Leetcode Top 100 Liked Questions(序号75~104)
75. Sort Colors
题意:红白蓝的颜色排序,使得相同的颜色放在一起,不要用排序
我的思路
哈希
代码 Runtime 4 ms Beats 28.23% Memory 8.3 MB Beats 9.95%
class Solution {
public:void sortColors(vector<int>& nums) {vector<int> ans; int n=nums.size();int vis[3]={0};for(int i=0;i<n;i++) vis[nums[i]]++;int v=0;for(int i=0;i<3;i++){for(int j=0;j<vis[i];j++){nums[v++]=i;}}}
};
标答
Dutch National Flag Problem荷兰国旗问题;
代码 计数排序 Runtime 0 ms Beats 100% Memory 8.3 MB Beats 41.44%
感觉和我之前做的差不多
class Solution {
public:void sortColors(vector<int>& nums) {int num0 = 0;int num1 = 0;int num2 = 0;for (int num:nums) {if( num==0 ) num0++;else if (num == 1) num1++; else if (num == 2) num2++; }for (int i=0; i< nums.size(); i++) {if( num0>0 ) {nums[i] = 0; num0--;} else if( num1>0 ) {nums[i] = 1; num1--;} else if( num2>0 ) {nums[i] = 2; num2--;}}}
};
代码 双指针 Runtime 0 ms Beats 100% Memory 8.3 MB Beats 41.44%
如果nums[mid]是0,low和mid交换,因为low负责0的部分,low++保证指针前面的都是0,low指针指到的就只有0或者1;如果nums[mid]是1,那么是正确的;如果nums[mid]是2,high指向的和mid指向的交换,high--来确保high的右边都是2,为什么mid不++?因为mid这时可能是0,所以需要循环一次在判断
class Solution {
public:void sortColors(vector<int>& nums) {int low = 0, mid = 0, high = nums.size()-1;while(mid <= high){if(nums[mid] == 0){swap(nums[low], nums[mid]); low++; mid++;}else if(nums[mid] == 1) mid++;else{swap(nums[mid], nums[high]); high--;}}}
};
76. Minimum Window Substring
题意:给出两个字符串s和t,找到minimum window substring
我的思路
不会
标答
用滑动窗口,窗口的开头在s字符串的初始位置,结尾在所有t的字符都在里面;当上面的窗口有了,就可以改变头指针来缩小窗口,步骤为:
1. 如果s比t小,那么直接返回;2. 记录t中的字母数量
3. 初始化;4. 尾指针遍历s
5. 一边遍历的时候一遍计算t中的字母数量;6. 如果数量剪完了,说明窗口形成了,现在要通过移动头指针实现窗口缩小了
7. 记录下最小的窗口长度和起始位置;8. 返回答案
注意:如何在头指针移动中判断当前节点是或否是t中的?在每个end指针移动的时候会把mp中的字母--,这时候就只有在t中的字母是大于等于0的了
代码 Runtime 7 ms Beats 92.29% Memory7.9 MB Beats 79.63%
class Solution {
public:string minWindow(string s, string t) {if(t.size()>s.size())return "";unordered_map<char,int>mp;for(int i=0;i<t.size();i++)mp[t[i]]++;int st=0,en=0,minli=99999,minst=0,coun=0;//st是头指针,en是尾指针,minli是最短的字符串长度,minst是头指针位置,coun是t字符个数for(;en<s.size();en++){if(mp[s[en]]>0) coun++;//属于字符串t的字母数量mp[s[en]]--;//无论它是不是字符串t中的字符,都要减减,这样子剪完就说明t字符都没了//变成负数就说明它减多了if(coun==t.size()){//字符串数量已经over了for(;st<en && (mp[s[st]]<0);){//注意这里是小于0,如果是大于0就说明减多了mp[s[st]]++;st++;//先加加,恢复mp原来的个数,之后st前进}if(en-st<minli){//看看头指针改变过后,最小字符串长度是否更新了minst=st;minli=en-st;}mp[s[st]]++;st++;//更新头指针,使得目前的字符串不合法coun--;//这时coun--,让尾指针可以接着更新}}string ans="";if (minli!=99999)ans= s.substr(minst,minli+1);return ans;}
};
78. Subsets
题意:给出一个数字集,输出所有的子集(包括空集)
我的思路
用递归?就像之前一样;有想过要不要用循环,但是发现用循环的话答案不对,就把循环消去了
代码 Runtime 0 ms Beats 100% Memory 7 MB Beats 78.17%
class Solution {
public:void sol(vector<int>& nums,int nxt,vector<int>& pol,vector<vector<int>> &ans){if(nxt==nums.size()){ans.push_back(pol);return ;}pol.push_back(nums[nxt]);//因为拿或者不拿sol(nums,nxt+1,pol,ans);pol.pop_back();sol(nums,nxt+1,pol,ans);}vector<vector<int>> subsets(vector<int>& nums) {vector<vector<int>> ans={}; vector<int> pol={};sol(nums,0,pol,ans);return ans;}
};
79. Word Search
题意:
我的思路
把所有可能性的都遍历一遍,如果是BFS的话,先把起点找到,之后环顾四周看看有没有(?
首先要准备一个队列,把(位置,第几个英文字母)找到的都放到队列里,之后bfs上下左右的看,如果在周围找不到下一个就return false,但是做到最后 超时了TLE
代码 超时代码
class Solution {
public:struct node{int x,y,id;vector<vector<bool> > vis=vector(6 , vector<bool>(6,0));};bool exist(vector<vector<char>>& board, string word) {queue<node>st,q;int n=board.size(),m=board[0].size();for(int i=0;i<n;i++){for(int j=0;j<m;j++){if(board[i][j]==word[0]){node temp;temp.x=i;temp.y=j;temp.id=0;temp.vis[i][j]=1;st.push(temp);}}}int dx[]={1,-1,0,0};int dy[]={0,0,1,-1};while(!st.empty()){while(!q.empty())q.pop();//清空node te=st.front();st.pop();q.push(te);while(!q.empty()){node now=q.front(); int nx,ny;if(now.id==word.size()-1)return 1;q.pop();for(int i=0;i<4;i++){nx=now.x+dx[i];ny=now.y+dy[i];if(nx>=0&&nx<n&&ny>=0&&ny<m&&!now.vis[nx][ny]){if(board[nx][ny]==word[now.id+1]){node ans;ans.x=nx;ans.y=ny;ans.id=now.id+1;ans.vis=now.vis;ans.vis[nx][ny]=1;q.push(ans);}}}}}return 0;}
};
标答
首先得到n和m,遍历地图,如果地图是第一个字符,那么就可以开始递归了
代码 Runtime 774 ms Beats 47.25% Memory 7.9 MB Beats 74.74%
在递归条件中先判断是不是这个字母,然后递归下去
class Solution {
public:bool solve(vector<vector<char>>& board,int x,int y,int c,int m,int n,string word){if(c==word.size())return 1;if(x<0||y<0||x>=m||y>=n)return 0;if(board[x][y]!=word[c])return 0;char ch=board[x][y];board[x][y]='#';//这一手就不会来回走了!if(solve(board,x+1,y,c+1,m,n,word)||solve(board,x,y+1,c+1,m,n,word)||solve(board,x-1,y,c+1,m,n,word)||solve(board,x,y-1,c+1,m,n,word))return 1;board[x][y]=ch;return 0;}bool exist(vector<vector<char>>& board, string word) {int m=board.size(), n=board[0].size();for(int i=0;i<m;i++){for(int j=0;j<n;j++){if(solve(board,i,j,0,m,n,word))return 1;}}return 0;}
};
优化代码 Runtime 0 ms Beats 100% Memory8.1 MB Beats 42.63%
优化途径
1. 不要把递归放在判断if里面,这样会费时
2. 先把字符串倒过来,如果最后一个字符的数量大于第一个字符的数量,再把字符串倒转回去;也就是先把字符数量少的先递归【但是我不知道为什么】
class Solution {
public:bool ispres(vector<vector<char>>& board, int i, int j, const string &word,int c,int m, int n){if(c==word.length()){return true;}if(i < 0 || j < 0 || i >= m || j >= n || board[i][j]!=word[c]){return false;}char ch = board[i][j];board[i][j] = '*';bool flag = ispres(board,i+1,j,word,c+1,m,n) || ispres(board,i-1,j,word,c+1,m,n) || ispres(board,i,j+1,word,c+1,m,n) || ispres(board,i,j-1,word,c+1,m,n);board[i][j] = ch;return flag;}bool exist(vector<vector<char>>& board, string word) {int m = board.size();int n = board[0].size();reverse(word.begin(), word.end());if (count(word.begin(), word.end(), word[0]) > count(word.begin(), word.end(), word[word.size() - 1])) reverse(word.begin(), word.end());for(int i = 0; i < m; i++){for(int j = 0; j < n; j++){if(board[i][j]==word[0]){if(ispres(board,i,j,word,0,m,n)){return true;}}}}return false;}
};
84. Largest Rectangle in Histogram
题意:
我的思路
如果是双指针的话,min(a[i],…,a[j])*(j-i+1)
那就首先是On^2的时间复杂度,但是预处理的话二维数组会爆栈
不会做
标答
代码 单调栈 Runtime 125 ms Beats 88.36% Memory77.3 MB Beats 73.1%
st里面从小到大的放置,相等的留下,ran是最近的比当前的要大的高度,height的数组最后加上0。求面积是弹出的最大值*(当前的位置减去栈中目前的位置-1)
为什么是当前的位置减去栈中目前的位置-1?
因为设这一轮被弹出的位置为x,高位h[x],弹出后的栈顶位置为y,因为h[y]小于等于h[x],也就是说,(y,x]的位置之间的高都是大于h[x];
【不然为什么(y,x]的位置之间的高要被弹出去呢】
同时(x,i)的位置之间的高也都是大于h[x]
【不然x就被弹出去了】
所以(y,i)之间,x是最小的;因此面积是h[ x ] * ( i - y - 1 )
注意:初始化ran,初始化h数组
为什么栈一开始不把-1放入?【就像32. Trapping Rain WaterLeetCode Top100 Liked 题单(序号19~33)】
因为32是栈,而这个是单调栈,需要比较站内的元素来决定是否弹出
相关:42. Trapping Rain WaterLeetCode Top100 Liked
class Solution {
public:int largestRectangleArea(vector<int>& h) {stack<int> st;int ans=0;h.push_back(0); int n=h.size();for(int i=0;i<n;i++){while(!st.empty()&&h[st.top()]>h[i]){//找到了高的int x=st.top(),y;st.pop();if(st.empty()) y=-1;else y=st.top();ans=max(ans,h[x]*(i-y-1));}st.push(i);}return ans;}
};
代码 Runtime 86 ms Beats 99.54% Memory74.7 MB Beats 99.53%
感觉和单调栈的原理差不多,但是没有看懂
class Solution {
public:int largestRectangleArea(const vector<int>& heights) {int end=-1;int n=heights.size();int maxArea=0;int counts[10001] = {};int sortedIndexes[10001] = {};for (int i=0;i<=n;++i) {int count = 1;while(true){if(end<0)break;const int index=sortedIndexes[end];if(i!=n&&heights[index]<heights[i])break;count+=counts[end];int area=(counts[end]+i-index-1)*heights[index];if(area>maxArea)maxArea=area;--end;}sortedIndexes[++end] = i;counts[end] = count;}return maxArea;}
};
94. Binary Tree Inorder Traversal
题意:输出二叉树中序排序
我的思路
就直接中序递归就可以了
注意:当root为空的时候要特判!!!
代码 Runtime0 ms Beats 100% Memory 8.2 MB Beats 97.70%
class Solution {
public:void p(TreeNode* root,vector<int>& ans){if(root->left !=NULL)p(root->left,ans);ans.push_back(root->val);if(root->right!=NULL)p(root->right,ans);}vector<int> inorderTraversal(TreeNode* root) {if(root==NULL)return {};vector<int> ans;p(root,ans);return ans;}
};
98. Validate Binary Search Tree
题意:判断树是不是平衡搜索树BST,左子树比中间的小,右子树比中间的大
我的思路
还是递归,就像前面的79 word search一样搜索,然后判断ans的顺序大小
注意:等于也不行!!!
注意:int&的时候不要传const int 进去
代码 Runtime13 ms Beats 42.85% Memory 21.7 MB Beats 33.33%
class Solution {
public:bool P(TreeNode* root,long long & r){if(root->left!=NULL){if(!P(root->left,r))return 0;}if(r>=root->val) return 0;r=root->val;if(root->right!=NULL){if(!P(root->right,r))return 0;}return 1;}bool isValidBST(TreeNode* root) {long long ans=-2147483649;return P(root,ans);}
};
标答
确实更加简洁快速
代码 Runtime4 ms Beats 94.78% Memory21.7 MB Beats 33.33%
class Solution {
public:bool isValidBST(TreeNode* root,long min,long max){if(root==NULL)return true;if(root->val>=max or root->val<=min)return false;return isValidBST(root->left,min,root->val)&&isValidBST(root->right,root->val,max);}bool isValidBST(TreeNode* root) {long max=LONG_MAX;long min=LONG_MIN;return isValidBST(root,min,max);}
};
101. Symmetric Tree
题意:看是不是对称的
我的思路
中序序列ans,两个指针从两端向中间核对
答案不正确,因为[1,2,2,2,null,2]也会判断成正确的,所以不可以
既然是递归,那就要让左子树的左子树 和 右子树的右子树对称;左子树的右子树 和 右子树的左子树对称
但是不会写
标答
递归函数的参数是左子树指针和右子树指针,然后判断左的值和右的值是否相同
然后判断左的左和右的右 左的右和右的左
这样递归下去
代码 Runtime 0 ms Beats 100% Memory 16.2 MB Beats 91.71%
class Solution {
public:bool p(TreeNode* l,TreeNode* r){if(l==NULL&&r==NULL)return 1;else if (l==NULL||r==NULL)return 0;if(l->val!=r->val)return 0;return p(l->left,r->right)&&p(l->right,r->left);//递归}bool isSymmetric(TreeNode* root) {if(root==NULL)return 1;return p(root->left,root->right);}
};
102. Binary Tree Level Order Traversal
题意:层序遍历
我的思路
一层的结尾放一个空指针,每次循环到空指针的时候,就在结尾放一个空指针;当循环弹出空指针同时队列为空的时候,就停止放入空指针
代码 Runtime 6 ms Beats 52.11% Memory13.3 MB Beats 92.55%
class Solution {
public:vector<vector<int>> levelOrder(TreeNode* root) {vector<vector<int>> ans;queue <TreeNode *>q;if(root==NULL)return ans;q.push(root);q.push(NULL);vector<int> sol;while(!q.empty()){TreeNode * top;top=q.front();q.pop();if(top!=NULL){sol.push_back(top->val);if(top->left!=NULL)q.push(top->left);if(top->right!=NULL)q.push(top->right);}else{ans.push_back(sol);sol={};if(!q.empty())q.push(NULL);}}return ans;}
};
标答
用一层的队列大小得出一层有多少个,来循环
代码 Runtime 3 ms Beats 88.93% Memory13.6 MB Beats 51.20%
class Solution {
public:vector<vector<int>> levelOrder(TreeNode* root) {vector<vector<int>>ans; if(root == NULL) return ans; queue<TreeNode*>q;q.push(root);while(!q.empty()){int size = q.size(); vector<int> level; for(int i =0;i<size;i++){TreeNode* node=q.front();q.pop();if(node->left!=NULL)q.push(node->left);if(node->right!=NULL)q.push(node->right);level.push_back(node->val);}ans.push_back(level);}return ans; }
};
104. Maximum Depth of Binary Tree
题意:求树的最大深度
我的思路
用层序遍历看看有几层或者用递归无线向下,那就先用层序遍历向下
代码 层序遍历 Runtime 0 ms Beats 100% Memory19 MB Beats 9.46%
class Solution {
public:int maxDepth(TreeNode* root) {queue<TreeNode*> q; if(root==NULL)return 0;q.push(root);int deep=0;while(!q.empty()){deep++;int n=q.size();for(int i=0;i<n;i++){if(q.front()->left!=NULL)q.push(q.front()->left);if(q.front()->right!=NULL)q.push(q.front()->right);q.pop();}}return deep;}
};
标答 递归
树的高度=max(左子树的高度,右子树的高度)+1
代码 递归 Runtime 8 ms Beats 61.27% Memory19 MB Beats 9.46%
class Solution {
public:int maxDepth(TreeNode* root) {if(root==NULL)return 0;return max(maxDepth(root->left),maxDepth(root->right))+1;}
};
相关文章:
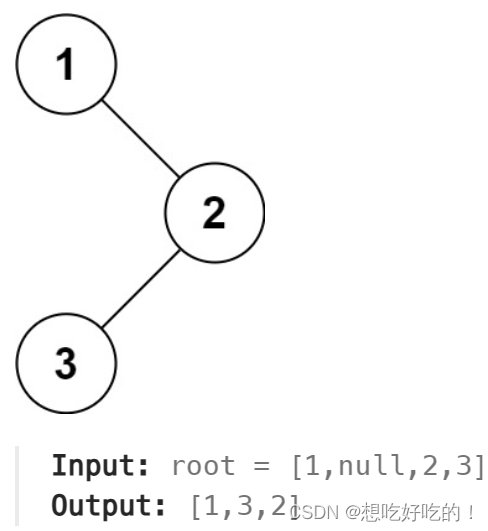
Leetcode Top 100 Liked Questions(序号75~104)
75. Sort Colors 题意:红白蓝的颜色排序,使得相同的颜色放在一起,不要用排序 我的思路 哈希 代码 Runtime 4 ms Beats 28.23% Memory 8.3 MB Beats 9.95% class Solution { public:void sortColors(vector<int>& nums) {vector…...

Shell编程之流程控制
目录 if判断 case语句 for循环 while循环 if判断 语法: if [ 条件判断表达式 ] then 程序 elif [ 条件判断表达式 ] then 程序 else 程序 fi 注意: [ 条件判断表达式 ],中括号和条件判断表达式之间必须有空格。if,elif…...
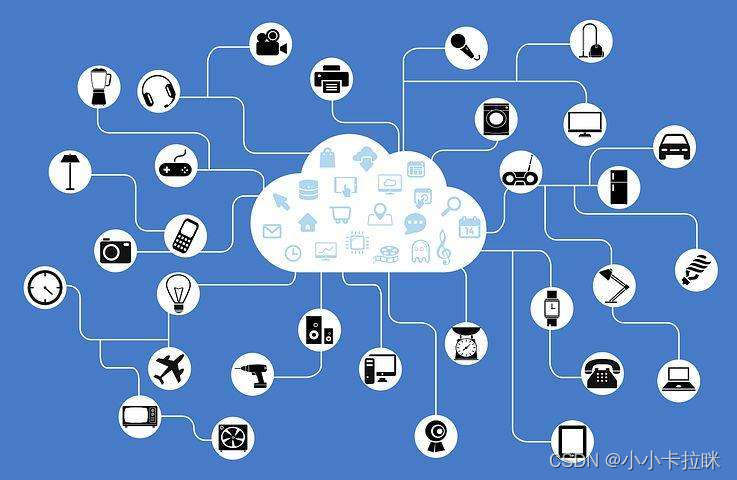
什么是Python爬虫分布式架构,可能遇到哪些问题,如何解决
目录 什么是Python爬虫分布式架构 1. 调度中心(Scheduler): 2. 爬虫节点(Crawler Node): 3. 数据存储(Data Storage): 4. 反爬虫处理(Anti-Scraping&…...
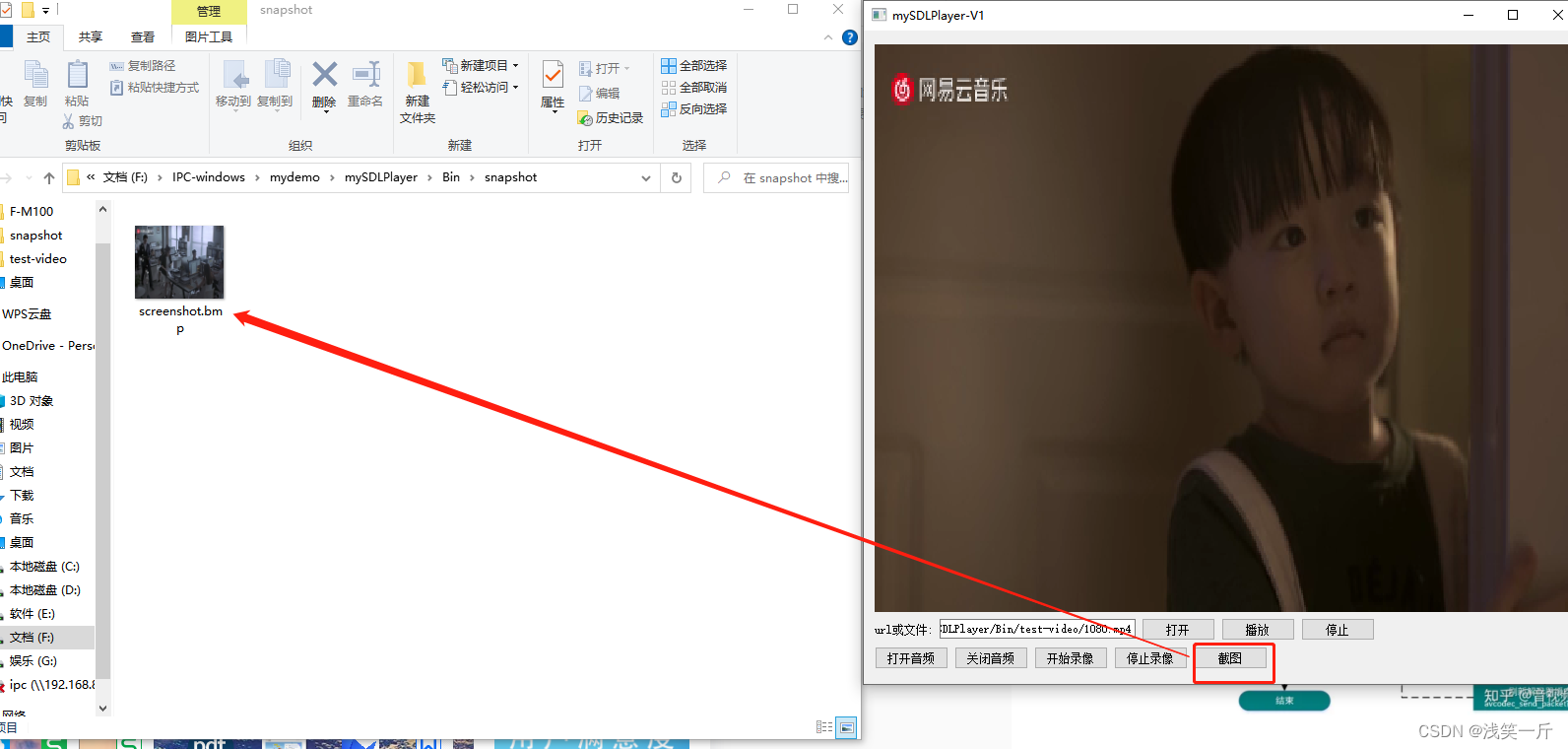
QT下使用ffmpeg+SDL实现音视频播放器,支持录像截图功能,提供源码分享与下载
前言: SDL是音视频播放和渲染的一个开源库,主要利用它进行视频渲染和音频播放。 SDL库下载路径:https://github.com/libsdl-org/SDL/releases/tag/release-2.26.3,我使用的是2.26.3版本,大家可以自行选择该版本或其他版…...
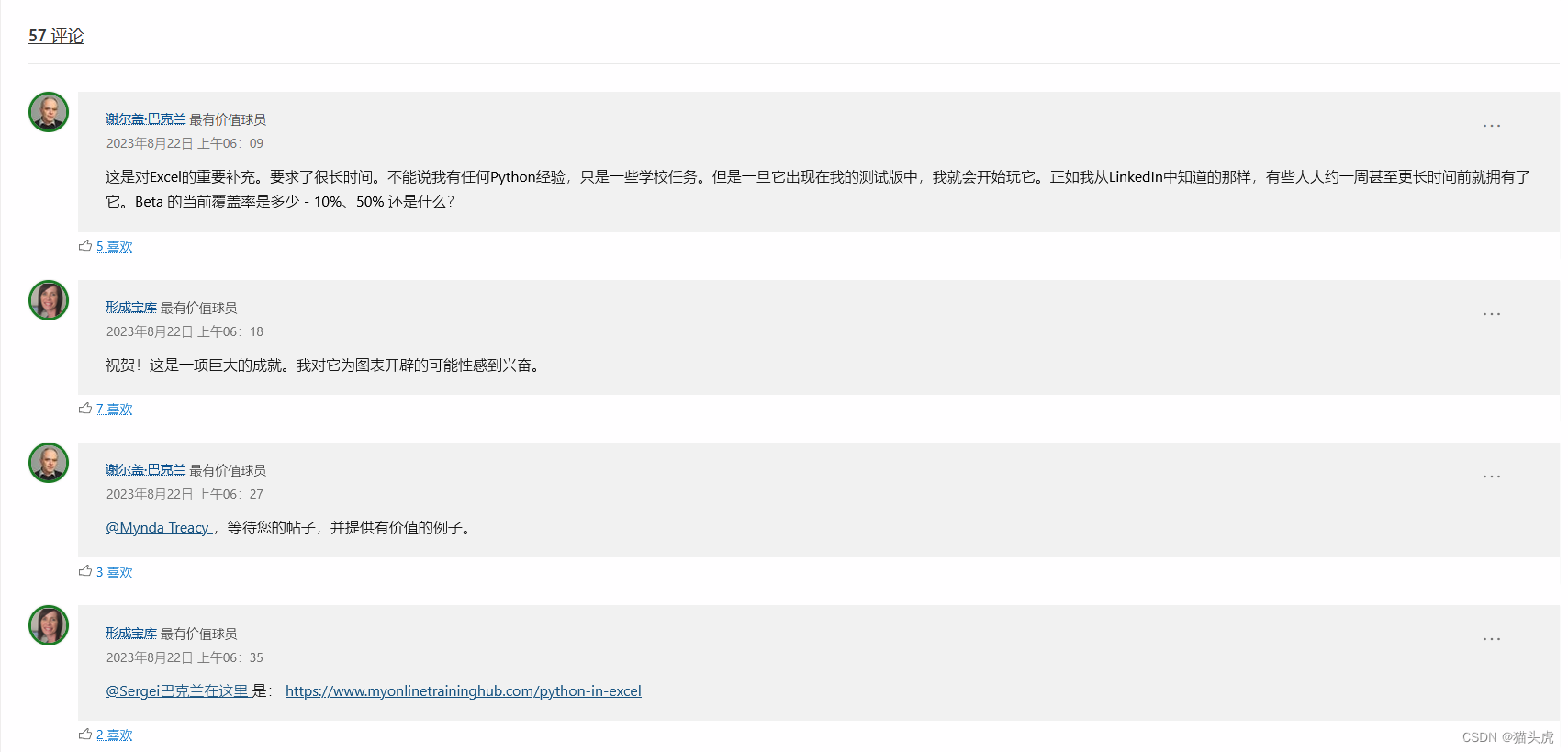
Microsoft Excel整合Python:数据分析的新纪元
🌷🍁 博主猫头虎 带您 Go to New World.✨🍁 🦄 博客首页——猫头虎的博客🎐 🐳《面试题大全专栏》 文章图文并茂🦕生动形象🦖简单易学!欢迎大家来踩踩~🌺 &a…...
【前端代码规范】
前端代码规范 vue3版本:【Vue&React】版本TS版本:【TS&JS】版本vite版本:【Webpack&Vite】版本Eslint版本:命名规则:【见名识意】项目命名:目录命名:JS/VUE文件CSS/SCSS文件命名:HTML文件命名:…...

postgresql-日期函数
postgresql-日期函数 日期时间函数计算时间间隔获取时间中的信息截断日期/时间创建日期/时间获取系统时间CURRENT_DATE当前事务开始时间 时区转换 日期时间函数 PostgreSQL 提供了以下日期和时间运算的算术运算符。 计算时间间隔 age(timestamp, timestamp)函数用于计算两…...
Android11去掉Setings里的投射菜单条目
Android11去掉【设置】--【已连接的设备】--【连接偏好设置】里的投射菜单条目,具体如下: commit 0c0583e6ddcdea21ec02db291d9a07d90f10aa59 Author: wzh <wzhincartech.com> Date: Wed Jul 21 16:37:13 2021 0800去掉投射菜单Change-Id: Id7f…...
fnm(Node.js 版本管理器)
fnm是什么? fnm是一款快速简单跨平台的 Node.js 版本管理器,使用 Rust 构建。 fnm怎么使用? 查看node 已安装列表 fnm list node 版本切换 fnm use 版本号 fnm use 16.0.0...
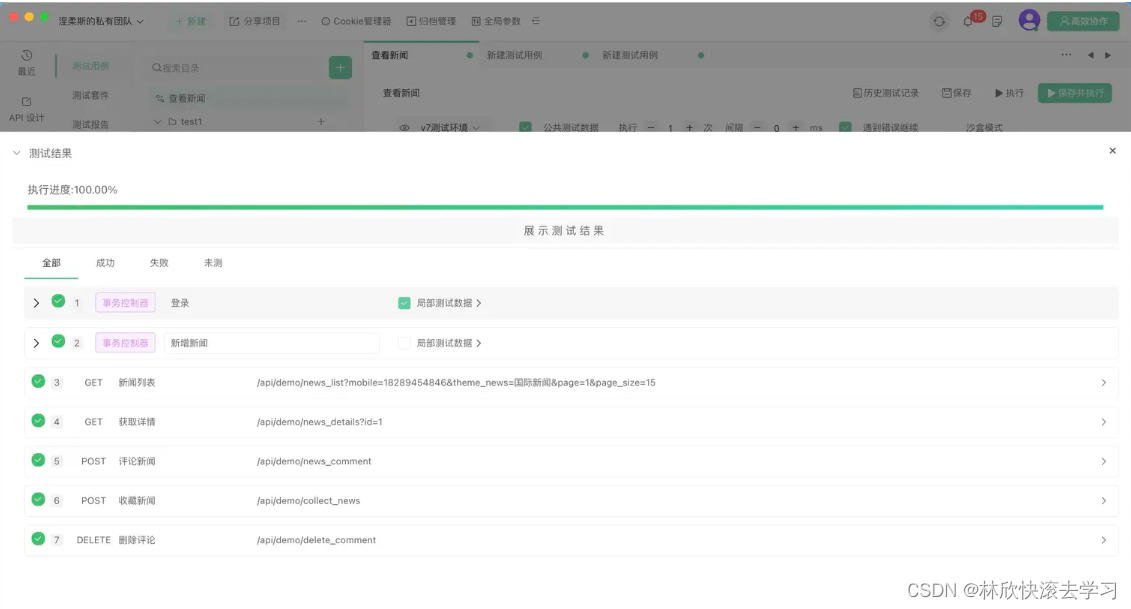
Apipost:为什么是开发者首选的API调试工具
文章目录 前言正文接口调试接口公共参数、环境全局参数的使用快速生成并导出接口文档研发协作接口压测和自动化测试结论 前言 Apipost是一款支持 RESTful API、SOAP API、GraphQL API等多种API类型,支持 HTTPS、WebSocket、gRPC多种通信协议的API调试工具。除此之外…...
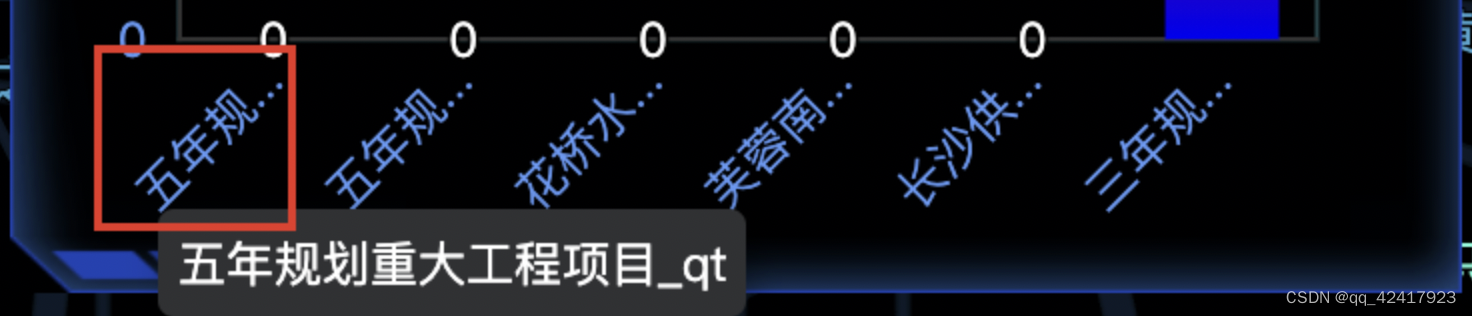
Echarts图表坐标轴文字太长,省略显示,鼠标放上显示全部(vue)
注意:记得加上这个,触发事件, triggerEvent: true,重点:下面就是处理函数,在实例化图表的时候使用,传入参数是echarts的实例 // 渲染echartsfirstBarChart() {const that thislet columnar echarts.init…...
C语言控制语句——跳转关键字
循环和switch专属的跳转:break循环专属的跳转:continue无条件跳转:goto break 循环的break说明 某一条件满足时,不再执行循环体中后续重复的代码,并退出循环 需求:一共吃5碗饭, 吃到第3碗吃饱了, 结束吃饭…...
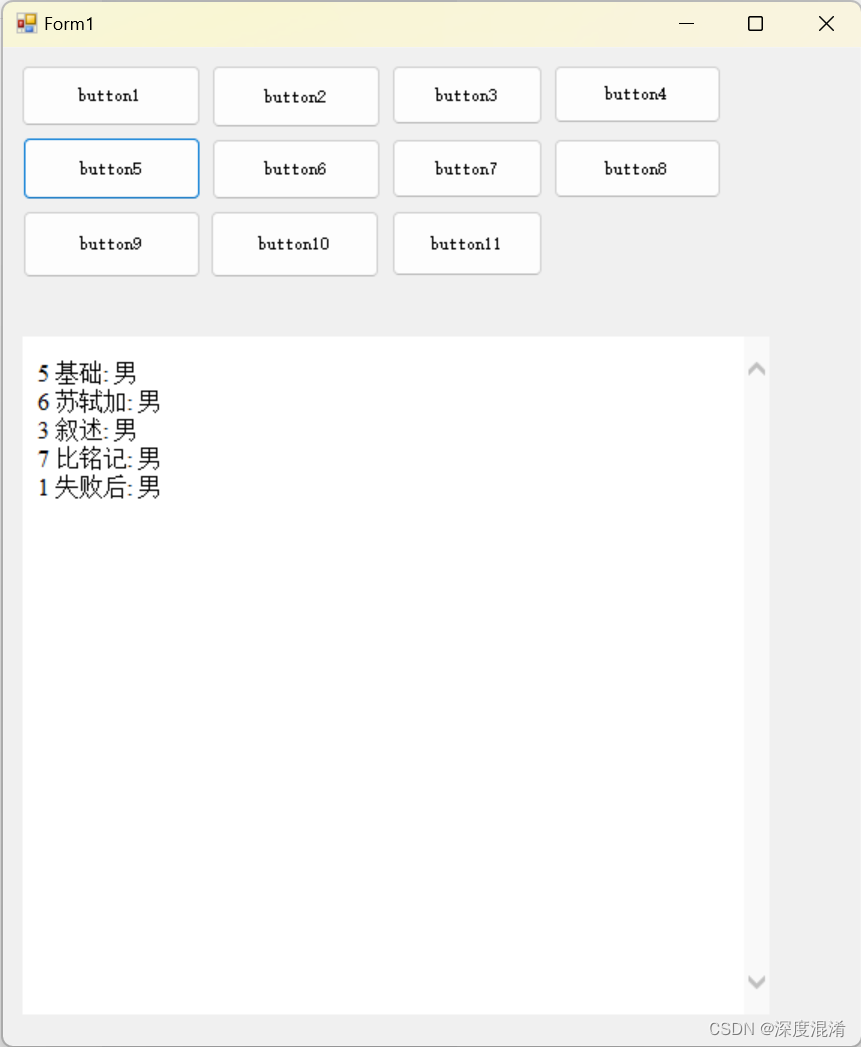
C#,《小白学程序》第五课:队列(Queue)
日常生活中常见的排队,软件怎么体现呢? 排队的基本原则是:先到先得,先到先吃,先进先出 1 文本格式 /// <summary> /// 《小白学程序》第五课:队列(Queue) /// 日常生活中常见…...
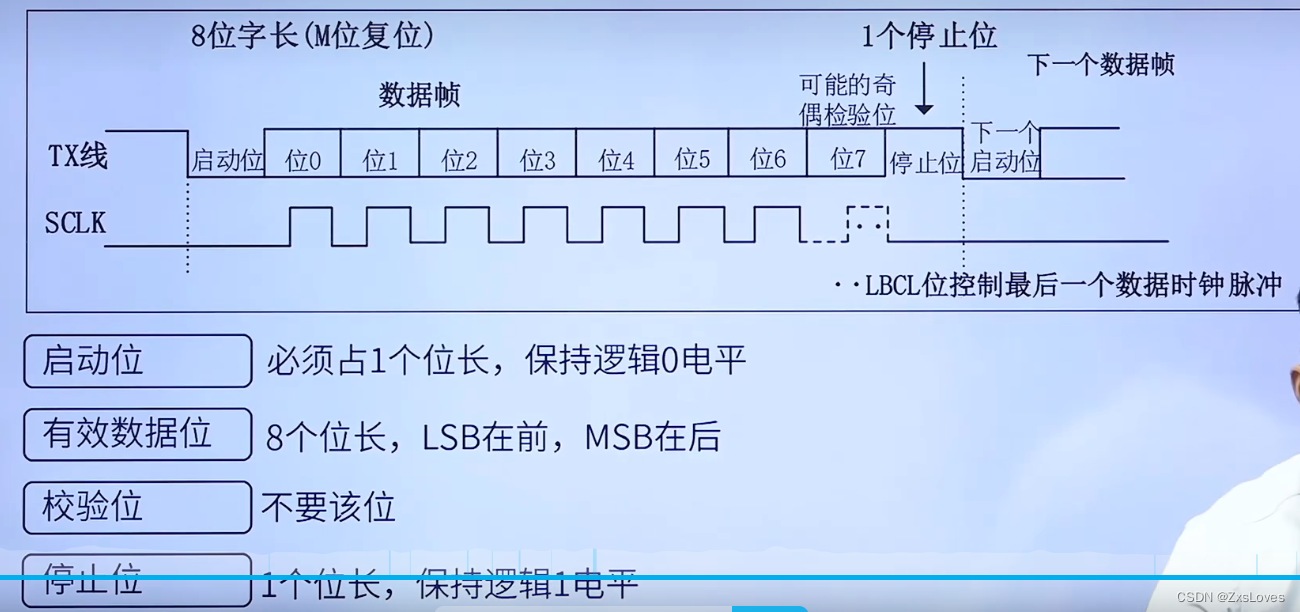
【【萌新的STM32学习25--- USART寄存器的介绍】】
萌新的STM32学习25- USART寄存器的介绍 STM32–USART寄存器介绍(F1) 控制寄存器1 (CR1) 位13: 使能USART UE 0: USART分频器和输出被禁止 1: USART模块使能 位12 : 配置8个数据位…...
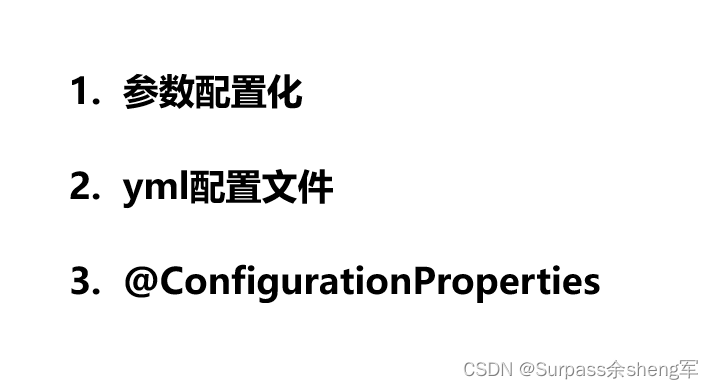
SpringBootWeb案例 Part 5
4. 配置文件 员工管理的增删改查功能我们已开发完成,但在我们所开发的程序中还一些小问题,下面我们就来分析一下当前案例中存在的问题以及如何优化解决。 4.1 参数配置化 在我们之前编写的程序中进行文件上传时,需要调用AliOSSUtils工具类&…...

【ES6】Promise.race的用法
Promise.race()方法同样是将多个 Promise 实例,包装成一个新的 Promise 实例。 const p Promise.race([p1, p2, p3]);上面代码中,只要p1、p2、p3之中有一个实例率先改变状态,p的状态就跟着改变。那个率先改变的 Promise 实例的返回值&#…...
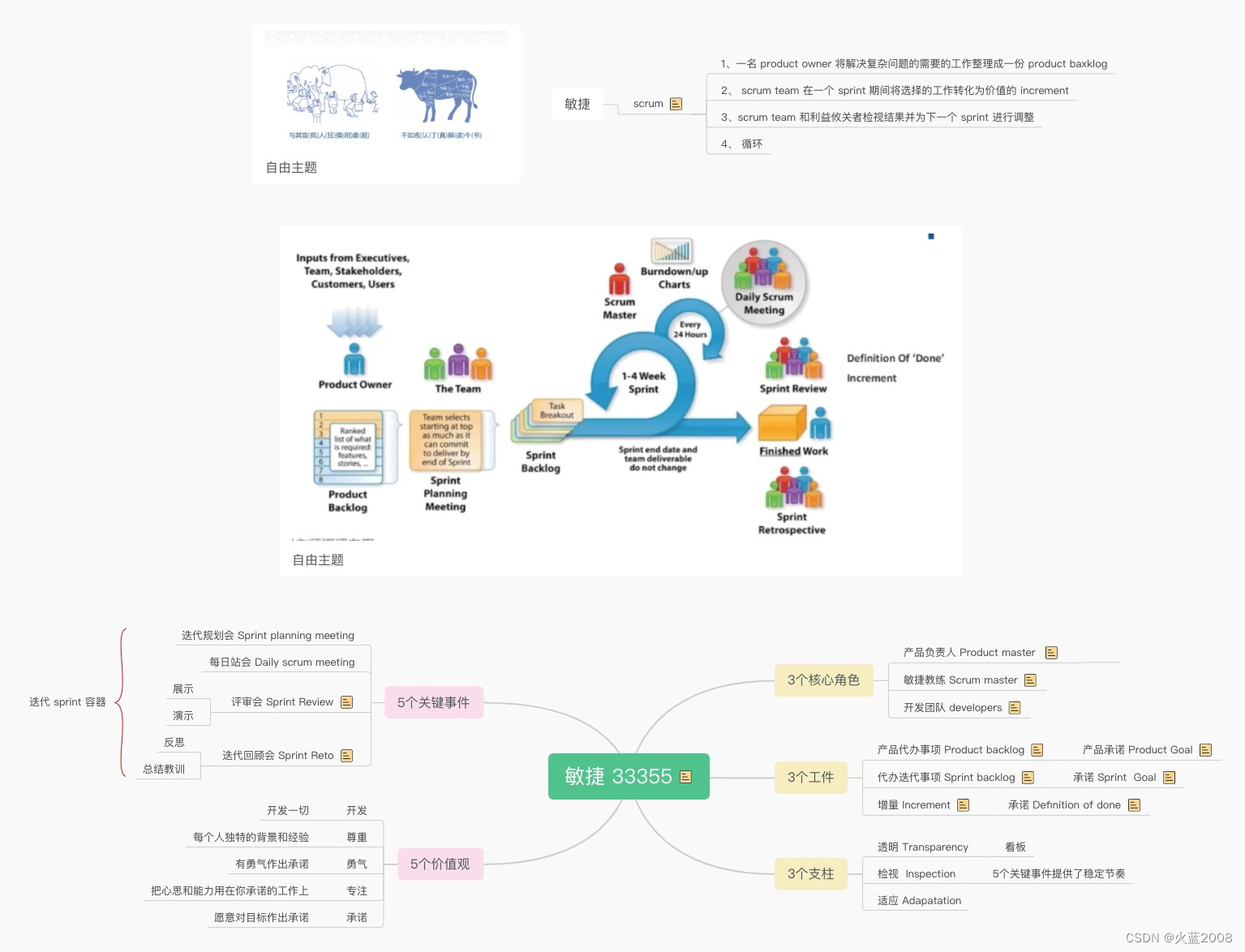
PMP - 敏捷 3355
三个核心 产品负责人 负责最大化投资回报(ROI),通过确定产品特性,把他们翻译成一个有优先级的列表 为下一个 sprint 决定在这个列表中哪些应该优先级最高,并且不断调整优先级以及调整这个列表 职责是定义需求、定义…...
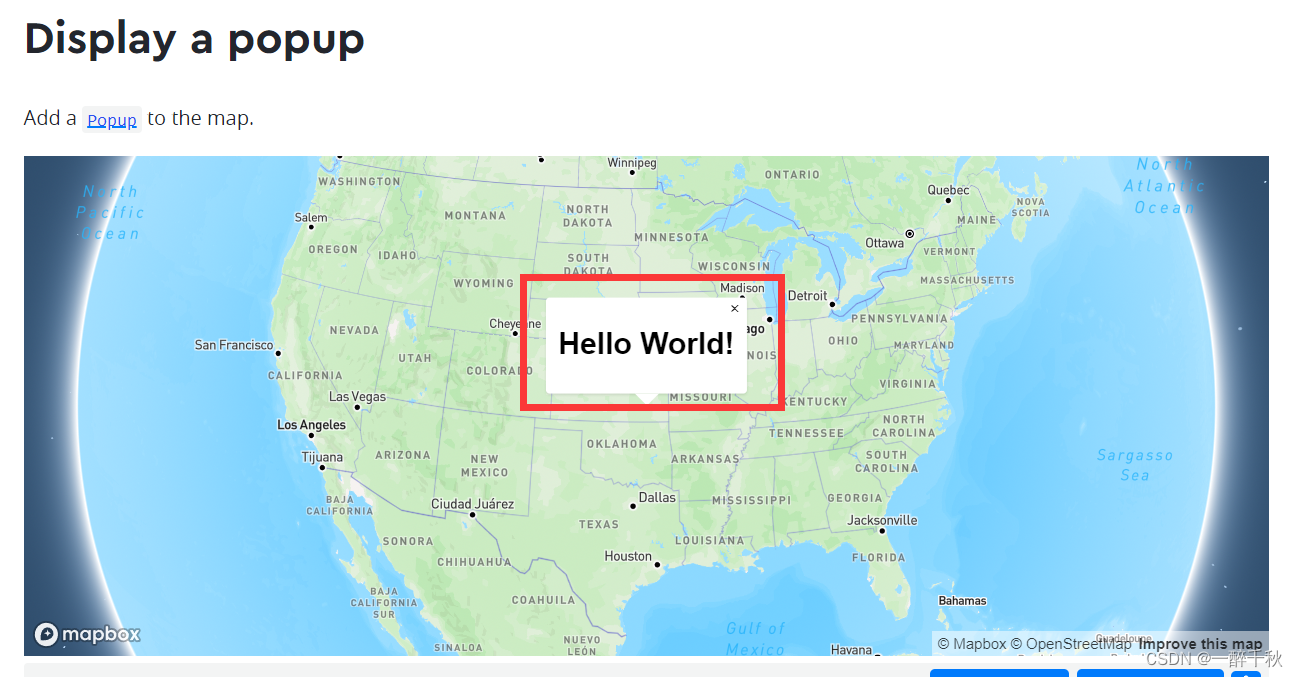
Mapbox-gl 关闭所有Popup,以及关闭按钮出现黑色边框bug
1.官方示例 var popup new mapboxgl.Popup().addTo(map);popup.remove(); 很明显,需要记录popup对象,管理起来比较麻烦。 2.本人采用div的方式关闭所有的popup,在map对象上新增加方法 map.closePopupmapView.popupClear function(){$(&q…...
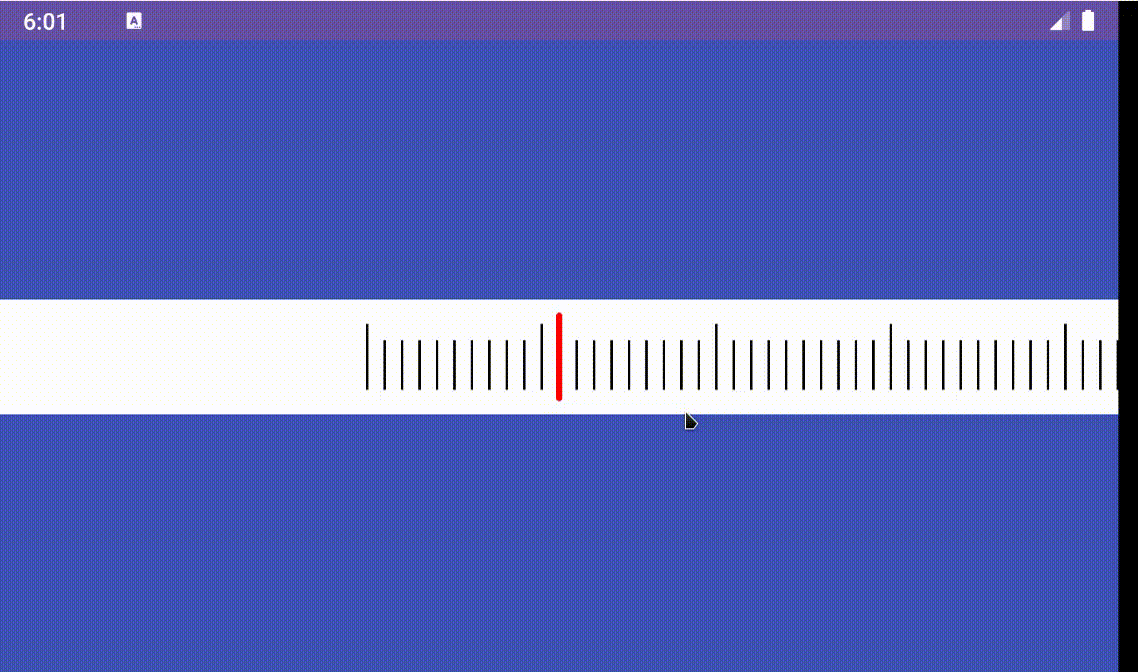
RE:从零开始的车载Android HMI(四) - 收音机刻度尺
最近比较忙,研究复杂的东西需要大量集中的时间,但是又抽不出来,就写点简单的东西吧。车载应用开发中有一个几乎避不开的自定义View,就是收音机的刻度条。本篇文章我们来研究如何绘制一个收音机的刻度尺。 本系列文章的目的是在讲…...
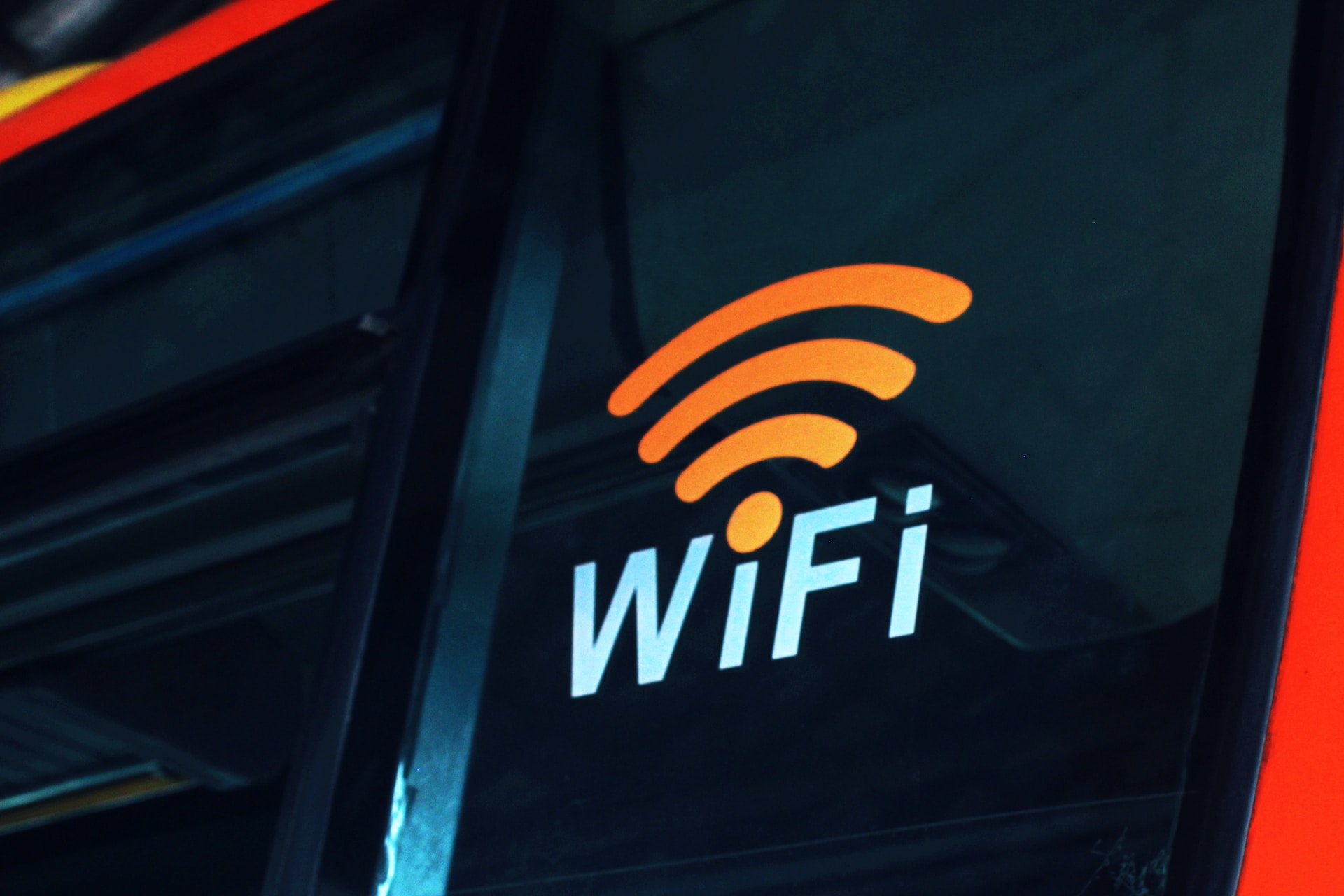
评估安全 Wi-Fi 接入:Cisco ISE、Aruba、Portnox 和 Foxpass
在当今不断变化的数字环境中,对 Wi-Fi 网络进行强大访问控制的需求从未像现在这样重要。各组织一直在寻找能够为其用户提供无缝而安全的体验的解决方案。 在本博客中,我们将深入探讨保护 Wi-Fi(和有线)网络的四种领先解决方案——…...
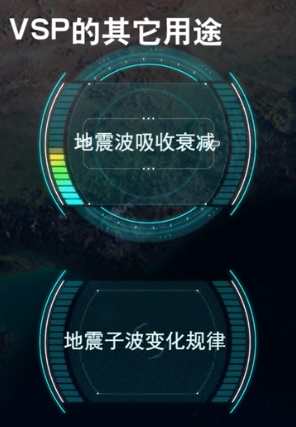
地震勘探——干扰波识别、井中地震时距曲线特点
目录 干扰波识别反射波地震勘探的干扰波 井中地震时距曲线特点 干扰波识别 有效波:可以用来解决所提出的地质任务的波;干扰波:所有妨碍辨认、追踪有效波的其他波。 地震勘探中,有效波和干扰波是相对的。例如,在反射波…...
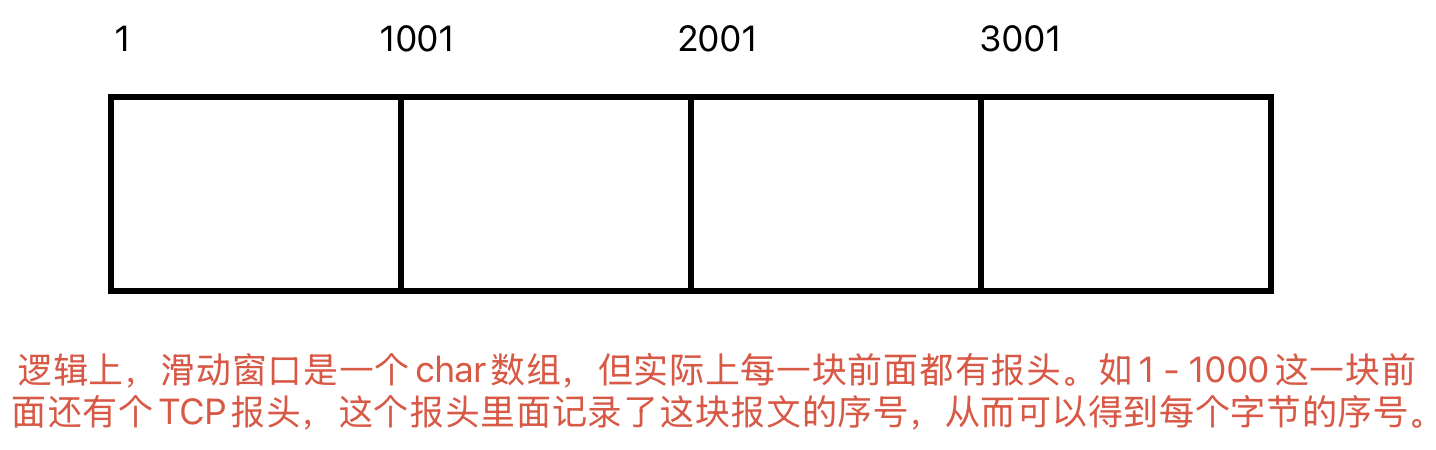
Linux相关概念和易错知识点(42)(TCP的连接管理、可靠性、面临复杂网络的处理)
目录 1.TCP的连接管理机制(1)三次握手①握手过程②对握手过程的理解 (2)四次挥手(3)握手和挥手的触发(4)状态切换①挥手过程中状态的切换②握手过程中状态的切换 2.TCP的可靠性&…...
在Ubuntu中设置开机自动运行(sudo)指令的指南
在Ubuntu系统中,有时需要在系统启动时自动执行某些命令,特别是需要 sudo权限的指令。为了实现这一功能,可以使用多种方法,包括编写Systemd服务、配置 rc.local文件或使用 cron任务计划。本文将详细介绍这些方法,并提供…...
论文解读:交大港大上海AI Lab开源论文 | 宇树机器人多姿态起立控制强化学习框架(一)
宇树机器人多姿态起立控制强化学习框架论文解析 论文解读:交大&港大&上海AI Lab开源论文 | 宇树机器人多姿态起立控制强化学习框架(一) 论文解读:交大&港大&上海AI Lab开源论文 | 宇树机器人多姿态起立控制强化…...
拉力测试cuda pytorch 把 4070显卡拉满
import torch import timedef stress_test_gpu(matrix_size16384, duration300):"""对GPU进行压力测试,通过持续的矩阵乘法来最大化GPU利用率参数:matrix_size: 矩阵维度大小,增大可提高计算复杂度duration: 测试持续时间(秒&…...
现有的 Redis 分布式锁库(如 Redisson)提供了哪些便利?
现有的 Redis 分布式锁库(如 Redisson)相比于开发者自己基于 Redis 命令(如 SETNX, EXPIRE, DEL)手动实现分布式锁,提供了巨大的便利性和健壮性。主要体现在以下几个方面: 原子性保证 (Atomicity)ÿ…...
Go语言多线程问题
打印零与奇偶数(leetcode 1116) 方法1:使用互斥锁和条件变量 package mainimport ("fmt""sync" )type ZeroEvenOdd struct {n intzeroMutex sync.MutexevenMutex sync.MutexoddMutex sync.Mutexcurrent int…...
C语言中提供的第三方库之哈希表实现
一. 简介 前面一篇文章简单学习了C语言中第三方库(uthash库)提供对哈希表的操作,文章如下: C语言中提供的第三方库uthash常用接口-CSDN博客 本文简单学习一下第三方库 uthash库对哈希表的操作。 二. uthash库哈希表操作示例 u…...
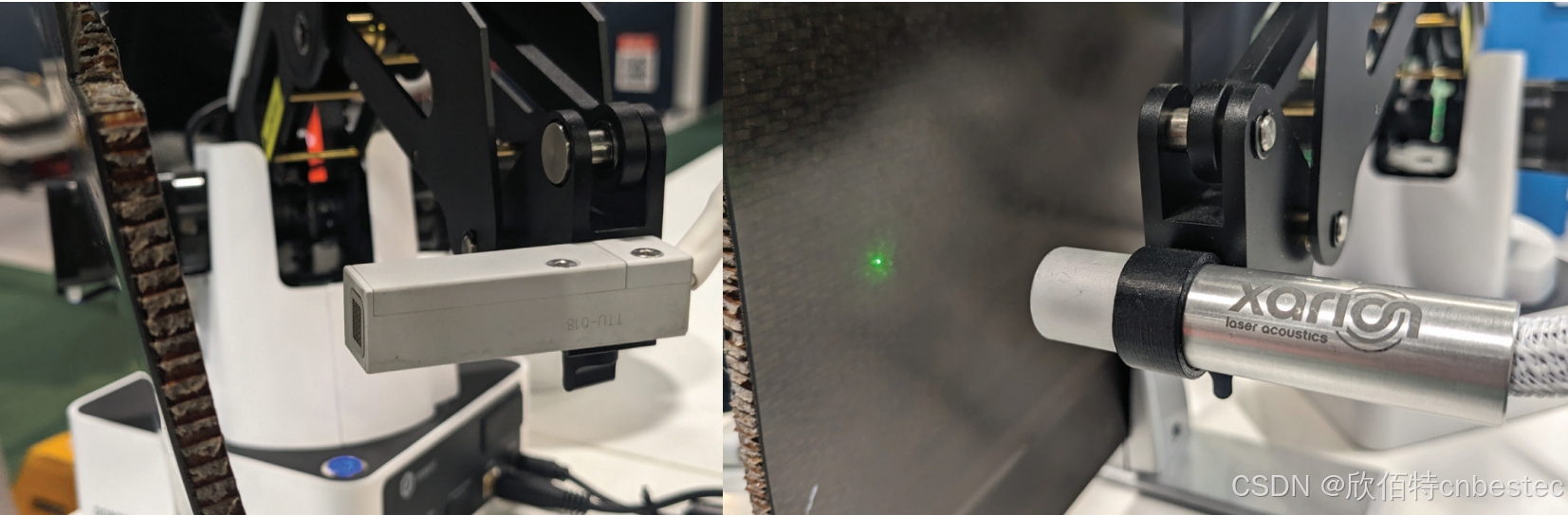
解析奥地利 XARION激光超声检测系统:无膜光学麦克风 + 无耦合剂的技术协同优势及多元应用
在工业制造领域,无损检测(NDT)的精度与效率直接影响产品质量与生产安全。奥地利 XARION开发的激光超声精密检测系统,以非接触式光学麦克风技术为核心,打破传统检测瓶颈,为半导体、航空航天、汽车制造等行业提供了高灵敏…...
适应性Java用于现代 API:REST、GraphQL 和事件驱动
在快速发展的软件开发领域,REST、GraphQL 和事件驱动架构等新的 API 标准对于构建可扩展、高效的系统至关重要。Java 在现代 API 方面以其在企业应用中的稳定性而闻名,不断适应这些现代范式的需求。随着不断发展的生态系统,Java 在现代 API 方…...