ElasticSearch Java API 操作
1.idea创建Maven项目
2.添加依赖
修改 pom.xml 文件
<dependency><groupId>org.elasticsearch</groupId><artifactId>elasticsearch</artifactId><version>7.8.0</version></dependency><!-- elasticsearch 的客户端 --><dependency><groupId>org.elasticsearch.client</groupId><artifactId>elasticsearch-rest-high-level-client</artifactId><version>7.8.0</version></dependency><!-- elasticsearch 依赖 2.x 的 log4j --><dependency><groupId>org.apache.logging.log4j</groupId><artifactId>log4j-api</artifactId><version>2.8.2</version></dependency><dependency><groupId>org.apache.logging.log4j</groupId><artifactId>log4j-core</artifactId><version>2.8.2</version></dependency><dependency><groupId>com.fasterxml.jackson.core</groupId><artifactId>jackson-databind</artifactId><version>2.9.9</version></dependency><!-- junit 单元测试 --><dependency><groupId>junit</groupId><artifactId>junit</artifactId><version>4.12</version></dependency>
3.客户端对象
创建 ES_test 类:
public class ES_test {public static void main(String[] args) throws IOException {// 创建客户端对象RestHighLevelClient client = new RestHighLevelClient(RestClient.builder(new HttpHost("localhost", 9200, "http")));// 关闭客户端连接client.close();}
}
localhost为 es服务器主机名(windows下部署,所以用localhost就可以)
9200端口为es 通信端口
执行代码:
4.ES api 操作
4.1 索引操作
4.1.1创建索引
/*** 创建索引* @param client 客户端对象* @param indexName 索引名称* @throws IOException*/public static void CreateIndex(RestHighLevelClient client,String indexName) throws IOException {// 创建索引 - 请求对象CreateIndexRequest request = new CreateIndexRequest(indexName);// 发送请求,获取响应CreateIndexResponse response = client.indices().create(request,RequestOptions.DEFAULT);boolean acknowledged = response.isAcknowledged();// 响应状态System.out.println("操作状态 = " + acknowledged);}
4.1.2 查看索引
/**** @param client 客户端对象* @param indexName 索引名称* @throws IOException*/public static void GetIndex(RestHighLevelClient client,String indexName) throws IOException {// 查询索引 - 请求对象GetIndexRequest request = new GetIndexRequest(indexName);// 发送请求,获取响应GetIndexResponse response = client.indices().get(request,RequestOptions.DEFAULT);System.out.println("aliases:"+response.getAliases());System.out.println("mappings:"+response.getMappings());System.out.println("settings:"+response.getSettings());}
4.1.3 删除索引
/**** @param client 客户端对象* @param indexName 索引名称* @throws IOException*/public static void DeleteIndex(RestHighLevelClient client,String indexName) throws IOException {// 删除索引 - 请求对象DeleteIndexRequest request = new DeleteIndexRequest(indexName);// 发送请求,获取响应AcknowledgedResponse response = client.indices().delete(request,RequestOptions.DEFAULT);// 操作结果System.out.println("操作结果 : " + response.isAcknowledged());}
4.2 文档操作
创建User 类
/*** @author MR.Liu* @version 1.0* @data 2023-07-19 15:28*/
public class User {private String name;private Integer age;private String sex;public String getName() {return name;}public void setName(String name) {this.name = name;}public Integer getAge() {return age;}public void setAge(Integer age) {this.age = age;}public String getSex() {return sex;}public void setSex(String sex) {this.sex = sex;}public User(String name, Integer age, String sex) {this.name = name;this.age = age;this.sex = sex;}public User() {}
}
4.2.1 新增文档
public static void InsertDoc(RestHighLevelClient client,String indexName,User user,String id) throws IOException {// 新增文档 - 请求对象IndexRequest request = new IndexRequest();// 设置索引及唯一性标识request.index(indexName).id(id);ObjectMapper objectMapper = new ObjectMapper();String productJson = objectMapper.writeValueAsString(user);// 添加文档数据,数据格式为 JSON 格式request.source(productJson, XContentType.JSON);// 客户端发送请求,获取响应对象IndexResponse response = client.index(request, RequestOptions.DEFAULT);3.打印结果信息System.out.println("_index:" + response.getIndex());System.out.println("_id:" + response.getId());System.out.println("_result:" + response.getResult());}
4.2.2 修改文档
public static void UpdateDoc(RestHighLevelClient client,String indexName,String id,String field,Object value) throws IOException {// 修改文档 - 请求对象UpdateRequest request = new UpdateRequest();// 配置修改参数request.index(indexName).id(id);// 设置请求体,对数据进行修改request.doc(XContentType.JSON, field, value);// 客户端发送请求,获取响应对象UpdateResponse response = client.update(request, RequestOptions.DEFAULT);System.out.println("_index:" + response.getIndex());System.out.println("_id:" + response.getId());System.out.println("_result:" + response.getResult());}
4.2.3 删除文档
public static void DeleteDoc(RestHighLevelClient client,String indexName,String id) throws IOException {//创建请求对象DeleteRequest request = new DeleteRequest().index(indexName).id(id);//客户端发送请求,获取响应对象DeleteResponse response = client.delete(request, RequestOptions.DEFAULT);//打印信息System.out.println(response.toString());}
4.2.4 批量新增文档
public static void ListInsertDoc(RestHighLevelClient client,String indexName) throws IOException {//创建批量新增请求对象BulkRequest request = new BulkRequest();request.add(new IndexRequest().index(indexName).id("10001").source(XContentType.JSON, "name","zhangsan"));request.add(new IndexRequest().index(indexName).id("10002").source(XContentType.JSON, "name","lisi"));request.add(new IndexRequest().index(indexName).id("10003").source(XContentType.JSON, "name","wangwu"));//客户端发送请求,获取响应对象BulkResponse responses = client.bulk(request, RequestOptions.DEFAULT);//打印结果信息System.out.println("took:" + responses.getTook());System.out.println("items:" + responses.getItems());}
4.2.5 批量删除文档
public static void ListDeleteDoc(RestHighLevelClient client,String indexName,String ... id) throws IOException {//创建批量删除请求对象BulkRequest request = new BulkRequest();for (int i=0;i<id.length;i++){System.out.println(id[i]);request.add(new DeleteRequest().index(indexName).id(id[i]));}//客户端发送请求,获取响应对象BulkResponse responses = client.bulk(request, RequestOptions.DEFAULT);//打印结果信息System.out.println("took:" + responses.getTook());System.out.println("items:" + responses.getItems());}
4.3 高级查询
4.3.1 请求体查询
4.3.1.1 查询索引中所有文档
public static void SelectIndexAllDoc(RestHighLevelClient client,String indexName) throws IOException {// 创建搜索请求对象SearchRequest request = new SearchRequest();request.indices(indexName);// 构建查询的请求体SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();// 查询所有数据sourceBuilder.query(QueryBuilders.matchAllQuery());request.source(sourceBuilder);SearchResponse response = client.search(request, RequestOptions.DEFAULT);// 查询匹配SearchHits hits = response.getHits();System.out.println("took:" + response.getTook());System.out.println("timeout:" + response.isTimedOut());System.out.println("total:" + hits.getTotalHits());System.out.println("MaxScore:" + hits.getMaxScore());System.out.println("hits========>>");for (SearchHit hit : hits) {//输出每条查询的结果信息System.out.println(hit.getSourceAsString());}System.out.println("<<========");}
4.3.1.2 term查询关键字
public static void TramSelectByKeyWords(RestHighLevelClient client,String indexName) throws IOException {// 创建搜索请求对象SearchRequest request = new SearchRequest();request.indices("student");// 构建查询的请求体SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();sourceBuilder.query(QueryBuilders.termQuery("age", "30"));request.source(sourceBuilder);SearchResponse response = client.search(request, RequestOptions.DEFAULT);// 查询匹配SearchHits hits = response.getHits();System.out.println("took:" + response.getTook());System.out.println("timeout:" + response.isTimedOut());System.out.println("total:" + hits.getTotalHits());System.out.println("MaxScore:" + hits.getMaxScore());System.out.println("hits========>>");for (SearchHit hit : hits) {//输出每条查询的结果信息System.out.println(hit.getSourceAsString());}System.out.println("<<========");}
4.3.1.3分页查询
public static void Paging(RestHighLevelClient client,String indexName) throws IOException {// 创建搜索请求对象SearchRequest request = new SearchRequest();request.indices("student");// 构建查询的请求体SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();sourceBuilder.query(QueryBuilders.matchAllQuery());// 分页查询// 当前页其实索引(第一条数据的顺序号),fromsourceBuilder.from(0);// 每页显示多少条 sizesourceBuilder.size(2);request.source(sourceBuilder);SearchResponse response = client.search(request, RequestOptions.DEFAULT);// 查询匹配SearchHits hits = response.getHits();System.out.println("took:" + response.getTook());System.out.println("timeout:" + response.isTimedOut());System.out.println("total:" + hits.getTotalHits());System.out.println("MaxScore:" + hits.getMaxScore());System.out.println("hits========>>");for (SearchHit hit : hits) {//输出每条查询的结果信息System.out.println(hit.getSourceAsString());}System.out.println("<<========");}
4.3.1.4排序查询
public static void SortOrderAll(RestHighLevelClient client,String indexName) throws IOException {// 创建搜索请求对象SearchRequest request = new SearchRequest();request.indices("student");// 构建查询的请求体SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();sourceBuilder.query(QueryBuilders.matchAllQuery());// 排序sourceBuilder.sort("age", SortOrder.ASC);request.source(sourceBuilder);SearchResponse response = client.search(request, RequestOptions.DEFAULT);// 查询匹配SearchHits hits = response.getHits();System.out.println("took:" + response.getTook());System.out.println("timeout:" + response.isTimedOut());System.out.println("total:" + hits.getTotalHits());System.out.println("MaxScore:" + hits.getMaxScore());System.out.println("hits========>>");for (SearchHit hit : hits) {//输出每条查询的结果信息System.out.println(hit.getSourceAsString());}System.out.println("<<========");}
4.3.1.5 过滤字段
public static void FilterByColumn(RestHighLevelClient client,String indexName) throws IOException {// 创建搜索请求对象SearchRequest request = new SearchRequest();request.indices("student");// 构建查询的请求体SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();sourceBuilder.query(QueryBuilders.matchAllQuery());//查询字段过滤String[] excludes = {};String[] includes = {"name", "age"};sourceBuilder.fetchSource(includes, excludes);request.source(sourceBuilder);SearchResponse response = client.search(request, RequestOptions.DEFAULT);// 查询匹配SearchHits hits = response.getHits();System.out.println("took:" + response.getTook());System.out.println("timeout:" + response.isTimedOut());System.out.println("total:" + hits.getTotalHits());System.out.println("MaxScore:" + hits.getMaxScore());System.out.println("hits========>>");for (SearchHit hit : hits) {//输出每条查询的结果信息System.out.println(hit.getSourceAsString());}System.out.println("<<========");}
4.3.1.6 Bool查询
public static void boolSelect(RestHighLevelClient client,String indexName) throws IOException {// 创建搜索请求对象SearchRequest request = new SearchRequest();request.indices("student");// 构建查询的请求体SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();BoolQueryBuilder boolQueryBuilder = QueryBuilders.boolQuery();// 必须包含boolQueryBuilder.must(QueryBuilders.matchQuery("age", "30"));// 一定不含boolQueryBuilder.mustNot(QueryBuilders.matchQuery("name", "zhangsan"));// 可能包含boolQueryBuilder.should(QueryBuilders.matchQuery("sex", "男"));sourceBuilder.query(boolQueryBuilder);request.source(sourceBuilder);SearchResponse response = client.search(request, RequestOptions.DEFAULT);// 查询匹配SearchHits hits = response.getHits();System.out.println("took:" + response.getTook());System.out.println("timeout:" + response.isTimedOut());System.out.println("total:" + hits.getTotalHits());System.out.println("MaxScore:" + hits.getMaxScore());System.out.println("hits========>>");for (SearchHit hit : hits) {//输出每条查询的结果信息System.out.println(hit.getSourceAsString());}System.out.println("<<========");}
4.3.1.7 范围查询
public static void RangeSelect(RestHighLevelClient client,String indexName) throws IOException {// 创建搜索请求对象SearchRequest request = new SearchRequest();request.indices("student");// 构建查询的请求体SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();RangeQueryBuilder rangeQuery = QueryBuilders.rangeQuery("age");// 大于等于rangeQuery.gte("30");// 小于等于rangeQuery.lte("40");sourceBuilder.query(rangeQuery);request.source(sourceBuilder);SearchResponse response = client.search(request, RequestOptions.DEFAULT);// 查询匹配SearchHits hits = response.getHits();System.out.println("took:" + response.getTook());System.out.println("timeout:" + response.isTimedOut());System.out.println("total:" + hits.getTotalHits());System.out.println("MaxScore:" + hits.getMaxScore());System.out.println("hits========>>");for (SearchHit hit : hits) {//输出每条查询的结果信息System.out.println(hit.getSourceAsString());}System.out.println("<<========");}
4.3.1.8 模糊查询
public static void VagueSelect(RestHighLevelClient client,String indexName) throws IOException {// 创建搜索请求对象SearchRequest request = new SearchRequest();request.indices("student");// 构建查询的请求体SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();sourceBuilder.query(QueryBuilders.fuzzyQuery("name","zhangsan").fuzziness(Fuzziness.ONE));request.source(sourceBuilder);SearchResponse response = client.search(request, RequestOptions.DEFAULT);// 查询匹配SearchHits hits = response.getHits();System.out.println("took:" + response.getTook());System.out.println("timeout:" + response.isTimedOut());System.out.println("total:" + hits.getTotalHits());System.out.println("MaxScore:" + hits.getMaxScore());System.out.println("hits========>>");for (SearchHit hit : hits) {//输出每条查询的结果信息System.out.println(hit.getSourceAsString());}System.out.println("<<========");}
4.3.2 高亮查询
public static void HighlightSelect(RestHighLevelClient client,String indexName) throws IOException {// 高亮查询SearchRequest request = new SearchRequest().indices("student");//2.创建查询请求体构建器SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();//构建查询方式:高亮查询TermsQueryBuilder termsQueryBuilder = QueryBuilders.termsQuery("name","zhangsan");//设置查询方式sourceBuilder.query(termsQueryBuilder);//构建高亮字段HighlightBuilder highlightBuilder = new HighlightBuilder();highlightBuilder.preTags("<font color='red'>");//设置标签前缀highlightBuilder.postTags("</font>");//设置标签后缀highlightBuilder.field("name");//设置高亮字段//设置高亮构建对象sourceBuilder.highlighter(highlightBuilder);//设置请求体request.source(sourceBuilder);//3.客户端发送请求,获取响应对象SearchResponse response = client.search(request, RequestOptions.DEFAULT);//4.打印响应结果SearchHits hits = response.getHits();System.out.println("took::"+response.getTook());System.out.println("time_out::"+response.isTimedOut());System.out.println("total::"+hits.getTotalHits());System.out.println("max_score::"+hits.getMaxScore());System.out.println("hits::::>>");for (SearchHit hit : hits) {String sourceAsString = hit.getSourceAsString();System.out.println(sourceAsString);//打印高亮结果Map<String, HighlightField> highlightFields = hit.getHighlightFields();System.out.println(highlightFields);}System.out.println("<<::::");}
4.3.3 聚合函数
4.3.3.1 最大值查询
public static void MaxSelect(RestHighLevelClient client,String indexName) throws IOException {// 高亮查询SearchRequest request = new SearchRequest().indices("student");SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();sourceBuilder.aggregation(AggregationBuilders.max("maxAge").field("age"));//设置请求体request.source(sourceBuilder);//3.客户端发送请求,获取响应对象SearchResponse response = client.search(request, RequestOptions.DEFAULT);//4.打印响应结果SearchHits hits = response.getHits();System.out.println(response);}
4.3.3.2 分组查询
public static void GroupSelect(RestHighLevelClient client,String indexName) throws IOException {// 高亮查询SearchRequest request = new SearchRequest().indices("student");SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();sourceBuilder.aggregation(AggregationBuilders.terms("age_groupby").field("age"));//设置请求体request.source(sourceBuilder);//3.客户端发送请求,获取响应对象SearchResponse response = client.search(request, RequestOptions.DEFAULT);//4.打印响应结果SearchHits hits = response.getHits();System.out.println(response);}
相关文章:
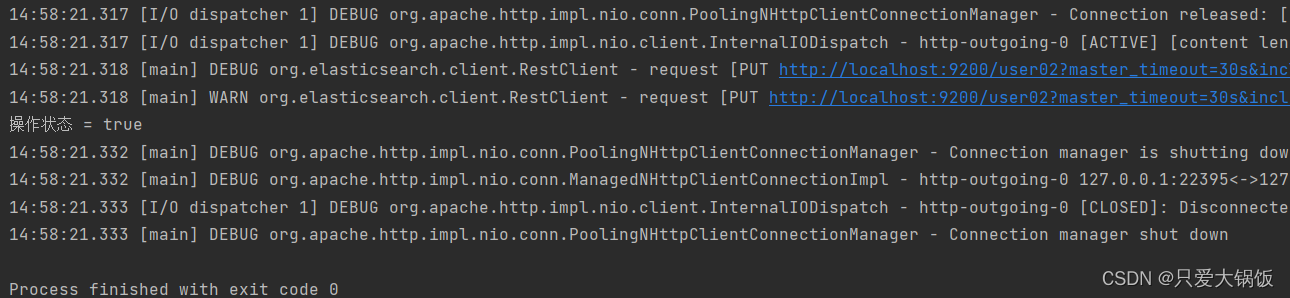
ElasticSearch Java API 操作
1.idea创建Maven项目 2.添加依赖 修改 pom.xml 文件 <dependency><groupId>org.elasticsearch</groupId><artifactId>elasticsearch</artifactId><version>7.8.0</version></dependency><!-- elasticsearch 的客户端 --…...
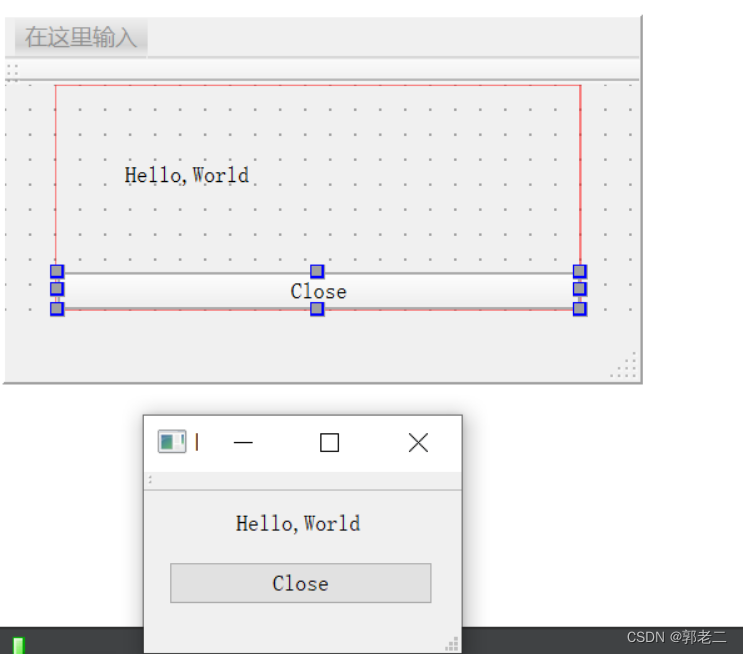
【Qt】QML-01:使用QtCreator10创建QML工程,并讲解第一个程序:Hello World
1、创建QML工程 1)新建工程 打开QtCreator10,依次点击“Create Project” --> “Application(Qt)” --> “Qt Quick Application(compat)” 注意:本人打算使用Qt5.15.2创建工程,而非Qt6,因此选择兼容低于Qt6版本的“Qt Quick Applicat…...
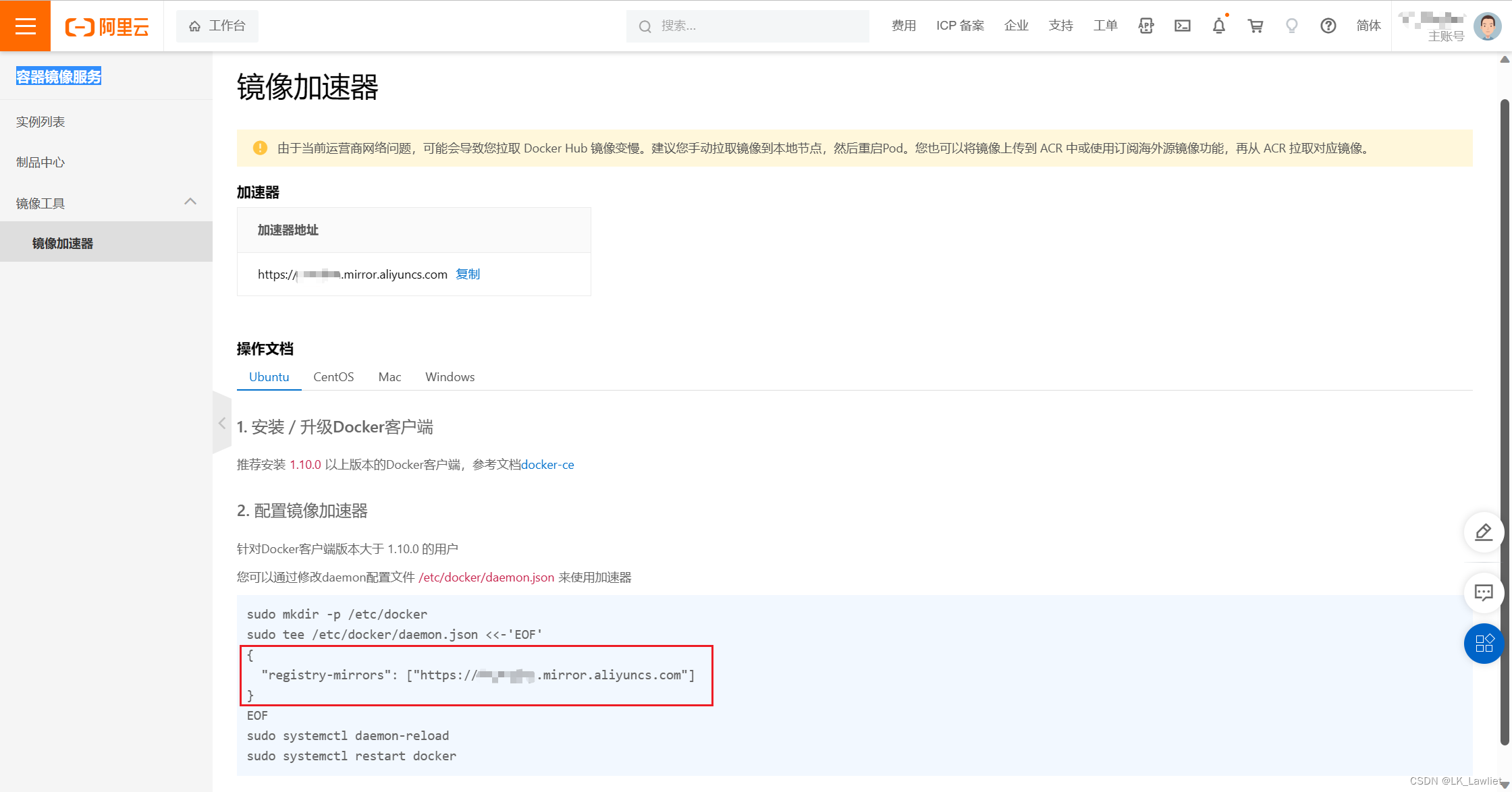
Docker的安装与部署
Docker 基本概念介绍 通俗理解:镜像是类,容器是对象实例 仓库 应用商店、镜像 下载的应用安装程序、容器 应用程序 镜像(Image) 这里面保存了应用和需要的依赖环境 为什么需要多个镜像?当开发、构建和运行容器化应用程序时,我们…...
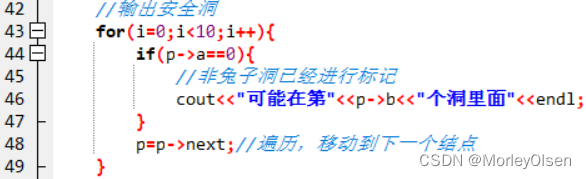
【数据结构】实验四:循环链表
实验四 循环链表 一、实验目的与要求 1)熟悉循环链表的类型定义和基本操作; 2)灵活应用循环链表解决具体应用问题。 二、实验内容 题目一:有n个小孩围成一圈,给他们从1开始依次编号,从编号为1的小孩开…...

【FPGA/D7】
2023年7月26日 串口传图到RAM并TFT显示 视频25note要求:接收两个字节数据合并为一个16位数据并写入ram: FIFO模型与应用场景 视频26 串口传图到RAM并TFT显示 视频25 note 存储器的使用,在开始读写或者结束读写的位置非常容易出现数据错误或…...
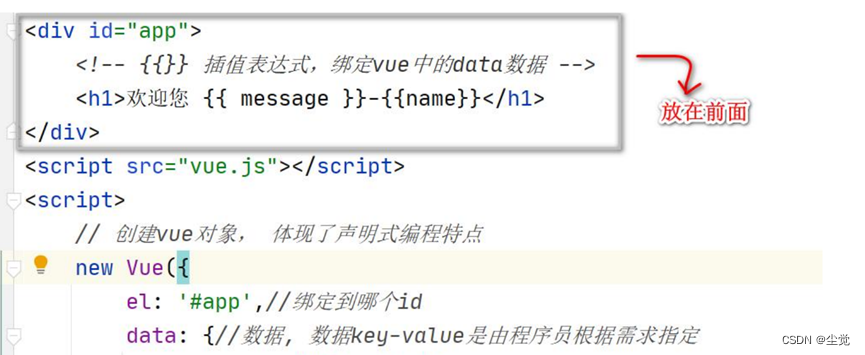
Vue的下载以及MVVM分析
😀前言本片文章是vue系列第一篇整理了vue的基础和发展史 🏠个人主页:尘觉主页 🧑个人简介:大家好,我是尘觉,希望我的文章可以帮助到大家,您的满意是我的动力😉Ƕ…...
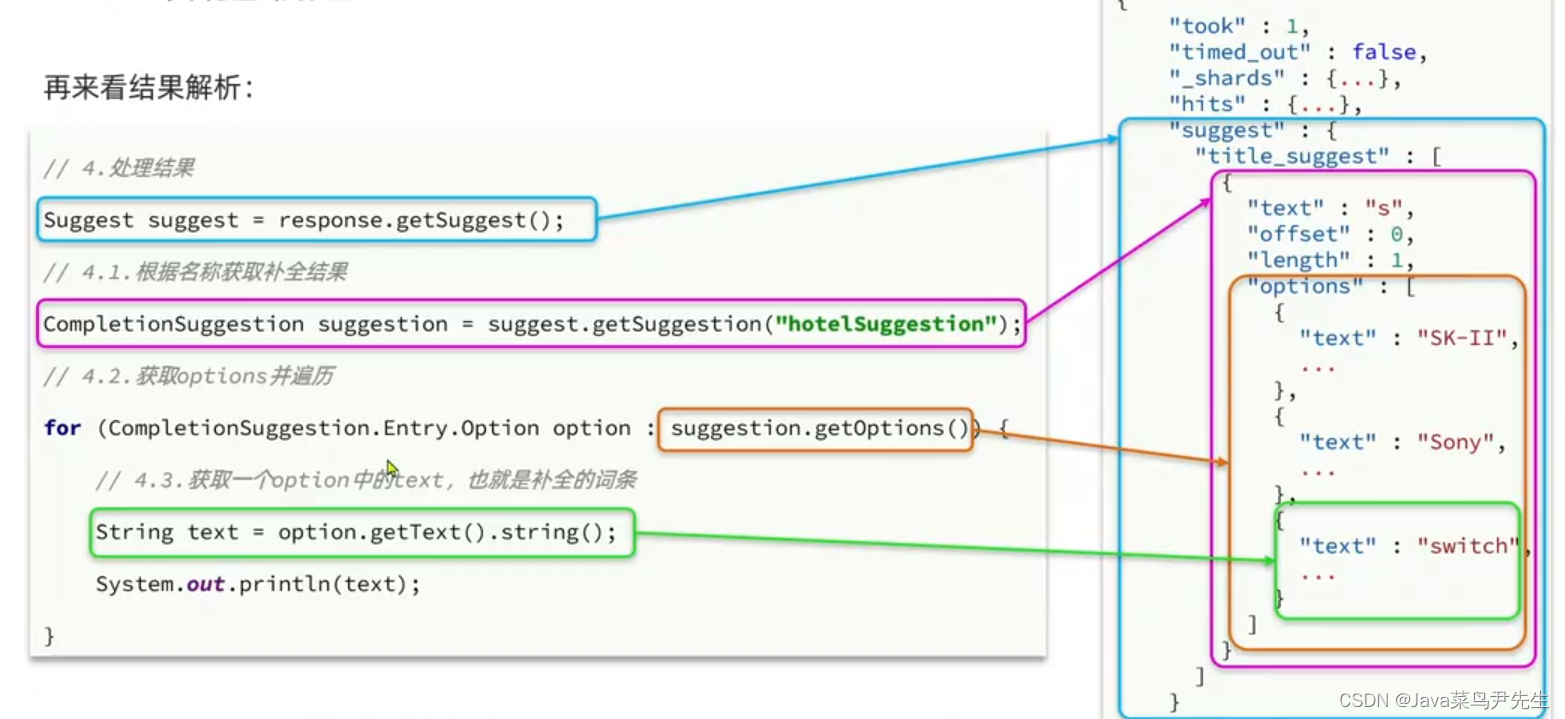
ElasticSearch学习--自动补全
目录 自定义分词器 介绍 配置自定义分词器 拼音分词器的问题编辑 总结 DSL自动补全查询 RestAPI实现自动补全 自定义分词器 介绍 自定义分词器只在当前库中有效 配置自定义分词器 拼音分词器的问题 总结 DSL自动补全查询 RestAPI实现自动补全...
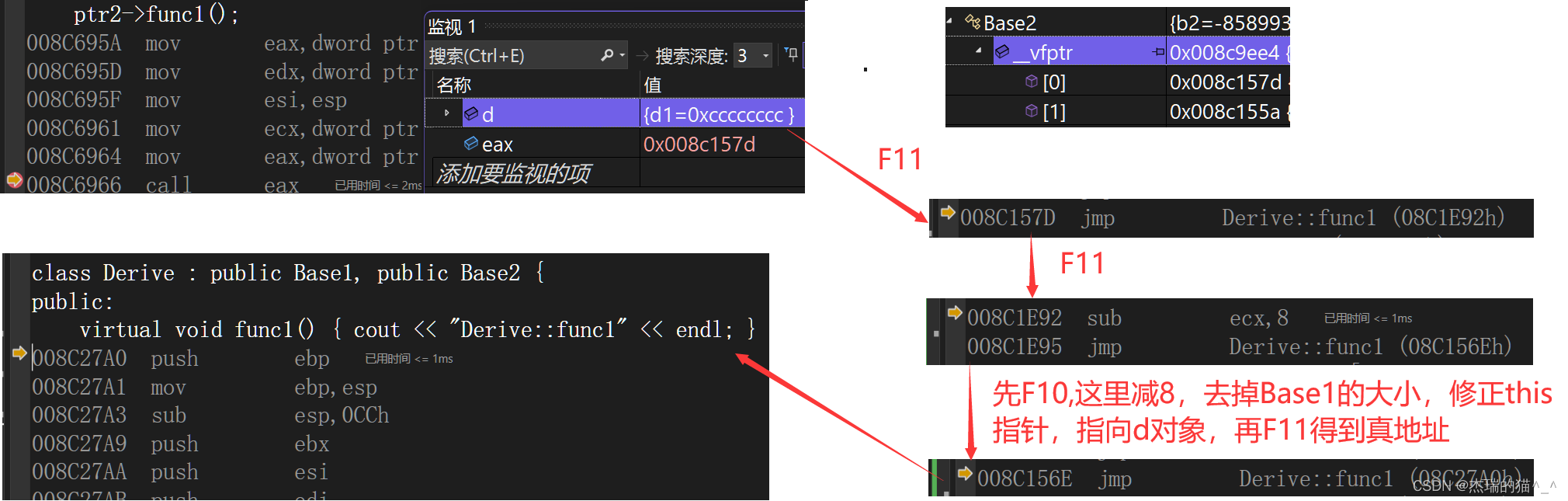
【C++】多态,虚函数表相关问题解决
文章目录 多态概念及其触发条件重写和协变(考点1)(考点2) 虚函数表及其位置(考点3) 多继承中的虚函数表 多态概念及其触发条件 多态的概念:通俗来说,就是多种形态。具体点就是去完成…...
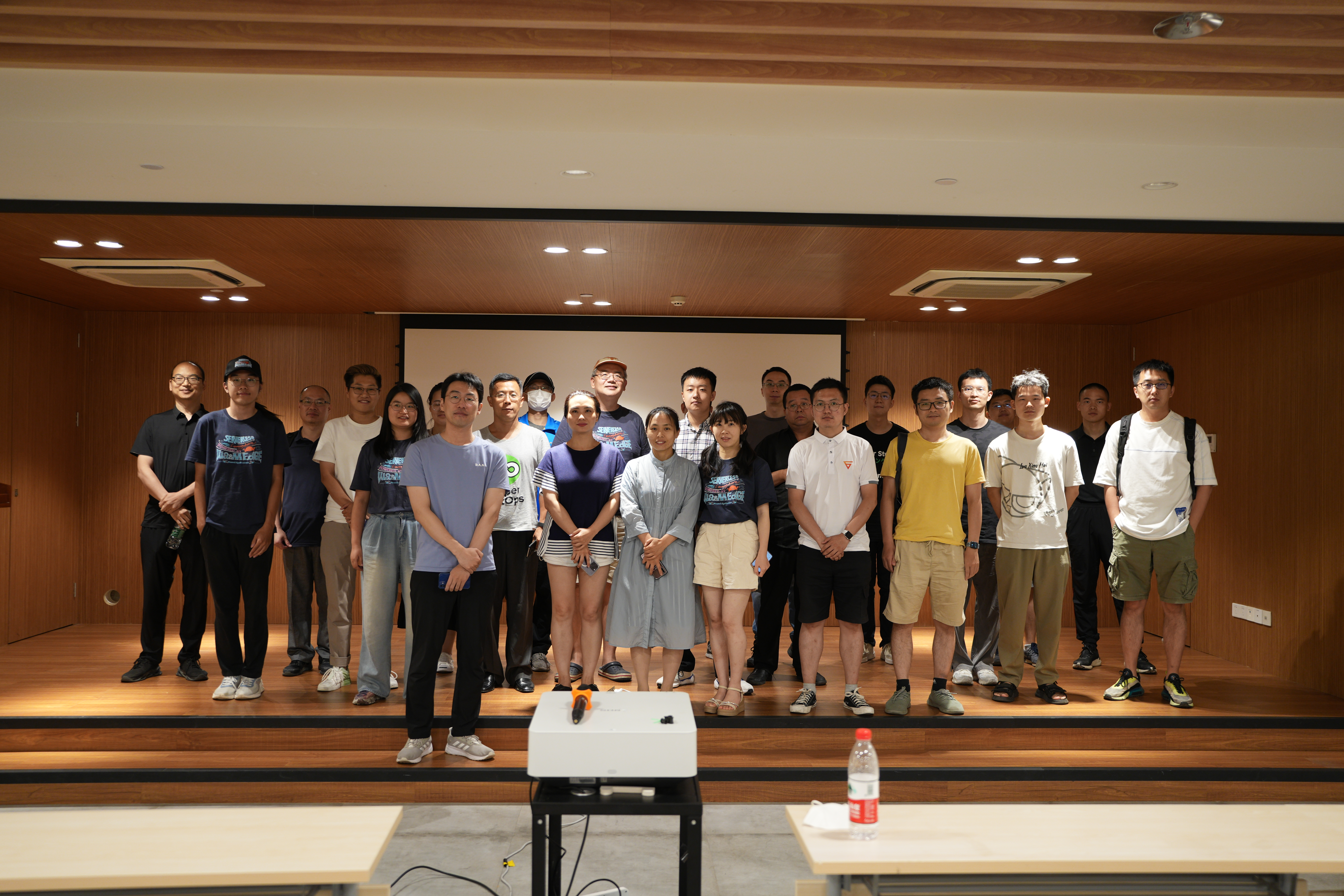
探索大型语言模型的开源人工智能基础设施:北京开源AI Meetup回顾
原文参见Explore open source AI Infra for Large Language Models: Highlights from the Open Source AI Meetup Beijing | Cloud Native Computing Foundation 背景介绍: 最近,在 ChatGPT 的成功推动下,大型语言模型及其应用程序的流行度激…...

Langchain 的 Conversation buffer window memory
Langchain 的 Conversation buffer window memory ConversationBufferWindowMemory 保存一段时间内对话交互的列表。它仅使用最后 K 个交互。这对于保持最近交互的滑动窗口非常有用,因此缓冲区不会变得太大。 我们首先来探讨一下这种存储器的基本功能。 示例代码&…...
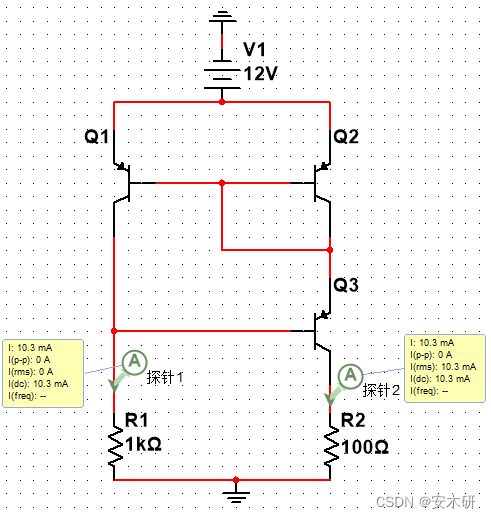
电流源电路
3.3.3电流源电路 镜像电流源 电路 分析 仿真 比例电流源 电路 分析 仿真 加射极输出器的电流源1 电路 分析 仿真 加射极输出器的电流源2 电路 分析 仿真 威尔逊电流源 电路 分析 仿真...

iOS开发-CMMotionManager传感器陀螺仪
iOS开发-CMMotionManager传感器陀螺仪 之前开发中遇到需要使用陀螺仪判断是否拍照时候水平判断,如果没有水平拍照,则给出提示。方便用户拍照合适的题目图片。 一、CMMotionManager CMMotionManager是什么 CMMotionManager 是 Core Motion 库的核心类&…...
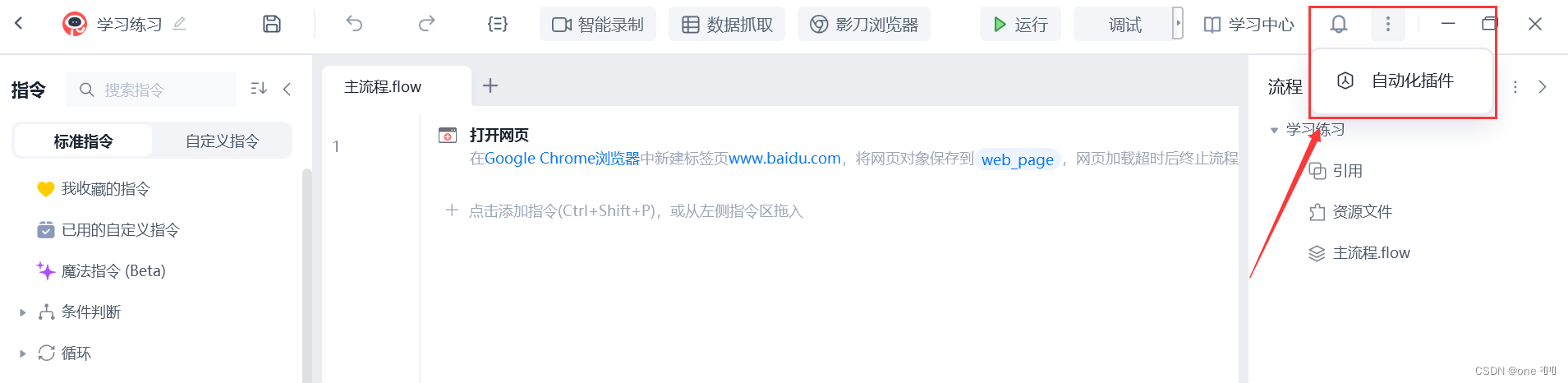
影刀下载,插件安装
1、下载 在影刀官网下载:www.yingdao.com 2、谷歌插件安装 参考: 影刀插件安装各种方式 浏览器安装插件说明 - 影刀帮助中心 安装说明:驱动外置 Chrome 需要安装插件,并且保证此插件处于开启状态 方式一:用户头…...

Linux的tcpdump命令详解
tcpdump 一款sniffer工具,是Linux上的抓包工具,嗅探器 补充说明 tcpdump命令 是一款抓包,嗅探器工具,它可以打印所有经过网络接口的数据包的头信息,也可以使用-w选项将数据包保存到文件中,方便以后分析。…...
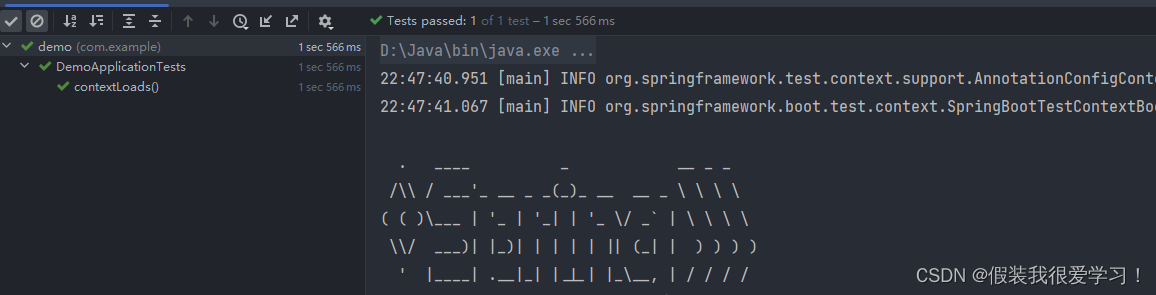
springboot运行报错Failed to load ApplicationContext for xxx
Failed to load ApplicationContext for报错解决方法 报错Failed to load ApplicationContext for 报错Failed to load ApplicationContext for 网上找了一堆方法都尝试了还是没用 包括添加mapperScan,添加配置类 配置pom文件 [外链图片转存失败,源站可能有防盗链机…...

[SQL挖掘机] - 内连接: inner join
介绍: 内连接是一种多表连接方式,用于将两个或多个表中的数据通过共同的列值进行匹配,并返回满足连接条件的匹配行。简单来说,内连接能够将相关联的数据组合在一起,以便进行更复杂和全面的数据分析。 内连接的工作原理如下&…...
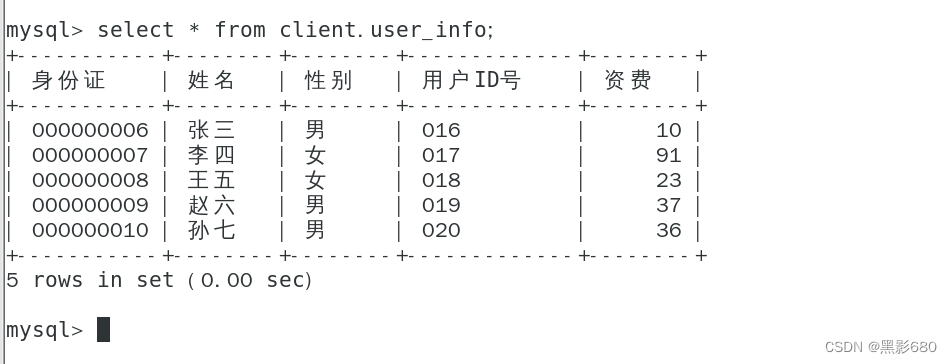
mysql(四)数据备份
目录 前言 一、概述 二、备份的类型 (一)物理与逻辑角度 (二)数据库备份策略角度 三、常见的备份方法 四、完整备份 (一)打包数据库文件备份 (二)备份工具备份 五、增量备份 六、操…...
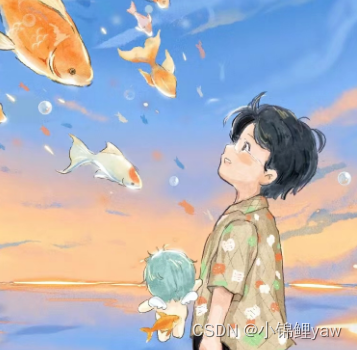
Spring 拦截器
上篇博客链接:SpringAOP详解 上篇博客我们提到使用AOP的环绕通知来完成统一的用户登陆验证虽然方便了许多,但随之而来也带来了新的问题: HttpSession不知道如何去获取,获取困难登录和注册的方法并不需要拦截,使用切点没办法定义哪…...

【libevent】http客户端3:简单封装
LibEventHttp: 适用于简单的http请求 LibEventHttp/* Copyright (c) MediaArea.net SARL. All Rights Reserved.** Use of this source code is governed by a BSD-style license that can* be found in the License.html file in the root of the source tree.*///--------…...
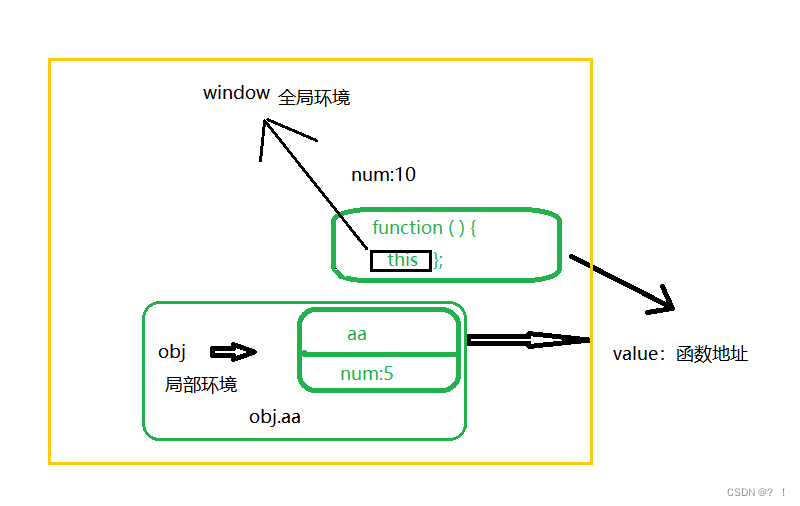
JavaScript的函数中this的指向
JavaScript的函数中this的指向 JavaScript 语言之所以有 this 的设计,跟内存里面的数据结构有关系。 以下例子来简单描述this在不同情况下所指向的对象。 var obj {aa: function(){console.log(this.num)},num: 5 };var aa obj.aa; var num 10;obj.aa(); // …...

Caddy 中实现自动 HTTPS
要在 Caddy 中实现自动 HTTPS,您可以按照以下步骤进行操作: 步骤 1:安装 Caddy 首先,您需要安装 Caddy 服务器。您可以从 Caddy 的官方网站(https://caddyserver.com/)下载适用于您的操作系统的最新版本。…...

SK5代理(socks5代理)在网络安全与爬虫应用中的优势与编写指南
一、SK5代理(socks5代理)的基本概念 SK5代理是一种网络代理协议,它允许客户端通过代理服务器与目标服务器进行通信。相较于HTTP代理,SK5代理在传输数据时更加高效且安全,它支持TCP和UDP协议,并且能够实现数…...
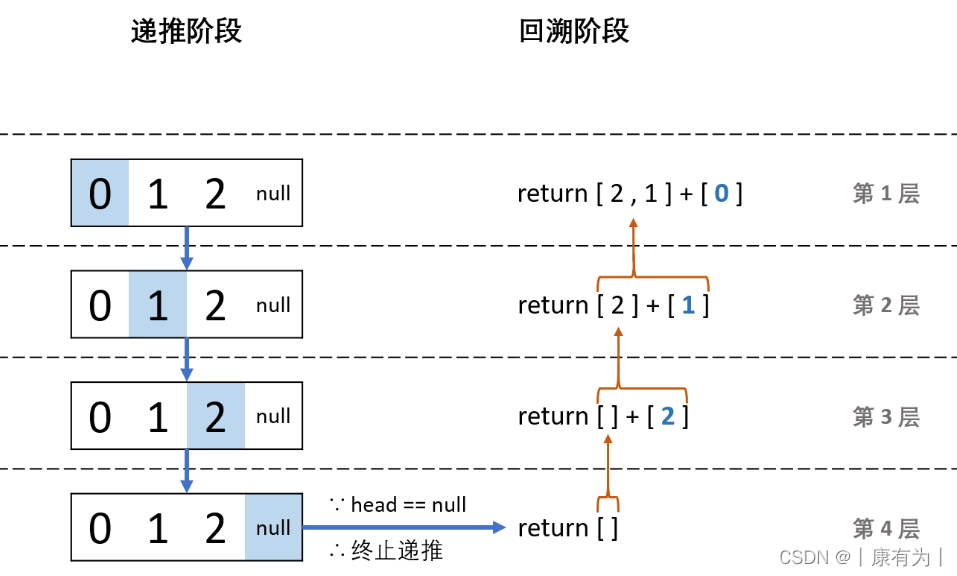
【LeetCode-简单】剑指 Offer 06. 从尾到头打印链表(详解)
题目 输入一个链表的头节点,从尾到头反过来返回每个节点的值(用数组返回)。 题目地址:剑指 Offer 06. 从尾到头打印链表 - 力扣(LeetCode) 方法1:栈 思路 题目要求我们将链表的从尾到投打印一…...
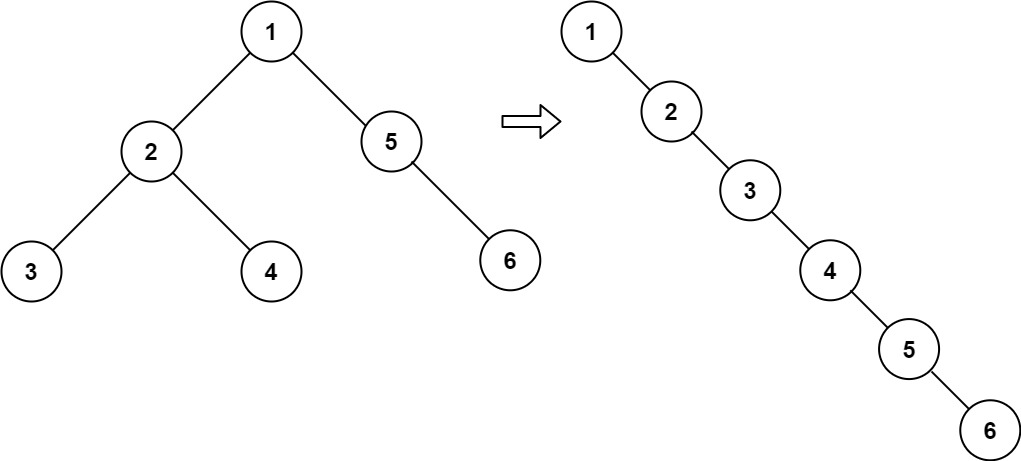
【LeetCode】114.二叉树展开为链表
题目 给你二叉树的根结点 root ,请你将它展开为一个单链表: 展开后的单链表应该同样使用 TreeNode ,其中 right 子指针指向链表中下一个结点,而左子指针始终为 null 。展开后的单链表应该与二叉树 先序遍历 顺序相同。 示例 1&…...
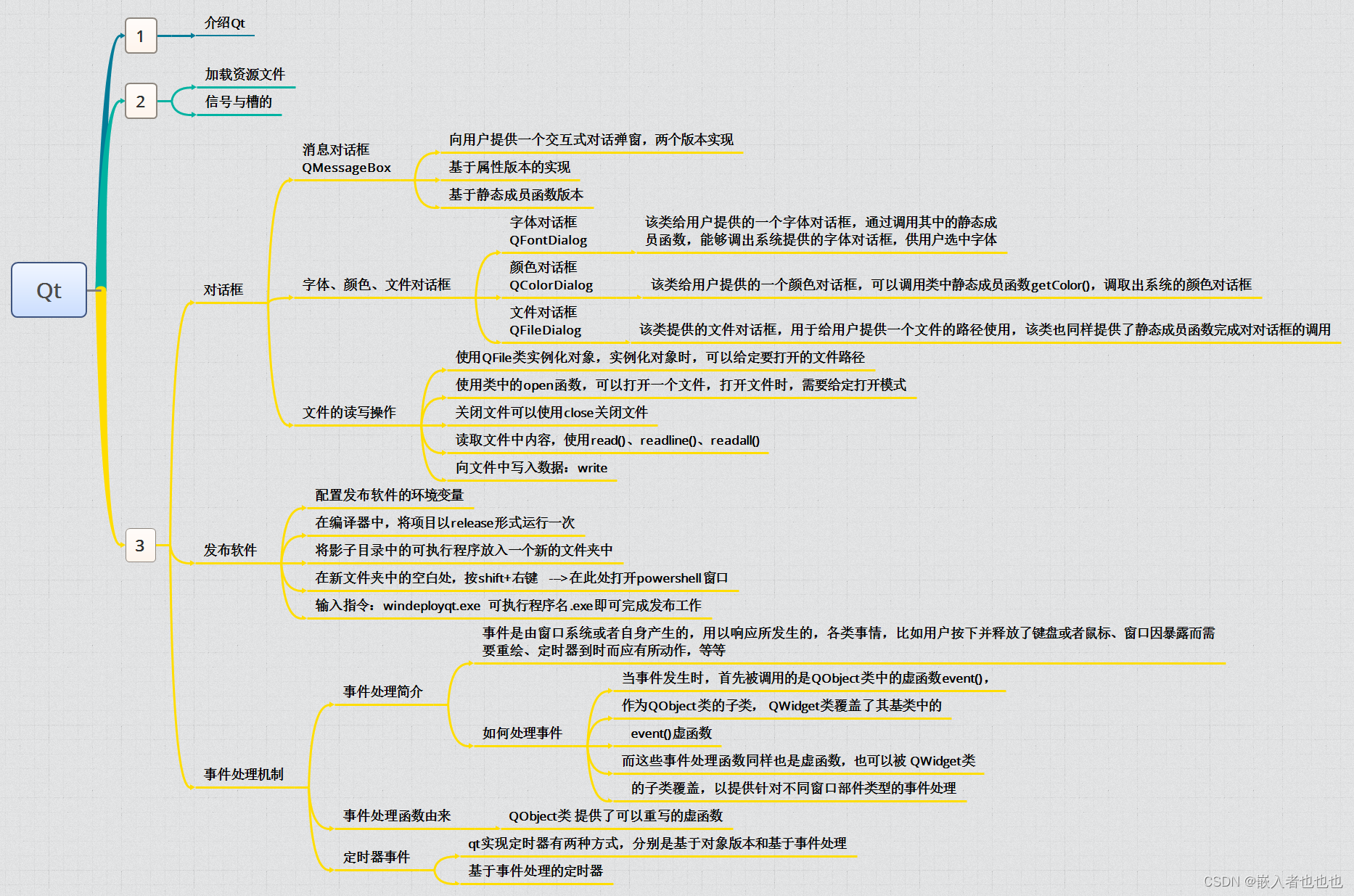
DAY3,Qt(完成闹钟的实现,定时器事件处理函数的使用)
1.完成闹钟的实现,到点播报文本框的内容; ---alarm.h---头文件 #ifndef ALARM_H #define ALARM_H#include <QWidget> #include <QTimerEvent> //定时器处理函数类 #include <QTime> //时间类 #include <QPushButton> //按钮…...

TL-ER3220G设置vlan
TL-ER3220G是企业宽带路由器。 自带5个RJ45接口。 其中接口1到接口4都可以接入宽带线路。最多可以并接4路。 本例由接口1接入宽带,默认接口2到接口4组成1个vlan,名称vlan。其中接口5特殊,带宽最大100M。 计划将接口2和接口4组成第一个vlan&…...

PHPWord 实现合并多个word文件
PHPWord 本来想着当调包侠呢,结果翻了一遍文档,没有这种操作支持,阿这😂 GPT 不出意外的一顿胡扯,给👨🦳气的要中风啦 思路 word 也就是docx结尾的文件本质上就是xml字符串, …...

rust持续学习Box::leak
Box就是unique_ptr 这个函数的功能是消费box返回一个全局变量! 写一个函数,想要真的返回全局变量,感觉用这个是个好的做法 fn Foo()->Option<&static mut A> {let a Box::new(A());Some(Box::leak(a)) }这样就能当真拿到这个全…...

通过SSH实现将本地端口反向代理到公网服务器
使用场景 有一台公网服务器,能够对外开放服务进行访问,但是这个公网服务器资源较低,无法运行太多服务 有一台闲置电脑可以全天候开机使用,且配置较好,可以部署多个服务,但是没有公网IP 需求:将…...
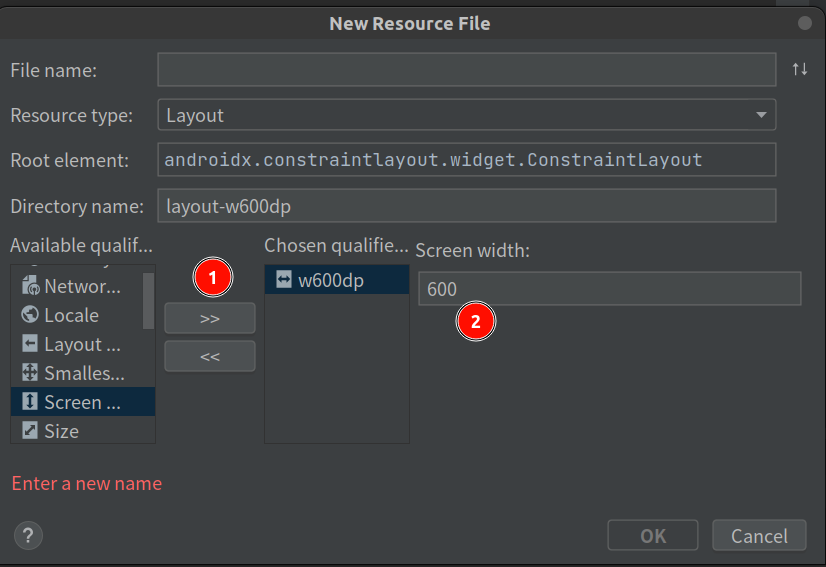
Fragment的基本用法、Fragment和活动间的通信、Fragment的生命周期、动态加载布局的技巧
一、Fragment的简单用法 1、制作Fragment 1.1 新建一个布局文件left_fragment.xml <?xml version"1.0" encoding"utf-8"?> <LinearLayout xmlns:android"http://schemas.android.com/apk/res/android"android:orientation"ve…...