=computed() =ref()
=computed() =ref()
在 Vue 中,computed()
和 ref()
是 Vue 3 组合式 API 的核心工具,它们分别用于 计算属性 和 响应式数据。以下是它们的区别和用法:
1. ref()
作用
- 用于创建响应式的单一数据。
- 可以是基本类型(如字符串、数字、布尔值)或对象类型。
- 当
ref
的值发生变化时,依赖它的组件会自动重新渲染。
用法
import { ref } from 'vue';const count = ref(0); // 创建一个响应式变量// 修改值
count.value++; // 必须通过 .value 修改或访问 ref 的值console.log(count.value); // 输出: 1
特点
- 基本类型:
ref
会将值包装为一个响应式对象,访问或修改时需要通过.value
。 - 对象类型:如果
ref
包裹的是对象,Vue 会自动将对象的属性变为响应式。
示例
import { ref } from 'vue';const user = ref({ name: 'Alice', age: 25 }); // 包裹一个对象// 修改对象属性
user.value.name = 'Bob';
console.log(user.value.name); // 输出: Bob
2. computed()
作用
- 用于定义基于其他响应式数据的 派生状态。
computed
的值会根据依赖的响应式数据自动更新。- 适合用来处理需要动态计算的值。
用法
import { ref, computed } from 'vue';const count = ref(0);// 定义一个计算属性
const doubleCount = computed(() => count.value * 2);console.log(doubleCount.value); // 输出: 0count.value++; // 修改 count 的值
console.log(doubleCount.value); // 输出: 2
特点
computed
的值是只读的,不能直接修改。- 如果需要可写的计算属性,可以传入
get
和set
方法。
可写计算属性
import { ref, computed } from 'vue';const count = ref(0);// 可写计算属性
const doubleCount = computed({get: () => count.value * 2,set: (newValue) => {count.value = newValue / 2; // 反向修改 count}
});doubleCount.value = 10; // 修改 doubleCount
console.log(count.value); // 输出: 5
3. ref()
和 computed()
的区别
特性 | ref() | computed() |
---|---|---|
用途 | 定义响应式的单一数据 | 定义基于其他响应式数据的派生状态 |
是否需要 .value | 是 | 是 |
是否可写 | 是 | 默认不可写(可通过 get 和 set 实现) |
依赖性 | 独立存在,不依赖其他响应式数据 | 依赖其他响应式数据 |
性能 | 直接存储值,简单高效 | 有缓存机制,只有依赖数据变化时才重新计算 |
4. 综合示例
以下是一个同时使用 ref()
和 computed()
的示例:
<script setup>
import { ref, computed } from 'vue';// 定义响应式数据
const price = ref(100);
const quantity = ref(2);// 定义计算属性
const total = computed(() => price.value * quantity.value);// 修改响应式数据
price.value = 150;console.log(total.value); // 输出: 300
</script><template><div><p>单价: {{ price }}</p><p>数量: {{ quantity }}</p><p>总价: {{ total }}</p></div>
</template>
5. 在 Options API 中的等价用法
在 Vue 3 的 Options API 中,ref()
和 computed()
的功能可以通过 data
和 computed
选项实现:
等价代码
<script>
export default {data() {return {price: 100, // 等价于 ref(100)quantity: 2 // 等价于 ref(2)};},computed: {total() {return this.price * this.quantity; // 等价于 computed(() => price.value * quantity.value)}}
};
</script><template><div><p>单价: {{ price }}</p><p>数量: {{ quantity }}</p><p>总价: {{ total }}</p></div>
</template>
6. 总结
ref()
:用于定义响应式的单一数据,适合存储基本类型或对象。computed()
:用于定义基于其他响应式数据的派生状态,具有缓存机制。- 组合使用:
ref()
定义基础数据,computed()
定义基于基础数据的动态值。
case 2
<script setup>
import { ref, computed } from 'vue'; // 原始数据
const data = ref([ { position: { x: 1, y: 2 } }, { position: { x: 3, y: 4 } }, { position: { x: 5, y: 6 } }
]); // 数据转换函数
const convertData = (sourceData) => { try { const paths = sourceData .filter(item => item?.position && typeof item.position === 'object') .map(item => item.position); return [{ paths: paths }]; } catch (error) { console.error('数据转换错误:', error); return [{ paths: [] }]; }
}; // 计算属性
const convertTodata = computed(() => convertData(data.value)); // 更新数据的方法
const updateData = (newData) => { data.value = newData;
};
</script> <template> <div class="p-4"> <h2 class="text-xl mb-4">转换后的数据:</h2> <pre class="bg-gray-100 p-4 rounded"> {{ JSON.stringify(convertTodata, null, 2) }} // 使用 JSON.stringify 将 convertTodata 的结果格式化为 JSON 字符串并显示在页面</pre> </div>
</template>
<script>
export default { name: 'DataConverter', // ref() -> data() data() { return { // const data = ref([...]) 变成: data: [ { position: { x: 1, y: 2 } }, { position: { x: 3, y: 4 } }, { position: { x: 5, y: 6 } } ] } }, // computed() -> computed: {} computed: { // const convertTodata = computed(() => ...) 变成: convertTodata() { return this.convertData(this.data); } }, methods: { convertData(sourceData) { try { const paths = sourceData .filter(item => item?.position && typeof item.position === 'object') .map(item => item.position); return [{ paths: paths }]; } catch (error) { console.error('数据转换错误:', error); return [{ paths: [] }]; } }, // const updateData = (newData) => { data.value = newData } 变成: updateData(newData) { this.data = newData; } }
};
</script> <template> <div class="p-4"> <h2 class="text-xl mb-4">转换后的数据:</h2> <pre class="bg-gray-100 p-4 rounded"> {{ JSON.stringify(convertTodata, null, 2) }} </pre> </div>
</template> <style scoped>
.p-4 { padding: 1rem;
} .text-xl { font-size: 1.25rem; line-height: 1.75rem;
} .mb-4 { margin-bottom: 1rem;
} .bg-gray-100 { background-color: #f3f4f6;
} .rounded { border-radius: 0.25rem;
}
</style>
主要转换规则
-
ref() 转换:
// Composition API const data = ref([...]) // Options API data() { return { data: [...] } }
-
computed() 转换:
// Composition API const result = computed(() => {...}) // Options API computed: { result() { return {...} } }
-
方法转换:
// Composition API const updateData = (newData) => { data.value = newData } // Options API methods: { updateData(newData) { this.data = newData } }
-
数据访问:
// Composition API data.value // Options API this.data
=computed() 能改为 =ref() 吗
在 Vue 3 中,computed()
和 ref()
是不同的工具,虽然它们都可以创建响应式数据,但它们的用途和行为不同。computed()
不建议直接改为 ref()
,因为它们的核心区别在于:
ref()
是用于存储和管理响应式的单一数据。computed()
是用于基于其他响应式数据派生出新的值,并且具有 缓存机制。
如果你想用 ref()
替代 computed()
,需要手动实现类似 computed()
的功能,但这通常不推荐,因为 computed()
已经为你处理了依赖追踪和缓存。=ref()
会丢失 computed()
的依赖追踪和缓存特性。
什么时候可以用 ref()
替代 computed()
?
如果你的计算逻辑非常简单,且不需要依赖缓存机制,可以用 ref()
和 watchEffect()
或 watch()
来实现类似的功能。
如何用 ref()
替代 computed()
?
以下是一个示例,展示如何用 ref()
和 watchEffect()
替代 computed()
:
原始代码:使用 computed()
import { ref, computed } from 'vue';const count = ref(0);
const doubleCount = computed(() => count.value * 2);console.log(doubleCount.value); // 输出: 0count.value++;
console.log(doubleCount.value); // 输出: 2
改为使用 ref()
和 watchEffect()
import { ref, watchEffect } from 'vue';const count = ref(0);
const doubleCount = ref(0);// 使用 watchEffect 手动更新 doubleCount
watchEffect(() => {doubleCount.value = count.value * 2;
});console.log(doubleCount.value); // 输出: 0count.value++;
console.log(doubleCount.value); // 输出: 2
对比分析
特性 | computed() | ref() + watchEffect() | watch |
---|---|---|---|
依赖追踪 | 自动追踪依赖 | 否, 使用 watchEffect 手动更新值 | 否, 使用 watch 手动更新值 |
缓存机制 | 有缓存,每次使用时使用的预先计算存储的的 cache,依赖变化时重新计算, 使用时使用的重新计算存储的的 new cache | 没有缓存,每次使用时重新计算,依赖变化时重新计算 | 否, 每次 data(){} 变化都会重新执行 |
代码复杂度 | 简洁,直接定义计算逻辑 | 需要手动更新值,代码稍显冗长 | |
适用场景 | 适合派生状态,依赖多个响应式数据 | 适合简单逻辑或不需要缓存的场景 |
完整示例:从 computed()
改为 ref()
假设你有一个组件,使用 computed()
来计算数据:
原始代码:使用 computed()
<script setup>
import { ref, computed } from 'vue';const price = ref(100);
const quantity = ref(2);// 计算总价
const total = computed(() => price.value * quantity.value);
</script><template><div><p>单价: {{ price }}</p><p>数量: {{ quantity }}</p><p>总价: {{ total }}</p></div>
</template>
改为使用 ref()
和 watchEffect()
<script setup>
import { ref, watchEffect } from 'vue';const price = ref(100);
const quantity = ref(2);// 使用 ref 存储总价
const total = ref(0);// 手动更新 total 的值
watchEffect(() => {total.value = price.value * quantity.value;
});
</script><template><div><p>单价: {{ price }}</p><p>数量: {{ quantity }}</p><p>总价: {{ total }}</p></div>
</template>
原始代码:使用 computed()
<script>
export default { name: 'DataConverter', // ref() -> data() data() { return { // const data = ref([...]) 变成: data: [ { position: { x: 1, y: 2 } }, { position: { x: 3, y: 4 } }, { position: { x: 5, y: 6 } } ] } }, // computed() -> computed: {} computed: { // const convertTodata = computed(() => ...) 变成: convertTodata() { return this.convertData(this.data); } }, methods: { convertData(sourceData) { try { const paths = sourceData .filter(item => item?.position && typeof item.position === 'object') .map(item => item.position); return [{ paths: paths }]; } catch (error) { console.error('数据转换错误:', error); return [{ paths: [] }]; } }, // const updateData = (newData) => { data.value = newData } 变成: updateData(newData) { this.data = newData; } }
};
</script> <template> <div class="p-4"> <h2 class="text-xl mb-4">转换后的数据:</h2> <pre class="bg-gray-100 p-4 rounded"> {{ JSON.stringify(convertTodata, null, 2) }} </pre> </div>
</template>
改为使用 ref()
和 watchEffect()
<template> <div class="p-4"> <h2 class="text-xl mb-4">转换后的数据:</h2> <pre class="bg-gray-100 p-4 rounded"> {{ JSON.stringify(convertTodata, null, 2) }} </pre> </div>
</template> <script>
export default { data() { return { // 原始数据 data1: [ { position: { x: 1, y: 2 } }, { position: { x: 3, y: 4 } }, { position: { x: 5, y: 6 } } ],irrelevantData: 'something', // 无关数据 // 将 computed 改为 data 中的属性 // 移除了 computed 属性:原来的计算属性被转换为 data 中的普通响应式数据// 无自动追踪依赖,需watch 无缓存机制 不是只有依赖变化时( data1.value 变化时)才重新计算,每次 data ( 指总的 data(){},例如 irrelevantData change ) 变化都会执行convertTodata: [{ paths: [] }],} }, // 监听 data ( 指总的 data(){},例如 irrelevantData change )的变化,更新 convertTodata watch: { data: { // 指总的 data(){}immediate: true, // 确保初始化时执行一次,立即执行一次 handler(newData) { // 当 data 变化时更新 convertTodata this.convertTodata = this.convertData(newData); } } }, methods: { convertData(sourceData) { try { const paths = sourceData .filter(item => item?.position && typeof item.position === 'object') .map(item => item.position); return [{ paths: paths }]; } catch (error) { console.error('数据转换错误:', error); return [{ paths: [] }]; } }, updateData(newData) { this.data = newData; } }
}
</script>
注意事项
-
性能问题:
computed()
有缓存机制,只有依赖的数据发生变化时才会重新计算。ref()
+watchEffect()
每次依赖变化都会重新执行逻辑,可能会带来性能开销。
-
代码简洁性:
computed()
更加简洁,适合大多数场景。ref()
+watchEffect()
需要手动更新值,代码稍显冗长。
-
推荐使用场景:
- 如果你的逻辑依赖多个响应式数据,并且需要缓存,优先使用
computed()
。 - 如果你的逻辑非常简单,或者不需要缓存,可以使用
ref()
+watchEffect()
。
- 如果你的逻辑依赖多个响应式数据,并且需要缓存,优先使用
总结
computed()
是首选:它更简洁、性能更好,适合大多数场景。ref()
替代computed()
:可以通过watchEffect()
手动实现,但代码复杂度会增加,且没有缓存机制。
参考:
=ref() =computed()
相关文章:
=computed() =ref()
computed() ref() 在 Vue 中,computed() 和 ref() 是 Vue 3 组合式 API 的核心工具,它们分别用于 计算属性 和 响应式数据。以下是它们的区别和用法: 1. ref() 作用 用于创建响应式的单一数据。可以是基本类型(如字符串、数字、…...
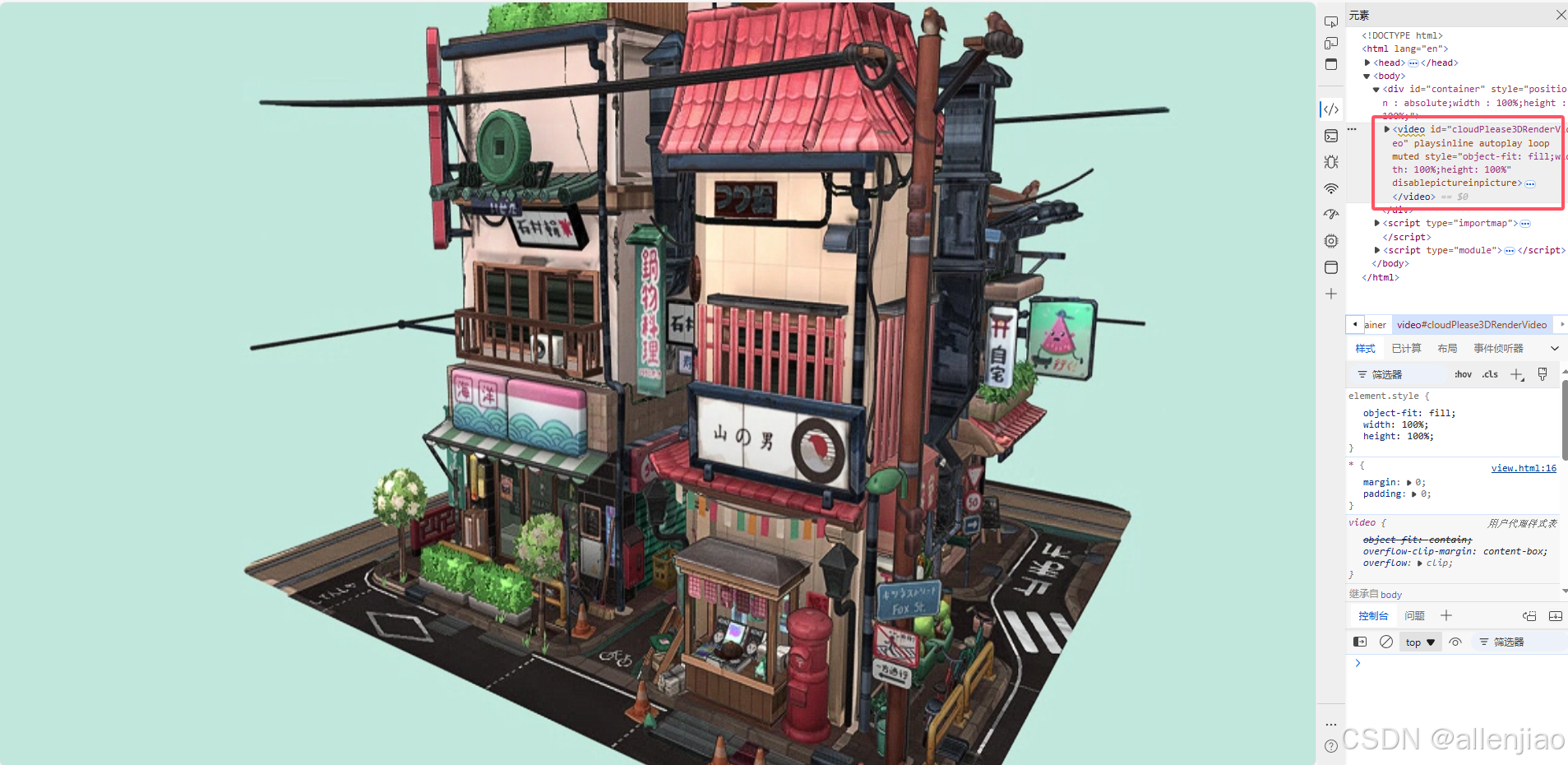
webgl threejs 云渲染(服务器渲染、后端渲染)解决方案
云渲染和流式传输共享三维模型场景 1、本地无需高端GPU设备即可提供三维项目渲染 云渲染和云流化媒体都可以让3D模型共享变得简单便捷。配备强大GPU的远程服务器早就可以处理密集的处理工作,而专有应用程序,用户也可以从任何个人设备查看全保真模型并与…...

【shell编程】函数、正则表达式、文本处理工具
函数 系统函数 常见内置命令 echo打印输出 #!/bin/bash # 输出普通文本 echo "Hello, World!"# 输出变量值 name"Alice" echo "Hello, $name"# 输出带有换行符的文本 echo -n "Hello, " # -n 选项不输出换行 echo "World!&quo…...
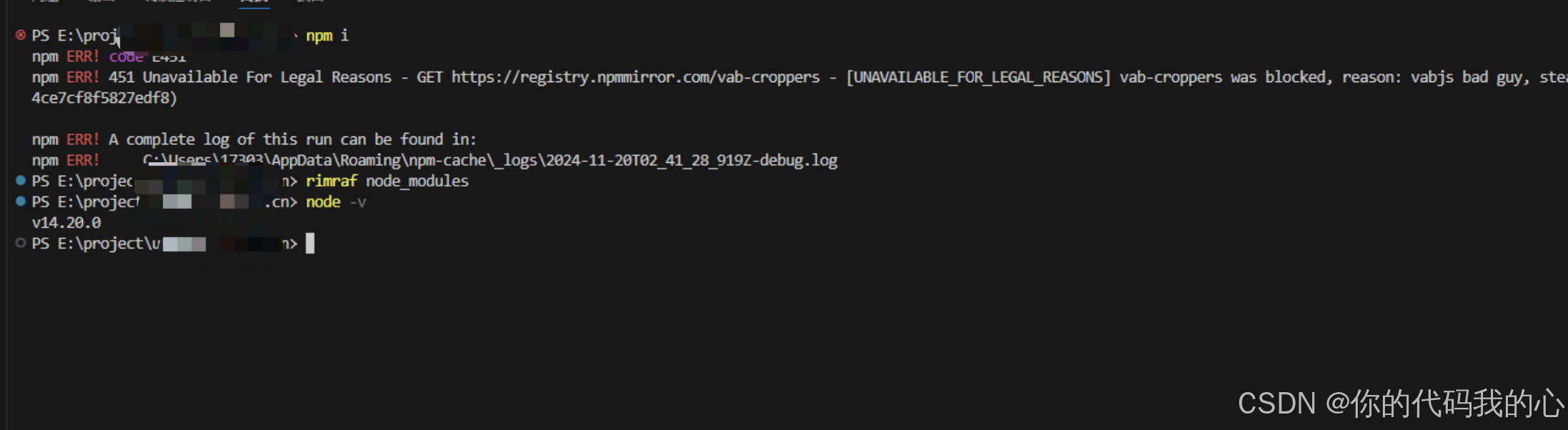
解决 npm xxx was blocked, reason: xx bad guy, steal env and delete files
问题复现 今天一位朋友说,vue2的老项目安装不老依赖,报错内容如下: npm install 451 Unavailable For Legal Reasons - GET https://registry.npmmirror.com/vab-count - [UNAVAILABLE_FOR_LEGAL_REASONS] vab-count was blocked, reas…...
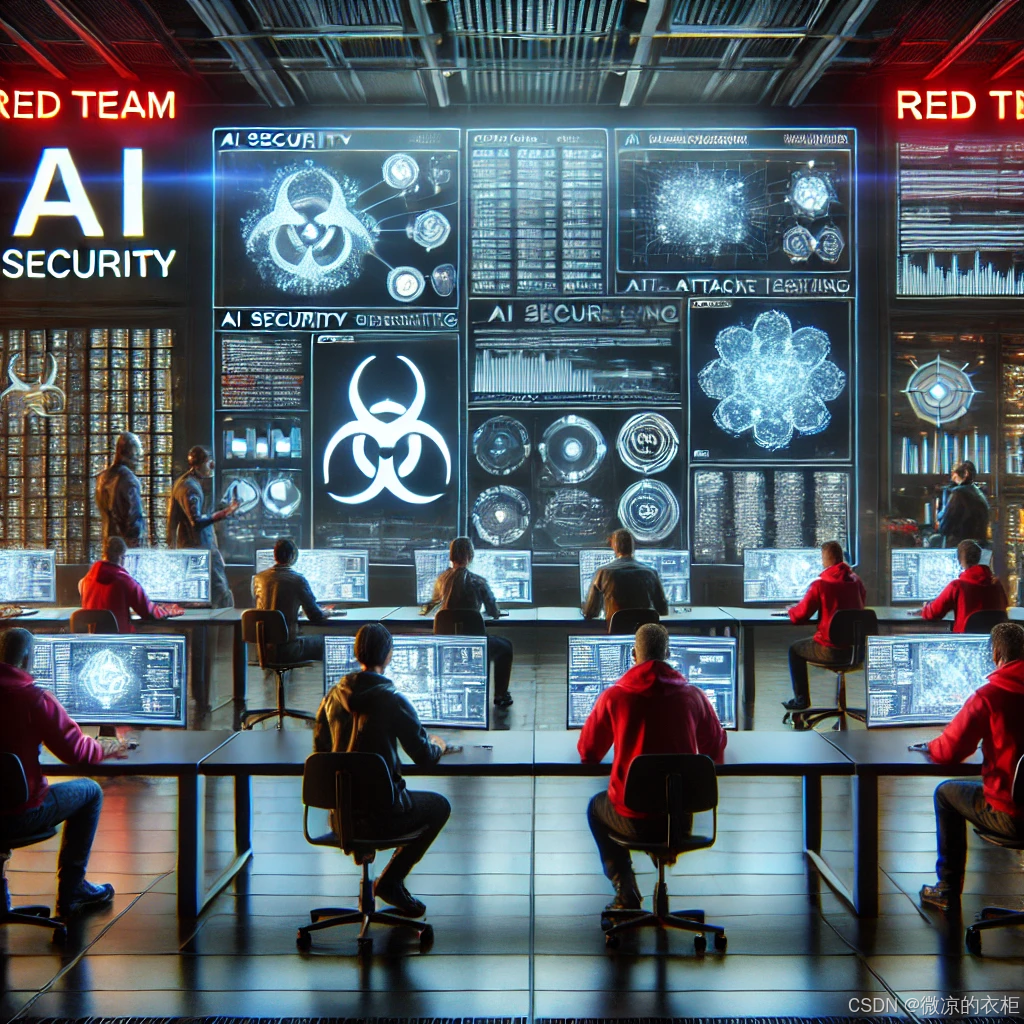
如何进行高级红队测试:OpenAI的实践与方法
随着人工智能(AI)技术的迅猛发展,AI模型的安全性和可靠性已经成为业界关注的核心问题之一。为了确保AI系统在实际应用中的安全性,红队测试作为一种有效的安全评估方法,得到了广泛应用。近日,OpenAI发布了两…...
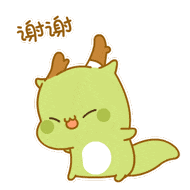
Java:二维数组
目录 1. 二维数组的基础格式 1.1 二维数组变量的创建 —— 3种形式 1.2 二维数组的初始化 \1 动态初始化 \2 静态初始化 2. 二维数组的大小 和 内存分配 3. 二维数组的不规则初始化 4. 遍历二维数组 4.1 for循环 编辑 4.2 for-each循环 5. 二维数组 与 方法 5.1…...
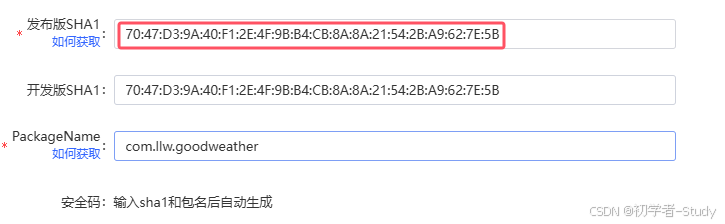
Android 天气APP(三十七)新版AS编译、更新镜像源、仓库源、修复部分BUG
上一篇:Android 天气APP(三十六)运行到本地AS、更新项目版本依赖、去掉ButterKnife 新版AS编译、更新镜像源、仓库源、修复部分BUG 前言正文一、更新镜像源① 腾讯源③ 阿里源 二、更新仓库源三、修复城市重名BUG四、地图加载问题五、源码 前…...
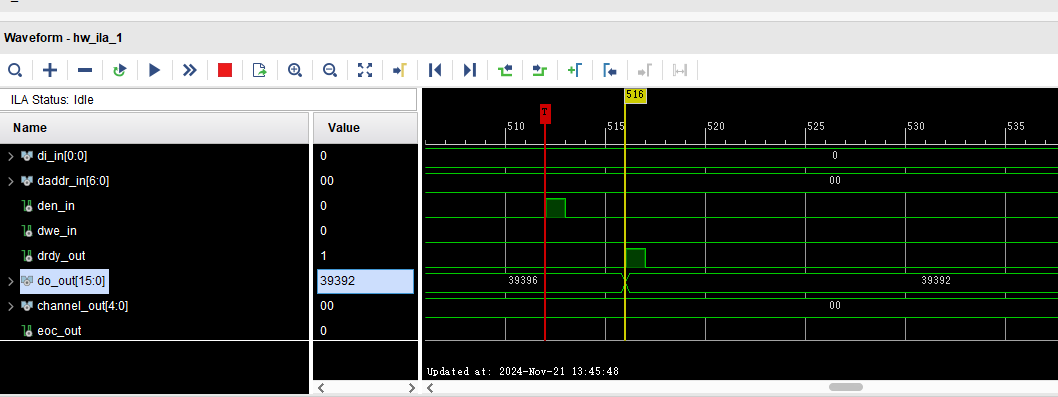
Xilinx IP核(3)XADC IP核
文章目录 1. XADC介绍2.输入要求3.输出4.XADC IP核使用5.传送门 1. XADC介绍 xadc在 所有的7系列器件上都有支持,通过将高质量模拟模块与可编程逻辑的灵活性相结合,可以为各种应用打造定制的模拟接口,XADC 包括双 12 位、每秒 1 兆样本 (MSP…...
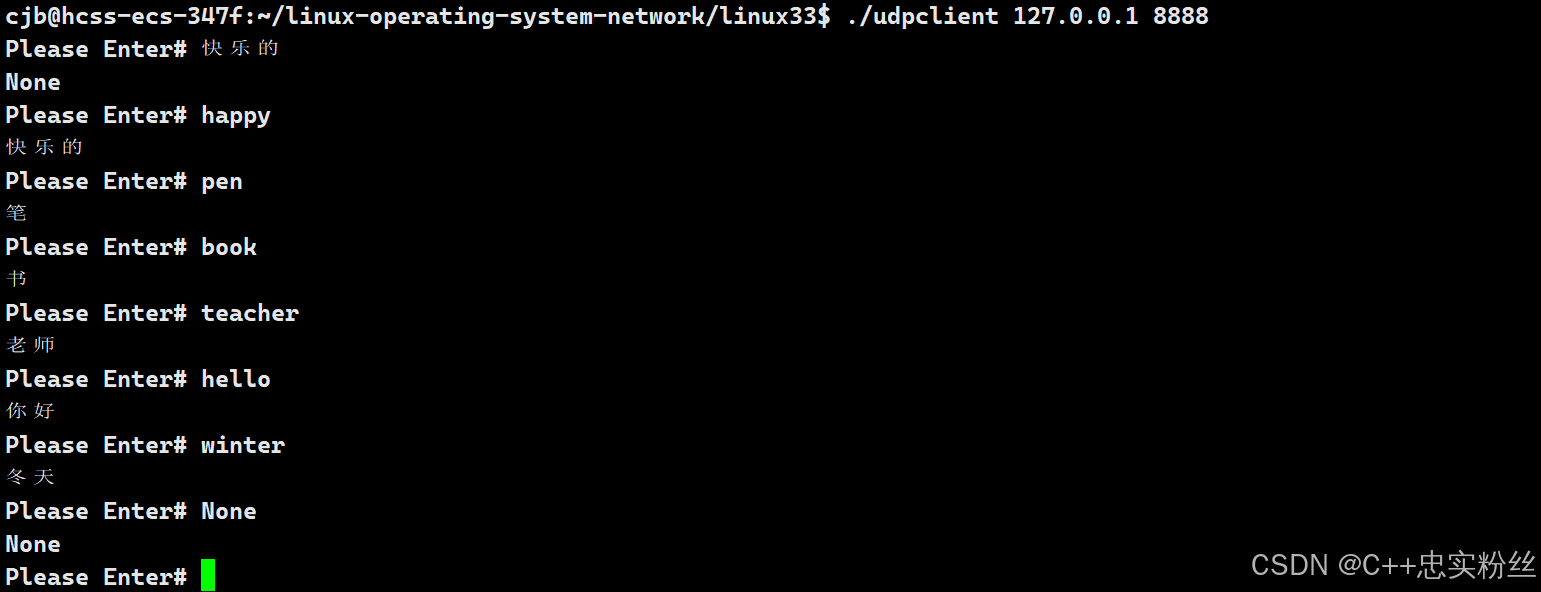
计算机网络socket编程(2)_UDP网络编程实现网络字典
个人主页:C忠实粉丝 欢迎 点赞👍 收藏✨ 留言✉ 加关注💓本文由 C忠实粉丝 原创 计算机网络socket编程(2)_UDP网络编程实现网络字典 收录于专栏【计算机网络】 本专栏旨在分享学习计算机网络的一点学习笔记,欢迎大家在评论区交流讨…...
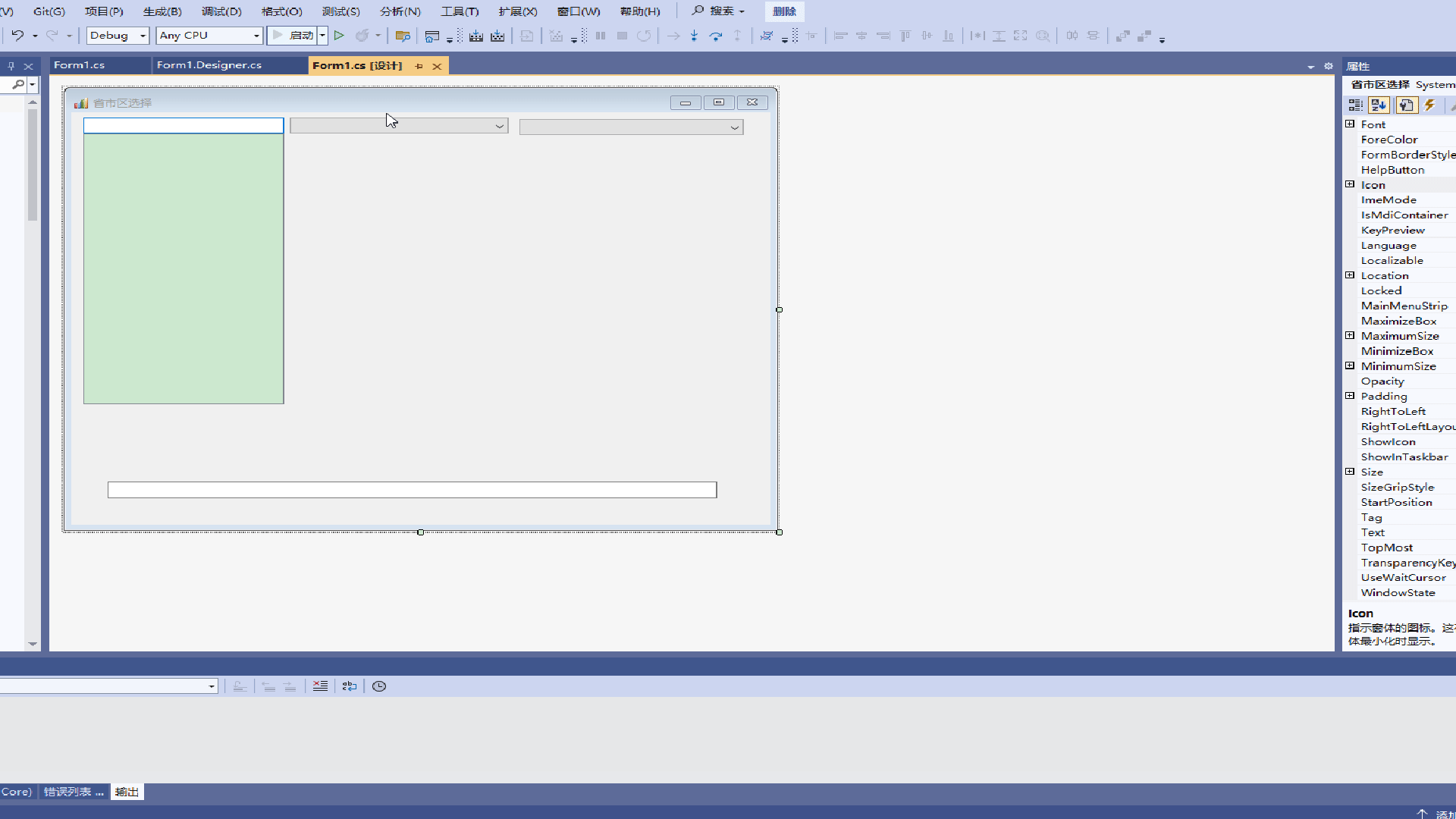
c#窗体列表框(combobox)应用——省市区列表选择实例
效果如下: designer.cs代码如下: using System.Collections.Generic;namespace 删除 {public partial class 省市区选择{private Dictionary<string, List<string>> provinceCityDictionary;private Dictionary<string,List<string&…...
Nginx 架构与设计
Nginx 是一个高性能的 HTTP 和反向代理服务器,同时也可以用作邮件代理和通用的 TCP/UDP 负载均衡器。它的架构设计以高并发、高可扩展性和高性能为目标,充分利用操作系统提供的多路复用机制和事件驱动模型。以下是 Nginx 的架构和设计特点: 1…...
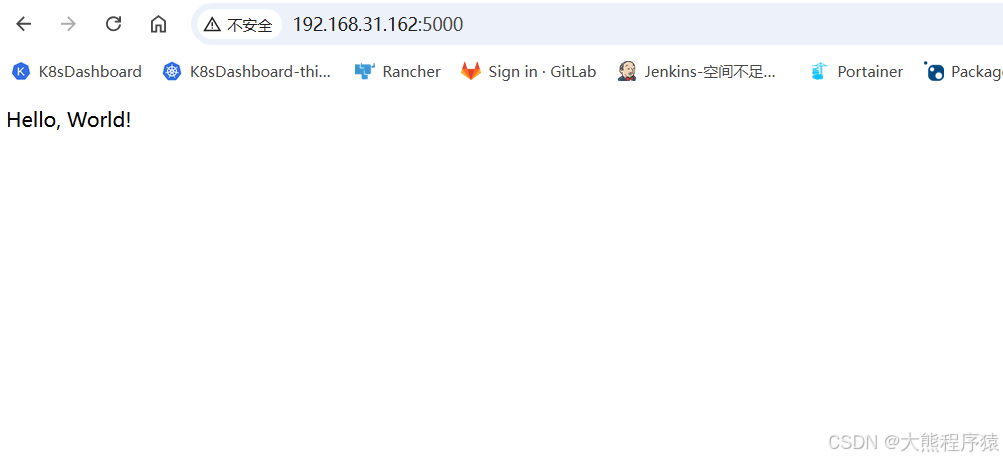
python Flask指定IP和端口
from flask import Flask, request import uuidimport json import osapp Flask(__name__)app.route(/) def hello_world():return Hello, World!if __name__ __main__:app.run(host0.0.0.0, port5000)...
多线程 相关面试集锦
什么是线程? 1、线程是操作系统能够进⾏运算调度的最⼩单位,它被包含在进程之中,是进程中的实际运作单位,可以使⽤多线程对 进⾏运算提速。 ⽐如,如果⼀个线程完成⼀个任务要100毫秒,那么⽤⼗个线程完成改…...
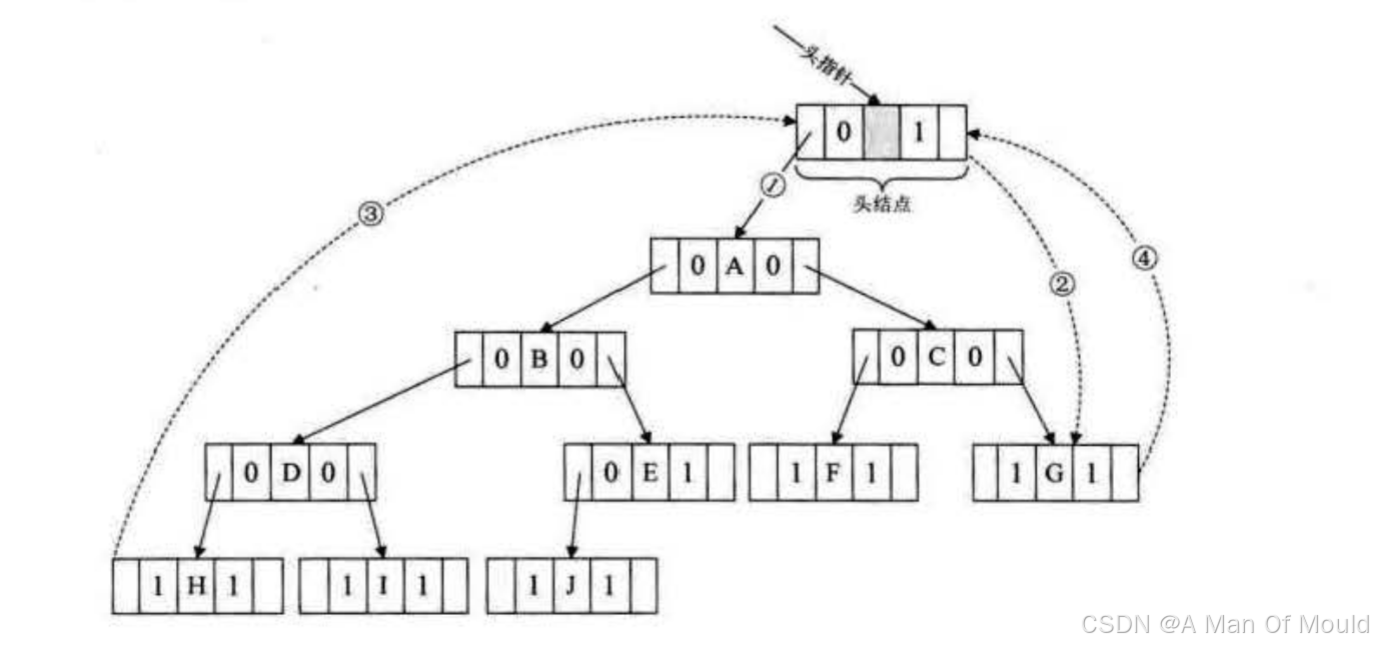
【数据结构】—— 线索二叉树
引入 我们现在提倡节约型杜会, 一切都应该节约为本。对待我们的程序当然也不例外,能不浪费的时间或空间,都应该考虑节省。我们再观察团下图的二叉树(链式存储结构),会发现指针域并不是都充分的利用了,有许…...

uni-app 发布媒介功能(自由选择媒介类型的内容) 设计
1.首先明确需求 我想做一个可以选择媒介的内容,来进行发布媒介的功能 (媒介包含:图片、文本、视频) 2.原型设计 发布-编辑界面 通过点击下方的加号,可以自由选择添加的媒介类型 但是因为预览中无法看到视频的效果&…...
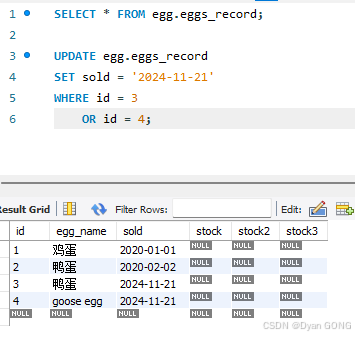
How to update the content of one column in Mysql
How to update the content of one column in Mysql by another column name? UPDATE egg.eggs_record SET sold 2024-11-21 WHERE id 3 OR id 4;UPDATE egg.eggs_record SET egg_name duck egg WHERE id 2;...
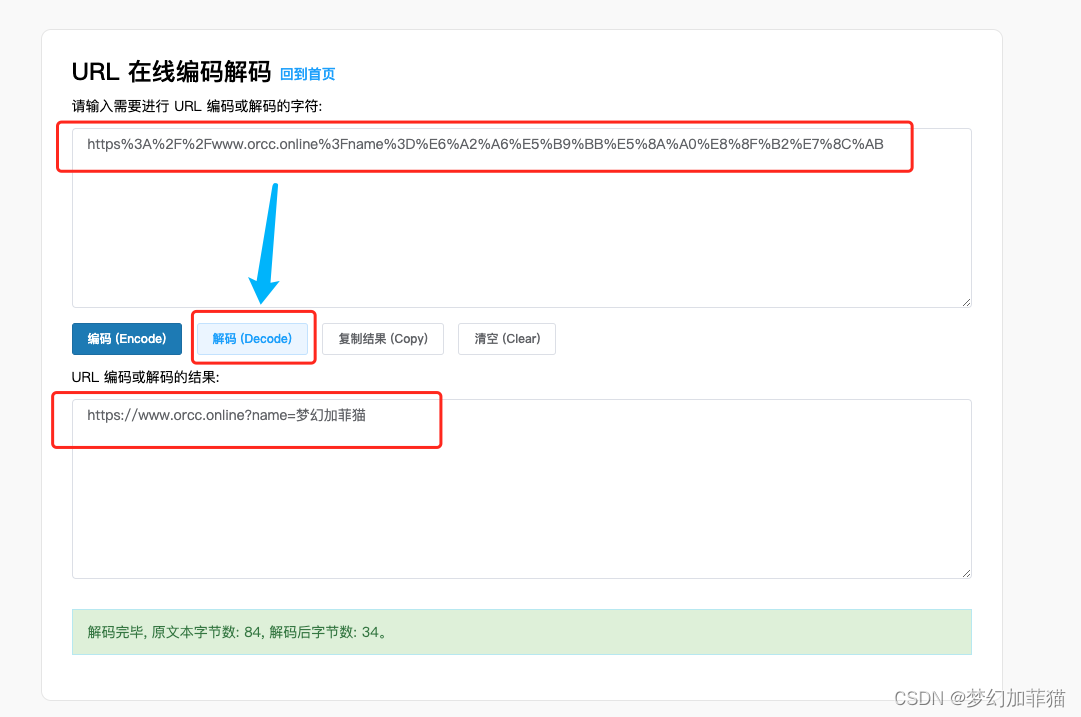
URL在线编码解码- 加菲工具
URL在线编码解码 打开网站 加菲工具 选择“URL编码解码” 输入需要编码/解码的内容,点击“编码”/“解码”按钮 编码: 解码: 复制已经编码/解码后的内容。...
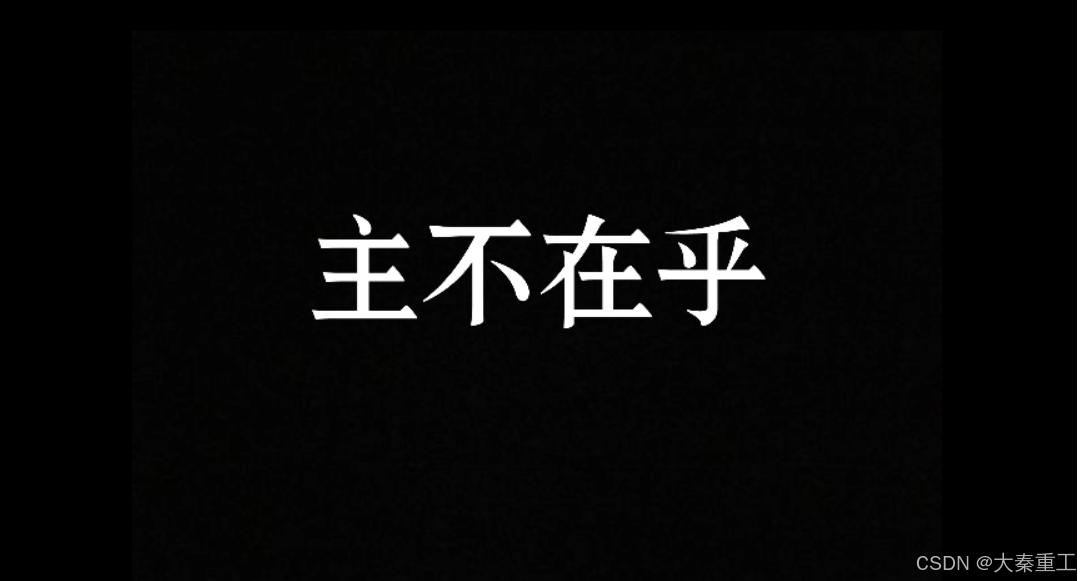
Python3 爬虫 Scrapy的安装
Scrapy是基于Python的分布式爬虫框架。使用它可以非常方便地实现分布式爬虫。Scrapy高度灵活,能够实现功能的自由拓展,让爬虫可以应对各种网站情况。同时,Scrapy封装了爬虫的很多实现细节,所以可以让开发者把更多的精力放在数据的…...

QT中QString类的各种使用
大部分的QString使用可以参考:QT中QString 类的使用--获取指定字符位置、截取子字符串等_qstring 取子串-CSDN博客 补充一种QString类的分离:Qt QString切割(Split()与Mid()函数详解)_qstring split-CSDN博客 1. Trimmed和Simplified函数(去除空白) trimmed:去除了…...
linux 网络安全不完全笔记
一、安装Centos 二、Linux网络网络环境设置 a.配置linux与客户机相连通 b.配置linux上网 三、Yum详解 yum 的基本操作 a.使用 yum 安装新软件 yum install –y Software b.使用 yum 更新软件 yum update –y Software c.使用 yum 移除软件 yum remove –y Software d.使用 yum …...
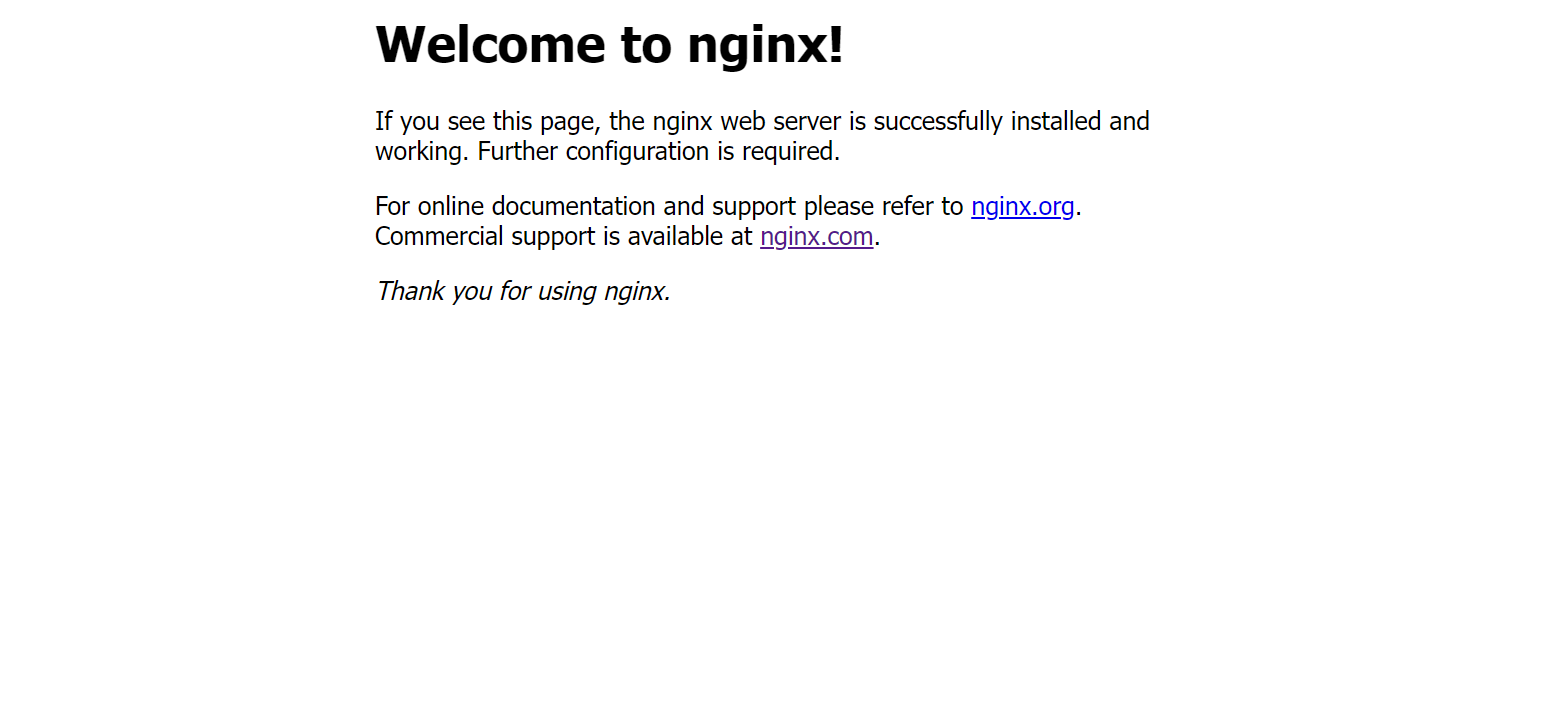
linux之kylin系统nginx的安装
一、nginx的作用 1.可做高性能的web服务器 直接处理静态资源(HTML/CSS/图片等),响应速度远超传统服务器类似apache支持高并发连接 2.反向代理服务器 隐藏后端服务器IP地址,提高安全性 3.负载均衡服务器 支持多种策略分发流量…...
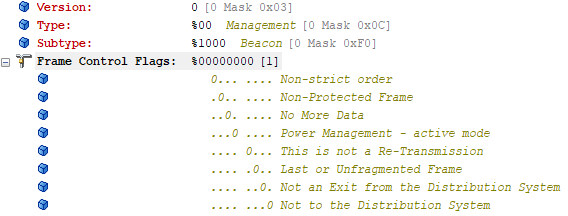
【WiFi帧结构】
文章目录 帧结构MAC头部管理帧 帧结构 Wi-Fi的帧分为三部分组成:MAC头部frame bodyFCS,其中MAC是固定格式的,frame body是可变长度。 MAC头部有frame control,duration,address1,address2,addre…...

中南大学无人机智能体的全面评估!BEDI:用于评估无人机上具身智能体的综合性基准测试
作者:Mingning Guo, Mengwei Wu, Jiarun He, Shaoxian Li, Haifeng Li, Chao Tao单位:中南大学地球科学与信息物理学院论文标题:BEDI: A Comprehensive Benchmark for Evaluating Embodied Agents on UAVs论文链接:https://arxiv.…...
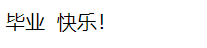
HTML 列表、表格、表单
1 列表标签 作用:布局内容排列整齐的区域 列表分类:无序列表、有序列表、定义列表。 例如: 1.1 无序列表 标签:ul 嵌套 li,ul是无序列表,li是列表条目。 注意事项: ul 标签里面只能包裹 li…...
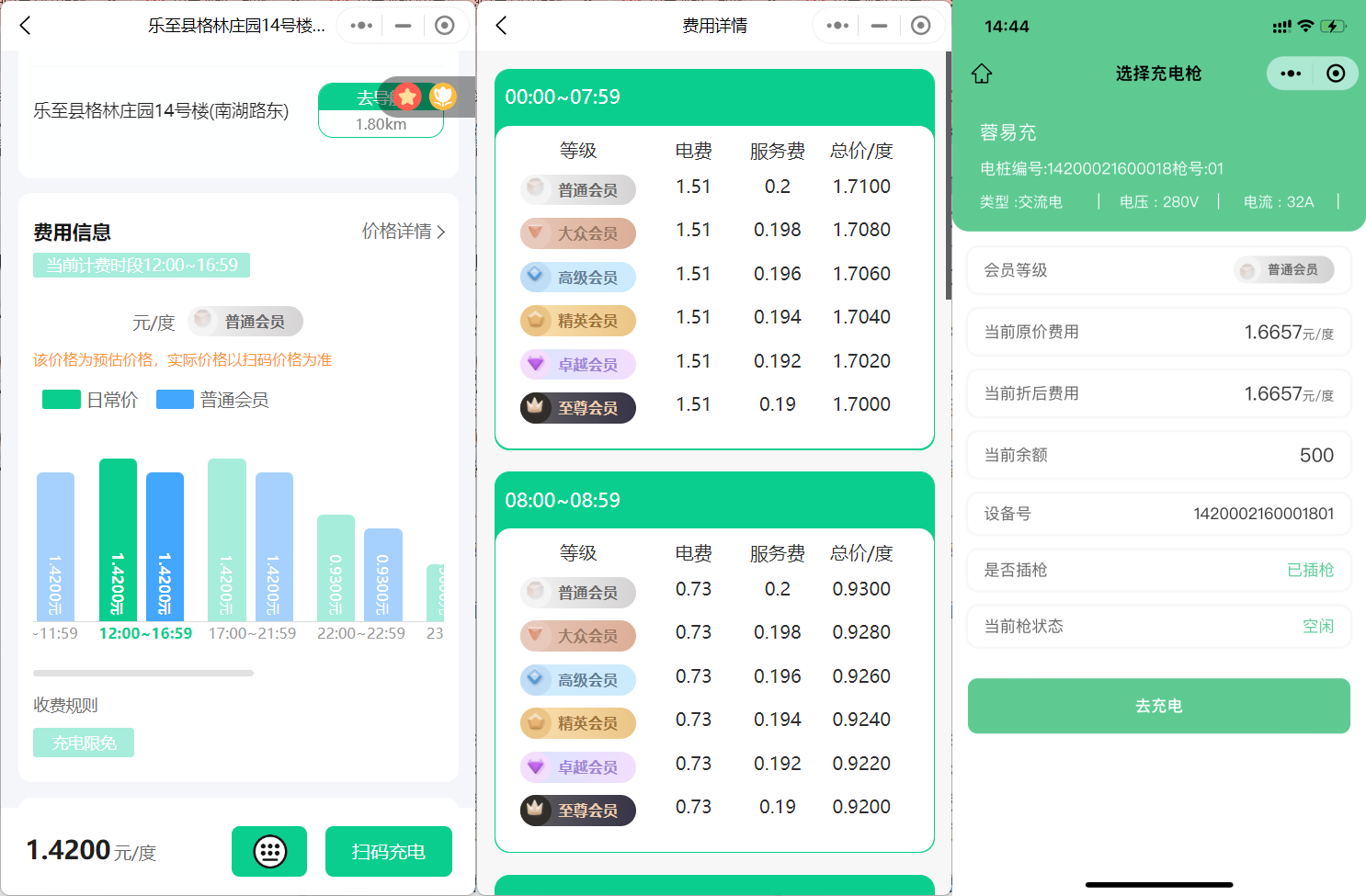
新能源汽车智慧充电桩管理方案:新能源充电桩散热问题及消防安全监管方案
随着新能源汽车的快速普及,充电桩作为核心配套设施,其安全性与可靠性备受关注。然而,在高温、高负荷运行环境下,充电桩的散热问题与消防安全隐患日益凸显,成为制约行业发展的关键瓶颈。 如何通过智慧化管理手段优化散…...
css的定位(position)详解:相对定位 绝对定位 固定定位
在 CSS 中,元素的定位通过 position 属性控制,共有 5 种定位模式:static(静态定位)、relative(相对定位)、absolute(绝对定位)、fixed(固定定位)和…...
CMake控制VS2022项目文件分组
我们可以通过 CMake 控制源文件的组织结构,使它们在 VS 解决方案资源管理器中以“组”(Filter)的形式进行分类展示。 🎯 目标 通过 CMake 脚本将 .cpp、.h 等源文件分组显示在 Visual Studio 2022 的解决方案资源管理器中。 ✅ 支持的方法汇总(共4种) 方法描述是否推荐…...
在web-view 加载的本地及远程HTML中调用uniapp的API及网页和vue页面是如何通讯的?
uni-app 中 Web-view 与 Vue 页面的通讯机制详解 一、Web-view 简介 Web-view 是 uni-app 提供的一个重要组件,用于在原生应用中加载 HTML 页面: 支持加载本地 HTML 文件支持加载远程 HTML 页面实现 Web 与原生的双向通讯可用于嵌入第三方网页或 H5 应…...
Webpack性能优化:构建速度与体积优化策略
一、构建速度优化 1、升级Webpack和Node.js 优化效果:Webpack 4比Webpack 3构建时间降低60%-98%。原因: V8引擎优化(for of替代forEach、Map/Set替代Object)。默认使用更快的md4哈希算法。AST直接从Loa…...
Kubernetes 网络模型深度解析:Pod IP 与 Service 的负载均衡机制,Service到底是什么?
Pod IP 的本质与特性 Pod IP 的定位 纯端点地址:Pod IP 是分配给 Pod 网络命名空间的真实 IP 地址(如 10.244.1.2)无特殊名称:在 Kubernetes 中,它通常被称为 “Pod IP” 或 “容器 IP”生命周期:与 Pod …...