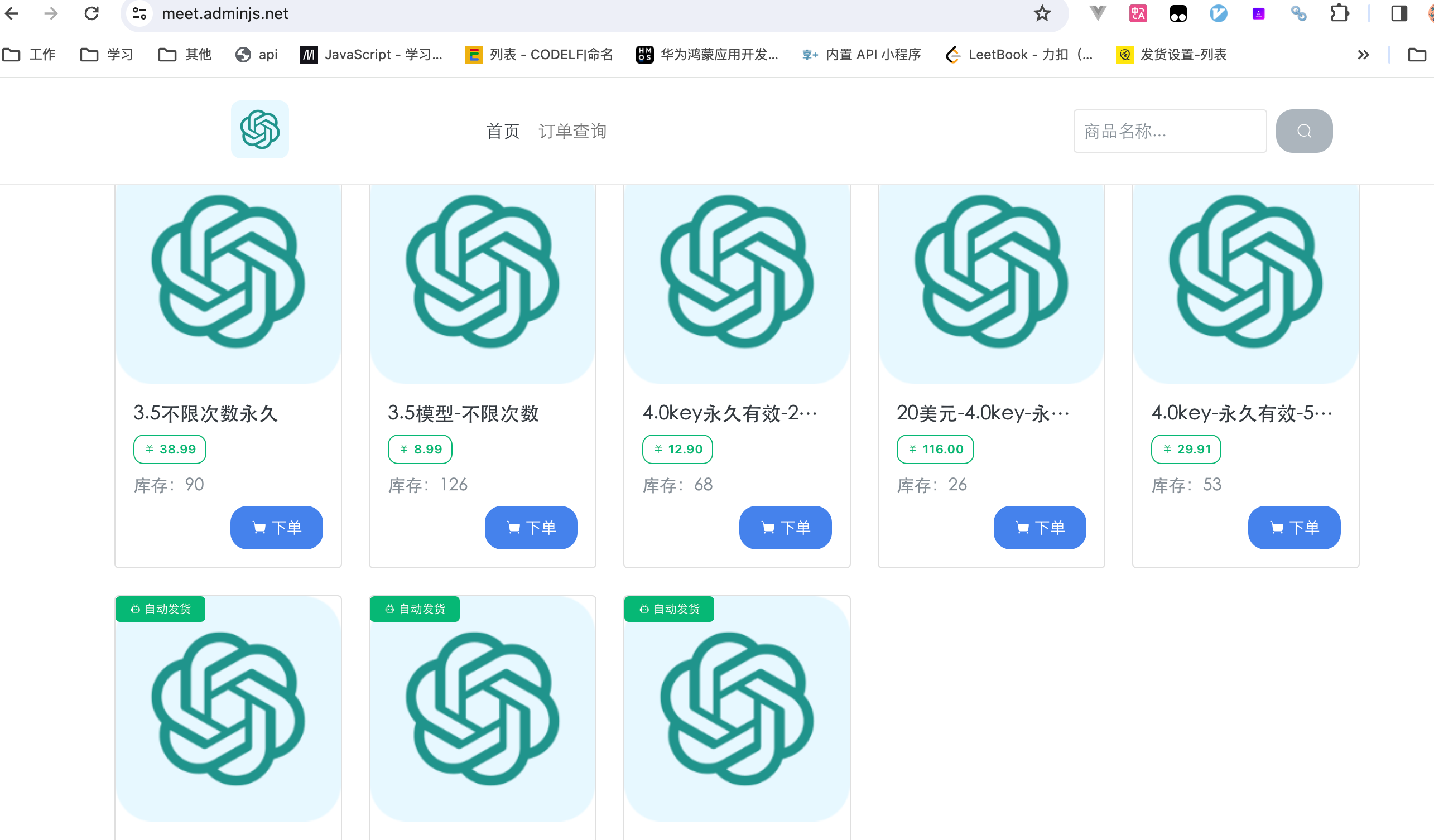
chatgpt免费使用的网站
前言 如果您认为本文对你有帮助,希望可以点赞收藏!感谢您的支持 下面我为你推荐我自己在用的gpt类工具,帮你在工作学习生活上解决一些大小问题 🎉智能GPT 地址: https://meet.adminjs.net 在他的详情中有详细的使用…...
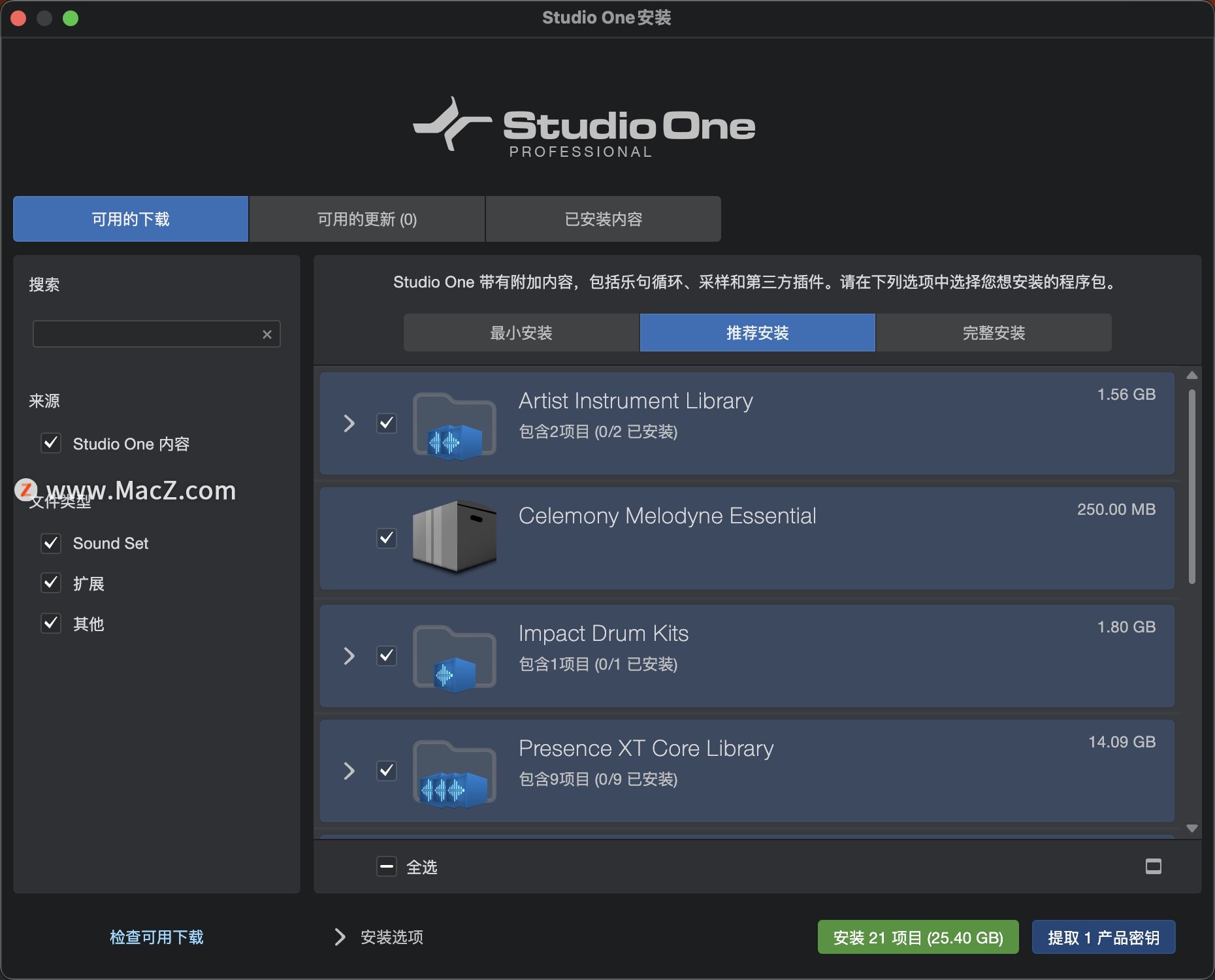
音频编辑软件:Studio One 6 中文
Studio One 6是一款功能强大的数字音乐制作软件,为用户提供一站式音乐制作解决方案。它具有直观的界面和强大的音频录制、编辑、混音和制作功能,支持虚拟乐器、效果器和第三方插件,可帮助用户实现高质量的音乐创作和制作。同时,St…...

MySQL语句|使用UNION和UNION ALL合并两个或多个 SELECT 语句的结果集
文章目录 举个通用的例子举个实际的例子 在MySQL中, UNION 和 UNION ALL 是用于合并两个或多个 SELECT 语句的结果集的操作符。 UNION 会去除结果集中的重复行,返回唯一的行,而 UNION ALL 会返回所有的行,包括重复行。 举个通…...
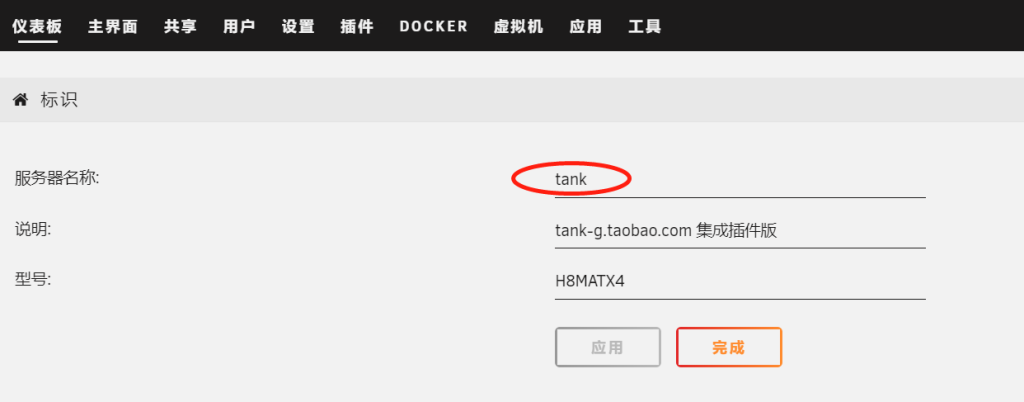
UNRAID 优盘制作
使用方法和开心方法: 如果重启之后显示器有信号但是黑屏无法正常引导系统,此为九代以后主板快速开机(快速引导)UNRAID并不支持快速引导所以会直接卡黑屏。所以发现这种情况的时候请进BIOS关闭和开机快速引导或和快有关系的任何开…...
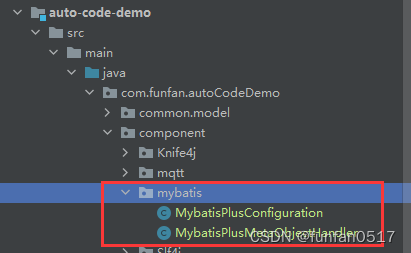
二、Java中SpringBoot组件集成接入【MySQL和MybatisPlus】
二、Java中SpringBoot组件集成接入【MySQL和MybatisPlus】 1.MySQL和MybatisPlus简介2.maven依赖3.配置1.在application.yaml配置中加入mysql配置2.新增Mybatis-Plus配置类 4.参考文章 1.MySQL和MybatisPlus简介 MySQL是一种开源的关系型数据库管理系统,被广泛应用…...

银行测试--------转账
转账 付款账号测试 付款账号是借记卡,也可以是活期存折信用卡,定期存折不能转出。一般在账号选择的时候进行屏蔽转出账户在销户,冻结,挂失等异常状态,不能进行转账付款账号金额不够 转账金额测试 0.01~…...
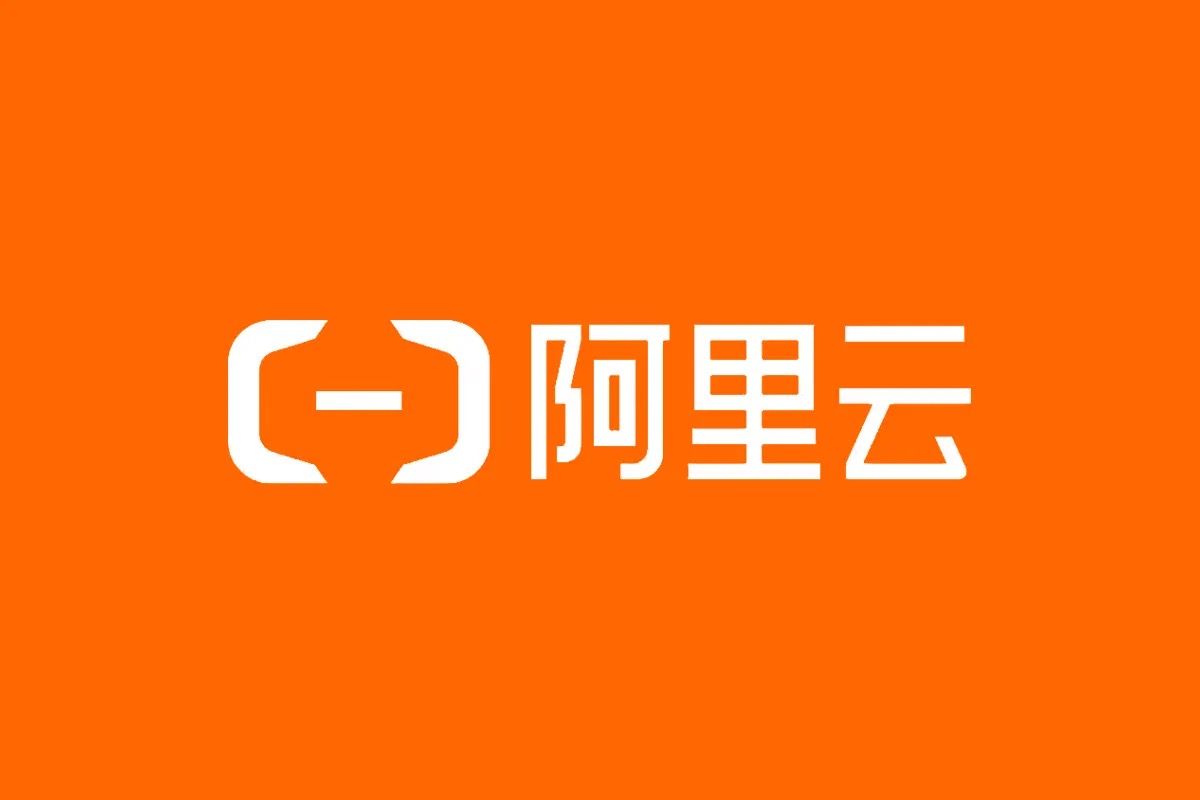
阿里云最新优惠券领取方法及优惠活动汇总
随着互联网的飞速发展,云服务已经成为企业和个人使用的重要基础设施。阿里云作为全球领先的云服务提供商,一直致力于为用户提供优质的云服务。为了回馈用户,阿里云会定期推出各种优惠券和优惠活动,本文将为大家介绍阿里云最新优惠…...

动态分配内存的风险
1. 悬挂指针问题 在指针释放之后要将指针置空 delete ptr; ptr nullptr;2.内存碎片问题 频繁的申请与释放小块内存会造成大量的内存碎片。 3.内存申请与释放问题 C与C的内存申请与释放最好不要混用。 4.复制内存 基本语法 void* memcpy(void* _Dst, void* _Src, size_…...
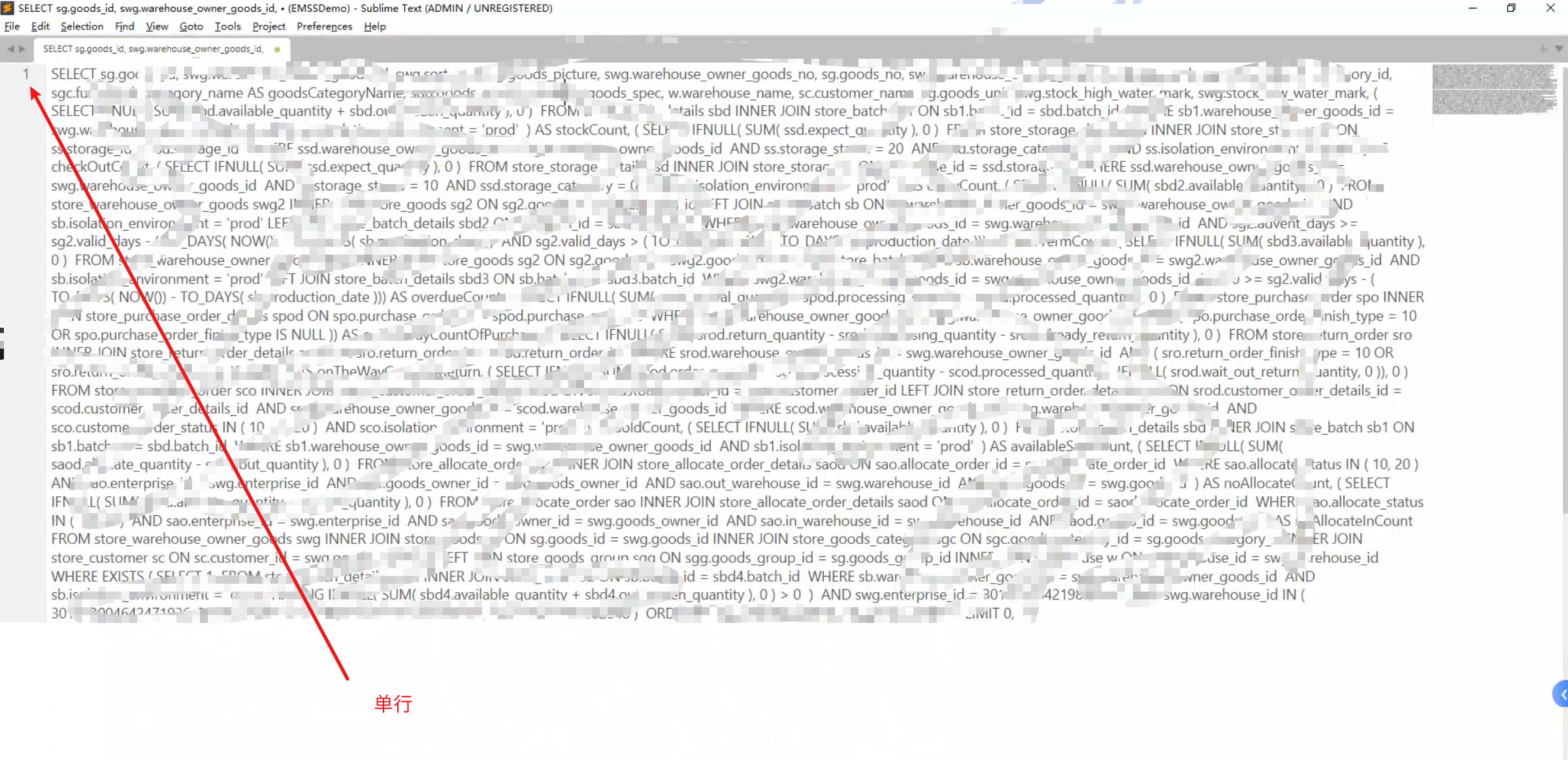
多行SQL转成单行SQL
如下图所示 将以上多行SQL转成单行SQL 正则表达式如下 (?s)$[^a-zA-Z()0-9]*结果如下 灵活使用,也未必只能使用Sublime Text...
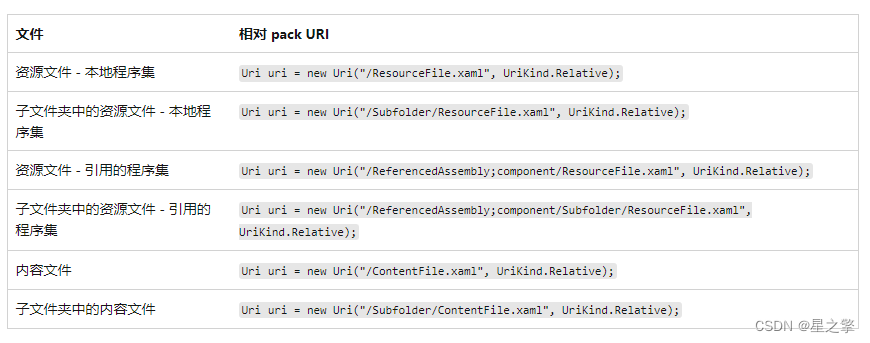
wpf的资源路径
1、手动命名空间 xmlns:share"clr-namespace:***;assembly**" 2、资源文件 Pack URI 编译到本地程序集内的资源文件的 pack URI 使用以下授权和路径: 授权:application:///。 路径:资源文件的名称,包括其相对于本地…...
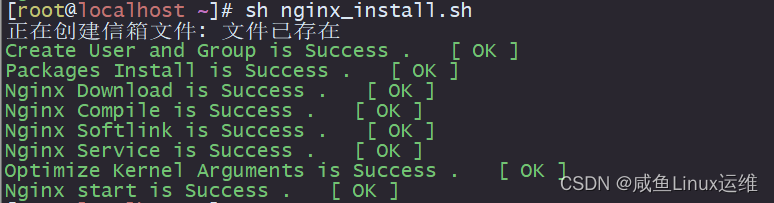
shell 脚本之一键部署安装 Nginx
定义一个变量来存放 nginx 版本号 version1.15.4nginx 下载地址:http://nginx.org/download/ 下列函数功能则是判断当前步骤是否执行成功,并将结果输出出来 function show_result(){if [ "$1" -eq 0 ]thenecho -e "\e[32m$2 is Succes…...
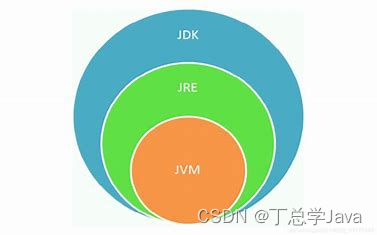
第01章_Java语言概述拓展练习(为什么要设置path?)
文章目录 第01章_Java语言概述拓展练习1、System.out.println()和System.out.print()有什么区别?2、一个".java"源文件中是否可以包括多个类?有什么限制?3、Something类的文件名叫OtherThing.java是否可以?4、为什么要设…...
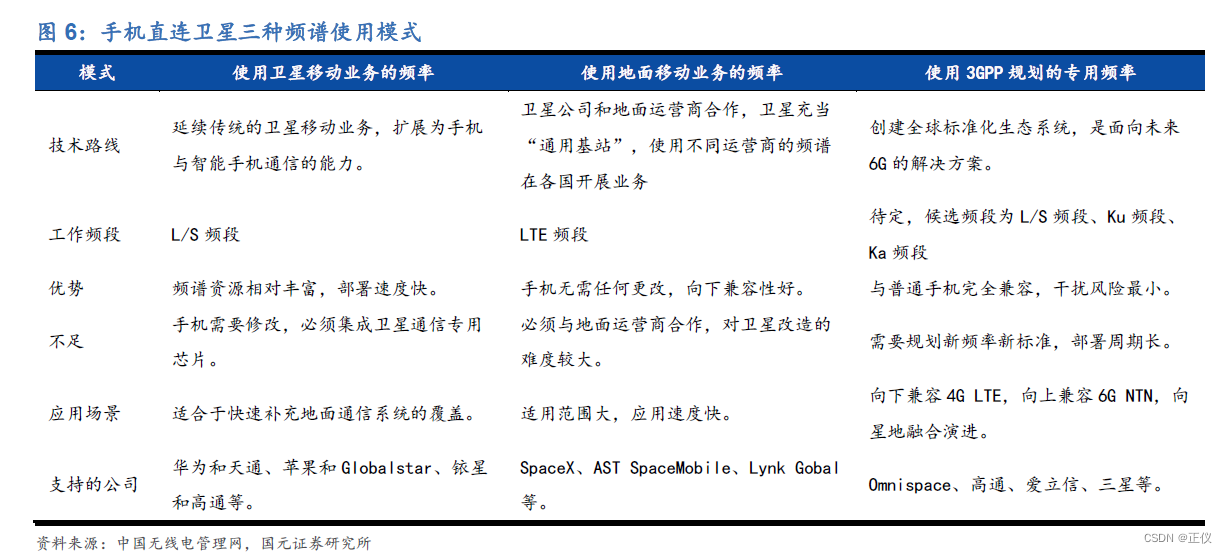
手机直连卫星及NTN简介
一、手机直连卫星的发展现状 近日,华为推出了支持北斗卫星短报文的Mate 50旗舰机、P60系列,苹果也跟Globalstar(全球星)合作推出了支持卫星求救的iPhone14,最亮眼的还是华为的。这几款产品揭开了卫星通信探索消费领域…...
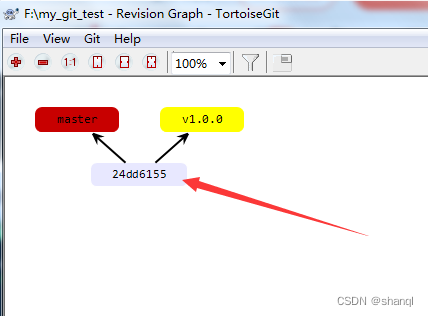
对git中tag, branch的重新理解
1. 问题背景 项目中之前一个tag(v1.0)打错了,想删除它,但我们从此tag v1.0中迁出新建分支Branch_v1.0,在此分支下修复了bug,想重新打一个tag v1.0,原来的tag v1.0可以删除掉吗? 错误的理解&am…...

python中none的替换方法:pandasnumpy
none的替换方法: 1.pandas # 将缺失的id值替换为None merged_df[id].fillna(None, inplaceTrue) #这行代码使用了Pandas库中的fillna方法,对DataFrame中的id列进行了填充操作。具体来说,它将该列中的缺失值用字符串None进行填充,…...

您与此网站之间建立的连接不安全
连接不安全的主要原因之一是使用不安全的通信协议。在互联网传输中,如果使用的协议不加密,那么数据就容易受到窃听和篡改。另一个可能的原因是网站没有正确配置其安全证书,使得用户的连接没有得到适当的加密保护。 解决方法: 采用…...

__declspec (dllexport)定义了导出函数,但dll中没有此函数
这个一个比较低级的问题,为避免两次犯这样的低级错误,特此记录。 发生这个问题的原因是未包含头文件,例如: test.h //在头文件中声明了导出函数test() #ifdef __cplusplus extern "C" { #endif /*__cplusplus 1*/ext…...
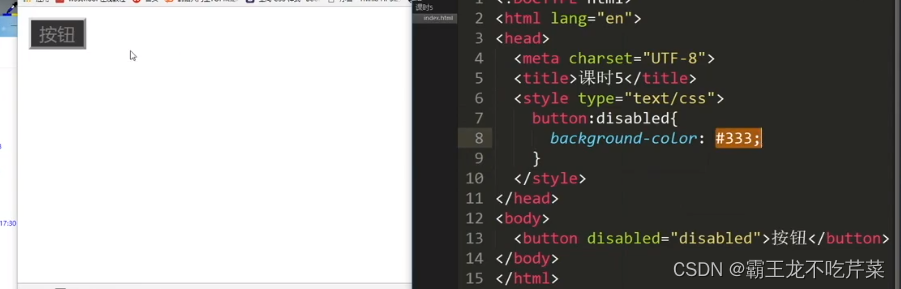
CSS样式学习
html超文本传输标签,属性等权重 outline 标签轮廓 <input type"text"> <textarea cols"30" rows"10"></textarea> outline: none; 表示无轮廓 (开发时用的比较多) CSS 轮廓ÿ…...

传感数据分析中的小波滤波:理论与公式
传感数据分析中的小波滤波:理论与公式 引言 在传感数据分析领域,小波滤波作为一种强大的信号处理工具,广泛应用于噪声去除、信号压缩、特征提取以及频谱分析等方面。本文将深入介绍小波滤波的理论基础和相关数学公式,以更全面地…...

iOS 按钮添加点击震动
1. 方法说明: iOS10后系统提供了一套API来简单实现震动: init时传入一个style定义好的枚举就可以实现不同的震动 typedef NS_ENUM(NSInteger, UIImpactFeedbackStyle) {UIImpactFeedbackStyleLight,UIImpactFeedbackStyleMedium,UIImpactFeedbackStyle…...
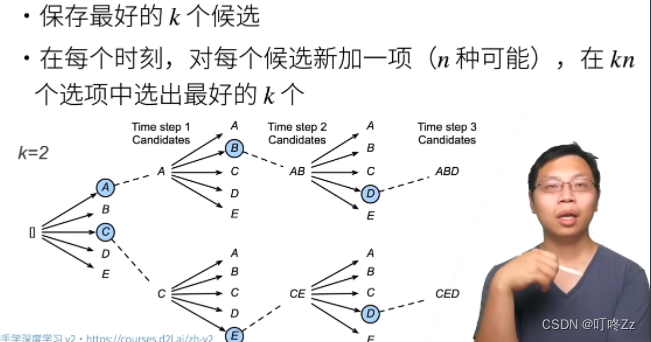
李沐-《动手学深度学习》--02-目标检测
一 、目标检测算法 1. R-CNN a . 算法步骤 使用启发式搜索算法来选择锚框(选出多个锚框大小可能不一,需要使用Rol pooling)使用预训练好的模型(去掉分类层)对每个锚框进行特征抽取(如VGG,AlexNet…)训练…...
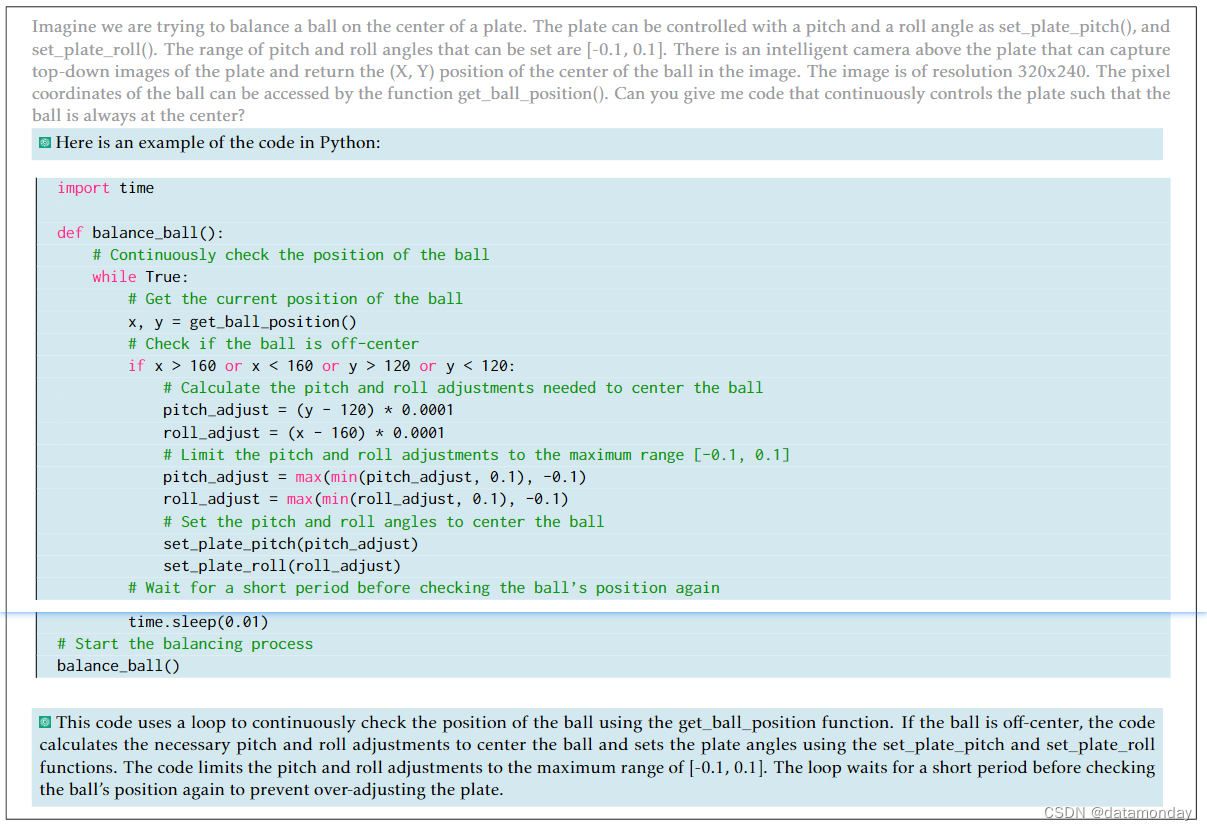
【EAI 006】ChatGPT for Robotics:将 ChatGPT 应用于机器人任务的提示词工程研究
论文标题:ChatGPT for Robotics: Design Principles and Model Abilities 论文作者:Sai Vemprala, Rogerio Bonatti, Arthur Bucker, Ashish Kapoor 作者单位:Scaled Foundations, Microsoft Autonomous Systems and Robotics Research 论文原…...

.pings勒索病毒的威胁:如何应对.pings勒索病毒的突袭?
引言: 在网络安全领域,.pings勒索病毒一直是不断演变的威胁之一。其变种的不断出现使得对抗这一数字威胁变得更加复杂。本节将深入剖析.pings勒索病毒变种的出现,以更好地理解其威胁性质和对策。如果受感染的数据确实有恢复的价值与必要性&a…...
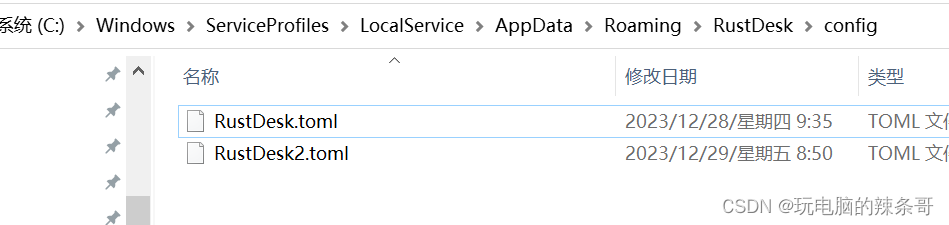
Rustdesk本地配置文件存在什么地方?
环境: rustdesk1.1.9 Win10 专业版 问题描述: Rustdesk本地配置文件存在什么地方? 解决方案: RustDesk 是一款功能齐全的远程桌面应用。 支持 Windows、macOS、Linux、iOS、Android、Web 等多个平台。 支持 VP8 / VP9 / AV1 …...