Unity阿里云OpenAPI 获取 Token的C#【记录】
获取Token
using UnityEngine;
using System;
using System.Text;
using System.Linq;
using Newtonsoft.Json.Linq;
using System.Security.Cryptography;
using UnityEngine.Networking;
using System.Collections.Generic;
using System.Globalization;
using Cysharp.Threading.Tasks;#if UNITY_EDITOR
using UnityEditor;
#endif/// <summary>
/// 获取阿里云的 Token 的代码
/// </summary>
public class AliTTSCtrl : MonoBehaviour
{private readonly string accessKeyId = "********"; private readonly string accessKeySecret = "********"; private readonly string accessKey = "********";private readonly string account = "********";private readonly string regionId = "cn-shanghai";private readonly string version = "2019-02-28";private readonly string action = "CreateToken";private readonly string formatType = "JSON";private readonly string signatureMethod = "HMAC-SHA1";private readonly string signatureVersion = "1.0";private DateTime expirationTime = DateTime.MinValue;void Start(){}// 获取当前有效的 Token[ContextMenu("获取 Token")]
#if UNITY_EDITOR[ExecuteInEditMode]
#endifpublic async UniTask<string> GetToken(){try {var res = CheckTokenExpireTime();if (res.Item1){return res.Item2;}else{//StartCoroutine(RefreshToken());var token = await PostTokenRequest();// 如果正在刷新 Token,可以考虑返回空字符串或者等待刷新完成return token;}}catch(Exception e){Debug.LogError($"错误: {e}");}throw new NullReferenceException("Token 无法获取");}/// <summary>/// 检查Token是否过期或者不存在/// </summary>/// <returns></returns>private (bool, string) CheckTokenExpireTime(){string tokenString = PlayerPrefs.GetString(accessKeyId, "");if (!string.IsNullOrEmpty(tokenString)){JObject token = JObject.Parse(tokenString);long expireTime = token["ExpireTime"].Value<long>();long currentTime = DateTimeOffset.UtcNow.ToUnixTimeSeconds();long timeLeft = expireTime - currentTime;string tokenID = token["Id"].Value<string>();if (timeLeft < 86400) // 24小时 = 86400秒{Debug.Log("Token 将在24小时内过期 True");return (false, null);}else{Debug.Log("Token 还可以使用 False");return (true, tokenID);}}return (false, null);}async UniTask<string> PostTokenRequest(){// 获取当前时间戳string timestamp = DateTime.UtcNow.ToString("yyyy-MM-ddTHH:mm:ssZ", CultureInfo.InvariantCulture);// 生成随机数string signatureNonce = Guid.NewGuid().ToString();// 构建请求参数字典 var parameters = new Dictionary<string, string>{{ "AccessKeyId", accessKeyId },{ "Action", action },{ "Format", formatType },{ "RegionId", regionId },{ "SignatureMethod", signatureMethod },{ "SignatureNonce", signatureNonce },{ "SignatureVersion", signatureVersion },{ "Timestamp", timestamp},{ "Version", version }};// 排序并构建规范化的查询字符串string queryString = EncodeDictionary(parameters);//Debug.Log("规范化的请求字符串: " + queryString);string stringToSign = "GET&" + EncodeText("/") + "&" + EncodeText(queryString);//Debug.Log("待签名的字符串: " + stringToSign);string signature = CalculateSignature(accessKeySecret, stringToSign);//Debug.Log("签名: " + signature);signature = EncodeText(signature);//Debug.Log("URL编码后的签名: " + signature);// 构建最终 URLstring url = $"https://nls-meta.cn-shanghai.aliyuncs.com/?Signature={signature}&{queryString}";//Debug.Log("url: " + url);using (UnityWebRequest www = UnityWebRequest.Get(url)){ var asyncOp = www.SendWebRequest();await asyncOp;var header = www.GetResponseHeaders();string headerStr = "";headerStr += www.uri.Host+"\n";//headerStr += www.uri. + "\n";foreach (var head in header){headerStr += $"{head.Key} : {head.Value} \n";}Debug.Log($"请求 Response: {headerStr}");//await www.SendWebRequest();if (www.result != UnityWebRequest.Result.Success){string textData = www.downloadHandler.text;Debug.LogError($"请求错误 Error:{www.result} + {www.error} -> {textData}");}else{// 解析返回的 JSON 数据string jsonResponse = www.downloadHandler.text;Debug.Log($"请求成功 Response: {jsonResponse}");JObject json = JObject.Parse(jsonResponse);JToken token = json["Token"];if (token != null && token["ExpireTime"] != null && token["Id"] != null){// 缓存 Token 数据PlayerPrefs.SetString(accessKeyId, token.ToString());PlayerPrefs.Save();long expireTime = token["ExpireTime"].Value<long>();long currentTime = DateTimeOffset.UtcNow.ToUnixTimeSeconds();// 将 Unix 时间戳转换为 DateTimeDateTime expiryDateTime = DateTimeOffset.FromUnixTimeSeconds(expireTime).UtcDateTime;DateTime currentDateTime = DateTimeOffset.FromUnixTimeSeconds(currentTime).UtcDateTime;TimeSpan timeLeft = expiryDateTime - currentDateTime;string formattedTime = $"{timeLeft.Days}天 {timeLeft.Hours}小时 {timeLeft.Minutes}分钟 {timeLeft.Seconds}秒";Debug.Log($"Token 数据保存成功 ; 当前时间:{currentDateTime.ToString("yyyy-MM-dd HH:mm:ss")} - 过期时间:{expiryDateTime.ToString("yyyy-MM-dd HH:mm:ss")} - 有效时长:{formattedTime}");return token.ToString();}else{Debug.Log("Token or required 的字段 不足或者丢失!");}}}return "";}private string EncodeText(string text){string encoded = UnityWebRequest.EscapeURL(text).Replace("+", "%20").Replace("*", "%2A").Replace("%7E", "~").Replace("%7E", "~");// 将所有小写十六进制代码转换为大写encoded = System.Text.RegularExpressions.Regex.Replace(encoded, "%[0-9a-f]{2}", m => m.Value.ToUpper());return encoded;}private string EncodeDictionary(Dictionary<string, string> dic){var items = dic.OrderBy(kvp => kvp.Key).Select(kvp =>$"{EncodeText(kvp.Key)}={EncodeText(kvp.Value)}");return string.Join("&", items);}private string CalculateSignature(string accessKeySecret, string stringToSign){// 将密钥字符串附加 "&" 后转换为字节var keyBytes = Encoding.UTF8.GetBytes(accessKeySecret + "&");// 将待签名字符串转换为字节var signBytes = Encoding.UTF8.GetBytes(stringToSign);using (var hmacsha1 = new HMACSHA1(keyBytes)){byte[] hashMessage = hmacsha1.ComputeHash(signBytes);string signature = Convert.ToBase64String(hashMessage);//signature = UnityWebRequest.EscapeURL(signature).Replace("+", "%20").Replace("*", "%2A").Replace("%7E", "~");return signature;// EncodeToUpper(signature);}}}
使用阿里云 TTS
using System;
using UnityEngine;
//using NativeWebSocket;
using System.Text.RegularExpressions;
using System.Net.Http;
using System.IO;
using UnityEngine.Networking;
using System.Runtime.CompilerServices;
using Cysharp.Threading.Tasks;//using System.Net.WebSockets;//public static class UnityWebRequestExtensions
//{
// public static TaskAwaiter<AsyncOperation> GetAwaiter(this UnityWebRequestAsyncOperation asyncOp)
// {
// TaskCompletionSource<AsyncOperation> tcs = new TaskCompletionSource<AsyncOperation>();
// asyncOp.completed += obj => tcs.SetResult(asyncOp);
// return tcs.Task.GetAwaiter();
// }
//}[Serializable]
public class Header
{public string message_id;public string task_id;public string @namespace;public string name;public string appkey;
}public class VoiceSynthesis : MonoBehaviour
{private AliTTSCtrl aliTTSCtrl;private TTSGeneral tTSGeneral;private AudioSource audioSource;void Start(){aliTTSCtrl = GetComponent<AliTTSCtrl>();audioSource = GetComponent<AudioSource>();}// 获取当前有效的 Token[ContextMenu("进行TTS")]
#if UNITY_EDITOR[ExecuteInEditMode]
#endifprivate async UniTask TTS(){if (aliTTSCtrl == null){aliTTSCtrl = GetComponent<AliTTSCtrl>();}if (audioSource == null){audioSource = GetComponent<AudioSource>();}var token = await aliTTSCtrl.GetToken();Debug.Log($"Token {token}");if (tTSGeneral == null){tTSGeneral = new TTSGeneral("******", token, audioSource);}await tTSGeneral.SpeakAsync();}void OnDestroy(){}private void OnApplicationQuit(){}
}public class TTSGeneral
{private string appKey = ""; private string accessToken = "";private AudioSource audioSource;private string ttsUrl = "https://nls-gateway-cn-shanghai.aliyuncs.com/stream/v1/tts";private string v;private UniTask<string> token;public TTSGeneral(string appKey,string accessToken,AudioSource audioSource){this.appKey = appKey;this.accessToken = accessToken;this.audioSource = audioSource;}public async UniTask<string> SynthesizeSpeech(string text,string format = "wav",int sampleRate = 16000,string voice = "siyue"){try{using (var client = new HttpClient()){var url = $"{ttsUrl}?appkey={appKey}&token={accessToken}&text={Uri.EscapeDataString(text)}&format={format}&sample_rate={sampleRate}&voice={voice}";HttpResponseMessage response = await client.GetAsync(url);response.EnsureSuccessStatusCode();byte[] audioBytes = await response.Content.ReadAsByteArrayAsync();// Save the audio file or play it directly in Unitystring path = Path.Combine(Application.persistentDataPath, "output.wav");File.WriteAllBytes(path, audioBytes);return path;}}catch (Exception ex){Debug.LogError($"Error synthesizing speech: {ex.Message}");throw;}}public async UniTask SpeakAsync(){string textToSpeak = "采用最先进的端到端语音识别框架,字错误率相比上一代系统相对下降10%至30%,并发推理速度相比业内主流推理推理框架提升10倍以上,同时支持实时和离线语音识别,毫秒级延迟。";string audioFilePath = null;try{audioFilePath = await SynthesizeSpeech(textToSpeak);}catch (Exception ex){Debug.LogError($"Error during speech synthesis: {ex.Message}");}//audioFilePath = path.Result;if (!string.IsNullOrEmpty(audioFilePath)){// Load and play the audio file in Unity using UnityWebRequestusing (UnityWebRequest www = UnityWebRequestMultimedia.GetAudioClip("file://" + audioFilePath, AudioType.WAV)){DownloadHandlerAudioClip downloadHandler = www.downloadHandler as DownloadHandlerAudioClip;downloadHandler.streamAudio = true; // Stream the audio to save memoryawait www.SendWebRequest();if (www.result == UnityWebRequest.Result.Success){AudioClip clip = downloadHandler.audioClip;if (audioSource != null){audioSource.clip = clip;audioSource.Play();}else{Debug.LogError($"必须有 AudioSource 组件才可以播放");}}else{Debug.LogError($"Failed to load audio file: {www.error}");}}}}
}//public class TTSStream
//{
// private WebSocket ws;
// private bool isSynthesisStarted = false;// async void ConnectWebSocket()
// {
// // 替换为你的 WebSocket 服务地址和 Token
// ws = new WebSocket("wss://nls-gateway-cn-beijing.aliyuncs.com/ws/v1?token=your-nls-token");
// ws.OnOpen += () =>
// {
// Debug.Log("WebSocket connected!");
// SendStartSynthesis();
// };// ws.OnError += (e) =>
// {
// Debug.LogError("Error: " + e);
// };
// ws.OnClose += (e) =>
// {
// Debug.Log("WebSocket closed!");
// };// ws.OnMessage += (bytes) =>
// {
// Debug.Log("Received message: " + bytes);
// };// Keep sending messages at every 0.3s
// //InvokeRepeating("SendWebSocketMessage", 0.0f, 0.3f);// await ws.Connect();
// }// void Update()
// {
//#if !UNITY_WEBGL || UNITY_EDITOR
// if (ws != null)
// {
// ws.DispatchMessageQueue();// }//#endif
// }// async void SendWebSocketMessage()
// {
// if (ws.State == WebSocketState.Open)
// {
// // Sending bytes
// await ws.Send(new byte[] { 10, 20, 30 });// // Sending plain text
// await ws.SendText("plain text message");
// }
// }// private async void SendStartSynthesis()
// {
// var header = GetHeader("StartSynthesis");
// var paramsJson = JsonUtility.ToJson(header);
// await ws.SendText(paramsJson);
// }// string GenerateUUID()
// {
// long d = DateTime.Now.Ticks;
// long d2 = (long)(DateTime.UtcNow - new DateTime(1970, 1, 1)).TotalMilliseconds * 1000;
// return Regex.Replace("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx", "[xy]", delegate (Match match)
// {
// int r = new System.Random().Next(16);
// if (d > 0)
// {
// r = ((int)(d + r) % 16) | 0;
// d = (long)Math.Floor(d / 16.0);
// }
// else
// {
// r = ((int)(d2 + r) % 16) | 0;
// d2 = (long)Math.Floor(d2 / 16.0);
// }
// return (match.Value == "x" ? r : (r & 0x3 | 0x8)).ToString("x");
// });
// }// Header GetHeader(string name)
// {
// return new Header
// {
// message_id = GenerateUUID(),
// task_id = GenerateUUID(), // 根据需要生成 task_id
// @namespace = "FlowingSpeechSynthesizer",
// name = name,
// appkey = "your-nls-appkey"
// };
// }// public async void CloseWs()
// {
// if (ws != null)
// {
// await ws.Close();
// ws = null;
// }
// }//}
使用阿里云 OCR
using System;
using System.Collections;
using System.Collections.Generic;using UnityEngine;
using System.IO;
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
using Imagine.WebAR;
using Cysharp.Threading.Tasks;
using Tea;
using AlibabaCloud.OpenApiClient.Models;
using AlibabaCloud.SDK.Ocr_api20210707.Models;
using AlibabaCloud.TeaUtil.Models;
using AlibabaCloud.SDK.Ocr_api20210707;
using AlibabaCloud.TeaUtil;
using AlibabaCloud.OpenApiClient;
using AlibabaCloud.OpenApiUtil;
using System.Reflection;public class AliOCR : MonoBehaviour
{public Texture2D texture;public RenderTexture textureRender;private OCRUnified oCR;private TextureExtractor_WarpedImage warpedImage;private readonly string accessKeyId = "********"; private readonly string accessKeySecret = "********"; // Start is called before the first frame updatevoid Start(){if (oCR == null){oCR = new OCRUnified(accessKeyId, accessKeySecret);}warpedImage = GetComponent<TextureExtractor_WarpedImage>();}public void OnImageFound(string id){if (warpedImage == null){warpedImage = GetComponent<TextureExtractor_WarpedImage>();}warpedImage.ExtractTexture(id);//string ocrContent = await OCR();Debug.Log($"执行 OCR 识别.....");try{OCR(); // 启动但不等待}catch (Exception ex){Debug.LogError("OCR failed 2 : " + ex.Message);}}public void OnImageLost(string id){}// 获取当前有效的 Token[ContextMenu("进行ORC")]
#if UNITY_EDITOR[ExecuteInEditMode]
#endifprivate void OCR(){Debug.Log($"执行 OCR 识别2 .....");if (oCR == null){oCR = new OCRUnified(accessKeyId, accessKeySecret);}try{//byte[] imageData = texture.EncodeToJPG();var imaStream = RenderTextureAsync();string res = oCR.Run(imaStream);//string res = await UniTask.Create(async () =>//{// // 直接调用同步方法// string result = oCR.Run(imaStream);// await UniTask.Yield(); // 等待至少下一帧// return result;//});if (res == ""){Debug.Log($"没有识别到任何文字:{res}");}else{Debug.Log($"识别到文字:{res}");}imaStream.Close();//return res;}catch (Exception ex){Debug.LogError("OCR failed2 : " + ex.Message);//return "OCR failed"; // 返回错误信息}}private Stream RenderTextureAsync(){// 等待渲染线程结束//await UniTask.WaitForEndOfFrame(this);// 临时激活渲染纹理的上下文RenderTexture.active = textureRender;// 创建一个新的 Texture2D 对象来接收像素Texture2D texture2D = new Texture2D(textureRender.width, textureRender.height, TextureFormat.RGB24, false);texture2D.ReadPixels(new Rect(0, 0, textureRender.width, textureRender.height), 0, 0);texture2D.Apply();// 重置渲染纹理的上下文RenderTexture.active = null;// 编码 Texture2D 数据为 JPGbyte[] jpgData = texture2D.EncodeToJPG();// 释放 Texture2D 对象
#if UNITY_EDITORDestroyImmediate(texture2D);
#elseDestroy(texture2D);
#endif// 将 JPG 数据写入内存流//using (MemoryStream stream = new MemoryStream())//{// stream.Write(jpgData, 0, jpgData.Length);// return stream;// // 这里可以添加额外的处理,如保存文件或发送数据//}return new MemoryStream(jpgData);}}/// <summary>
/// OCR 统一识别
/// </summary>
public class OCRUnified
{private AlibabaCloud.OpenApiClient.Client aliClient;private AlibabaCloud.SDK.Ocr_api20210707.Client aliClient2;public OCRUnified(string accessKeyId, string accessKeySecret){aliClient = CreateClient(accessKeyId, accessKeySecret);aliClient2 = CreateClient2(accessKeyId, accessKeySecret);}public string Run(Stream textureBody){try{Debug.Log($"执行 OCR 识别3 .....");AlibabaCloud.OpenApiClient.Models.Params params_ = CreateApiInfo();Debug.Log($" 1 OCR 创建 API 成功 .....");// query paramsDictionary<string, object> queries = new Dictionary<string, object>() { };queries["Type"] = "Advanced";queries["OutputFigure"] = true;//byte[] imageData = texture.EncodeToJPG();// 需要安装额外的依赖库,直接点击下载完整工程即可看到所有依赖。//Stream body = new MemoryStream(imageData) ;//AlibabaCloud.DarabonbaStream.StreamUtil.ReadFromFilePath("<your-file-path>");// runtime optionsAlibabaCloud.TeaUtil.Models.RuntimeOptions runtime = new AlibabaCloud.TeaUtil.Models.RuntimeOptions();Debug.Log($" 2 OCR 创建 RuntimeOptions 成功 .....");AlibabaCloud.OpenApiClient.Models.OpenApiRequest request = new AlibabaCloud.OpenApiClient.Models.OpenApiRequest{Query = AlibabaCloud.OpenApiUtil.Client.Query(queries),Stream = textureBody,};Debug.Log($" 3 OCR 创建 OpenApiRequest 成功 .....");// 复制代码运行请自行打印 API 的返回值// 返回值实际为 Map 类型,可从 Map 中获得三类数据:响应体 body、响应头 headers、HTTP 返回的状态码 statusCode。//Debug.Log($"执行 OCR params_:{params_.ToString()} - request:{request} - runtime:{runtime}");Dictionary<string, object> res = null;try{Debug.Log($"params : {ConvertToJson(params_)}");Debug.Log($"request : {ConvertToJson(request)}");Debug.Log($"runtime : {ConvertToJson(runtime)}");res = aliClient.CallApi(params_, request, runtime); //new Dictionary<string, object>();}catch (Exception ex){Debug.LogError($"CallApi 错误 {ex}");}Debug.Log($" 4 OCR 请求 成功 .....");string jsonString = JsonConvert.SerializeObject(res, Formatting.Indented);if (res.ContainsKey("statusCode")){var statusCode = res["statusCode"];int code = int.Parse(statusCode.ToString());if (code == 200){// 打印 JSON 字符串到控制台Debug.Log(jsonString);// 将 JSON 字符串解析为 JObjectJObject jsonObject = JObject.Parse(jsonString);// 从 JObject 获取 "Data" 下的 "Content"string content = jsonObject["body"]["Data"]["Content"].ToString();Debug.Log($"content = {content}");return content;//PrintDictionary(res);}else{var strRes = $"识别异常 {code} -> {jsonString} ";Debug.LogError(strRes);return strRes;}}return $"不是有效的返回 {jsonString}";}catch (Exception ex){var strRes = $"ORC 错误: {ex}";Debug.LogError(strRes);return strRes;}}public static string ConvertToJson(object obj){var properties = obj.GetType().GetProperties(BindingFlags.Public | BindingFlags.Instance);var result = new System.Text.StringBuilder();result.Append("{");bool first = true;foreach (var property in properties){if (!first){result.Append(", ");}else{first = false;}try{var value = property.GetValue(obj, null);string jsonValue = value != null ? value.ToString() : "null";result.AppendFormat("\"{0}\": {1}", property.Name, jsonValue);}catch (Exception ex){// Handle the exception if the property cannot be serialized to JSONresult.AppendFormat("\"{0}\": \"Error: {1}\"", property.Name, ex.Message);}}result.Append("}");return result.ToString();}public static void PrintJson(object obj){Debug.Log(ConvertToJson(obj));}// 打印字典的方法//void PrintDictionary(Dictionary<string, object> dictionary)//{// foreach (KeyValuePair<string, object> entry in dictionary)// {// // 检查值的类型,如果是列表或数组,需要特别处理// if (entry.Value is List<string>)// {// List<string> list = entry.Value as List<string>;// Debug.Log(entry.Key + ": [" + string.Join(", ", list) + "]");// }// else// {// Debug.Log(entry.Key + ": " + entry.Value);// }// }//}/// <term><b>Description:</b></term>/// <description>/// <para>使用AK&SK初始化账号Client</para>public AlibabaCloud.OpenApiClient.Client CreateClient(string accessKeyId, string accessKeySecret){// 工程代码泄露可能会导致 AccessKey 泄露,并威胁账号下所有资源的安全性。以下代码示例仅供参考。// 建议使用更安全的 STS 方式,更多鉴权访问方式请参见:https://help.aliyun.com/document_detail/378671.html。AlibabaCloud.OpenApiClient.Models.Config config = new AlibabaCloud.OpenApiClient.Models.Config{// 必填,请确保代码运行环境设置了环境变量 ALIBABA_CLOUD_ACCESS_KEY_ID。AccessKeyId = accessKeyId,// Environment.GetEnvironmentVariable("ALIBABA_CLOUD_ACCESS_KEY_ID"),// 必填,请确保代码运行环境设置了环境变量 ALIBABA_CLOUD_ACCESS_KEY_SECRET。AccessKeySecret = accessKeySecret //Environment.GetEnvironmentVariable("ALIBABA_CLOUD_ACCESS_KEY_SECRET"),};// Endpoint 请参考 https://api.aliyun.com/product/ocr-apiconfig.Endpoint = "ocr-api.cn-hangzhou.aliyuncs.com";return new AlibabaCloud.OpenApiClient.Client(config);}public AlibabaCloud.SDK.Ocr_api20210707.Client CreateClient2(string accessKeyId, string accessKeySecret){// 工程代码泄露可能会导致 AccessKey 泄露,并威胁账号下所有资源的安全性。以下代码示例仅供参考。// 建议使用更安全的 STS 方式,更多鉴权访问方式请参见:https://help.aliyun.com/document_detail/378671.html。AlibabaCloud.OpenApiClient.Models.Config config = new AlibabaCloud.OpenApiClient.Models.Config{// 必填,请确保代码运行环境设置了环境变量 ALIBABA_CLOUD_ACCESS_KEY_ID。AccessKeyId = accessKeyId,//Environment.GetEnvironmentVariable("ALIBABA_CLOUD_ACCESS_KEY_ID"),// 必填,请确保代码运行环境设置了环境变量 ALIBABA_CLOUD_ACCESS_KEY_SECRET。AccessKeySecret = accessKeySecret,// Environment.GetEnvironmentVariable("ALIBABA_CLOUD_ACCESS_KEY_SECRET"),};// Endpoint 请参考 https://api.aliyun.com/product/ocr-apiconfig.Endpoint = "ocr-api.cn-hangzhou.aliyuncs.com";return new AlibabaCloud.SDK.Ocr_api20210707.Client(config);}public string Run2(Stream textureBody){//AlibabaCloud.SDK.Ocr_api20210707.Client client = CreateClient();// 需要安装额外的依赖库,直接点击下载完整工程即可看到所有依赖。Stream bodyStream = AlibabaCloud.DarabonbaStream.StreamUtil.ReadFromFilePath("<your-file-path>");var dd = new AlibabaCloud.SDK.Ocr_api20210707.Models.DataSubImagesFigureInfoValue();AlibabaCloud.SDK.Ocr_api20210707.Models.RecognizeAllTextRequest recognizeAllTextRequest = new AlibabaCloud.SDK.Ocr_api20210707.Models.RecognizeAllTextRequest{Type = "Advanced",OutputFigure = true,Body = textureBody,};AlibabaCloud.TeaUtil.Models.RuntimeOptions runtime = new AlibabaCloud.TeaUtil.Models.RuntimeOptions();try{// 复制代码运行请自行打印 API 的返回值recognizeAllTextRequest.Validate();Debug.Log($" 验证通过 ");var res = aliClient2.RecognizeAllTextWithOptions(recognizeAllTextRequest, runtime);return res.Body.Data.Content;}catch (TeaException error){// 此处仅做打印展示,请谨慎对待异常处理,在工程项目中切勿直接忽略异常。// 错误 messageDebug.Log($"Tea错误 : {error.Message}");// 诊断地址Debug.Log($"Tea错误2 : {error.Data["Recommend"]}");AlibabaCloud.TeaUtil.Common.AssertAsString(error.Message);}catch (Exception _error){Debug.Log($"错误 : {_error}");TeaException error = new TeaException(new Dictionary<string, object>{{ "message", _error.Message }});// 此处仅做打印展示,请谨慎对待异常处理,在工程项目中切勿直接忽略异常。// 错误 messageDebug.Log($"错误2 : {error.Message}");// 诊断地址Debug.Log($"错误3 : {error.Data["Recommend"]}");AlibabaCloud.TeaUtil.Common.AssertAsString(error.Message);}return "";}/// <term><b>Description:</b></term>/// <description>/// <para>API 相关</para>public AlibabaCloud.OpenApiClient.Models.Params CreateApiInfo(){AlibabaCloud.OpenApiClient.Models.Params params_ = new AlibabaCloud.OpenApiClient.Models.Params{// 接口名称Action = "RecognizeAllText",// 接口版本Version = "2021-07-07",// 接口协议Protocol = "HTTPS",// 接口 HTTP 方法Method = "POST",AuthType = "AK",Style = "V3",// 接口 PATHPathname = "/",// 接口请求体内容格式ReqBodyType = "json",// 接口响应体内容格式BodyType = "json",};return params_;}public void RecognizeAllTextWithOptions(){}}
相关文章:
Unity阿里云OpenAPI 获取 Token的C#【记录】
获取Token using UnityEngine; using System; using System.Text; using System.Linq; using Newtonsoft.Json.Linq; using System.Security.Cryptography; using UnityEngine.Networking; using System.Collections.Generic; using System.Globalization; using Cysharp.Thr…...
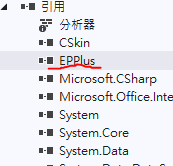
winfrom项目,引用EPPlus.dll实现将DataTable 中的数据保存到Excel文件
最近研究不安装office也可以保存Excel文件,在网上查询资料找到这个方法。 第一步:下载EPPlus.dll文件(自行去网上搜索下载) 第二步:引用到需要用的项目中,如图所示: 第三步:写代码…...
【C++基础】多线程并发场景下的同步方法
如果在多线程程序中对全局变量的访问没有进行适当的同步控制(例如使用互斥锁、原子变量等),会导致多个线程同时访问和修改全局变量时发生竞态条件(race condition)。这种竞态条件可能会导致一系列不确定和严重的后果。…...
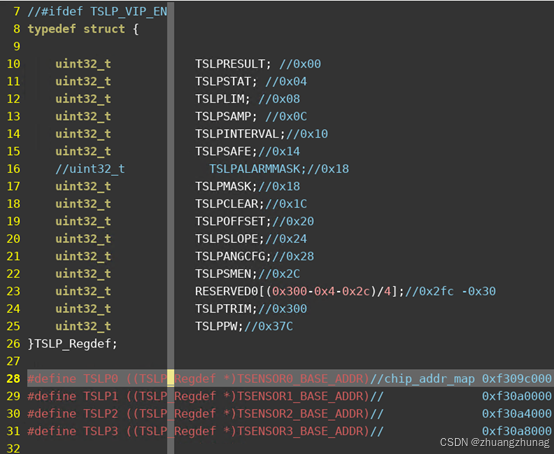
C语言#define TSLP0 (TSLP_Regdef *)TSENSORO BASE ADDR)的含义?
在C语言中,#define指令用于定义宏。宏是一种预处理器指令,它允许你为代码片段指定一个名称,以便在编译时进行替换。 从你的描述来看,似乎你想定义一个名为 TSLP0 的宏,其值是某个寄存器地址。假设 TSENSORO_BASE_ADDR…...
微信小程序wxs实现UTC转北京时间
微信小程序实现UTC转北京时间 打脸一刻:最近在迭代原生微信小程序,好一段时间没写原生的,有点不习惯; 咦,更新数据咋不生效呢?原来还停留在 this.xxx; 哟,事件又没反应了?…...
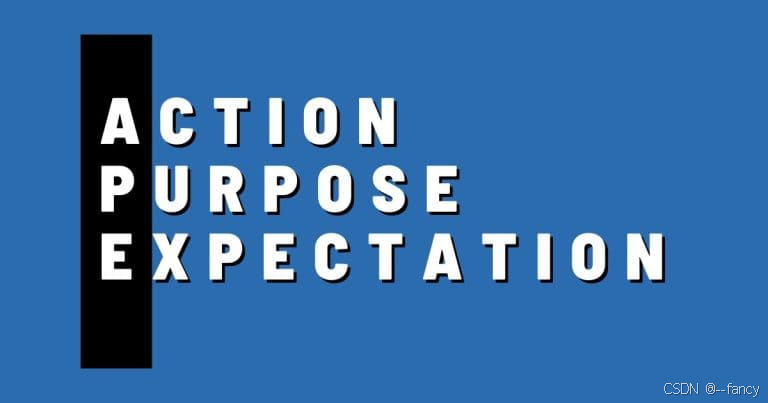
提示词的艺术 ---- AI Prompt 进阶(提示词框架)
提示词的艺术 ---- AI Prompt 进阶(提示词框架) 写在前面 上周发布了一篇《提示词的艺术----AI Prompt撰写指南》,旨在帮助读者理解提示词的作用,以及简单的提示词撰写指南。本篇作为进阶内容,将给出常用的提示词框架…...
WPF常见面试题解答
以下是WPF(Windows Presentation Foundation)面试中常见的问题及解答,涵盖基础概念、高级功能和实际应用,帮助你更好地准备面试: 基础概念 什么是WPF? WPF是微软开发的用于构建桌面应用程序的UI框架&#x…...
TypeScript 学习
TypeScript 类型 准备本地环境 初始化 TypeScript 项目, 生成 package.json 文件: npm init -y安装 typescript: yarn add typescript -D初始化 TypeScript 配置文件: npx tsc--init输出: Created a new tsconfig.json with:target: es2016module: commonjsstrict: true…...
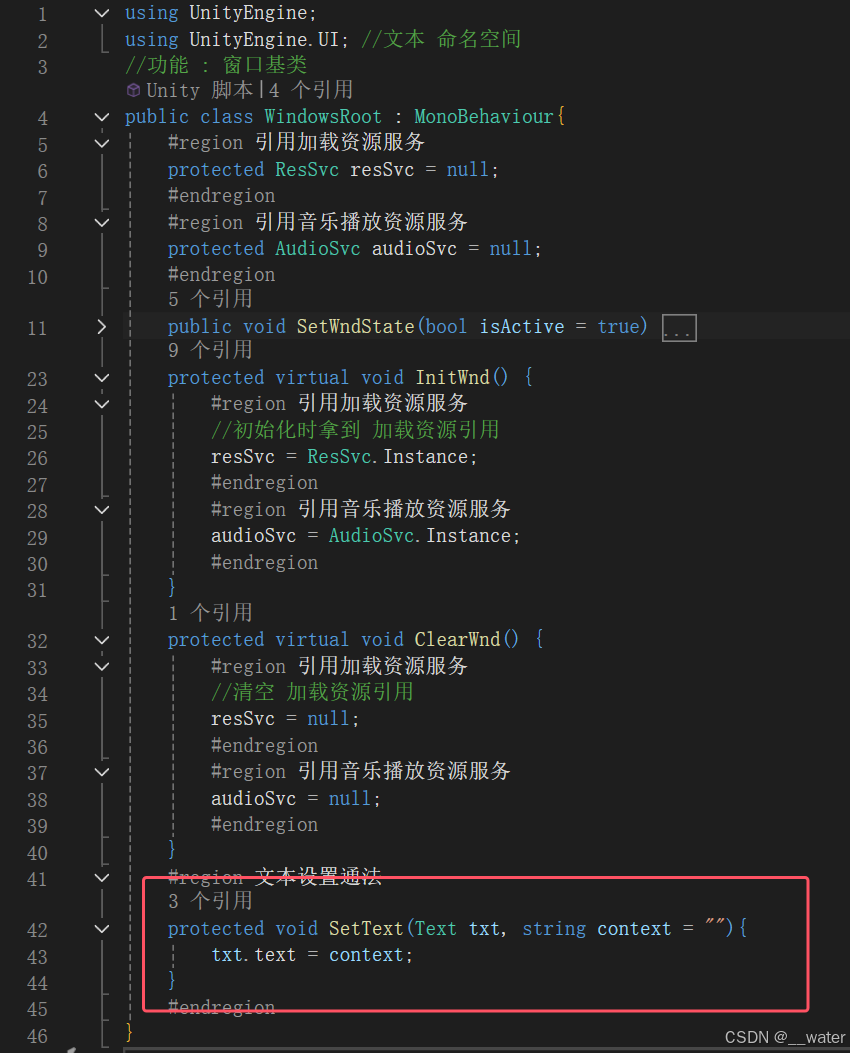
24_游戏启动逻辑梳理总结
首先这个项目从游戏根入口GameRoot.cs的初始化开始 分为 服务层初始化Svc.cs 与 业务系统层初始化Sys.cs 而服务层 分为 资源加载服务层ResSvc.cs 与 音乐播放服务层AudioSvc.cs 而在 资源加载服务层ResSvc.cs中 初始化了 名字的 配置文件 而音乐播放服务层AudioSvc.cs 暂时没…...
C++/CLI(Common Language Runtime)关键点详解
C++/CLI(Common Language Runtime)是 Microsoft Visual C++ 的一个扩展,允许使用 .NET Framework 的功能,同时保留对本机 C++ 代码的访问。当您需要在 C++ 和 C# 之间进行互操作时,C++/CLI 是一种常见的选择,因为它可以作为桥梁,将托管代码(如 C#)与非托管代码(如 C+…...
Transfoemr的解码器(Decoder)与分词技术
在自然语言处理(NLP)领域,解码器(Decoder)和分词技术是两个至关重要的概念。解码器是序列生成任务的核心组件,而分词则是将文本数据转换为可处理形式的基础步骤。 一、解码器(Decoder&…...
CDSN 2024博客之星总评选-主题文章创作,我的AI之路-起手篇
CDSN 2024博客之星总评选-主题文章创作,我的AI之路-起手篇 一. 回顾自己的机器学习之路二. 2024年的大模型学习三. 对自己的期望 一. 回顾自己的机器学习之路 自2019年起,我开始涉足机器学习领域,最初接触的是通过模型实现自动化的任务&…...
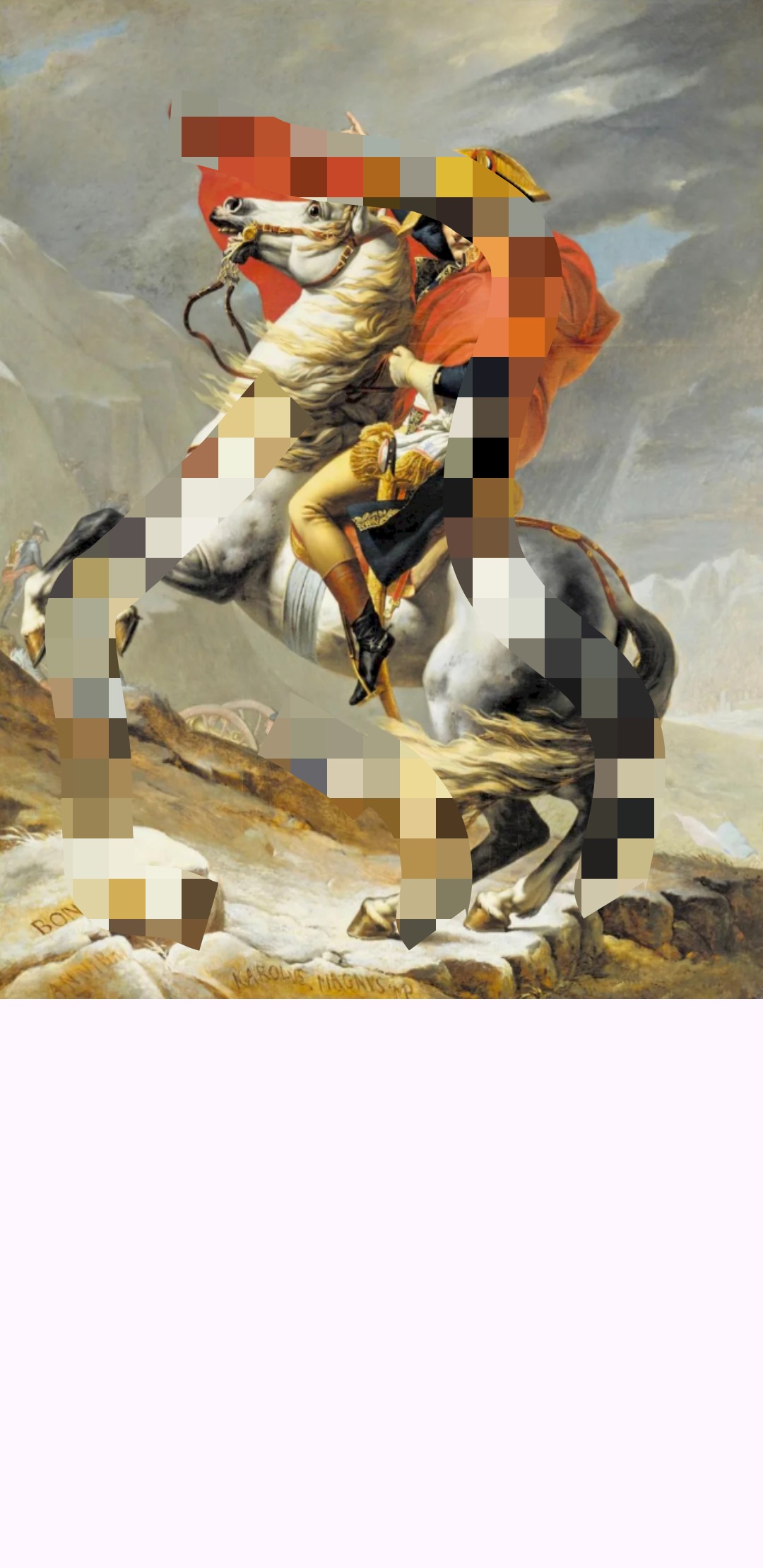
Android BitmapShader简洁实现马赛克,Kotlin(二)
Android BitmapShader简洁实现马赛克,Kotlin(二) 这一篇 Android BitmapShader简洁实现马赛克,Kotlin(一)-CSDN博客 遗留一个问题,xml定义的MyView为wrap_content的宽高,如果改成其…...
蓝桥杯 阶乘的和(C++完整代码+详细分析)
题目描述 原题链接 阶乘的和 问题描述 给定 n 个数 Ai,问能满足 m! 为 ∑(Ai!) 的因数的最大的 m 是多少。其中 m! 表示 m 的阶乘,即 123⋯m。 输入格式 输入的第一行包含一个整数 n。 第二行包含 n 个整数,分别表示 Ai,相…...
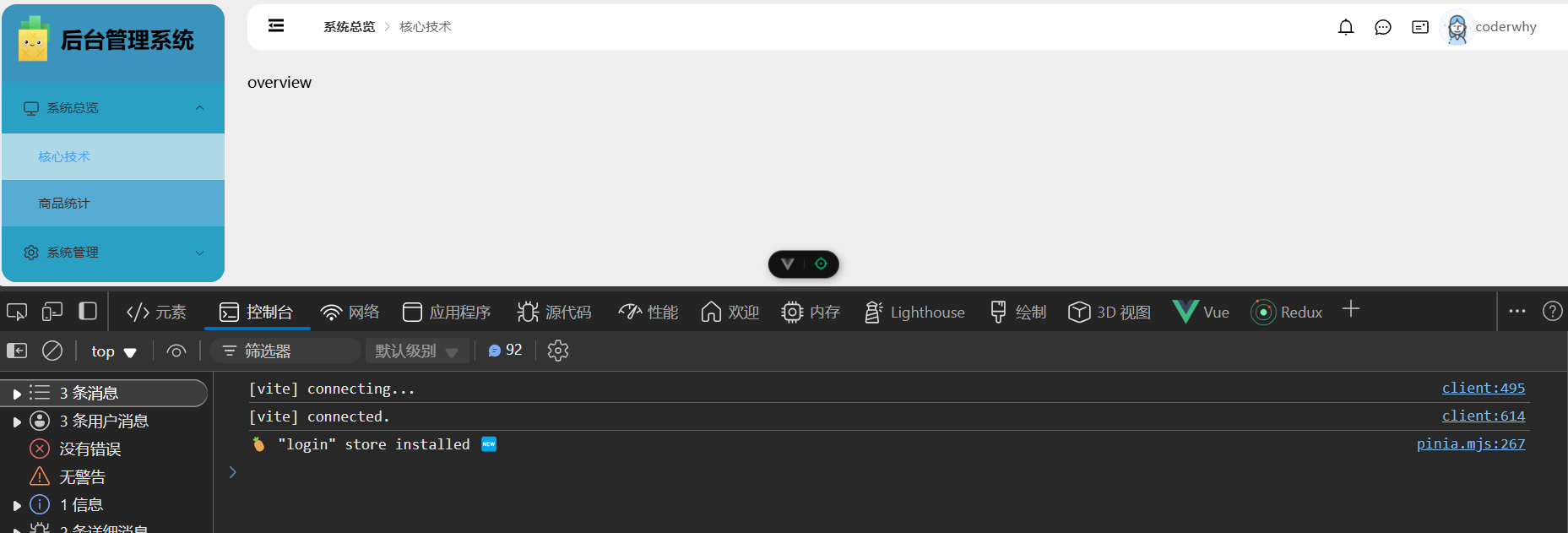
【Bug 记录】el-sub-menu 第一次进入默认不高亮
项目场景: 项目场景:el-sub-menu 第一次进入默认不高亮 问题描述 例如:sub-menu 的 index 后端默认传过来是 number,我们需要手动转为 string,否则会有警告,而且第一次进入 sub-menu 默认不高亮。 解决方…...
SpringCloud两种注册中心
SpringCloud 基本概念 系统架构 我们之前做的所有的项目都属于单体架构,下面我们将要学习更适合大型项目的分布式架构 单体架构: 将业务的所有功能几种在一个项目中开发,打成一个包部署。 优点:架构简单、部署成本低 缺点&am…...
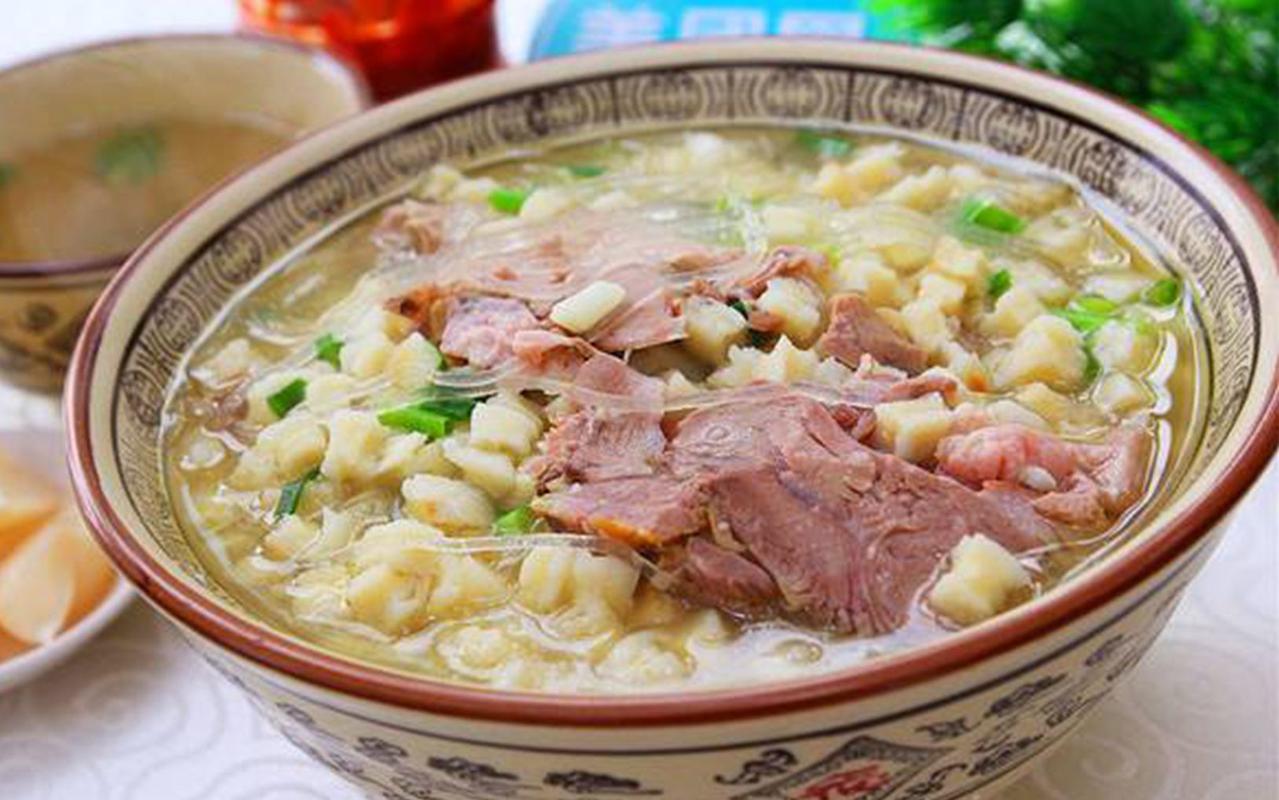
陕西羊肉泡馍:味蕾上的西北风情
陕西羊肉泡馍:味蕾上的西北风情 在广袤的西北地区,有一道美食以其独特的口感、丰富的营养价值和深厚的文化底蕴,成为了无数食客心中的佳肴——陕西羊肉泡馍。这道传统美食,不仅承载着陕西人民的饮食智慧,更以其醇厚的味道和暖胃耐饥的特性,赢得了国内外食客的一致赞誉。 历史渊…...
蓝桥杯试题:整数反转
一、题目要求: 给定一个整数,请将该数各个位上数字反转得到一个新数。新数也应满足整数的常见形式,即除非给定的原数为零,否则反转后得到的新数的最高位数字不应为零 二、题目分析代码演示: 该程序的主要功能是接收一个整数输入&…...
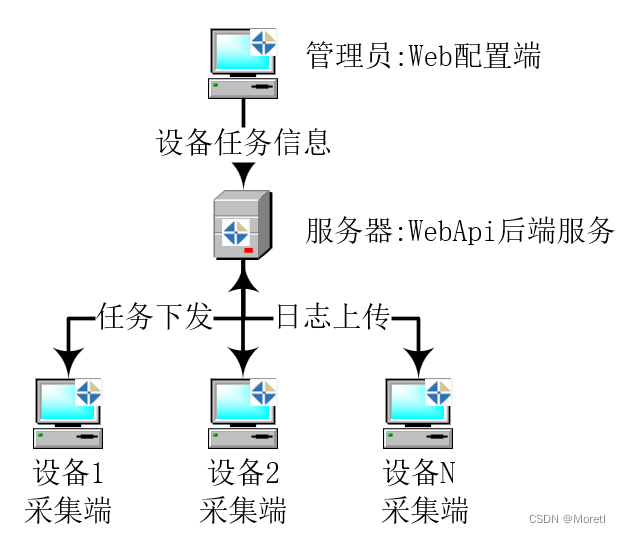
Moretl FileSync增量文件采集工具
永久免费: <下载> <使用说明> 我们希望Moretl FileSync是一款通用性很好的文件日志采集工具,解决工厂环境下,通过共享目录采集文件,SMB协议存在的安全性,兼容性的问题. 同时,我们发现工厂设备日志一般为增量,为方便MES,QMS等后端系统直接使用数据,我们推出了增量采…...
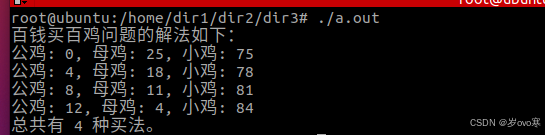
day1代码练习
输出3-100以内的完美数,(完美数:因子和(因子不包含自身)数本身) #include <stdio.h>// 判断一个数是否为完美数的函数 int panduan(int n) {if (n < 2) {return 0; // 小于2的数不可能是完美数}int sum 1; // 因子和初始化为1(因…...

XCTF-web-easyupload
试了试php,php7,pht,phtml等,都没有用 尝试.user.ini 抓包修改将.user.ini修改为jpg图片 在上传一个123.jpg 用蚁剑连接,得到flag...
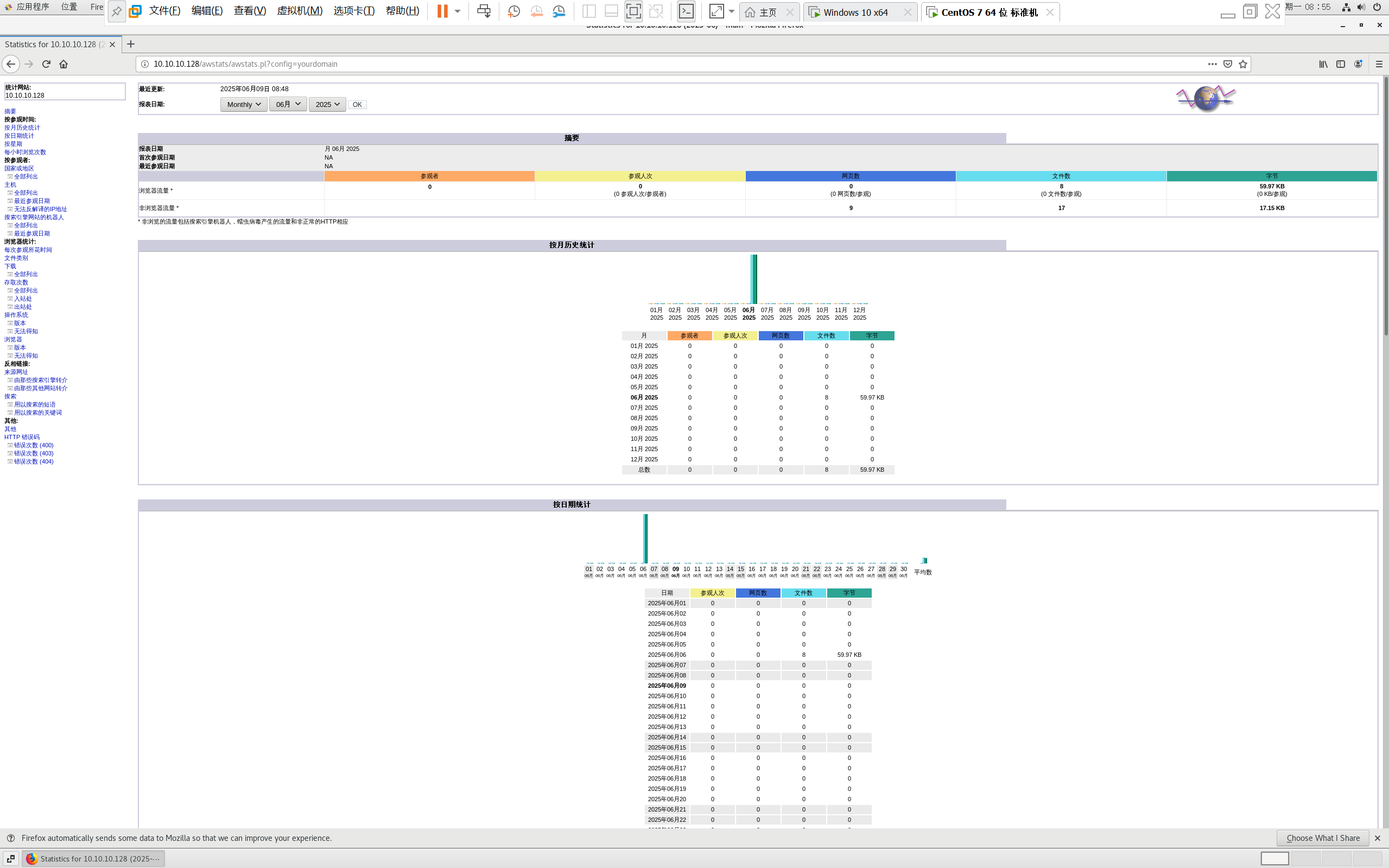
centos 7 部署awstats 网站访问检测
一、基础环境准备(两种安装方式都要做) bash # 安装必要依赖 yum install -y httpd perl mod_perl perl-Time-HiRes perl-DateTime systemctl enable httpd # 设置 Apache 开机自启 systemctl start httpd # 启动 Apache二、安装 AWStats࿰…...
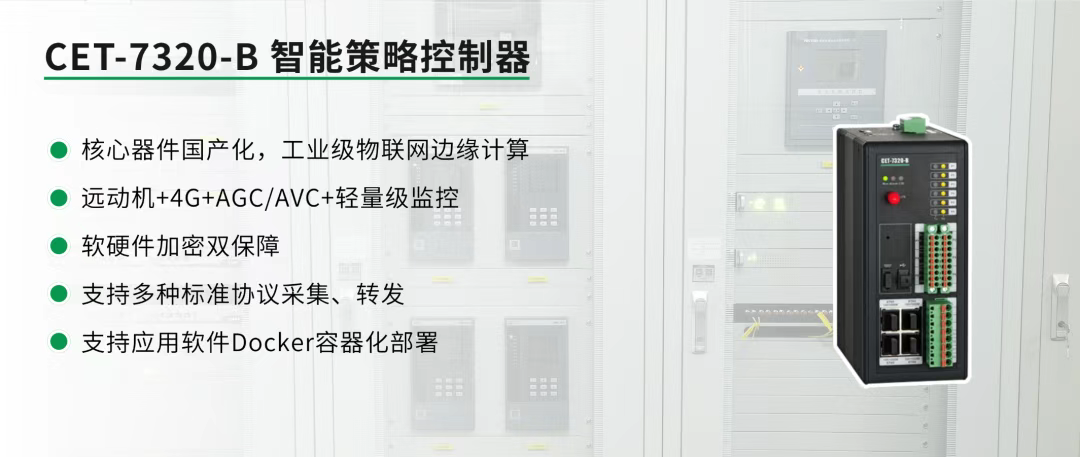
屋顶变身“发电站” ,中天合创屋面分布式光伏发电项目顺利并网!
5月28日,中天合创屋面分布式光伏发电项目顺利并网发电,该项目位于内蒙古自治区鄂尔多斯市乌审旗,项目利用中天合创聚乙烯、聚丙烯仓库屋面作为场地建设光伏电站,总装机容量为9.96MWp。 项目投运后,每年可节约标煤3670…...
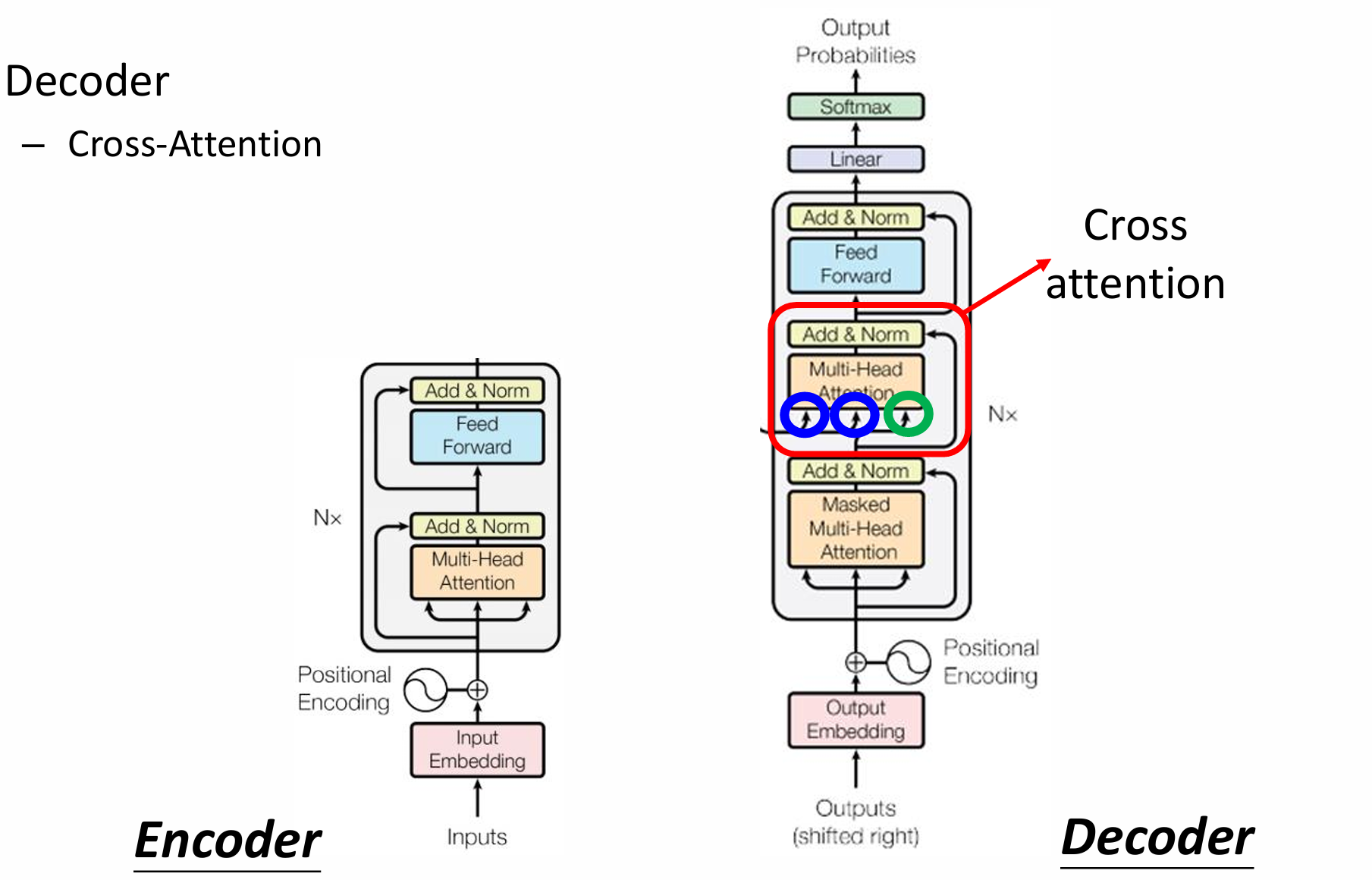
自然语言处理——Transformer
自然语言处理——Transformer 自注意力机制多头注意力机制Transformer 虽然循环神经网络可以对具有序列特性的数据非常有效,它能挖掘数据中的时序信息以及语义信息,但是它有一个很大的缺陷——很难并行化。 我们可以考虑用CNN来替代RNN,但是…...
【JavaSE】绘图与事件入门学习笔记
-Java绘图坐标体系 坐标体系-介绍 坐标原点位于左上角,以像素为单位。 在Java坐标系中,第一个是x坐标,表示当前位置为水平方向,距离坐标原点x个像素;第二个是y坐标,表示当前位置为垂直方向,距离坐标原点y个像素。 坐标体系-像素 …...
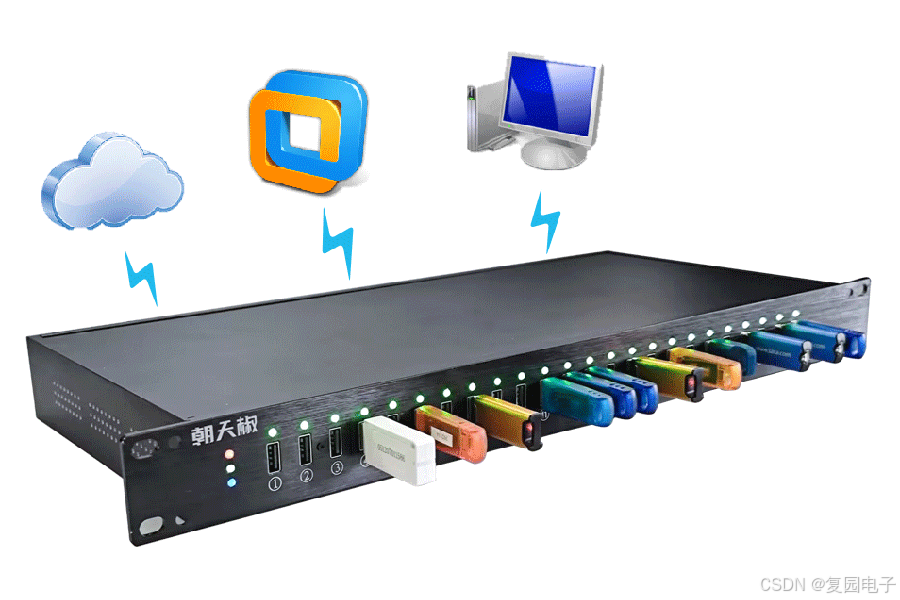
USB Over IP专用硬件的5个特点
USB over IP技术通过将USB协议数据封装在标准TCP/IP网络数据包中,从根本上改变了USB连接。这允许客户端通过局域网或广域网远程访问和控制物理连接到服务器的USB设备(如专用硬件设备),从而消除了直接物理连接的需要。USB over IP的…...
Redis的发布订阅模式与专业的 MQ(如 Kafka, RabbitMQ)相比,优缺点是什么?适用于哪些场景?
Redis 的发布订阅(Pub/Sub)模式与专业的 MQ(Message Queue)如 Kafka、RabbitMQ 进行比较,核心的权衡点在于:简单与速度 vs. 可靠与功能。 下面我们详细展开对比。 Redis Pub/Sub 的核心特点 它是一个发后…...
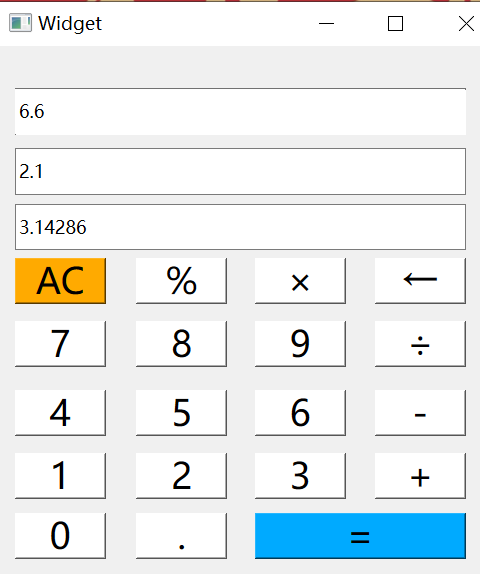
6.9-QT模拟计算器
源码: 头文件: widget.h #ifndef WIDGET_H #define WIDGET_H#include <QWidget> #include <QMouseEvent>QT_BEGIN_NAMESPACE namespace Ui { class Widget; } QT_END_NAMESPACEclass Widget : public QWidget {Q_OBJECTpublic:Widget(QWidget *parent nullptr);…...
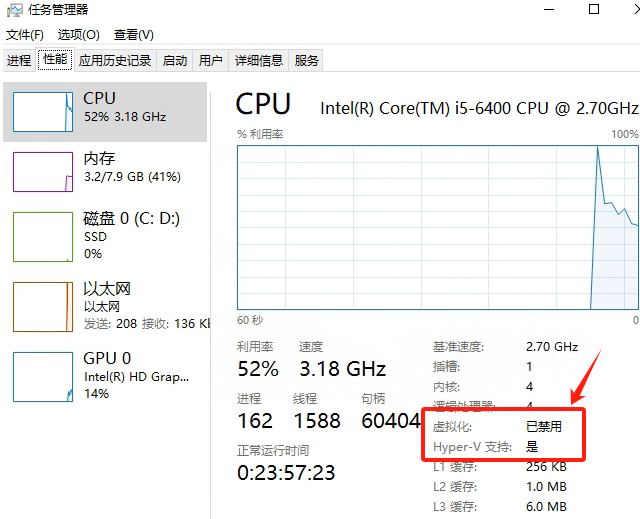
Windows电脑能装鸿蒙吗_Windows电脑体验鸿蒙电脑操作系统教程
鸿蒙电脑版操作系统来了,很多小伙伴想体验鸿蒙电脑版操作系统,可惜,鸿蒙系统并不支持你正在使用的传统的电脑来安装。不过可以通过可以使用华为官方提供的虚拟机,来体验大家心心念念的鸿蒙系统啦!注意:虚拟…...
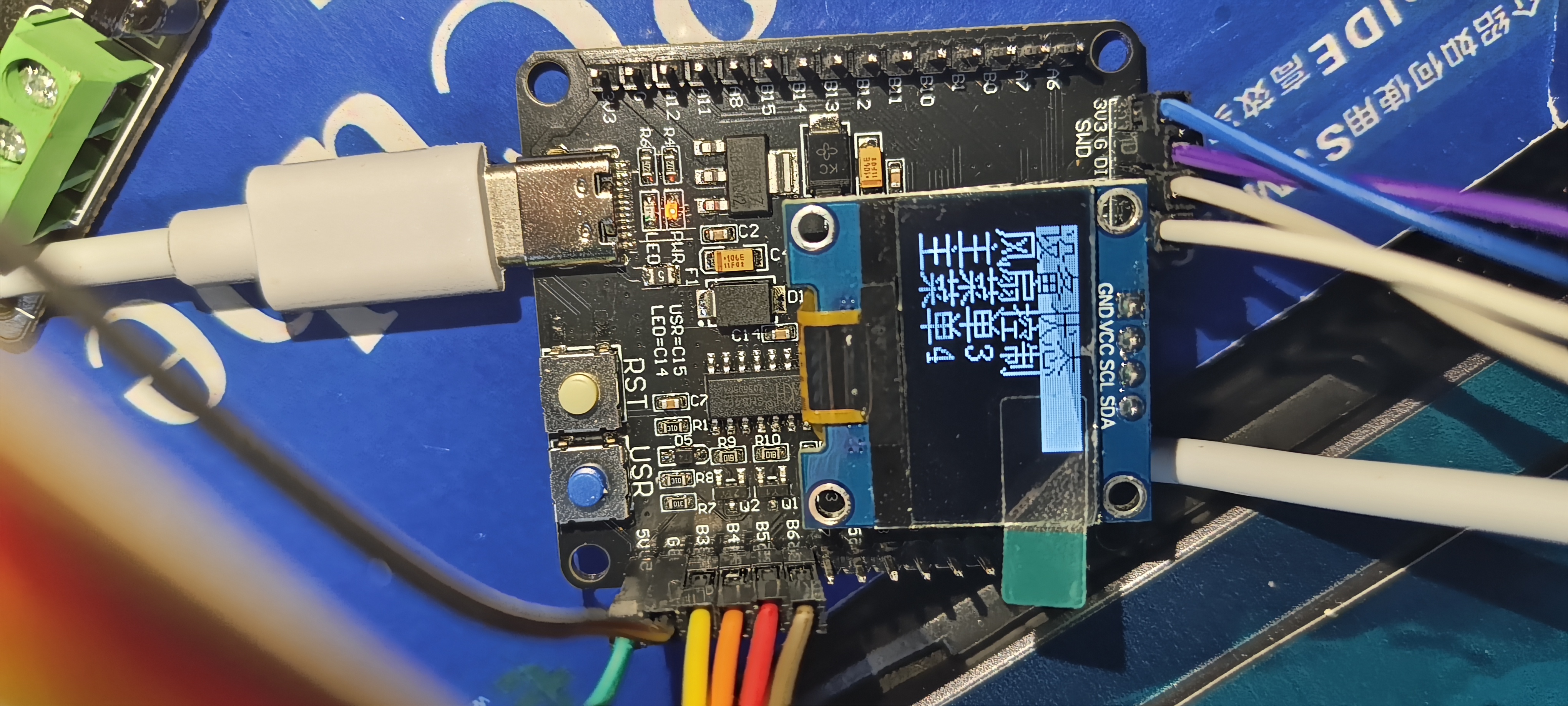
基于江科大stm32屏幕驱动,实现OLED多级菜单(动画效果),结构体链表实现(独创源码)
引言 在嵌入式系统中,用户界面的设计往往直接影响到用户体验。本文将以STM32微控制器和OLED显示屏为例,介绍如何实现一个多级菜单系统。该系统支持用户通过按键导航菜单,执行相应操作,并提供平滑的滚动动画效果。 本文设计了一个…...