POI 和 EasyExcel 操作 Excel
一、概述
目前操作 Excel 比较流行的就是 Apache POI 和阿里巴巴的 easyExcel。
1.1 POI 简介
Apache POI 是用 Java 编写的免费开源的跨平台的 Java API,Apache POI 提供 API 给 Java 程序对 Microsoft Office 格式文档读和写的常用功能。POI 为 “Poor Obfuscation Implementation” 的首字母缩写,意为“简洁版的模糊实现”。其常用的结构如下:
HSSF -- 提供读写 03 版本的 Excel 常用功能。
XSSF -- 提供读写 07 版本的 Excel 常用功能。
HWPF -- 提供读写 Word 格式的常用功能
HSLF -- 提供读写 ppt 格式的常用功能。
HDGF -- 提供读写 visio 格式的常用功能
1.2 easyExcel 简介
easyExcel 是阿里巴巴开源的一个 excel 处理框架,以使用简单、节省内存著称。easyExcel 能大大减少占用内存的主要原因是在解析 Excel 时没有将文件数据一次性全部加载到内存中,而是从磁盘上一行行读取数据,逐个解析。
easyExcel 官网地址:https://github.com/alibaba/easyexcel
1.3 xls 和 xlsx 区别
常用的 excel 文档有两种结尾形式,分别为 xls 和 xlsx,其中以 xls 结尾的文档属于 03 版本的,它里面最多可以存储 65536 行数据。而以 xlsx 结尾的文档属于 07 版本的,它理论上可以存储无限行数据,这就是两者之前的区别。
二、POI 常用操作
2.1 添加 maven 依赖
<dependencies><!--xls(03 版本)--><dependency><groupId>org.apache.poi</groupId><artifactId>poi</artifactId><version>3.9</version></dependency><!--xlsx(07 版本)--><dependency><groupId>org.apache.poi</groupId><artifactId>poi-ooxml</artifactId><version>3.9</version></dependency><!-- 日期格式化工具--><dependency><groupId>joda-time</groupId><artifactId>joda-time</artifactId><version>2.10.1</version></dependency><!--test--><dependency><groupId>junit</groupId><artifactId>junit</artifactId><version>4.12</version></dependency></dependencies>
2.2 写入 Excel 操作
2.2.1 一般文件写入
2.2.1.1 03 版本
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.joda.time.DateTime;
import org.junit.Test;import java.io.FileOutputStream;public class ExcelWriteTest {String PATH ="F:\\idea_home\\poi-excel\\";@Testpublic void testWrite03() throws Exception {// 1、创建一个工作簿Workbook workbook = new HSSFWorkbook();// 2、创建一个工作表Sheet sheet = workbook.createSheet("我是 sheet1 页");// 3、创建一行Row row1 = sheet.createRow(0);// 4、创建一个单元格Cell cell11 = row1.createCell(0);cell11.setCellValue("我是第一行第一个单元格");Cell cell12 = row1.createCell(1);cell12.setCellValue("我是第一行第二个单元格");// 第二行Row row2 = sheet.createRow(1);Cell cell21 = row2.createCell(0);cell21.setCellValue("我是第二行第一个单元格");Cell cell22 = row2.createCell(1);String time = new DateTime().toString("yyyy-MM-dd HH:mm:ss");cell22.setCellValue(time);// 03 版本的使用 xls 结尾FileOutputStream fileOutputStream = new FileOutputStream(PATH+"统计表03类型.xls");workbook.write(fileOutputStream);fileOutputStream.close();System.out.println("Excel03 写入完成了");}
}
2.2.2.2 07 版本
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.joda.time.DateTime;
import org.junit.Test;import java.io.FileOutputStream;public class ExcelWriteTest {String PATH ="F:\\idea_home\\poi-excel\\";@Testpublic void testWrite07() throws Exception {// 1、创建一个工作簿Workbook workbook = new XSSFWorkbook();// 2、创建一个工作表Sheet sheet = workbook.createSheet("我是 sheet1 页");// 3、创建一行Row row1 = sheet.createRow(0);// 4、创建一个单元格Cell cell11 = row1.createCell(0);cell11.setCellValue("我是第一行第一个单元格");Cell cell12 = row1.createCell(1);cell12.setCellValue("我是第一行第二个单元格");// 第二行Row row2 = sheet.createRow(1);Cell cell21 = row2.createCell(0);cell21.setCellValue("我是第二行第一个单元格");Cell cell22 = row2.createCell(1);String time = new DateTime().toString("yyyy-MM-dd HH:mm:ss");cell22.setCellValue(time);// 07 版本的使用 xlsx 结尾FileOutputStream fileOutputStream = new FileOutputStream(PATH+"统计表07类型.xlsx");workbook.write(fileOutputStream);fileOutputStream.close();System.out.println("Excel07 写入完成了");}
}
2.2.2 大文件写入
2.2.2.1 03 版本
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.junit.Test;import java.io.FileOutputStream;public class ExcelWriteTest {String PATH ="F:\\idea_home\\poi-excel\\";@Testpublic void testWrite03BigData() throws Exception {long begin = System.currentTimeMillis();// 1、创建一个工作簿Workbook workbook = new HSSFWorkbook();// 2、创建一个工作表Sheet sheet = workbook.createSheet();// 3、写入数据for(int rowNum =0;rowNum<65537;rowNum++){Row row = sheet.createRow(rowNum);for(int cellNum=0;cellNum<10;cellNum++){Cell cell = row.createCell(cellNum);cell.setCellValue(cellNum);}}System.out.println("over");FileOutputStream fileOutputStream = new FileOutputStream(PATH+"统计表03大数据类型.xls");workbook.write(fileOutputStream);fileOutputStream.close();long end = System.currentTimeMillis();System.out.println((double)(end-begin)/1000);}
}
缺点:最多只能处理 65536 行,否则会抛出异常。
优点:写入过程中写入缓存,不操作磁盘,最后一次性写入磁盘,速度快。将 65537 改成65536 再次执行程序,结果如下,可以看到 1.692s 就完成了写入操作,速度还是很快的。
2.2.2.2 07 版本
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.junit.Test;import java.io.FileOutputStream;public class ExcelWriteTest {String PATH ="F:\\idea_home\\poi-excel\\";@Testpublic void testWrite07BigData() throws Exception {long begin = System.currentTimeMillis();// 1、创建一个工作簿Workbook workbook = new XSSFWorkbook();// 2、创建一个工作表Sheet sheet = workbook.createSheet();// 3、写入数据for(int rowNum =0;rowNum<100000;rowNum++){Row row = sheet.createRow(rowNum);for(int cellNum=0;cellNum<10;cellNum++){Cell cell = row.createCell(cellNum);cell.setCellValue(cellNum);}}System.out.println("over");FileOutputStream fileOutputStream = new FileOutputStream(PATH+"统计表07大数据类型.xlsx");workbook.write(fileOutputStream);fileOutputStream.close();long end = System.currentTimeMillis();System.out.println((double)(end-begin)/1000);}
}
缺点:写数据时速度非常慢,非常耗内存,也会发生内存溢出,如100万条。
优点:可以写较大的数据量,如20万条。
2.2.2.3 07 版本优化
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.streaming.SXSSFWorkbook;
import org.junit.Test;import java.io.FileOutputStream;public class ExcelWriteTest {String PATH ="F:\\idea_home\\poi-excel\\";@Testpublic void testWrite07BigDataS() throws Exception {long begin = System.currentTimeMillis();// 1、创建一个工作簿Workbook workbook = new SXSSFWorkbook();// 2、创建一个工作表Sheet sheet = workbook.createSheet();// 3、写入数据for(int rowNum =0;rowNum<100000;rowNum++){Row row = sheet.createRow(rowNum);for(int cellNum=0;cellNum<10;cellNum++){Cell cell = row.createCell(cellNum);cell.setCellValue(cellNum);}}System.out.println("over");FileOutputStream fileOutputStream = new FileOutputStream(PATH+"统计表07大数据类型优化.xlsx");workbook.write(fileOutputStream);// 清除产生的临时文件((SXSSFWorkbook)workbook).dispose();fileOutputStream.close();long end = System.currentTimeMillis();System.out.println((double)(end-begin)/1000);}
}
优点:可以写非常大的数据量,如 100万 条甚至更多条,数据速度快,占用更少的内存。
需要注意的是:代码在过程中会产生临时文件,需要清理临时文件。默认有 100 条记录被保存在内存中,如果超过这数量,则最前面的数据被写入临时文件。如果想自定义内存中数据的数量,可以使用 new SXSSFWorkbook(数量) 。
SXSSFWorkbook 来至官方的解释:实现 “BigGridDemo” 策略的流式 XSSFWorkbook 版本。这允许写入非常大的文件而不会耗尽内存,因为任何时候只有可配置的行部分被保存在内存中。请注意,仍然可能会消耗大量内存,这些内存基于您正在使用的功能,例如合并区域,注释……仍然只存储在内存中,因此如果广泛使用,可能需要大量内存。
2.3 读取 Excel 操作
2.3.1 03 版本
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.*;
import org.junit.Test;
import java.io.FileInputStream;public class ExcelRead {String PATH ="F:\\idea_home\\poi-excel\\";@Testpublic void testRead03() throws Exception {// 1、获取文件流FileInputStream fileInputStream = new FileInputStream(PATH+"统计表03类型.xls");// 2、创建文件簿,使用 excel 能操作的这边都可以操作Workbook workbook = new HSSFWorkbook(fileInputStream);// 3、得到表Sheet sheet = workbook.getSheetAt(0);// 4、得到行Row row = sheet.getRow(0);// 5、得到列Cell cell = row.getCell(0);// 读取值的时候需要注意类型,String 和数字调用的方法是不同的。System.out.println(cell.getStringCellValue());fileInputStream.close();}
}
2.3.2 07 版本
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.junit.Test;
import java.io.FileInputStream;public class ExcelRead {String PATH ="F:\\idea_home\\poi-excel\\";@Testpublic void testRead07() throws Exception {// 1、获取文件流FileInputStream fileInputStream = new FileInputStream(PATH+"统计表07类型.xlsx");// 2、创建文件簿,使用 excel 能操作的这边都可以操作Workbook workbook = new XSSFWorkbook(fileInputStream);// 3、得到表Sheet sheet = workbook.getSheetAt(0);// 4、得到行Row row = sheet.getRow(0);// 5、得到列Cell cell = row.getCell(1);// 读取值的时候需要注意类型,String 和数字调用的方法是不同的。System.out.println(cell.getStringCellValue());fileInputStream.close();}
}
2.3.3 读取不同类型
表格的内容如下所示:
代码如下所示:
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFDateUtil;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.*;
import org.joda.time.DateTime;
import org.junit.Test;
import java.io.FileInputStream;
import java.util.Date;public class ExcelRead {String PATH ="F:\\idea_home\\poi-excel\\";@Testpublic void testCellType() throws Exception{// 1、获取文件流FileInputStream fileInputStream = new FileInputStream(PATH+"03商品信息.xls");// 2、创建文件簿,使用 excel 能操作的这边都可以操作Workbook workbook = new HSSFWorkbook(fileInputStream);// 3、得到表Sheet sheet = workbook.getSheetAt(0);// 4、获取标题内容Row rowTitle = sheet.getRow(0);if(rowTitle != null){// 获取列的数量int cellCount = rowTitle.getPhysicalNumberOfCells();for(int cellNum=0;cellNum<cellCount;cellNum++){Cell cell = rowTitle.getCell(cellNum);if(cell != null){// 获取列的类型int cellType = cell.getCellType();// 获取具体的列名String cellValue = cell.getStringCellValue();System.out.print(cellValue+" | ");}}}System.out.println();// 5、获取表中的内容// 获取有多少行的记录int rowCount = sheet.getPhysicalNumberOfRows();for(int rowNum=1;rowNum<rowCount;rowNum++){// 获取第一行数据Row rowData = sheet.getRow(rowNum);if(rowData !=null){// 读取行中的列int cellCount = rowTitle.getPhysicalNumberOfCells();for(int cellNum=0;cellNum<cellCount;cellNum++){System.out.print("["+(rowNum+1)+"-"+(cellNum+1)+"]");Cell cell = rowData.getCell(cellNum);// 匹配类的数据类型if(cell != null){int cellType = cell.getCellType();String cellValue="";switch(cellType){case HSSFCell.CELL_TYPE_STRING: //字符串System.out.print("【STRING】");cellValue = cell.getStringCellValue();break;case HSSFCell.CELL_TYPE_BOOLEAN: //布尔System.out.print("【BOOLEAN】");cellValue = String.valueOf(cell.getBooleanCellValue());break;case HSSFCell.CELL_TYPE_BLANK: //空System.out.print("【BLANK】");break;case HSSFCell.CELL_TYPE_NUMERIC: //数字(分为日期和普通数字)System.out.print("【NUMERIC】");if(HSSFDateUtil.isCellDateFormatted(cell)){ // 日期System.out.print("【日期】");Date date = cell.getDateCellValue();cellValue = new DateTime(date).toString("yyyy-MM-dd");}else{// 非日期格式,转换成字符串格式System.out.print("【转化为字符串输出】");cell.setCellType(HSSFCell.CELL_TYPE_STRING);cellValue = cell.toString();}break;case HSSFCell.CELL_TYPE_ERROR: //字符串System.out.print("【数据类型错误】");break;}System.out.println(cellValue);}}}}fileInputStream.close();}
}
2.3.4 读取公式
操作的表格内容如下所示:
代码如下所示:
import org.apache.poi.hssf.usermodel.HSSFFormulaEvaluator;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.*;
import org.junit.Test;
import java.io.FileInputStream;public class ExcelRead {String PATH ="F:\\idea_home\\poi-excel\\";@Testpublic void testFormula() throws Exception {FileInputStream fileInputStream = new FileInputStream(PATH + "03求和.xls");// 1.创建一个工作簿。使得excel能操作的,这边他也能操作。Workbook workbook = new HSSFWorkbook(fileInputStream);// 2.得到表。Sheet sheet = workbook.getSheetAt(0);Row row = sheet.getRow(6);Cell cell = row.getCell(0);// 拿到计算公司FormulaEvaluator formulaEvaluator = new HSSFFormulaEvaluator((HSSFWorkbook) workbook);// 输出单元格内容int cellType = cell.getCellType();switch (cellType){case Cell.CELL_TYPE_FORMULA:String cellFormula = cell.getCellFormula();System.out.println(cellFormula);// 计算CellValue evaluate = formulaEvaluator.evaluate(cell);String cellValue = evaluate.formatAsString();System.out.println(cellValue);break;}}
}
三、EasyExcel 常用操作
3.1 添加 maven 依赖
<dependencies><!-- 主要是这个依赖,剩下的依赖都是测试用到的 --><dependency><groupId>com.alibaba</groupId><artifactId>easyexcel</artifactId><version>3.0.5</version></dependency><dependency><groupId>lambada</groupId><artifactId>lambada</artifactId><version>1.0.3</version></dependency><dependency><groupId>org.projectlombok</groupId><artifactId>lombok</artifactId><version>RELEASE</version><scope>compile</scope></dependency><!--test--><dependency><groupId>junit</groupId><artifactId>junit</artifactId><version>4.12</version></dependency><dependency><groupId>com.alibaba</groupId><artifactId>fastjson</artifactId><version>1.2.7</version></dependency></dependencies>
3.2 写操作
先模拟一个实体类,如下所示:
import com.alibaba.excel.annotation.ExcelIgnore;
import com.alibaba.excel.annotation.ExcelProperty;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.Setter;import java.util.Date;@Getter
@Setter
@EqualsAndHashCode
public class DemoData {@ExcelProperty("字符串标题")private String string;@ExcelProperty("日期标题")private Date date;@ExcelProperty("数字标题")private Double doubleData;/*** 忽略这个字段*/@ExcelIgnoreprivate String ignore;
}
然后写入文档即可,如下所示:
import com.alibaba.excel.EasyExcel;
import com.alibaba.excel.util.ListUtils;
import org.junit.Test;import java.util.Date;
import java.util.List;public class TestWrite {@Testpublic void simpleWrite() {String PATH ="F:\\idea_home\\poi-excel\\easyexcel统计表03类型.xlsx";EasyExcel.write(PATH, DemoData.class).sheet("模板").doWrite(() -> {// 分页查询数据return data();});}private List<DemoData> data() {List<DemoData> list = ListUtils.newArrayList();for (int i = 0; i < 10; i++) {DemoData data = new DemoData();data.setString("字符串" + i);data.setDate(new Date());data.setDoubleData(0.56);list.add(data);}return list;}
}
3.3 读操作
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.Setter;import java.util.Date;@Getter
@Setter
@EqualsAndHashCode
public class DemoData {private String string;private Date date;private Double doubleData;
}
import com.alibaba.excel.EasyExcel;
import com.alibaba.excel.read.listener.PageReadListener;
import com.alibaba.fastjson.JSON;
import org.junit.Test;public class TestRead {@Testpublic void simpleRead() {String PATH ="F:\\idea_home\\poi-excel\\easyexcel统计表03类型.xlsx";// 这里 需要指定读用哪个class去读,然后读取第一个sheet 文件流会自动关闭// 这里每次会读取3000条数据 然后返回过来 直接调用使用数据就行EasyExcel.read(PATH, DemoData.class, new PageReadListener<DemoData>(dataList -> {for (DemoData demoData : dataList) {System.out.println(JSON.toJSONString(demoData));}})).sheet().doRead();}
}
3.4 更多操作
详细的文档地址:https://www.yuque.com/easyexcel/doc/easyexcel
相关文章:
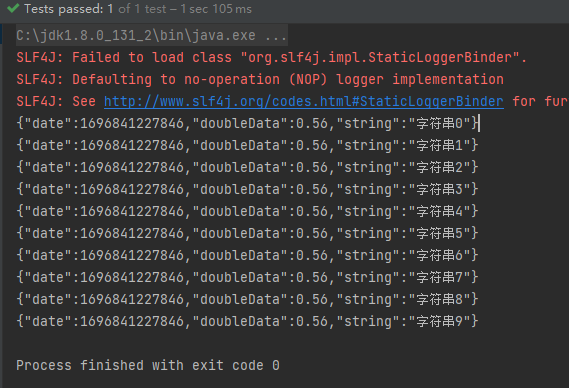
POI 和 EasyExcel 操作 Excel
一、概述 目前操作 Excel 比较流行的就是 Apache POI 和阿里巴巴的 easyExcel。 1.1 POI 简介 Apache POI 是用 Java 编写的免费开源的跨平台的 Java API,Apache POI 提供 API 给 Java 程序对 Microsoft Office 格式文档读和写的常用功能。POI 为 “Poor Obfuscati…...
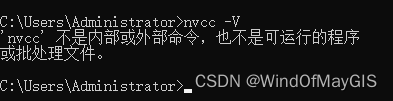
pytorch算力与有效性分析
pytorch Windows中安装深度学习环境参考文档机器环境说明3080机器 Windows11qt_env 满足遥感CS软件分割、目标检测、变化检测的需要gtrs 主要是为了满足遥感监测管理平台(BS)系统使用的,无深度学习环境内容swin_env 与 qt_env 基本一致od 用于…...

Sublime text启用vim模式
官方教程:https://www.sublimetext.com/docs/vintage.html vintage的github:https://github.com/sublimehq/Vintage...

爬虫进阶-反爬破解6(Nodejs+Puppeteer实现登陆官网+实现滑动验证码全自动识别)
一、NodejsPuppeteer实现登陆官网 1.环境说明 Nodejs——直接从官网下载最新版本,并安装 使用npm安装puppeteer:npm install puppeteer npm install xxx -registry https://registry.npm.taobao.org Chromium会自动下载,前提是网络通畅 2.实践操作…...
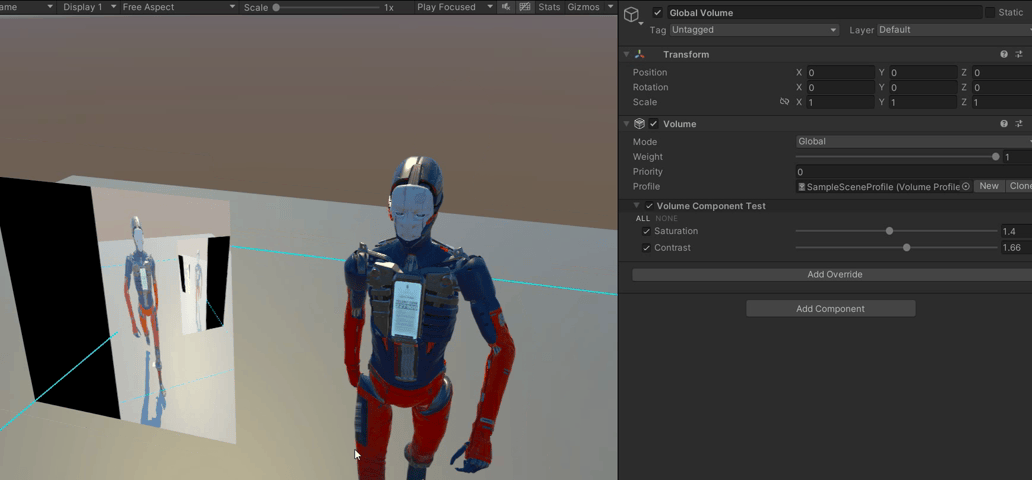
【Unity】RenderFeature笔记
【Unity】RenderFeature笔记 RenderFeature是在urp中添加的额外渲染pass,并可以将这个pass插入到渲染列队中的任意位置。内置渲染管线中Graphics 的功能需要在RenderFeature里实现,常见的如DrawMesh和Blit 可以实现的效果包括但不限于 后处理,可以编写…...
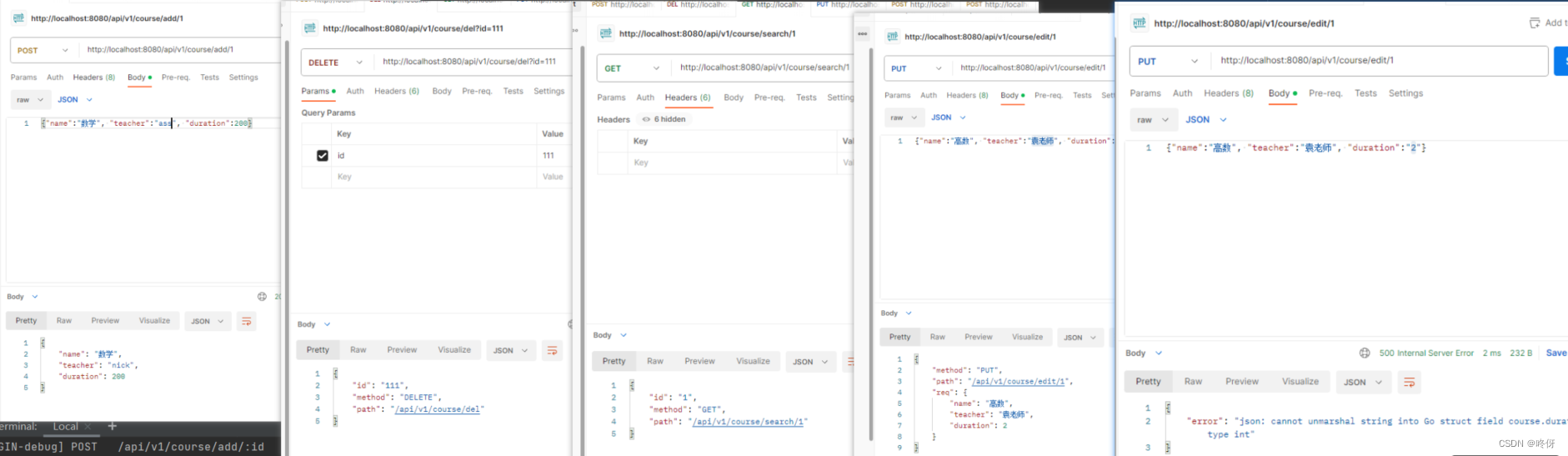
golang gin——controller 模型绑定与参数校验
controller 模型绑定与参数校验 gin框架提供了多种方法可以将请求体的内容绑定到对应struct上,并且提供了一些预置的参数校验 绑定方法 根据数据源和类型的不同,gin提供了不同的绑定方法 Bind, shouldBind: 从form表单中去绑定对象BindJSON, shouldB…...
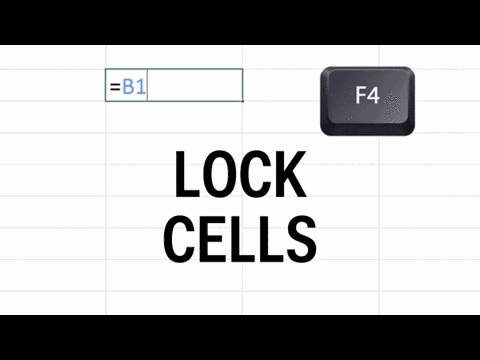
办公技巧:Excel日常高频使用技巧
目录 1. 快速求和?用 “Alt ” 2. 快速选定不连续的单元格 3. 改变数字格式 4. 一键展现所有公式 “CTRL ” 5. 双击实现快速应用函数 6. 快速增加或删除一列 7. 快速调整列宽 8. 双击格式刷 9. 在不同的工作表之间快速切换 10. 用F4锁定单元格 1. 快速求…...
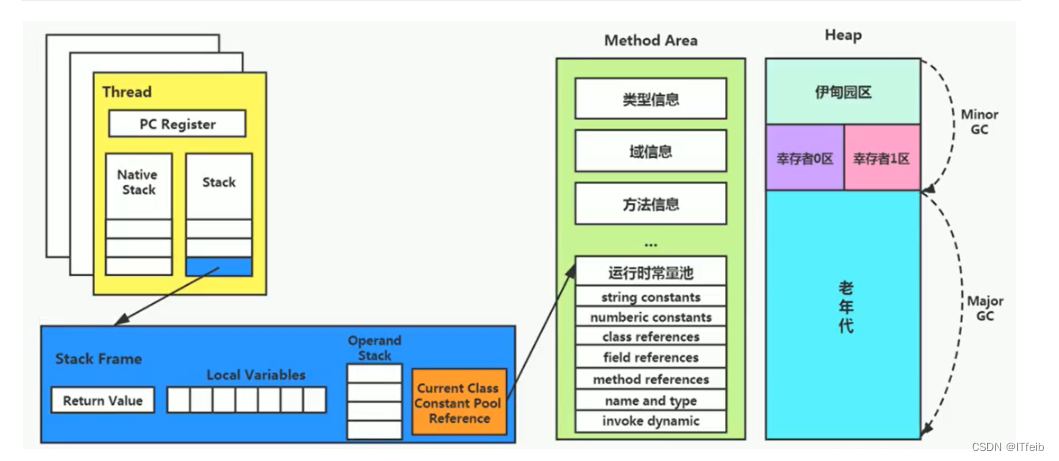
【jvm--方法区】
文章目录 1. 栈、堆、方法区的交互关系2. 方法区的内部结构3. 运行时常量池4. 方法区的演进细节5. 方法区的垃圾回收 1. 栈、堆、方法区的交互关系 方法区的基本理解: 方法区(Method Area)与 Java 堆一样,是各个线程共享的内存区…...
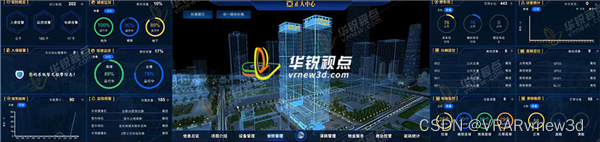
智慧楼宇3D数据可视化大屏交互展示实现了楼宇能源的高效、智能、精细化管控
智慧园区是指将物联网、大数据、人工智能等技术应用于传统建筑和基础设施,以实现对园区的全面监控、管理和服务的一种建筑形态。通过将园区内设备、设施和系统联网,实现数据的传输、共享和响应,提高园区的管理效率和运营效益,为居…...
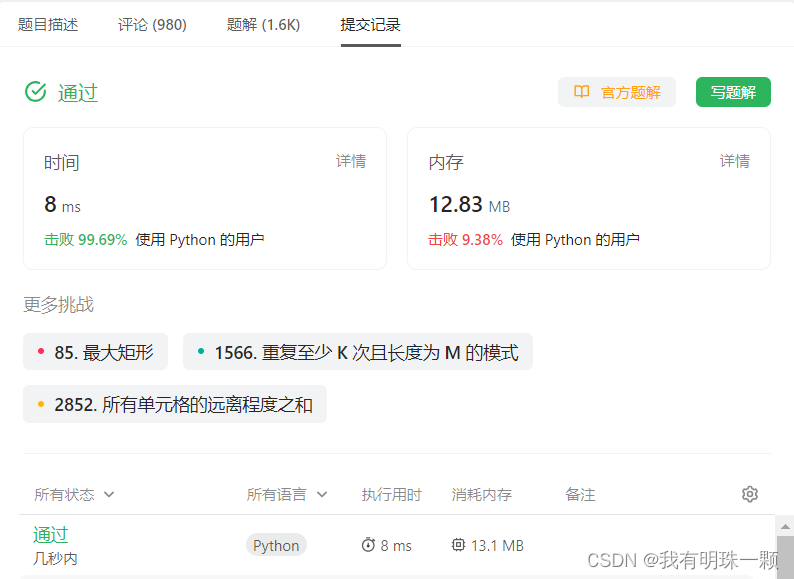
算法题:摆动序列(贪心算法解决序列问题)
这道题是一道贪心算法题,如果前两个数是递增,则后面要递减,如果不符合则往后遍历,直到找到符合的。(完整题目附在了最后) 代码如下: class Solution(object):def wiggleMaxLength(self, nums):…...
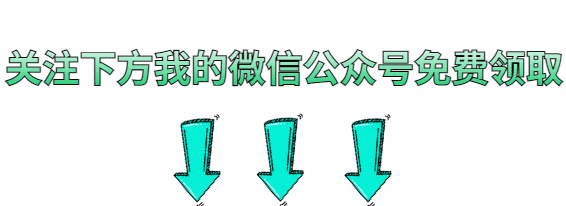
接口自动化测试yaml+requests+allure技术,你学会了吗?
前言 接口自动化测试是在软件开发过程中常用的一种测试方式,通过对接口进行自动化测试,可以提高测试效率、降低测试成本。在接口自动化测试中,yaml、requests和allure三种技术经常被使用。 一、什么是接口自动化测试 接口自动化测试是指通…...

android 获取局域网其他设备ip
Android 通过读取本地Arp表获取当前局域网内其他设备信息_手机查看arp-CSDN博客...

angular中使用 ngModel 自定义组件
要创建一个自定义的 Angular 组件,并使用 ngModel 进行双向数据绑定,您可以按照以下步骤操作: 创建自定义组件:首先,使用 Angular CLI 或手动创建一个新的组件。在组件的模板中,添加一个输入元素或其他适合…...

kubernetes pod日志查看用户创建
目录 1.创建用户 1.1证书创建 1.2创建用户 1.3允许用户登陆 1.4切换用户 1.5删除用户 2.RBAC 1.创建用户 1.1证书创建 进入证书目录 # cd /etc/kubernetes/pki创建key # openssl genrsa -out user1.key 2048 Generating RSA private key, 2048 bit long modulus .....…...
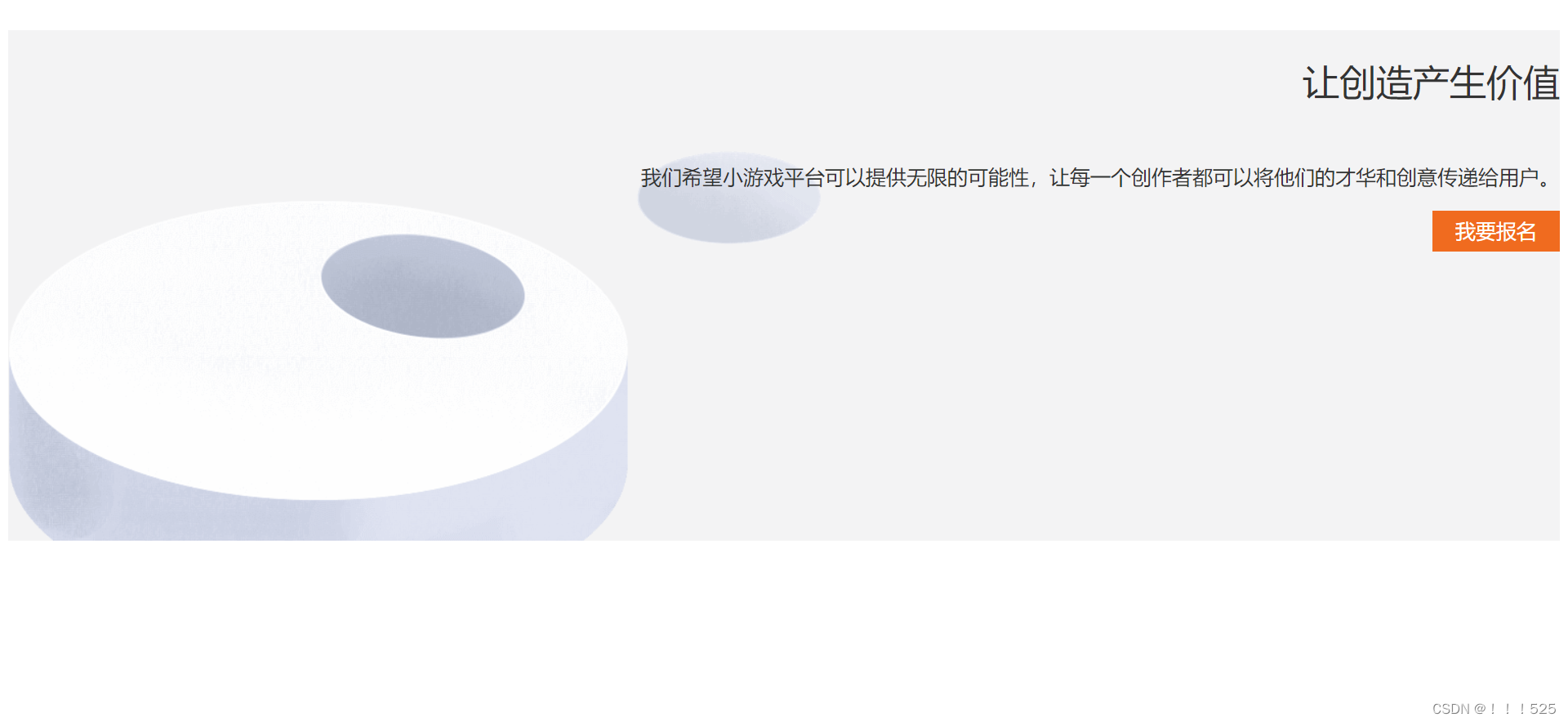
HTML5+CSSday4综合案例二——banner效果
bannerCSS展示图: <!DOCTYPE html> <html lang"en"> <head><meta charset"UTF-8"><meta http-equiv"X-UA-Compatible" content"IEedge"><meta name"viewport" content"wi…...

关于红包雨功能的探索
【高并发优化手段】基于Springboot项目 【红包雨功能的】环境部署(弹性伸缩、负载均衡、Redis读写分离、云服务器部署) jemeter压测【2万用户每秒5次请求在30秒内处理完请求】 【红包雨压测】提供2万用户30秒内5次请求的并发服务支持 使用工厂模式、策略…...
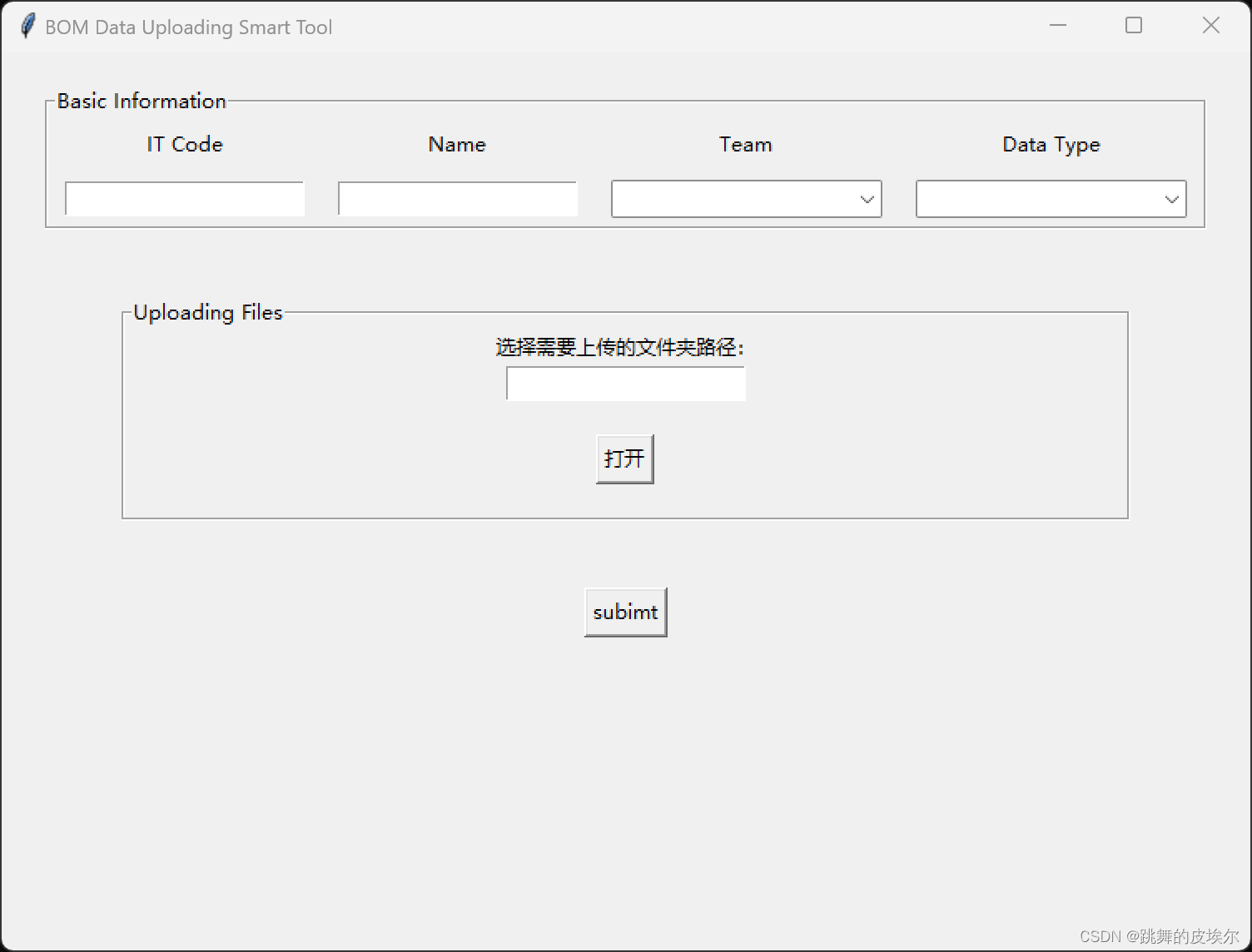
【已解决】Python打包文件执行报错:ModuleNotFoundError: No module named ‘pymssql‘
【已解决】Python打包文件执行报错:ModuleNotFoundError: No module named pymssql 1、问题2、原因3、解决 1、问题 今天打包一个 tkinter pymssql 的项目的时候,打包过程很顺利,但是打开软件的时候,报错 ModuleNotFoundError: …...

华为云云耀云服务器L实例评测|测试CentOS的网络配置和访问控制
目录 引言 1 理解几个基础概念 2 配置VPC、子网以及路由表 3 配置安全组策略和访问控制规则 3.1 安全组策略和访问控制简介 3.2 配置安全组策略 3.3 安全组的最佳实践 结论 引言 在云计算时代,网络配置和访问控制是确保您的CentOS虚拟机在云环境中安全运行的…...

CSP模拟51联测13 B.狗
CSP模拟51联测13 B.狗 文章目录 CSP模拟51联测13 B.狗题目大意题目描述输入格式输出格式样例样例 1inputoutput 思路 题目大意 题目描述 小G养了很多狗。 小G一共有 n n n\times n nn 条狗,在一个矩阵上。小G想让狗狗交朋友,一条狗狗最多只能交一个…...
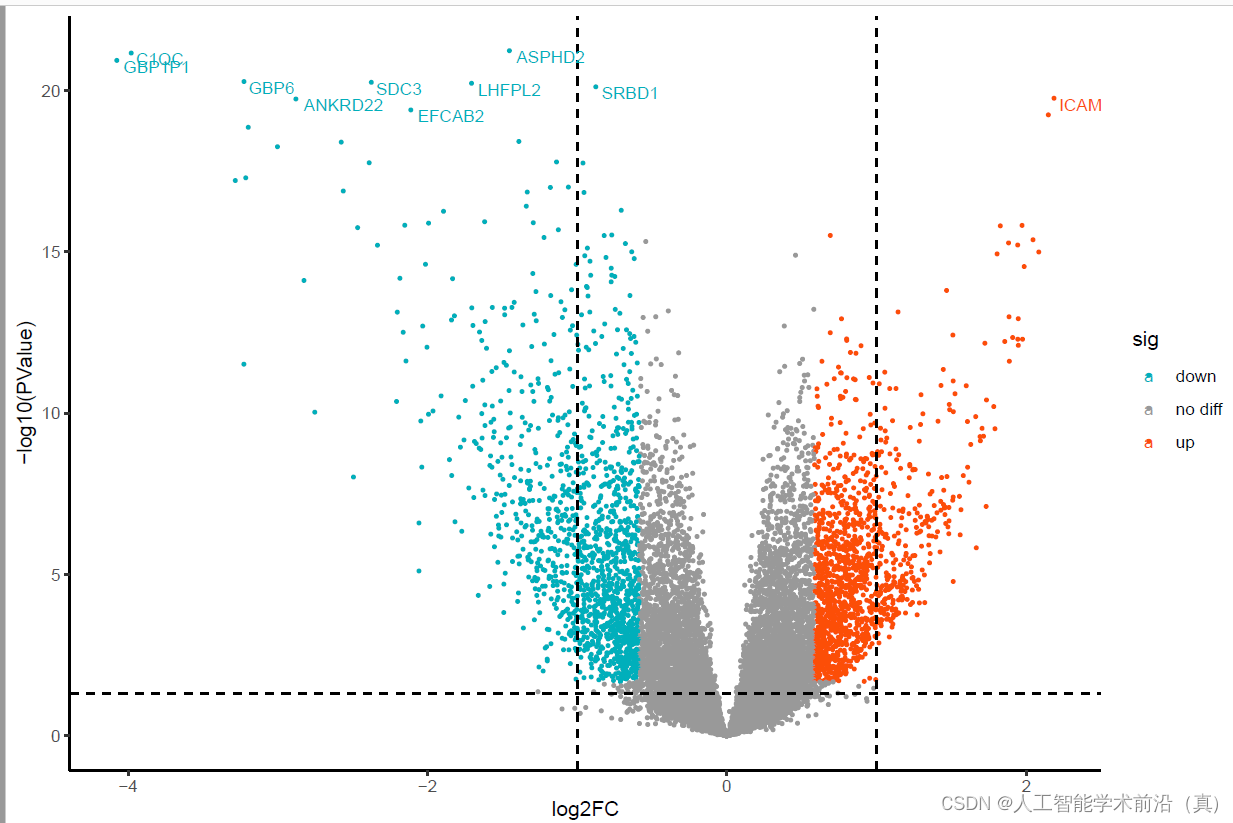
GEO生信数据挖掘(七)差异基因分析
上节,我们使用结核病基因数据,做了一个数据预处理的实操案例。例子中结核类型,包括结核,潜隐进展,对照和潜隐,四个类别。本节延续上个数据,进行了差异分析。 差异分析 计算差异指标step12 加载…...
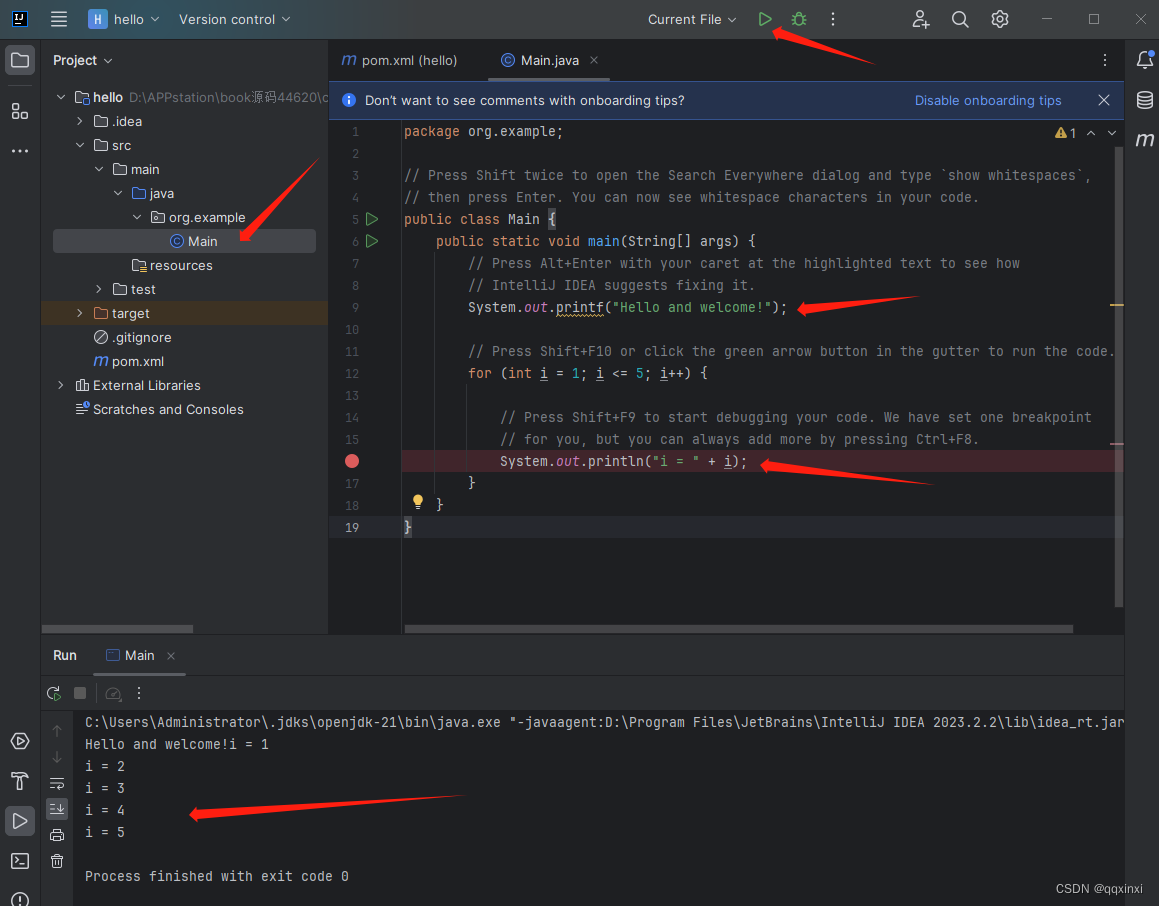
JAVA-SpringBoot入门Demo用IDEA建立helloworld
使用编辑器IDEA做SpringBoot项目最近几年比较红红,作为JAVA语言翻身的技术,用户量激增。由于java平台原来的占有率,相比net core在某些方面更有优势。 我把本次我下载完成后Maven项目的过程记录下来了,仅供参考! 安装J…...
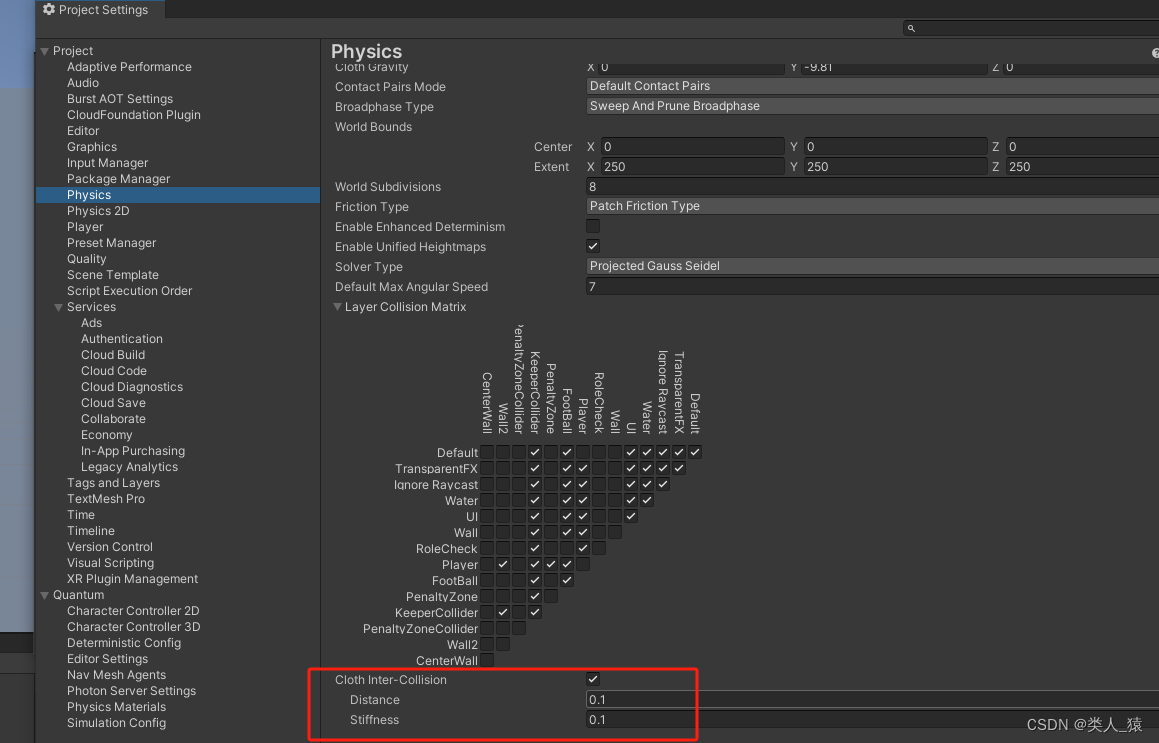
Unity布料系统Cloth
Unity布料系统Cloth 介绍布料系统Cloth(Unity组件)组件上的一些属性布料系统的使用布料约束Select面板Paint面板Gradient Tool面板 布料碰撞布料碰撞碰撞适用 介绍 布料系统我第一次用是做人物的裙摆自然飘动,当时我用的是UnityChan这个unity官方自带的插件做的裙摆…...
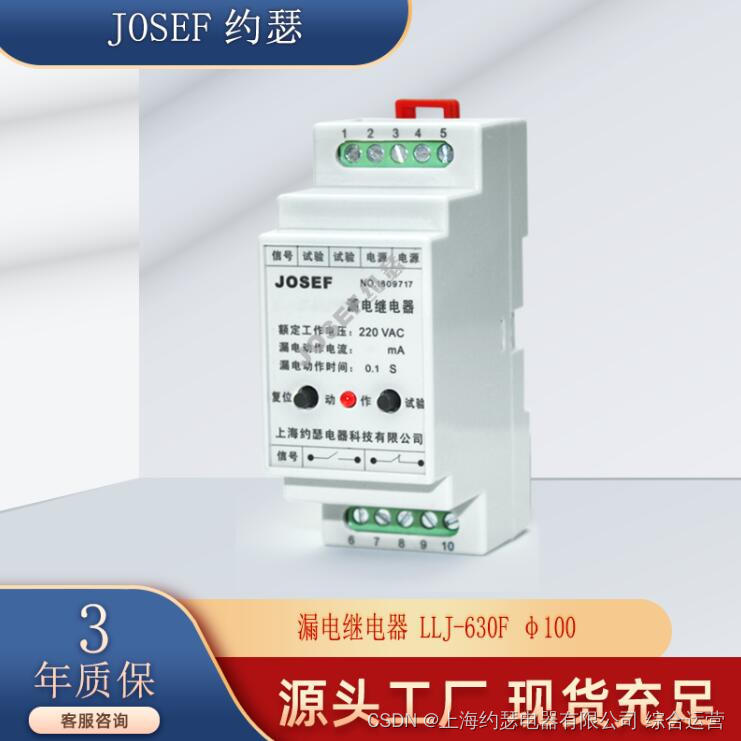
漏电继电器 LLJ-630F φ100 导轨安装 分体式结构 LLJ-630H(S) AC
系列型号: LLJ-10F(S)漏电继电器LLJ-15F(S)漏电继电器LLJ-16F(S)漏电继电器 LLJ-25F(S)漏电继电器LLJ-30F(S)漏电继电器LLJ-32F(S)漏电继电器 LLJ-60F(S)漏电继电器LLJ-63F(S)漏电继电器LLJ-80F(S)漏电继电器 LLJ-100F(S)漏电继电器LLJ-120F(S)漏电继电器LLJ-125F(S…...
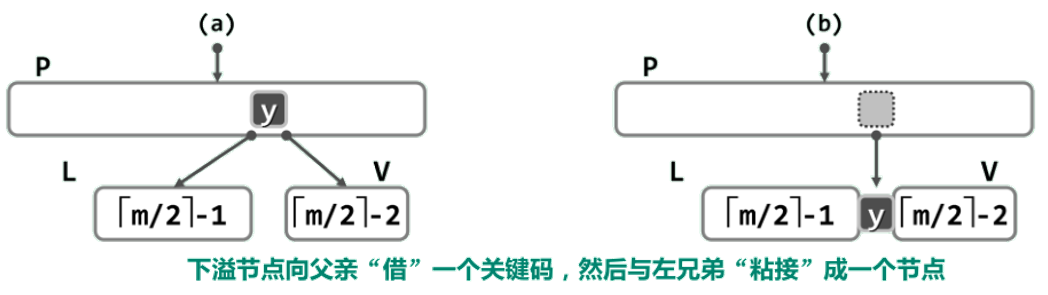
数据结构和算法(10):B-树
B-树:大数据 现代电子计算机发展速度空前,就存储能力而言,情况似乎也是如此:如今容量以TB计的硬盘也不过数百元,内存的常规容量也已达到GB量级。 然而从实际应用的需求来看,问题规模的膨胀却远远快于存储能…...
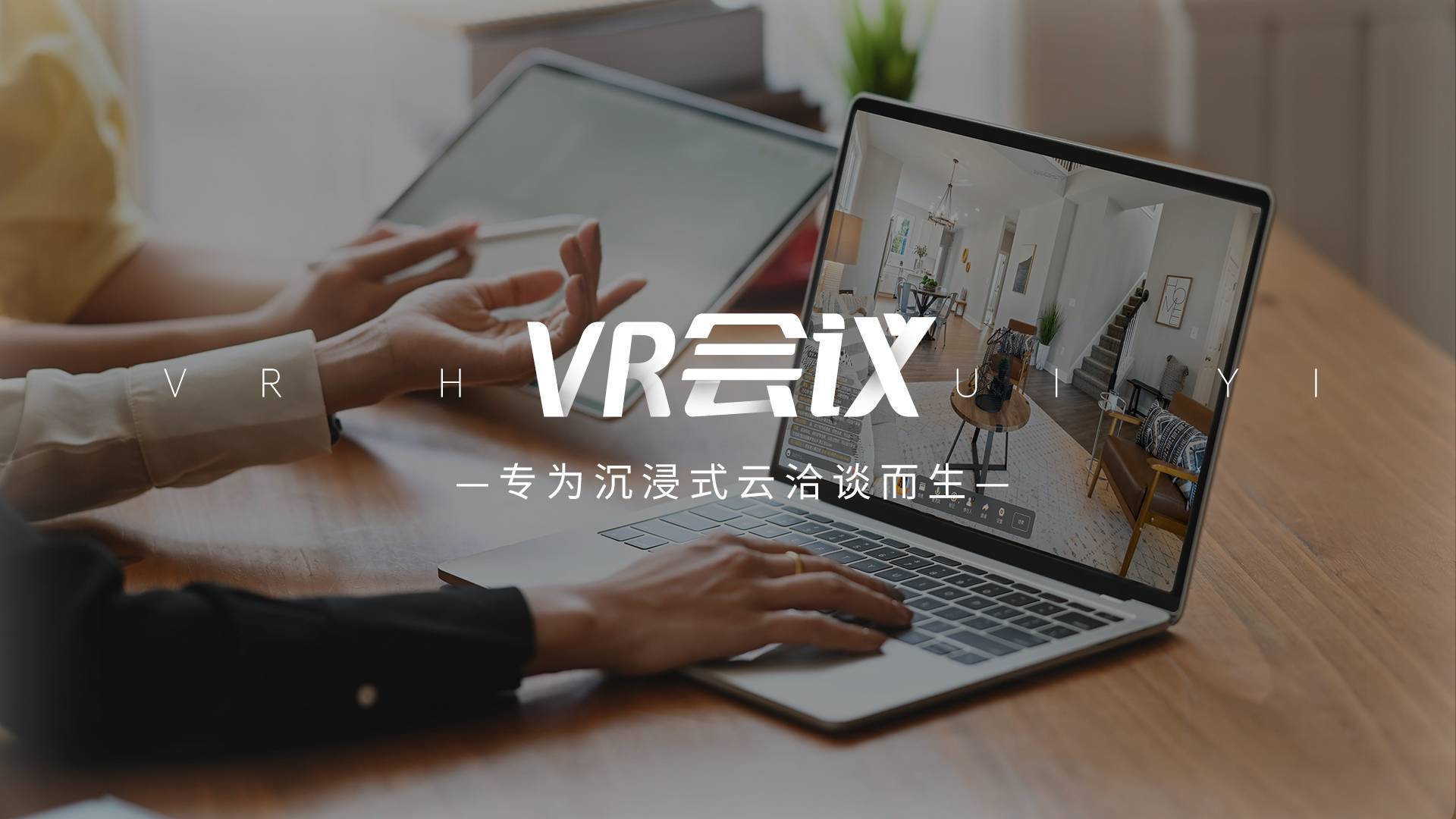
VR会议:远程带看功能,专为沉浸式云洽谈而生
随着科技的不断发展,VR技术已经成为当今市场上较为热门的新型技术之一了,而VR会议远程带看功能,更是为用户提供更加真实、自然的沉浸式体验。 随着5G技术的发展,传统的图文、视频这种展示形式已经无法满足消费者对信息真实性的需求…...
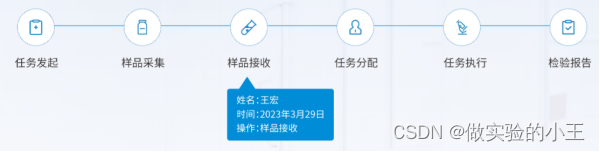
实验室管理系统LIMS
在数字化浪潮中,越来越多的企业开始有数字化转型的意识。对于实验室而言,数字化转型是指运用新一代数字技术,促进实验室业务、生产、研发、管理、服务、供应链等方面的转型与升级,实现实验室业务“人、机、料、法、环”的多维度发…...
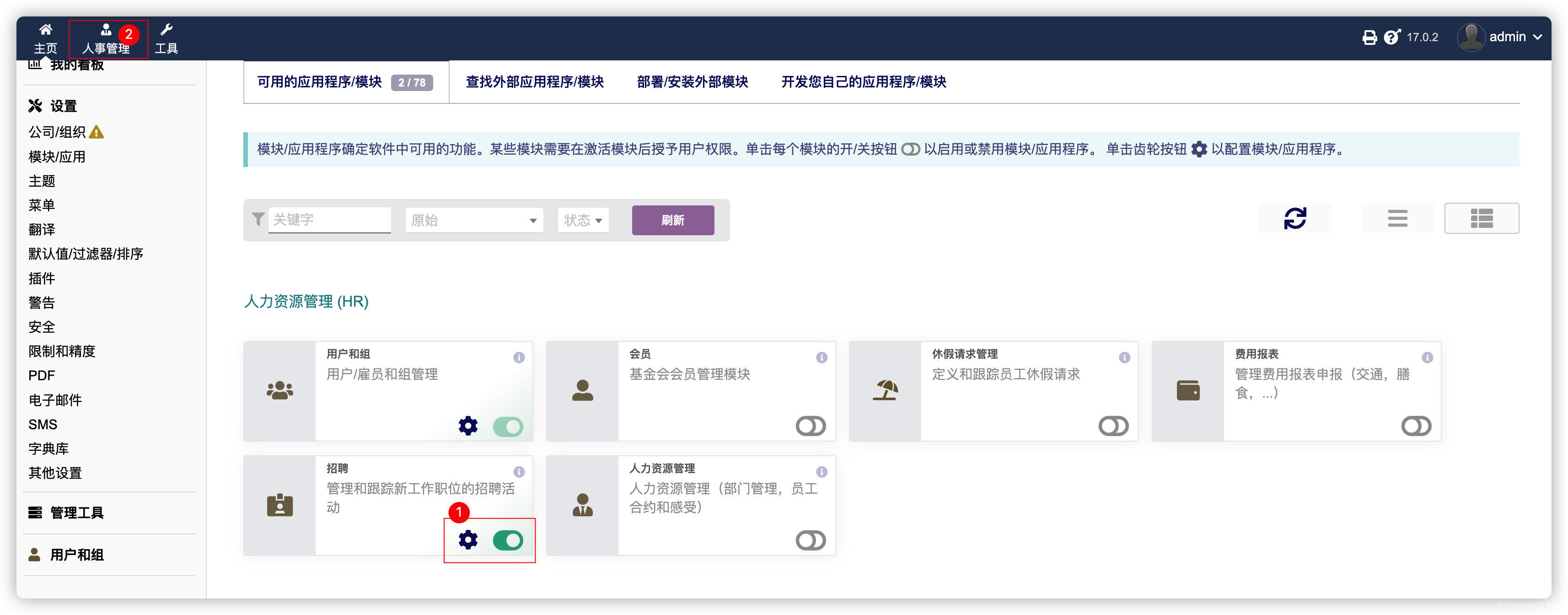
开源ERP和CRM套件Dolibarr
什么是 Dolibarr ? Dolibarr ERP & CRM 是一个现代软件包,用于管理您组织的活动(联系人、供应商、发票、订单、库存、议程…)。它是开源软件(用 PHP 编写),专为中小型企业、基金会和自由职业…...
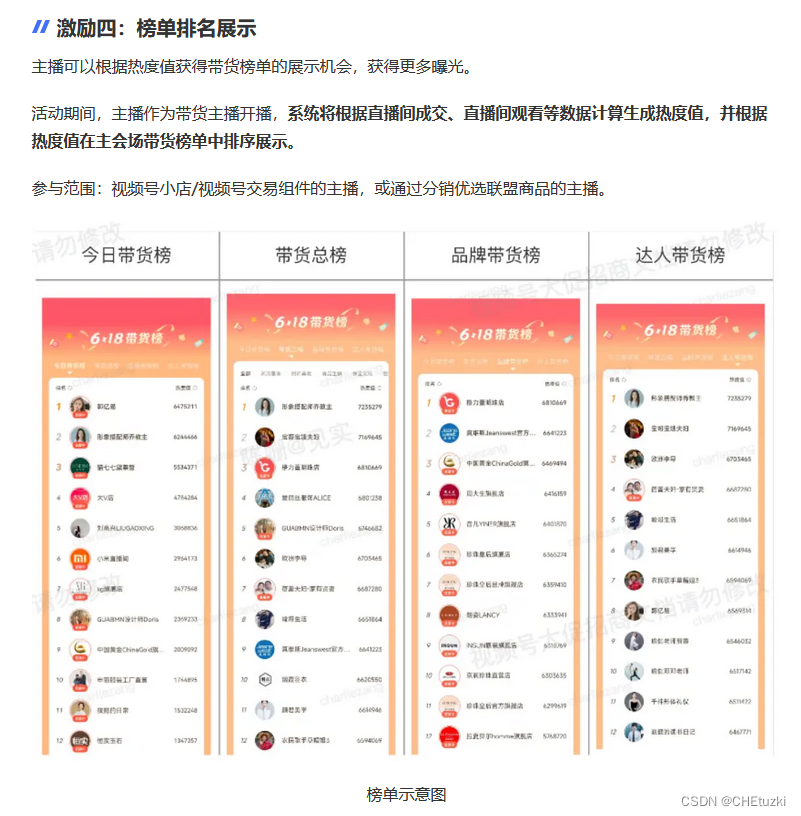
视频号双11激励政策,快来看一看
双十一即将来临,不少平台都公布了自己的双十一政策。这篇文章,我们来看看视频号推出的激励政策,看有哪些需要准备的。...
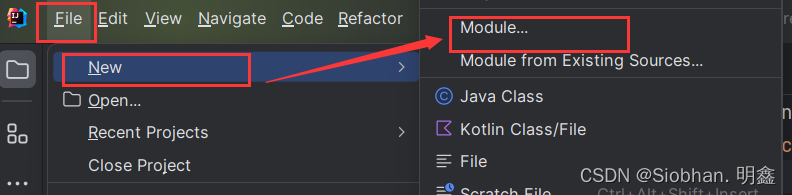
Maven最新版本安装及配置
Maven是一个Java项目管理和构建工具,它可以定义项目结构、项目依赖,并使用统一的方式进行自动化构建,是Java项目不可缺少的工具。 本章我们详细介绍如何使用Maven。 一、Maven是什么? 如果每一个项目都自己搞一套配置…...

探索ClickHouse——使用MaterializedPostgreSQL同步PostgreSQL数据库
安装PostgreSQL sudo apt install postgresql修改配置 sudo vim /etc/postgresql/14/main/postgresql.conf 解开并修改wal_level 的配置项 wal_level logical 重启服务 /etc/init.d/postgresql restartRestarting postgresql (via systemctl): postgresql.service AUTHENTI…...