【Node.js】路由
基础使用
写法一:
// server.js
const http = require('http');
const fs = require('fs');
const route = require('./route')
http.createServer(function (req, res) {const myURL = new URL(req.url, 'http://127.0.0.1')route(res, myURL.pathname)res.end()
}).listen(3000, ()=> {console.log("server start")
})
// route.js
const fs = require('fs')
function route(res, pathname) {switch (pathname) {case '/login':res.writeHead(200, { 'Content-Type': 'text/html;charset=utf-8' })res.write(fs.readFileSync('./static/login.html'), 'utf-8')break;case '/home':res.writeHead(200, { 'Content-Type': 'text/html;charset=utf-8' })res.write(fs.readFileSync('./static/home.html'), 'utf-8')breakdefault:res.writeHead(404, { 'Content-Type': 'text/html;charset=utf-8' })res.write(fs.readFileSync('./static/404.html'), 'utf-8')break}
}
module.exports = route
写法二:
// route.js
const fs = require('fs')const route = {"/login": (res) => {res.writeHead(200, { 'Content-Type': 'text/html;charset=utf-8' })res.write(fs.readFileSync('./static/login.html'), 'utf-8')},"/home": (res) => {res.writeHead(200, { 'Content-Type': 'text/html;charset=utf-8' })res.write(fs.readFileSync('./static/home.html'), 'utf-8')},"/404": (res) => {res.writeHead(404, { 'Content-Type': 'text/html;charset=utf-8' })res.write(fs.readFileSync('./static/404.html'), 'utf-8')},"/favicon.ico": (res) => {res.writeHead(200, { 'Content-Type': 'image/x-icon;charset=utf-8' })res.write(fs.readFileSync('./static/favicon.ico'))}
}
module.exports = route
// server.js
const http = require('http');
const fs = require('fs');
const route = require('./route')
http.createServer(function (req, res) {const myURL = new URL(req.url, 'http://127.0.0.1')try {route[myURL.pathname](res)} catch (error) {route['/404'](res)}res.end()
}).listen(3000, ()=> {console.log("server start")
})
注册路由
// api.js
function render(res, data, type = "") {res.writeHead(200, { 'Content-Type': `${type ? type : 'application/json'};charset=utf-8` })res.write(data)res.end()
}
const apiRouter = {'api/login':(res)=> [render(res, `{ok:1}`)]
}
module.exports = apiRouter
// route.js
const fs = require('fs')function render(res, path, type="") {res.writeHead(200, { 'Content-Type': `${type?type:'text/html'};charset=utf-8` })res.write(fs.readFileSync(path), 'utf-8')res.end()
}const route = {"/login": (res) => {render(res, './static/login.html')},"/home": (res) => {render(res, './static/home.html')},"/404": (res) => {res.writeHead(404, { 'Content-Type': 'text/html;charset=utf-8' })res.write(fs.readFileSync('./static/404.html'), 'utf-8')res.end()},"/favicon.ico": (res) => {render(res, './static/favicon.ico', 'image/x-icon')}
}
module.exports = route
// server.js
const http = require('http');
const Router = {}
const use = (obj) => {Object.assign(Router, obj)
}
const start = () => {http.createServer(function (req, res) {const myURL = new URL(req.url, 'http://127.0.0.1')try {Router[myURL.pathname](res)} catch (error) {Router['/404'](res)}}).listen(3000, () => {console.log("server start")})
}
exports.start = start
exports.use = use
// index.js
const server = require('./server');
const route = require('./route');
const api = require('./api');
// 注册路由
server.use(route)
server.use(api)
server.start()
get 和 post
// api.js
function render(res, data, type = "") {res.writeHead(200, { 'Content-Type': `${type ? type : 'application/json'};charset=utf-8` })res.write(data)res.end()
}
const apiRouter = {'/api/login': (req,res) => {const myURL = new URL(req.url, 'http:/127.0.0.1')if(myURL.searchParams.get('username') === 'admin' && myURL.searchParams.get('password') === '123456') {render(res, `{"ok":1)`)} else {render(res, `{"ok":0}`)}},'/api/loginpost': (req, res) => {let post = ''req.on('data', chunk => {post += chunk})req.on('end', () => {console.log(post)post = JSON.parse(post)if(post.username === 'admin' && post.password === '123456') {render(res, `{"ok":1}`)}else {render(res, `{"ok":0}`)}})}
}
module.exports = apiRouter
// route.js
const fs = require('fs')function render(res, path, type="") {res.writeHead(200, { 'Content-Type': `${type?type:'text/html'};charset=utf-8` })res.write(fs.readFileSync(path), 'utf-8')res.end()
}const route = {"/login": (req,res) => {render(res, './static/login.html')},"/home": (req,res) => {render(res, './static/home.html')},"/404": (req,res) => {res.writeHead(404, { 'Content-Type': 'text/html;charset=utf-8' })res.write(fs.readFileSync('./static/404.html'), 'utf-8')res.end()},"/favicon.ico": (req,res) => {render(res, './static/favicon.ico', 'image/x-icon')}
}
module.exports = route
// server.js
const http = require('http');
const Router = {}
const use = (obj) => {Object.assign(Router, obj)
}
const start = () => {http.createServer(function (req, res) {const myURL = new URL(req.url, 'http://127.0.0.1')try {Router[myURL.pathname](req, res)} catch (error) {Router['/404'](req, res)}}).listen(3000, () => {console.log("server start")})
}
exports.start = start
exports.use = use
// index.js
const server = require('./server');
const route = require('./route');
const api = require('./api');
// 注册路由
server.use(route)
server.use(api)
server.start()
<!-- login.html -->
<!DOCTYPE html>
<html lang="en"><head><meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><title>Document</title>
</head><body><div>用户名:<input type="text" class="username"></div><div>密码:<input type="password" class="password"></div><div><button class="login">登录-get</button><button class="loginpost">登录-post</button></div><script>const ologin = document.querySelector('.login')const ologinpost = document.querySelector('.loginpost')const password = document.querySelector('.password')const username = document.querySelector('.username')// get 请求ologin.onclick = () => {// console.log(username.value, password.value)fetch(`/api/login?username=${username.value}&password=${password.value}`).then(res => res.text()).then(res => {console.log(res)})}// post 请求ologinpost.onclick = () => {fetch('api/loginpost',{method: 'post',headers: {'Content-Type': 'application/json'},body: JSON.stringify({username: username.value,password: password.value})}).then(res => res.text()).then(res=> {console.log(res)})}</script>
</body></html>
静态资源管理
成为静态资源文件夹static
,可以直接输入类似于login.html
或者login
进行访问(忽略static/
)。
<!-- login.html -->
<html lang="en"><head><meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><title>Document</title><link rel="stylesheet" href="/css/login.css">
</head><body><div>用户名:<input type="text" class="username"></div><div>密码:<input type="password" class="password"></div><div><button class="login">登录-get</button><button class="loginpost">登录-post</button></div><script src="/js/login.js"></script>
</body></html>
/* login.css */
div {background-color: pink;
}
// login.js
const ologin = document.querySelector('.login')
const ologinpost = document.querySelector('.loginpost')
const password = document.querySelector('.password')
const username = document.querySelector('.username')
// get 请求
ologin.onclick = () => {// console.log(username.value, password.value)fetch(`/api/login?username=${username.value}&password=${password.value}`).then(res => res.text()).then(res => {console.log(res)})
}
// post 请求
ologinpost.onclick = () => {fetch('api/loginpost', {method: 'post',headers: {'Content-Type': 'application/json'},body: JSON.stringify({username: username.value,password: password.value})}).then(res => res.text()).then(res => {console.log(res)})
}
// api.js
function render(res, data, type = "") {res.writeHead(200, { 'Content-Type': `${type ? type : 'application/json'};charset=utf-8` })res.write(data)res.end()
}
const apiRouter = {'/api/login': (req, res) => {const myURL = new URL(req.url, 'http:/127.0.0.1')if (myURL.searchParams.get('username') === 'admin' && myURL.searchParams.get('password') === '123456') {render(res, `{"ok":1)`)} else {render(res, `{"ok":0}`)}},'/api/loginpost': (req, res) => {let post = ''req.on('data', chunk => {post += chunk})req.on('end', () => {console.log(post)post = JSON.parse(post)if (post.username === 'admin' && post.password === '123456') {render(res, `{"ok":1}`)} else {render(res, `{"ok":0}`)}})}
}
module.exports = apiRouter
// index.js
const server = require('./server');
const route = require('./route');
const api = require('./api');
// 注册路由
server.use(route)
server.use(api)
server.start()
// route.js
const fs = require('fs')
const mime = require('mime')
const path = require('path')
function render(res, path, type = "") {res.writeHead(200, { 'Content-Type': `${type ? type : 'text/html'};charset=utf-8` })res.write(fs.readFileSync(path), 'utf-8')res.end()
}const route = {"/login": (req, res) => {render(res, './static/login.html')},"/": (req, res) => {render(res, './static/home.html')},"/home": (req, res) => {render(res, './static/home.html')},"/404": (req, res) => {// 校验静态资源能否读取if(readStaticFile(req, res)) {return }res.writeHead(404, { 'Content-Type': 'text/html;charset=utf-8' })res.write(fs.readFileSync('./static/404.html'), 'utf-8')res.end()},// 静态资源下没有必要写该 ico 文件加载// "/favicon.ico": (req, res) => {// render(res, './static/favicon.ico', 'image/x-icon')// }
}// 静态资源管理
function readStaticFile(req, res) {const myURL = new URL(req.url, 'http://127.0.0.1:3000')// __dirname 获取当前文件夹的绝对路径 path 有以统一形式拼接路径的方法,拼接绝对路径const pathname = path.join(__dirname, '/static', myURL.pathname)if (myURL.pathname === '/') return falseif (fs.existsSync(pathname)) {// 在此访问静态资源// mime 存在获取文件类型的方法// split 方法刚好截取文件类型render(res, pathname, mime.getType(myURL.pathname.split('.')[1]))return true} else {return false}
}
module.exports = route
// server.js
const http = require('http');
const Router = {}
const use = (obj) => {Object.assign(Router, obj)
}
const start = () => {http.createServer(function (req, res) {const myURL = new URL(req.url, 'http://127.0.0.1')try {Router[myURL.pathname](req, res)} catch (error) {Router['/404'](req, res)}}).listen(3000, () => {console.log("server start")})
}
exports.start = start
exports.use = use
相关文章:
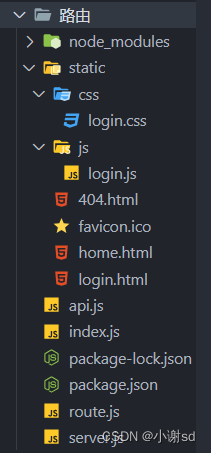
【Node.js】路由
基础使用 写法一: // server.js const http require(http); const fs require(fs); const route require(./route) http.createServer(function (req, res) {const myURL new URL(req.url, http://127.0.0.1)route(res, myURL.pathname)res.end() }).listen…...
matlab 2ask 4ask 信号调制
1 matlab 2ask close all clear all clcL =1000;Rb=2822400;%码元速率 Fs =Rb*8; Fc=Rb*30;%载波频率 Ld =L*Fs/Rb;%产生载波信号 t =0:1/Fs:L/Rb;carrier&...
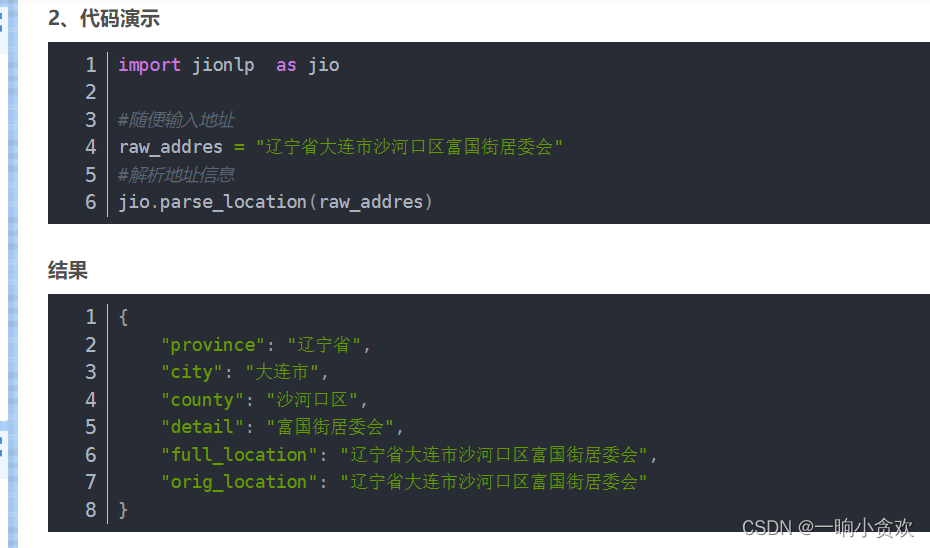
Python利用jieba分词提取字符串中的省市区(字符串无规则)
目录 背景库(jieba)代码拓展结尾 背景 今天的需求就是在一串字符串中提取包含,省、市、区,该字符串不是一个正常的地址;,如下字符串 "安徽省、浙江省、江苏省、上海市,冷运标快首重1kg价格xx元,1.01kg(含)-5kg(不含)续重价…...
MuLogin防关联浏览器帮您一键实现Facebook账号多开
导言: 在当今数字化时代,社交媒体应用程序的普及程度越来越高。Facebook作为全球最大的社交媒体平台之一,拥有数十亿的用户。然而,对于一些用户来说,只拥有一个Facebook账号可能无法满足他们的需求。有时,…...

【C语言】每日一题(半月斩)——day4
目录 选择题 1、设变量已正确定义,以下不能统计出一行中输入字符个数(不包含回车符)的程序段是( ) 2、运行以下程序后,如果从键盘上输入 65 14<回车> ,则输出结果为( &…...
Are you sure you want to continue connecting (yes/no) 每次ssh进
Lunix scp等命令不需要输入yes确认方法_scp不需要确认-CSDN博客 方法一:连接时加入StrictHostKeyCheckingno ssh -o StrictHostKeyCheckingno root192.168.1.100 方法二:修改/etc/ssh/ssh_config配置文件,添加: StrictHostKeyC…...
网络与信息系统安全设计规范
1、总则 1.1、目的 为规范XXXXX单位信息系统安全设计过程,确保整个信息安全管理体系在信息安全设计阶段符合国家相关标准和要求,特制订本规范。 1.2、范围 本规范适用于XXXXX单位在信息安全设计阶段的要求和规范管理。 1.3、职责 网络安全与信息化…...
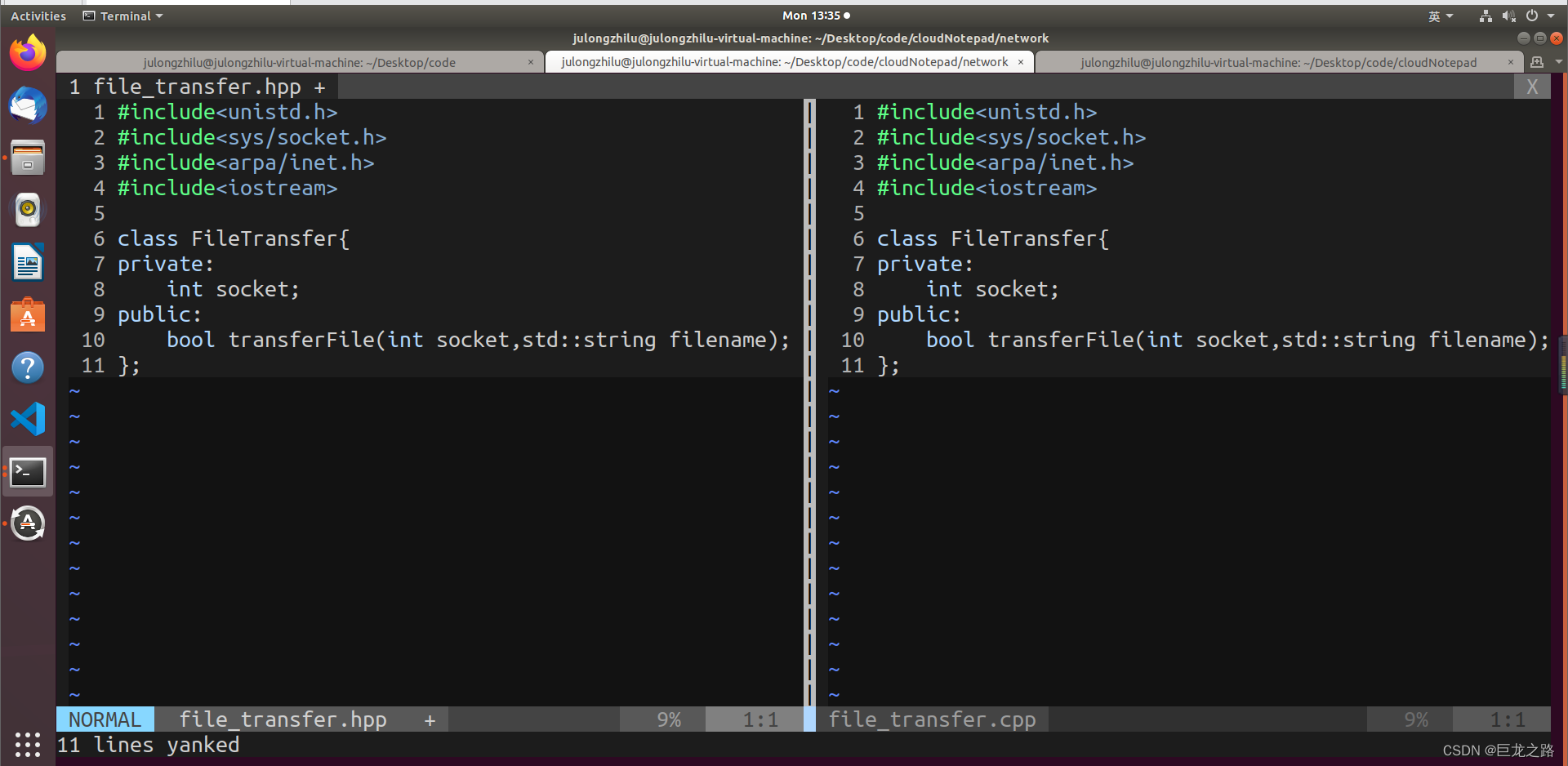
在Linux怎么用vim实现把一个文件里面的文本复制到另一个文件里面
2023年10月9日,周一下午 我昨天遇到了这个问题,但在网上没找到图文并茂的博客,于是我自己摸索出解决办法后,决定写一篇图文并茂的博客。 情景 假设现在我要用vim把file_transfer.cpp的内容复制到file_transfer.hpp里面 第一步 …...
CCAK—云审计知识证书学习
目录 一、CCAK云审计知识证书概述 二、云治理概述 三、云信任 四、构建云合规计划 <...
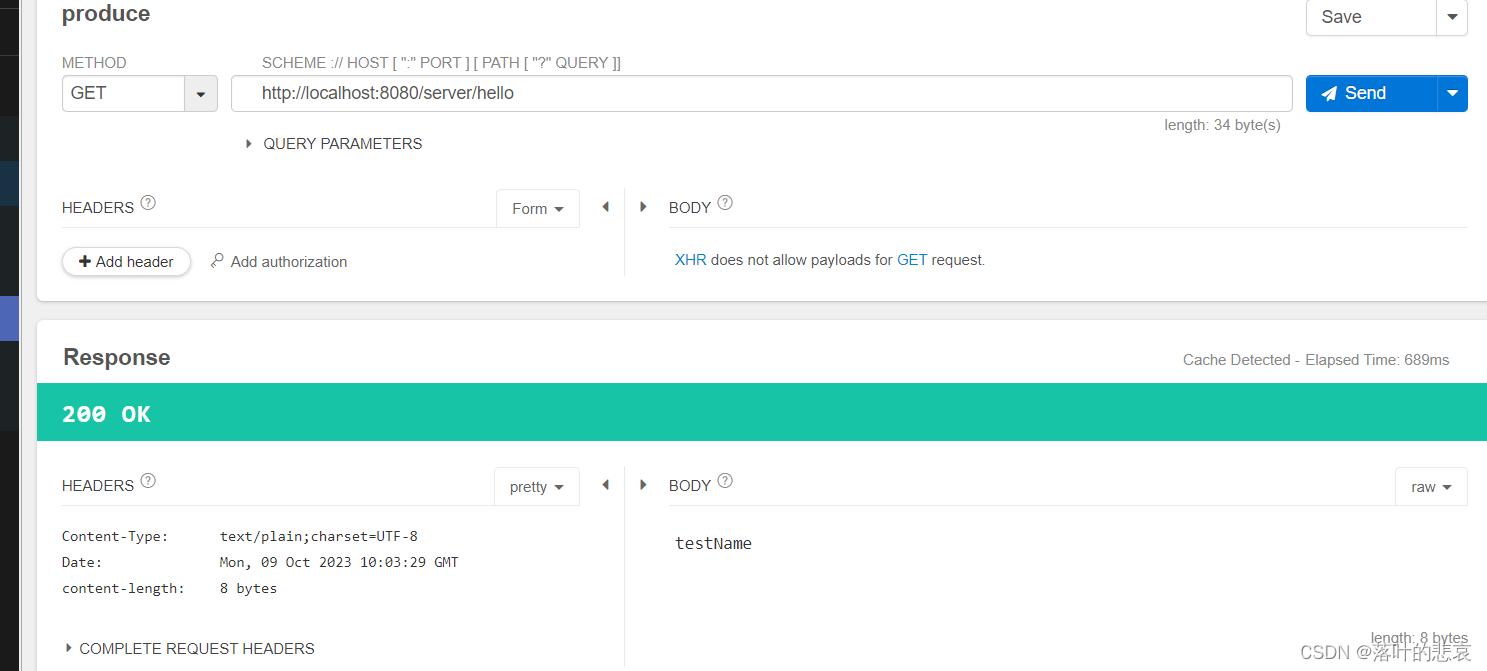
3.springcloudalibaba gateway项目搭建
文章目录 前言一、搭建gateway项目1.1 pom配置1.2 新增配置如下 二、新增server服务2.1 pom配置2.2新增测试接口如下 三、测试验证3.1 分别启动两个服务,查看nacos是否注册成功3.2 测试 总结 前言 前面已经完成了springcloudalibaba项目搭建,接下来搭建…...
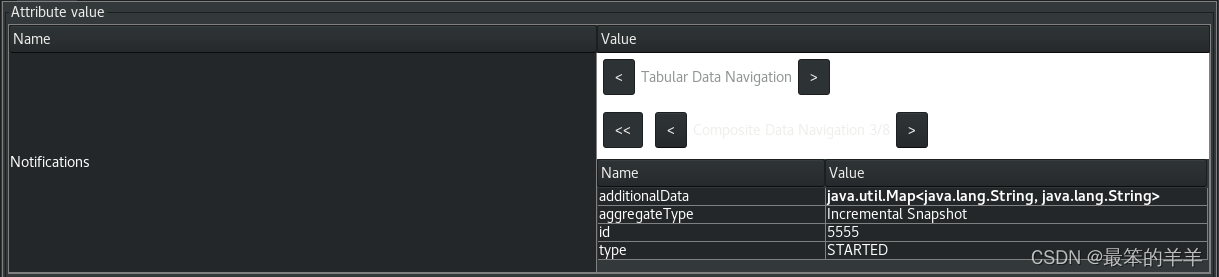
Debezium日常分享系列之:Debezium 2.3.0.Final发布
Debezium日常分享系列之:Debezium 2.3.0.Final发布 一、重大改变二、PostgreSQL / MySQL 安全连接更改三、JDBC 存储编码更改四、新功能和改进五、Kubernetes 的 Debezium Server Operator六、新的通知子系统七、新的可扩展信号子系统八、JMX 信号和通知集成九、新的…...
js为什么是单线程?
基础 js为什么是单线程? 多线程问题 类比操作系统,多线程问题有: 单一资源多线程抢占,引起死锁问题;线程间同步数据问题; 总结 为了简单: 更简单的dom渲染。js可以操控dom,而一…...
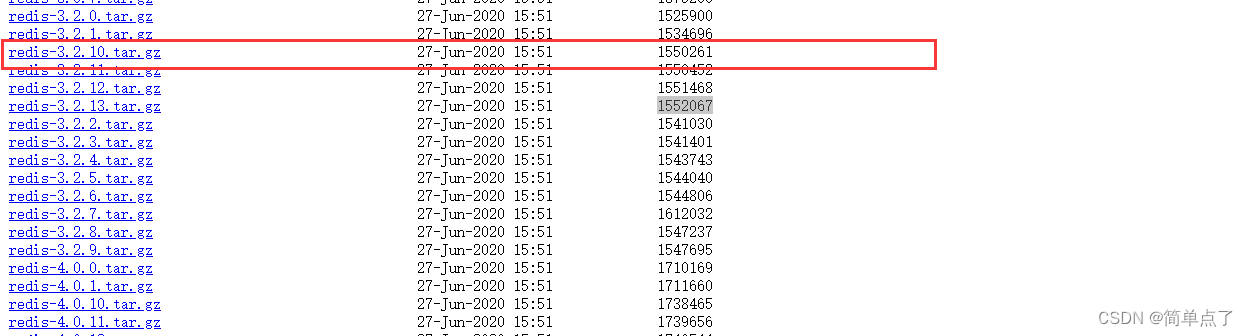
centos安装redis教程
centos安装redis教程 安装的版本为centos7.9下的redis3.2.100版本 1.下载地址 Index of /releases/ 使用xftp将redis传上去。 2.解压 tar -zxvf 文件名.tar.gz 3.安装 首先,确保系统已经安装了GCC编译器和make工具。可以使用以下命令进行安装: sudo y…...
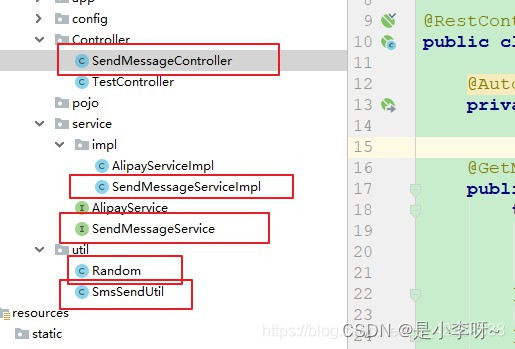
把短信验证码储存在Redis
校验短信验证码 接着上一篇博客https://blog.csdn.net/qq_42981638/article/details/94656441,成功实现可以发送短信验证码之后,一般可以把验证码存放在redis中,并且设置存放时间,一般短信验证码都是1分钟或者90s过期,…...
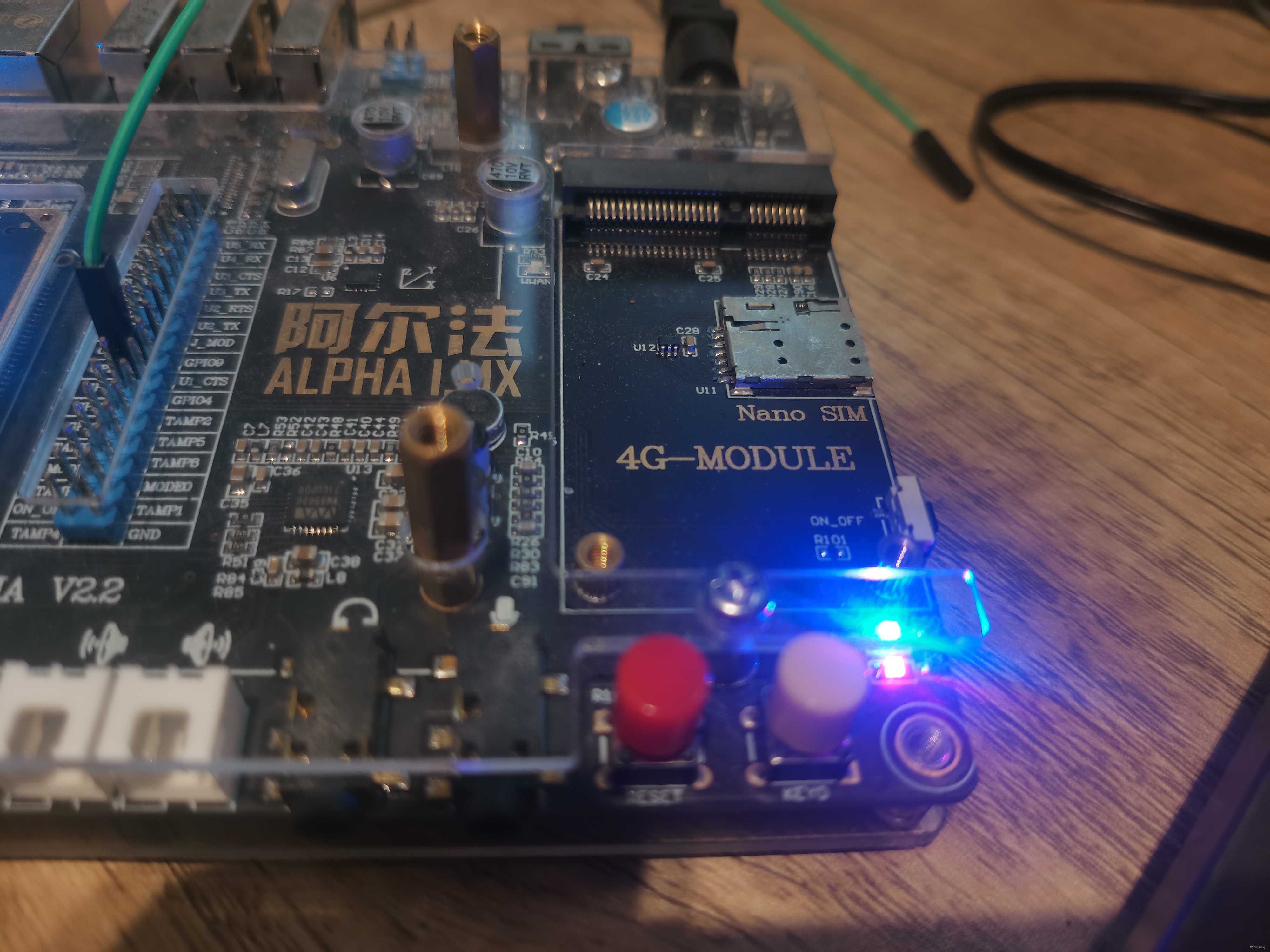
【已编译资料】基于正点原子alpha开发板的第三篇系统移植
系统移植的三大步骤如下: 系统uboot移植系统linux移植系统rootfs制作 一言难尽,踩了不少坑,当时只是想学习驱动开发,发现必须要将第三篇系统移植弄好才可以学习后面驱动,现将移植好的文件分享出来: 仓库&…...
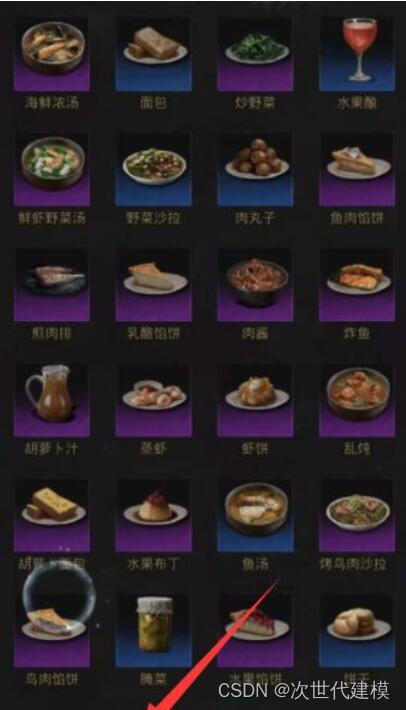
地下城堡3魂之诗食谱,地下城堡3菜谱37种
地下城堡3魂之诗食谱大全,让你解锁制作各种美食的方法!不同的食材搭配不同的配方制作,食物效果和失效也迥异。但有时候我们可能会不知道如何制作这些食物,下面为您介绍地下城堡3菜谱37种。 关注【娱乐天梯】,获取内部福…...
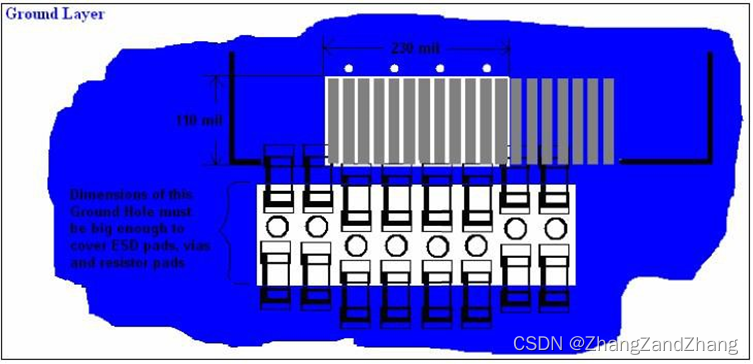
HDMI 基于 4 层 PCB 的布线指南
HDMI 基于 4 层 PCB 的布线指南 简介 HDMI 规范文件里面规定其差分线阻抗要求控制在 100Ω 15%,其中 Rev.1.3a 里面规定相对放宽了一些,容忍阻抗失控在 100Ω 25%范围内,不要超过 250ps。 通常,在 PCB 设计时,注意控…...
理解Go中的布尔逻辑
布尔数据类型(bool)可以是两个值之一,true或false。布尔值在编程中用于比较和控制程序流程。 布尔值表示与数学逻辑分支相关的真值,它指示计算机科学中的算法。布尔(Boolean)一词以数学家乔治布尔(George Boole)命名,总是以大写字母B开头。 …...
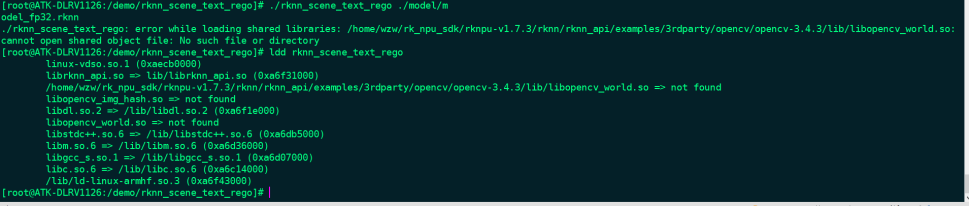
rv1126-rknpu-v1.7.3添加opencv库
rv1126所使用的rknn sdk里默认是不带opencv库的,官方所用的例程里也没有使用opencv,但是这样在进行图像处理的时候有点麻烦了,这里有两种办法: 一是先用python将所需要的图片处理好后在转化为bin格式文件,在使用c或c进行读取&…...
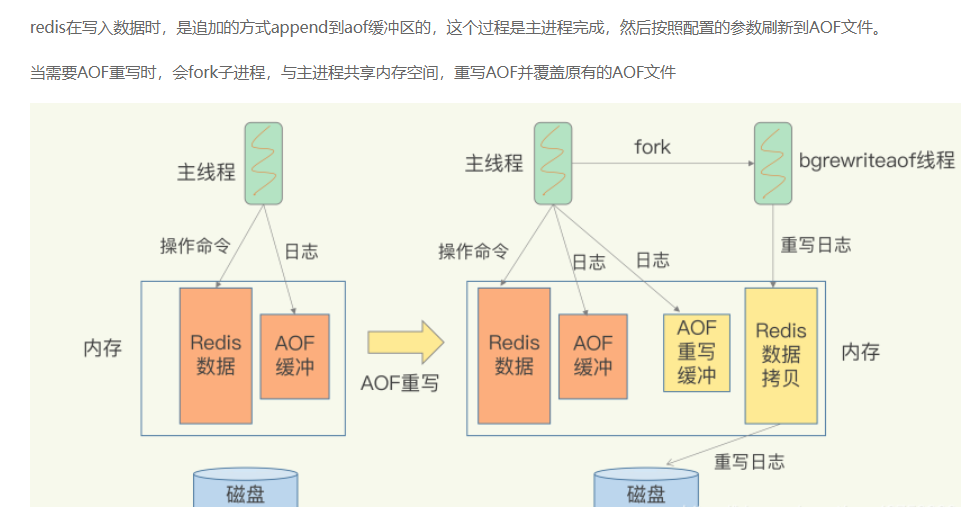
【Redis】Redis持久化深度解析
原创不易,注重版权。转载请注明原作者和原文链接 文章目录 Redis持久化介绍RDB原理Fork函数与写时复制关于写时复制的思考 RDB相关配置 AOF原理AOF持久化配置AOF文件解读AOF文件修复AOF重写AOF缓冲区与AOF重写缓存区AOF缓冲区可以替代AOF重写缓冲区吗AOF相关配置写后…...

Chapter03-Authentication vulnerabilities
文章目录 1. 身份验证简介1.1 What is authentication1.2 difference between authentication and authorization1.3 身份验证机制失效的原因1.4 身份验证机制失效的影响 2. 基于登录功能的漏洞2.1 密码爆破2.2 用户名枚举2.3 有缺陷的暴力破解防护2.3.1 如果用户登录尝试失败次…...
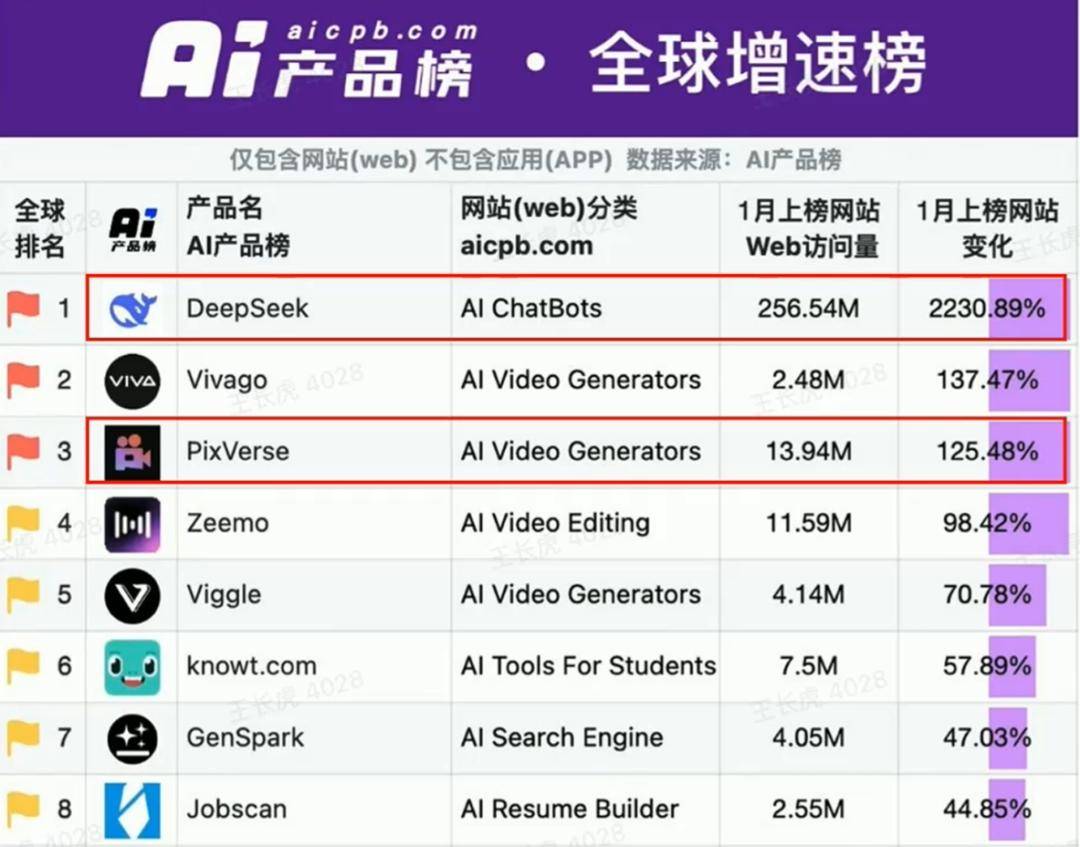
多模态2025:技术路线“神仙打架”,视频生成冲上云霄
文|魏琳华 编|王一粟 一场大会,聚集了中国多模态大模型的“半壁江山”。 智源大会2025为期两天的论坛中,汇集了学界、创业公司和大厂等三方的热门选手,关于多模态的集中讨论达到了前所未有的热度。其中,…...
java_网络服务相关_gateway_nacos_feign区别联系
1. spring-cloud-starter-gateway 作用:作为微服务架构的网关,统一入口,处理所有外部请求。 核心能力: 路由转发(基于路径、服务名等)过滤器(鉴权、限流、日志、Header 处理)支持负…...
利用ngx_stream_return_module构建简易 TCP/UDP 响应网关
一、模块概述 ngx_stream_return_module 提供了一个极简的指令: return <value>;在收到客户端连接后,立即将 <value> 写回并关闭连接。<value> 支持内嵌文本和内置变量(如 $time_iso8601、$remote_addr 等)&a…...
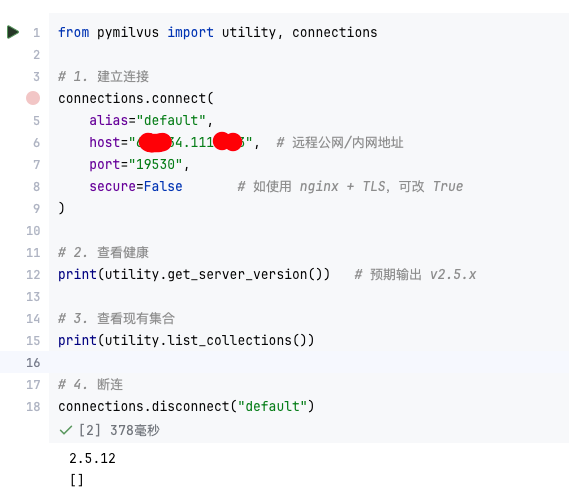
【大模型RAG】Docker 一键部署 Milvus 完整攻略
本文概要 Milvus 2.5 Stand-alone 版可通过 Docker 在几分钟内完成安装;只需暴露 19530(gRPC)与 9091(HTTP/WebUI)两个端口,即可让本地电脑通过 PyMilvus 或浏览器访问远程 Linux 服务器上的 Milvus。下面…...
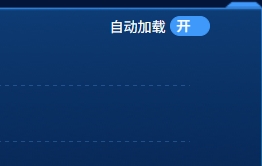
el-switch文字内置
el-switch文字内置 效果 vue <div style"color:#ffffff;font-size:14px;float:left;margin-bottom:5px;margin-right:5px;">自动加载</div> <el-switch v-model"value" active-color"#3E99FB" inactive-color"#DCDFE6"…...
ffmpeg(四):滤镜命令
FFmpeg 的滤镜命令是用于音视频处理中的强大工具,可以完成剪裁、缩放、加水印、调色、合成、旋转、模糊、叠加字幕等复杂的操作。其核心语法格式一般如下: ffmpeg -i input.mp4 -vf "滤镜参数" output.mp4或者带音频滤镜: ffmpeg…...
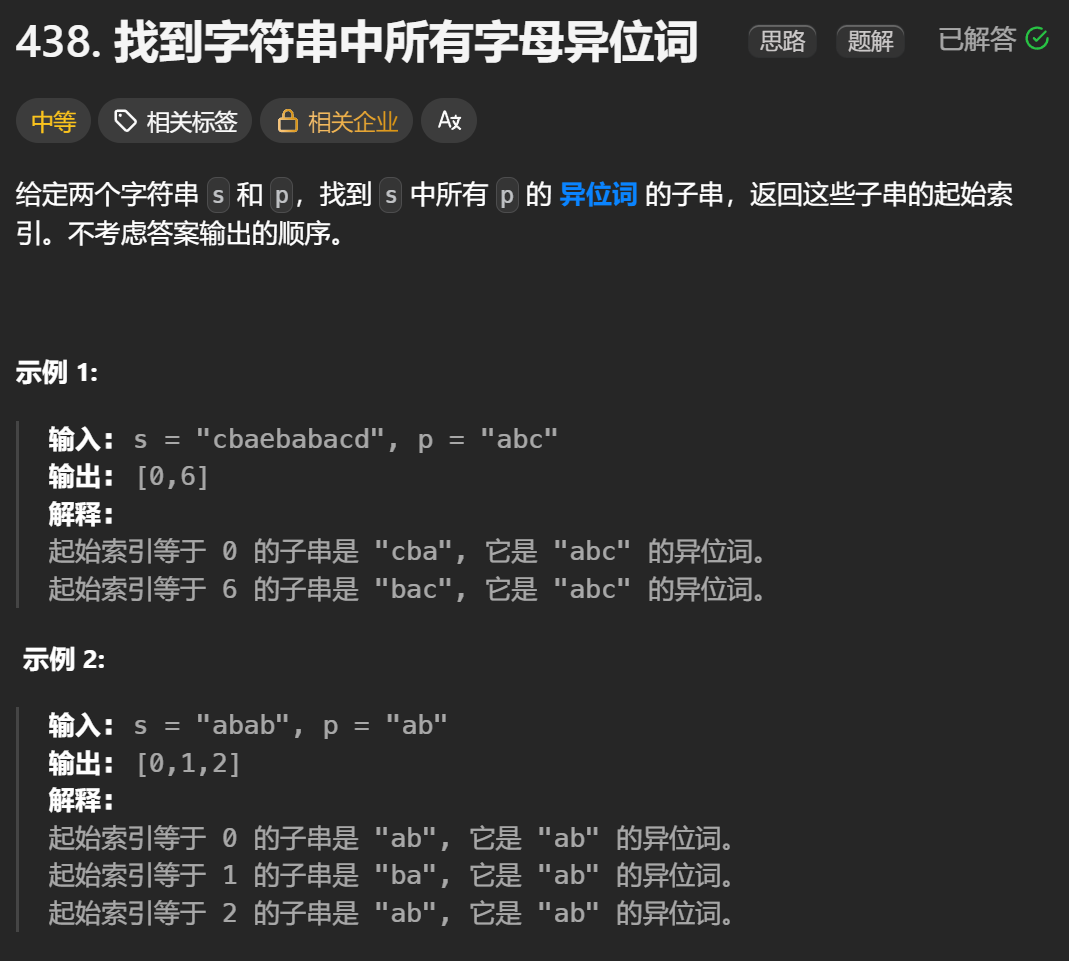
12.找到字符串中所有字母异位词
🧠 题目解析 题目描述: 给定两个字符串 s 和 p,找出 s 中所有 p 的字母异位词的起始索引。 返回的答案以数组形式表示。 字母异位词定义: 若两个字符串包含的字符种类和出现次数完全相同,顺序无所谓,则互为…...
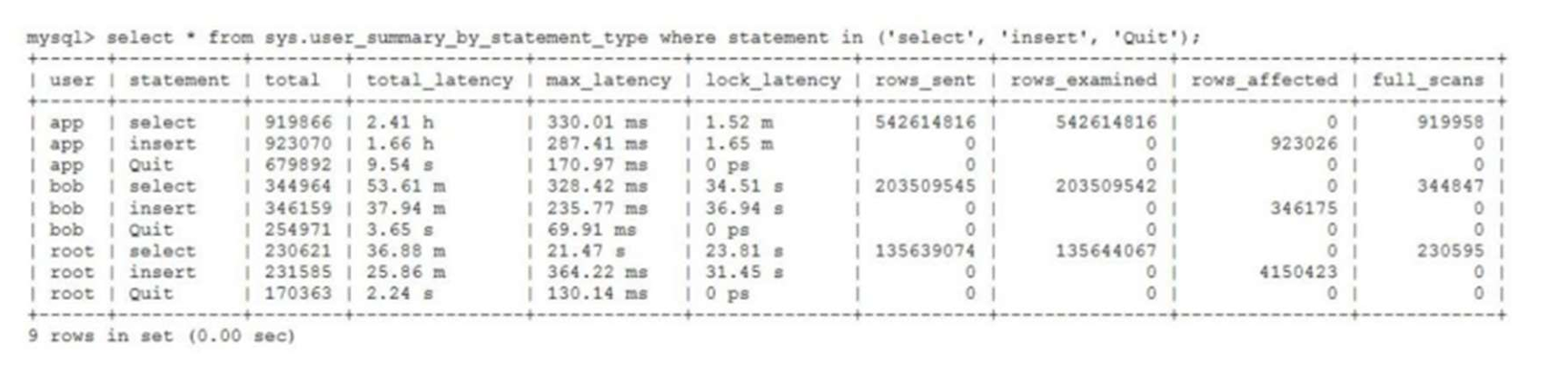
MySQL 8.0 OCP 英文题库解析(十三)
Oracle 为庆祝 MySQL 30 周年,截止到 2025.07.31 之前。所有人均可以免费考取原价245美元的MySQL OCP 认证。 从今天开始,将英文题库免费公布出来,并进行解析,帮助大家在一个月之内轻松通过OCP认证。 本期公布试题111~120 试题1…...
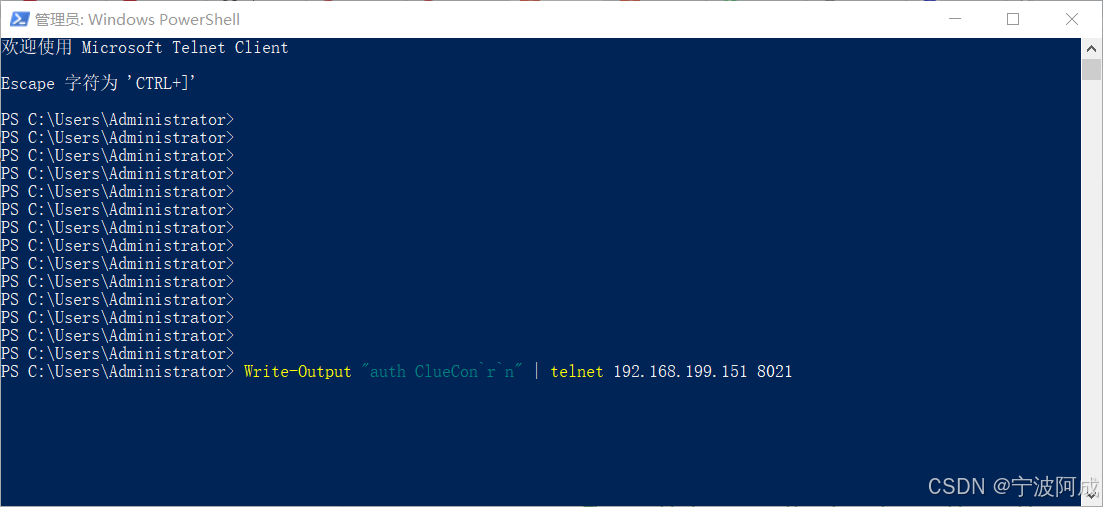
用docker来安装部署freeswitch记录
今天刚才测试一个callcenter的项目,所以尝试安装freeswitch 1、使用轩辕镜像 - 中国开发者首选的专业 Docker 镜像加速服务平台 编辑下面/etc/docker/daemon.json文件为 {"registry-mirrors": ["https://docker.xuanyuan.me"] }同时可以进入轩…...