python INI文件操作与configparser内置库
目录
INI文件
configparser内置库
类与方法
操作实例
导入INI
查询所有节的列表
判断某个节是否存在
查询某个节的所有键的列表
判断节下是否存在某个键
增加节点
删除节点
增加节点的键
修改键值
保存修改结果
获取键值
获取节点所有键值
INI文件
即Initialization File的缩写,是Windows系统配置文件所采用的存储格式,用于统管Windows的各项配置。虽然Windows 95之后引入了注册表的概念,使得许多参数和初始化信息被存储在注册表中,但在某些场合,INI文件仍然具有其不可替代的地位。
INI文件是一种按照特殊方式排列的文本文件,其格式规范包括节(section)、键(name)和值(value)。节用方括号括起来,单独占一行,用于表示一个段落,区分不同用途的参数区。键(也称为属性)单独占一行,用等号连接键名和键值,例如“name=value”。注释使用英文分号(;)开头,单独占一行,分号后面的文字直到该行结尾都作为注释处理。
INI文件在Windows系统中非常常见,其中最重要的是“System.ini”、“System32.ini”和“Win.ini”等文件。这些文件主要存放用户所做的选择以及系统的各种参数。用户可以通过修改INI文件来改变应用程序和系统的很多配置。当然,我们自己编写程序时也可以把INI文件作为配置和管理参数的工具,比如python中就有内置库configparser可以方便地配置和管理程序的参数。
configparser内置库
类与方法
Intrinsic defaults can be specified by passing them into the ConfigParser constructor as a dictionary.
class:
ConfigParser -- responsible for parsing a list of configuration files, and managing the parsed database.
methods:
__init__(defaults=None, dict_type=_default_dict, allow_no_value=False,
delimiters=('=', ':'), comment_prefixes=('#', ';'),
inline_comment_prefixes=None, strict=True,
empty_lines_in_values=True, default_section='DEFAULT',
interpolation=<unset>, converters=<unset>):
Create the parser. When `defaults` is given, it is initialized into the dictionary or intrinsic defaults. The keys must be strings, the values must be appropriate for %()s string interpolation.
When `dict_type` is given, it will be used to create the dictionary objects for the list of sections, for the options within a section, and for the default values.
When `delimiters` is given, it will be used as the set of substrings that divide keys from values.
When `comment_prefixes` is given, it will be used as the set of substrings that prefix comments in empty lines. Comments can be indented.
When `inline_comment_prefixes` is given, it will be used as the set of substrings that prefix comments in non-empty lines.
When `strict` is True, the parser won't allow for any section or option
duplicates while reading from a single source (file, string or
dictionary). Default is True.
When `empty_lines_in_values` is False (default: True), each empty line marks the end of an option. Otherwise, internal empty lines of a multiline option are kept as part of the value.
When `allow_no_value` is True (default: False), options without values are accepted; the value presented for these is None.
When `default_section` is given, the name of the special section is named accordingly. By default it is called ``"DEFAULT"`` but this can be customized to point to any other valid section name. Its current value can be retrieved using the ``parser_instance.default_section`` attribute and may be modified at runtime.
When `interpolation` is given, it should be an Interpolation subclass instance. It will be used as the handler for option value pre-processing when using getters. RawConfigParser objects don't do any sort of interpolation, whereas ConfigParser uses an instance of BasicInterpolation. The library also provides a ``zc.buildout`` inspired ExtendedInterpolation implementation.
When `converters` is given, it should be a dictionary where each key represents the name of a type converter and each value is a callable implementing the conversion from string to the desired datatype. Every converter gets its corresponding get*() method on the parser object and section proxies.
sections()
Return all the configuration section names, sans DEFAULT.
has_section(section)
Return whether the given section exists.
has_option(section, option)
Return whether the given option exists in the given section.
options(section)
Return list of configuration options for the named section.
read(filenames, encoding=None)
Read and parse the iterable of named configuration files, given by name. A single filename is also allowed. Non-existing files are ignored. Return list of successfully read files.
read_file(f, filename=None)
Read and parse one configuration file, given as a file object.
The filename defaults to f.name; it is only used in error messages (if f has no `name` attribute, the string `<???>` is used).
read_string(string)
Read configuration from a given string.
read_dict(dictionary)
Read configuration from a dictionary. Keys are section names, values are dictionaries with keys and values that should be present in the section. If the used dictionary type preserves order, sections and their keys will be added in order. Values are automatically converted to strings.
get(section, option, raw=False, vars=None, fallback=_UNSET)
Return a string value for the named option. All % interpolations are expanded in the return values, based on the defaults passed into the constructor and the DEFAULT section. Additional substitutions may be provided using the `vars` argument, which must be a dictionary whose contents override any pre-existing defaults. If `option` is a key in `vars`, the value from `vars` is used.
getint(section, options, raw=False, vars=None, fallback=_UNSET)
Like get(), but convert value to an integer.
getfloat(section, options, raw=False, vars=None, fallback=_UNSET)
Like get(), but convert value to a float.
getboolean(section, options, raw=False, vars=None, fallback=_UNSET)
Like get(), but convert value to a boolean (currently case insensitively defined as 0, false, no, off for False, and 1, true, yes, on for True). Returns False or True.
items(section=_UNSET, raw=False, vars=None)
If section is given, return a list of tuples with (name, value) for each option in the section. Otherwise, return a list of tuples with (section_name, section_proxy) for each section, including DEFAULTSECT.
remove_section(section)
Remove the given file section and all its options.
remove_option(section, option)
Remove the given option from the given section.
set(section, option, value)
Set the given option.
write(fp, space_around_delimiters=True)
Write the configuration state in .ini format. If `space_around_delimiters` is True (the default), delimiters between keys and values are surrounded by spaces.
操作实例
就以我电脑上的win.ini的内容作操作对象,为防止乱改windows参数,把win.ini复制到源代码目录中并改名为exam.ini。
; for 16-bit app support
[fonts]
[extensions]
[mci extensions]
[files]
[Mail]
MAPI=1
导入INI
>>> import configparser
>>> parser = configparser.ConfigParser()
>>> parser.read('exam.ini')
['exam.ini']
查询所有节的列表
>>> parser.sections()
['fonts', 'extensions', 'mci extensions', 'files', 'Mail']
判断某个节是否存在
>>> parser.has_section('fonts')
True
>>> parser.has_section('font')
False
>>> parser.has_section('files')
True
查询某个节的所有键的列表
>>> parser.options('Mail')
['mapi']
>>> parser.options('mail')
Traceback (most recent call last):
File "<pyshell#21>", line 1, in <module>
parser.options('mail')
File "D:\Program Files\Python\Lib\configparser.py", line 661, in options
raise NoSectionError(section) from None
configparser.NoSectionError: No section: 'mail'
>>> parser.options('files')
[]
>>> parser.options('Files')
Traceback (most recent call last):
File "<pyshell#31>", line 1, in <module>
parser.options('Files')
File "D:\Program Files\Python\Lib\configparser.py", line 661, in options
raise NoSectionError(section) from None
configparser.NoSectionError: No section: 'Files'
注意:节名区别字母大小写。
判断节下是否存在某个键
>>> parser.has_option('Mail','mapi')
True
>>> parser.has_option('Mail','Mapi')
True
>>> parser.has_option('Mail','MAPI')
True
>>> parser.has_option('Mail','abc')
False
>>> parser.has_option('Mail','ABC')
False
注意:键名不区别字母大小写。
增加节点
>>> parser.add_section('Names')
>>> parser.sections()
['fonts', 'extensions', 'mci extensions', 'files', 'Mail', 'Names']
>>> parser.add_section('names')
>>> parser.sections()
['fonts', 'extensions', 'mci extensions', 'files', 'Mail', 'Names', 'names']
注意:增加已在节,会抛错DuplicateSectionError(section)
>>> parser.add_section('names')
Traceback (most recent call last):
File "<pyshell#47>", line 1, in <module>
parser.add_section('names')
File "D:\Program Files\Python\Lib\configparser.py", line 1189, in add_section
super().add_section(section)
File "D:\Program Files\Python\Lib\configparser.py", line 645, in add_section
raise DuplicateSectionError(section)
configparser.DuplicateSectionError: Section 'names' already exists
正确用法,配合has_section()一起使用
>>> if not parser.has_section('Level'):
... parser.add_section('Level')
...
>>> parser.sections()
['fonts', 'extensions', 'files', 'Mail', 'names', 'Level']
删除节点
>>> parser.sections()
['fonts', 'extensions', 'mci extensions', 'files', 'Mail', 'Names', 'names']
>>> parser.remove_section('Names')
True
>>> parser.remove_section('file')
False
>>> parser.remove_section('mci extensions')
True
>>> parser.sections()
['fonts', 'extensions', 'files', 'Mail', 'names']
注意:是否删除成功,由返回值True或False来判断。
增加节点的键
>>> parser.options('Mail')
['mapi']
>>> if not parser.has_option("Mail", "names"):
... parser.set("Mail", "names", "")
...
...
>>> parser.options('Mail')
['mapi', 'names']
修改键值
与增加键一样用set(),但value参数不为空。
>>> parser.set("Mail", "names", "Hann")
或者写成:
parser.set(section="Mail", option="names", value="Hann")
保存修改结果
>>> parser.write(fp=open('exam.ini', 'w'))
注意:增删等改变ini文件内容的操作都要write才能得到保存。
获取键值
>>> parser.get("Mail", "names")
'Hann'
>>> parser.get("Mail", "Names")
'Hann'
>>> parser.get("mail", "Names")
Traceback (most recent call last):
File "<pyshell#81>", line 1, in <module>
parser.get("mail", "Names")
File "D:\Program Files\Python\Lib\configparser.py", line 759, in get
d = self._unify_values(section, vars)
File "D:\Program Files\Python\Lib\configparser.py", line 1130, in _unify_values
raise NoSectionError(section) from None
configparser.NoSectionError: No section: 'mail'
注意:再次证明节名区别大小写,键名不区别大小写。
获取节点所有键值
>>> parser.items('Mail')
[('mapi', '1'), ('name', '"Hann"'), ('names', 'Tom'), ('kates', '')]
完整示例代码
import configparser # 创建一个配置解析器
parser = configparser.ConfigParser() # 读取INI文件
parser.read('exam.ini') # 检查是否成功读取了文件
if len(parser.sections()) == 0: print("INI文件为空或未找到指定的节。")
else: # 获取所有节的列表 sections = parser.sections() print("INI文件中的节:") for section in sections: print(section) # 获取指定节下的所有选项 section_name = 'Mail'if section_name in parser: options = parser[section_name] print(f"节 '{section_name}' 中的选项:") for option in options: print(f"{option}: {parser[section_name][option]}") # 获取指定节下的单个选项的值 option_name = 'Name' # 假设我们要获取的选项的名字是 'example_option' if option_name in options: value = parser.get(section_name, option_name) print(f"节 '{section_name}' 中 '{option_name}' 的值为:{value}") # 修改指定节下的单个选项的值 new_value = 'Name' parser.set(section_name, option_name, new_value) print(f"已将节 '{section_name}' 中 '{option_name}' 的值修改为:{new_value}") # 添加一个新的选项到指定节 new_option_name = 'new_option' new_option_value = 'option_value' parser.set(section_name, new_option_name, new_option_value) print(f"已在节 '{section_name}' 中添加了新选项 '{new_option_name}',其值为:{new_option_value}") # 删除指定节下的单个选项 parser.remove_option(section_name, new_option_name) print(f"已删除节 '{section_name}' 中的选项 '{new_option_name}'") # 添加一个新的节 new_section_name = 'new_section' parser.add_section(new_section_name) print(f"已添加新节 '{new_section_name}'") # 将修改后的配置写回文件 with open('exam.ini', 'w') as configfile: parser.write(configfile) print("修改已写回INI文件。") else: print(f"INI文件中未找到节 '{section_name}'。")
完
相关文章:
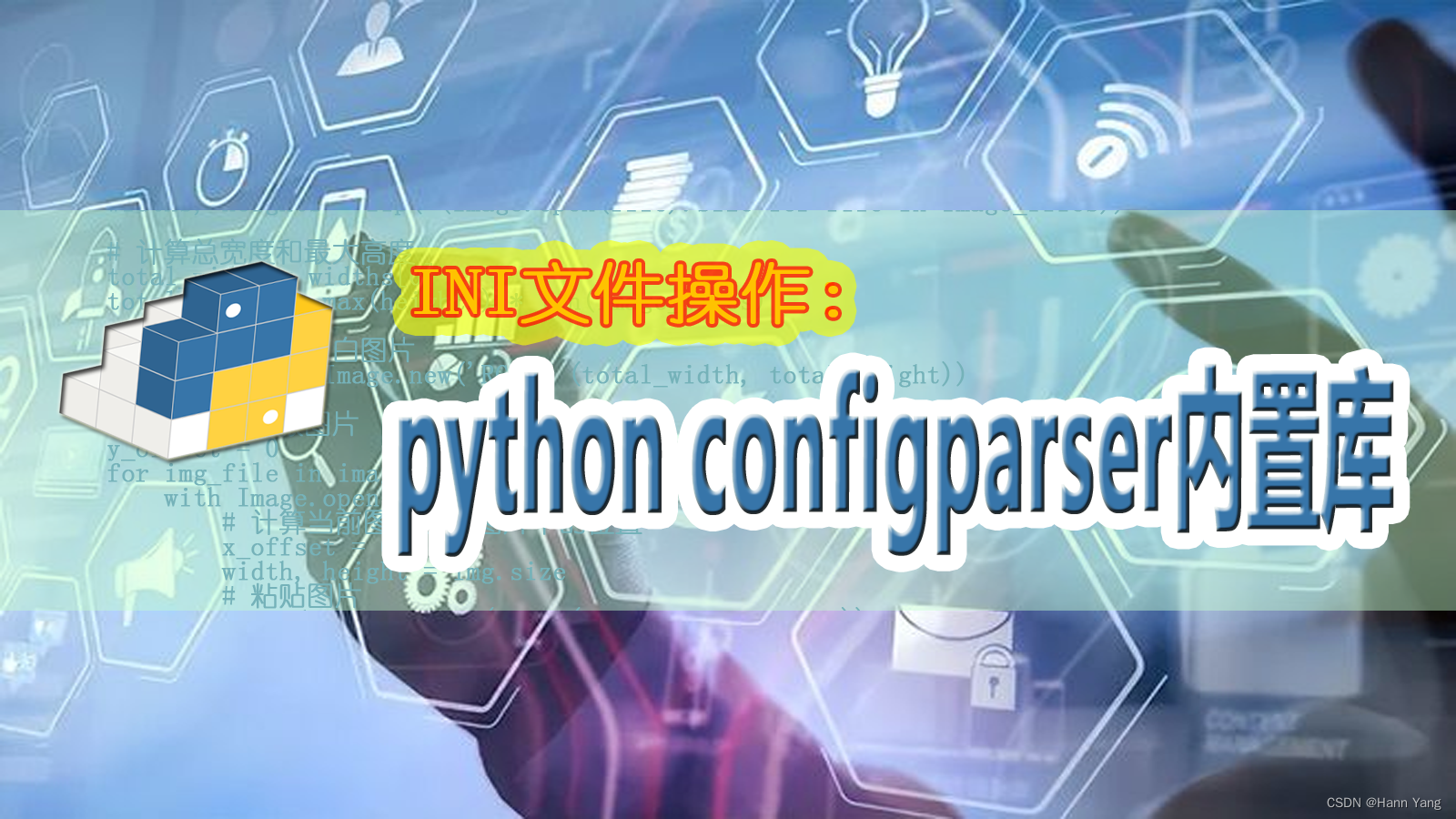
python INI文件操作与configparser内置库
目录 INI文件 configparser内置库 类与方法 操作实例 导入INI 查询所有节的列表 判断某个节是否存在 查询某个节的所有键的列表 判断节下是否存在某个键 增加节点 删除节点 增加节点的键 修改键值 保存修改结果 获取键值 获取节点所有键值 INI文件 即Initiali…...

软考笔记--软件系统质量属性
一.软件系统质量属性的概念 软件系统的质量就是“软件系统与明确地和隐含的定义的需求相一致的程度”。更具体地说,软件系统质量就是软件与明确地叙述的功能和性能需求文档中明确描述的开发标准以及任何专业开发的软件产品都应该具有的隐含特征相一致的程度。从管理…...
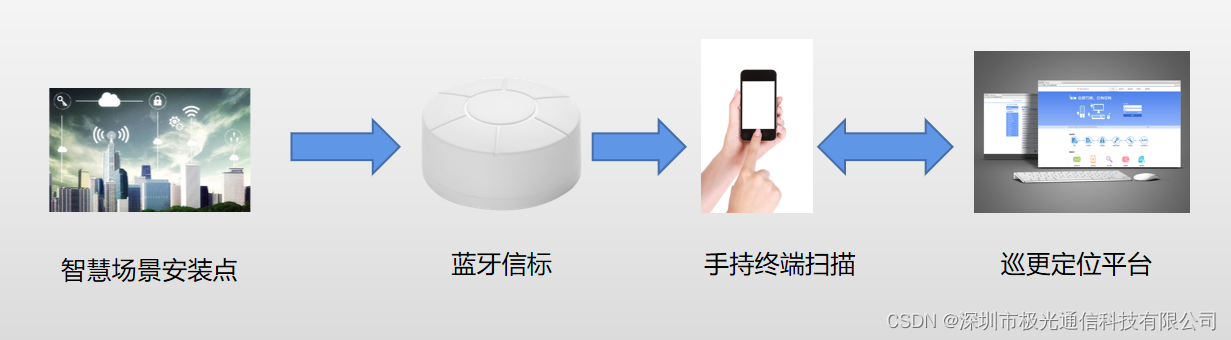
新型设备巡检方案-手机云巡检
随着科技的不断发展,设备巡检工作也在逐步向智能化、高效化方向转变。传统的巡检方式往往需要人工逐个设备检查,耗时耗力,效率低下,同时还容易漏检和误检。而新型设备巡检应用—手机蓝牙云巡检的出现,则为设备巡检工作…...

node.js 下 mysql2 的 CURD 功能极简封装
此封装适合于使用 SQL 直接操作数据库的小型后端项目,更多功能请查阅MySQL2官网 // 代码保存到单独的 js 文件const mysql require(mysql2/promise)const debug true let conn/*** 执行 SQL 语句* param {String} sql* param {*} params* returns {Array}*/ const…...
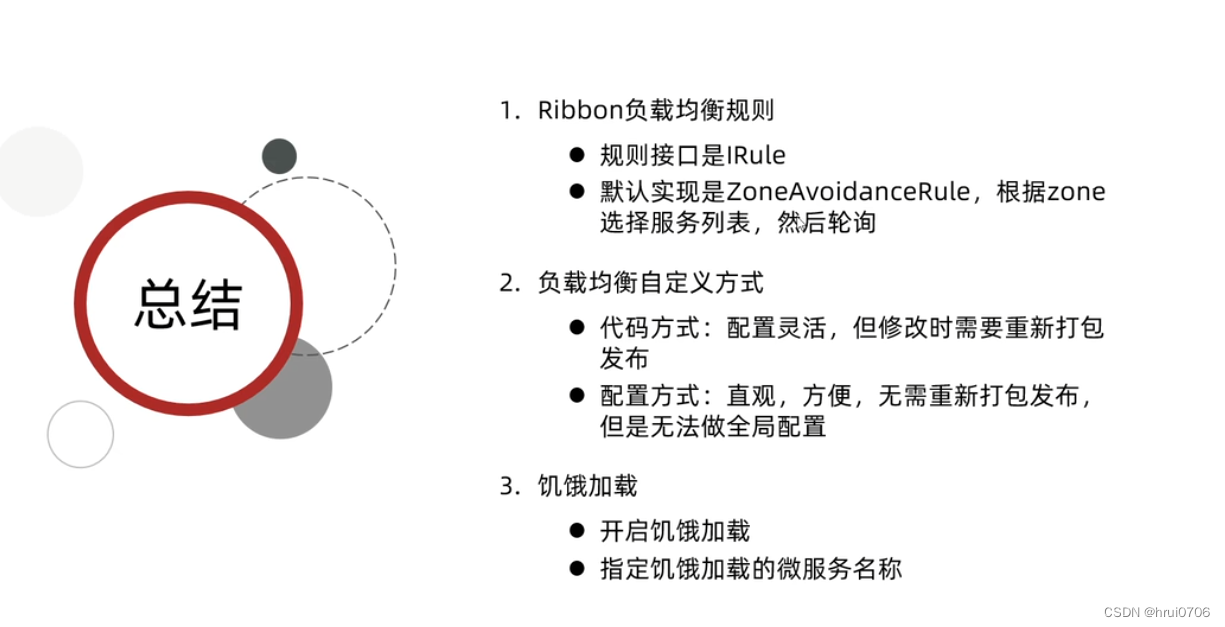
Cloud-Eureka服务治理-Ribbon负载均衡
构建Cloud父工程 父工程只做依赖版本管理 不引入依赖 pom.xml <packaging>pom</packaging><parent><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-parent</artifactId><version>2.3.9.RELEA…...
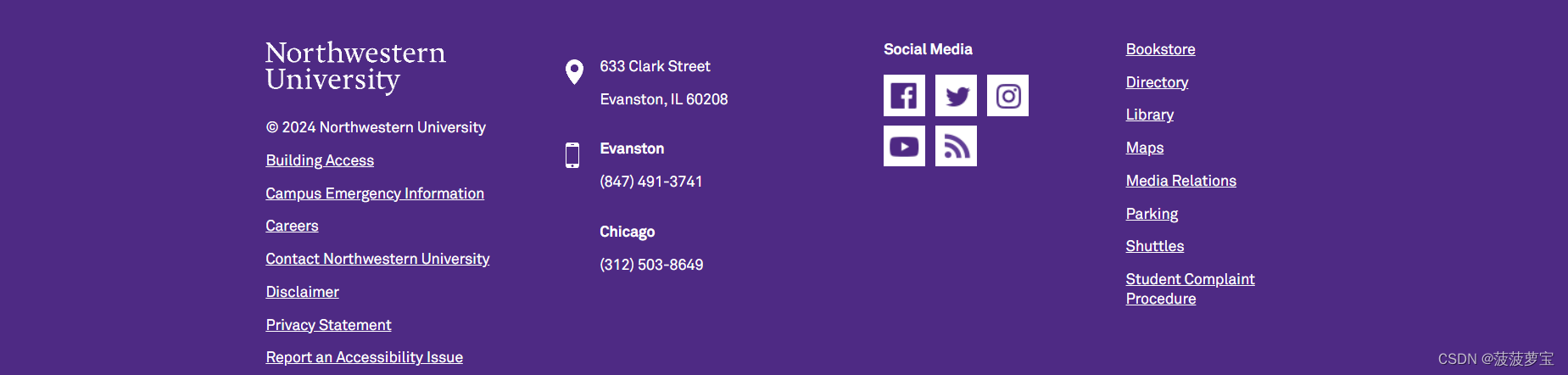
Northwestern University-844计算机科学与技术/软件工程-机试指南【考研复习】
本文提到的西北大学是位于密歇根湖泊畔的西北大学。西北大学(英语:Northwestern University,简称:NU)是美国的一所著名私立研究型大学。它由九人于1851年创立,目标是建立一所为西北领地地区的人服务的大学。…...

【Linux的网络编程】
1、OSI的七层网络模型有哪些,每一层有什么作用? 答:(1)应用层:负责处理不同应用程序之间的通信,需要满足提供的协议,确保数据发送方和接收方的正确。 (2)表…...

vue-seamless-scroll 点击事件不生效
问题:在使用此插件时发现,列表内容前几行还是能正常点击的,但是从第二次出现的列表开始就没有点击事件了 原因:因为html元素是复制出来的(滚动组件是将后面的复制出来一份,进行填铺页面,方便滚动…...

前端工程部署步骤小记
安装mqtt: “mqtt”: “^4.3.7”, npm install git panjiacheng 后台demo下载zip 1、npm install --registryhttps://registry.npmmirror.com 2、npm run dev 前端demo创建 1、安装npm 2、npm install vuenext 3、npm install -g vue/cli 查看版本 vue --version 4、更新插件…...

TS常见问题
文章目录 1. 什么是 TypeScript?它与 JavaScript 有什么区别?2. TS 泛型、接口、泛型工具record、Pick、Omit3. TS unknow和any的区别,如何告诉编译器unknow一定是某个类型?4. 元组与常规数组的区别5. 什么是泛型,有什么作用&…...

linux系统nginx常用命令
查nginx位置 find / -name nginx nginx目录:/usr/local/ 查看nginx进程号 ps -ef |grep nginx 停止进程 kill 2072 启动 ./sbin/nginx /usr/local/nginx/sbin/nginx -t -c /usr/local/nginx/conf/nginx.conf 启动并校验校验配置文件 ./sbin/nginx -t 看到如下显…...
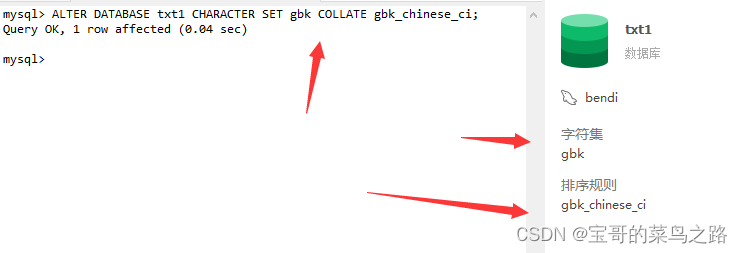
MySQl基础入门③
上一遍内容 接下来我们都使用navicat软件来操作数据了。 1.新建数据库 先创建我门自己的一个数据库 鼠标右键点击bendi那个绿色海豚的图标,然后选择新建数据库。 数据库名按自己喜好的填,不要写中文, 在 MySQL 8.0 中,最优的字…...
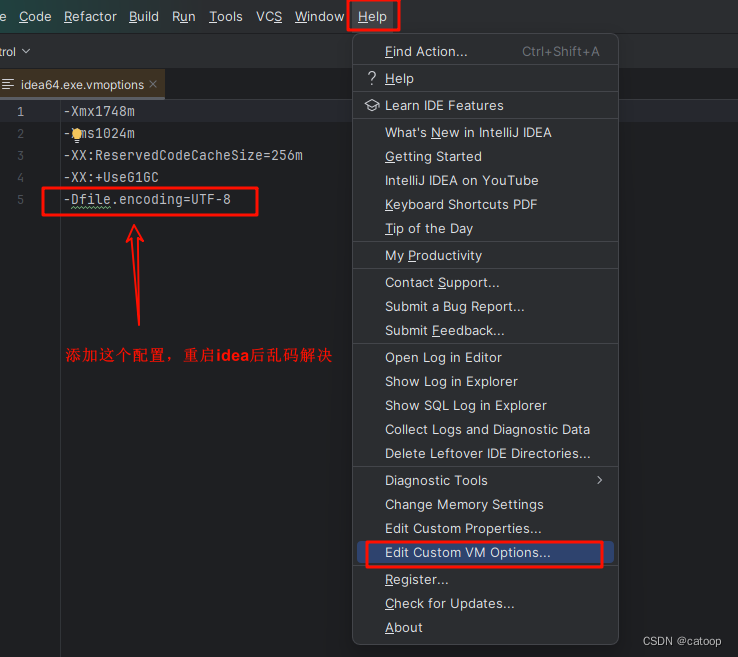
idea Gradle 控制台中文乱码
如下图所示,idea 中的 Gradle 控制台中文乱码: 解决方法,如下图所示: 注意:如果你的 idea 使用 crack 等方式破解了,那么你可能需要在文件 crack-2023\jetbra\vmoptions\idea.vmoptions 中进行配置…...

嵌入式学习day31 网络
网络: 数据传输,数据共享 1.网络协议模型: OSI协议模型 应用层 实际发送的数据 表示层 发送的数据是否加密 会话层 是否建立会话连接 传输层 数据传输的方式(数据报…...
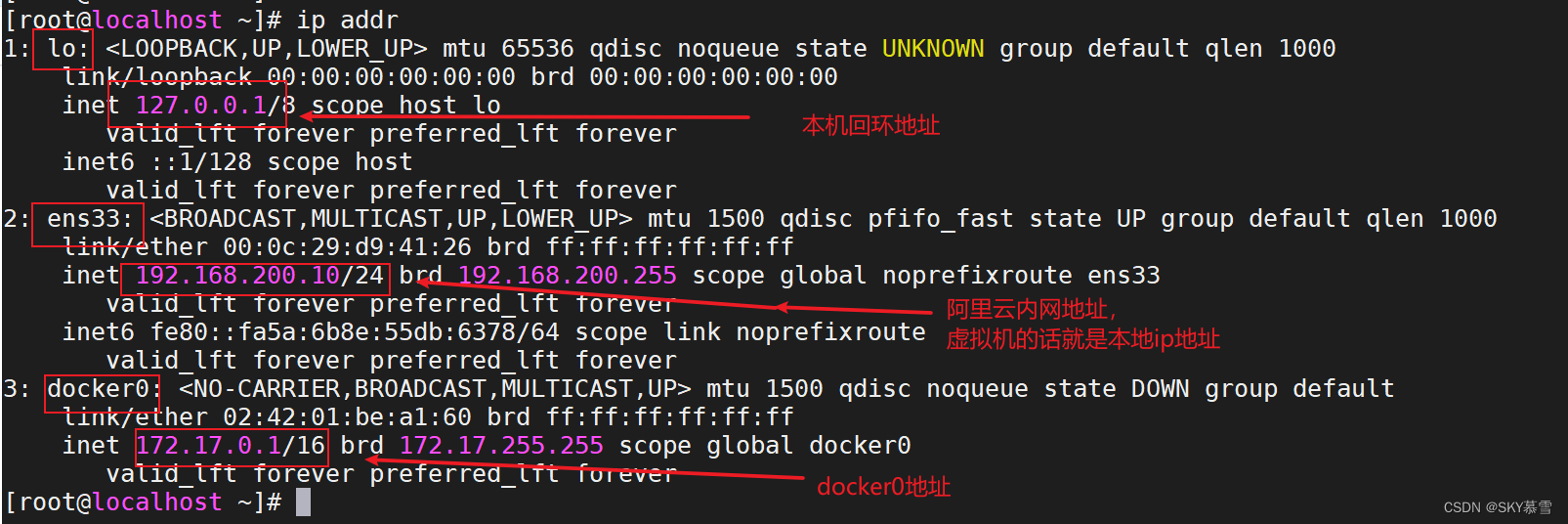
Docker网络+原理+link+自定义网络
目录 一、理解Docker网络 1.1 运行tomcat容器 1.2 查看容器内部网络地址 1.3 测试连通性 二、原理 2.1 查看网卡信息 2.2 再启动一个容器测试网卡 2.3 测试tomcat01 和tomcat02是否可以ping通 2.4 只要删除容器,对应网桥一对就没了 2.5 结论 三、--link 3.…...
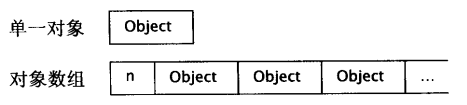
Effective C++ 学习笔记 条款16 成对使用new和delete时要采取相同形式
以下动作有什么错? std::string *stringArray new std::string[100]; // ... delete stringArray;每件事看起来都井然有序,使用了new,也搭配了对应的delete。但还是有某样东西完全错误:你的程序行为未定义。至少,str…...

PokéLLMon 源码解析(四)
.\PokeLLMon\poke_env\exceptions.py """ This module contains exceptions. """# 定义一个自定义异常类 ShowdownException,继承自内置异常类 Exception class ShowdownException(Exception):"""This exception is …...

区块链基础知识01
区块链:区块链技术是一种高级数据库机制,允许在企业网络中透明地共享信息。区块链数据库将数据存储在区块中,而数据库则一起链接到一个链条中。数据在时间上是一致的,在没有网络共识的情况下,不能删除或修改链条。 即&…...
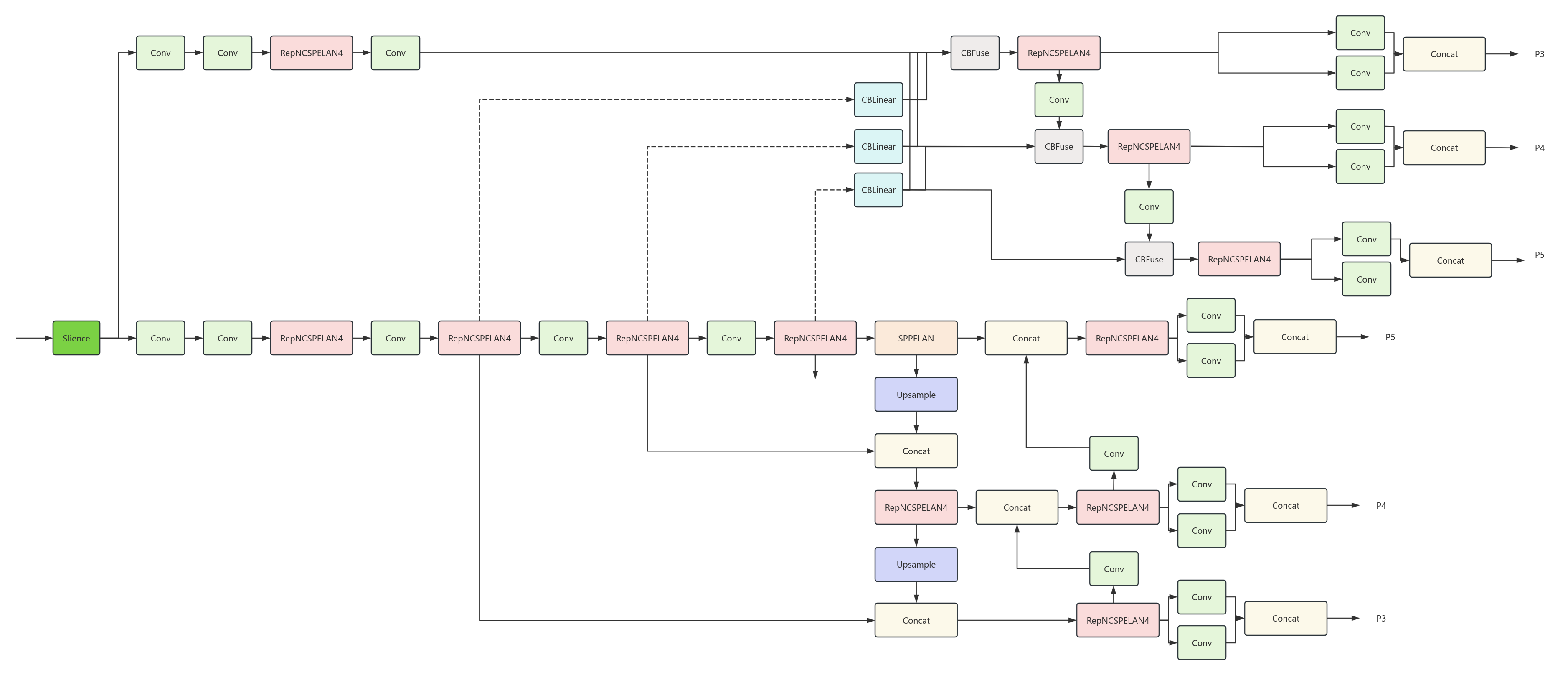
YOLOv9(2):YOLOv9网络结构
1. 前言 本文仅以官方提供的yolov9.yaml来进行简要讲解。 讲解之前,还是要做一些简单的铺垫。 Slice层不做任何的操作,纯粹是做一个占位层。这样一来,在parse_model时,ch[n]可表示第n层的输出通道。 Detect和DDetect主要区别还…...
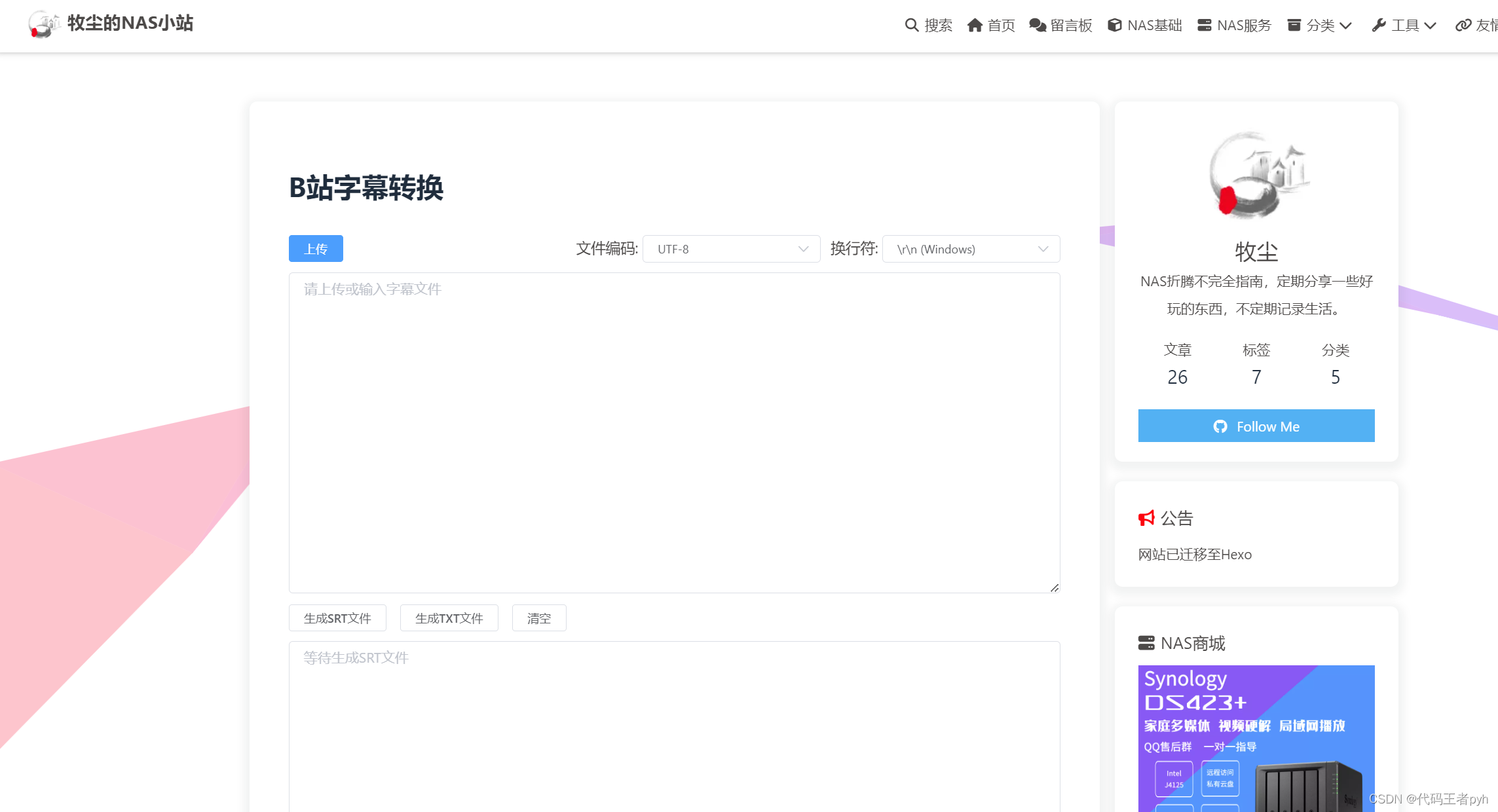
提取b站字幕(视频字幕、AI字幕)
提取b站字幕(视频字幕、AI字幕) 1. 打开视频 2. 按 F12 进行开发者界面 视频自己的紫米输入的是 json,如果是AI字幕则需要输入 ai_subtitle 3. 进入这个网址:https://www.dreamlyn.cn/bsrt...
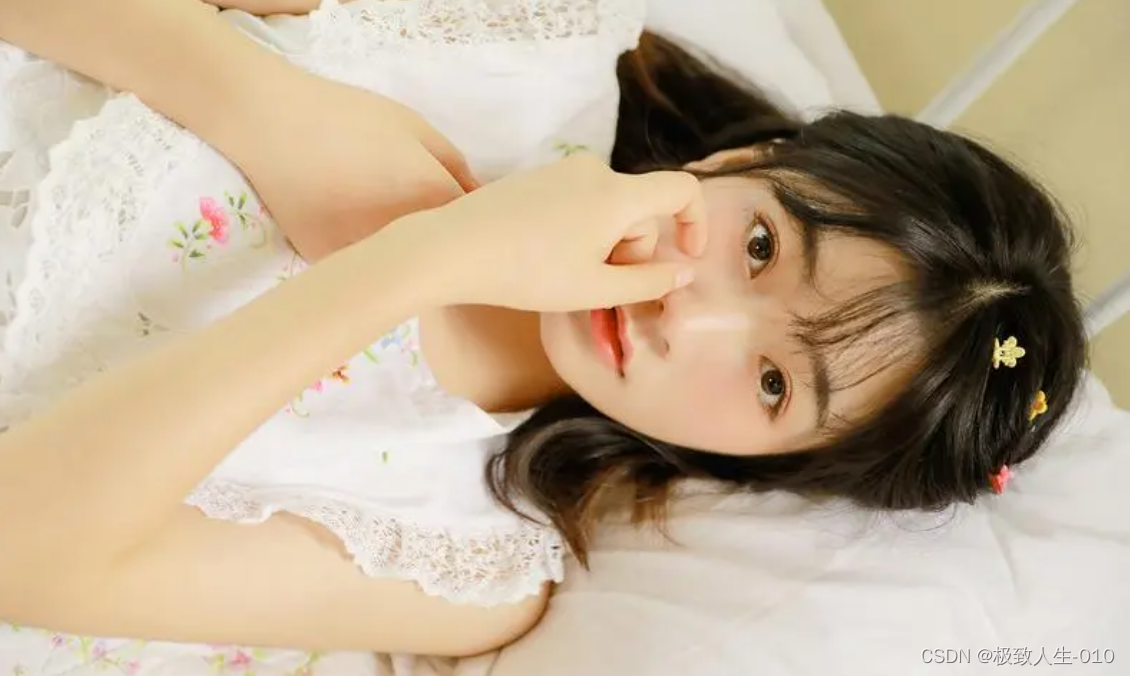
JAVA程序员如何快速熟悉新项目?
文章目录 Java程序员快速熟悉一个新项目的步骤通常包括以下几个方面:实例展示:Java程序员加入新项目时可能遇到的技术难题及其解决方案包括: Java程序员快速熟悉一个新项目的步骤通常包括以下几个方面: 理解项目背景和目标&#x…...
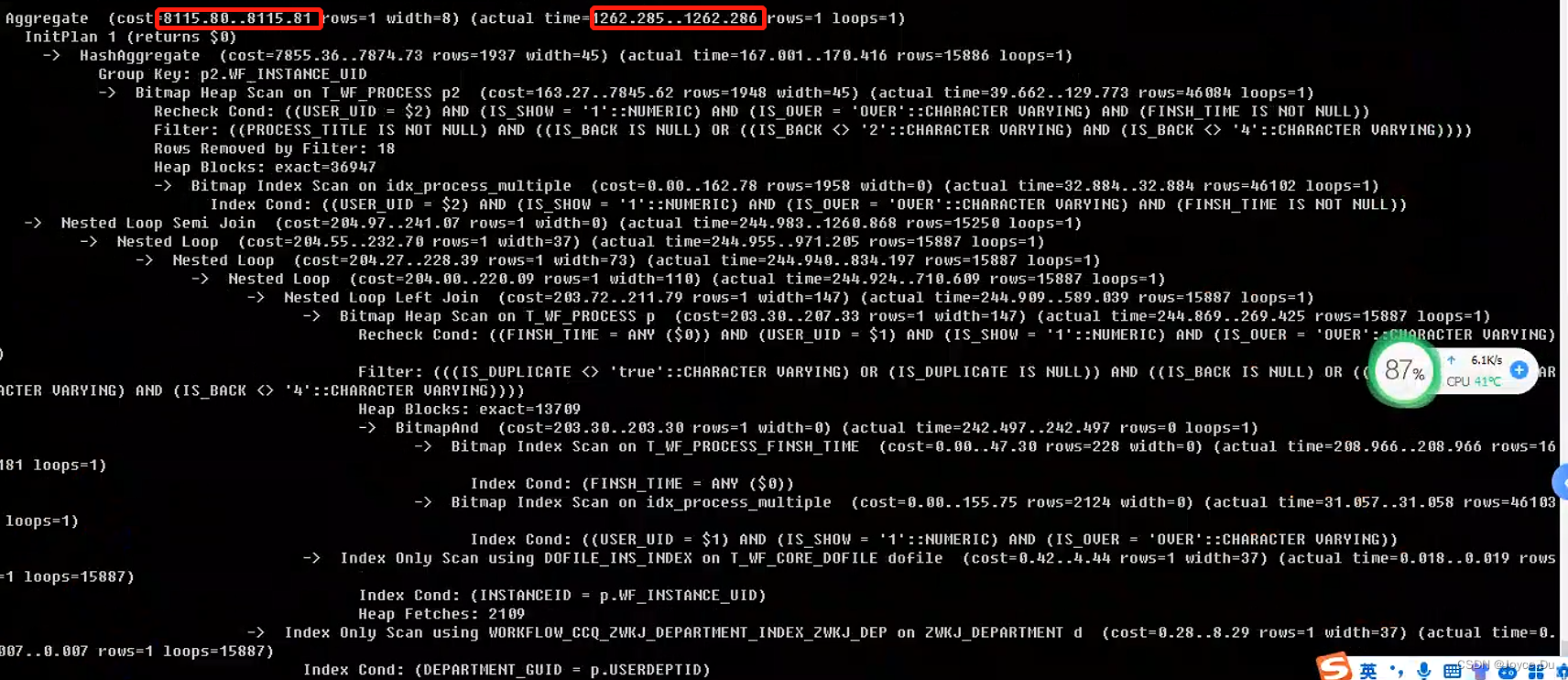
慢sql优化记录1
慢sql为: select count(*) from t_wf_process p left join t_wf_core_dofile dofile on p.wf_instance_uid dofile.instanceid join zwkj_department d on p.userdeptid d.department_guid ,t_wf_core_item i,wf_node n where (p.IS_DUPLICATE ! true or p.IS_DU…...
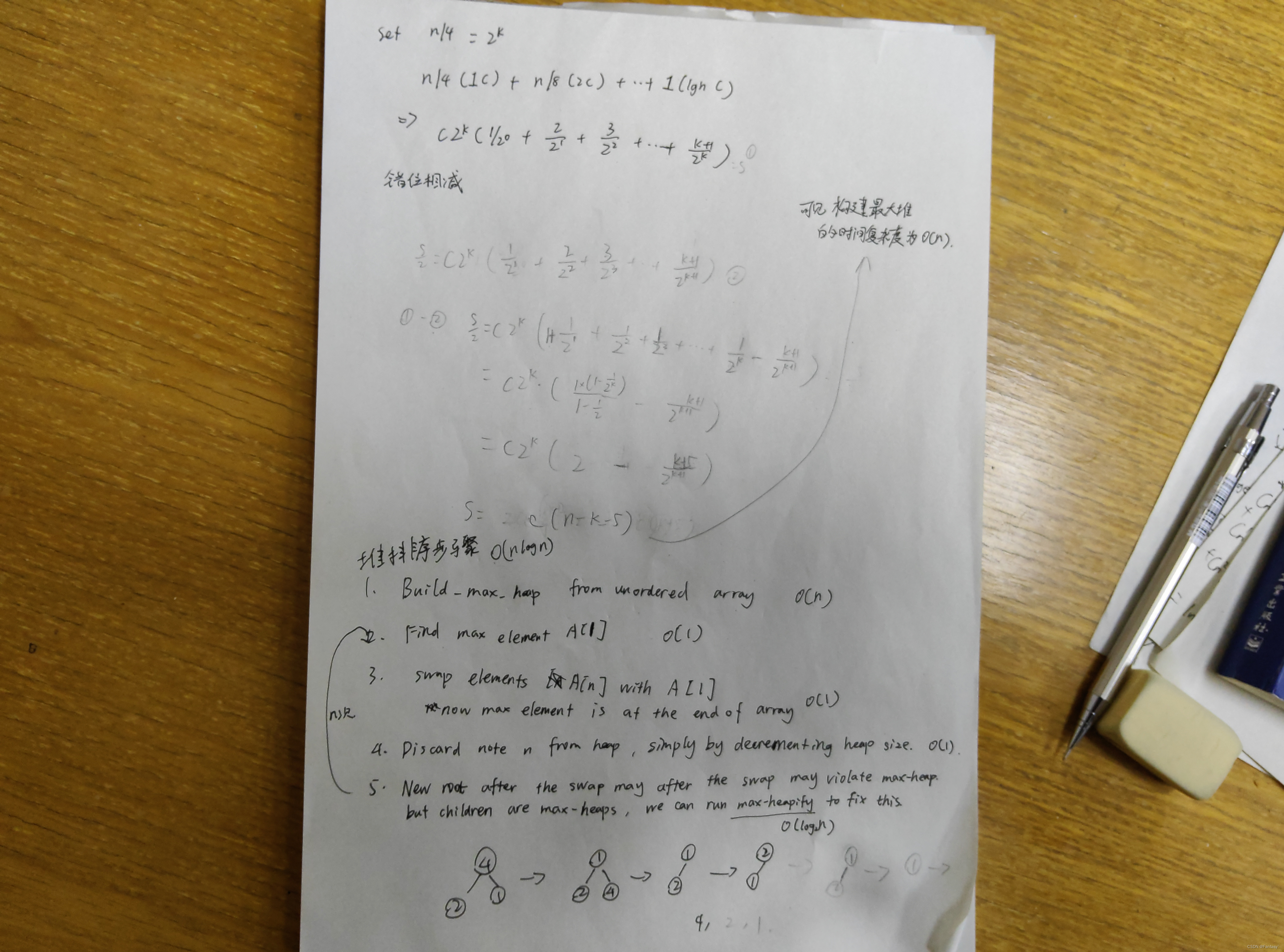
堆和堆排序
堆排序是一种与插入排序和并归排序十分不同的算法。 优先级队列 Priority Queue 优先级队列是类似于常规队列或堆栈数据结构的抽象数据类型(ADT)。优先级队列中的每个元素都有一个相关联的优先级key。在优先级队列中,高优先级的元素优先于…...
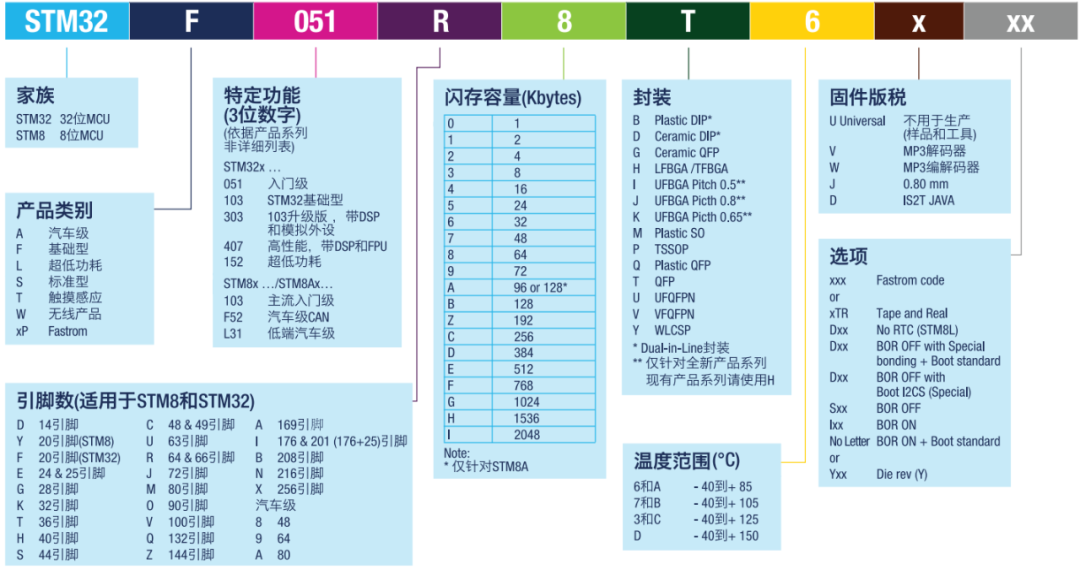
STM32 | 零基础 STM32 第一天
零基础 STM32 第一天 一、认知STM32 1、STM32概念 STM32:意法半导体基于ARM公司的Cortex-M内核开发的32位的高性能、低功耗单片机。 ST:意法半导体 M:基于ARM公司的Cortex-M内核的高性能、低功耗单片机 32:32位单片机 2、STM32开发的产品 STM32开发的产品&a…...
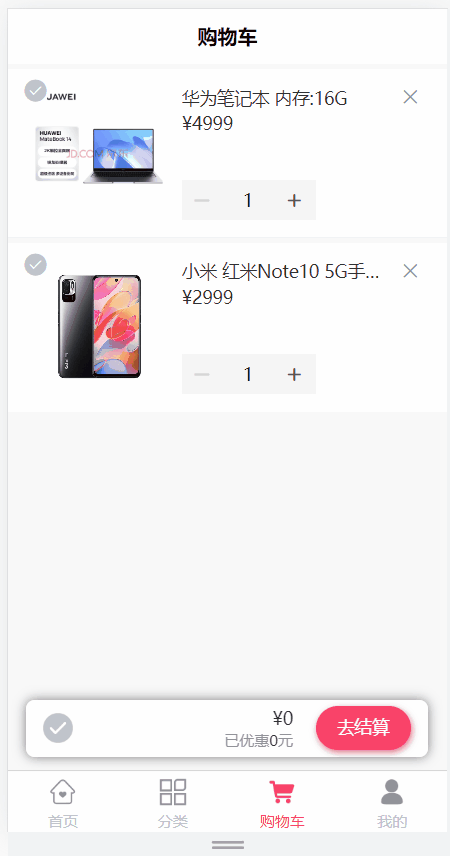
day16_购物车(添加购物车,购物车列表查询,删除购物车商品,更新选中商品状态,完成购物车商品的全选,清空购物车)
文章目录 购物车模块1 需求说明2 环境搭建3 添加购物车3.1 需求说明3.2 远程调用接口开发3.2.1 ProductController3.2.2 ProductService 3.3 openFeign接口定义3.3.1 环境搭建3.3.2 接口定义3.3.3 降级类定义 3.4 业务后端接口开发3.4.1 添加依赖3.4.2 修改启动类3.4.3 CartInf…...

基于Spring Boot的图书个性化推荐系统 ,计算机毕业设计(带源码+论文)
源码获取地址: 码呢-一个专注于技术分享的博客平台一个专注于技术分享的博客平台,大家以共同学习,乐于分享,拥抱开源的价值观进行学习交流http://www.xmbiao.cn/resource-details/1765769136268455938...
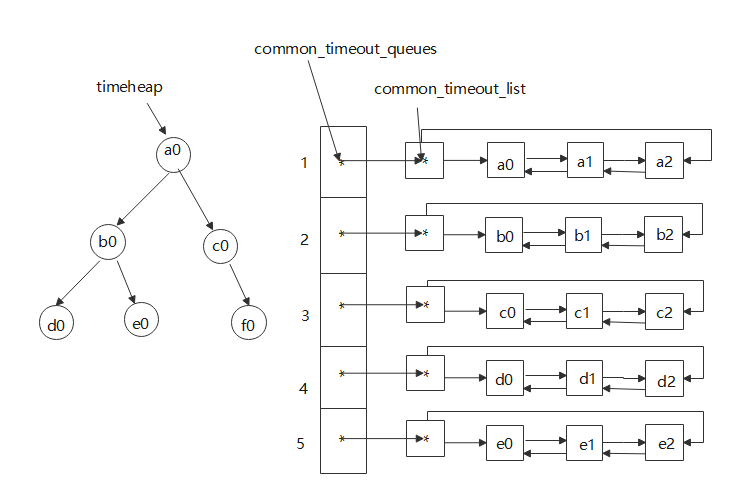
libevent源码解析:定时器事件(三)
文章目录 前言一、用例小根堆管理定时器事件小根堆和链表管理定时器事件区别 二、基本数据结构介绍结构体成员分析小根堆和链表common_timeout图示 三、源码分析小根堆管理定时器事件event_newevent_addevent_dispatch 链表common_timeout管理定时器事件event_base_init_common…...
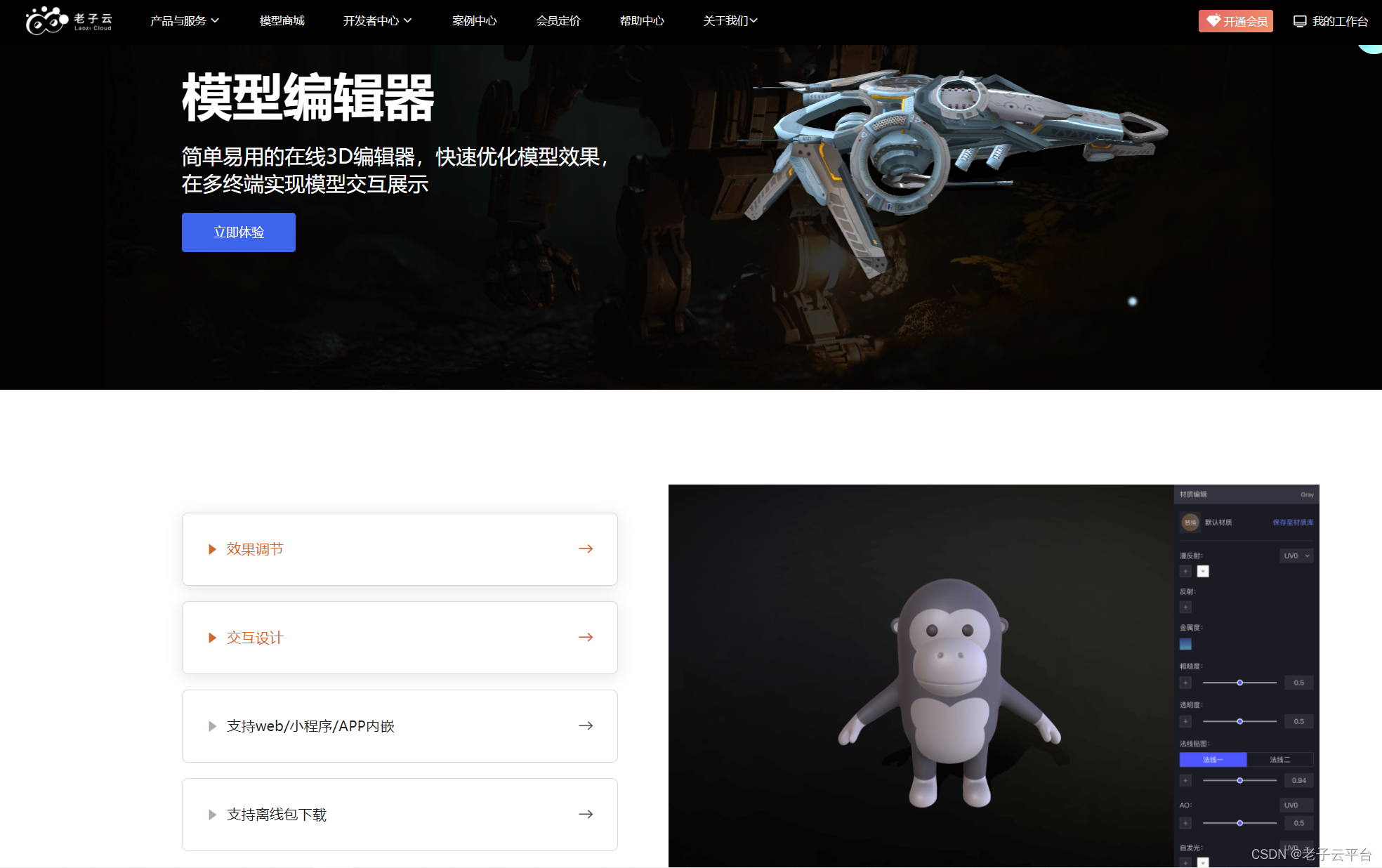
3D资产管理
3D 资产管理是指组织、跟踪、优化和分发 3D 模型和资产以用于游戏、电影、AR/VR 体验等各种应用的过程。 3D资产管理也称为3D内容管理。 随着游戏、电影、建筑、工程等行业中 3D 内容的增长,实施有效的资产管理工作流程对于提高生产力、减少错误、简化工作流程以及使…...
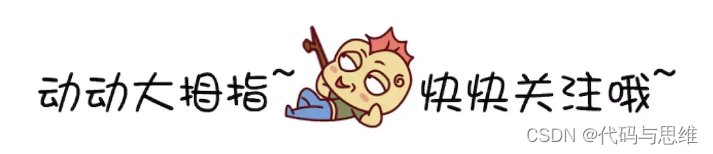
鸿蒙Harmony应用开发—ArkTS声明式开发(基础手势:Blank)
空白填充组件,在容器主轴方向上,空白填充组件具有自动填充容器空余部分的能力。仅当父组件为Row/Column/Flex时生效。 说明: 该组件从API Version 7开始支持。后续版本如有新增内容,则采用上角标单独标记该内容的起始版本。 子组件…...
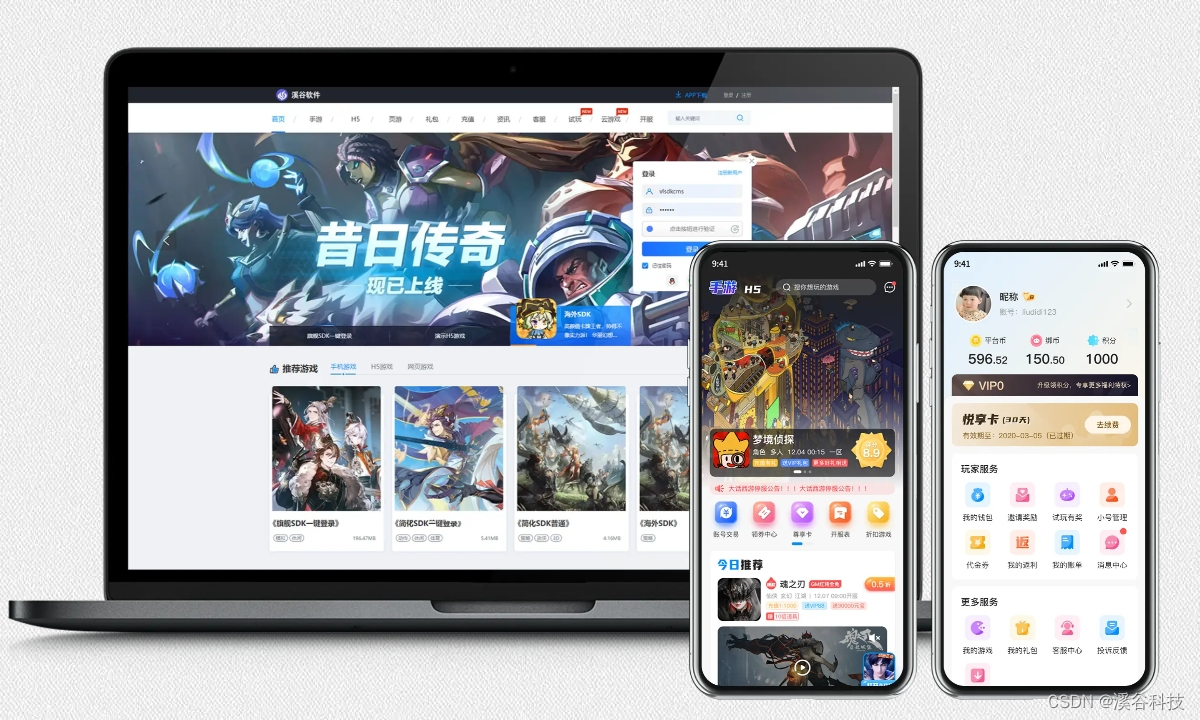
【手游联运平台搭建】游戏平台的作用
随着科技的不断发展,游戏行业也在不断壮大,而游戏平台作为连接玩家与游戏的桥梁,发挥着越来越重要的作用。游戏平台不仅为玩家提供了便捷的游戏体验,还为游戏开发者提供了广阔的市场和推广渠道。本文将从多个方面探讨游戏平台的作…...