轻量封装WebGPU渲染系统示例<51>- 视差贴图(Parallax Map)(源码)
视差纹理是一种片段着色阶段增强材质表面凹凸细节的技术。
这里在WebGPU的实时渲染材质管线中实现了视差贴图计算,以便增强相关的纹理细节表现力。
当前示例源码github地址:
https://github.com/vilyLei/voxwebgpu/blob/feature/material/src/voxgpu/sample/ParallaxTexTest.ts
当前示例运行效果:
此示例基于此渲染系统实现,当前示例TypeScript源码如下:
export class ParallaxTexTest {private mRscene = new RendererScene();initialize(): void {console.log("ParallaxTexTest::initialize() ...");this.loadImg();}initSys(): void {this.mRscene.initialize({canvasWith: 512,canvasHeight: 512,mtplEnabled: true,rpassparam:{multisampled: true}});this.initScene();this.initEvent();}private mPixels: Uint8ClampedArray;private mPixelsW = 128;private mPixelsH = 128;getRandomColor(s?: number): ColorDataType {if (s === undefined) {s = 1.0;}let i = 5;let j = Math.floor(Math.random() * this.mPixelsW);let k = i * this.mPixelsW + j;let vs = this.mPixels;k *= 4;let cs = [s * vs[k] / 255.0, s * vs[k + 1] / 255.0, s * vs[k + 2] / 255.0];return cs;}private loadImg(): void {let img = new Image();img.onload = evt => {this.mPixelsW = img.width;this.mPixelsH = img.height;let canvas = document.createElement("canvas");canvas.width = img.width;canvas.height = img.height;let ctx = canvas.getContext('2d');ctx.drawImage(img, 0, 0);this.mPixels = ctx.getImageData(0, 0, img.width, img.height).data;this.initSys();}img.src = 'static/assets/colorPalette.jpg';}private mLightData: MtLightDataDescriptor;private createLightData(): MtLightDataDescriptor {let ld = { pointLights: [], directionLights: [], spotLights: [] } as MtLightDataDescriptor;let total = 5;let scale = 3.0;for (let i = 0; i < total; ++i) {for (let j = 0; j < total; ++j) {let position = [-500 + 250 * j, 290 + Math.random() * 30, -500 + 250 * i];position[0] += Math.random() * 60 - 30;position[2] += Math.random() * 60 - 30;let color = this.getRandomColor(scale);let factor1 = 0.00001;let factor2 = 0.00002;let pLight = new PointLight({ color, position, factor1, factor2 });ld.pointLights.push(pLight);if (Math.random() > 0.5) {position = [-500 + 150 * j, 290 + Math.random() * 50, -500 + 150 * i];position[0] += Math.random() * 160 - 80;position[2] += Math.random() * 160 - 80;color = this.getRandomColor(scale);let direction = [(Math.random() - 0.5) * 8, -1, (Math.random() - 0.5) * 8];let degree = Math.random() * 10 + 5;let spLight = new SpotLight({ position, color, direction, degree, factor1, factor2 });ld.spotLights.push(spLight);}}}let dLight = new DirectionLight({ color: [0.5, 0.5, 0.5], direction: [-1, -1, 0] });ld.directionLights.push(dLight);return ld;}private createBillboard(pv: Vector3DataType, c: ColorDataType, type: number): void {let rc = this.mRscene;let diffuseTex0 = { diffuse: { url: "static/assets/flare_core_03.jpg" } };if (type > 1) {diffuseTex0 = { diffuse: { url: "static/assets/circleWave_disp.png" } };}let billboard = new BillboardEntity({ size: 10, textures: [diffuseTex0] });billboard.color = c;billboard.alpha = 1;billboard.transform.setPosition(pv);rc.addEntity(billboard);}private createBillboards(): void {let lightData = this.mLightData;let pls = lightData.pointLights;for (let i = 0; i < pls.length; i++) {let lp = pls[i];this.createBillboard(lp.position, lp.color, 1);}let spls = lightData.spotLights;for (let i = 0; i < spls.length; i++) {let lp = spls[i];this.createBillboard(lp.position, lp.color, 2);}}private initScene(): void {let rc = this.mRscene;let mtpl = rc.renderer.mtpl;this.mLightData = this.createLightData();mtpl.light.lightData = this.mLightData;mtpl.shadow.param.intensity = 0.4;mtpl.shadow.param.radius = 4;let position = [-30, 220, -50];let materials = this.createMaterials(true);let sphere: SphereEntity;let total = 3;let py = 150;for (let i = 0; i < total; ++i) {for (let j = 0; j < total; ++j) {if (total > 2) {position = [-350 + 350 * j, py, -350 + 350 * i];} else {position = [0, py, 0];}let rotation = [0, Math.random() * 360, 0];let materials = this.createMaterials(true);if (sphere) {let sph = new SphereEntity({geometry: sphere.geometry,materials,transform: { position, rotation }});rc.addEntity(sph);} else {sphere = new SphereEntity({radius: 110.0,materials,transform: { position, rotation }});rc.addEntity(sphere);}}}position = [0, 0, 0];materials = this.createMaterials(true, false, 'back');let plane = new PlaneEntity({axisType: 1,materials,extent: [-600, -600, 1200, 1200],transform: { position }});rc.addEntity(plane);this.createBillboards();}private createArmTextures(): WGTextureDataDescriptor[] {const albedoTex = { albedo: { url: `static/assets/pbrtex/rough_plaster_broken_diff_1k.jpg` } };const normalTex = { normal: { url: `static/assets/pbrtex/rough_plaster_broken_nor_1k.jpg` } };const armTex = { arm: { url: `static/assets/pbrtex/rough_plaster_broken_arm_1k.jpg` } };const parallaxTex = { parallax: { url: `static/assets/pbrtex/rough_plaster_broken_disp_1k.jpg` } };let envTex = { specularEnv: {} };let textures = [envTex,albedoTex,normalTex,armTex,parallaxTex] as WGTextureDataDescriptor[];return textures;}private createMaterials(shadowReceived = false, shadow = true, faceCullMode = 'back', uvParam?: number[]): BaseMaterial[] {let textures0 = this.createArmTextures();let material0 = this.createMaterial(textures0, ["solid"], 'less', faceCullMode);this.applyMaterialPPt(material0, shadowReceived, shadow);let list = [material0];if (uvParam) {for (let i = 0; i < list.length; ++i) {list[i].property.uvParam.value = uvParam;}}return list;}private applyMaterialPPt(material: BaseMaterial, shadowReceived = false, shadow = true): void {let ppt = material.property;ppt.ambient.value = [0.2, 0.2, 0.2];ppt.albedo.value = this.getRandomColor(1.0);ppt.arms.roughness = Math.random() * 0.95 + 0.05;ppt.arms.metallic = 0.2;ppt.armsBase.value = [0, 1.0, 0];ppt.specularFactor.value = [0.1, 0.1, 0.1];ppt.shadow = shadow;ppt.lighting = true;ppt.shadowReceived = shadowReceived;}private createMaterial(textures: WGTextureDataDescriptor[], blendModes: string[], depthCompare = 'less', faceCullMode = 'back'): BaseMaterial {let pipelineDefParam = {depthWriteEnabled: true,faceCullMode,blendModes,depthCompare};let material = new BaseMaterial({ pipelineDefParam });material.addTextures(textures);return material;}private initEvent(): void {const rc = this.mRscene;rc.addEventListener(MouseEvent.MOUSE_DOWN, this.mouseDown);new MouseInteraction().initialize(rc, 0, false).setAutoRunning(true);}private mouseDown = (evt: MouseEvent): void => { };run(): void {this.mRscene.run();}
}
相关文章:
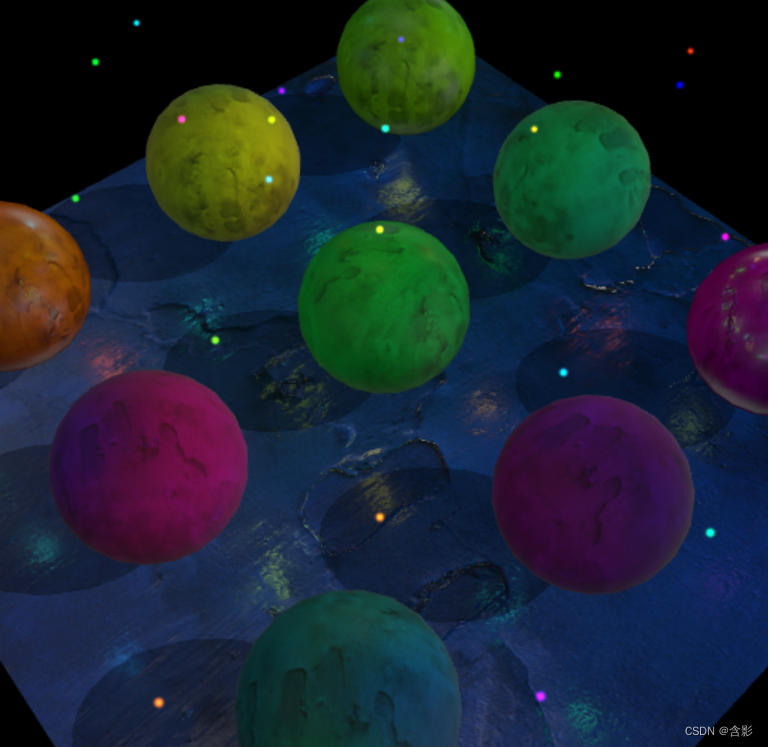
轻量封装WebGPU渲染系统示例<51>- 视差贴图(Parallax Map)(源码)
视差纹理是一种片段着色阶段增强材质表面凹凸细节的技术。 这里在WebGPU的实时渲染材质管线中实现了视差贴图计算,以便增强相关的纹理细节表现力。 当前示例源码github地址: https://github.com/vilyLei/voxwebgpu/blob/feature/material/src/voxgpu/sample/Para…...
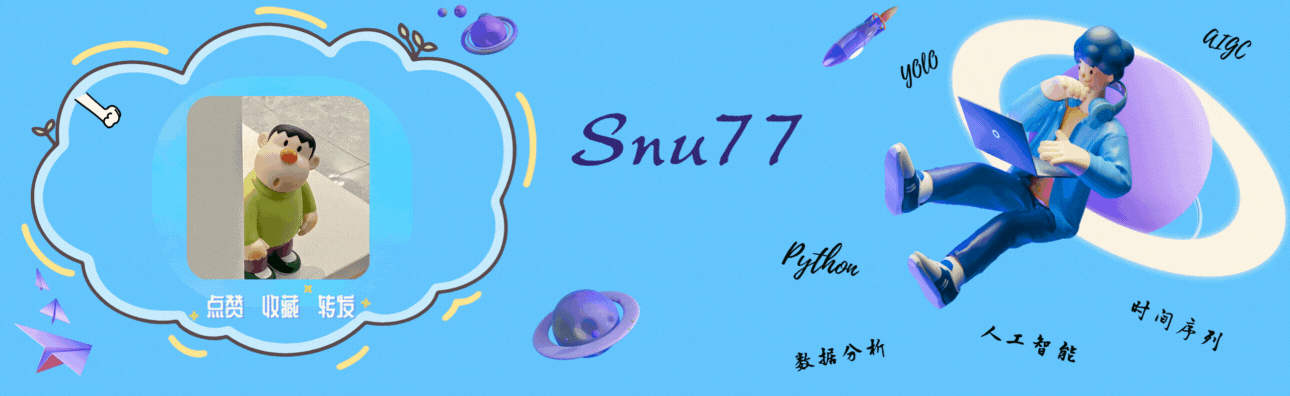
YOLOv8改进 | 2023主干篇 | 华为最新VanillaNet主干替换Backbone实现大幅度长点
一、本文介绍 本文给大家来的改进机制是华为最新VanillaNet网络,其是今年最新推出的主干网络,VanillaNet是一种注重极简主义和效率的神经网络架构。它的设计简单,层数较少,避免了像深度架构和自注意力这样的复杂操作(需要注意的是…...
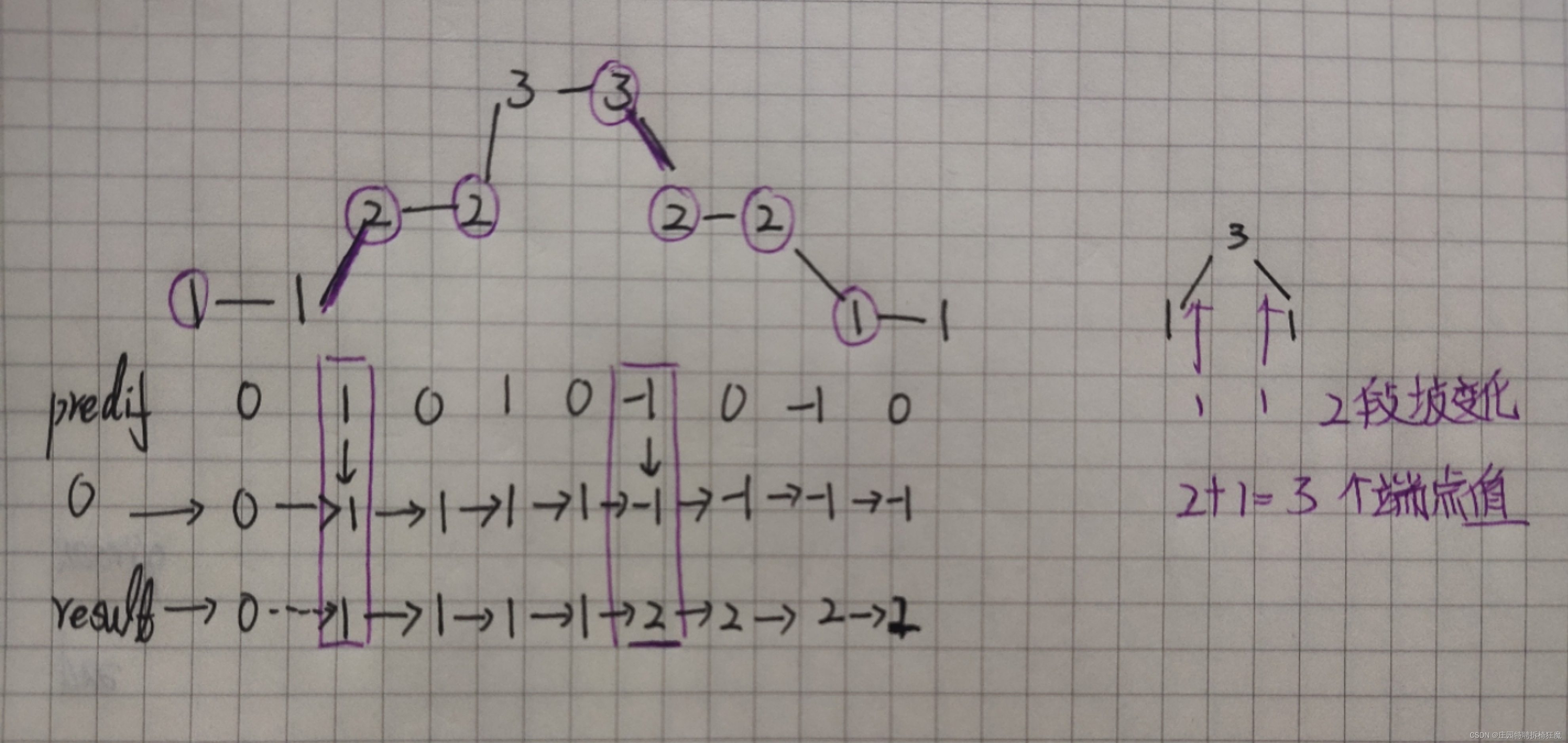
Leetcode 376 摆动序列
题意理解: 如果连续数字之间的差严格地在正数和负数之间交替,则数字序列称为 摆动序列 如果是摆动序列,前后差值呈正负交替出现 为保证摆动序列尽可能的长,我们可以尽可能的保留峰值,,删除上下坡的中间值&…...
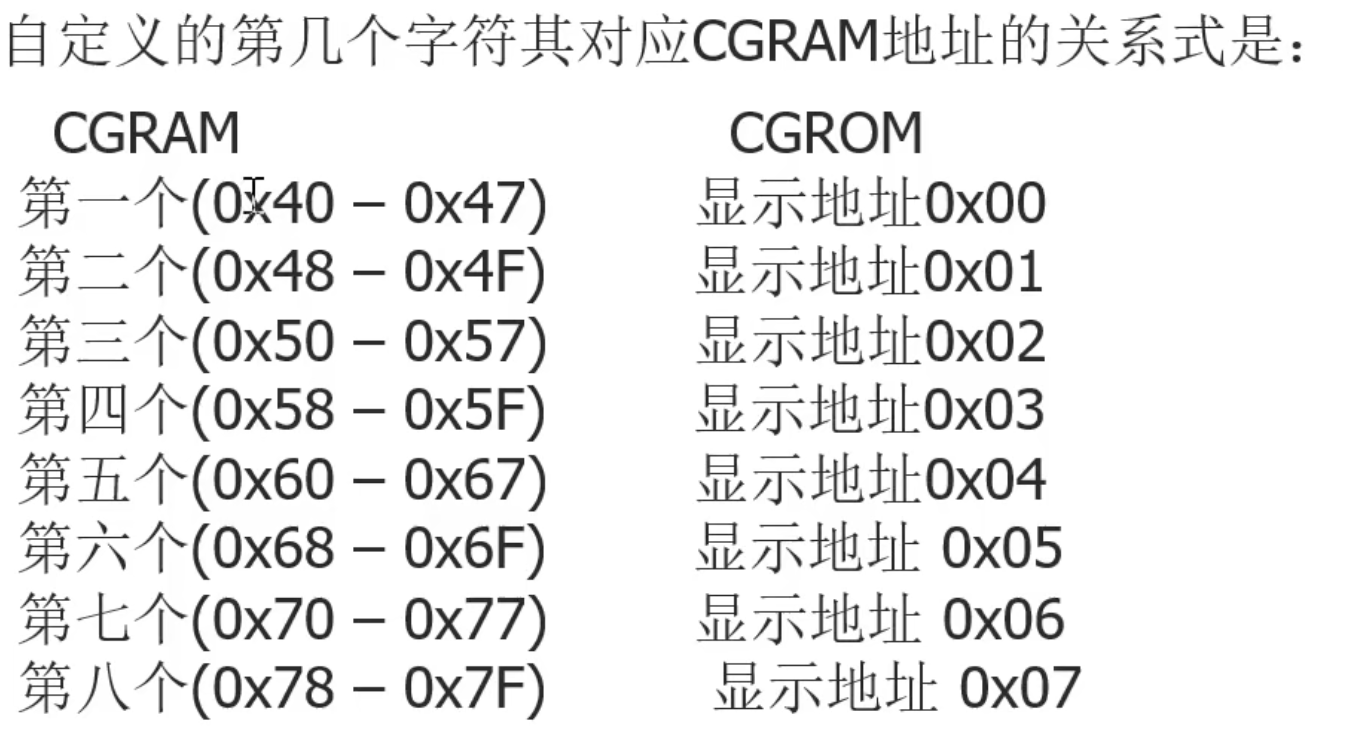
51单片机控制1602LCD显示屏输出自定义字符二
51单片机控制1602LCD显示屏输出自定义字符二 1.概述 1602LCD除了内置的字符外还提供自定义字符功能,当内置的字符中没有我们想要输出的字符时,我们就可以自己创造字符让他显示,下面介绍1602如何创建自定义字符。 2.1602LCD创建字符原理 自…...
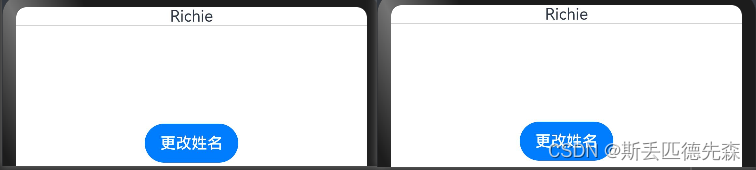
HarmonyOS自学-Day2(@Builder装饰器)
目录 文章声明⭐⭐⭐让我们开始今天的学习吧!Builder装饰器:自定义构建函数Builder介绍Builder使用说明自定义组件中创建自定义构建函数全局自定义构建函数 Builder参数传递规则按引用传递参数按值传递参数 文章声明⭐⭐⭐ 该文章为我(有编程…...
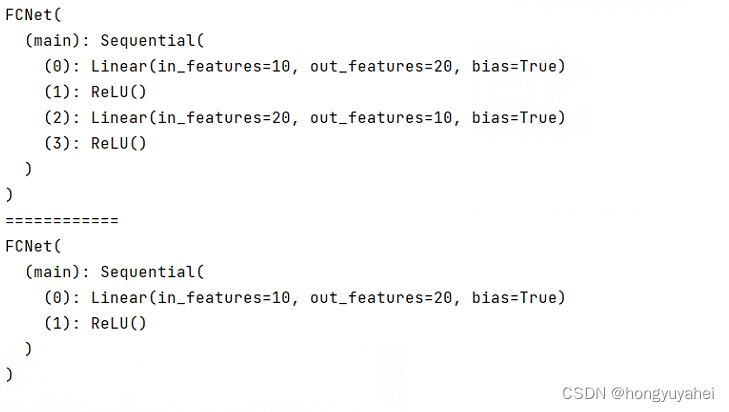
bottom-up-attention-vqa-master 成功复现!!!
代码地址 1、create_dictionary.py 建立词典和使用预训练的glove向量 (1)create_dictionary() 遍历每个question文件取出所关注的question部分,qs 遍历qs,对每个问题的文本内容进行分词,并将分词结果添加到字典中&…...

BigDecimal中divide方法详解
BigDecimal中divide方法详解 大家好,我是免费搭建查券返利机器人赚佣金就用微赚淘客系统3.0的小编,也是冬天不穿秋裤,天冷也要风度的程序猿!今天,让我们一起深入探讨Java中BigDecimal的divide方法,揭开这个…...
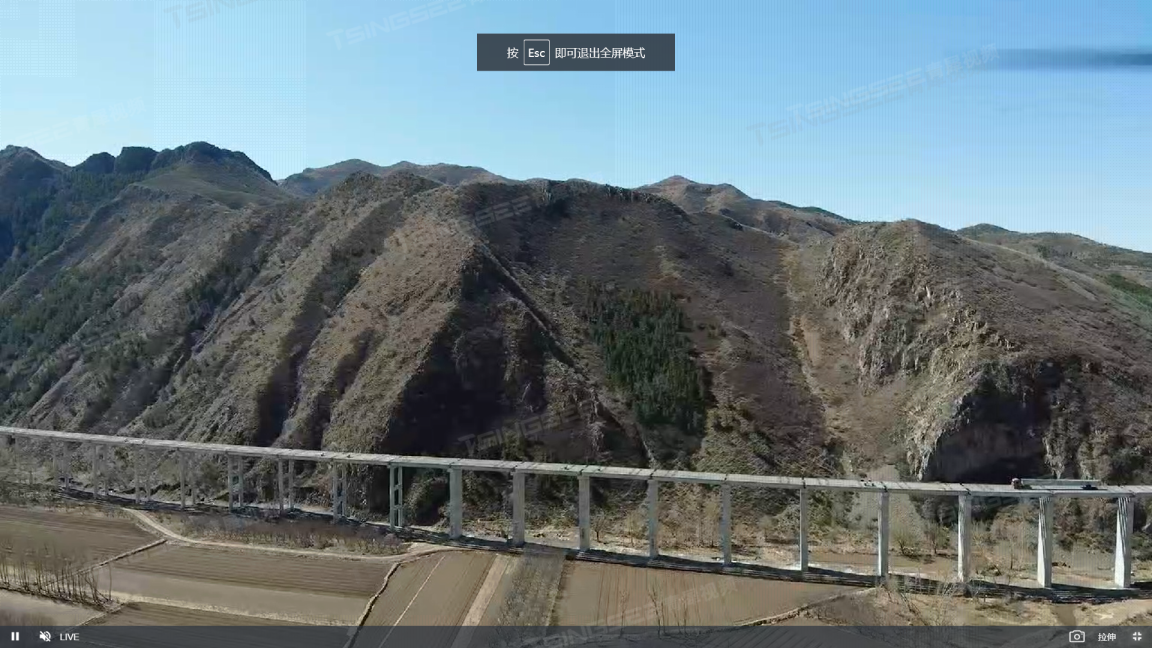
视频推拉流EasyDSS互联网直播/点播平台构建户外无人机航拍直播解决方案
一、背景分析 近几年,国内无人机市场随着航拍等业务走进大众,出现爆发式增长。无人机除了在民用方面的应用越来越多,在其他领域也已经开始广泛应用,比如公共安全、应急搜救、农林、环保、交通 、通信、气象、影视航拍等。无人机使…...
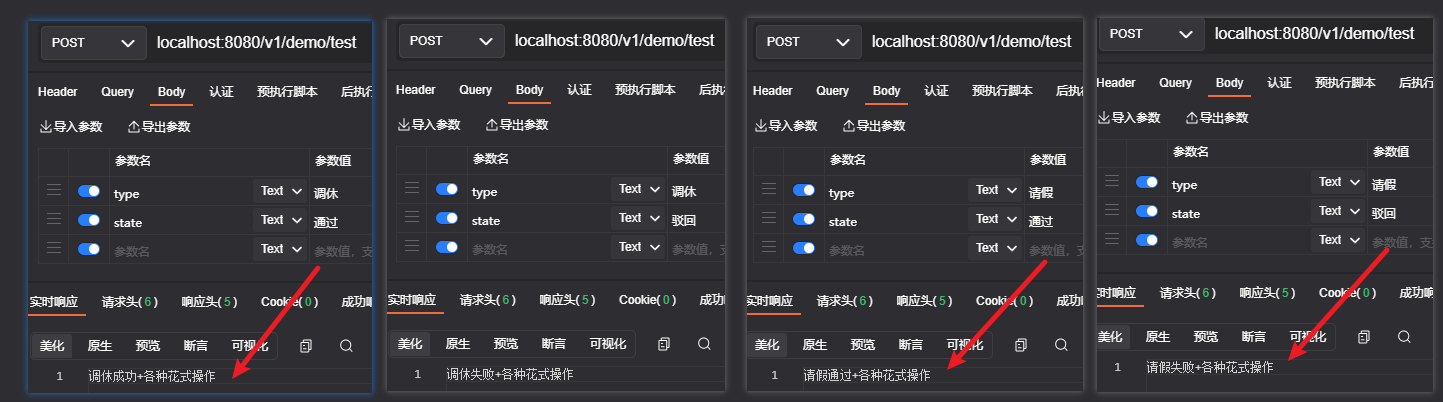
行为型设计模式-策略模式(Strategy Pattern)
策略模式 策略模式:百度百科中引述为:指对象有某个行为,但是在不同的场景中,该行为有不同的实现算法。 策略模式是对算法的包装,是把使用算法的责任和算法本身分割开来,委派给不同的对象管理。策略模式通…...
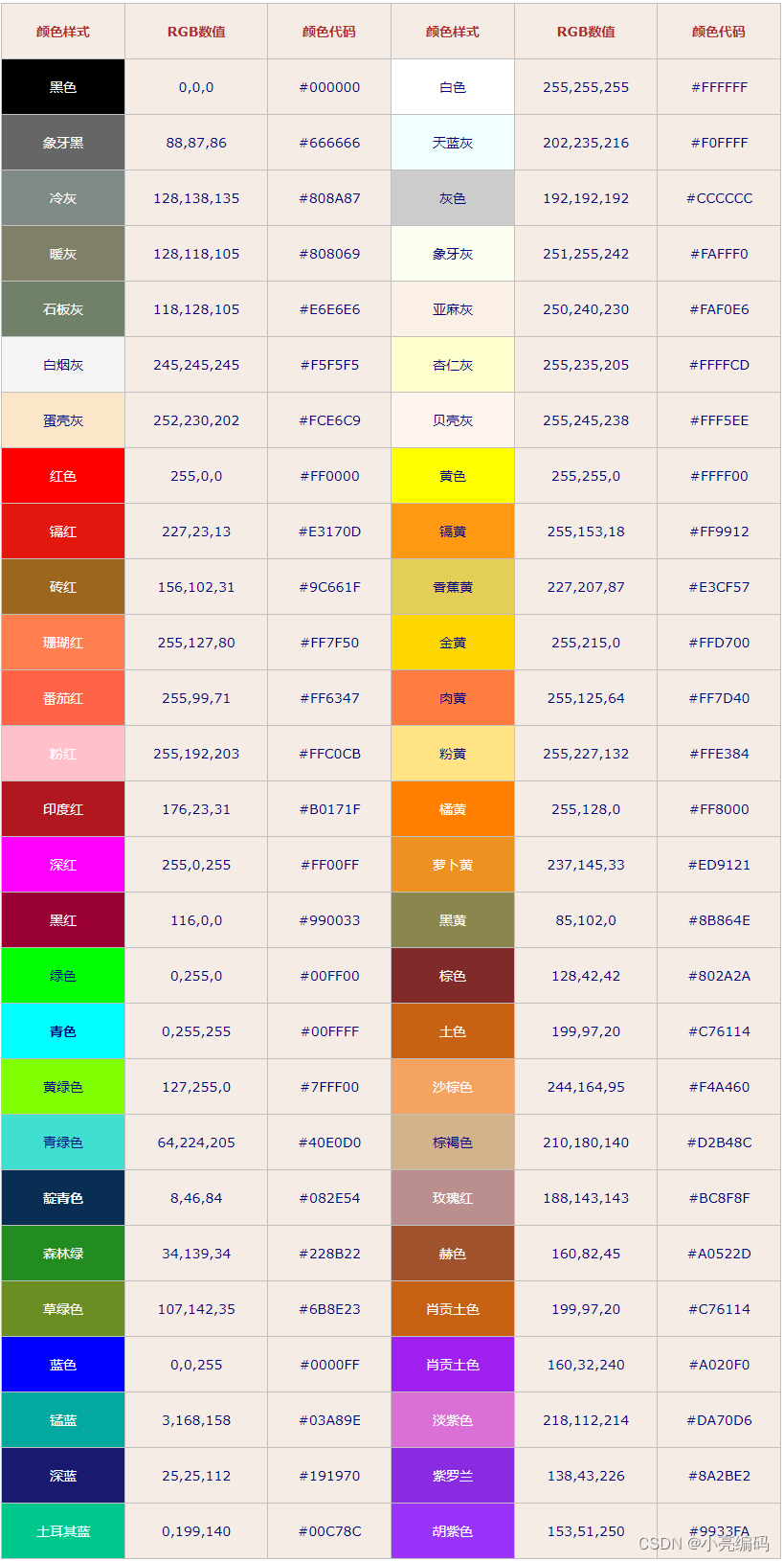
html中RGB和RGBA颜色表示法
文章目录 RGB什么是RGBRGB颜色模式的取值范围RGB常用颜色对照表 RGBA什么是RGBARGBA颜色模式的取值范围 总结 RGB 什么是RGB RGB是一种颜色空间,其中R代表红色(Red)、G代表绿色(Green)、B代表蓝色(Blue&a…...

【BEV感知】BEVFormer 融合多视角相机空间特征和时序特征的端到端框架 ECCV 2022
前言 本文分享BEV感知方案中,具有代表性的方法:BEVFormer。 基本思想:使用可学习的查询Queries表示BEV特征,查找图像中的空间特征和先前BEV地图中的时间特征。 它基于Deformable Attention实现了一种融合多视角相机空间特征和时序特征的端到端框架,适用于多种自动驾驶感…...

git拉取hugging face代码失败:443
报错信息:fatal: unable to access http://huggingface.co/THUDM/chatglm2-6b/: OpenSSL SSL_connect: Connection reset by peer in connection to huggingface.co:443 解决方法:(127.0.0.1:7890配置为自己的实际代理ip及端口) …...
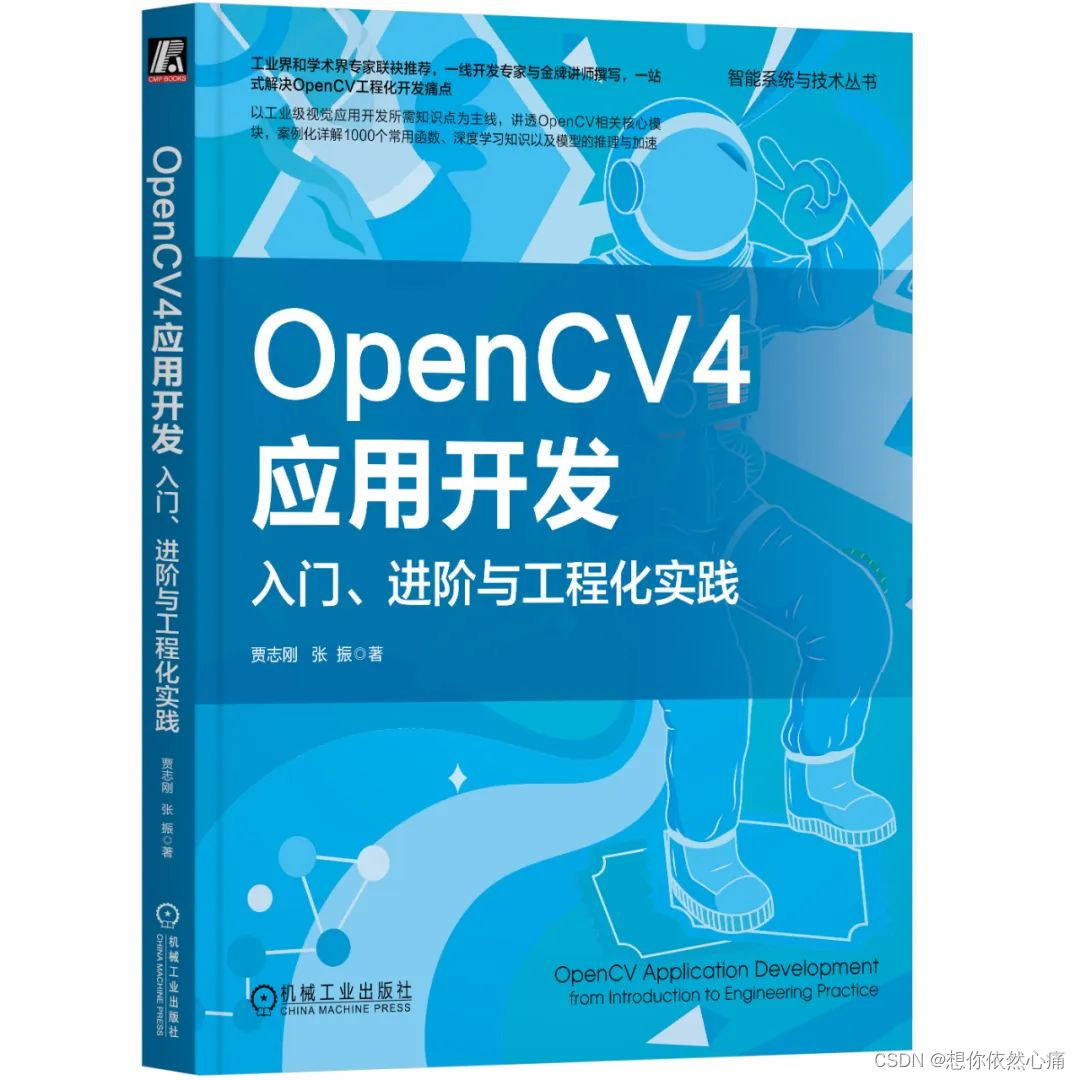
【赠书活动】OpenCV4工业缺陷检测的六种方法
文章目录 前言机器视觉缺陷检测工业上常见缺陷检测方法延伸阅读推荐语 赠书活动 前言 随着工业制造的发展,对产品质量的要求越来越高。工业缺陷检测是确保产品质量的重要环节,而计算机视觉技术的应用能够有效提升工业缺陷检测的效率和精度。 OpenCV是一…...
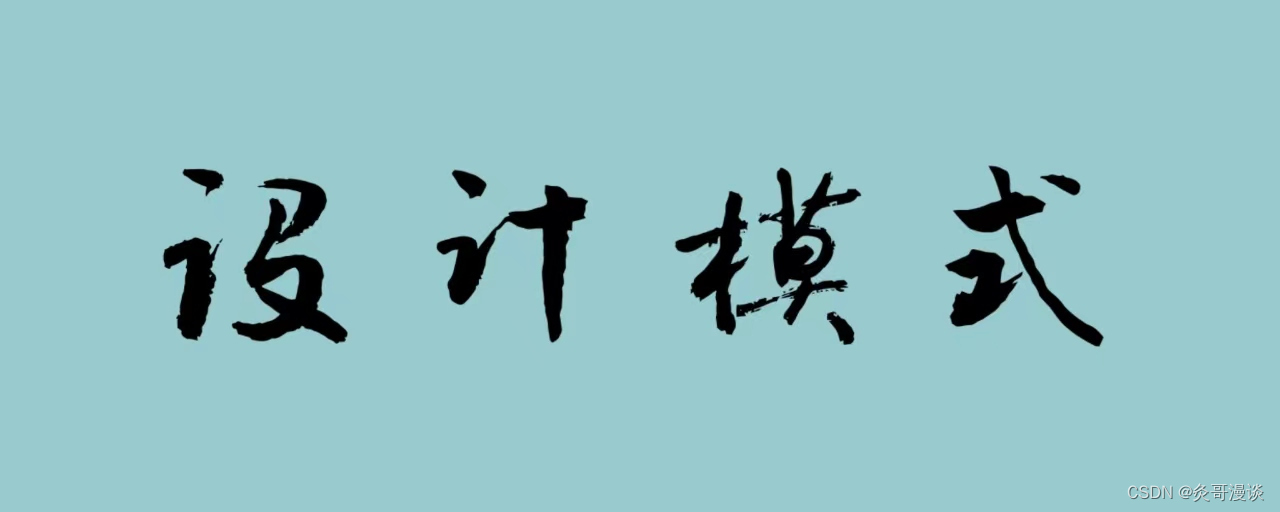
设计模式之创建型设计模式(一):单例模式 原型模式
单例模式 Singleton 1、什么是单例模式 在软件设计中,单例模式是一种创建型设计模式,其主要目的是确保一个类只有一个实例,并提供一个全局访问点。 这意味着无论何时需要该类的实例,都可以获得相同的实例,而不会创建…...
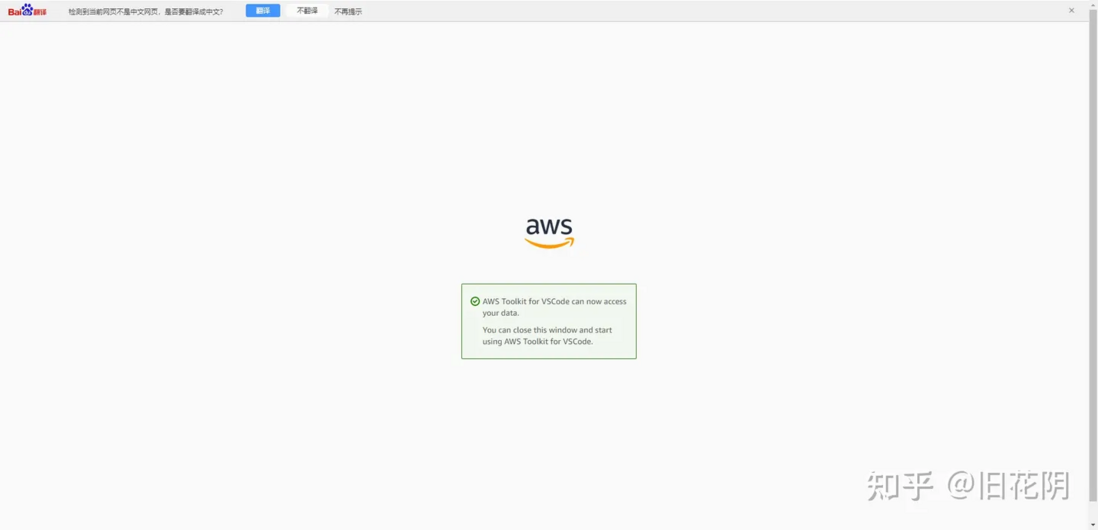
Amazon CodeWhisperer 在 vscode 的应用
文章作者:旧花阴 CodeWhisperer 是一款可以帮助程序员更快、更安全地编写代码的工具,可以在他们的开发环境中实时提供代码建议和推荐。亚马逊云科技发布的这款代码生成工具 CodeWhisperer 最大的优势就是对于个人用户免费。以在 vscode 为例,演示安装过程…...
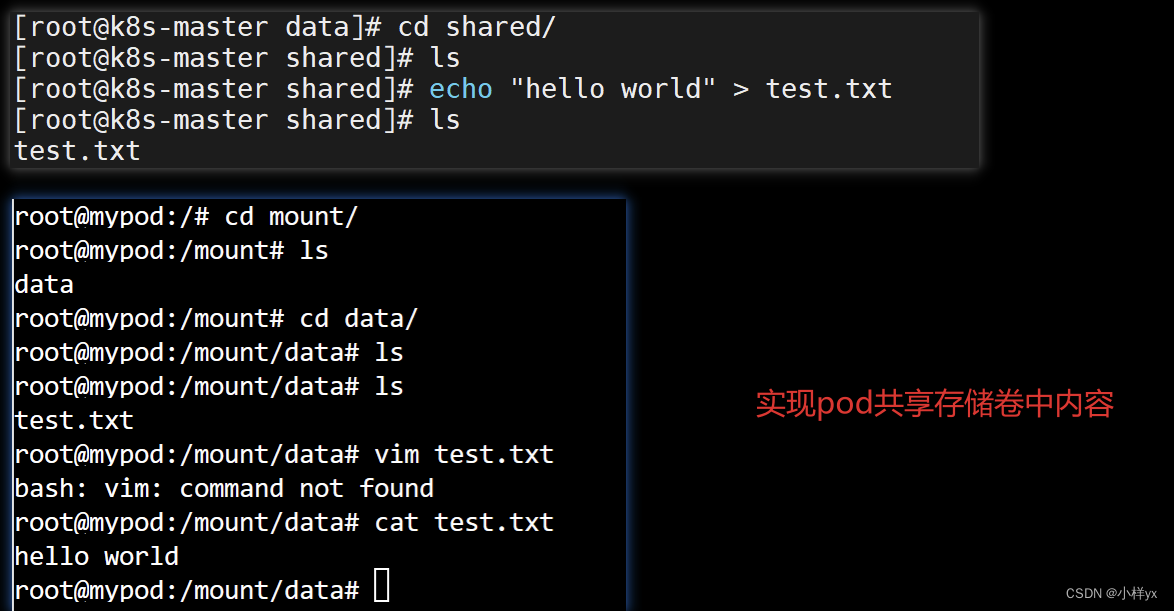
【Java】基于fabric8io库操作k8s集群实战(pod、deployment、service、volume)
目录 前言一、基于fabric8io操作pod1.1 yaml创建pod1.2 fabric8io创建pod案例 二、基于fabric8io创建Service(含Deployment)2.1 yaml创建Service和Deployment2.2 fabric8io创建service案例 三、基于fabric8io操作Volume3.1 yaml配置挂载存储卷3.2 基于fa…...

uniapp微信小程序下载保存图片流到本地,base64
我们在开发时下载图片或文件,地址基本上都是https的格式,下面来说一下后端返回base64的文件流,是如何下载的 必须把返回的流去掉这一部分:data:image/png;base64,否则下载不了 如我自己的流: data:image/…...
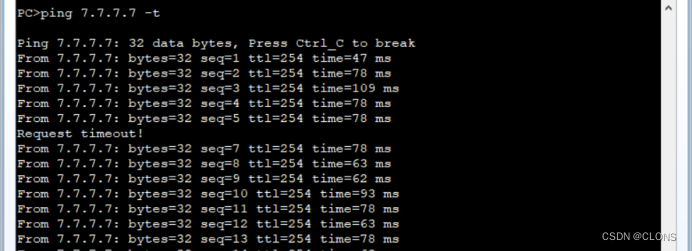
华为数通——企业双出口冗余
目标:默认数据全部经过移动上网,联通低带宽。 R1 [ ]ip route-static 0.0.0.0 24 12.1.1.2 目的地址 掩码 下一条 [ ]ip route-static 0.0.0.0 24 13.1.1.3 preference 65 目的地址 掩码 下一条 设置优先级为65 R…...
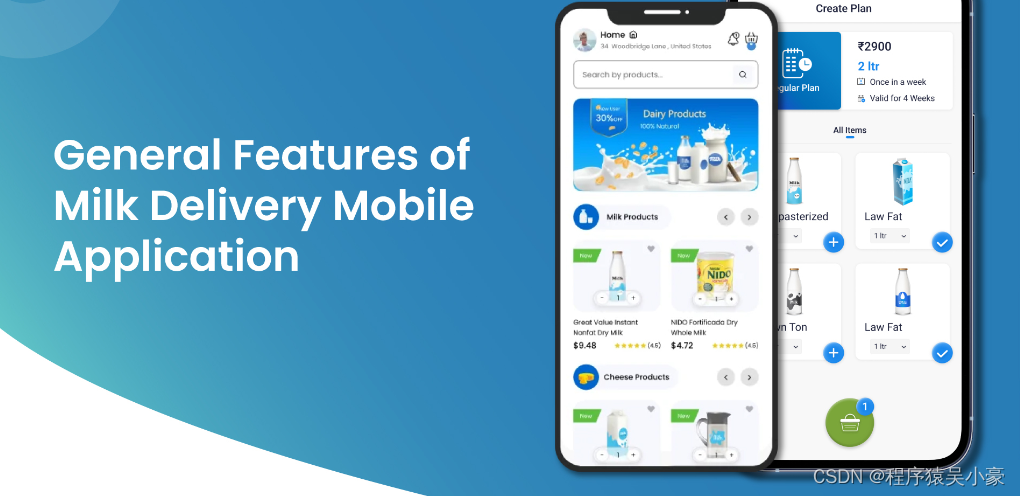
送奶APP开发:终极指南
您是否有兴趣使用新鲜牛奶和乳制品,但不想每天早上去乳制品店或最近的商店?借助技术,订购日常用品(例如杂货和牛奶)变得更加简单。 DailyMoo 是最受欢迎的送奶应用,收入达数百万人民币。因此,投…...
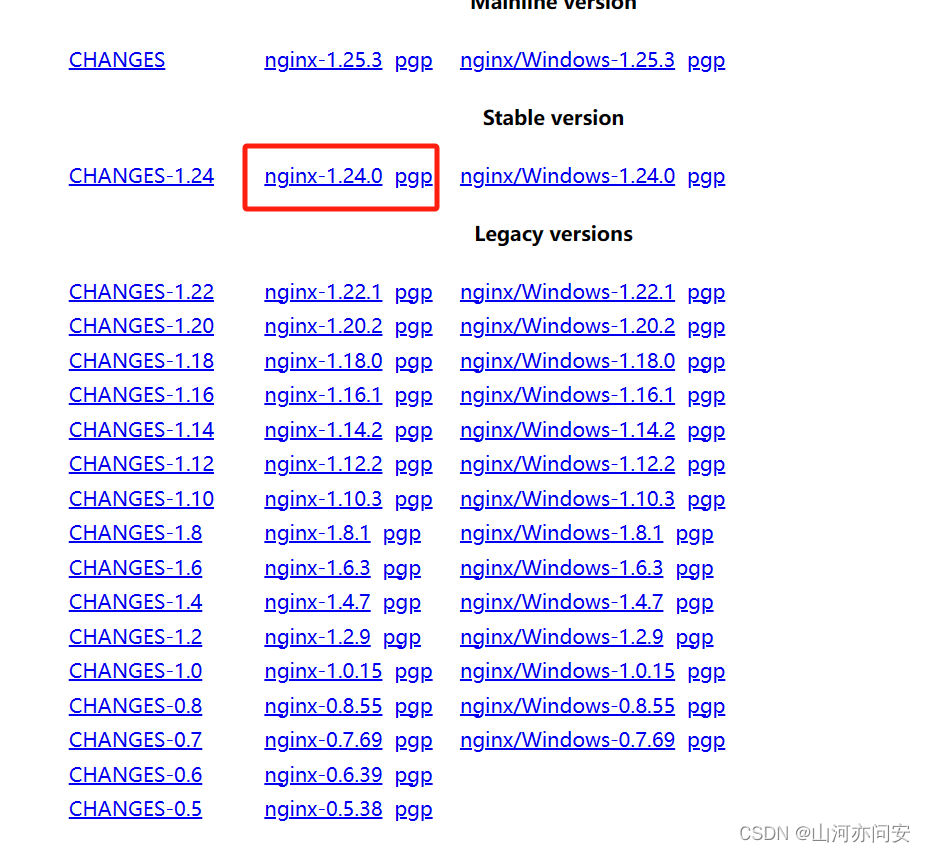
Ngnix之反向代理、负载均衡、动静分离
目录 1. Ngnix 1.1 Linux系统Ngnix下载安装 1.2 反向代理 正向代理(Forward Proxy): 反向代理(Reverse Proxy): 1.3 负载均衡 1.4 动静分离 1. Ngnix Nginx是一个高性能的开源Web服务器࿰…...
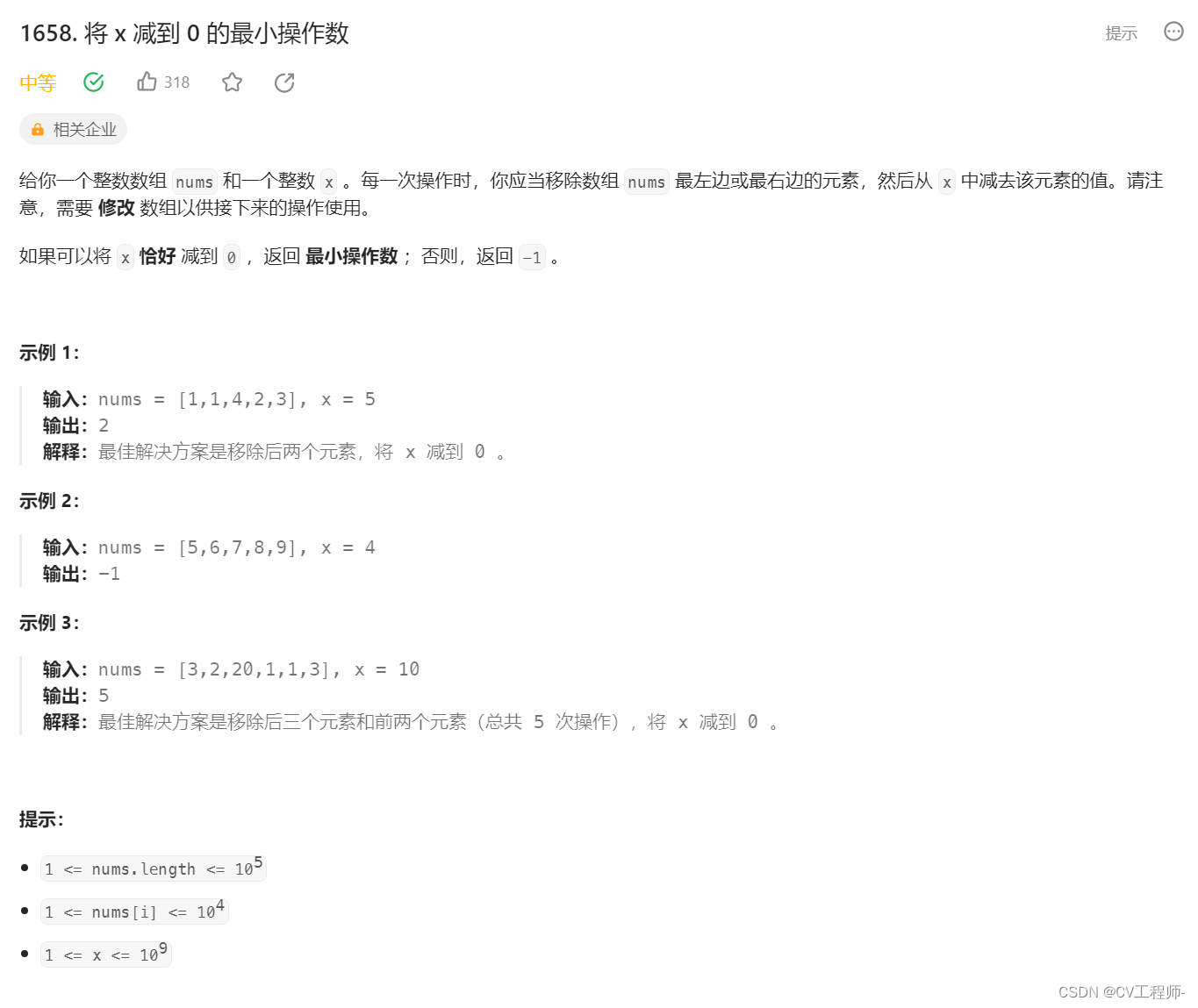
(C++)将x减到0的最小操作数--滑动窗口
个人主页:Lei宝啊 愿所有美好如期而遇 力扣(LeetCode)官网 - 全球极客挚爱的技术成长平台备战技术面试?力扣提供海量技术面试资源,帮助你高效提升编程技能,轻松拿下世界 IT 名企 Dream Offer。https://le…...
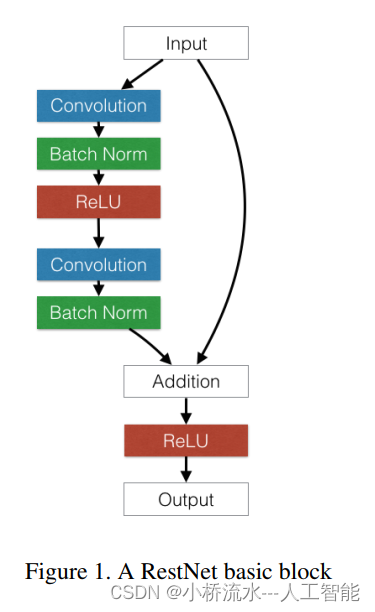
回答某位同学的问题:残差网络常用来分类,可以用于回归预测吗?
残差网络可以用于回归预测,以下是我的观点: 残差网络最初是用于计算机视觉和语音识别等分类任务,但它也可以用于回归预测。在回归预测任务中,我们预测的目标变量通常是一个连续值,而不是一个离散的类别。使用残差网络进行回归预测的主要思路是: 定义一个…...

C语言初学5:运算符
一、算数运算符 假设变量 A 的值为 10 运算符描述实例A先赋值后运算C A C为10 A为11A--C A-- C为10 A为9A先运算后赋值C A C为11 A为11--AC --A C为9 A为9 二、位运算符 运算符描述实例&对两个操作数的每一位执行逻辑与操作,如果两个相应的位都为 1&…...
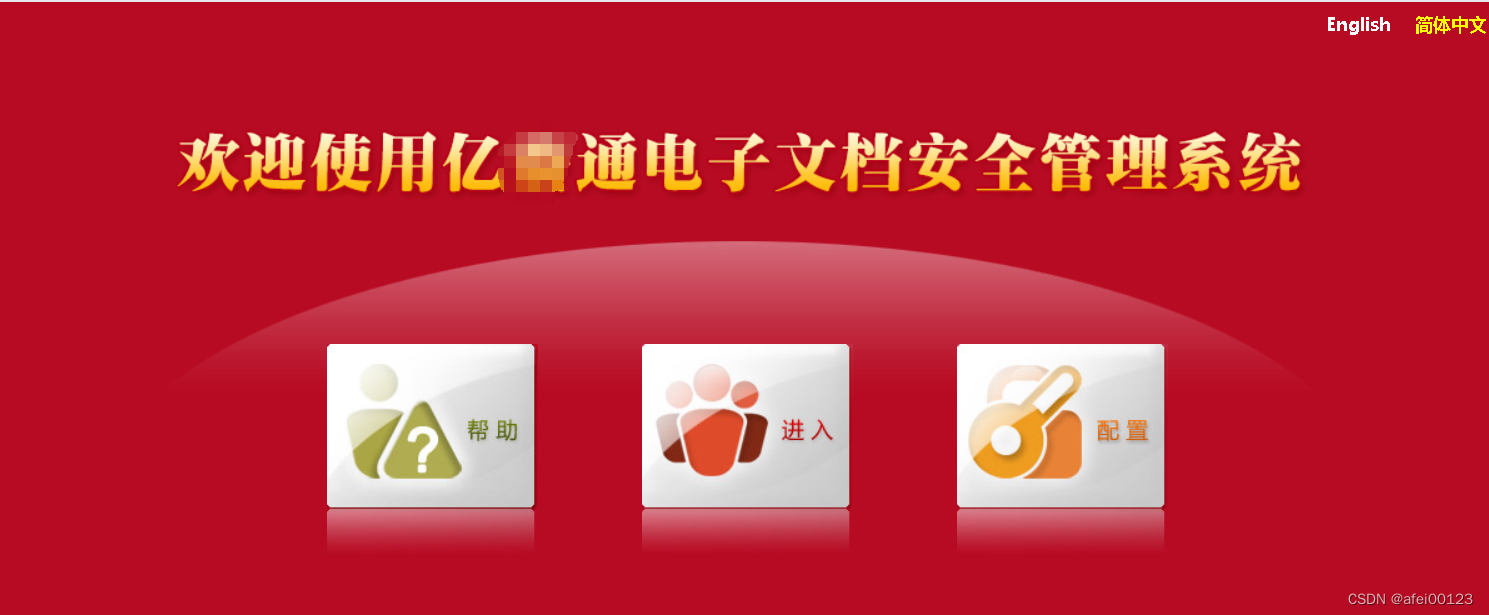
亿某通电子文档安全管理系统任意文件上传漏洞 CNVD-2023-59471
1.漏洞概述 亿某通电子文档安全管理系统是一款电子文档安全防护软件,该系统利用驱动层透明加密技术,通过对电子文档的加密保护,防止内部员工泄密和外部人员非法窃取企业核心重要数据资产。亿赛通电子文档安全管理系统UploadFileFromClientServiceForClient接口处存在任意文件…...
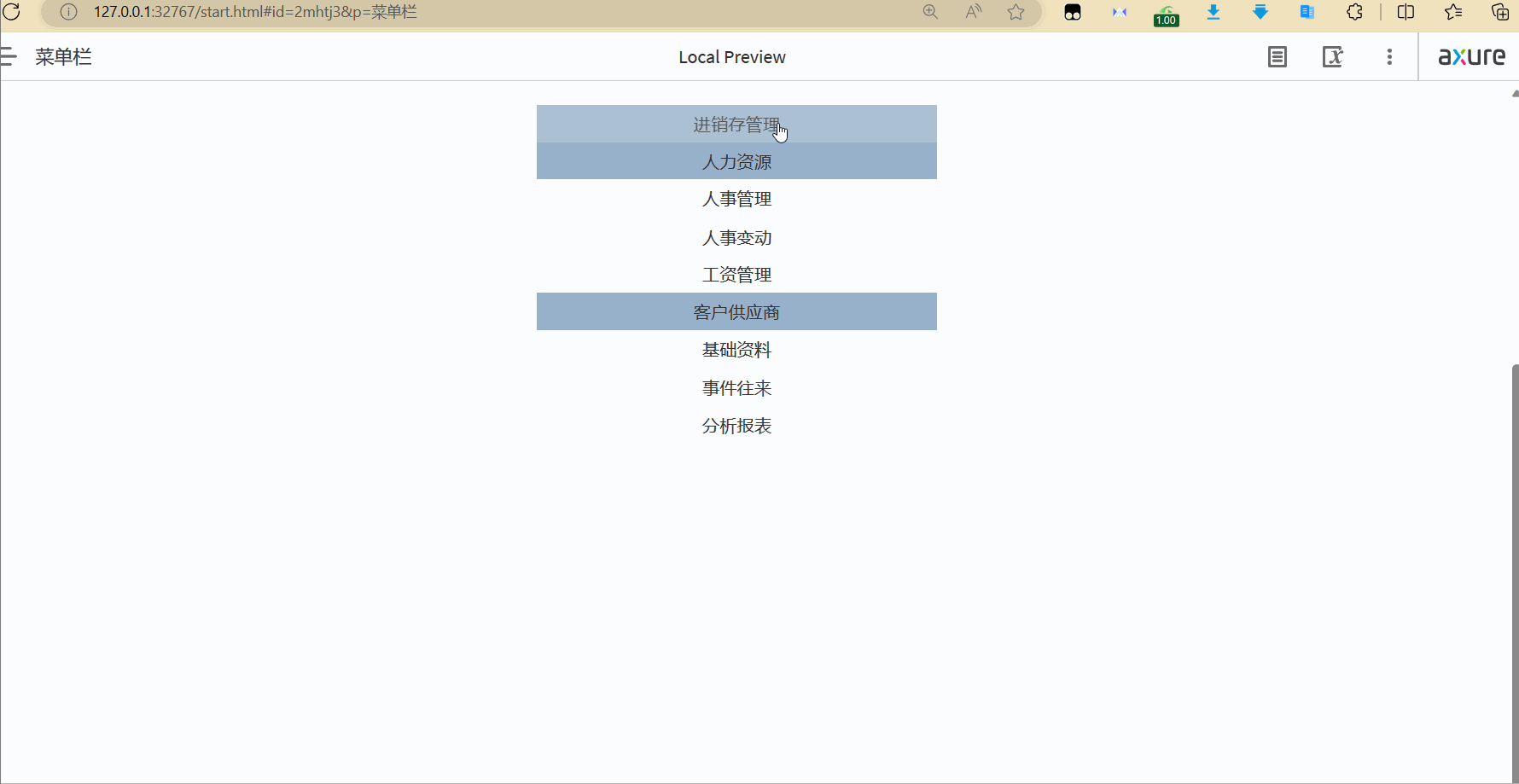
产品入门第四讲:Axure动态面板
📚📚 🏅我是默,一个在CSDN分享笔记的博主。📚📚 🌟在这里,我要推荐给大家我的专栏《Axure》。🎯🎯 🚀无论你是编程小白,还…...
【数据结构】哈希表算法总结
知识概览(哈希表) 哈希表可以将一些值域较大的数映射到较小的空间内,通常用x mod 质数的方式进行映射。为什么用质数呢?这样的质数还要离2的整数幂尽量远。这可以从数学上证明,这样冲突最小。取余还是会出现冲突情况。…...

微信小程序单图上传和多图上传
图片上传主要用到 1、wx.chooseImage(Object object) 从本地相册选择图片或使用相机拍照。 参数 Object object 属性类型默认值必填说明countnumber9否最多可以选择的图片张数sizeTypeArray.<string>[original, compressed]否所选的图片的尺寸sourceTypeArray.<s…...

github入门基础操作
GitHub是一个基于Git版本控制系统的代码托管平台,它提供了一个方便的平台,让开发者可以在上面存储、管理和分享代码。如果你是一个开发者,那么学习如何使用GitHub是非常重要的,因为它可以帮助你更好地管理你的代码和协作开发。 在…...

Android Studio(3.6.2版本)安装 java2smali 插件,java2smali 插件的使用方法简述
一、Android Studio(3.6.2版本)安装 java2smali 插件 1、左上角File—>Setting,如下图 2、Setting界面中:点击Plugins—>选择右侧上方Marketplace—>搜索栏输入java2smali,如下图 3、点击Install按钮—>点…...

vscode使用remote ssh到server上 - Node进程吃满CPU
起因:Node进程吃满CPU 分析 我发现每次使用vscode的remote插件登陆到server后,就会出现node进程,不太清楚干什么用的,但是绝对和它有关。 查找原因 首先找到了这篇文章,解决了rg进程的问题: https://blo…...