openssl3.2 - 官方demo学习 - mac - poly1305.c
文章目录
- openssl3.2 - 官方demo学习 - mac - poly1305.c
- 概述
- 笔记
- END
openssl3.2 - 官方demo学习 - mac - poly1305.c
概述
MAC算法为Poly1305,
加密算法为AES-128-ECB, 用key初始化加密算法
加密算法进行padding填充
对加密算法的key加密, 放入MAC_key后16字节, 将MAC_key的前16字节清空, 作为要用的MAC_key
拿MAC_key来初始化MAC上下文
对明文进行MAC操作.
官方建议:
Poly1305不能单独使用, 必须和其他加密算法一起对输入(MAC_key)进行处理
绝对禁止将nonce(MAC_key)直接传给Poly1305
不同会话的nonce(MAC_key)禁止重用(相同).
在实际应用绝对禁止将nonce(MAC_key)硬编码
看来nonce对于Poly1305应用的安全性影响很大(知道了MAC_key, 就可以伪造MAC值)
笔记
/*!
\file poly1305.c
\note
openssl3.2 - 官方demo学习 - mac - poly1305.cMAC算法为Poly1305,
加密算法为AES-128-ECB, 用key初始化加密算法
加密算法进行padding填充对加密算法的key加密, 放入MAC_key后16字节, 将MAC_key的前16字节清空, 作为要用的MAC_key
拿MAC_key来初始化MAC上下文
对明文进行MAC操作.官方建议:
Poly1305不能单独使用, 必须和其他加密算法一起对输入(MAC_key)进行处理
绝对禁止将nonce(MAC_key)直接传给Poly1305
不同会话的nonce(MAC_key)禁止重用(相同).
在实际应用绝对禁止将nonce(MAC_key)硬编码
看来nonce对于Poly1305应用的安全性影响很大(知道了MAC_key, 就可以伪造MAC值)
*//** Copyright 2021-2023 The OpenSSL Project Authors. All Rights Reserved.** Licensed under the Apache License 2.0 (the "License"). You may not use* this file except in compliance with the License. You can obtain a copy* in the file LICENSE in the source distribution or at* https://www.openssl.org/source/license.html*/#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/core_names.h>
#include <openssl/evp.h>
#include <openssl/params.h>
#include <openssl/err.h>#include "my_openSSL_lib.h"/** This is a demonstration of how to compute Poly1305-AES using the OpenSSL* Poly1305 and AES providers and the EVP API.** Please note that:** - Poly1305 must never be used alone and must be used in conjunction with* another primitive which processes the input nonce to be secure;** - you must never pass a nonce to the Poly1305 primitive directly;** - Poly1305 exhibits catastrophic failure (that is, can be broken) if a* nonce is ever reused for a given key.** If you are looking for a general purpose MAC, you should consider using a* different MAC and looking at one of the other examples, unless you have a* good familiarity with the details and caveats of Poly1305.** This example uses AES, as described in the original paper, "The Poly1305-AES* message authentication code":* https://cr.yp.to/mac/poly1305-20050329.pdf** The test vectors below are from that paper.*//** Hard coding the key into an application is very bad.* It is done here solely for educational purposes.* These are the "r" and "k" inputs to Poly1305-AES.*/
static const unsigned char test_r[] = {0x85, 0x1f, 0xc4, 0x0c, 0x34, 0x67, 0xac, 0x0b,0xe0, 0x5c, 0xc2, 0x04, 0x04, 0xf3, 0xf7, 0x00
};static const unsigned char test_k[] = {0xec, 0x07, 0x4c, 0x83, 0x55, 0x80, 0x74, 0x17,0x01, 0x42, 0x5b, 0x62, 0x32, 0x35, 0xad, 0xd6
};/** Hard coding a nonce must not be done under any circumstances and is done here* purely for demonstration purposes. Please note that Poly1305 exhibits* catastrophic failure (that is, can be broken) if a nonce is ever reused for a* given key.*/
static const unsigned char test_n[] = {0xfb, 0x44, 0x73, 0x50, 0xc4, 0xe8, 0x68, 0xc5,0x2a, 0xc3, 0x27, 0x5c, 0xf9, 0xd4, 0x32, 0x7e
};/* Input message. */
static const unsigned char test_m[] = {0xf3, 0xf6
};static const unsigned char expected_output[] = {0xf4, 0xc6, 0x33, 0xc3, 0x04, 0x4f, 0xc1, 0x45,0xf8, 0x4f, 0x33, 0x5c, 0xb8, 0x19, 0x53, 0xde
};/** A property query used for selecting the POLY1305 implementation.*/
static char *propq = NULL;int main(int argc, char **argv)
{int ret = EXIT_FAILURE;EVP_CIPHER *aes = NULL;EVP_CIPHER_CTX *aesctx = NULL;EVP_MAC *mac = NULL;EVP_MAC_CTX *mctx = NULL;unsigned char composite_key[32];unsigned char out[16];OSSL_LIB_CTX *library_context = NULL;size_t out_len = 0;int aes_len = 0;library_context = OSSL_LIB_CTX_new();if (library_context == NULL) {fprintf(stderr, "OSSL_LIB_CTX_new() returned NULL\n");goto end;}/* Fetch the Poly1305 implementation */mac = EVP_MAC_fetch(library_context, "POLY1305", propq);if (mac == NULL) {fprintf(stderr, "EVP_MAC_fetch() returned NULL\n");goto end;}/* Create a context for the Poly1305 operation */mctx = EVP_MAC_CTX_new(mac);if (mctx == NULL) {fprintf(stderr, "EVP_MAC_CTX_new() returned NULL\n");goto end;}/* Fetch the AES implementation */aes = EVP_CIPHER_fetch(library_context, "AES-128-ECB", propq);if (aes == NULL) {fprintf(stderr, "EVP_CIPHER_fetch() returned NULL\n");goto end;}/* Create a context for AES */aesctx = EVP_CIPHER_CTX_new();if (aesctx == NULL) {fprintf(stderr, "EVP_CIPHER_CTX_new() returned NULL\n");goto end;}/* Initialize the AES cipher with the 128-bit key k */if (!EVP_EncryptInit_ex(aesctx, aes, NULL, test_k, NULL)) {fprintf(stderr, "EVP_EncryptInit_ex() failed\n");goto end;}/** Disable padding for the AES cipher. We do not strictly need to do this as* we are encrypting a single block and thus there are no alignment or* padding concerns, but this ensures that the operation below fails if* padding would be required for some reason, which in this circumstance* would indicate an implementation bug.*/if (!EVP_CIPHER_CTX_set_padding(aesctx, 0)) {fprintf(stderr, "EVP_CIPHER_CTX_set_padding() failed\n");goto end;}/** Computes the value AES_k(n) which we need for our Poly1305-AES* computation below.*/if (!EVP_EncryptUpdate(aesctx, composite_key + 16, &aes_len,test_n, sizeof(test_n))) {fprintf(stderr, "EVP_EncryptUpdate() failed\n");goto end;}/** The Poly1305 provider expects the key r to be passed as the first 16* bytes of the "key" and the processed nonce (that is, AES_k(n)) to be* passed as the second 16 bytes of the "key". We already put the processed* nonce in the correct place above, so copy r into place.*/memcpy(composite_key, test_r, 16);/* Initialise the Poly1305 operation */if (!EVP_MAC_init(mctx, composite_key, sizeof(composite_key), NULL)) {fprintf(stderr, "EVP_MAC_init() failed\n");goto end;}/* Make one or more calls to process the data to be authenticated */if (!EVP_MAC_update(mctx, test_m, sizeof(test_m))) {fprintf(stderr, "EVP_MAC_update() failed\n");goto end;}/* Make one call to the final to get the MAC */if (!EVP_MAC_final(mctx, out, &out_len, sizeof(out))) {fprintf(stderr, "EVP_MAC_final() failed\n");goto end;}printf("Generated MAC:\n");BIO_dump_indent_fp(stdout, out, (int)out_len, 2);putchar('\n');if (out_len != sizeof(expected_output)) {fprintf(stderr, "Generated MAC has an unexpected length\n");goto end;}if (CRYPTO_memcmp(expected_output, out, sizeof(expected_output)) != 0) {fprintf(stderr, "Generated MAC does not match expected value\n");goto end;}ret = EXIT_SUCCESS;
end:EVP_CIPHER_CTX_free(aesctx);EVP_CIPHER_free(aes);EVP_MAC_CTX_free(mctx);EVP_MAC_free(mac);OSSL_LIB_CTX_free(library_context);if (ret != EXIT_SUCCESS)ERR_print_errors_fp(stderr);return ret;
}
END
相关文章:

openssl3.2 - 官方demo学习 - mac - poly1305.c
文章目录 openssl3.2 - 官方demo学习 - mac - poly1305.c概述笔记END openssl3.2 - 官方demo学习 - mac - poly1305.c 概述 MAC算法为Poly1305, 加密算法为AES-128-ECB, 用key初始化加密算法 加密算法进行padding填充 对加密算法的key加密, 放入MAC_key后16字节, 将MAC_key的…...

【Python 千题 —— 基础篇】不吉利的数字
题目描述 题目描述 在西方,“13”被称为不吉利的数字,这是因为耶稣与13个弟子共进晚餐时耶稣的第13个弟子出卖了耶稣,且耶稣受难的日期是13日。所以西方的门牌号会跳过13号,假设这栋楼有16户,请为这栋楼的每一户设立门牌号。 输入描述 无 输出描述 依次输出这栋楼每…...
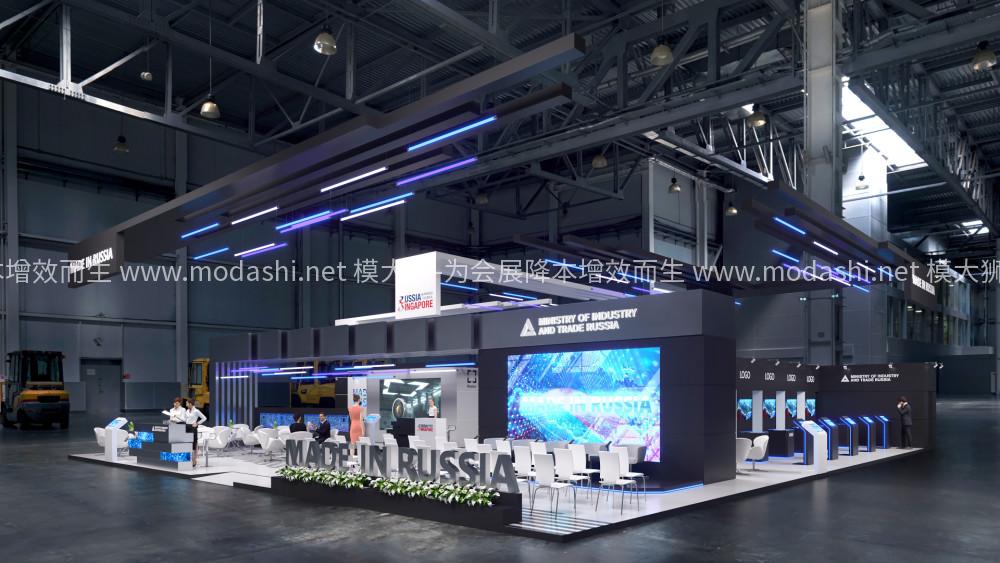
3d模型未响应打不开怎么办---模大狮模型网
在进行3D建模和设计工作时,有时可能会遇到3D模型无法打开的情况,这给工作流程带来了困扰。本文将为您介绍一些常见的原因以及解决3D模型未响应无法打开问题的方法。 一、文件格式检查 首先,确保您使用的文件格式与所使用的软件兼容。不同的3…...
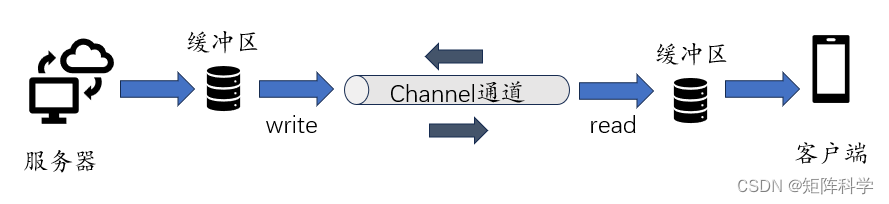
Java-NIO 开篇(1)
NIO简介 高性能的Java通信,离不开Java NIO组件,现在主流的技术框架或中间件服务器,都使用了Java NIO组件,譬如Tomcat、 Jetty、 Netty、Redis、RabbitMQ等的网络通信模块。在1.4版本之前, Java IO类库是阻塞式IO&…...
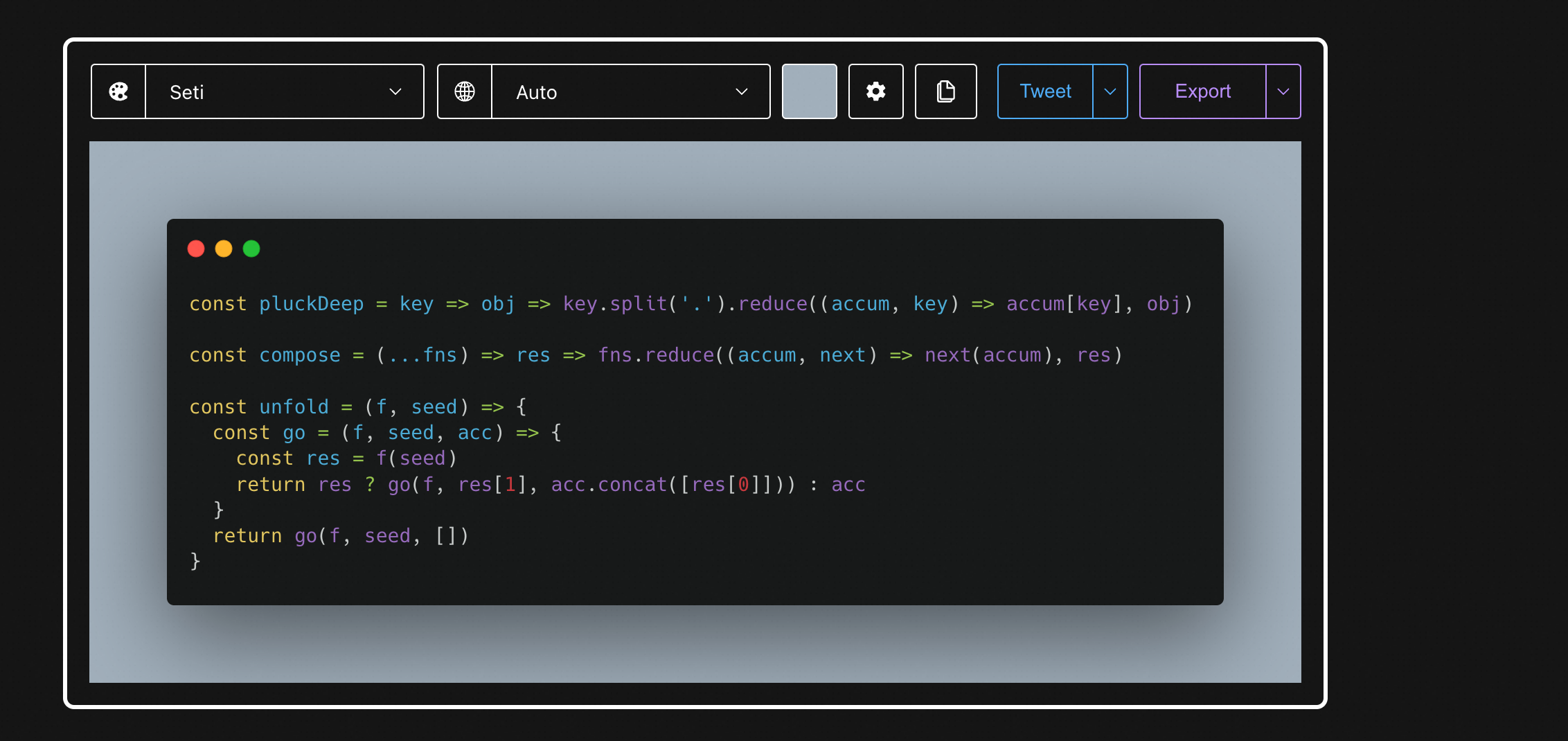
VSCode 插件推荐
前言 关于开发用的插件就不做赘述了,网上面有很多文章都做了推荐,本文推荐几个好看的插件。 文件图标主题 Vscode icons Material Icon Theme 字体主题 推荐 One Dark Pro 其他 推荐一个生成好看代码的网址 https://carbon.now.sh/...
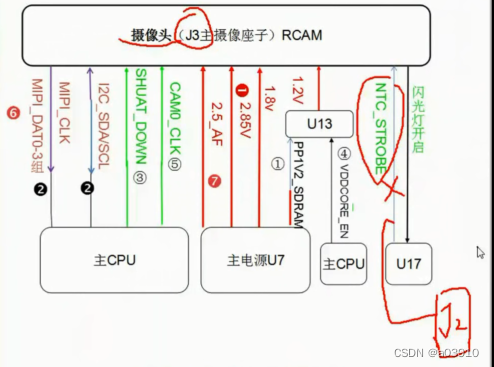
摄像部分时序
(1).,后摄像。 (2).,前摄像。 RCAM_TO_LEDDRV_STROBE_EN_CONN表面意思是:后置摄像头到led驱动闪光灯_使能。从时序图中看起来是连接到U17的,发现果然如此。 闪光灯温度检测,是检测闪光灯的温度。所以时序图…...
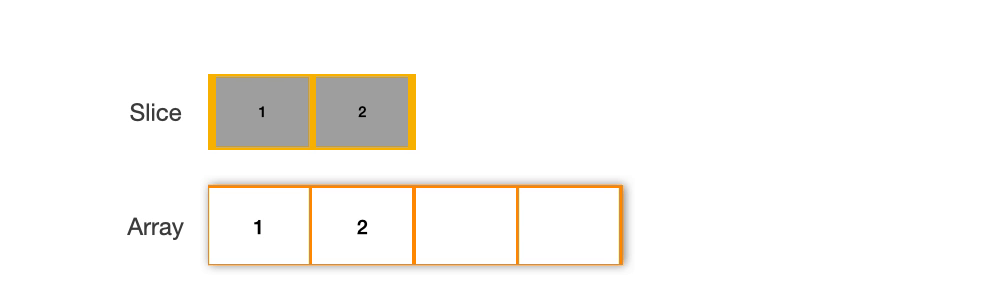
为什么 Golang Fasthttp 选择使用 slice 而非 map 存储请求数据
文章目录 Slice vs Map:基本概念内存分配和性能Fasthttp 中的 SliceMap性能优化的深层原因HTTP Headers 的特性CPU 预加载特性 结论 Fasthttp 是一个高性能的 Golang HTTP 框架,它在设计上做了许多优化以提高性能。其中一个显著的设计选择是使用 slice 而…...

C#设计模式教程(7):适配器模式
适配器模式的定义 适配器模式(Adapter Pattern)是一种结构型设计模式,它允许不兼容的接口之间能够相互合作。适配器的作用是解决那些因接口不兼容而不能一起工作的类的问题,它通过包装一个类的接口转换成另一个期望的接口。 适配器模式主要分为两种: 类适配器(Class Ad…...

1818:红与黑【解析】-------深度优先搜索
1818:红与黑 描述 有一间长方形的房子,地上铺了红色、黑色两种颜色的正方形瓷砖。你站在其中一块黑色的瓷砖上,只能向相邻的黑色瓷砖移动。请写一个程序,计算你总共能够到达多少块黑色的瓷砖。 输入 包括多个数据集合。每个数据集合的第一行…...
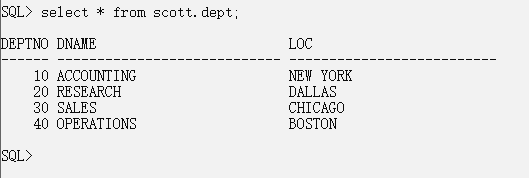
实验三 Oracle数据库的创建和管理
🕺作者: 主页 我的专栏C语言从0到1探秘C数据结构从0到1探秘Linux 😘欢迎关注:👍点赞🙌收藏✍️留言 🏇码字不易,你的👍点赞🙌收藏❤️关注对我真的很重要&…...
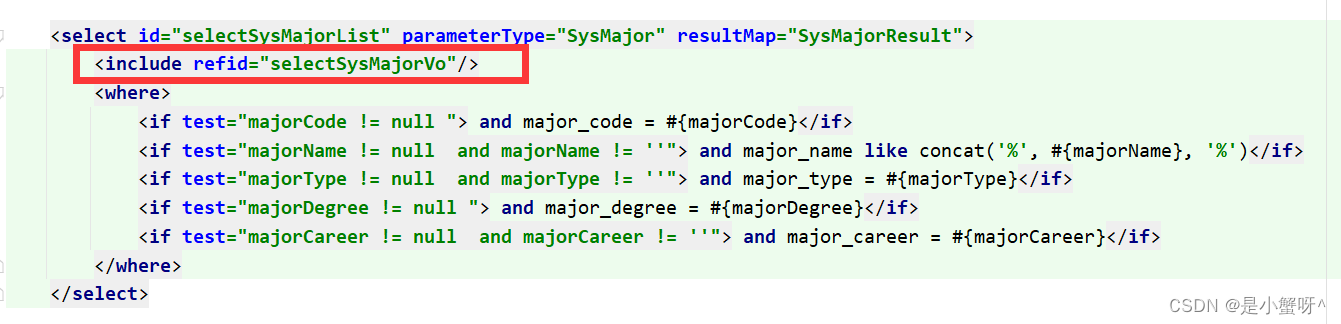
Mysql:重点且常用的 SQL 标签整理
目录 1 <resultMap> 标签 2 <sql> 标签 3 <where> 标签 4 <if> 标签 5 <trim> 标签 6 <foreach> 标签 7 <set> 标签 1 <resultMap> 标签 比如以下代码: <resultMap type"SysCollege" id&qu…...
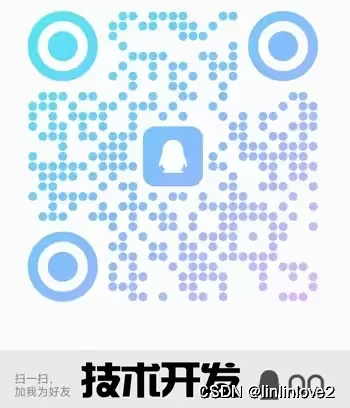
云锁防火墙编译安装nginx-plugin模块
一般情况下,当用户安装云锁的时候,云锁会自动适配nginx版本,使用我们已经预编译好的包含云锁模块的nginx备份并替换掉您当前系统中使用的nginx。卸载时,会将系统原始nginx文件替换回来。因此,云锁可保护使用nginx搭建的…...
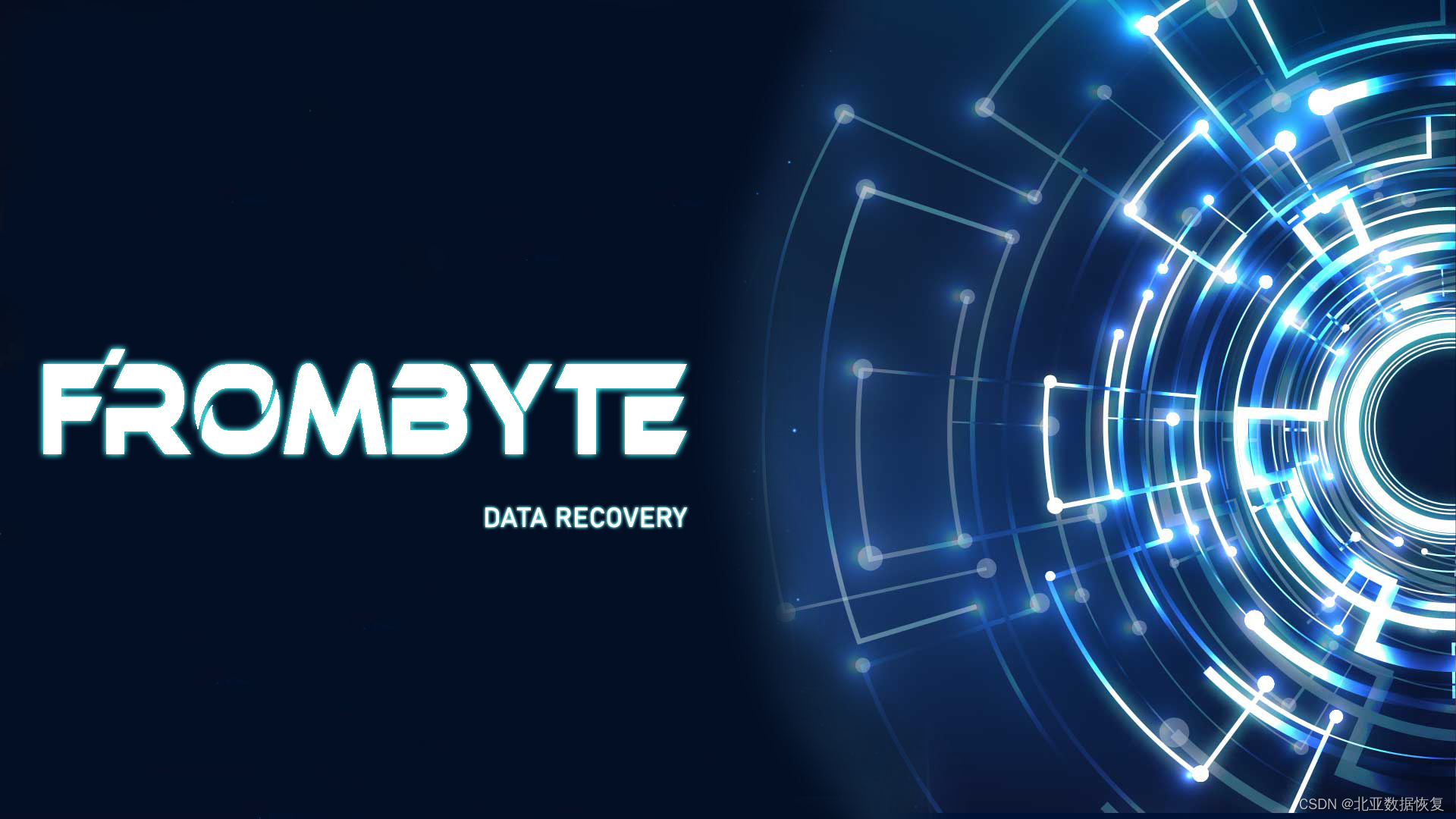
【服务器数据恢复】服务器迁移数据时lun数据丢失的数据恢复案例
服务器数据恢复环境&服务器故障: 一台安装Windows操作系统的服务器。工作人员在迁移该服务器中数据时突然无法读取数据,服务器管理界面出现报错。经过检查发现服务器中一个lun的数据丢失。 服务器数据恢复过程: 1、将故障服务器中所有磁盘…...
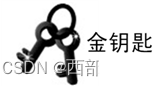
6.4.2转换文件
6.4.2转换文件 利用Swf2VideoConverter2可以很方便地将Flash动画(*.swf)转换为其它的视频格式。 1.单击“添加”按钮,在弹出的下拉菜单中选择“添加文件”,在弹出的“Open Swf Files(打开Swf文件)”窗口中选择swf文件(如:那些花…...
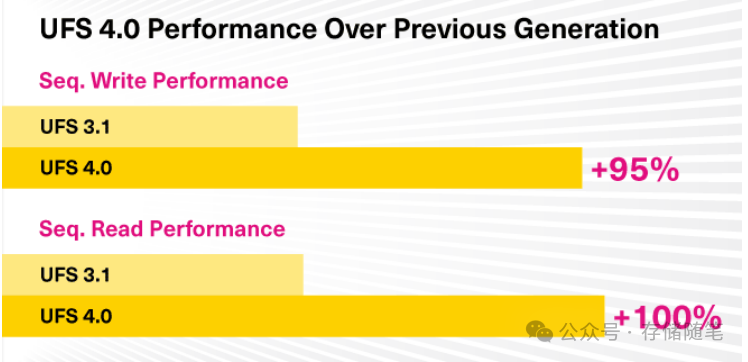
智能驾驶新浪潮:SSD与UFS存储技术如何破浪前行?-UFS篇
如果说SSD是赛道上的超级跑车,那UFS更像是专为智能汽车定制的高性能轻量化赛车。UFS采用串行接口技术,像是闪电侠一样,将数据传输的速度推向新高,大幅缩短了系统启动时间和应用程序加载时间,这对追求即时反应的ADAS系统…...

TS 学习笔录(持续更新中)
TS学习笔录 1、TS 数据类型有哪些?2、元组是什么?3、union(联合类型)& Literal(字面量类型)?4、any 和 unknown 的区别?5、Object 对象类型?6、type 、interface 、 class 之间…...
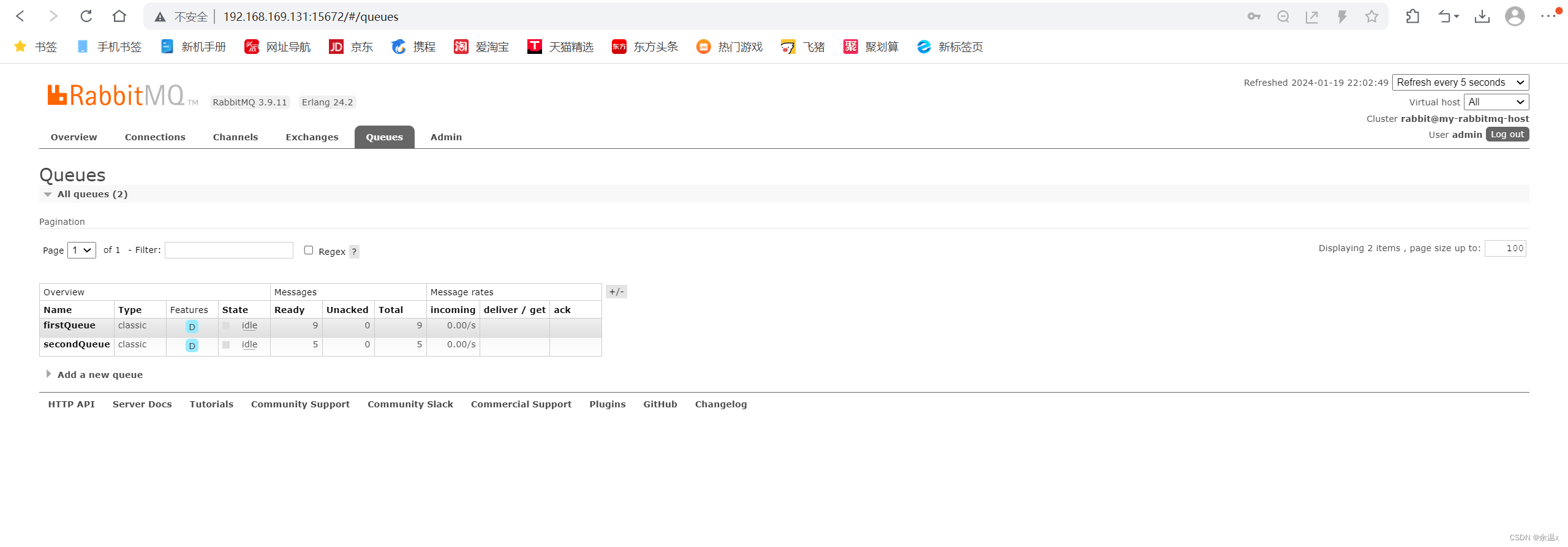
RabbitMQ安装和使用
简介 RabbitMQ是一套开源(MPL)的消息队列服务软件,是由LShift提供的一个Advanced Message Queuing Protocol (AMQP) 的开源实现,由以高性能、健壮以及可伸缩性出名的Erlang写成。所有主要的编程语言均有与代理接口通讯的客户端库…...
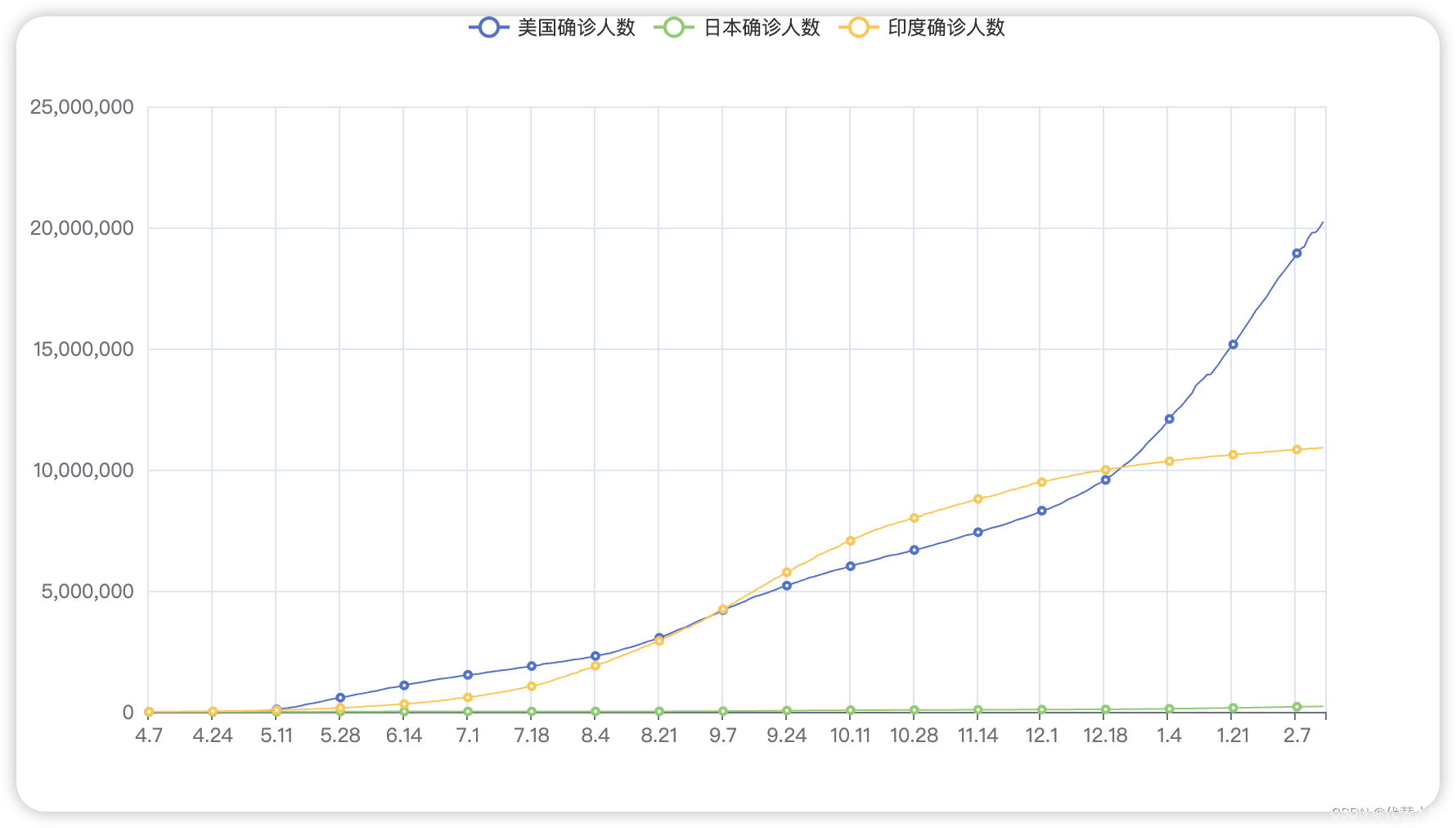
使用pyechart创建折线图
import json from pyecharts.charts import Line from pyecharts import options# 首先使用文件打开数据 f_us open(Desktop/python/Project/数据可视化/美国.txt,r,encoding"UTF-8") f_rb open(Desktop/python/Project/数据可视化/日本.txt,r,encoding"UTF-8…...

Vue3+Ts:使用i18n实现国际化与全局动态下拉框框切换语言
Vue3Ts:使用i18n实现国际化与全局动态下拉框框切换语言 一、下载依赖:二、创建ts文件并配置main.ts三,如何使用1.在<template>中使用2.在setup中使用 四、全局下拉框动态切换 一、下载依赖: npm install vue-i18nnex二、创…...
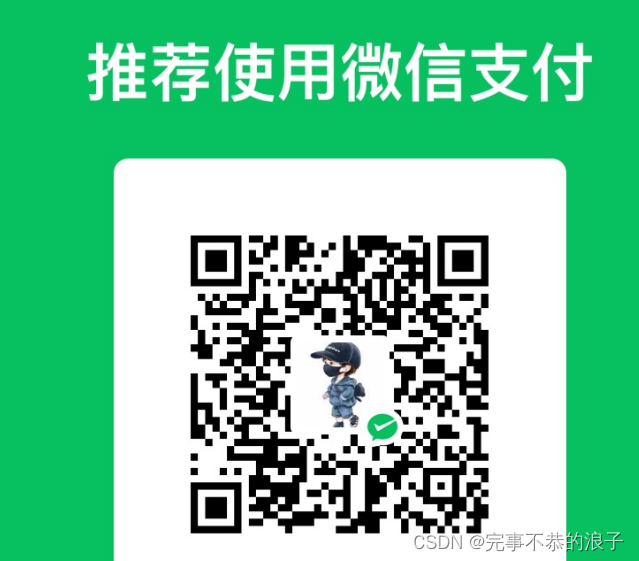
多目标优化中常用的差分进化算法DE【2】
# 多目标优化中常用的进化算法 1、链接一 2、链接二 #后续继续补充多目标的差分进化算法MODE的应用 此链接介绍很详细,此处用来分享学习,后续有问题会继续进行补充。 如果你觉得不错,佛系随缘打赏,感谢,你的支持是…...
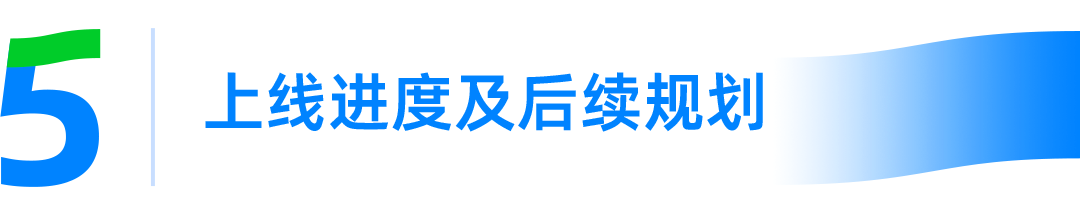
游卡:OceanBase在游戏核心业务的规模化降本实践
从 2023 年 9 月测试 OceanBase,到如今 3 个核心业务应用 OceanBase,国内最早卡牌游戏研发者之一的游卡仅用了两个月。是什么原因让游卡放弃游戏行业通用的 MySQL方案,选择升级至 OceanBase?杭州游卡网络技术有限公司(…...

LightDB - oracle_fdw 过滤条件下推增强【24.1】
LightDB - oracle_fdw 过滤条件下推增强【24.1】 1. 字符串比较下推1.1 示例 2. 隐式转换下推2.1 示例 3. nvl 和trim 下推3.1 示例 LightDB 在24.1版本对oracle_fdw 的where下推进行了增强,新增对如下两种情况进行下推: 字符串比较下推,如 …...
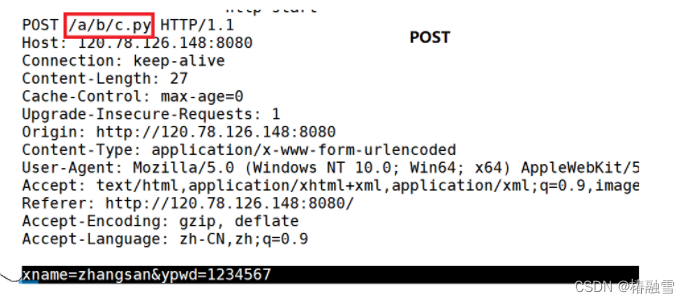
【计算机网络】HTTP协议以及简单的HTTP服务器实现
文章目录 一、HTTP协议1.认识URL2.urlencode和urldecode3.HTTP协议格式4.HTTP的方法5.HTTP的状态码6.HTTP常见Header7.重定向8.长连接9.会话保持10.基本工具 二、简单的HTTP服务器实现1.err.hpp2.log.hpp3.procotol.hpp4.Sock.hpp5.Util.hpp6.httpServer.hpp7.httpServer.cc8.总…...
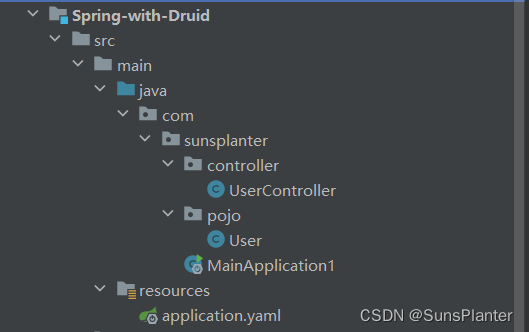
04 SpringBoot整合Druid/MyBatis/事务/AOP+打包项目
整合Druid 项目结构: 引入依赖: <?xml version"1.0" encoding"UTF-8"?> <project xmlns"http://maven.apache.org/POM/4.0.0"xmlns:xsi"http://www.w3.org/2001/XMLSchema-instance"xsi:schemaL…...

C++程序编译时的_GLIBCXX_USE_CXX11_ABI参数的值选择,适配昇腾Transformer推理加速库与LLM推理模型库
目录 2024/1/19日更新确定已安装G编译测试程序获取宏值安装对应的Transformer LLM推理模型库和Transformer推理加速库小结 2024/1/19日更新 具体使用cxx11abi0 还是cxx11abi1 可通过python命令查询 import torch torch.compiled_with_cxx11_abi()若返回True 则使用 cxx11abi1…...

什么是站群服务器?
网站群服务器是管理多个网站的强大工具,可以帮助站长轻松管理和维护多个网站,提高网站运营效率。在本文中,我们将讨论站点组服务器的优势,以及为什么它是网站管理员不可或缺的工具。 介绍站群服务器 网站群服务器是一个集中管理…...
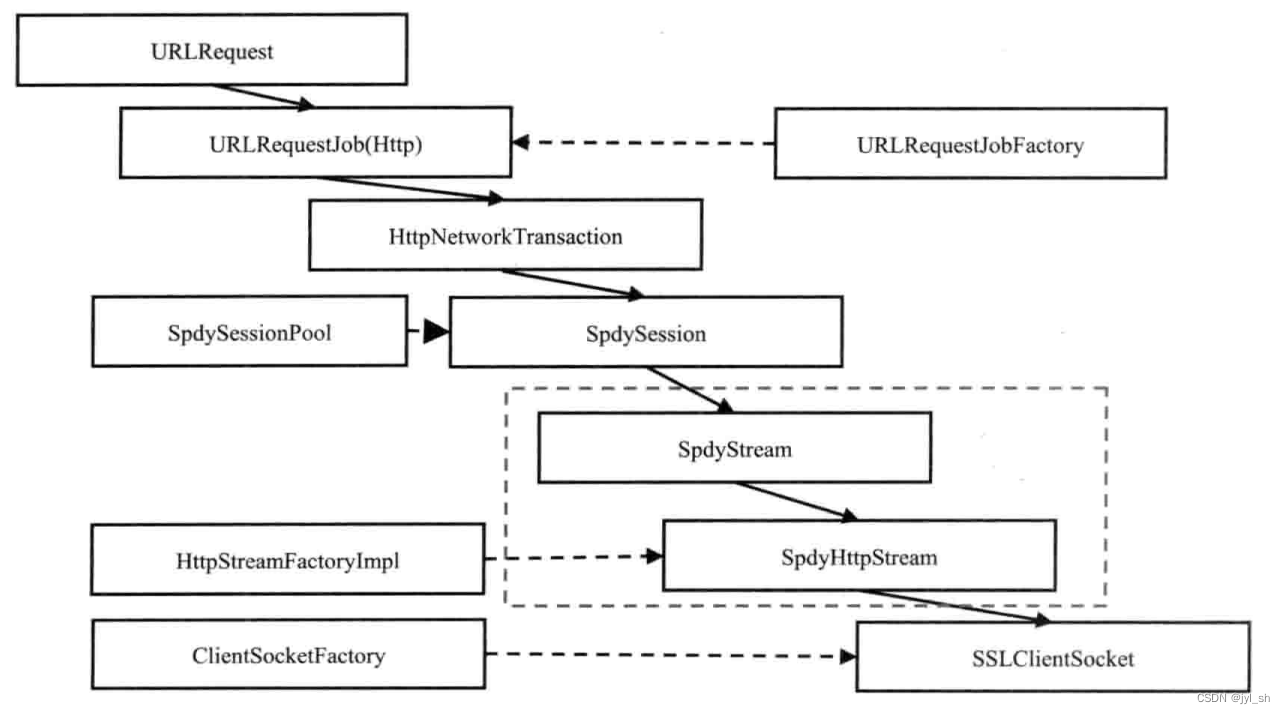
《WebKit 技术内幕》之四(3): 资源加载和网络栈
3. 网络栈 3.1 WebKit的网络设施 WebKit的资源加载其实是交由各个移植来实现的,所以WebCore其实并没有什么特别的基础设施,每个移植的网络实现是非常不一样的。 从WebKit的代码结构中可以看出,网络部分代码的确比较少的,它们都在…...
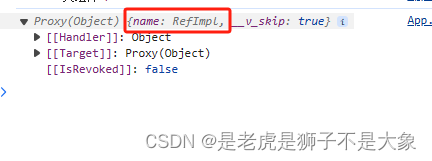
vue3-模板引用
//1.调用ref函数 -> ref对象 const h1Ref ref(null) const comRef ref(null) //组件挂载完毕之后才能获取 onMounted(()>{console.log(h1Ref.value);console.log(comRef.value); })<div class"father"><!-- 通过ref标识绑定ref对象 --><h2 re…...
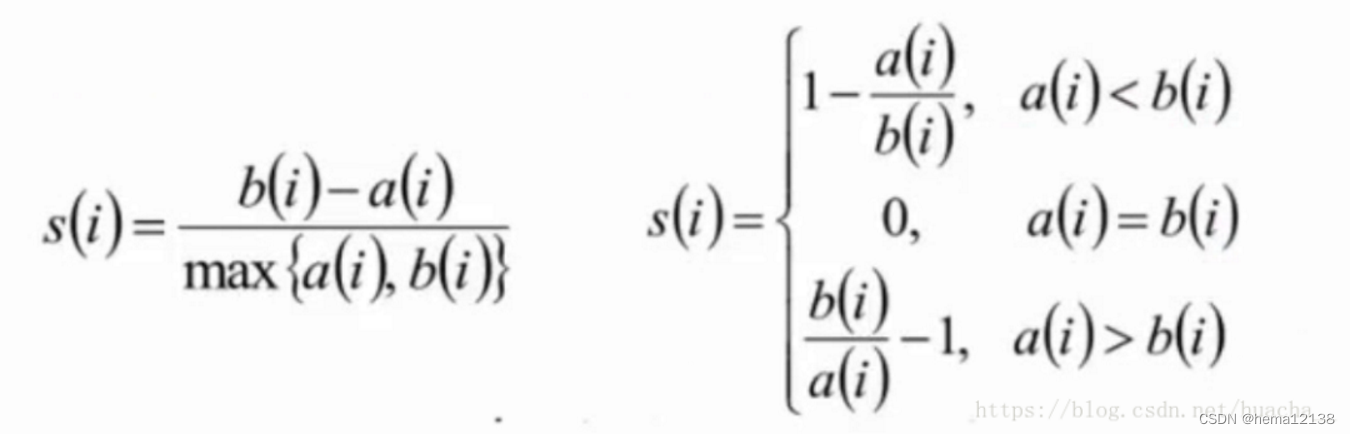
聚类模型评估指标
聚类模型评估指标-轮廓系数 计算样本i到同簇其它样本到平均距离ai,ai越小,说明样本i越应该被聚类到该簇(将ai称为样本i到簇内不相似度);计算样本i到其它某簇Cj的所有样本的平均距离bij,称为样本i与簇Cj的…...
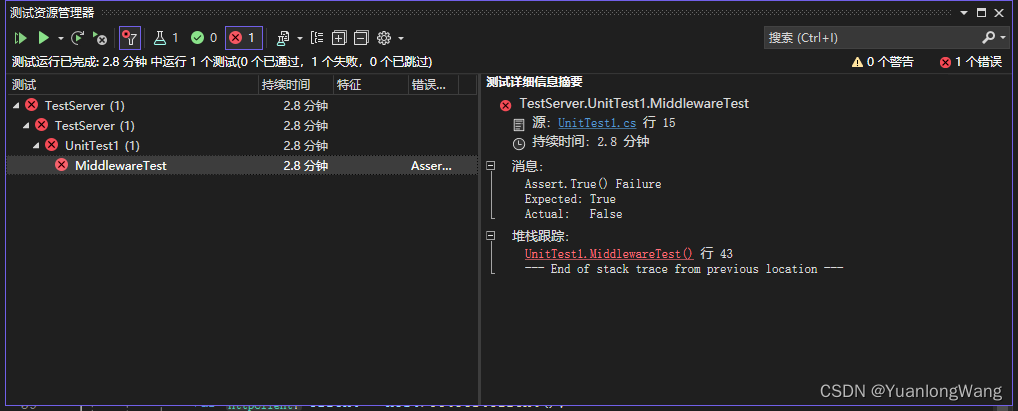
测试 ASP.NET Core 中间件
正常情况下,中间件会在主程序入口统一进行实例化,这样如果想单独测试某一个中间件就很不方便,为了能测试单个中间件,可以使用 TestServer 单独测试。 这样便可以: 实例化只包含需要测试的组件的应用管道。发送自定义请…...