Chromium 中chrome.history扩展接口c++实现
一、前端 chrome.history定义
使用 chrome.history
API 与浏览器的已访问网页的记录进行交互。您可以在浏览器的历史记录中添加、移除和查询网址。如需使用您自己的版本替换历史记录页面,请参阅覆盖网页。
更多参考:chrome.history | API | Chrome for Developers (google.cn)
示例
若要试用此 API,请安装 chrome-extension-samples 中的 history API 示例 存储库
二、history接口在c++定义
chrome\common\extensions\api\history.json
out\Debug\gen\chrome\common\extensions\api\history.cc
src\out\Debug\gen\chrome\common\extensions\api\history.h
// Copyright 2012 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.[{"namespace": "history","description": "Use the <code>chrome.history</code> API to interact with the browser's record of visited pages. You can add, remove, and query for URLs in the browser's history. To override the history page with your own version, see <a href='override'>Override Pages</a>.","types": [{"id": "TransitionType","type": "string","enum": ["link", "typed", "auto_bookmark", "auto_subframe", "manual_subframe", "generated", "auto_toplevel", "form_submit", "reload", "keyword", "keyword_generated"],"description": "The <a href='#transition_types'>transition type</a> for this visit from its referrer."},{"id": "HistoryItem","type": "object","description": "An object encapsulating one result of a history query.","properties": {"id": {"type": "string", "minimum": 0, "description": "The unique identifier for the item."},"url": {"type": "string", "optional": true, "description": "The URL navigated to by a user."},"title": {"type": "string", "optional": true, "description": "The title of the page when it was last loaded."},"lastVisitTime": {"type": "number", "optional": true, "description": "When this page was last loaded, represented in milliseconds since the epoch."},"visitCount": {"type": "integer", "optional": true, "description": "The number of times the user has navigated to this page."},"typedCount": {"type": "integer", "optional": true, "description": "The number of times the user has navigated to this page by typing in the address."}}},{"id": "VisitItem","type": "object","description": "An object encapsulating one visit to a URL.","properties": {"id": {"type": "string", "minimum": 0, "description": "The unique identifier for the corresponding $(ref:history.HistoryItem)."},"visitId": {"type": "string", "description": "The unique identifier for this visit."},"visitTime": {"type": "number", "optional": true, "description": "When this visit occurred, represented in milliseconds since the epoch."},"referringVisitId": {"type": "string", "description": "The visit ID of the referrer."},"transition": {"$ref": "TransitionType","description": "The <a href='#transition_types'>transition type</a> for this visit from its referrer."},"isLocal": { "type": "boolean", "description": "True if the visit originated on this device. False if it was synced from a different device." }}},{"id": "UrlDetails","type": "object","properties": {"url": {"type": "string", "description": "The URL for the operation. It must be in the format as returned from a call to history.search."}}}],"functions": [{"name": "search","type": "function","description": "Searches the history for the last visit time of each page matching the query.","parameters": [{"name": "query","type": "object","properties": {"text": {"type": "string", "description": "A free-text query to the history service. Leave empty to retrieve all pages."},"startTime": {"type": "number", "optional": true, "description": "Limit results to those visited after this date, represented in milliseconds since the epoch. If not specified, this defaults to 24 hours in the past."},"endTime": {"type": "number", "optional": true, "description": "Limit results to those visited before this date, represented in milliseconds since the epoch."},"maxResults": {"type": "integer", "optional": true, "minimum": 0, "description": "The maximum number of results to retrieve. Defaults to 100."}}}],"returns_async": {"name": "callback","parameters": [{ "name": "results", "type": "array", "items": { "$ref": "HistoryItem"} }]}},{"name": "getVisits","type": "function","description": "Retrieves information about visits to a URL.","parameters": [{"name": "details","$ref": "UrlDetails"}],"returns_async": {"name": "callback","parameters": [{ "name": "results", "type": "array", "items": { "$ref": "VisitItem"} }]}},{"name": "addUrl","type": "function","description": "Adds a URL to the history at the current time with a <a href='#transition_types'>transition type</a> of \"link\".","parameters": [{"name": "details","$ref": "UrlDetails"}],"returns_async": {"name": "callback","optional": true,"parameters": []}},{"name": "deleteUrl","type": "function","description": "Removes all occurrences of the given URL from the history.","parameters": [{"name": "details","$ref": "UrlDetails"}],"returns_async": {"name": "callback","optional": true,"parameters": []}},{"name": "deleteRange","type": "function","description": "Removes all items within the specified date range from the history. Pages will not be removed from the history unless all visits fall within the range.","parameters": [{"name": "range","type": "object","properties": {"startTime": { "type": "number", "description": "Items added to history after this date, represented in milliseconds since the epoch." },"endTime": { "type": "number", "description": "Items added to history before this date, represented in milliseconds since the epoch." }}}],"returns_async": {"name": "callback","parameters": []}},{"name": "deleteAll","type": "function","description": "Deletes all items from the history.","parameters": [],"returns_async": {"name": "callback","parameters": []}}],"events": [{"name": "onVisited","type": "function","description": "Fired when a URL is visited, providing the HistoryItem data for that URL. This event fires before the page has loaded.","parameters": [{ "name": "result", "$ref": "HistoryItem"}]},{"name": "onVisitRemoved","type": "function","description": "Fired when one or more URLs are removed from the history service. When all visits have been removed the URL is purged from history.","parameters": [{"name": "removed","type": "object","properties": {"allHistory": { "type": "boolean", "description": "True if all history was removed. If true, then urls will be empty." },"urls": { "type": "array", "items": { "type": "string" }, "optional": true}}}]}]}
]
三、history API接口定义文件:
chrome\browser\extensions\api\bookmarks\bookmarks_api.h
chrome\browser\extensions\api\bookmarks\bookmarks_api.cc
// Copyright 2012 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.#ifndef CHROME_BROWSER_EXTENSIONS_API_HISTORY_HISTORY_API_H_
#define CHROME_BROWSER_EXTENSIONS_API_HISTORY_HISTORY_API_H_#include <string>
#include <vector>#include "base/memory/raw_ptr.h"
#include "base/scoped_observation.h"
#include "base/task/cancelable_task_tracker.h"
#include "base/values.h"
#include "chrome/common/extensions/api/history.h"
#include "components/history/core/browser/history_service.h"
#include "components/history/core/browser/history_service_observer.h"
#include "extensions/browser/browser_context_keyed_api_factory.h"
#include "extensions/browser/event_router.h"
#include "extensions/browser/extension_function.h"class Profile;namespace extensions {// Observes History service and routes the notifications as events to the
// extension system.
class HistoryEventRouter : public history::HistoryServiceObserver {public:HistoryEventRouter(Profile* profile,history::HistoryService* history_service);HistoryEventRouter(const HistoryEventRouter&) = delete;HistoryEventRouter& operator=(const HistoryEventRouter&) = delete;~HistoryEventRouter() override;private:// history::HistoryServiceObserver.void OnURLVisited(history::HistoryService* history_service,const history::URLRow& url_row,const history::VisitRow& new_visit) override;void OnURLsDeleted(history::HistoryService* history_service,const history::DeletionInfo& deletion_info) override;void DispatchEvent(Profile* profile,events::HistogramValue histogram_value,const std::string& event_name,base::Value::List event_args);raw_ptr<Profile> profile_;base::ScopedObservation<history::HistoryService,history::HistoryServiceObserver>history_service_observation_{this};
};class HistoryAPI : public BrowserContextKeyedAPI, public EventRouter::Observer {public:explicit HistoryAPI(content::BrowserContext* context);~HistoryAPI() override;// KeyedService implementation.void Shutdown() override;// BrowserContextKeyedAPI implementation.static BrowserContextKeyedAPIFactory<HistoryAPI>* GetFactoryInstance();// EventRouter::Observer implementation.void OnListenerAdded(const EventListenerInfo& details) override;private:friend class BrowserContextKeyedAPIFactory<HistoryAPI>;raw_ptr<content::BrowserContext> browser_context_;// BrowserContextKeyedAPI implementation.static const char* service_name() {return "HistoryAPI";}static const bool kServiceIsNULLWhileTesting = true;// Created lazily upon OnListenerAdded.std::unique_ptr<HistoryEventRouter> history_event_router_;
};template <>
void BrowserContextKeyedAPIFactory<HistoryAPI>::DeclareFactoryDependencies();// Base class for history function APIs.
class HistoryFunction : public ExtensionFunction {protected:~HistoryFunction() override {}bool ValidateUrl(const std::string& url_string,GURL* url,std::string* error);bool VerifyDeleteAllowed(std::string* error);base::Time GetTime(double ms_from_epoch);Profile* GetProfile() const;
};// Base class for history funciton APIs which require async interaction with
// chrome services and the extension thread.
class HistoryFunctionWithCallback : public HistoryFunction {public:HistoryFunctionWithCallback();protected:~HistoryFunctionWithCallback() override;// The task tracker for the HistoryService callbacks.base::CancelableTaskTracker task_tracker_;
};class HistoryGetVisitsFunction : public HistoryFunctionWithCallback {public:DECLARE_EXTENSION_FUNCTION("history.getVisits", HISTORY_GETVISITS)protected:~HistoryGetVisitsFunction() override {}// ExtensionFunction:ResponseAction Run() override;// Callback for the history function to provide results.void QueryComplete(history::QueryURLResult result);
};class HistorySearchFunction : public HistoryFunctionWithCallback {public:DECLARE_EXTENSION_FUNCTION("history.search", HISTORY_SEARCH)protected:~HistorySearchFunction() override {}// ExtensionFunction:ResponseAction Run() override;// Callback for the history function to provide results.void SearchComplete(history::QueryResults results);
};class HistoryAddUrlFunction : public HistoryFunction {public:DECLARE_EXTENSION_FUNCTION("history.addUrl", HISTORY_ADDURL)protected:~HistoryAddUrlFunction() override {}// ExtensionFunction:ResponseAction Run() override;
};class HistoryDeleteAllFunction : public HistoryFunctionWithCallback {public:DECLARE_EXTENSION_FUNCTION("history.deleteAll", HISTORY_DELETEALL)protected:~HistoryDeleteAllFunction() override {}// ExtensionFunction:ResponseAction Run() override;// Callback for the history service to acknowledge deletion.void DeleteComplete();
};class HistoryDeleteUrlFunction : public HistoryFunction {public:DECLARE_EXTENSION_FUNCTION("history.deleteUrl", HISTORY_DELETEURL)protected:~HistoryDeleteUrlFunction() override {}// ExtensionFunction:ResponseAction Run() override;
};class HistoryDeleteRangeFunction : public HistoryFunctionWithCallback {public:DECLARE_EXTENSION_FUNCTION("history.deleteRange", HISTORY_DELETERANGE)protected:~HistoryDeleteRangeFunction() override {}// ExtensionFunction:ResponseAction Run() override;// Callback for the history service to acknowledge deletion.void DeleteComplete();
};} // namespace extensions#endif // CHROME_BROWSER_EXTENSIONS_API_HISTORY_HISTORY_API_H_
四、看下扩展调用history.getVisits 堆栈:
总结:
相关文章:
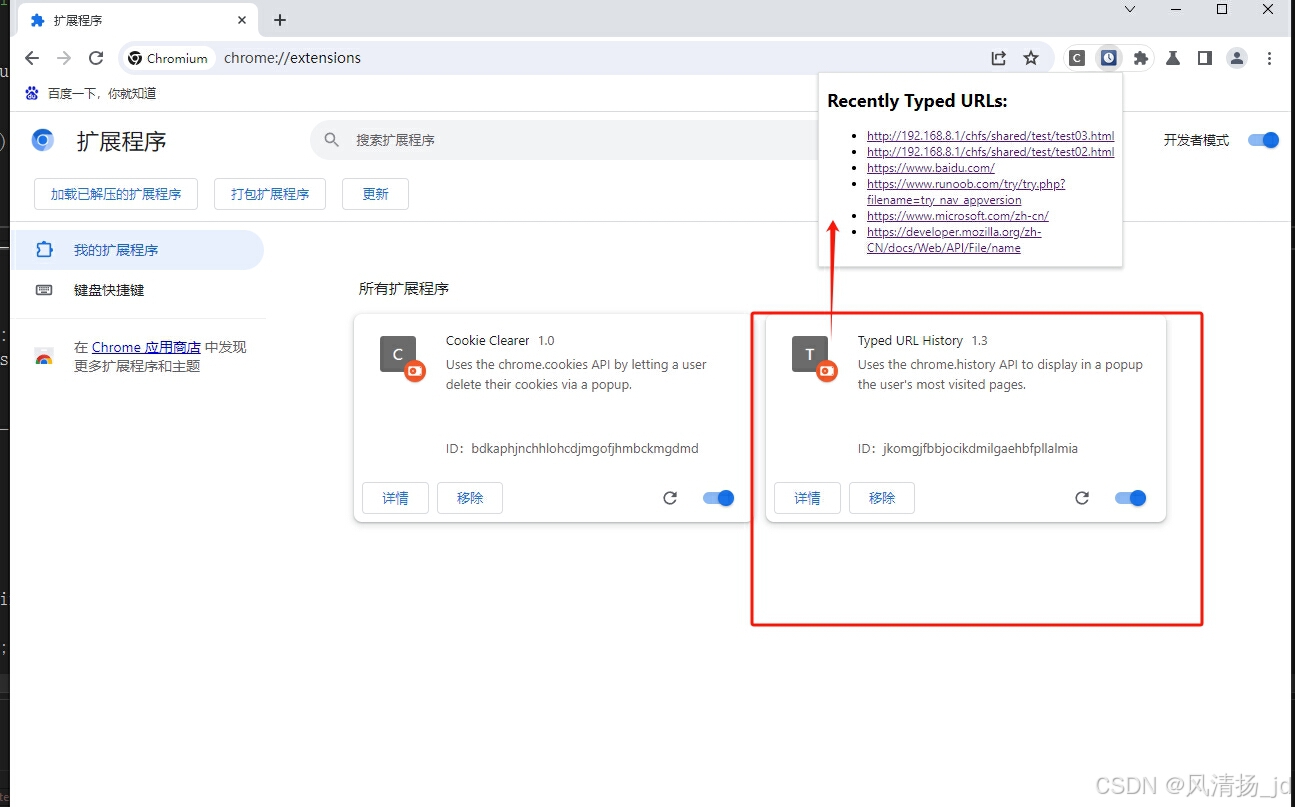
Chromium 中chrome.history扩展接口c++实现
一、前端 chrome.history定义 使用 chrome.history API 与浏览器的已访问网页的记录进行交互。您可以在浏览器的历史记录中添加、移除和查询网址。如需使用您自己的版本替换历史记录页面,请参阅覆盖网页。 更多参考:chrome.history | API | Chrome…...
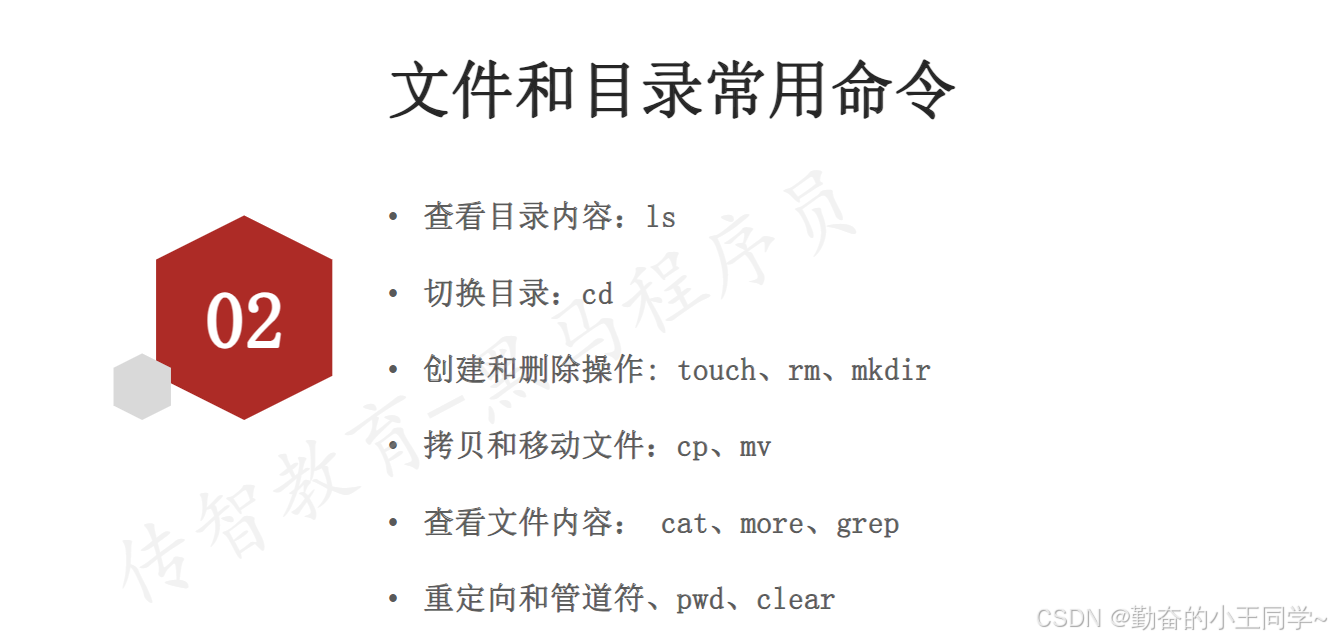
(Linux和数据库)1.Linux操作系统和常用命令
了解Linux操作系统介绍 除了办公和玩游戏之外不用Linux,其他地方都要使用Linux(it相关) iOS的本质是unix(unix是付费版本的操作系统) unix和Linux之间很相似 Linux文件系统和目录 bin目录--放工具使用的 操作Linux远程…...
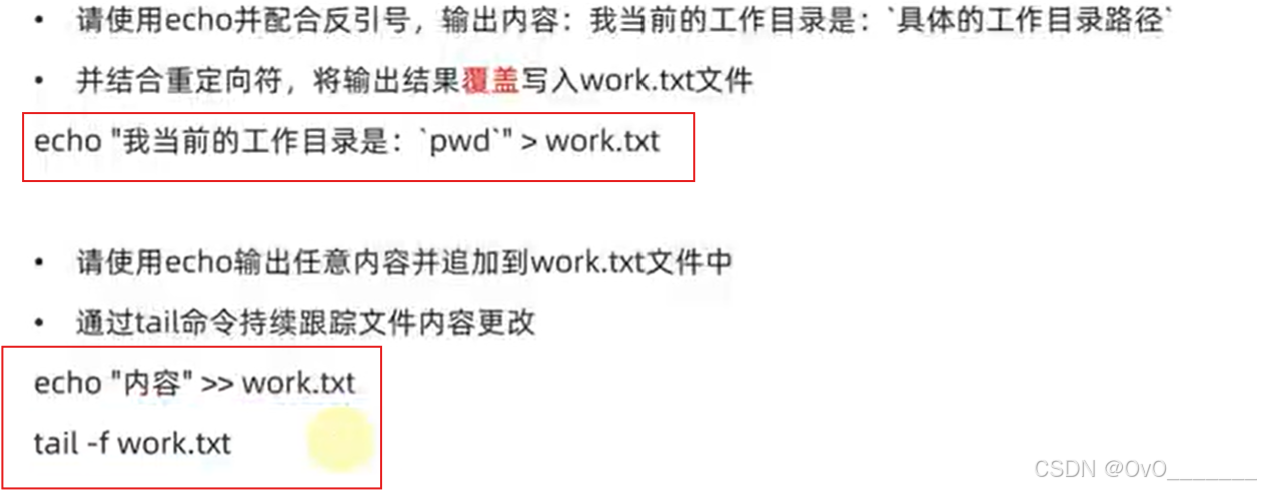
Linux——echo-tail-重定向符
echo命令 类似printf 输出 反引号 重定向符 > 和 >> > 覆盖 >> 追加 tail命令 查看文件尾部内容,追踪文件最新更改 tail -num 从尾部往上读num行,默认10行 tail -f 持续跟踪...
GitHub Copilot 使用手册(一)--配置
一、 什么是GitHub Copilot GitHub Copilot 是GitHub和OpenAI合作开发的一个人工智能工具,在使用Visual Studio Code、Microsoft Visual Studio、Vim、Cursor或JetBrains等IDE时可以协助用户编写代码等工作,实现虚拟的结对编程。 二、 GitHub Copilot …...
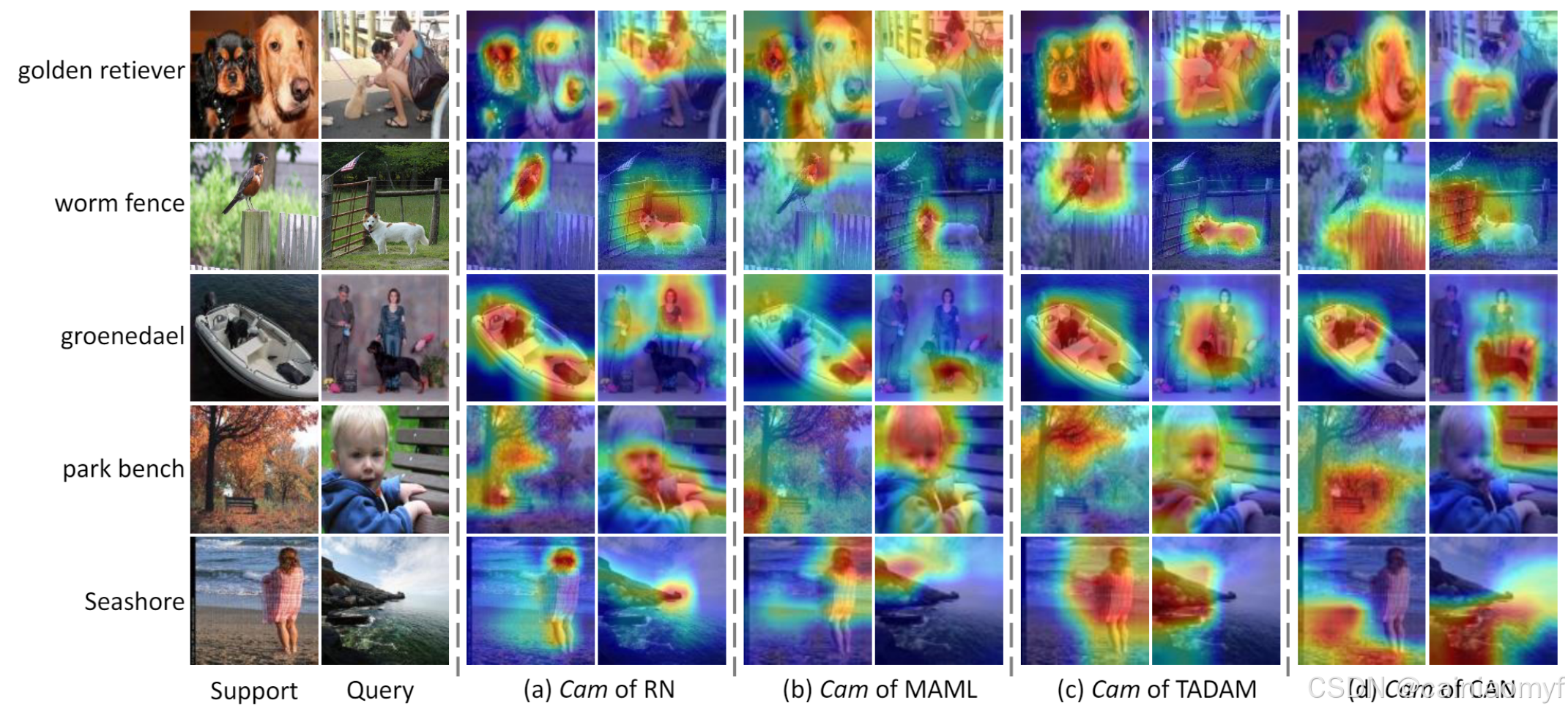
【论文阅读】Cross Attention Network for Few-shot Classification
用于小样本分类的交叉注意力网络 引用:Hou, Ruibing, et al. “Cross attention network for few-shot classification.” Advances in neural information processing systems 32 (2019). 论文地址:下载地址 论文代码:https://github.com/bl…...
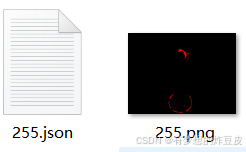
CV图像处理小工具——json文件转P格式mask
CV图像处理小工具——json文件转P格式mask import cv2 import json import numpy as np import osdef func(file_path: str) -> np.ndarray:try:with open(file_path, moder, encoding"utf-8") as f:configs json.load(f)# 检查JSON是否包含必要的字段if "…...
Typora 快捷键操作大全
Typora 是一款简洁的 Markdown 编辑器,它提供了一些快捷键来帮助用户更高效地编辑文档。以下是一些常用的 Typora 快捷键,这些快捷键可能会根据操作系统有所不同(Windows 和 macOS): 常用格式化快捷键 加粗ÿ…...
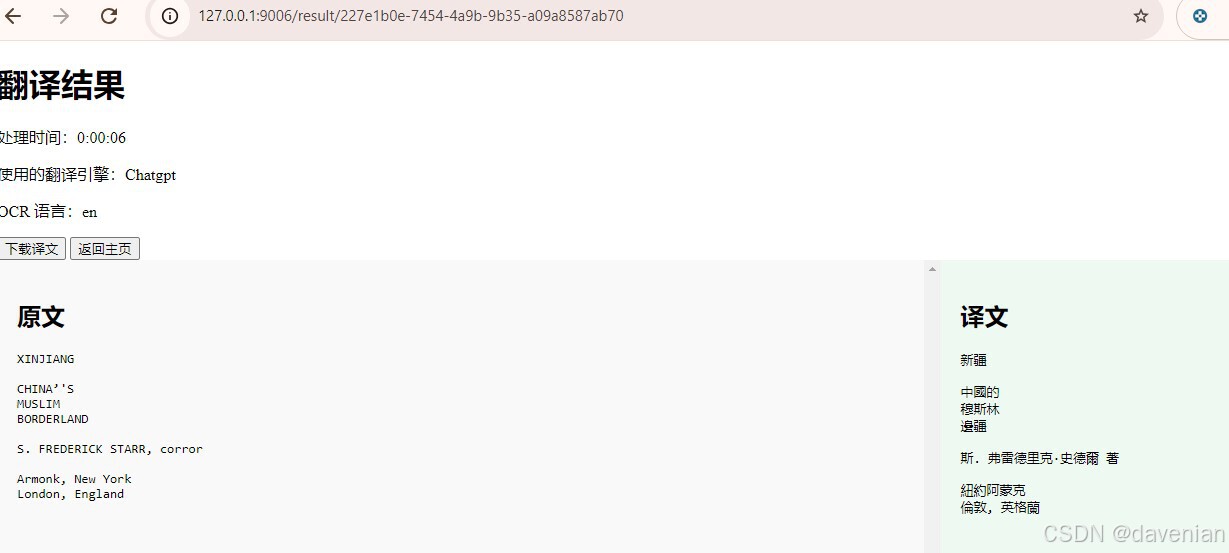
<Project-8.1.1 pdf2tx-mm> Python 调用 ChatGPT API 翻译PDF内容 历程心得
原因 用ZhipuAI,测试用的PDF里,有国名西部省穆斯林,翻译结果返回 “系统检测到输入或生成内容可能包含不安全或敏感内容,请您避免输入易产生敏感内容的提 示语,感谢您的配合” 。想过先替换掉省名、民族名等ÿ…...
JDK1.1主要特性
JDK 1.1,也被称为Java Development Kit 1.1,是Java编程语言的第一个更新版本,由Sun Microsystems公司在1997年发布。JDK 1.1在JDK 1.0的基础上进行了许多重要的改进和扩展,进一步巩固了Java作为一种强大、安全的编程语言和平台的地…...
软件测试工作中-商城类项目所遇bug点
商城的 bug 1、跨设备同步问题 当用户在不同设备上使用同一个账户时,购物车数据无法正确同步这可能是由于购物车数据存储和同步机制不完善,导致购物车内容在设备之间无法实时更新。怎么解决:开发把同步机制代码修改了一下,就不会出现这个 bug 了。 2、数…...
Java多线程面试题
1.进程和线程的区别 程序由指令和数据组成,但这些指令要运行,数据要读写,就必须将指令加载至 CPU中,数据加载至内存。在指令运行过程中还需要用到磁盘、网络等设备。进程就是用来加载指令、管理内存、管理 IO 的。 当一个程序被运…...
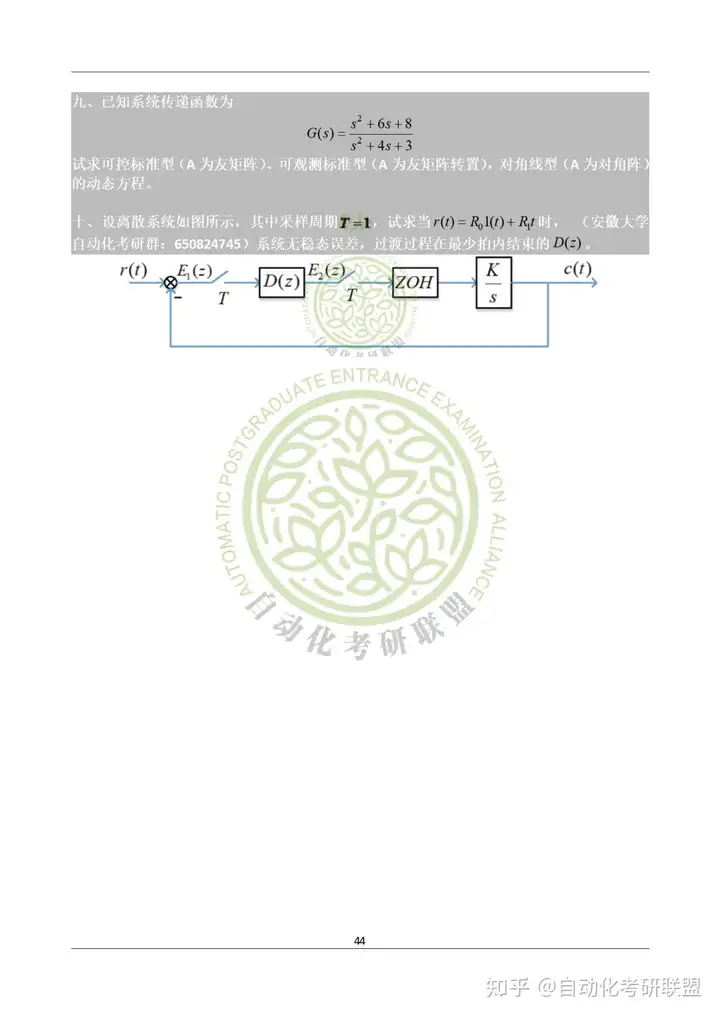
安徽大学《2022年+2023年831自动控制原理真题》 (完整版)
本文内容,全部选自自动化考研联盟的:《安徽大学831自控考研资料》的真题篇。后续会持续更新更多学校,更多年份的真题,记得关注哦~ 目录 2022年真题 2023年真题 Part1:2022年2023年完整版真题 2022年真题 2023年真题…...
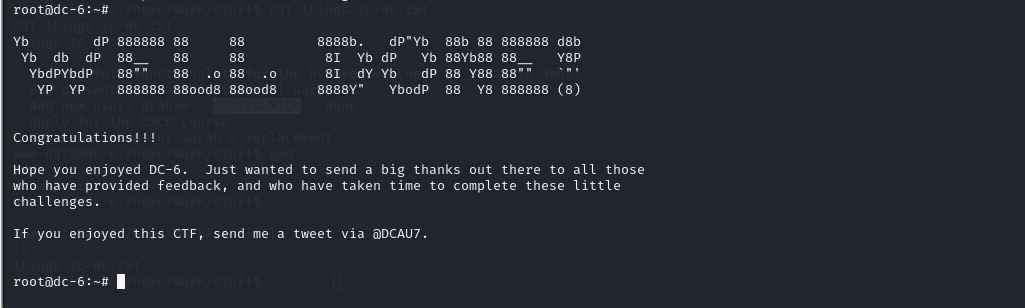
Vulnhub靶场案例渗透[6]- DC6
文章目录 1. 靶场搭建2. 信息收集2.1 确定靶机ip2.2 主机信息收集2.3 主机目录扫描2.4 网站用户名和密码爆破 3. 反弹shell4. 提权 1. 靶场搭建 靶场源地址 检验下载文件的检验码,对比没问题使用vmware打开 # windwos 命令 Get-FileHash <filePath> -Algori…...
FreeSWITCH 分机网关路由
不废话了,直接贴代码: --[[作用:分机网关呼叫第一个参数: 分机号码第二个参数: 被叫号码第三个参数: 主叫号码使用例子:<extension name"usergw"><condition><action applicatio…...
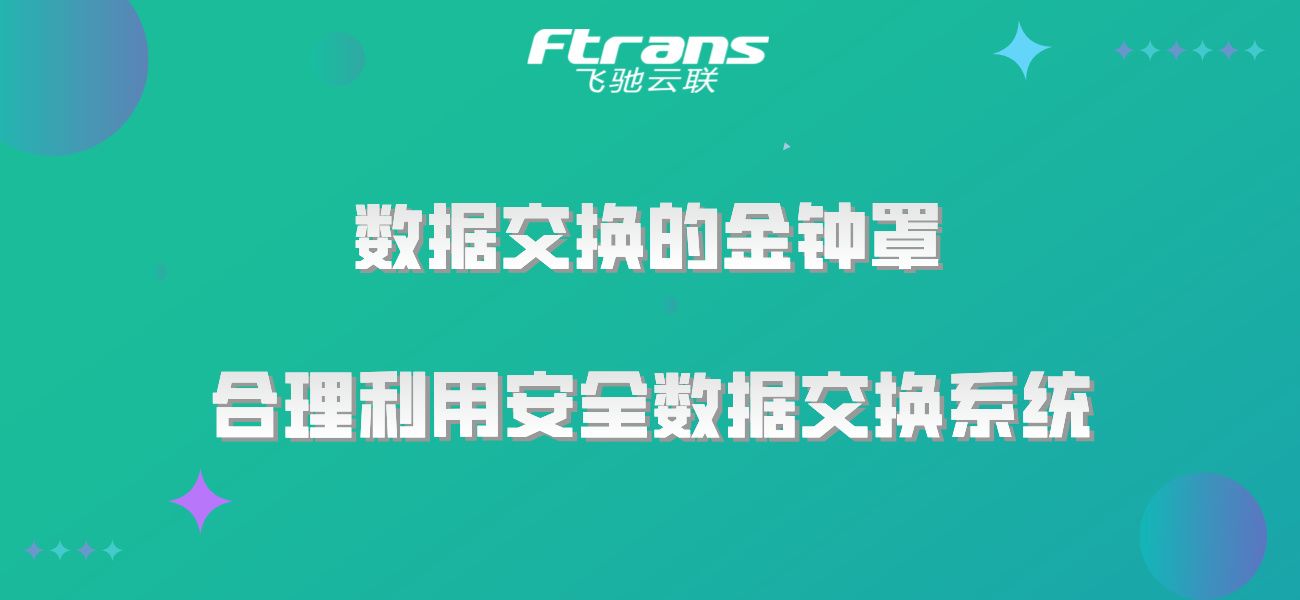
数据交换的金钟罩:合理利用安全数据交换系统,确保信息安全
政府单位为了保护网络不受外部威胁和内部误操作的影响,通常会进行网络隔离,隔离成内网和外网。安全数据交换系统是专门设计用于在不同的网络环境(如内部不同网络,内部网络和外部网络)之间安全传输数据的解决方案。 使用…...
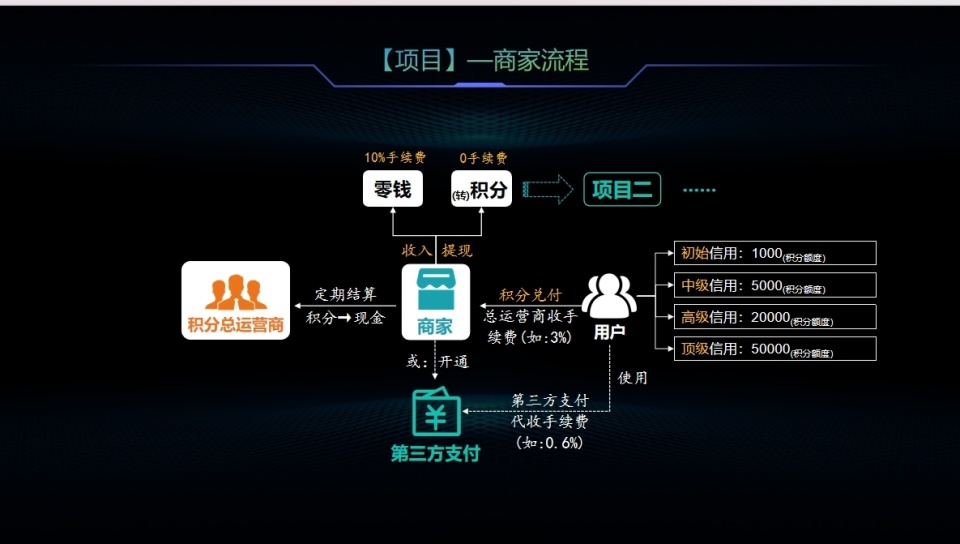
区块链积分系统:重塑支付安全与商业创新的未来
在当今社会,数字化浪潮席卷全球,支付安全与风险管理议题日益凸显。随着交易频次与规模的不断扩大,传统支付体系正面临前所未有的效率、合规性和安全挑战。 区块链技术,凭借其去中心化、高透明度以及数据不可篡改的特性,…...
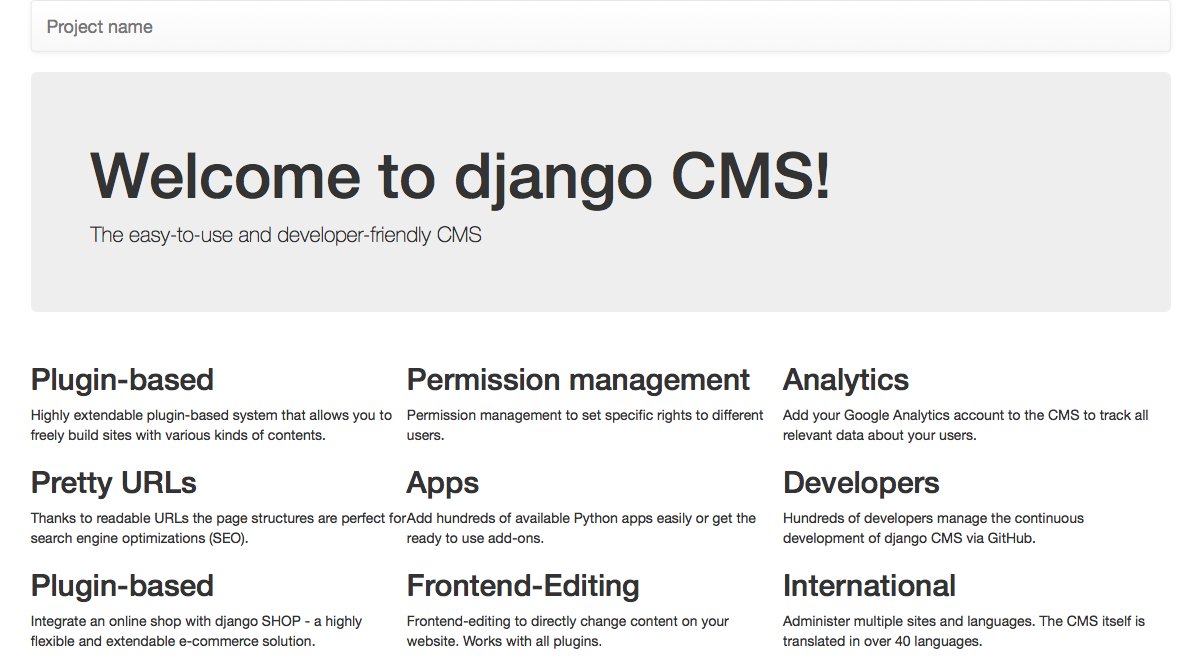
Django学习笔记十三:优秀案例学习
Django CMS 是一个基于 Django 框架的开源内容管理系统,它允许开发者轻松地创建和管理网站内容。Django CMS 提供了一个易于使用的界面来实现动态网站的快速开发,并且具有丰富的内容管理功能和多种插件扩展。以下是 Django CMS 的一些核心特性和如何开始…...
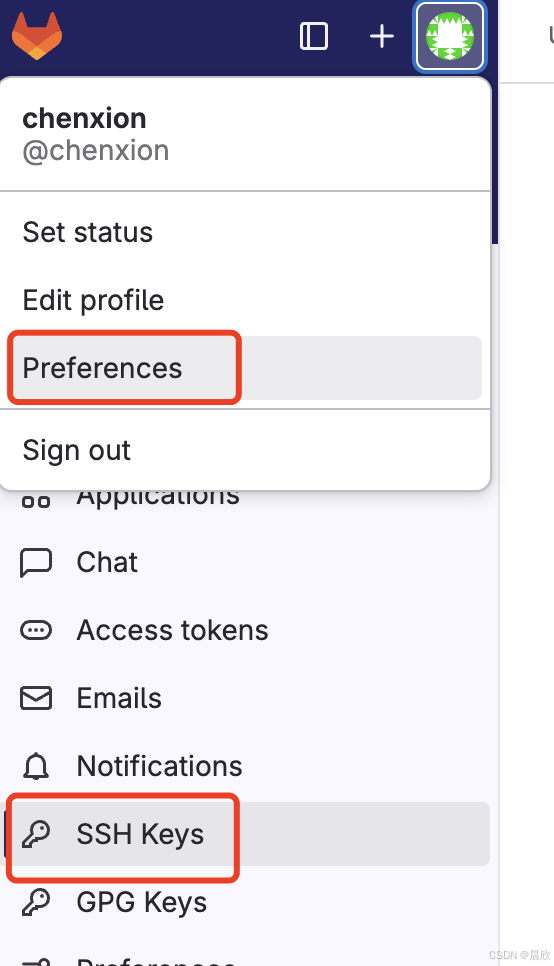
SSH 公钥认证:从gitlab clone项目repo到本地
这篇文章的分割线以下文字内容由 ChatGPT 生成(我稍微做了一些文字上的调整和截图的补充),我review并实践后觉得内容没有什么问题,由此和大家分享。 假如你想通过 git clone git10.12.5.19:your_project.git 命令将 git 服务器上…...
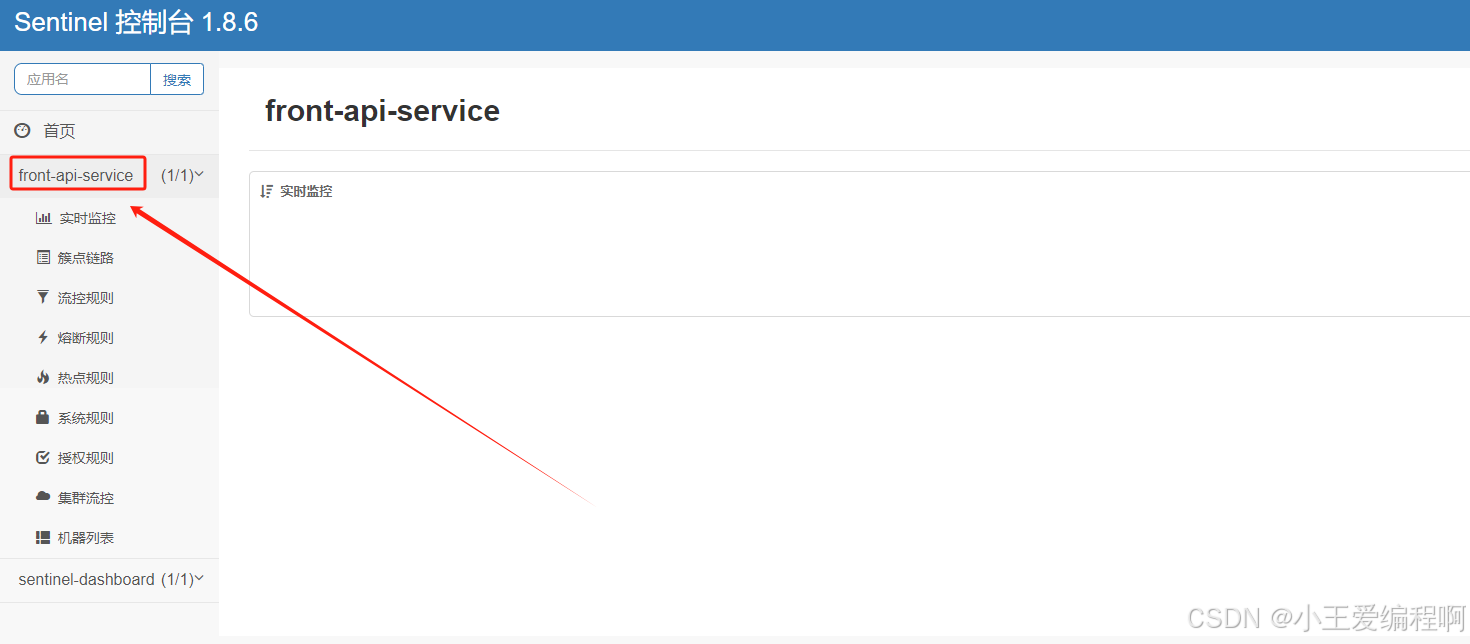
linux 搭建sentinel
1.下载 linux执行下面的命令下载包 wget https://github.com/alibaba/Sentinel/releases/download/1.8.6/sentinel-dashboard-1.8.6.jar2.启动 nohup java -Dserver.port9090 -Dcsp.sentinel.dashboard.serverlocalhost:9090 -Dproject.namesentinel-dashboard -jar sentin…...
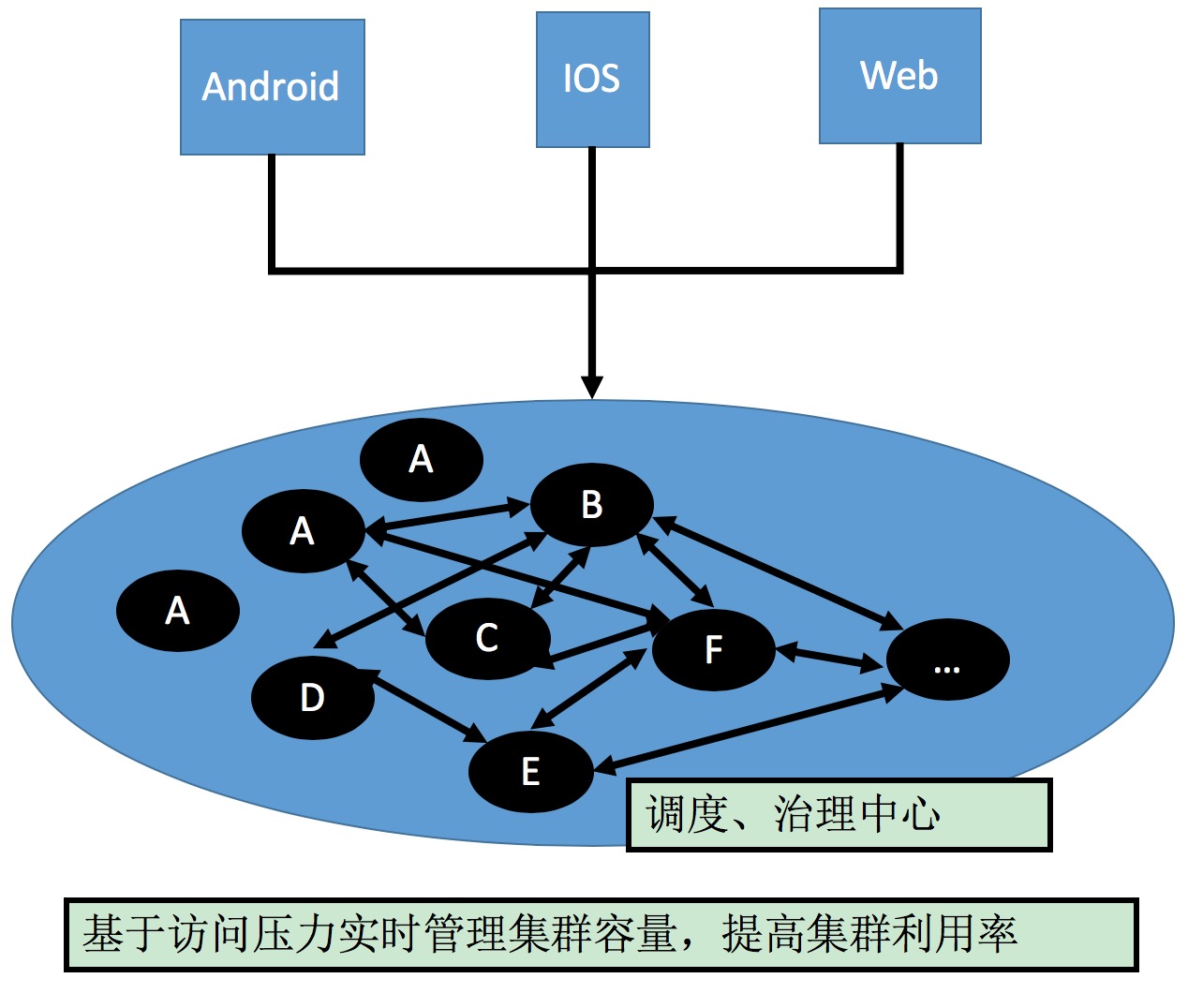
微服务发展历程
服务架构演进 服务架构演进过程:抽取各个模块独立维护,独立部署的过程。 初创公司2 ~ 3个研发人员,ALL IN ONE 的框架开发效率最高。随着队伍的壮大,产品,用户,商品成立独立小组,拆出相应的模块…...
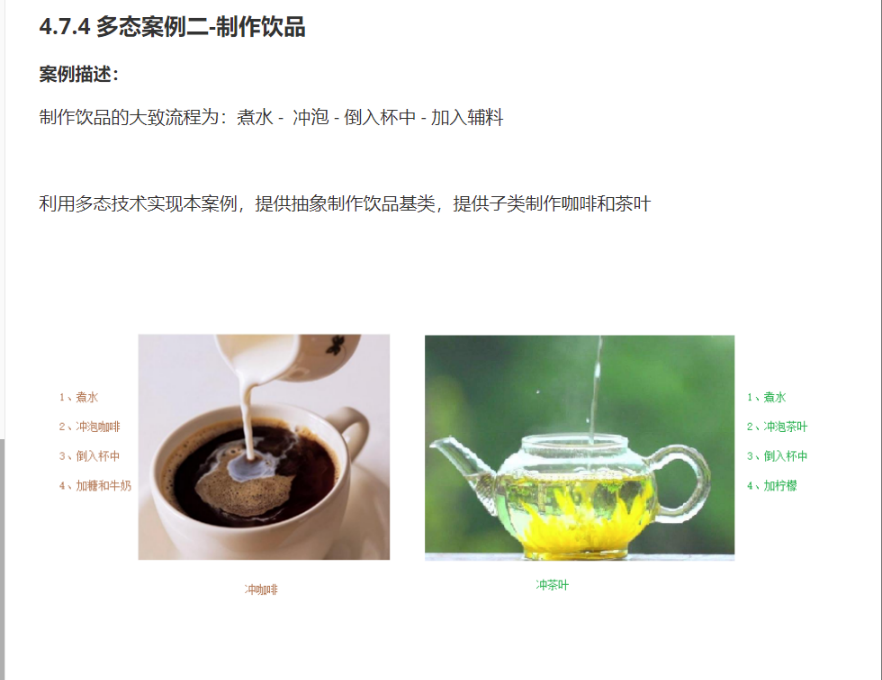
C++_核心编程_多态案例二-制作饮品
#include <iostream> #include <string> using namespace std;/*制作饮品的大致流程为:煮水 - 冲泡 - 倒入杯中 - 加入辅料 利用多态技术实现本案例,提供抽象制作饮品基类,提供子类制作咖啡和茶叶*//*基类*/ class AbstractDr…...
MySQL 隔离级别:脏读、幻读及不可重复读的原理与示例
一、MySQL 隔离级别 MySQL 提供了四种隔离级别,用于控制事务之间的并发访问以及数据的可见性,不同隔离级别对脏读、幻读、不可重复读这几种并发数据问题有着不同的处理方式,具体如下: 隔离级别脏读不可重复读幻读性能特点及锁机制读未提交(READ UNCOMMITTED)允许出现允许…...
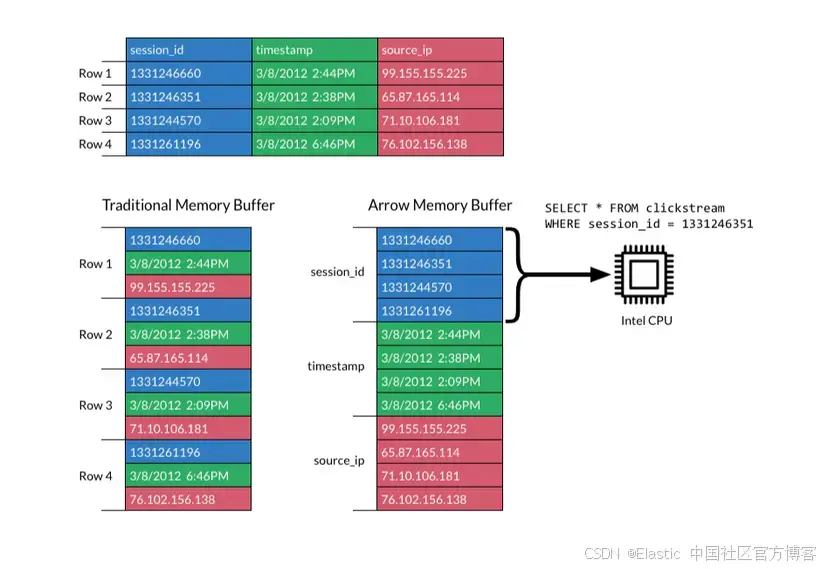
JavaScript 中的 ES|QL:利用 Apache Arrow 工具
作者:来自 Elastic Jeffrey Rengifo 学习如何将 ES|QL 与 JavaScript 的 Apache Arrow 客户端工具一起使用。 想获得 Elastic 认证吗?了解下一期 Elasticsearch Engineer 培训的时间吧! Elasticsearch 拥有众多新功能,助你为自己…...
【位运算】消失的两个数字(hard)
消失的两个数字(hard) 题⽬描述:解法(位运算):Java 算法代码:更简便代码 题⽬链接:⾯试题 17.19. 消失的两个数字 题⽬描述: 给定⼀个数组,包含从 1 到 N 所有…...
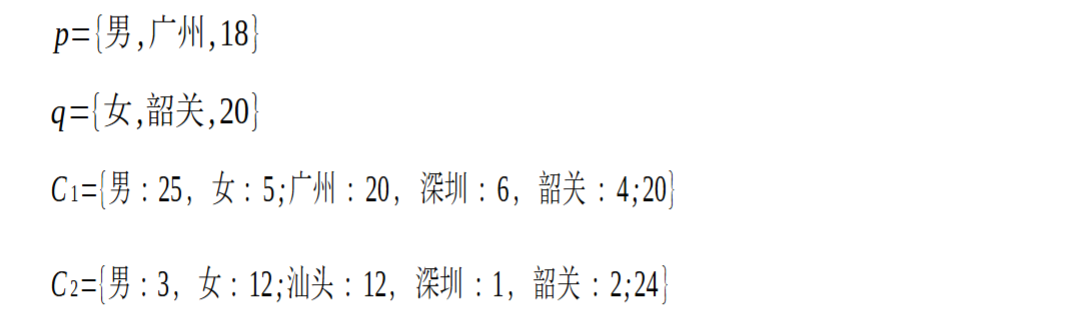
SCAU期末笔记 - 数据分析与数据挖掘题库解析
这门怎么题库答案不全啊日 来简单学一下子来 一、选择题(可多选) 将原始数据进行集成、变换、维度规约、数值规约是在以下哪个步骤的任务?(C) A. 频繁模式挖掘 B.分类和预测 C.数据预处理 D.数据流挖掘 A. 频繁模式挖掘:专注于发现数据中…...
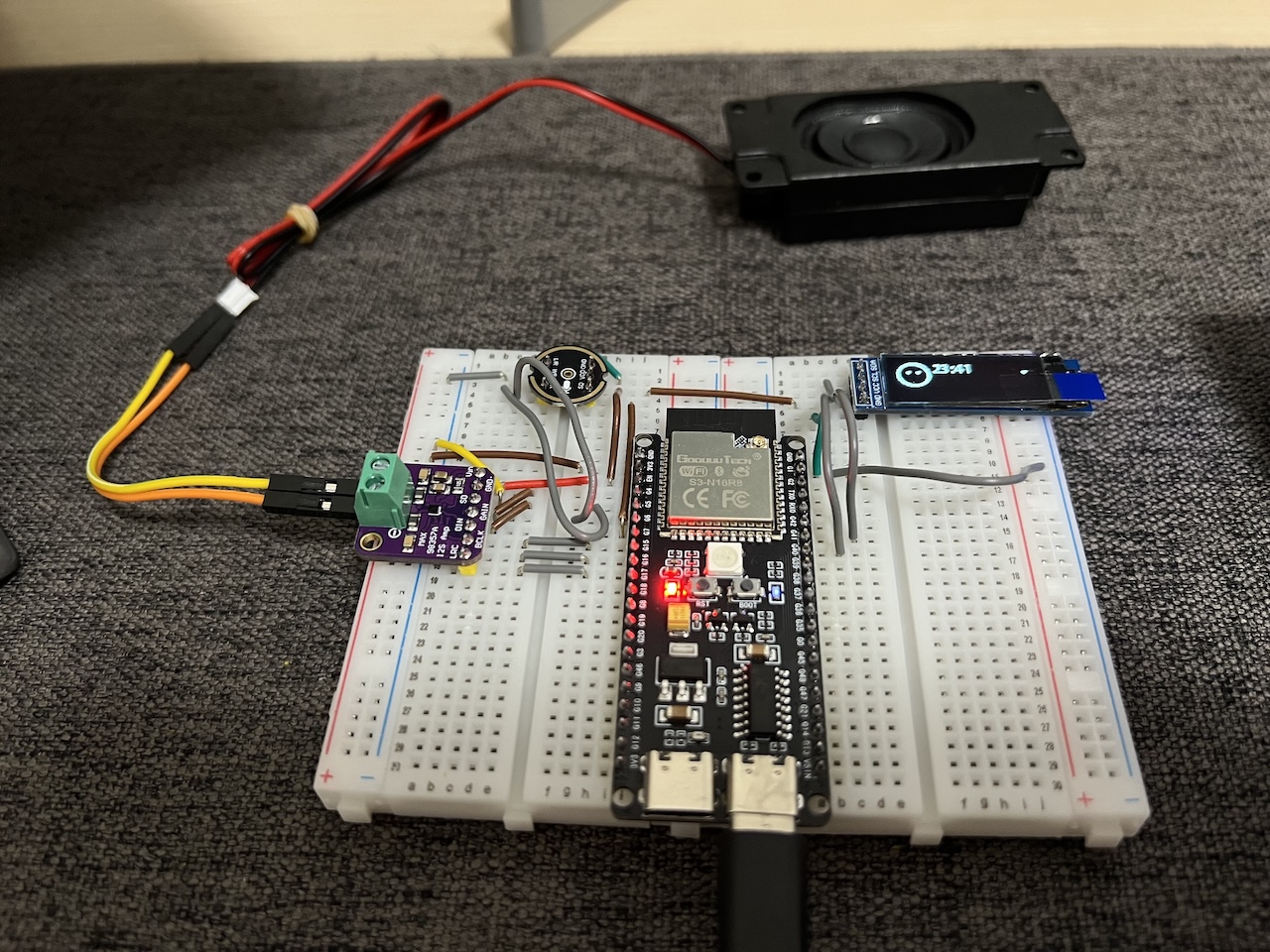
DIY|Mac 搭建 ESP-IDF 开发环境及编译小智 AI
前一阵子在百度 AI 开发者大会上,看到基于小智 AI DIY 玩具的演示,感觉有点意思,想着自己也来试试。 如果只是想烧录现成的固件,乐鑫官方除了提供了 Windows 版本的 Flash 下载工具 之外,还提供了基于网页版的 ESP LA…...
linux 下常用变更-8
1、删除普通用户 查询用户初始UID和GIDls -l /home/ ###家目录中查看UID cat /etc/group ###此文件查看GID删除用户1.编辑文件 /etc/passwd 找到对应的行,YW343:x:0:0::/home/YW343:/bin/bash 2.将标红的位置修改为用户对应初始UID和GID: YW3…...
CRMEB 框架中 PHP 上传扩展开发:涵盖本地上传及阿里云 OSS、腾讯云 COS、七牛云
目前已有本地上传、阿里云OSS上传、腾讯云COS上传、七牛云上传扩展 扩展入口文件 文件目录 crmeb\services\upload\Upload.php namespace crmeb\services\upload;use crmeb\basic\BaseManager; use think\facade\Config;/*** Class Upload* package crmeb\services\upload* …...
什么?连接服务器也能可视化显示界面?:基于X11 Forwarding + CentOS + MobaXterm实战指南
文章目录 什么是X11?环境准备实战步骤1️⃣ 服务器端配置(CentOS)2️⃣ 客户端配置(MobaXterm)3️⃣ 验证X11 Forwarding4️⃣ 运行自定义GUI程序(Python示例)5️⃣ 成功效果
mysql已经安装,但是通过rpm -q 没有找mysql相关的已安装包
文章目录 现象:mysql已经安装,但是通过rpm -q 没有找mysql相关的已安装包遇到 rpm 命令找不到已经安装的 MySQL 包时,可能是因为以下几个原因:1.MySQL 不是通过 RPM 包安装的2.RPM 数据库损坏3.使用了不同的包名或路径4.使用其他包…...