将整数,结构体,结构体数组,链表写到文件
在之前的学习中,忘文件中写的内容都是字符串或字符,本节学习如何写入其他各种类型的数据。
回看write和read函数的形式:
ssize_t write(int fd, const void *buf, size_t count);
ssize_t read(int fd, void *buf, size_t count);
其中,第二个参数都是一个无类型的指针,只不过之前一直将这里定义为一个字符串,其实,这个指针可以指向各种形式数据的地址。
写入一个整数
demo7.c:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>int main()
{int fd; // file descriptionint data = 100;int data2 = 0;fd = open("./file1",O_RDWR|O_CREAT|O_TRUNC, 0600);printf("file description = %d, open successfully!\n",fd);write(fd, &data, sizeof(int));lseek(fd,0,SEEK_SET);read(fd, &data2, sizeof(int));printf("context:%d\n",data2);close(fd); //close after writing return 0;
}
运行代码:
注意,如果此时打开file1:(此时需要使用vi打开)
发现是乱码,但是这并不影响程序运行时的读取和写入。
写入一个结构体
demo7.c:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>struct Test
{int i;char c;
};int main()
{int fd; // file descriptionstruct Test data = {30,'k'};struct Test data2;fd = open("./file1",O_RDWR|O_CREAT|O_TRUNC, 0600);printf("file description = %d, open successfully!\n",fd);write(fd, &data, sizeof(struct Test));lseek(fd,0,SEEK_SET);read(fd, &data2, sizeof(struct Test));printf("data.i:%d,data.c=%c\n",data2.i,data2.c);close(fd); //close after writing return 0;
}
运行代码:
写入一个结构体数组
demo7.c:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>struct Test
{int i;char c;
};int main()
{int fd; // file descriptionstruct Test data[2] = {{30,'k'},{100,'p'}};struct Test data2[2];fd = open("./file1",O_RDWR|O_CREAT|O_TRUNC, 0600);printf("file description = %d, open successfully!\n",fd);write(fd, &data, sizeof(struct Test)*2);lseek(fd,0,SEEK_SET);read(fd, &data2, sizeof(struct Test)*2);printf("data[0].i:%d,data[0].c=%c\n",data2[0].i,data2[0].c);printf("data[1].i:%d,data[1].c=%c\n",data2[1].i,data2[1].c);close(fd); //close after writing return 0;
}
运行代码:
写入一个链表
demo7.c:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>struct Test
{int data;struct Test *next;
};int main()
{int fd; // file descriptionstruct Test head = {20,NULL};struct Test node1 = {30,NULL};struct Test node2 = {40,NULL};head.next = &node1;node1.next = &node2;fd = open("./file1",O_RDWR|O_CREAT|O_TRUNC, 0600);printf("file description = %d, open successfully!\n",fd);write(fd, &head, sizeof(struct Test));write(fd, &node1, sizeof(struct Test));write(fd, &node2, sizeof(struct Test));lseek(fd,0,SEEK_SET);struct Test node_read_1 = {0,NULL};struct Test node_read_2 = {0,NULL};struct Test node_read_3 = {0,NULL};read(fd, &node_read_1, sizeof(struct Test)); read(fd, &node_read_2, sizeof(struct Test));read(fd, &node_read_3, sizeof(struct Test));printf("no.1=%d\n",node_read_1.data);printf("no.2=%d\n",node_read_2.data);printf("no.3=%d\n",node_read_3.data);close(fd);return 0;
}
运行代码:
但是以上的代码有点笨,且容错性太低,首先读取和写入应该封装成函数,并且我认为通常在读取链表的时候,不一定知道链表有多少元素,所以应该一边用尾插法动态创建链表一边读取。
改进代码demo8.c:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>struct Test
{int data;struct Test *next;
};struct Test *insertBehind(struct Test *head, struct Test *new)
{struct Test *p = head;if(p == NULL){head = new;return head;}while(p->next != NULL){p = p->next;} //将p先移动到链表的尾部p->next = new;return head;
}void writeLink2File(int fd,struct Test *p){while(p != NULL){write(fd, p, sizeof(struct Test));p = p->next;}
}void readLinkFromFile(int fd,struct Test *head){struct Test *new;int size = lseek(fd,0,SEEK_END); //先计算文件大小lseek(fd,0,SEEK_SET); //然后不要忘记把光标移到文件头while(1){new = (struct Test *)malloc(sizeof(struct Test));read(fd, new, sizeof(struct Test));printf("link data:%d\n",new->data);if(lseek(fd,0,SEEK_CUR) == size){ //每次读取一个数据之后,动态创建下一个链表节点之前,都要判断“当前光标是否已经在文件尾”,如果是,说明读取已经完成printf("read finish\n");break;}head = insertBehind(head,new);}}int main()
{int fd; // file descriptionstruct Test head = {20,NULL};struct Test node1 = {30,NULL};struct Test node2 = {40,NULL};head.next = &node1;node1.next = &node2;fd = open("./file1",O_RDWR|O_CREAT|O_TRUNC, 0600);printf("file description = %d, open successfully!\n",fd);writeLink2File(fd,&head);struct Test *head_recv = NULL; //准备创建一个新的链表用于接收readLinkFromFile(fd,head_recv);close(fd);return 0;
}
改进代码运行:
相关文章:
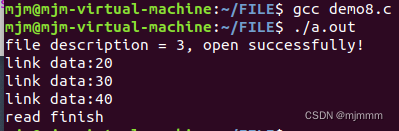
将整数,结构体,结构体数组,链表写到文件
在之前的学习中,忘文件中写的内容都是字符串或字符,本节学习如何写入其他各种类型的数据。 回看write和read函数的形式: ssize_t write(int fd, const void *buf, size_t count); ssize_t read(int fd, void *buf, size_t count); 其中&a…...
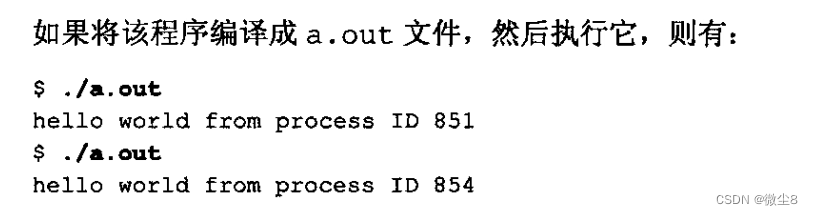
UNIX基础知识:UNIX体系结构、登录、文件和目录、输入和输出、程序和进程、出错处理、用户标识、信号、时间值、系统调用和库函数
引言: 所有的操作系统都为运行在其上的程序提供服务,比如:执行新程序、打开文件、读写文件、分配存储区、获得系统当前时间等等 1. UNIX体系结构 从严格意义上来说,操作系统可被定义为一种软件,它控制计算机硬件资源&…...
IDEA2021.3.1-优化设置IDEA2021.3.1-优化设置、快捷方式改为eclipse、快捷键等
IDEA2021.3.1-优化设置IDEA2021.3.1-优化设置、快捷方式改为eclipse、快捷键等 一、主题设置二、鼠标悬浮提示三、显示方法分隔符四、代码提示忽略大小写五、自动导包六、取消单行显示tabs七、设置字体八、配置类文档注释信息模板九、设置文件编码9.1、所有地方设置为UTF-89.2、…...
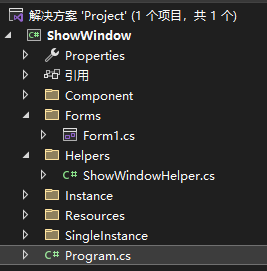
使用C#的窗体显示与隐藏动画效果方案 - 开源研究系列文章
今天继续研究C#的WinForm的显示动画效果。 上次我们实现了无边框窗体的显示动画效果(见博文:基于C#的无边框窗体动画效果的完美解决方案 - 开源研究系列文章 ),这次介绍的是未在任务栏托盘中窗体的显示隐藏动画效果的实现代码。 1、 项目目录;…...
09_Vue3中的 toRef 和 toRefs
toRdf 作用:创建一个 ref 对象,其 value 值指向另一个对象中的某个属性。语法: const name toRef(person,name) 应用:要将响应式对象中的某个属性单独提供给外部使用时。扩展:toRef 与 toRefs 功能一致࿰…...
JAVA获取视频音频时长 文件大小 MultipartFileUtil和file转换
java 获取视频时长_java获取视频时长_似夜晓星辰的博客-CSDN博客 <dependency><groupId>ws.schild</groupId><artifactId>jave-all-deps</artifactId><version>2.5.1</version></dependency>Slf4j public class VideoTimeUtil…...
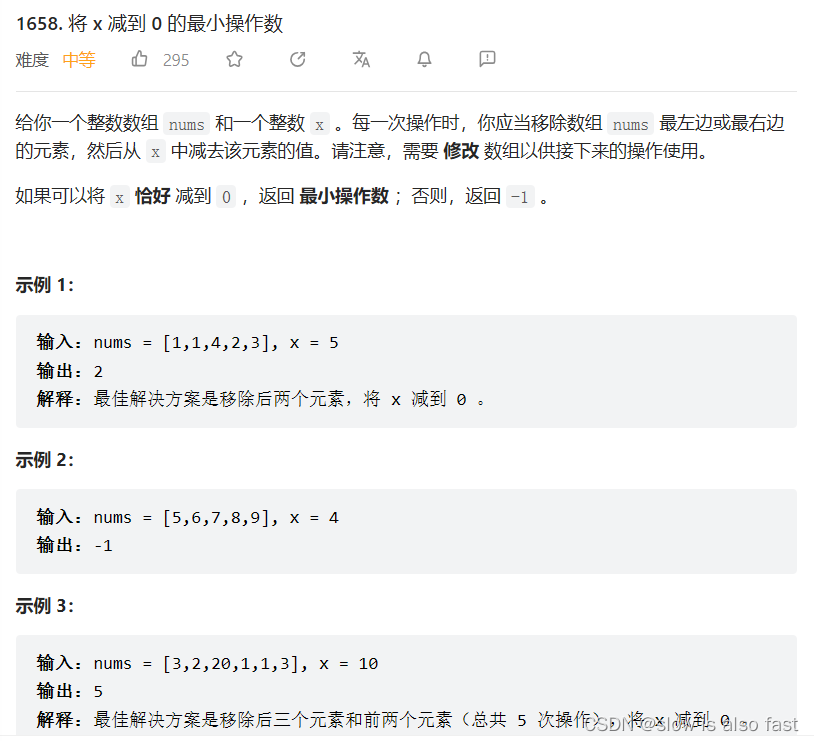
刷题笔记 day9
1658 将 x 减到 0 的最小操作数 解析:1. 当数组的两端的数都大于x时,直接返回 -1。 2. 当数组所有数之和小于 x 时 ,直接返回 -1。 3. 数组中可以将 x 消除为0,那么可以从左边减小为 0 ;可以从右边减小为 0 ࿱…...
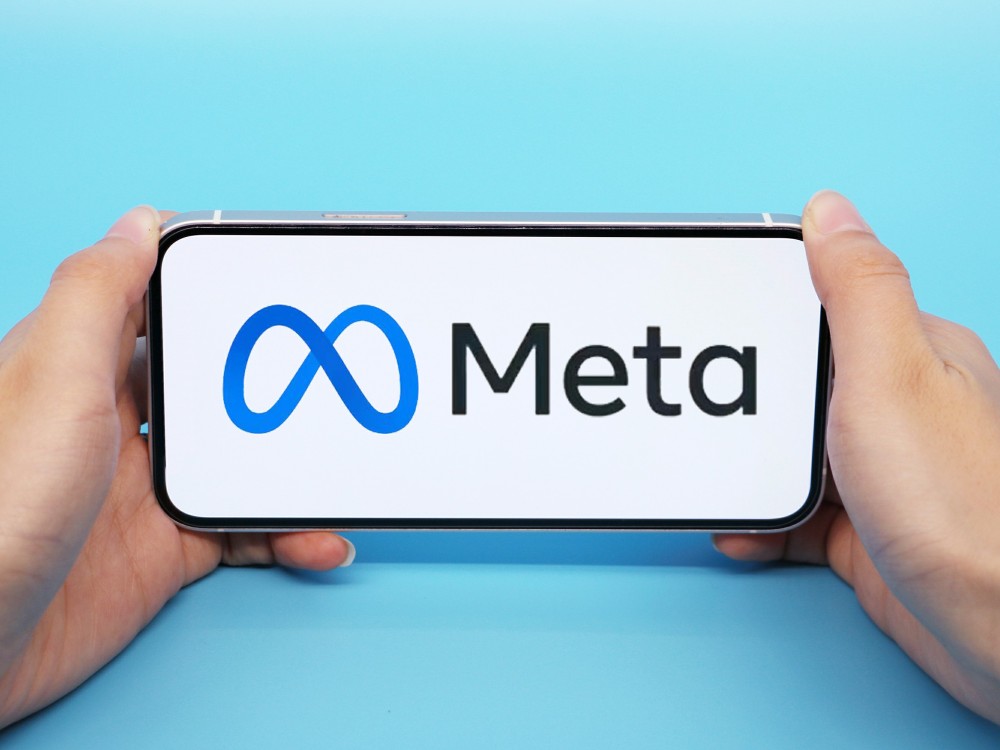
小白解密ChatGPT大模型训练;Meta开源生成式AI工具AudioCraft
🦉 AI新闻 🚀 Meta开源生成式AI工具AudioCraft,帮助用户创作音乐和音频 摘要:美国公司Meta开源了一款名为AudioCraft的生成式AI工具,可以通过文本提示生成音乐和音频。该工具包含三个核心组件:MusicGen用…...
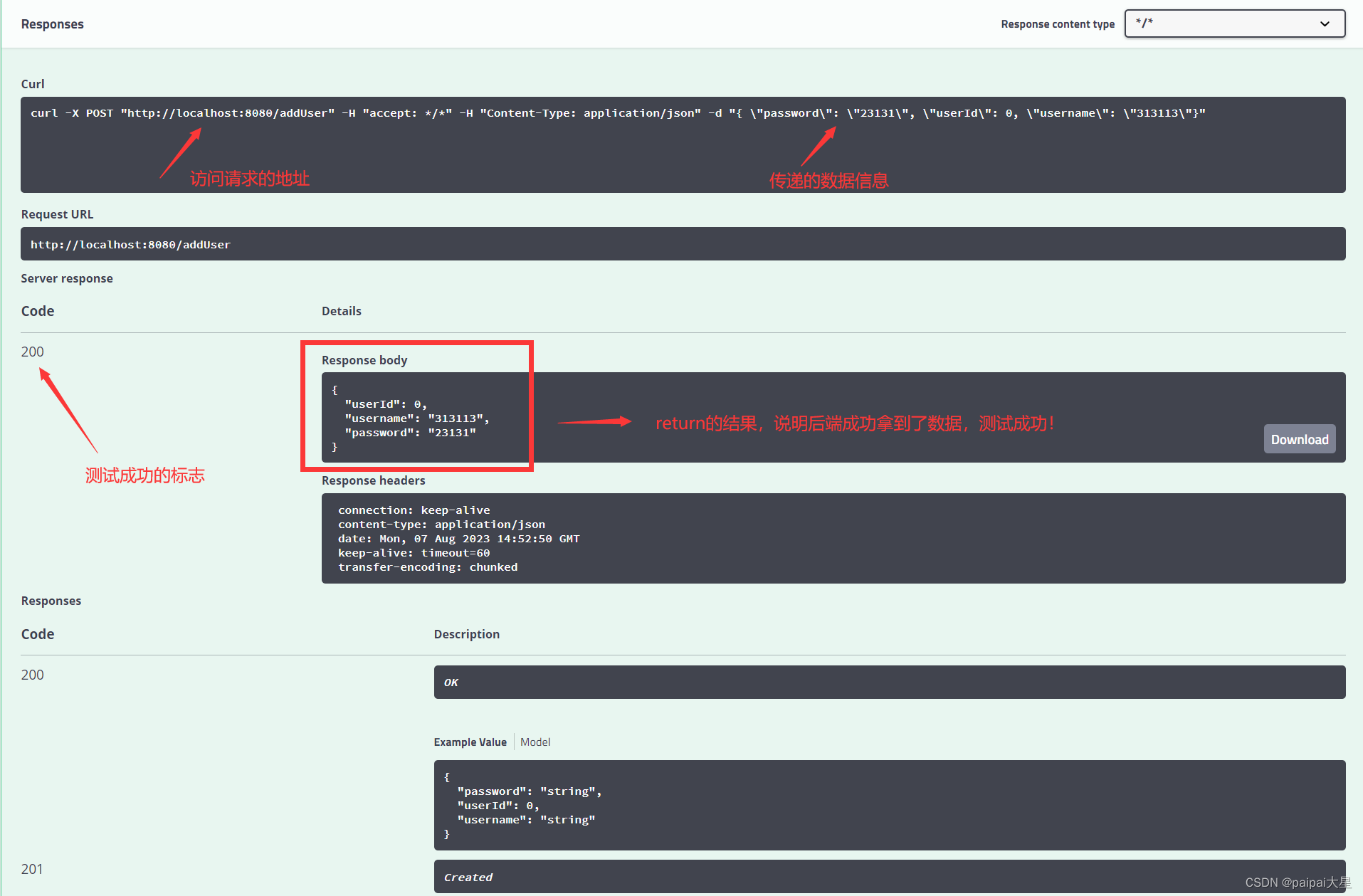
1 swagger简单案例
1.1 加入依赖 <!--swagger图形化接口--><dependency><groupId>io.springfox</groupId><artifactId>springfox-swagger2</artifactId><version>2.9.2</version> </dependency><dependency><groupId>io.spri…...
Flutter写一个android底部导航栏框架
废话不多说,上代码: import package:flutter/material.dart;void main() {runApp(MyApp()); }class MyApp extends StatelessWidget {overrideWidget build(BuildContext context) {return MaterialApp(title: Bottom Navigation Bar,theme: ThemeData(…...
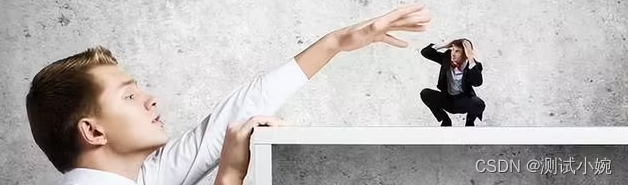
关于自动化测试用例失败重试的一些思考
自动化测试用例失败重跑有助于提高自动化用例的稳定性,那我们来看一下,python和java生态里都有哪些具体做法? 怎么做 如果是在python生态里,用pytest做测试驱动,那么可以通过pytest的插件pytest-rerunfailures来实现…...
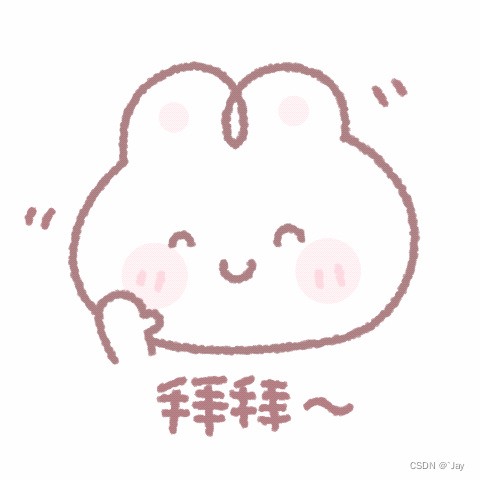
JS逆向之顶像滑块
本教程仅限于学术探讨,也没有专门针对某个网站而编写,禁止用于非法用途、商业活动、恶意滥用技术等,否则后果自负。观看则同意此约定。如有侵权,请告知删除,谢谢! 目录 一、接口请求流程 二、C1包 三、ac 四…...
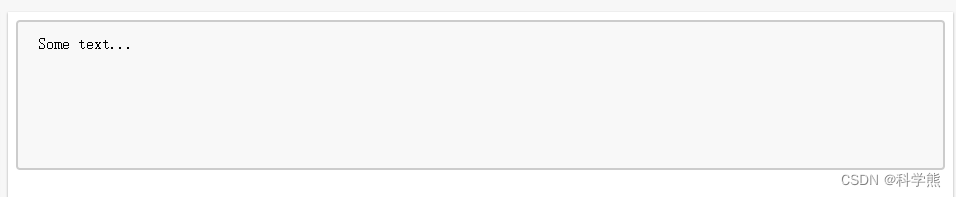
【css】textarea-通过resize:none 禁止拖动设置大小
使用 resize 属性可防止调整 textareas 的大小(禁用右下角的“抓取器”): 没有设置resize:none 代码: <!DOCTYPE html> <html> <head> <style> textarea {width: 100%;height: 150px;padding: 12px 20p…...
Linux内核学习小结
网上学习总结的一些资料,加上个人的一些总结。 Linux内核可以分成基础层和应用层。 基础层包括数据结构,内核同步机制,内存管理,任务调度。 应用层包括文件系统,设备和驱动,网络,虚拟化等。文件…...
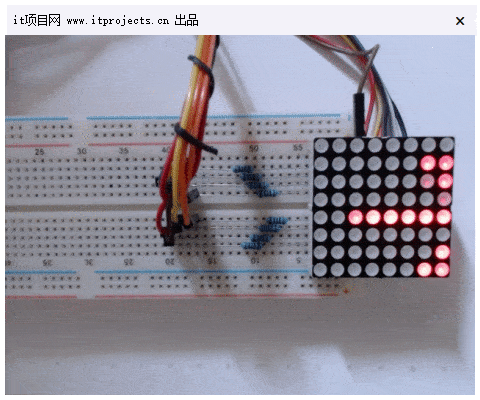
八、ESP32控制8x8点阵屏
引脚的说明如下 上图中 C表示column 列的意思,所有的C接高电压,即控制esp32中输出1L表示line 行的意思,所有的L接低电压,即控制esp32中输出为01. 运行效果 2. 点阵屏引脚...
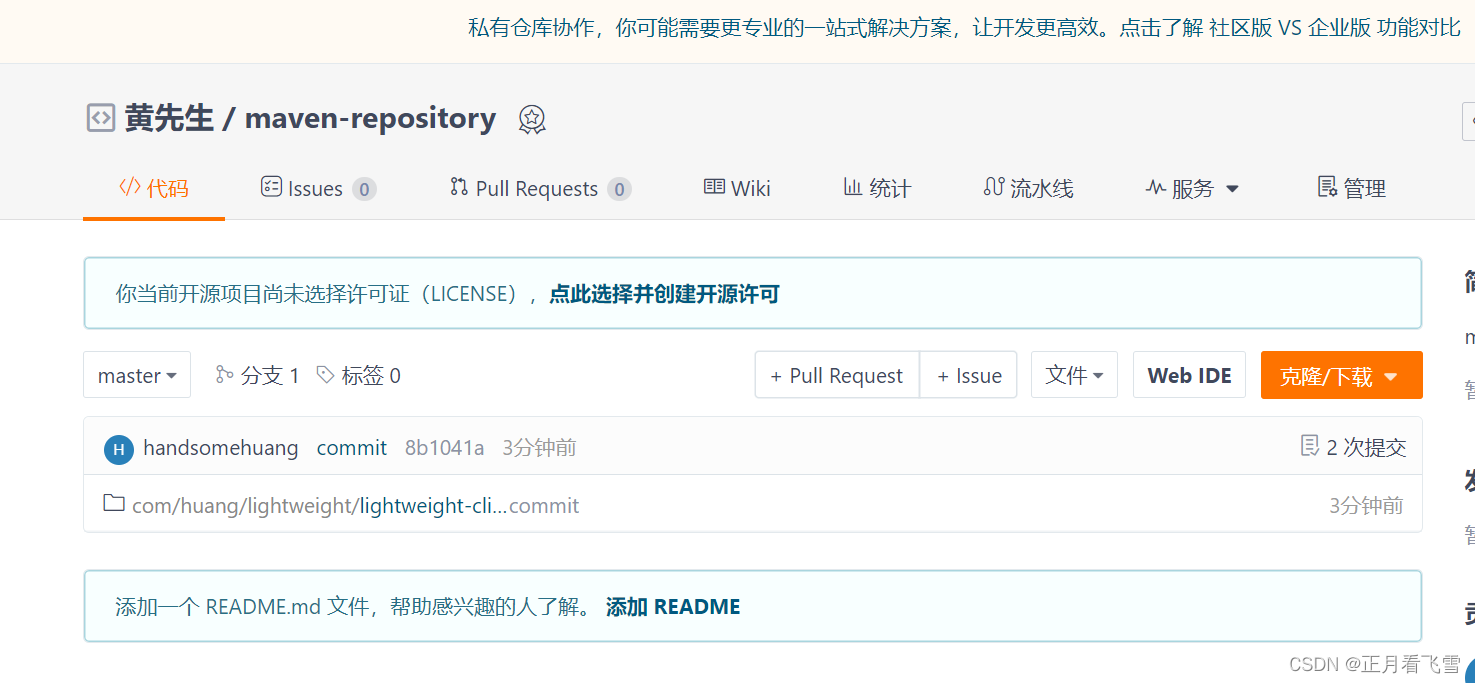
使用gitee创建远程maven仓库
1. 创建一个项目作为远程仓库 2. 打包项目发布到远程仓库 id随意,url是打包到哪个文件夹里面 在需要打包的项目的pom中添加 <distributionManagement><repository><id>handsomehuang-maven</id><url>file:D:/workspace/java/2023/re…...
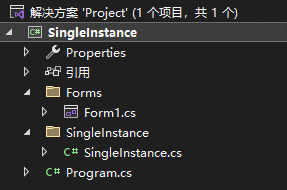
基于C#的应用程序单例唯一运行的完美解决方案 - 开源研究系列文章
今次介绍一个应用程序单例唯一运行方案的代码。 我们知道,有些应用程序在操作系统中需要单例唯一运行,因为程序多开的话会对程序运行效果有影响,最基本的例子就是打印机,只能运行一个实例。这里将笔者单例运行的代码共享出来&…...
2023-08-07力扣今日二题
链接: 剑指 Offer 29. 顺时针打印矩阵 题意: 如题 解: 麻烦的简单题,具体操作类似走地图,使用一个长度四的数组表示移动方向 我这边的思路是如果按正常的方向没有路走了,那转向下一个方向一定有路&am…...
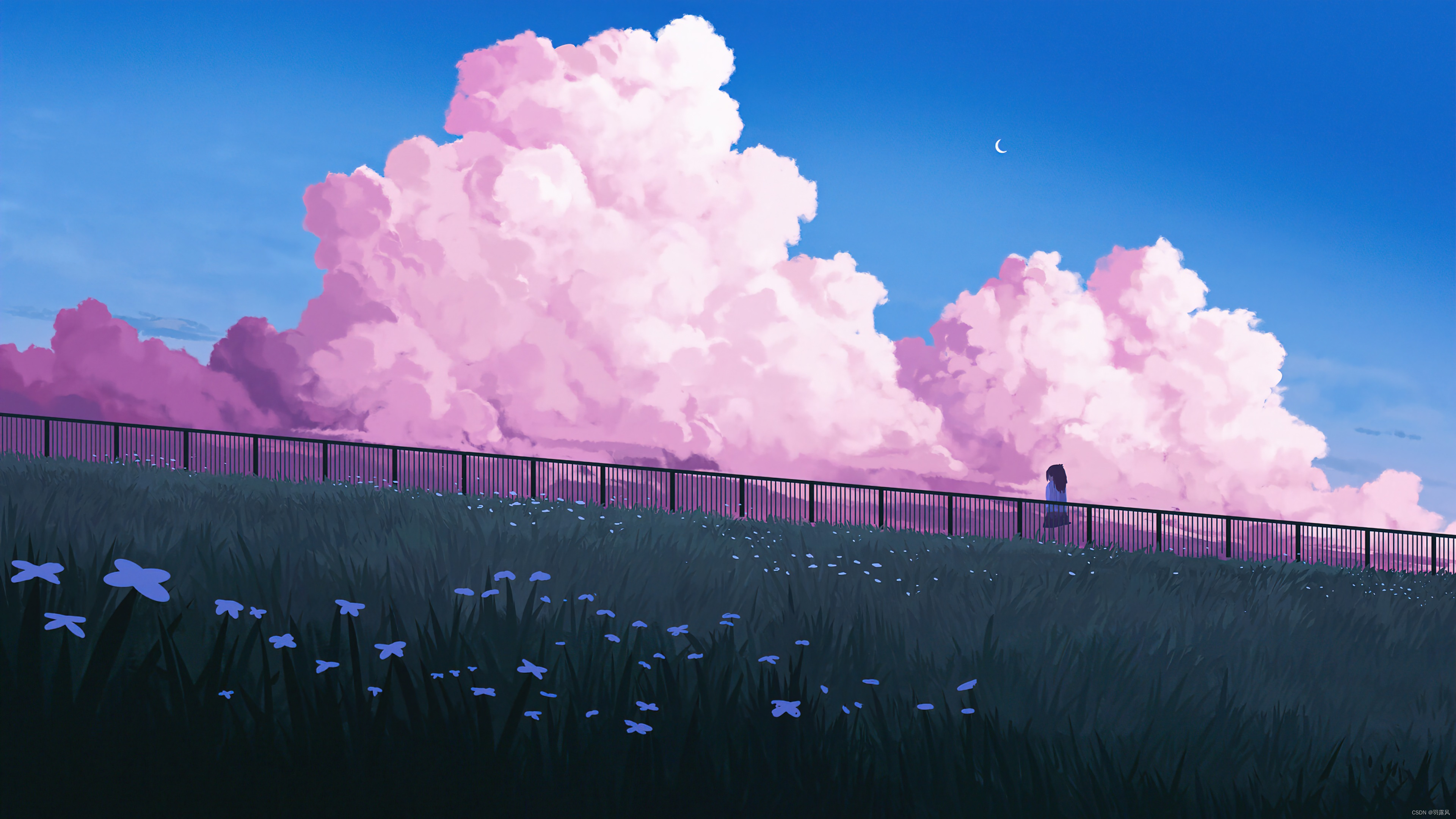
Spring接口ApplicationRunner的作用和使用介绍
在Spring框架中,ApplicationRunner接口是org.springframework.boot.ApplicationRunner接口的一部分。它是Spring Boot中用于在Spring应用程序启动完成后执行特定任务的接口。ApplicationRunner的作用是在Spring应用程序完全启动后,执行一些初始化任务或处…...
奶牛排队 java 思维题
👨🏫 5133. 奶牛排队 题目描述 约翰的农场有 n n n 头奶牛,每一头奶牛都有一个正整数编号。 不同奶牛的编号不同。 现在,这 n n n 头牛按某种顺序排成一队,每头牛都拿出一张纸条写下了其前方相邻牛的编号以及其…...
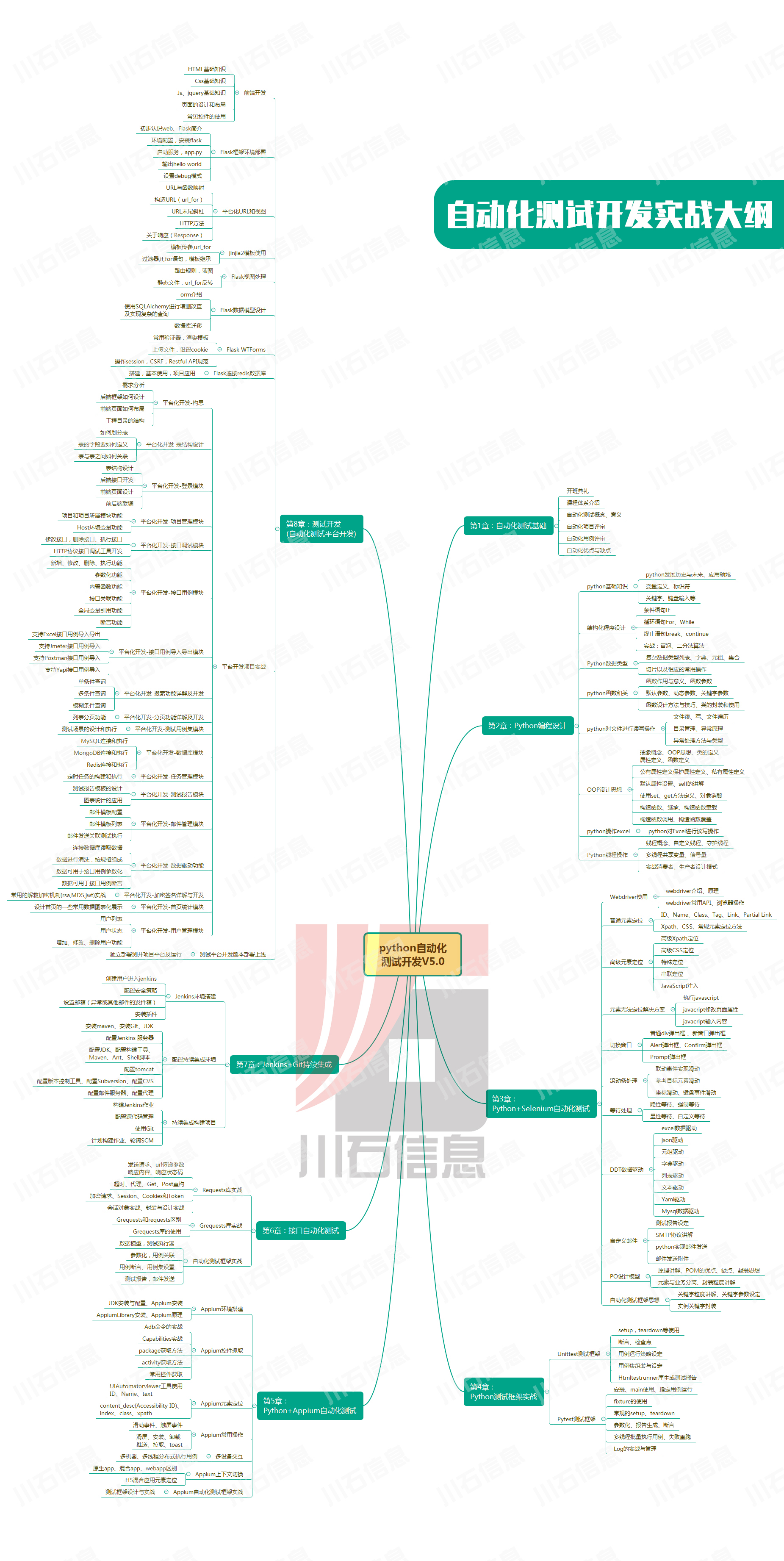
接口测试中缓存处理策略
在接口测试中,缓存处理策略是一个关键环节,直接影响测试结果的准确性和可靠性。合理的缓存处理策略能够确保测试环境的一致性,避免因缓存数据导致的测试偏差。以下是接口测试中常见的缓存处理策略及其详细说明: 一、缓存处理的核…...
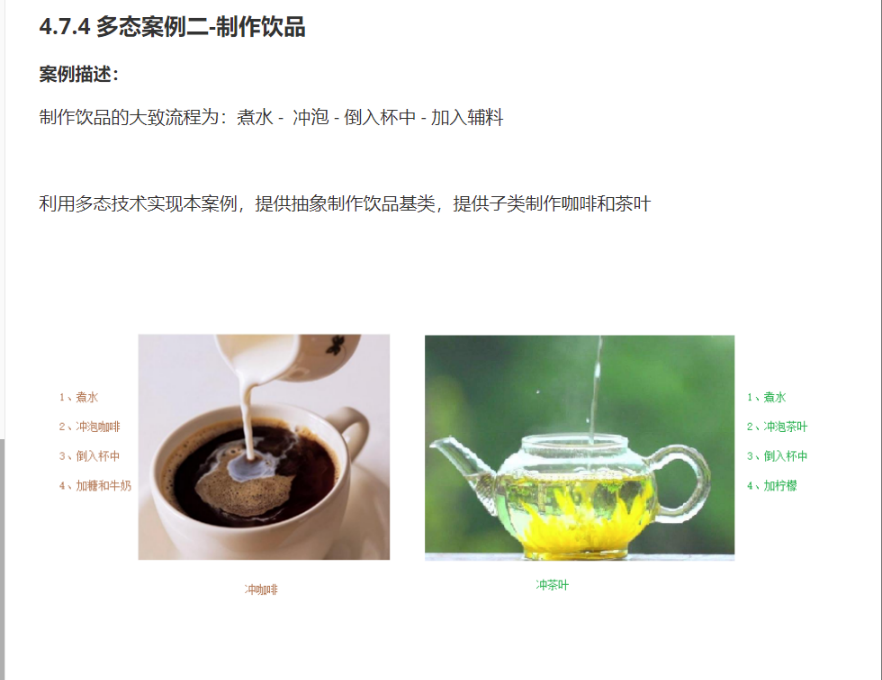
C++_核心编程_多态案例二-制作饮品
#include <iostream> #include <string> using namespace std;/*制作饮品的大致流程为:煮水 - 冲泡 - 倒入杯中 - 加入辅料 利用多态技术实现本案例,提供抽象制作饮品基类,提供子类制作咖啡和茶叶*//*基类*/ class AbstractDr…...
利用ngx_stream_return_module构建简易 TCP/UDP 响应网关
一、模块概述 ngx_stream_return_module 提供了一个极简的指令: return <value>;在收到客户端连接后,立即将 <value> 写回并关闭连接。<value> 支持内嵌文本和内置变量(如 $time_iso8601、$remote_addr 等)&a…...
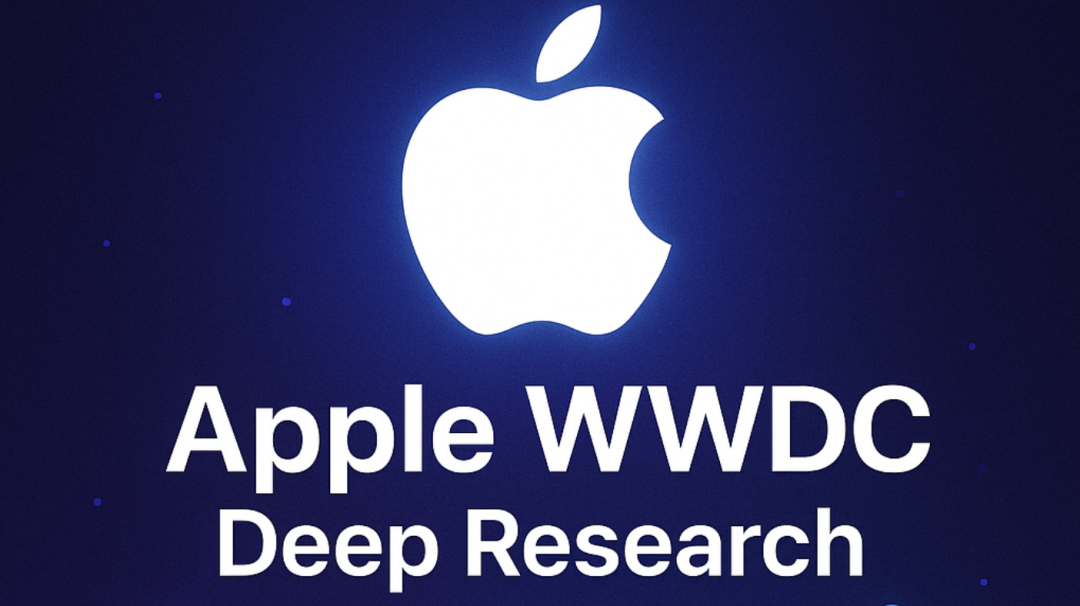
从WWDC看苹果产品发展的规律
WWDC 是苹果公司一年一度面向全球开发者的盛会,其主题演讲展现了苹果在产品设计、技术路线、用户体验和生态系统构建上的核心理念与演进脉络。我们借助 ChatGPT Deep Research 工具,对过去十年 WWDC 主题演讲内容进行了系统化分析,形成了这份…...
从零实现富文本编辑器#5-编辑器选区模型的状态结构表达
先前我们总结了浏览器选区模型的交互策略,并且实现了基本的选区操作,还调研了自绘选区的实现。那么相对的,我们还需要设计编辑器的选区表达,也可以称为模型选区。编辑器中应用变更时的操作范围,就是以模型选区为基准来…...
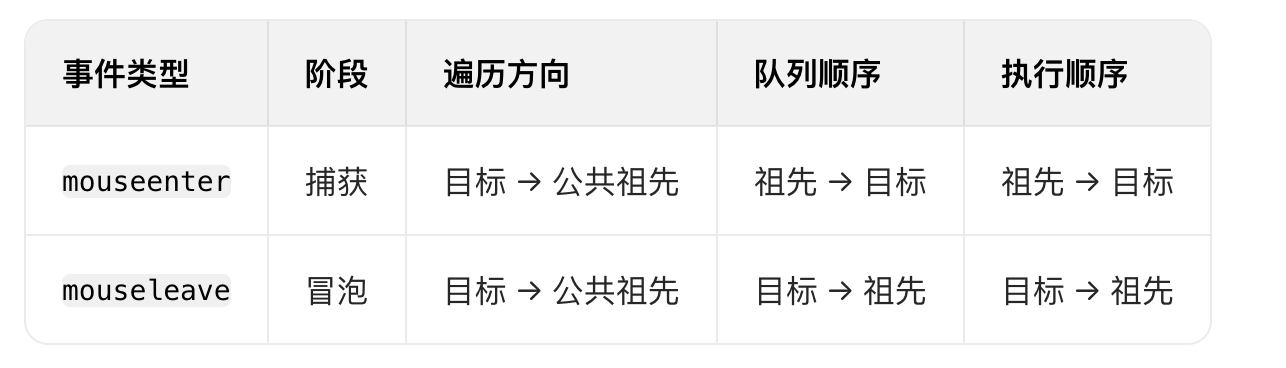
React19源码系列之 事件插件系统
事件类别 事件类型 定义 文档 Event Event 接口表示在 EventTarget 上出现的事件。 Event - Web API | MDN UIEvent UIEvent 接口表示简单的用户界面事件。 UIEvent - Web API | MDN KeyboardEvent KeyboardEvent 对象描述了用户与键盘的交互。 KeyboardEvent - Web…...
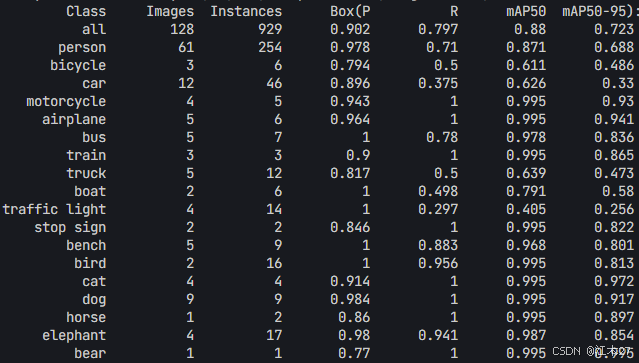
Yolov8 目标检测蒸馏学习记录
yolov8系列模型蒸馏基本流程,代码下载:这里本人提交了一个demo:djdll/Yolov8_Distillation: Yolov8轻量化_蒸馏代码实现 在轻量化模型设计中,**知识蒸馏(Knowledge Distillation)**被广泛应用,作为提升模型…...
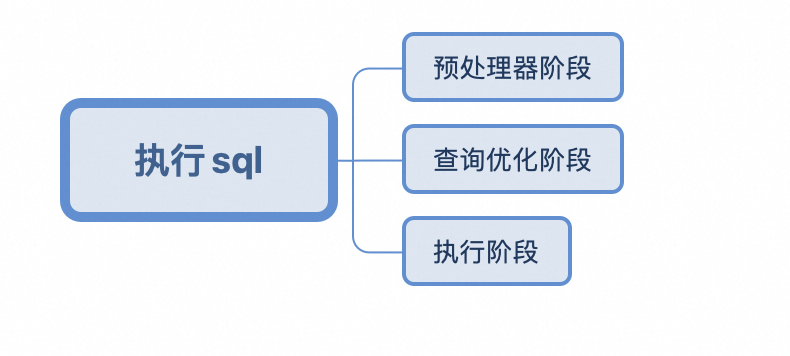
Mysql中select查询语句的执行过程
目录 1、介绍 1.1、组件介绍 1.2、Sql执行顺序 2、执行流程 2.1. 连接与认证 2.2. 查询缓存 2.3. 语法解析(Parser) 2.4、执行sql 1. 预处理(Preprocessor) 2. 查询优化器(Optimizer) 3. 执行器…...
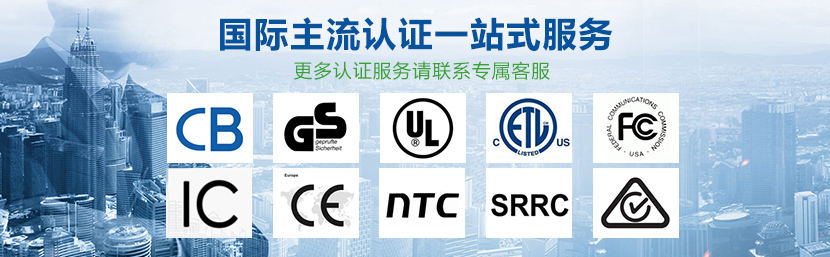
手机平板能效生态设计指令EU 2023/1670标准解读
手机平板能效生态设计指令EU 2023/1670标准解读 以下是针对欧盟《手机和平板电脑生态设计法规》(EU) 2023/1670 的核心解读,综合法规核心要求、最新修正及企业合规要点: 一、法规背景与目标 生效与强制时间 发布于2023年8月31日(OJ公报&…...
嵌入式常见 CPU 架构
架构类型架构厂商芯片厂商典型芯片特点与应用场景PICRISC (8/16 位)MicrochipMicrochipPIC16F877A、PIC18F4550简化指令集,单周期执行;低功耗、CIP 独立外设;用于家电、小电机控制、安防面板等嵌入式场景8051CISC (8 位)Intel(原始…...