QCC30XX如何查找本地地址码
查找本地地址段/**********************************************************************
Copyright (c) 2016 - 2017 Qualcomm Technologies International, Ltd.
FILE NAME
sink_private_data.c
DESCRIPTION
This module works as a container for all private and common data that is used across sink
qpplication. It provides API to access the data from outside. It interacts with configuration
entities to read/write configuration data. More over this module contains module specific
run time data (as a common runtime data for entire sink application), and those data also
exposed using defined API's
NOTES
Module does not have any intelligence to manipulate the data, or verify the data or its
contents ,it is the user to decide hoe to use this data and when to update the data.
*/
#include <stdlib.h>
#include <ps.h>
#include <vmtypes.h>
#include <bdaddr.h>
#include <byte_utils.h>
#include "sink_private_data.h"
#include "sink_dut.h"
#include "sink_malloc_debug.h"
#include "sink_configmanager.h"
#include <local_device.h>
#ifdef DEBUG_SINK_PRIVATE_DATA
#define SINK_DATA_DEBUG(x) DEBUG(x)
#define SINK_DATA_ERROR(x) TOLERATED_ERROR(x)
#else
#define SINK_DATA_DEBUG(x)
#define SINK_DATA_ERROR(x)
#endif
#ifdef DEBUG_MAIN
#define MAIN_DEBUG(x) DEBUG(x)
#else
#define MAIN_DEBUG(x)
#endif
/* Referance to Global Data for sink private module */
typedef struct __sinkdata_globaldata_t
{
unsigned panic_reconnect:1; /* Are we using panic action? Bit to inidcate panic reconnection action is used */
unsigned paging_in_progress:1; /* Bit to indicate that device is curretly paging whilst in connectable state*/
unsigned powerup_no_connection:1; /* Bit to indicate device has powered and no connections yet */
unsigned confirmation:1; /* Bit to indicate user auth confirmation status */
unsigned SinkInitialising:1; /* Bit to indicate sink is in initialising state */
unsigned PowerOffIsEnabled:1; /* Bit to indicate power off is enabled */
unsigned debug_keys_enabled:1; /* Bit to indicate debug keys enabled */
unsigned stream_protection_state:2; /* Holds stream protection state */
unsigned MultipointEnable:1; /* Bit to indicate multipoint enabled */
unsigned _spare1_:6;
unsigned gEventQueuedOnConnection:8; /* variable to hold evet queued while in connection */
unsigned dfu_access:1; /* Link Policy expedites DFU data transfer */
unsigned display_link_keys:1; /* Bit used to indicate if link keys should be displayed */
unsigned _spare2_:6;
uint16 NoOfReconnectionAttempts; /* Holdes current number of reconnection attempts */
uint16 connection_in_progress; /* flag used to block role switch requests until all connections are complete or abandoned */
#ifdef ENABLE_SQIFVP
unsigned partitions_mounted:8;
unsigned unused:8;
#endif
bdaddr *linkloss_bd_addr; /** bdaddr of a2dp device that had the last link loss. */
tp_bdaddr *confirmation_addr;
bdaddr local_bd_addr; /* Local BD Address of the sink device available in ps */
power_table *user_power_table; /* pointer to user power table if available in ps */
}sinkdata_globaldata_t;
/* PSKEY for BD ADDRESS */
#define PSKEY_BDADDR 0x0001
#define LAP_MSW_OFFSET 0
#define LAP_LSW_OFFSET 1
#define UAP_OFFSET 2
#define NAP_OFFSET 3
/* Global data strcuture element for sink private data */
static sinkdata_globaldata_t gSinkData;
#define GSINKDATA gSinkData
/**********************************************************************
*************** External Interface Function Implemetations **********************
***********************************************************************/
/**********************************************************************
Interfaces for accessing Configurable Items
*/
/**********************************************************************
Interfaces for Initializing Local Address, which read the local address.
*/
bool sinkDataInitLocalBdAddrFromPs(void)
{
bool result = FALSE;
uint16 size = PS_SIZE_ADJ(sizeof(GSINKDATA.local_bd_addr));
uint16* bd_addr_data = (uint16*)PanicUnlessNew(bdaddr);
BdaddrSetZero(&GSINKDATA.local_bd_addr);
if(size == PsFullRetrieve(PSKEY_BDADDR, bd_addr_data, size))
{
GSINKDATA.local_bd_addr.nap = bd_addr_data[NAP_OFFSET];
GSINKDATA.local_bd_addr.uap = bd_addr_data[UAP_OFFSET];
GSINKDATA.local_bd_addr.lap = MAKELONG(bd_addr_data[LAP_LSW_OFFSET], bd_addr_data[LAP_MSW_OFFSET]);
SINK_DATA_DEBUG(("CONF: PSKEY_BDADDR [%04x %02x %06lx]\n",
GSINKDATA.local_bd_addr.nap, GSINKDATA.local_bd_addr.uap, GSINKDATA.local_bd_addr.lap));
result = TRUE;
}
else
{
GSINKDATA.local_bd_addr = LocalDeviceGetBdAddr();
}
MAIN_DEBUG(("CONF: PSKEY_BDADDR [%04x %02x %06lx]\n",
GSINKDATA.local_bd_addr.nap, GSINKDATA.local_bd_addr.uap, GSINKDATA.local_bd_addr.lap));
free(bd_addr_data);
return result;
}
/**********************************************************************
Interfaces for checking reconnect on panic configuration is enabled or not
*/
bool sinkDataIsReconnectOnPanic(void)
{
bool reconnect_on_panic = FALSE;
sinkdata_readonly_config_def_t *read_configdata = NULL;
SINK_DATA_DEBUG(("SinkData:sinkDataIsReconnectOnPanic()\n"));
if (configManagerGetReadOnlyConfig(SINKDATA_READONLY_CONFIG_BLK_ID, (const void **)&read_configdata))
{
reconnect_on_panic = read_configdata->ReconnectOnPanic;
configManagerReleaseConfig(SINKDATA_READONLY_CONFIG_BLK_ID);
}
SINK_DATA_DEBUG(("SinkData:reconnect_on_panic = %d \n",reconnect_on_panic));
return (reconnect_on_panic)?TRUE : FALSE;
}
/**********************************************************************
Interfaces for checking power of after PDL reset configuration is enabled or not
*/
bool sinkDataIsPowerOffAfterPDLReset(void)
{
bool poweroff_pdl_reset = FALSE;
sinkdata_readonly_config_def_t *read_configdata = NULL;
SINK_DATA_DEBUG(("SinkData:sinkDataIsPowerOffAfterPDLReset()\n"));
if (configManagerGetReadOnlyConfig(SINKDATA_READONLY_CONFIG_BLK_ID, (const void **)&read_configdata))
{
poweroff_pdl_reset = read_configdata->PowerOffAfterPDLReset;
configManagerReleaseConfig(SINKDATA_READONLY_CONFIG_BLK_ID);
}
SINK_DATA_DEBUG(("SinkData: poweroff_pdl_reset = %d \n",poweroff_pdl_reset));
return (poweroff_pdl_reset)?TRUE : FALSE;
}
/**********************************************************************
Interfaces for checking does sink shoule be in discoverable mode all time
configuration is enabled or not
*/
bool sinkDataIsDiscoverableAtAllTimes(void)
{
bool discoverable_alltime = FALSE;
sinkdata_readonly_config_def_t *read_configdata = NULL;
SINK_DATA_DEBUG(("SinkData:sinkDataIsDiscoverableAtAllTimes()\n"));
if (configManagerGetReadOnlyConfig(SINKDATA_READONLY_CONFIG_BLK_ID, (const void **)&read_configdata))
{
discoverable_alltime = read_configdata->RemainDiscoverableAtAllTimes;
configManagerReleaseConfig(SINKDATA_READONLY_CONFIG_BLK_ID);
}
SINK_DATA_DEBUG(("SinkData: discoverable_alltime = %d\n",discoverable_alltime));
return (discoverable_alltime)?TRUE : FALSE;
}
/**********************************************************************
Interfaces for checking DisablePowerOffAfterPowerOn configuration is enabled or not
*/
bool sinkDataCheckDisablePowerOffAfterPowerOn(void)
{
bool disable_poweroff = FALSE;
sinkdata_readonly_config_def_t *read_configdata = NULL;
SINK_DATA_DEBUG(("SinkData:sinkDataCheckDisablePowerOffAfterPowerOn()\n"));
if (configManagerGetReadOnlyConfig(SINKDATA_READONLY_CONFIG_BLK_ID, (const void **)&read_configdata))
{
disable_poweroff = read_configdata->DisablePowerOffAfterPowerOn;
configManagerReleaseConfig(SINKDATA_READONLY_CONFIG_BLK_ID);
}
SINK_DATA_DEBUG(("SinkData: disable_poweroff = %d\n",disable_poweroff));
return (disable_poweroff)?TRUE : FALSE;
}
/**********************************************************************
Interfaces for checking pairing mode on connection failure configuration is enabled or not
*/
bool sinkDataEntrePairingModeOnConFailure(void)
{
bool failuretoconnect = FALSE;
sinkdata_readonly_config_def_t *read_configdata = NULL;
SINK_DATA_DEBUG(("SinkData:sinkDataPairingModeOnConnectionFailure()\n"));
if (configManagerGetReadOnlyConfig(SINKDATA_READONLY_CONFIG_BLK_ID, (const void **)&read_configdata))
{
failuretoconnect = read_configdata->EnterPairingModeOnFailureToConn;
configManagerReleaseConfig(SINKDATA_READONLY_CONFIG_BLK_ID);
}
SINK_DATA_DEBUG(("SinkData: failuretoconnect = %d\n",failuretoconnect));
return (failuretoconnect)?TRUE : FALSE;
}
/**********************************************************************
Interfaces for checking Power Off OnlyIf VReg Enble is low configuration is enabled or not
*/
bool sinkDataIsPowerOffOnlyIfVRegEnlow(void)
{
bool vreg_enlow = FALSE;
sinkdata_readonly_config_def_t *read_configdata = NULL;
SINK_DATA_DEBUG(("SinkData:sinkDataIsPowerOffOnlyIfVRegEnlow()\n"));
if (configManagerGetReadOnlyConfig(SINKDATA_READONLY_CONFIG_BLK_ID, (const void **)&read_configdata))
{
vreg_enlow = read_configdata->PowerOffOnlyIfVRegEnLow;
configManagerReleaseConfig(SINKDATA_READONLY_CONFIG_BLK_ID);
}
SINK_DATA_DEBUG(("SinkData: vreg_enlow = %d\n",vreg_enlow));
return (vreg_enlow)?TRUE : FALSE;
}
/**********************************************************************/
bool sinkDataAllowAutomaticPowerOffWhenCharging(void)
{
bool power_off_when_charging = FALSE;
sinkdata_readonly_config_def_t *read_configdata = NULL;
SINK_DATA_DEBUG(("SinkData:sinkDataAllowAutomaticPowerOffWhenCharging()\n"));
if (configManagerGetReadOnlyConfig(SINKDATA_READONLY_CONFIG_BLK_ID, (const void **)&read_configdata))
{
power_off_when_charging = read_configdata->AllowAutomaticPowerOffWhenCharging;
configManagerReleaseC
相关文章:

QCC30XX如何查找本地地址码
查找本地地址段/********************************************************************** Copyright (c) 2016 - 2017 Qualcomm Technologies International, Ltd. FILE NAME sink_private_data.c DESCRIPTION This module works as a container for all private and common…...
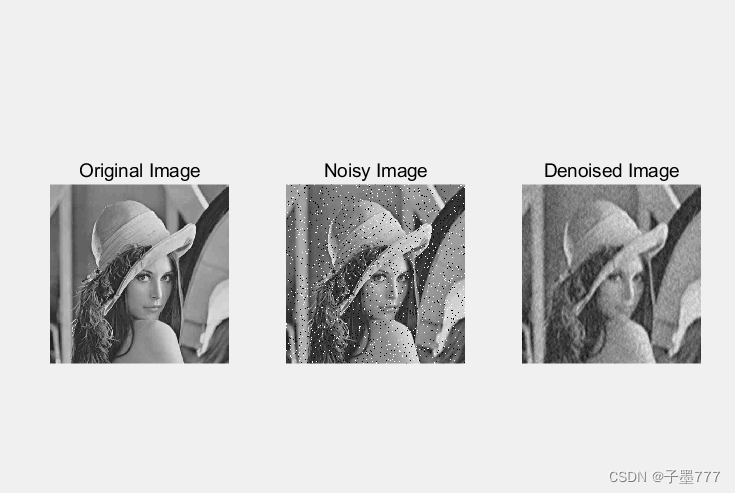
基于 DCT 的图像滤波
需求分析 对于图像去噪这一需求,我们可以通过DCT(离散余弦变换)算法来实现。DCT是一种基于频域的变换技术,可以将图像从空间域转换为频域,然后通过滤波等处理方式进行去噪。 针对这一需求,我们需要进行以下…...

spdlog日志库源码:自定义异常类spdlog_ex
自定义异常类spdlog_ex 标准库异常类(std::exception)系列,能满足大多数使用异常的场景,但对系统调用异常及错误信息缺乏支持。spdlog通过继承std::exception,扩展对系统调用的支持,实现自定义异常类spdlo…...

3.每日LeetCode-数组类,爬楼梯(Go,Java,Python)
目录 题目 解法 Go Java Python 代码地址:leetcode: 每日leetcode刷题 题目 题号70. 爬楼梯 假设你正在爬楼梯。需要 n 阶你才能到达楼顶。 每次你可以爬 1 或 2 个台阶。你有多少种不同的方法可以爬到楼顶呢? 示例 1: 输入ÿ…...

单节点11.2.0.3参数文件恢复到RAC11.2.0.4启动失败
问题描述 通过pfile生成spfile失败,提示DATA磁盘不存在 SQL> create spfileDATA/DXJ/spfiledxj.ora from pfile/home/oracle/initdxj20240529.ora; create spfileDATA/DXJ/spfiledxj.ora from pfile/home/oracle/initdxj20240529.ora * ERROR at line 1: ORA-1…...
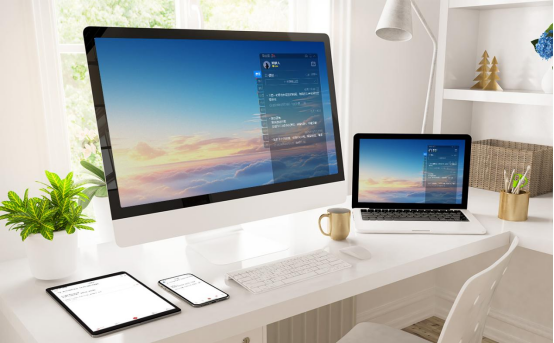
Windows电脑高颜值桌面便利贴,便签怎么设置
在这个看颜值的时代,我们不仅在衣着打扮上追求时尚与美观,就连电脑桌面也不愿放过。一张唯美的壁纸,几款别致的小工具,总能让我们的工作空间焕发出不一样的光彩。如果你也热衷于打造高颜值的电脑桌面,那么,…...

代码随想录35期Day54-Java
Day54题目 LeetCode392判断子序列 核心思想:公共子序列长度达到需要判断的字符串的长度,说明是子序列 class Solution {public boolean isSubsequence(String s, String t) {if("".equals(s)) return true;int[][] dp new int[s.length()1][t.length()1];for(int…...

Ubuntu使用sudo命令
在Ubuntu系统中,使用管理员权限通常涉及到使用sudo命令。这是因为Ubuntu默认情况下不直接允许root用户登录,而是通过sudo命令来执行需要管理员权限的任务。以下是一些常见的使用管理员权限的方法: 1. 使用sudo命令 运行单个命令 如果只需要…...
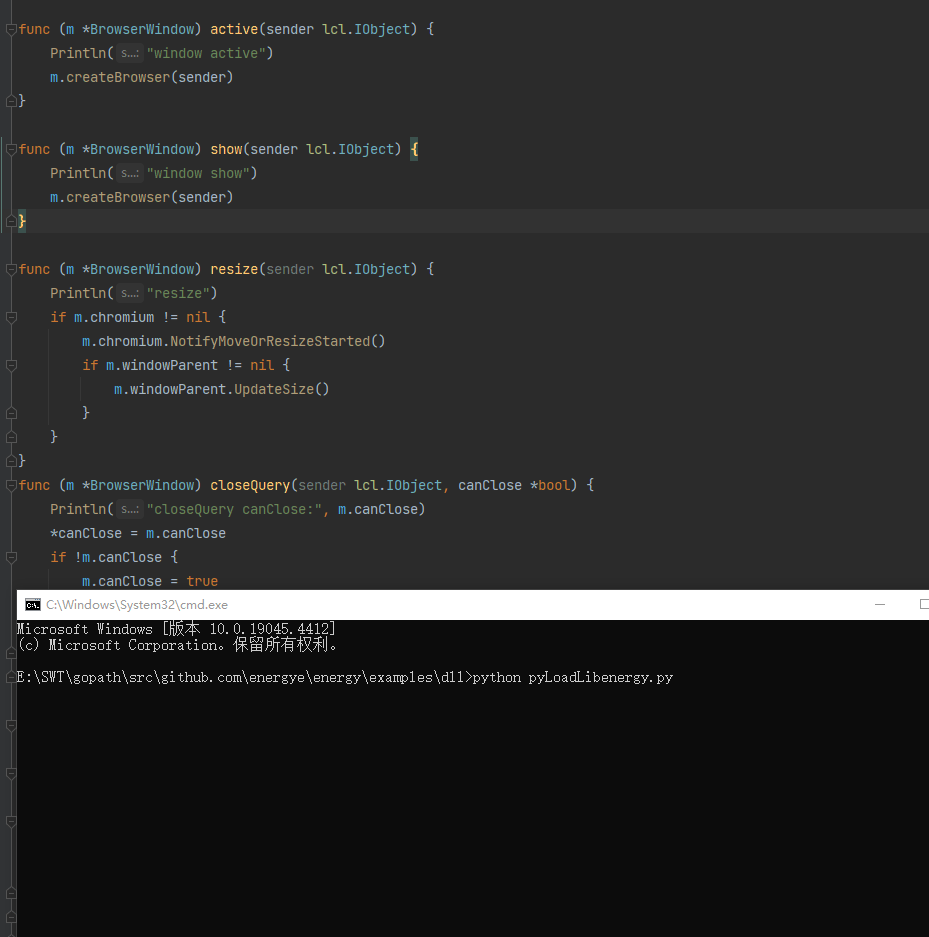
三方语言中调用, Go Energy GUI编译的dll动态链接库CEF
如何在其它编程语言中调用energy编译的dll动态链接库,以使用CEF 或 LCL库 Energy是Go语言基于LCL CEF开发的跨平台GUI框架, 具有很容易使用CEF 和 LCL控件库 interface 便利 示例链接 正文 为方便起见使用 python 调用 go energy 编译的dll 准备 系统&#x…...
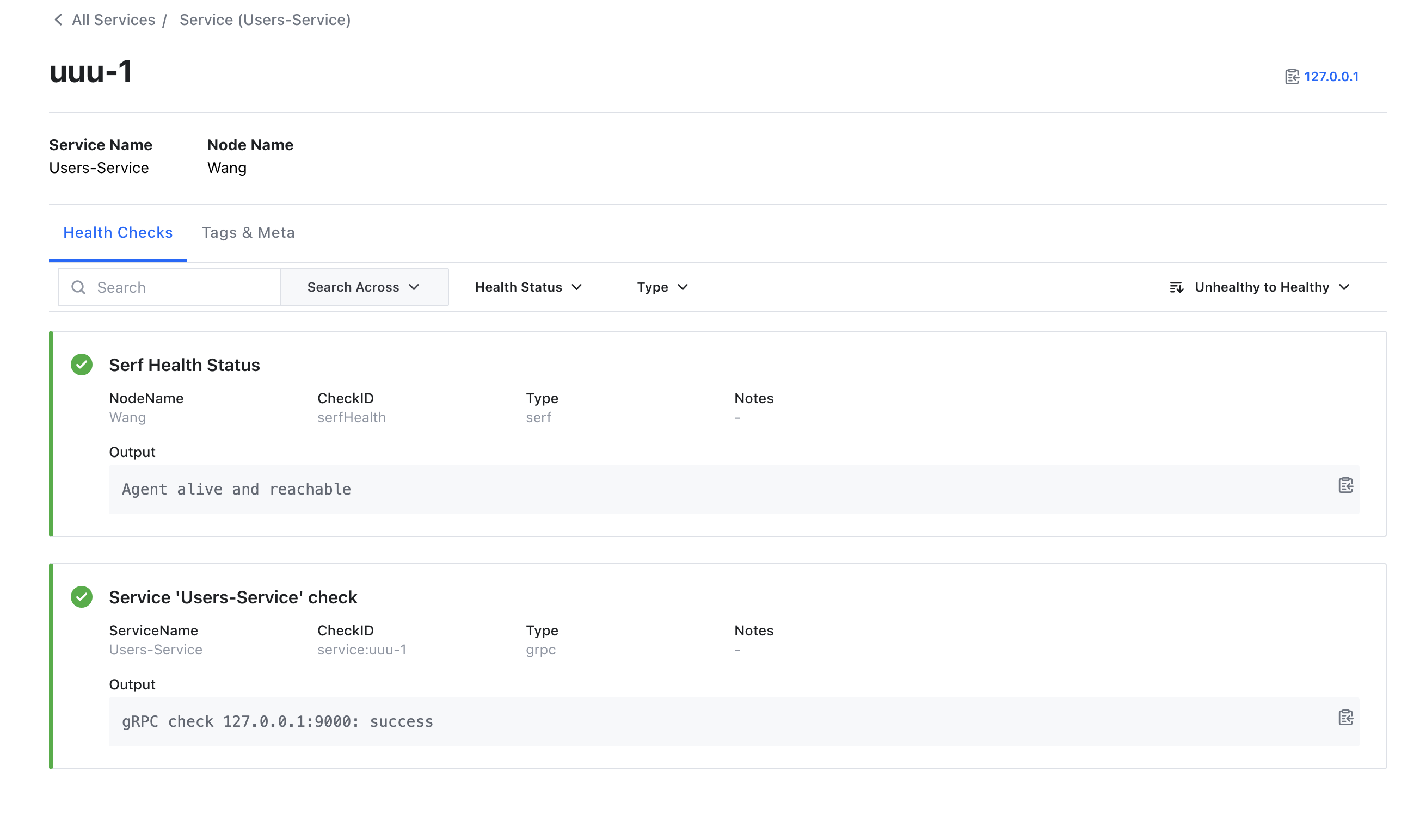
Go微服务: Grpc服务注册在Consul的示例(非Go-Micro)
概述 现在,我们使用consul客户端的api来把GRPC服务实现注册到consul上,非Go-Micro的形式其实,consul官方提供了对应的接口调用来实现,golang中的consul/api包对其进行了封装我们使用consul/api来进行展示 目录结构 gitee.com/g…...
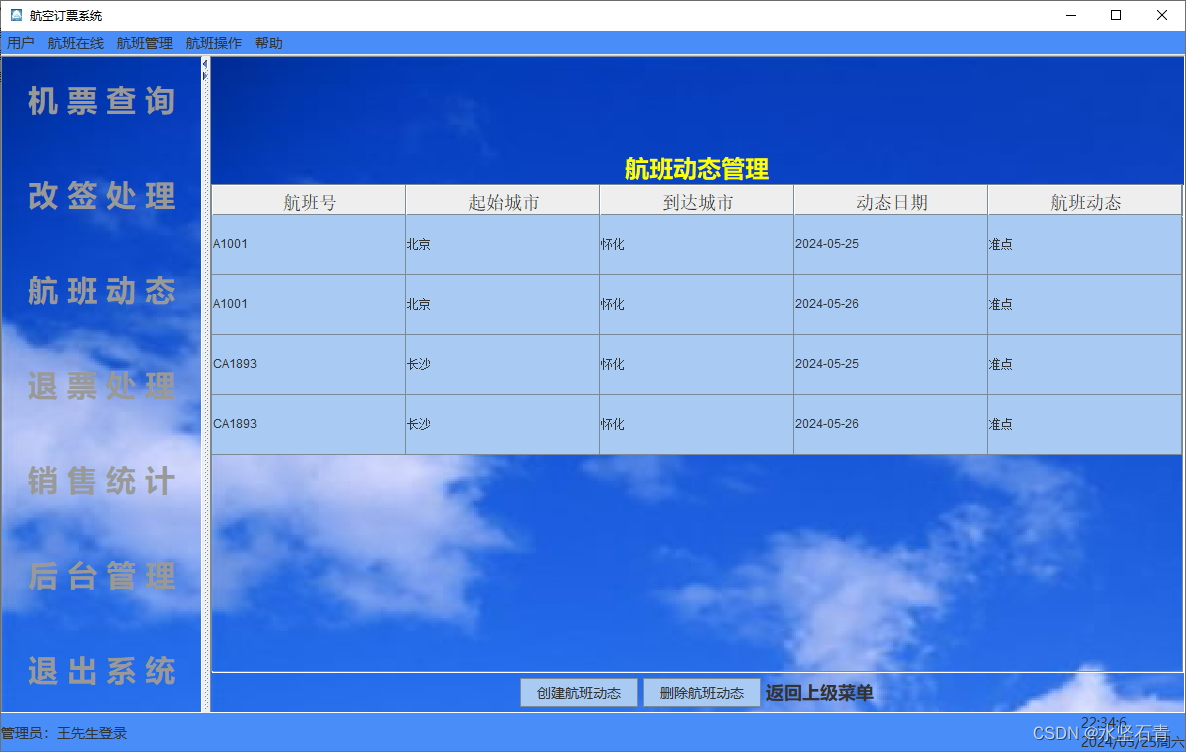
Java+Swing+Mysql实现飞机订票系统
一、系统介绍 1.开发环境 操作系统:Win10 开发工具 :Eclipse2021 JDK版本:jdk1.8 数据库:Mysql8.0 2.技术选型 JavaSwingMysql 3.功能模块 4.数据库设计 1.用户表(users) 字段名称 类型 记录内容…...

2024 rk
1.mysql、redis分布式锁 case: 商品秒杀 1)使用 MySQL 作为分布式锁来实现商品秒杀功能可能存在以下几个缺点; 使用 MySQL 作为分布式锁来实现商品秒杀功能可能存在以下几个缺点: 单点故障:如果使用单个 MySQL 实例作为分布式锁的存储介质…...

Java实现多张图片合并保存到pdf中
Java实现多张图片合并保存到pdf中 1、依赖–maven <dependency><groupId>org.apache.pdfbox</groupId><artifactId>pdfbox</artifactId><version>2.0.24</version></dependency>2、上代码 package com.hxlinks.hxiot.contro…...
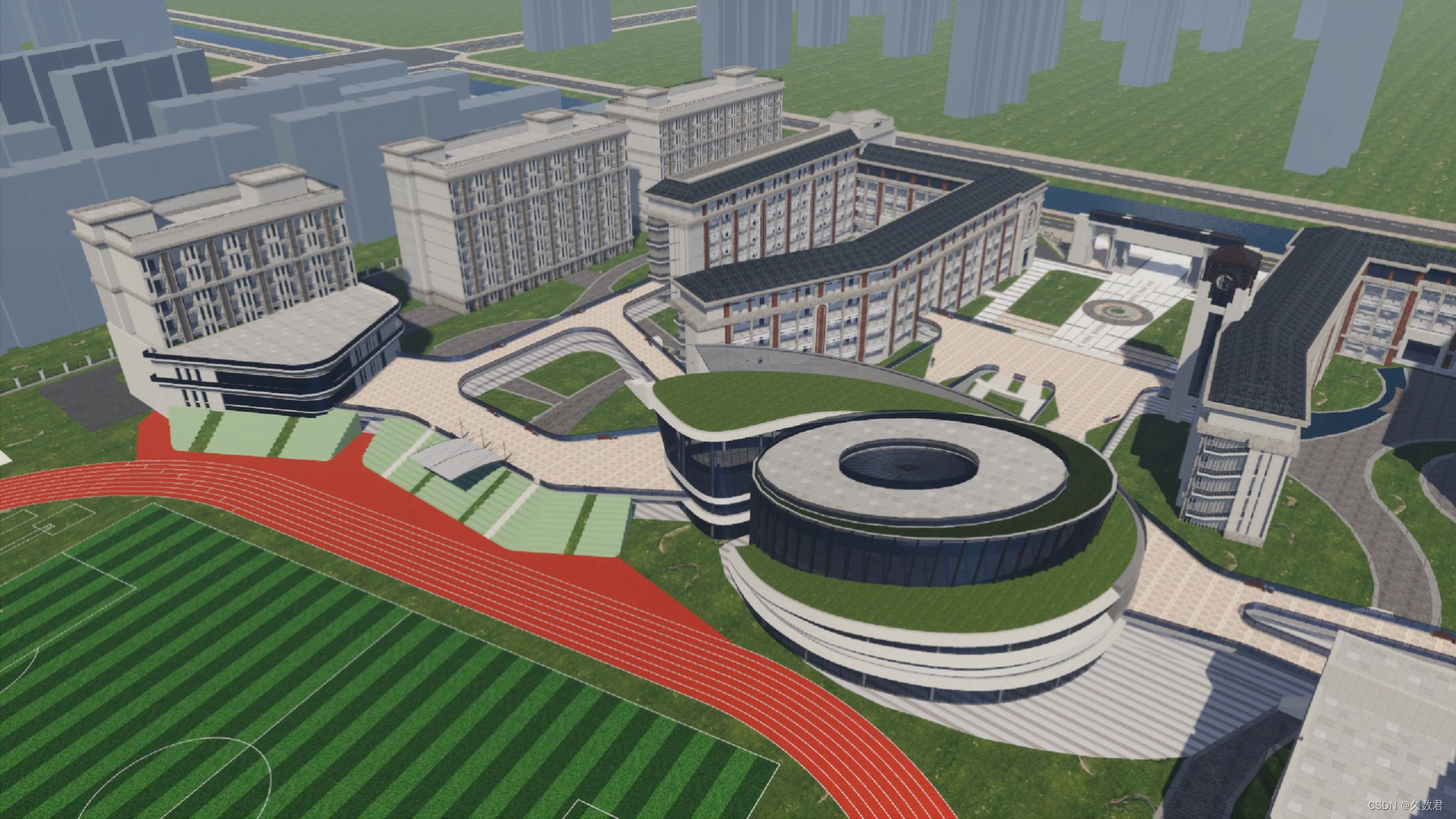
揭秘智慧校园:可视化技术引领教育新篇章
随着科技的飞速发展,我们的生活方式正在经历一场前所未有的变革。而在这场变革中,学校作为培养未来人才的重要基地,也在不断地探索与创新。 一、什么是校园可视化? 校园可视化,就是通过先进的信息技术,将学…...
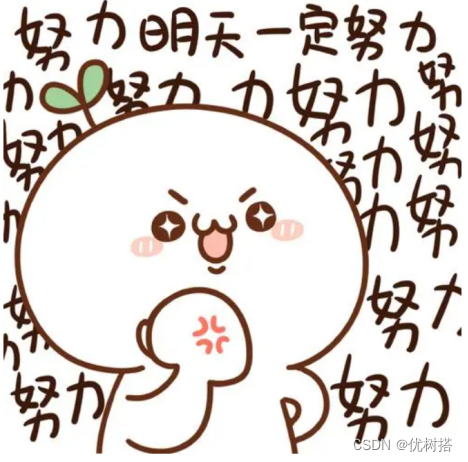
基础9 探索图形化编程的奥秘:从物联网到工业自动化
办公室内,明媚的阳光透过窗户洒落,为每张办公桌披上了一层金色的光辉。同事们各自忙碌着,键盘敲击声、文件翻页声和低声讨论交织在一起,营造出一种忙碌而有序的氛围。空气中氤氲着淡淡的咖啡香气和纸张的清新味道,令人…...

RPC-----RCF
RPC RPC(Remote Procedure Call Protocol)——远程过程调用协议。 RCF...

StarRocks中,这些配置项是表属性的一部分
CREATE TABLE warehouse.ads_order_all_df ( so_id varchar(200) NULL COMMENT "销售订单主表标识", so_code varchar(200) NULL COMMENT "销售订单主表表号" ) ENGINEOLAP DUPLICATE KEY(so_id) COMMENT "OLAP" DISTRIBUTED BY HASH(dt) …...

Activity->Activity生命周期
<四大组件 android:name"xxx"android:exported"true" // 该组边能够被其他组件启动android:enabled"true" // 该组件能工与用户交互 </四大组件>Activity常用生命周期 启动Activity 2024-05-29 03:53:57.401 21372-21372 yang …...
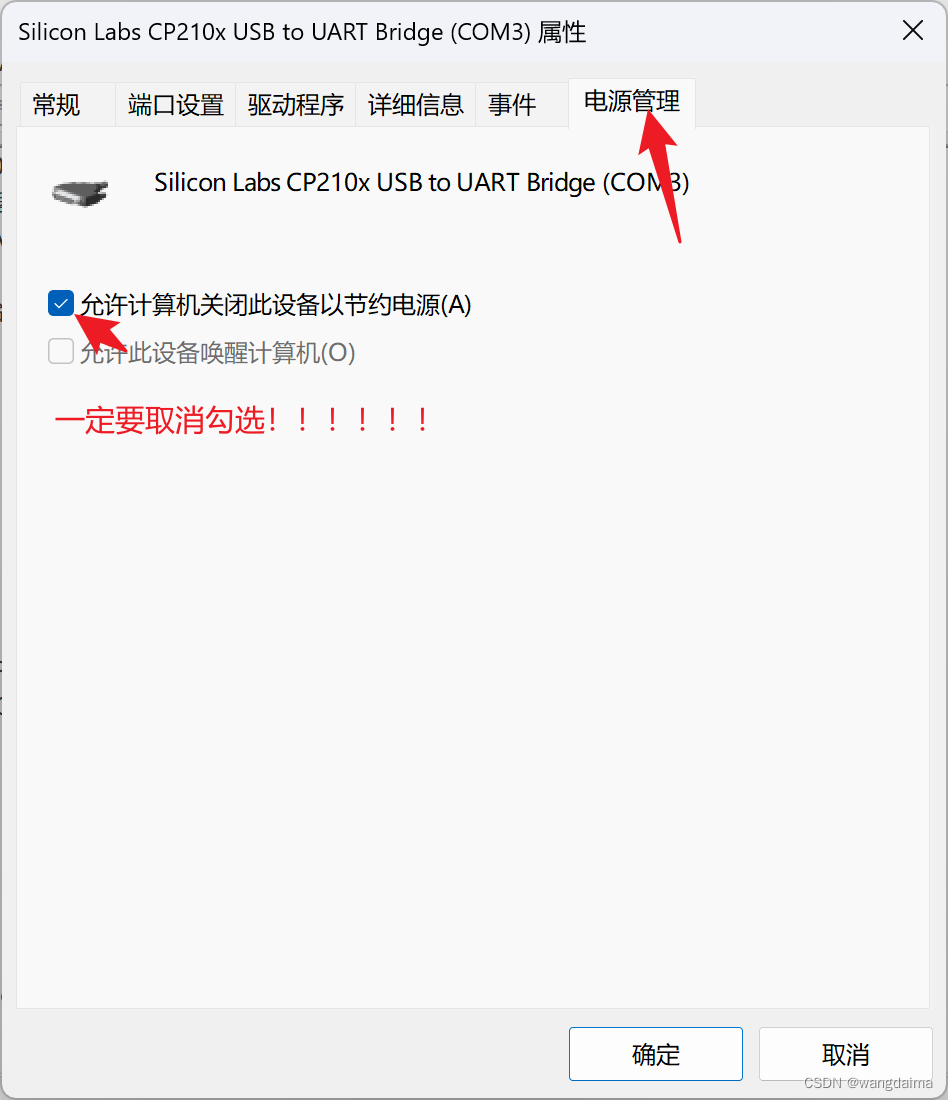
乐鑫ESP串口驱动安装,安装cp210x驱动
windows11安装cp210x驱动: 1:第一步官网下载驱动: 官网地址如下: CP210x USB to UART Bridge VCP Drivers - Silicon Labs 第二步:解压文件夹并安装如图所示: 3:第三步安装成功后会给你个提示…...
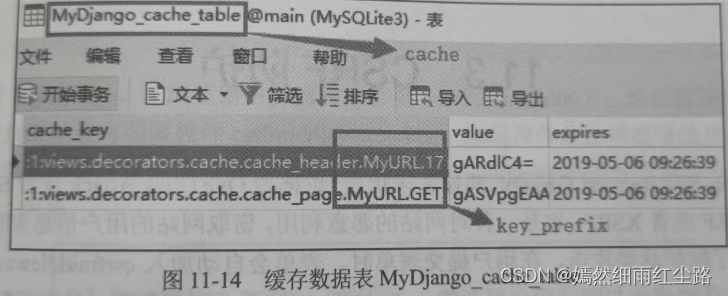
Django缓存
由于Django是动态网站,所有每次请求均会去数据进行相应的操作,当程序访问量大时,耗时必然会更加明显,最简单解决方式是使用:缓存,缓存将一个某个views的返回值保存至内存或者memcache中,若某个时…...

Python 元组
(1)元组中只包含一个元素时,需要在元素后面添加逗号: tup1 (50,); (2)元组中的元素值是不允许修改的,但我们可以对元组进行连接组合: tup1 (12, 34.56); tup2 (abc, xyz);# 以…...
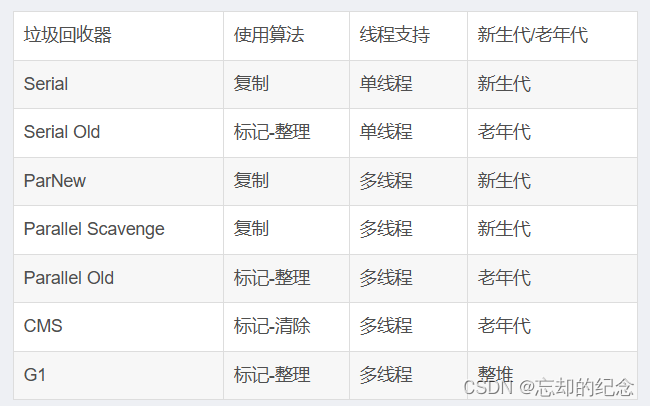
JAVA面试题大全(十八)
1、说一下 jvm 的主要组成部分?及其作用? 类加载器(ClassLoader)运行时数据区(Runtime Data Area)执行引擎(Execution Engine)本地库接口(Native Interface)…...
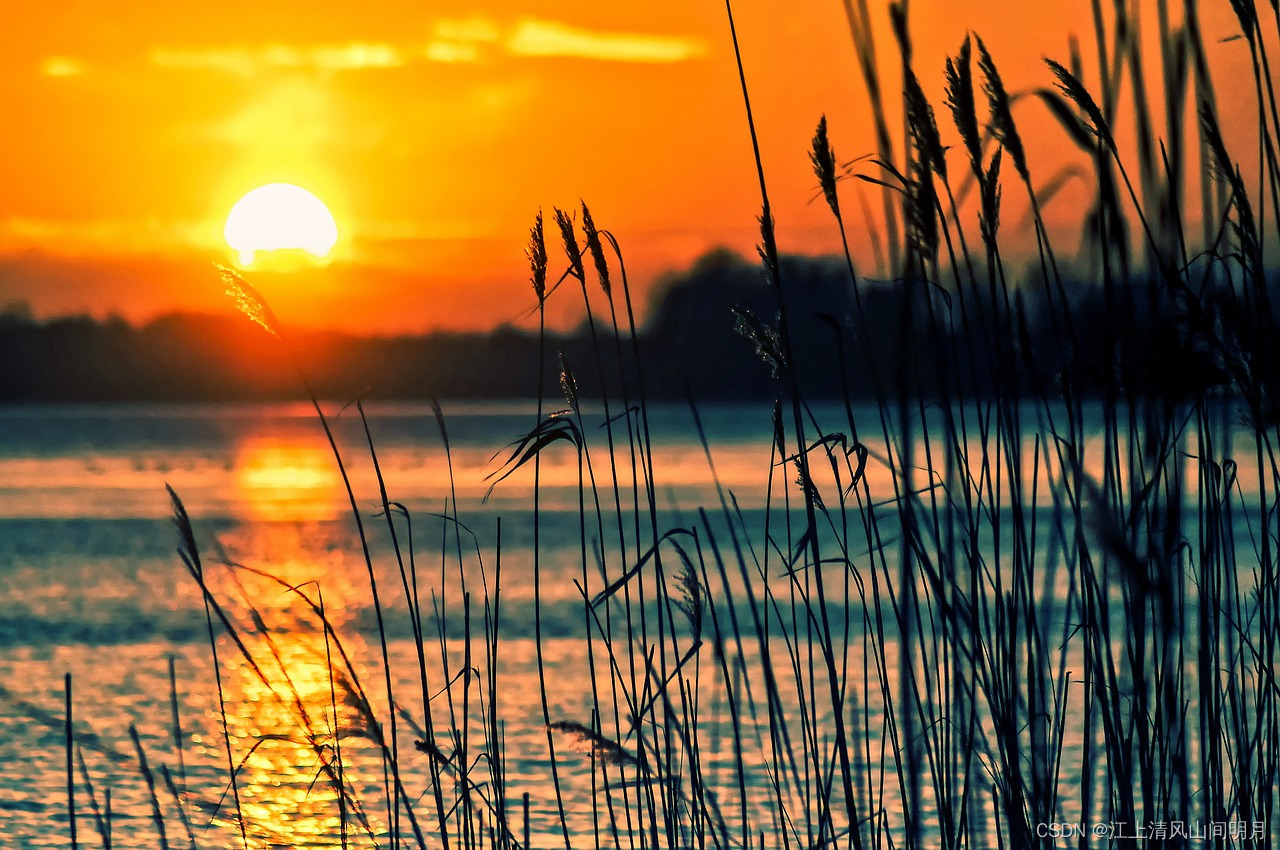
如何利用Firebase Hosting来托管网站
文章目录 如何利用Firebase Hosting来托管网站前提条件详细步骤1. 安装 Firebase CLI2. 登录 Firebase3. 初始化 Firebase 项目4. 准备网站文件5. 部署到 Firebase6. 配置自定义域名(可选) 常见问题 如何利用Firebase Hosting来托管网站 以下是更详细的…...
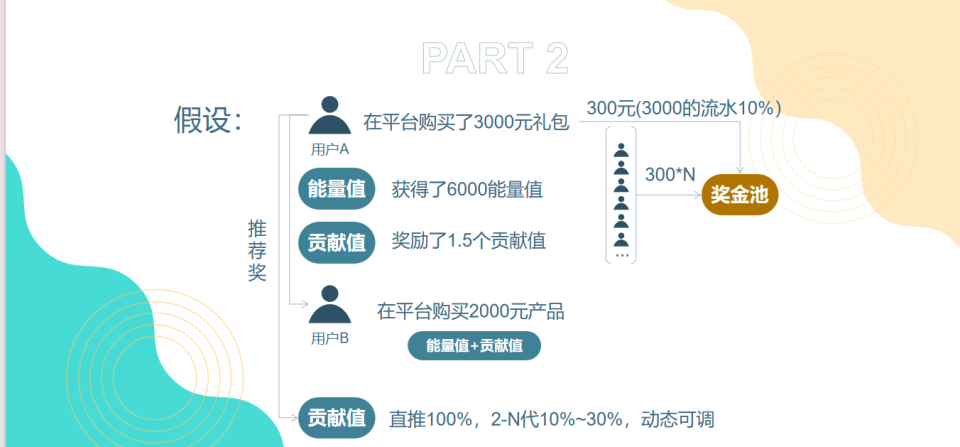
揭秘“循环消费”模式:消费即收益,购物新体验
亲爱的朋友们,大家好,我是李华。今天,我要为大家介绍一种正在悄然兴起的商业模式——“循环消费”。你是否曾想过,在消费的同时,还能获得额外的收益和回馈?这种新型模式正在逐渐改变我们的购物体验。 近期&…...
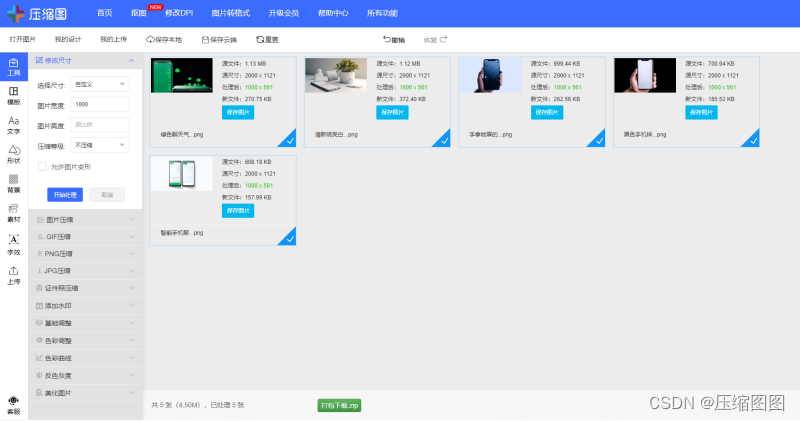
图片怎样在线改像素大小?电脑快速修改图片大小的方法
在设计图片的时候下载的图片尺寸一般会比较大,在网上使用经常会因为尺寸的问题导致无法正常上传,那么如何快速在线改图片大小呢?想要修改图片尺寸可以在直接选择网上的图片改大小工具的功能来快速完成修改,操作简单方便使用&#…...
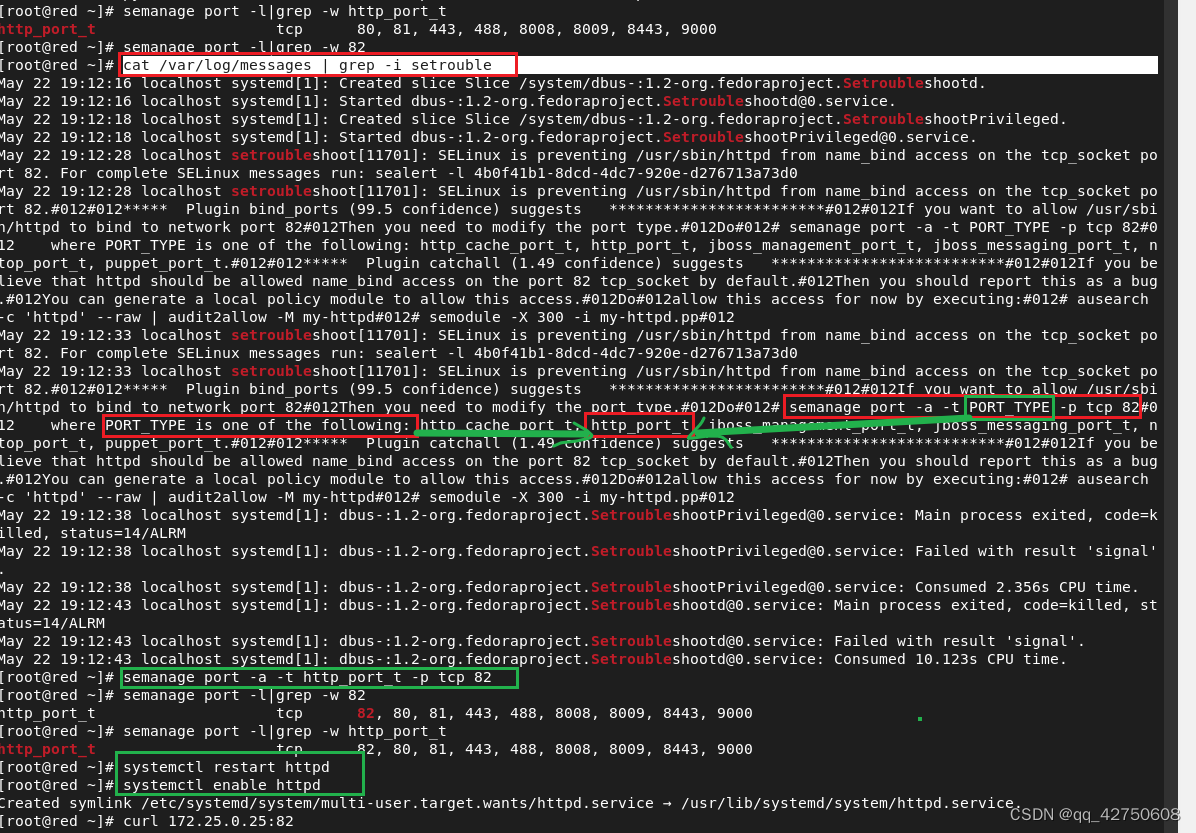
SELINUX=enforcing时无法启动httpd服务的解决方案(semanage命令以及setroubleshoot-server插件的妙用)
一、问题描述: 当/etc/selinux/conf被要求必须是SELINUXenforcing,不被允许使用setenforce 0宽松模式 我们启动httpd就会报错: Job for httpd.service failed because the control process exited with error code. See "systemctl s…...
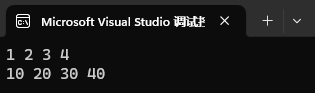
【C++】list的使用方法和模拟实现
❤️欢迎来到我的博客❤️ 前言 list是可以在常数范围内在任意位置进行插入和删除的序列式容器,并且该容器可以前后双向迭代list的底层是双向链表结构,双向链表中每个元素存储在互不相关的独立节点中,在节点中通过指针指向其前一个元素和后…...
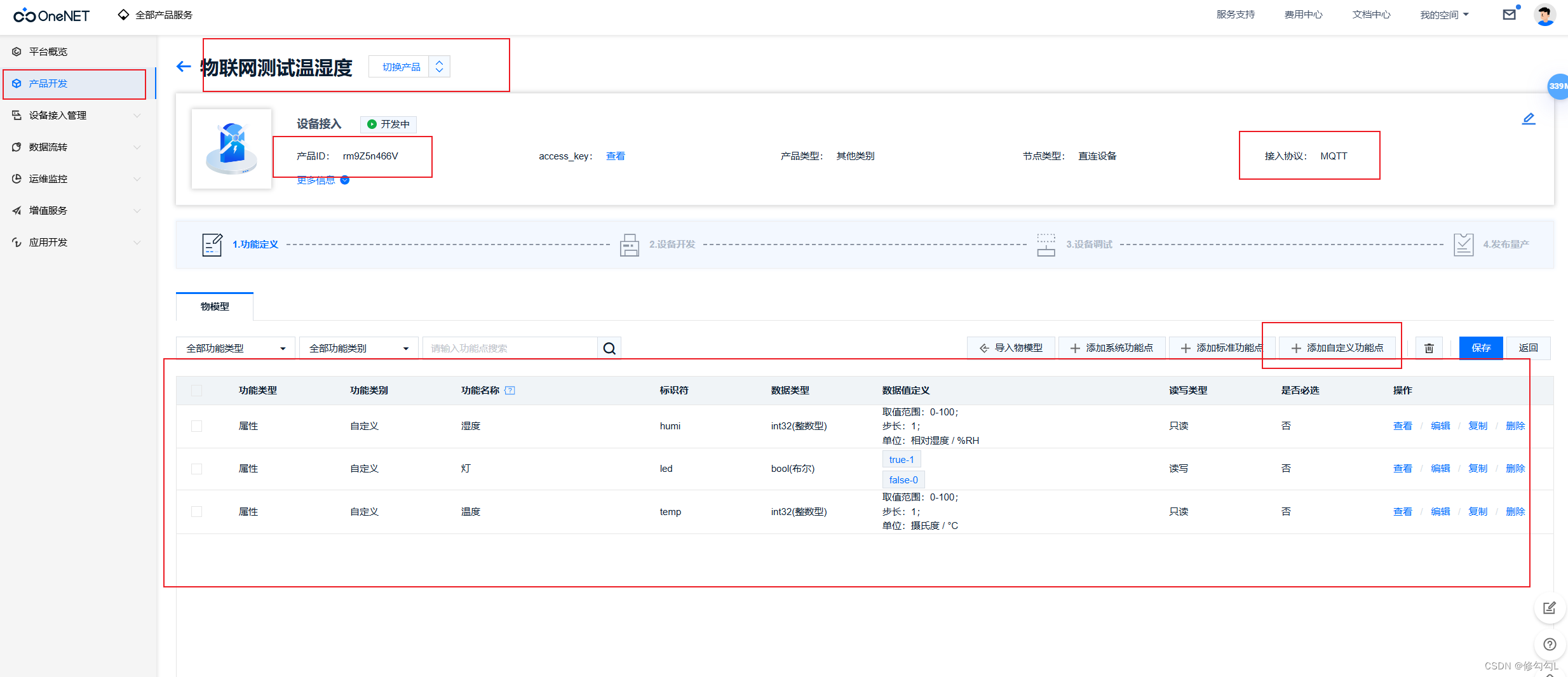
【物联网实战项目】STM32C8T6+esp8266/mqtt+dht11+onenet+uniapp
一、实物图 前端uniapp效果图(实现与onenet同步更新数据) 首先要确定接线图和接线顺序: 1、stm32c8t6开发板连接stlinkv2下载线 ST-LINK V2STM323.3V3.3VSWDIOSWIOSWCLKSWCLKGNDGND 2、ch340串口连接底座(注意RXD和TXD的连接方式…...

Pyhton 二叉树层级遍历
class TreeNode:def __init__(self, val0, leftNone, rightNone):self.val valself.left leftself.right rightclass Solution:def levelOrder(self, root: Optional[TreeNode]) -> List[List[int]]:res []# 空节点,直接返回if not root:return resque [roo…...

Flutter 中的 FadeTransition 小部件:全面指南
Flutter 中的 FadeTransition 小部件:全面指南 在 Flutter 中,动画是一种吸引用户注意力并提供流畅用户体验的强大工具。FadeTransition 是 Flutter 提供的一个动画小部件,它允许子组件在不透明度上进行渐变,从而实现淡入和淡出效…...