【业务功能篇92】微服务-springcloud-多线程-异步处理-异步编排-CompletableFutrue
三、CompletableFutrue
一个商品详情页
- 展示SKU的基本信息 0.5s
- 展示SKU的图片信息 0.6s
- 展示SKU的销售信息 1s
- spu的销售属性 1s
- 展示规格参数 1.5s
- spu详情信息 1s
1.ComplatableFuture介绍
Future是Java 5添加的类,用来描述一个异步计算的结果。你可以使用 isDone
方法检查计算是否完成,或者使用 get
阻塞住调用线程,直到计算完成返回结果,你也可以使用 cancel
方法停止任务的执行。
虽然 Future
以及相关使用方法提供了异步执行任务的能力,但是对于结果的获取却是很不方便,只能通过阻塞或者轮询的方式得到任务的结果。阻塞的方式显然和我们的异步编程的初衷相违背,轮询的方式又会耗费无谓的CPU资源,而且也不能及时地得到计算结果,为什么不能用观察者设计模式当计算结果完成及时通知监听者呢?
很多语言,比如Node.js,采用回调的方式实现异步编程。Java的一些框架,比如Netty,自己扩展了Java的 Future
接口,提供了 addListener
等多个扩展方法;Google guava也提供了通用的扩展Future;Scala也提供了简单易用且功能强大的Future/Promise异步编程模式。
作为正统的Java类库,是不是应该做点什么,加强一下自身库的功能呢?
在Java 8中, 新增加了一个包含50个方法左右的类: CompletableFuture,提供了非常强大的Future的扩展功能,可以帮助我们简化异步编程的复杂性,提供了函数式编程的能力,可以通过回调的方式处理计算结果,并且提供了转换和组合CompletableFuture的方法。
CompletableFuture类实现了Future接口,所以你还是可以像以前一样通过 get
方法阻塞或者轮询的方式获得结果,但是这种方式不推荐使用。
CompletableFuture和FutureTask同属于Future接口的实现类,都可以获取线程的执行结果。
2.创建异步对象
CompletableFuture 提供了四个静态方法来创建一个异步操作。
static CompletableFuture<Void> runAsync(Runnable runnable)
public static CompletableFuture<Void> runAsync(Runnable runnable, Executor executor)
public static <U> CompletableFuture<U> supplyAsync(Supplier<U> supplier)
public static <U> CompletableFuture<U> supplyAsync(Supplier<U> supplier, Executor executor)
方法分为两类:
- runAsync 没有返回结果
- supplyAsync 有返回结果
private static ThreadPoolExecutor executor = new ThreadPoolExecutor(5,50,10, TimeUnit.SECONDS,new LinkedBlockingQueue<>(100), Executors.defaultThreadFactory(),new ThreadPoolExecutor.AbortPolicy());public static void main(String[] args) throws ExecutionException, InterruptedException {System.out.println("main -- 线程开始了...");// 获取CompletableFuture对象CompletableFuture<Void> voidCompletableFuture = CompletableFuture.runAsync(() -> {System.out.println("线程开始了...");int i = 100/50;System.out.println("线程结束了...");},executor);System.out.println("main -- 线程结束了...");System.out.println("------------");CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {System.out.println("线程开始了...");int i = 100 / 50;System.out.println("线程结束了...");return i;}, executor);System.out.println("获取的线程的返回结果是:" + future.get() );}
3.whenXXX和handle方法
当CompletableFuture的计算结果完成,或者抛出异常的时候,可以执行特定的Action。主要是下面的方法:
public CompletableFuture<T> whenComplete(BiConsumer<? super T,? super Throwable> action);
public CompletableFuture<T> whenCompleteAsync(BiConsumer<? super T,? super Throwable> action);
public CompletableFuture<T> whenCompleteAsync(BiConsumer<? super T,? super Throwable> action, Executor executor);public CompletableFuture<T> exceptionally(Function<Throwable,? extends T> fn);public <U> CompletableFuture<U> handle(BiFunction<? super T, Throwable, ? extends U> fn) ;
public <U> CompletableFuture<U> handleAsync(BiFunction<? super T, Throwable, ? extends U> fn) ;
public <U> CompletableFuture<U> handleAsync(BiFunction<? super T, Throwable, ? extends U> fn, Executor executor) ;
相关方法的说明:
- whenComplete 可以获取异步任务的返回值和抛出的异常信息,但是不能修改返回结果
- execptionlly 当异步任务跑出了异常后会触发的方法,如果没有抛出异常该方法不会执行
- handle 可以获取异步任务的返回值和抛出的异常信息,而且可以显示的修改返回的结果
/*** CompletableFuture的介绍*/
public class CompletableFutureDemo2 {private static ThreadPoolExecutor executor = new ThreadPoolExecutor(5,50,10, TimeUnit.SECONDS,new LinkedBlockingQueue<>(100), Executors.defaultThreadFactory(),new ThreadPoolExecutor.AbortPolicy());public static void main(String[] args) throws ExecutionException, InterruptedException {CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {System.out.println("线程开始了...");int i = 100 / 5;System.out.println("线程结束了...");return i;}, executor).handle((res,exec)->{System.out.println("res = " + res + ":exec="+exec);return res * 10;});// 可以处理异步任务之后的操作System.out.println("获取的线程的返回结果是:" + future.get() );}/* public static void main(String[] args) throws ExecutionException, InterruptedException {CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {System.out.println("线程开始了...");int i = 100 / 5;System.out.println("线程结束了...");return i;}, executor).whenCompleteAsync((res,exec)->{System.out.println("res = " + res);System.out.println("exec = " + exec);}).exceptionally((res)->{ // 在异步任务显示的抛出了异常后才会触发的方法System.out.println("res = " + res);return 10;});// 可以处理异步任务之后的操作System.out.println("获取的线程的返回结果是:" + future.get() );}*//* public static void main(String[] args) throws ExecutionException, InterruptedException {CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {System.out.println("线程开始了...");int i = 100 / 0;System.out.println("线程结束了...");return i;}, executor).whenCompleteAsync((res,exec)->{System.out.println("res = " + res);System.out.println("exec = " + exec);});// 可以处理异步任务之后的操作System.out.println("获取的线程的返回结果是:" + future.get() );}*/
}
4.线程串行方法
thenApply 方法:当一个线程依赖另一个线程时,获取上一个任务返回的结果,并返回当前任务的返回值。
thenAccept方法:消费处理结果。接收任务的处理结果,并消费处理,无返回结果。
thenRun方法:只要上面的任务执行完成,就开始执行thenRun,只是处理完任务后,执行 thenRun的后续操作
带有Async默认是异步执行的。这里所谓的异步指的是不在当前线程内执行。
public <U> CompletableFuture<U> thenApply(Function<? super T,? extends U> fn)
public <U> CompletableFuture<U> thenApplyAsync(Function<? super T,? extends U> fn)
public <U> CompletableFuture<U> thenApplyAsync(Function<? super T,? extends U> fn, Executor executor)public CompletionStage<Void> thenAccept(Consumer<? super T> action);
public CompletionStage<Void> thenAcceptAsync(Consumer<? super T> action);
public CompletionStage<Void> thenAcceptAsync(Consumer<? super T> action,Executor executor);public CompletionStage<Void> thenRun(Runnable action);
public CompletionStage<Void> thenRunAsync(Runnable action);
public CompletionStage<Void> thenRunAsync(Runnable action,Executor executor);
/*** CompletableFuture的介绍*/
public class CompletableFutureDemo3 {private static ThreadPoolExecutor executor = new ThreadPoolExecutor(5,50,10, TimeUnit.SECONDS,new LinkedBlockingQueue<>(100), Executors.defaultThreadFactory(),new ThreadPoolExecutor.AbortPolicy());/*** 线程串行的方法* thenRun:在前一个线程执行完成后,开始执行,不会获取前一个线程的返回结果,也不会返回信息* thenAccept:在前一个线程执行完成后,开始执行,获取前一个线程的返回结果,不会返回信息* thenApply: 在前一个线程执行完成后。开始执行,获取前一个线程的返回结果,同时也会返回信息* @param args* @throws ExecutionException* @throws InterruptedException*/public static void main(String[] args) throws ExecutionException, InterruptedException {CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {System.out.println("线程开始了..." + Thread.currentThread().getName());int i = 100 / 5;System.out.println("线程结束了..." + Thread.currentThread().getName());return i;}, executor).thenApply(res -> {System.out.println("res = " + res);return res * 100;});// 可以处理异步任务之后的操作System.out.println("获取的线程的返回结果是:" + future.get() );}/*public static void main(String[] args) throws ExecutionException, InterruptedException {CompletableFuture<Void> voidCompletableFuture = CompletableFuture.supplyAsync(() -> {System.out.println("线程开始了..." + Thread.currentThread().getName());int i = 100 / 5;System.out.println("线程结束了..." + Thread.currentThread().getName());return i;}, executor).thenAcceptAsync(res -> {System.out.println(res + ":" + Thread.currentThread().getName());}, executor);// 可以处理异步任务之后的操作//System.out.println("获取的线程的返回结果是:" + future.get() );}*//*public static void main(String[] args) throws ExecutionException, InterruptedException {CompletableFuture<Void> voidCompletableFuture = CompletableFuture.supplyAsync(() -> {System.out.println("线程开始了..."+Thread.currentThread().getName());int i = 100 / 5;System.out.println("线程结束了..."+Thread.currentThread().getName());return i;}, executor).thenRunAsync(() -> {System.out.println("线程开始了..."+Thread.currentThread().getName());int i = 100 / 5;System.out.println("线程结束了..."+Thread.currentThread().getName());}, executor);// 可以处理异步任务之后的操作//System.out.println("获取的线程的返回结果是:" + future.get() );}*/}
5.两个都完成
上面介绍的相关方法都是串行的执行,接下来看看需要等待两个任务执行完成后才会触发的几个方法
- thenCombine :可以获取前面两线程的返回结果,本身也有返回结果
- thenAcceptBoth:可以获取前面两线程的返回结果,本身没有返回结果
- runAfterBoth:不可以获取前面两线程的返回结果,本身也没有返回结果
/*** @param args* @throws ExecutionException* @throws InterruptedException*/public static void main(String[] args) throws ExecutionException, InterruptedException {CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> {System.out.println("任务1 线程开始了..." + Thread.currentThread().getName());int i = 100 / 5;System.out.println("任务1 线程结束了..." + Thread.currentThread().getName());return i;}, executor);CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> {System.out.println("任务2 线程开始了..." + Thread.currentThread().getName());int i = 100 /10;System.out.println("任务2 线程结束了..." + Thread.currentThread().getName());return i;}, executor);// runAfterBothAsync 不能获取前面两个线程的返回结果,本身也没有返回结果CompletableFuture<Void> voidCompletableFuture = future1.runAfterBothAsync(future2, () -> {System.out.println("任务3执行了");},executor);// thenAcceptBothAsync 可以获取前面两个线程的返回结果,本身没有返回结果CompletableFuture<Void> voidCompletableFuture1 = future1.thenAcceptBothAsync(future2, (f1, f2) -> {System.out.println("f1 = " + f1);System.out.println("f2 = " + f2);}, executor);// thenCombineAsync: 既可以获取前面两个线程的返回结果,同时也会返回结果给阻塞的线程CompletableFuture<String> stringCompletableFuture = future1.thenCombineAsync(future2, (f1, f2) -> {return f1 + ":" + f2;}, executor);// 可以处理异步任务之后的操作System.out.println("获取的线程的返回结果是:" + stringCompletableFuture.get() );}
6.两个任务完成一个
在上面5个基础上我们来看看两个任务只要有一个完成就会触发任务3的情况
- runAfterEither:不能获取完成的线程的返回结果,自身也没有返回结果
- acceptEither:可以获取线程的返回结果,自身没有返回结果
- applyToEither:既可以获取线程的返回结果,自身也有返回结果
/*** @param args* @throws ExecutionException* @throws InterruptedException*/public static void main(String[] args) throws ExecutionException, InterruptedException {CompletableFuture<Object> future1 = CompletableFuture.supplyAsync(() -> {System.out.println("任务1 线程开始了..." + Thread.currentThread().getName());int i = 100 / 5;System.out.println("任务1 线程结束了..." + Thread.currentThread().getName());return i;}, executor);CompletableFuture<Object> future2 = CompletableFuture.supplyAsync(() -> {System.out.println("任务2 线程开始了..." + Thread.currentThread().getName());int i = 100 /10;try {Thread.sleep(5000);} catch (InterruptedException e) {e.printStackTrace();}System.out.println("任务2 线程结束了..." + Thread.currentThread().getName());return i+"";}, executor);// runAfterEitherAsync 不能获取前面完成的线程的返回结果,自身也没有返回结果future1.runAfterEitherAsync(future2,()->{System.out.println("任务3执行了....");},executor);// acceptEitherAsync 可以获取前面完成的线程的返回结果 自身没有返回结果future1.acceptEitherAsync(future2,(res)->{System.out.println("res = " + res);},executor);// applyToEitherAsync 既可以获取完成任务的线程的返回结果 自身也有返回结果CompletableFuture<String> stringCompletableFuture = future1.applyToEitherAsync(future2, (res) -> {System.out.println("res = " + res);return res + "-->OK";}, executor);// 可以处理异步任务之后的操作System.out.println("获取的线程的返回结果是:" + stringCompletableFuture.get() );}
7.多任务组合
allOf:等待所有任务完成
anyOf:只要有一个任务完成
public static CompletableFuture<Void> allOf(CompletableFuture<?>... cfs);public static CompletableFuture<Object> anyOf(CompletableFuture<?>... cfs);
/*** @param args* @throws ExecutionException* @throws InterruptedException*/public static void main(String[] args) throws ExecutionException, InterruptedException {CompletableFuture<Object> future1 = CompletableFuture.supplyAsync(() -> {System.out.println("任务1 线程开始了..." + Thread.currentThread().getName());int i = 100 / 5;System.out.println("任务1 线程结束了..." + Thread.currentThread().getName());return i;}, executor);CompletableFuture<Object> future2 = CompletableFuture.supplyAsync(() -> {System.out.println("任务2 线程开始了..." + Thread.currentThread().getName());int i = 100 /10;try {Thread.sleep(5000);} catch (InterruptedException e) {e.printStackTrace();}System.out.println("任务2 线程结束了..." + Thread.currentThread().getName());return i+"";}, executor);CompletableFuture<Object> future3 = CompletableFuture.supplyAsync(() -> {System.out.println("任务3 线程开始了..." + Thread.currentThread().getName());int i = 100 /10;System.out.println("任务3 线程结束了..." + Thread.currentThread().getName());return i+"";}, executor);CompletableFuture<Object> anyOf = CompletableFuture.anyOf(future1, future2, future3);anyOf.get();System.out.println("主任务执行完成..." + anyOf.get());CompletableFuture<Void> allOf = CompletableFuture.allOf(future1, future2, future3);allOf.get();// 阻塞在这个位置,等待所有的任务执行完成System.out.println("主任务执行完成..." + future1.get() + " :" + future2.get() + " :" + future3.get());}
相关文章:
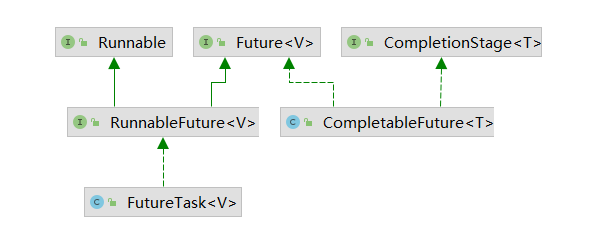
【业务功能篇92】微服务-springcloud-多线程-异步处理-异步编排-CompletableFutrue
三、CompletableFutrue 一个商品详情页 展示SKU的基本信息 0.5s展示SKU的图片信息 0.6s展示SKU的销售信息 1sspu的销售属性 1s展示规格参数 1.5sspu详情信息 1s 1.ComplatableFuture介绍 Future是Java 5添加的类,用来描述一个异步计算的结果。你可以使用 isDone方…...
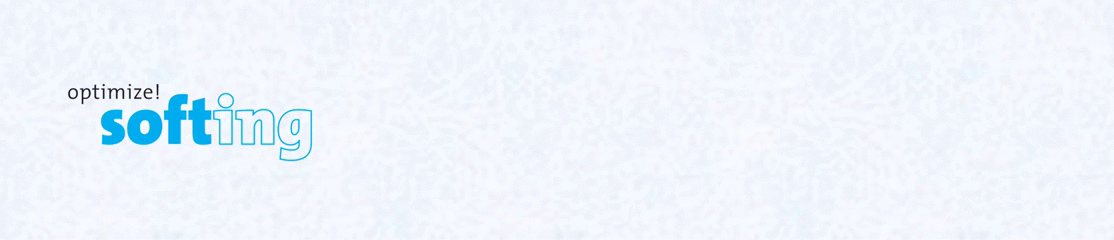
CAN FD的一致性测试 助力汽车电子智能化
后起之秀——CAN FD:随着各个行业的快速发展,消费者对汽车电子智能化的诉求越来越强烈,这使得整车厂将越来越多的电子控制系统加入到了汽车控制中,且在传统汽车、新能源汽车、ADAS和自动驾驶等汽车领域中也无不催生着更高的需求&a…...
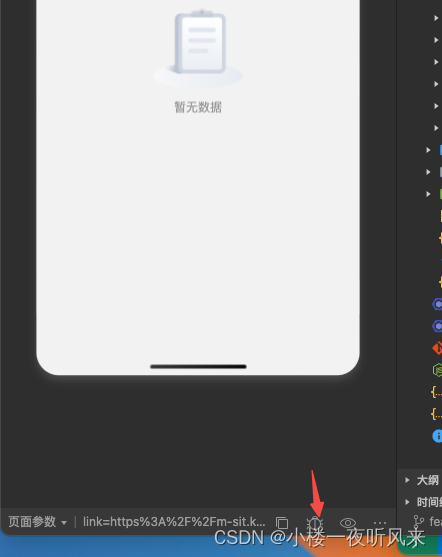
微信短链跳转到小程序指定页面调试
首先说下背景:后端给了短链地址,但是无法跳转到指定页面。总是在小程序首页。指定的页面我们是h5页面。排查步骤如下: 1、通过快速URL Scheme 编译。上部普通编译 下拉找到此选项。 、 2、按照小程序的要求的URL Scheme输入。另外后端给的…...
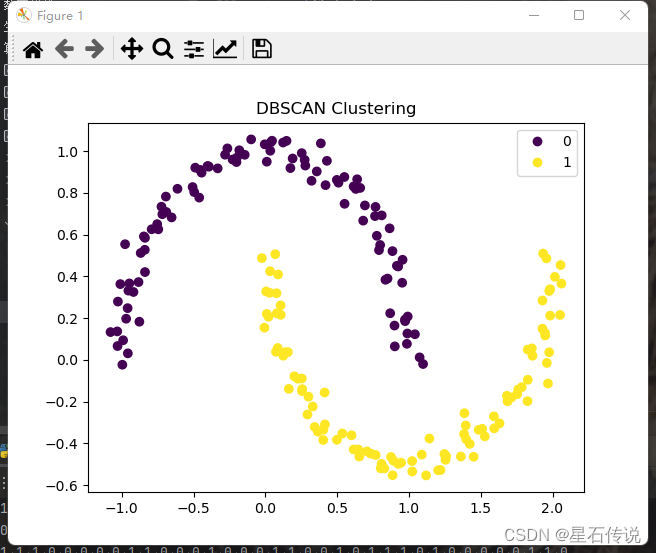
机器学习——聚类算法一
机器学习——聚类算法一 文章目录 前言一、基于numpy实现聚类二、K-Means聚类2.1. 原理2.2. 代码实现2.3. 局限性 三、层次聚类3.1. 原理3.2. 代码实现 四、DBSCAN算法4.1. 原理4.2. 代码实现 五、区别与相同点1. 区别:2. 相同点: 总结 前言 在机器学习…...
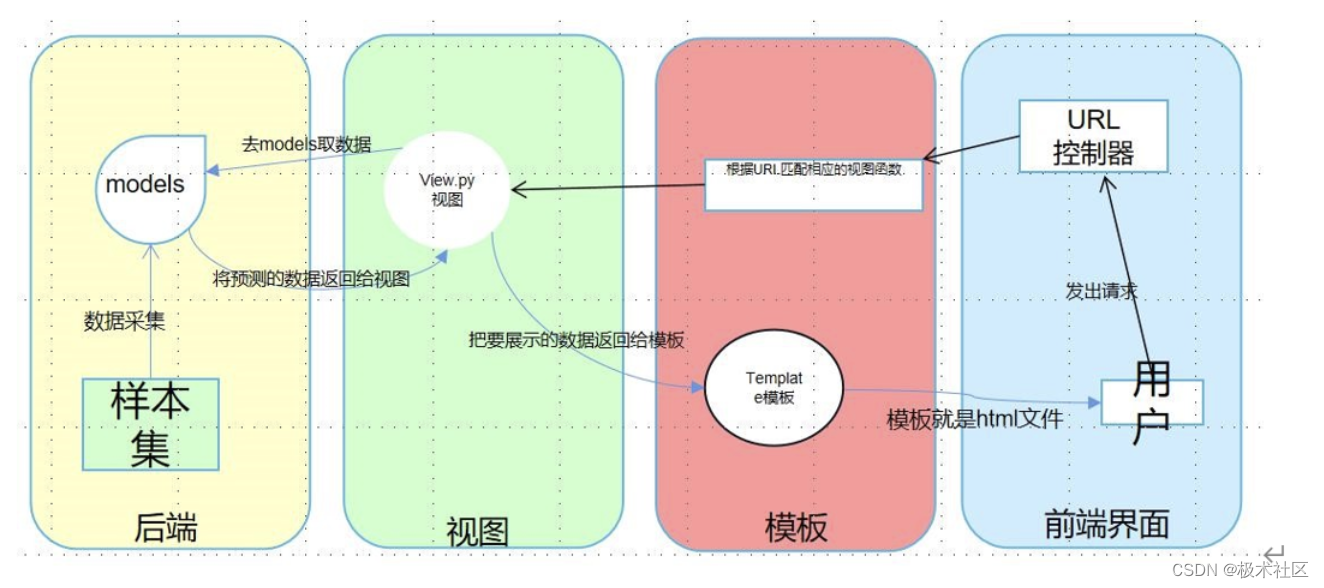
【2023研电赛】安谋科技企业命题三等奖作品: 短临天气预报AI云图分析系统
本文为2023年第十八届中国研究生电子设计竞赛安谋科技企业命题三等奖分享,参加极术社区的【有奖活动】分享2023研电赛作品扩大影响力,更有丰富电子礼品等你来领!,分享2023研电赛作品扩大影响力,更有丰富电子礼品等你来…...
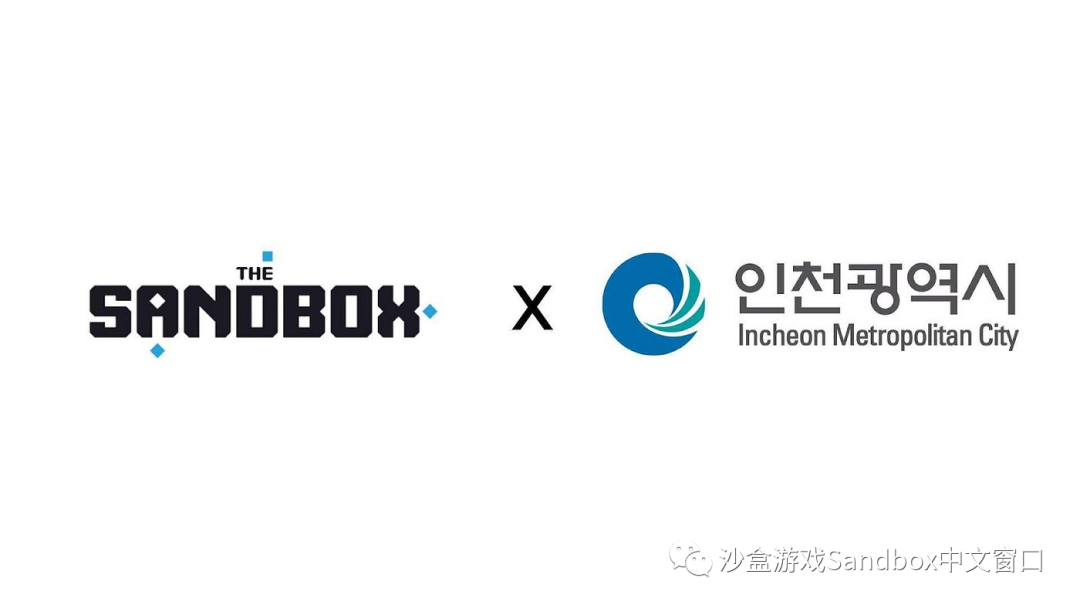
The Sandbox 与韩国仁川市合作,打造身临其境的城市体验内容
简要概括 ● The Sandbox 与仁川市联手展示城市魅力,打造创新形象。 ● 本次合作包含多种多样的活动,如 NFT 捐赠活动和针对元宇宙创作者的培训计划。 我们非常高兴地宣布与仁川市合作,共同打造身临其境的城市体验。 双方合作的目的是在国…...
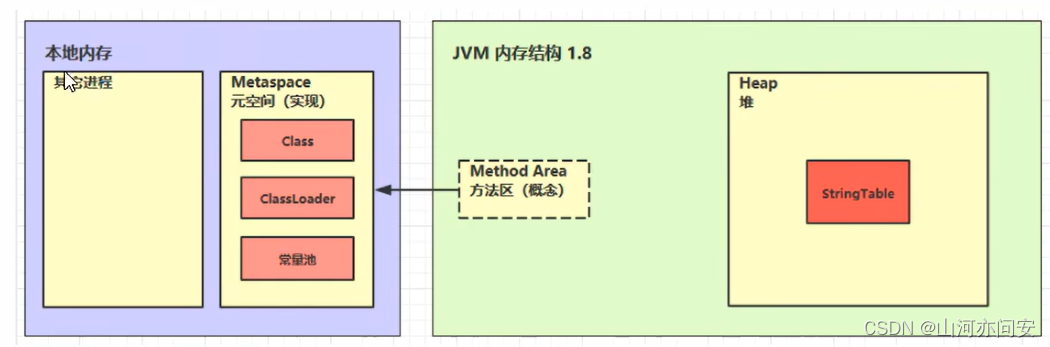
JVM之堆和方法区
目录 1.堆 1.1 堆的结构 1.1.1 新生代(Young Generation) 1.1.2 年老代(Old Generation) 1.1.3 永久代/元空间(Permanent Generation/Metaspace) 1.2 堆的内存溢出 1.3 堆内存诊断 1.3.1 jmap 1.3.2…...
Java 中的 IO 和 NIO
Java 中的 IO 和 NIO Java IO 介绍Java NIO(New IO)介绍windows 安装 ffmpeg完整示例参考文献 Java IO 介绍 Java IO(Input/Output)流是用于处理输入和输出数据的机制。它提供了一种标准化的方式来读取和写入数据,可以…...

Linux-crontab使用问题解决
添加定时进程 终端输入: crontab -e选择文本编辑方式,写入要运行的脚本,以及时间要求。 注意,如果有多个运行指令分两种情况: 1.多个运行指令之间没有耦合关系,分别独立,则可以直接分为两个…...
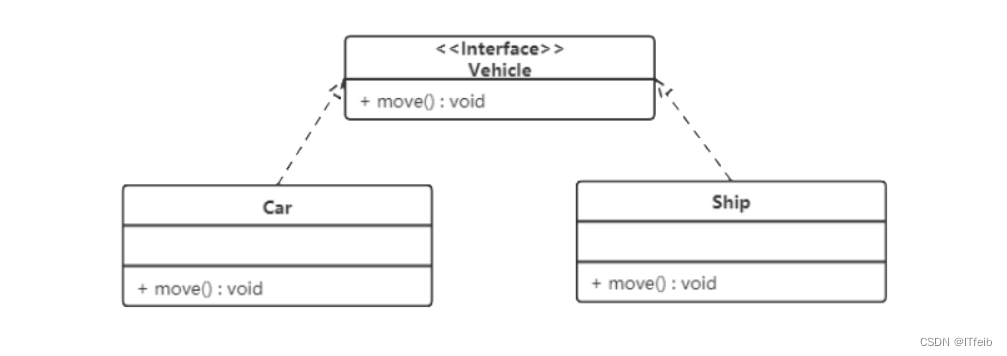
【设计模式】
文章目录 设计模式分类UML图类与类之间关系的表示方式 设计原则 设计模式分类 创建型模式 用于描述“怎样创建对象”,它的主要特点是“将对象的创建与使用分离”。单例、原型、工厂、抽象工厂、建造者等 5 种创建型模式。 结构型模式 用于描述如何将类或对象按某种…...
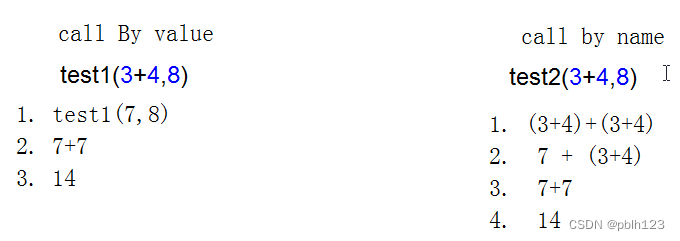
2023_Spark_实验四:SCALA基础
一、在IDEA中执行以下语句 或者用windows徽标R 输入cmd 进入命令提示符 输入scala直接进入编写界面 1、Scala的常用数据类型 注意:在Scala中,任何数据都是对象。例如: scala> 1 res0: Int 1scala> 1.toString res1: String 1scala…...
【深入解析spring cloud gateway】04 Global Filters
上一节学习了GatewayFilter。 回忆一下一个关键点: GateWayFilterFactory的本质就是:针对配置进行解析,为指定的路由,添加Filter,以便对请求报文进行处理。 一、原理分析 GlobalFilter又是啥?先看一下接口…...
c++搜索基础进阶
搜索算法基础 搜索算法是利用计算机的高性能来有目的的穷举一个问题的部分或所有的可能情况,从而求出问题的解的一种方法。搜索过程实际上是根据初始条件和扩展规则构造一棵解答树并寻找符合目标状态的节点的过程。 所有的搜索算法从其最终的算法实现上来看&#…...
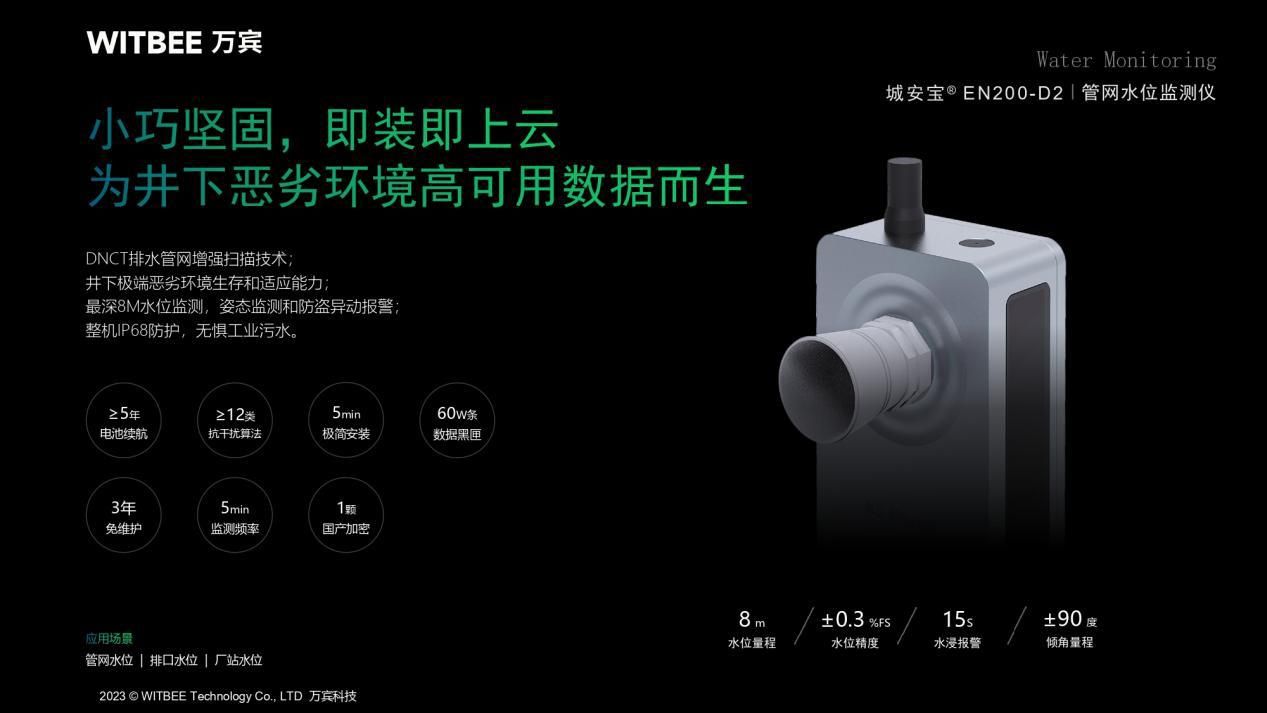
管网水位监测的必要性
城市燃气、桥梁、供水、排水、热力、电力、电梯、通信、轨道交通、综合管廊、输油管线等,担负着城市的信息传递、能源输送、排涝减灾等重要任务,是维系城市正常运行、满足群众生产生活需要的重要基础设施,是城市的生命线。基础设施生命线就像…...

无涯教程-Android - 系统架构
Android操作系统是一堆软件组件,大致分为五个部分和四个主要层,如体系结构图中所示。 Linux内核 底层是Linux-Linux 3.6,带有大约115个补丁,这在设备硬件之间提供了一定程度的抽象,并且包含所有必需的硬件驱动程序&am…...
await接受成功的promise,失败的promise用try catch
在 JavaScript 中,await 关键字用于等待一个 Promise 对象的解决(fulfillment)。下面是一个示例: async function example() {try {const result await doSomethingAsync();console.log(result); // 如果 Promise 成功解决&…...
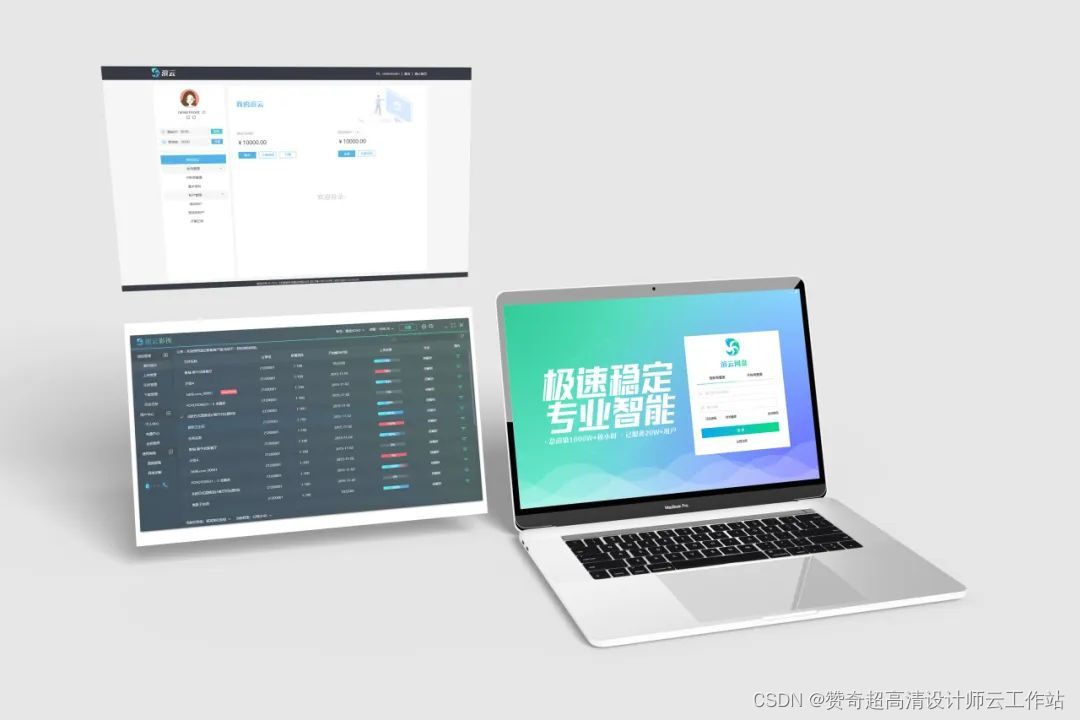
赞奇科技参与华为云828 B2B企业节,云工作站入选精选产品解决方案
8月27日,由华为云携手上万家伙伴共同发起的第二届 828 B2B 企业节拉开帷幕,围绕五大系列活动,为万千中小企业带来精细化商机对接。 聚焦行业数字化所需最优产品,举办超1000场供需对接会,遍及20多个省100多个城市&…...
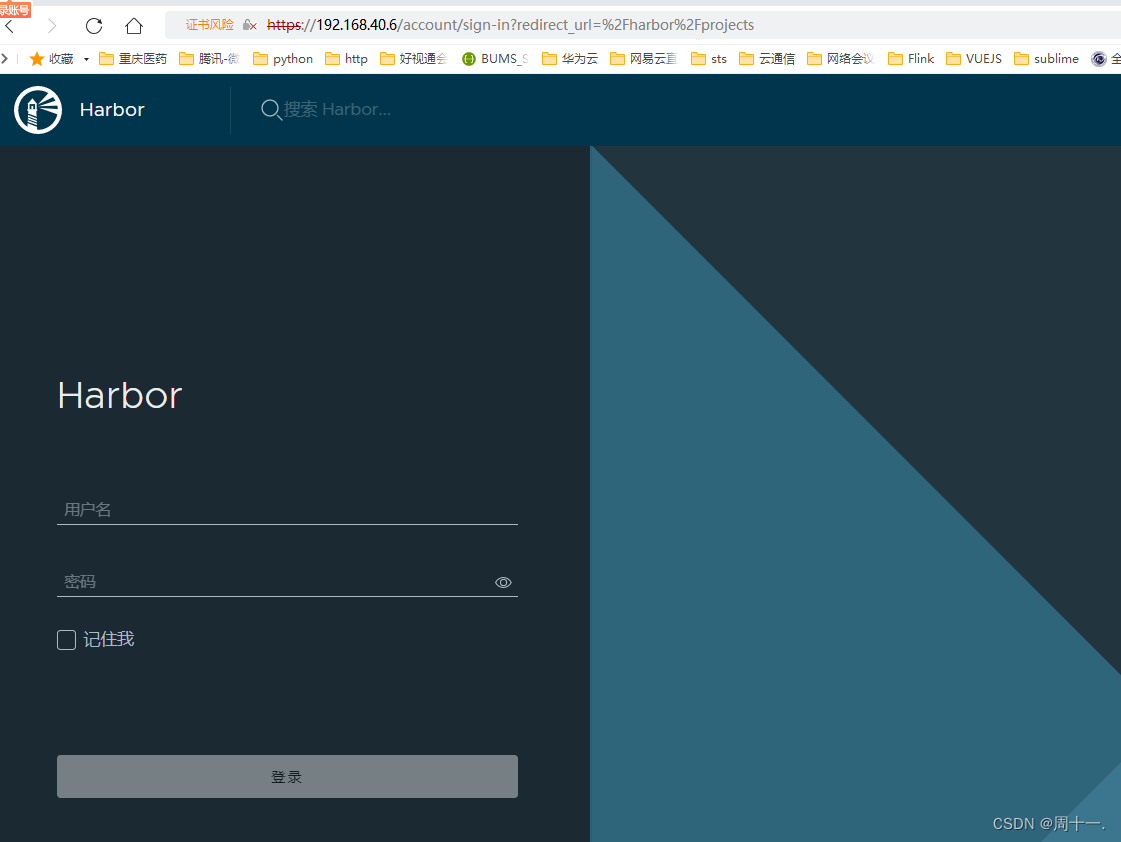
Docker私有镜像仓库(Harbor)安装
Docker私有镜像仓库(Harbor)安装 1、什么是Harbor Harbor是类似与DockerHub 一样的镜像仓库。Harbor是由VMware公司开源的企业级的Docker Registry管理项目,它包括权限管理(RBAC)、LDAP、日志审核、管理界面、自我注册、镜像复制和中文支持等功能。Docker容器应用的…...
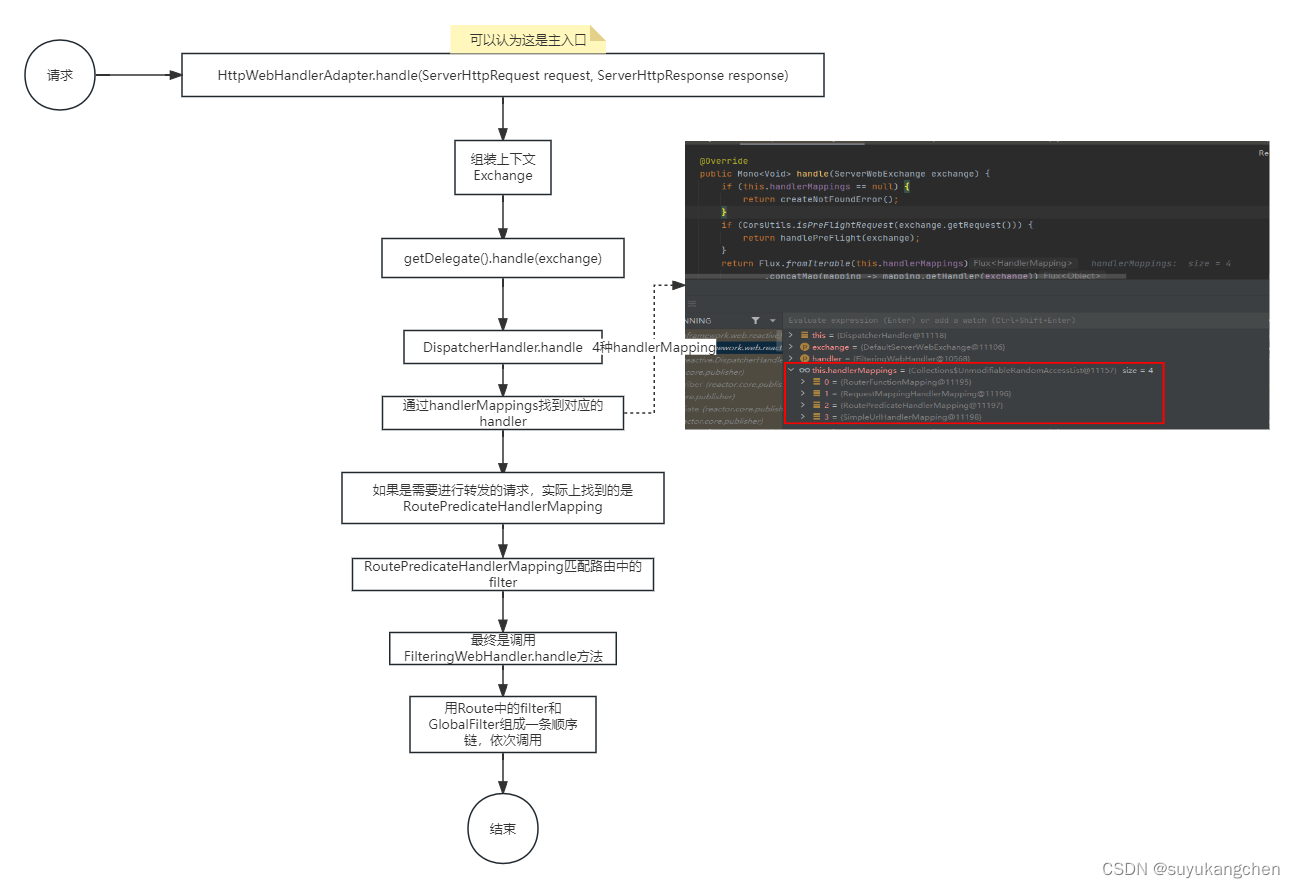
【深入解析spring cloud gateway】06 gateway源码简要分析
上一节做了一个很简单的示例,微服务通过注册到eureka上,然后网关通过服务发现访问到对应的微服务。本节将简单地对整个gateway请求转发过程做一个简单的分析。 一、核心流程 主要流程: Gateway Client向 Spring Cloud Gateway 发送请求请求…...
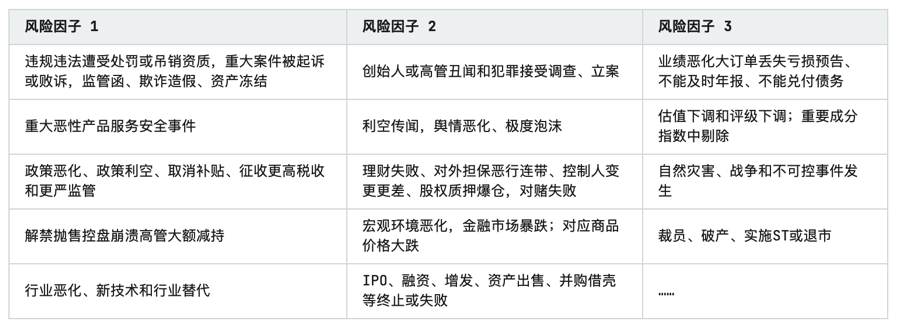
2023年行研行业研究报告
第一章 行业概述 1.1 行研行业 行业定义为同一类别的经济活动,这涉及生产相似产品、应用相同生产工艺或提供同类服务的集合,如食品饮料行业、服饰行业、机械制造行业、金融服务行业和移动互联网行业等。 为满足全球金融业的需求,1999年8月…...
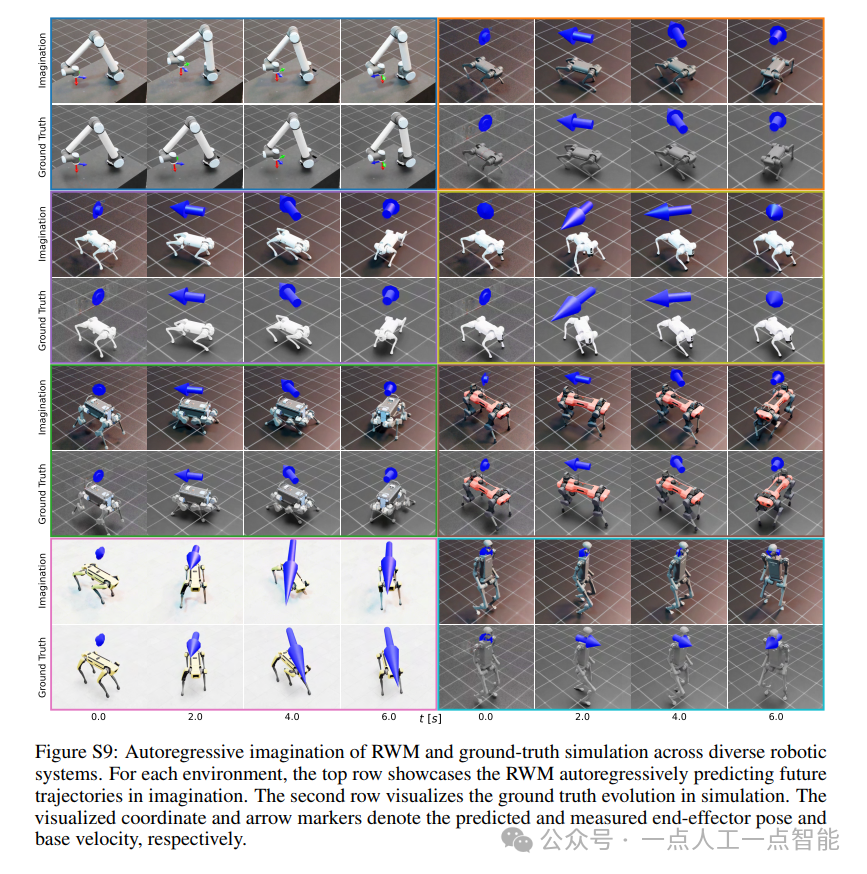
未来机器人的大脑:如何用神经网络模拟器实现更智能的决策?
编辑:陈萍萍的公主一点人工一点智能 未来机器人的大脑:如何用神经网络模拟器实现更智能的决策?RWM通过双自回归机制有效解决了复合误差、部分可观测性和随机动力学等关键挑战,在不依赖领域特定归纳偏见的条件下实现了卓越的预测准…...
HTML 语义化
目录 HTML 语义化HTML5 新特性HTML 语义化的好处语义化标签的使用场景最佳实践 HTML 语义化 HTML5 新特性 标准答案: 语义化标签: <header>:页头<nav>:导航<main>:主要内容<article>&#x…...
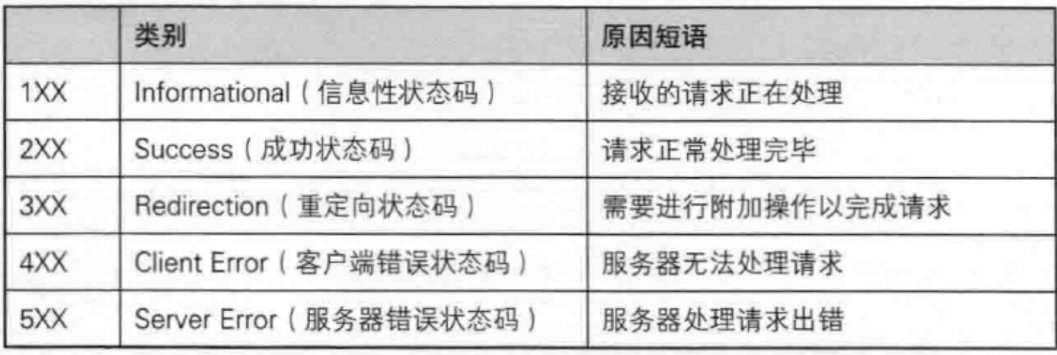
【JavaEE】-- HTTP
1. HTTP是什么? HTTP(全称为"超文本传输协议")是一种应用非常广泛的应用层协议,HTTP是基于TCP协议的一种应用层协议。 应用层协议:是计算机网络协议栈中最高层的协议,它定义了运行在不同主机上…...
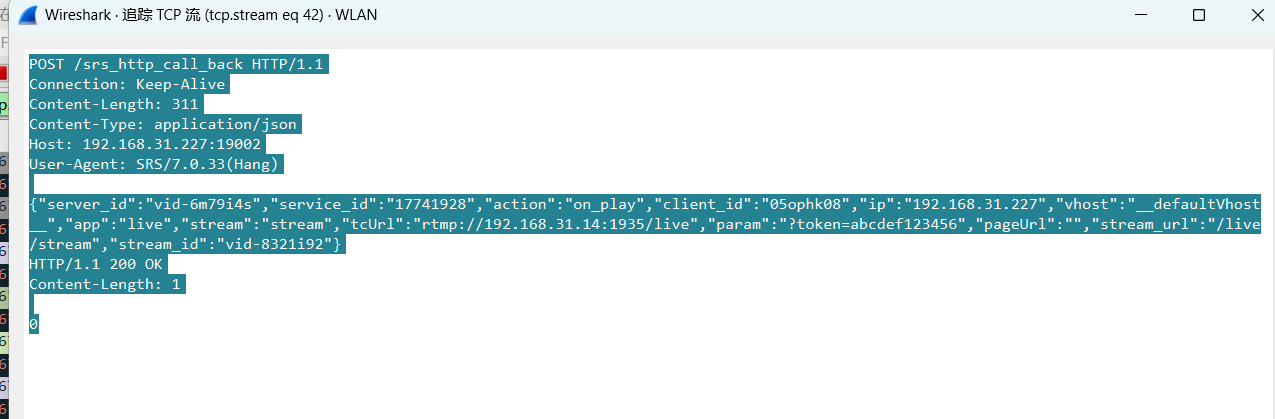
srs linux
下载编译运行 git clone https:///ossrs/srs.git ./configure --h265on make 编译完成后即可启动SRS # 启动 ./objs/srs -c conf/srs.conf # 查看日志 tail -n 30 -f ./objs/srs.log 开放端口 默认RTMP接收推流端口是1935,SRS管理页面端口是8080,可…...
ffmpeg(四):滤镜命令
FFmpeg 的滤镜命令是用于音视频处理中的强大工具,可以完成剪裁、缩放、加水印、调色、合成、旋转、模糊、叠加字幕等复杂的操作。其核心语法格式一般如下: ffmpeg -i input.mp4 -vf "滤镜参数" output.mp4或者带音频滤镜: ffmpeg…...
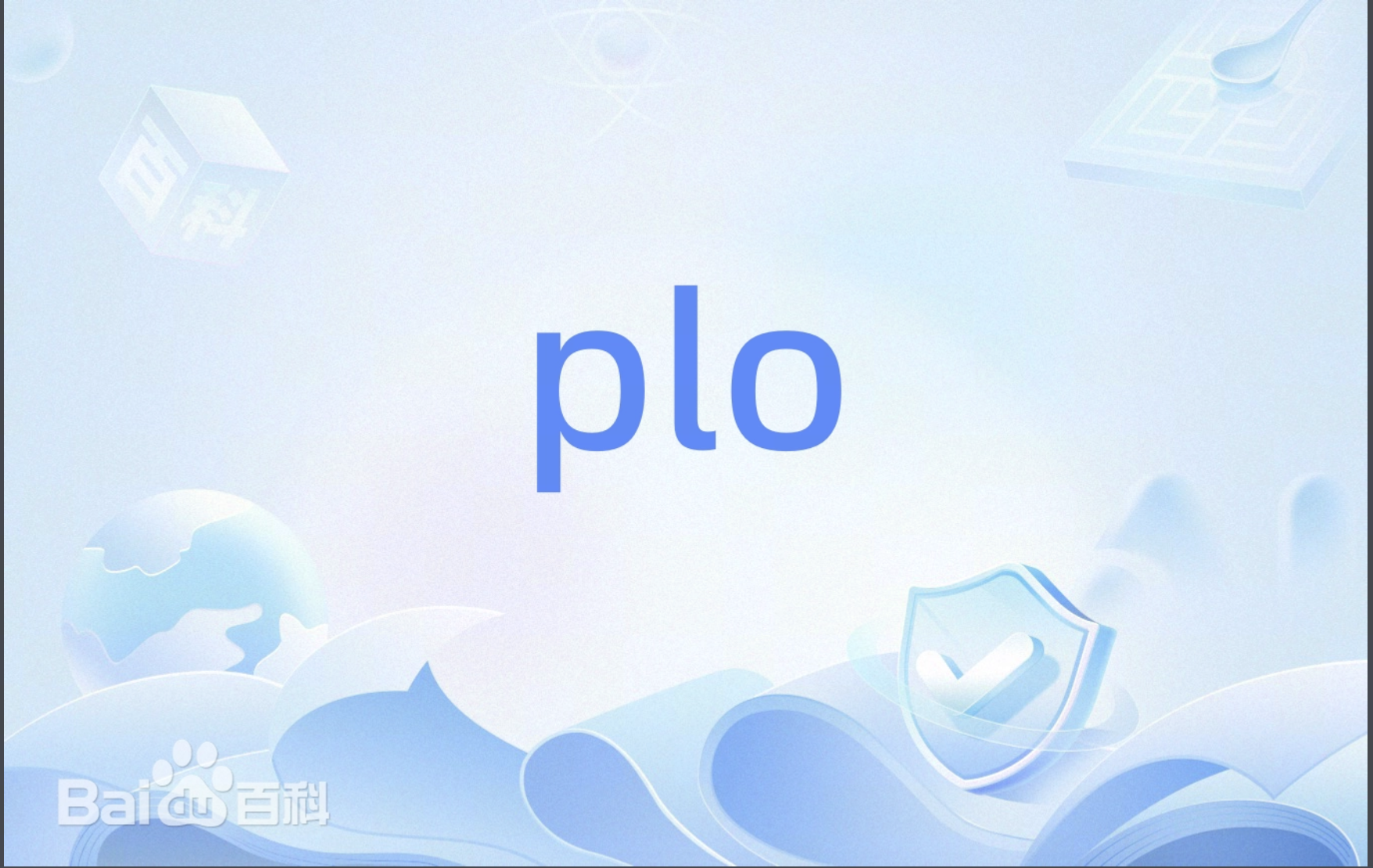
PL0语法,分析器实现!
简介 PL/0 是一种简单的编程语言,通常用于教学编译原理。它的语法结构清晰,功能包括常量定义、变量声明、过程(子程序)定义以及基本的控制结构(如条件语句和循环语句)。 PL/0 语法规范 PL/0 是一种教学用的小型编程语言,由 Niklaus Wirth 设计,用于展示编译原理的核…...
2023赣州旅游投资集团
单选题 1.“不登高山,不知天之高也;不临深溪,不知地之厚也。”这句话说明_____。 A、人的意识具有创造性 B、人的认识是独立于实践之外的 C、实践在认识过程中具有决定作用 D、人的一切知识都是从直接经验中获得的 参考答案: C 本题解…...
动态 Web 开发技术入门篇
一、HTTP 协议核心 1.1 HTTP 基础 协议全称 :HyperText Transfer Protocol(超文本传输协议) 默认端口 :HTTP 使用 80 端口,HTTPS 使用 443 端口。 请求方法 : GET :用于获取资源,…...
Caliper 配置文件解析:fisco-bcos.json
config.yaml 文件 config.yaml 是 Caliper 的主配置文件,通常包含以下内容: test:name: fisco-bcos-test # 测试名称description: Performance test of FISCO-BCOS # 测试描述workers:type: local # 工作进程类型number: 5 # 工作进程数量monitor:type: - docker- pro…...
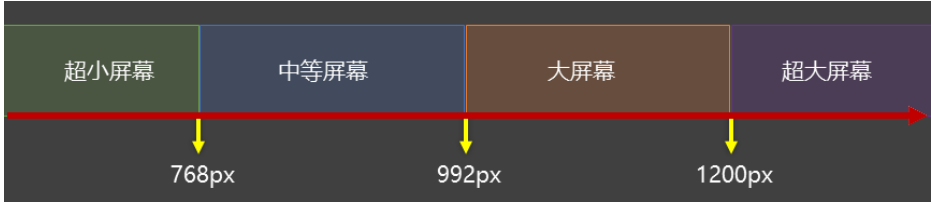
CSS3相关知识点
CSS3相关知识点 CSS3私有前缀私有前缀私有前缀存在的意义常见浏览器的私有前缀 CSS3基本语法CSS3 新增长度单位CSS3 新增颜色设置方式CSS3 新增选择器CSS3 新增盒模型相关属性box-sizing 怪异盒模型resize调整盒子大小box-shadow 盒子阴影opacity 不透明度 CSS3 新增背景属性ba…...