Race Condition竞争条件
Race Condition
- Question – why was there no race condition in the first solution (where at most N – 1) buffers can be filled?
- Processes P0 and P1 are creating child processes using the fork() system call
- Race condition on kernel variable next_available_pid which represents the next available process identifier (pid)
- Unless there is a mechanism to prevent P0 and P1 from accessing the variable next_available_pid the same pid could be assigned to two different processes!
进程 P0 和 P1 正在使用 fork() 系统调用创建子进程
内核变量 next_available_pid 代表下一个可用进程标识符 (pid) 的竞赛条件
除非有机制阻止 P0 和 P1 访问变量 next_available_pid,否则相同的 pid 可能被分配给两个不同的进程!
solution
Memory Barrier
• Memory model are the memory guarantees a computer architecture makes to application programs. • Memory models may be either:
• Strongly ordered – where a memory modification of one processor is immediately visible to all other processors. • Weakly ordered – where a memory modification of one processor may not be immediately visible to all other processors.
• A memory barrier is an instruction that forces any change in memory to be propagated (made visible) to all other processors.
内存模型是计算机体系结构为应用程序提供的内存保证。- 内存模型可以是
- 强有序 - 一个处理器的内存修改会立即被所有其他处理器看到。- 弱有序--一个处理器的内存修改可能不会立即被所有其他处理器看到。
- 内存障碍是一种指令,它强制将内存中的任何变化传播(使其可见)到所有其他处理器。
Memory Barrier Instructions
• When a memory barrier instruction is performed, the system ensures that all loads and stores are completed before any subsequent load or store operations are performed.
• Therefore, even if instructions were reordered, the memory barrier ensures that the store operations are completed in memory and visible to other processors before future load or store operations are performed.
执行内存障碍指令时,系统会确保在执行任何后续加载或存储操作之前,完成所有加载和存储操作。
- 因此,即使指令被重新排序,内存屏障也能确保存储操作在内存中完成,并在今后执行加载或存储操作前对其他处理器可见。
Memory Barrier Example
• Returning to the example of slides 6.17 - 6.18 • We could add a memory barrier to the following instructions to ensure Thread 1 outputs 100:
• Thread 1 now performs while (!flag) memory_barrier(); print x
• Thread 2 now performs x = 100; memory_barrier(); flag = true • For Thread 1 we are guaranteed that the value of flag is loaded before the value of x. • For Thread 2 we ensure that the assignment to x occurs before the assignment flag.
Synchronization Hardware
• Many systems provide hardware support for implementing the critical section code.
• Uniprocessors – could disable interrupts
• Currently running code would execute without preemption
• Generally too inefficient on multiprocessor systems
• Operating systems using this not broadly scalable
• We will look at three forms of hardware support:
1. Hardware instructions 2. Atomic variables Hardware Instructions
• Special hardware instructions that allow us to either test-and-modify the content of a word, or to swap the contents of two words atomically (uninterruptedly.) • Test-and-Set instruction • Compare-and-Swap instruction
Atomic Variables
• Typically, instructions such as compare-and-swap are used as building blocks for other synchronization tools. • One tool is an atomic variable that provides atomic (uninterruptible) updates on basic data types such as integers and booleans.
• For example: • Let sequence be an atomic variable • Let increment() be operation on the atomic variable sequence • The Command: increment(&sequence); ensures sequence is incremented without interruption:
Mutex Locks
• Previous solutions are complicated and generally inaccessible to application programmers • OS designers build software tools to solve critical section problem • Simplest is mutex lock
• Boolean variable indicating if lock is available or not • Protect a critical section by
• First acquire() a lock • Then release() the lock • Calls to acquire() and release() must be atomic • Usually implemented via hardware atomic instructions such as compare-and-swap. • But this solution requires busy waiting • This lock therefore called a spinlock
Semaphore
• Synchronization tool that provides more sophisticated ways (than Mutex locks) for processes to synchronize their activities.
• Semaphore S – integer variable • Can only be accessed via two indivisible (atomic) operations • wait() and signal()
• Originally called P() and V() Semaphore (Cont.)
• Definition of the wait() operation wait(S) { while (S value--; if (S->value < 0) { add this process to S->list; block(); } } signal(semaphore *S) { S->value++; if (S->value list; wakeup(P); } }
Problems with Semaphores • Incorrect use of semaphore operations:
• signal(mutex) …. wait(mutex) • wait(mutex) … wait(mutex) • Omitting of wait (mutex) and/or signal (mutex) • These – and others – are examples of what can occur when semaphores and other synchronization tools are used incorrectly.
相关文章:
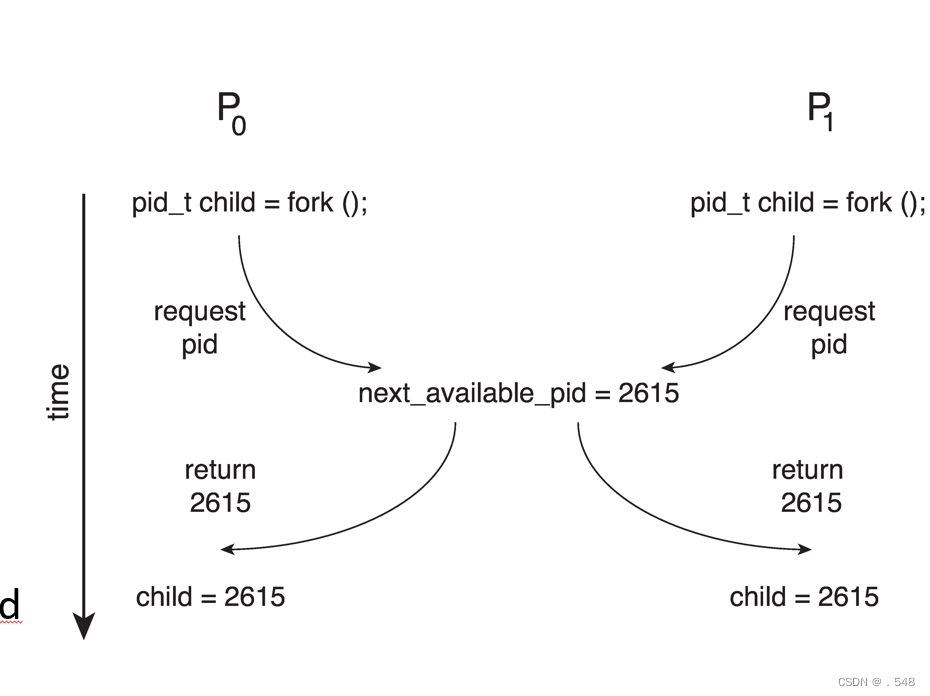
Race Condition竞争条件
Race Condition Question – why was there no race condition in the first solution (where at most N – 1) buffers can be filled?Processes P0 and P1 are creating child processes using the fork() system callRace condition on kernel variable next_available_pid…...

docker 删除本地镜像释放磁盘空间
时间一长,本地镜像文件特别多: 1 linux 配置crontab 定期删除 crontab l 查看 crontab e 编辑 30 3 * * * /home/mqq/gengmingming/cleanImage-realize.sh > /home/mqq/gengmingming/cleanImage-realize.log 2>&12 cleanImage-realize.sh …...
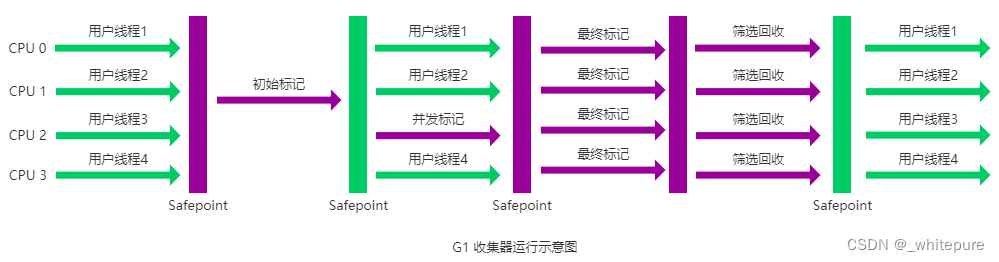
JVM中的垃圾回收器
文章目录 垃圾回收器发展史垃圾回收器分类按线程数分类按工作模式分类按处理方式分类 查看默认垃圾收集器评估垃圾回收器性能指标吞吐量暂停时间吞吐量对比暂停时间 7种经典的垃圾回收器垃圾回收器与垃圾分代垃圾收集器的组合关系Serial GCParNew GCParallel Scavenge GCSerial…...

记录一些可用的AI工具网站
记录一些可用的AI工具网站 AI对话大模型AI图片生成AI乐曲生成AI视频生成AI音频分离 AI对话大模型 当前时代巅峰,Microsoft Copilot:https://copilot.microsoft.com AI图片生成 stable diffusion模型资源分享社区,civitai:https…...
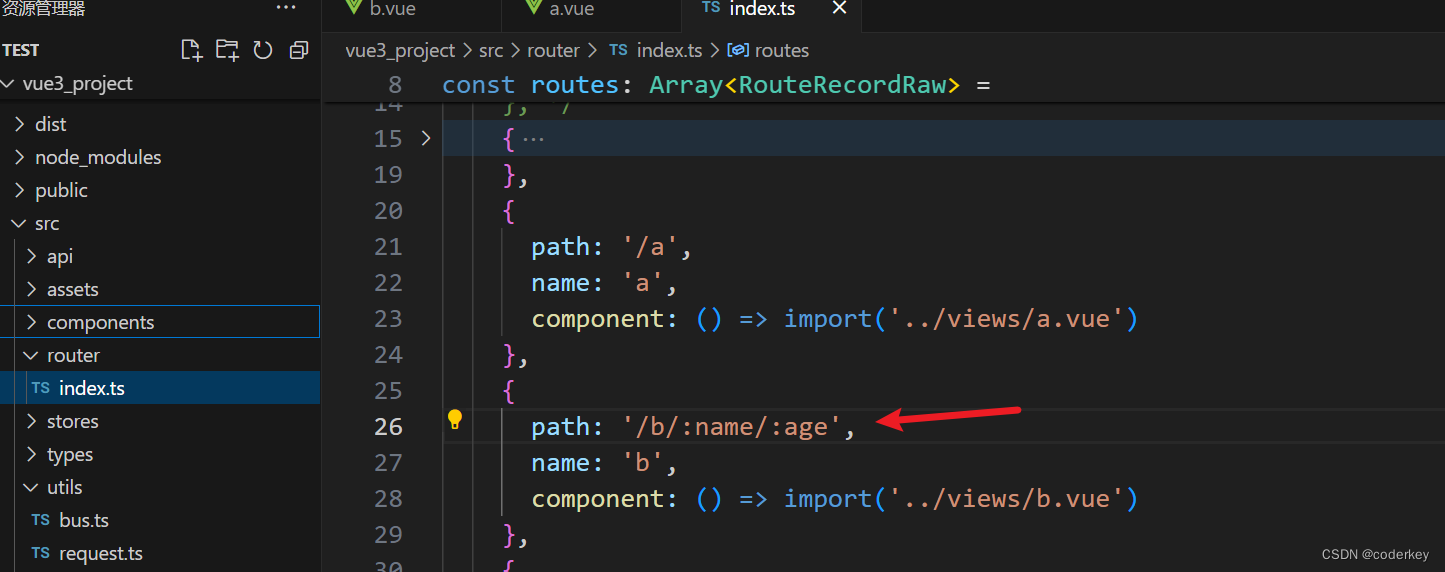
vue3页面传参
一,用query传参 方法: router.push({path: ‘路由地址’, query: ‘参数’}) 例子:a页面携带参数跳转到b页面并且b页面拿到a页面传递过来的参数 在路由router.ts配置 a页面: <template><div >a页面</div>…...

QNX OS微内核系统
微内核架构 微内核(Microkernel)架构是一种操作系统架构模式,其核心思想是尽量将操作系统的基本功能压缩在最小的核心中,而将其他服务(如设备驱动、文件系统、网络协议等)放在用户空间中运行,从而增加系统的灵活性和安全性,这种架构有几个主要特点和优势: 最小化核心…...
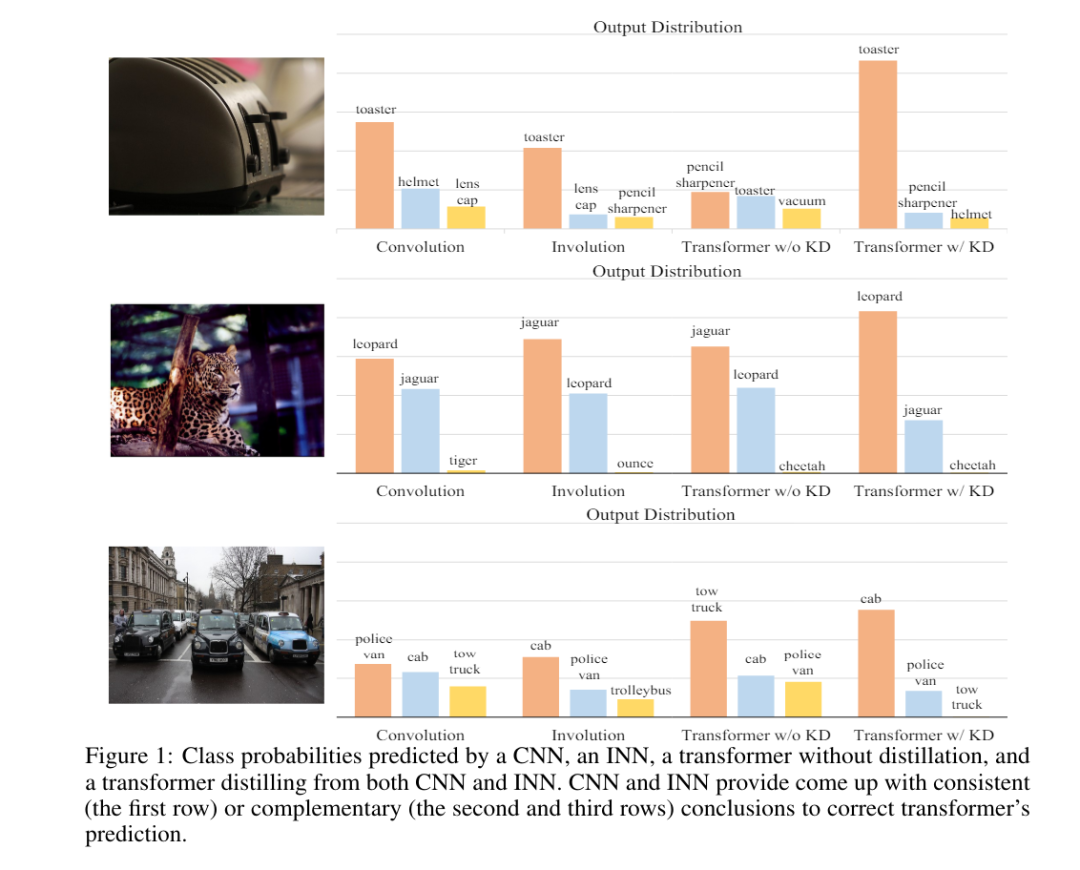
ViT:5 Knowledge Distillation
实时了解业内动态,论文是最好的桥梁,专栏精选论文重点解读热点论文,围绕着行业实践和工程量产。若在某个环节出现卡点,可以回到大模型必备腔调或者LLM背后的基础模型重新阅读。而最新科技(Mamba,xLSTM,KAN)…...
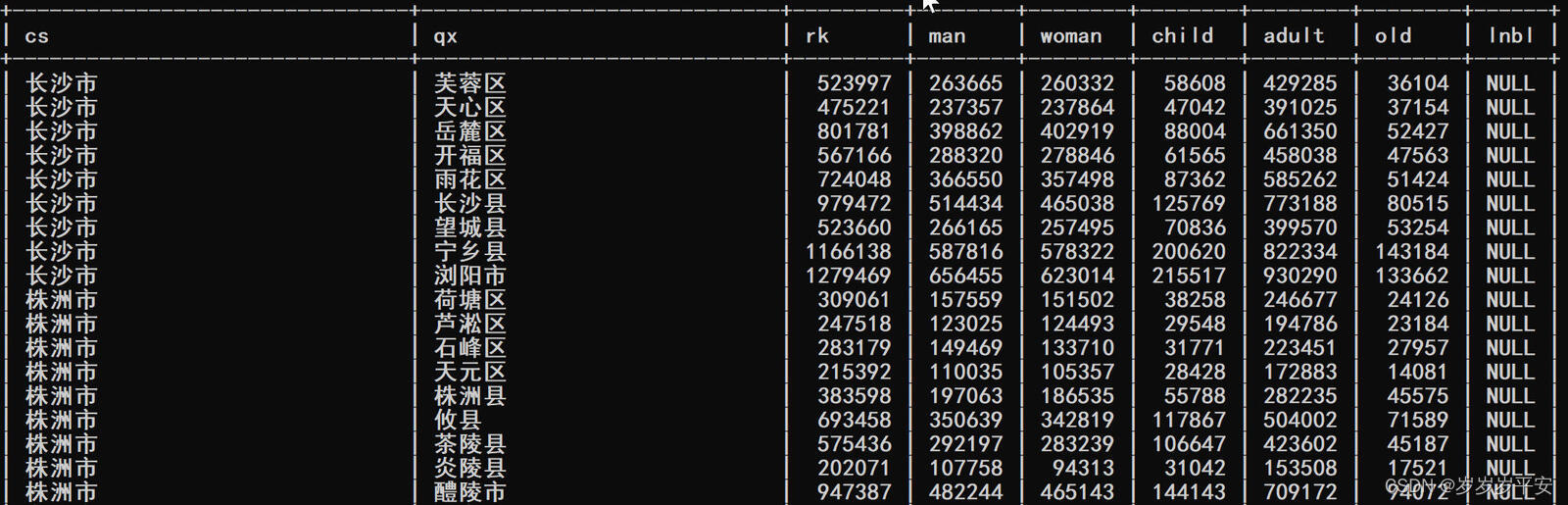
2024头歌数据库期末综合(部分题)
目录 第7关:数据查询三 任务描述 知识补充 答案 第8关:数据查询四 任务描述 知识补充 答案 本篇博客声明:所有题的答案不在一起,可以去作者博客专栏寻找其它文章。 第7关:数据查询三 任务描述 本关任务&#x…...

【Flask】学习
参考B站视频:https://www.bilibili.com/video/BV1v7411M7us/ 目录 第一讲 什么是 flask 修饰器、路由规则 flask 变量规则,灵活传参数据类型:str、int、float(正浮点数,传int会报错)、path、uuid app.…...
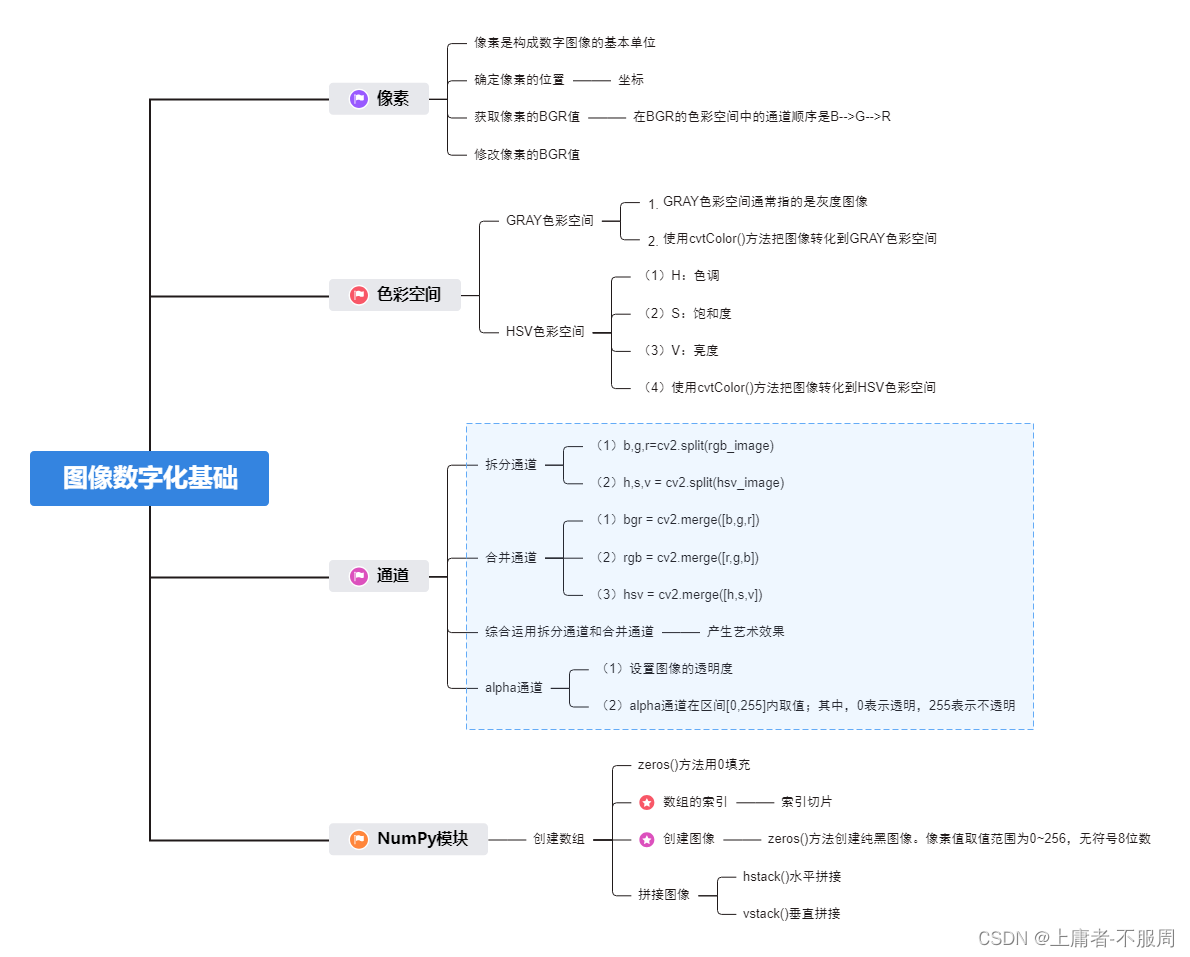
图像数字化基础
一、像素 1、获取图像指定位置的像素 import cv2 image cv2.imread("E:\\images\\2.png") px image[291,218] print("坐标(291,218)上的像素的BGR值是:",px) (1)RGB色彩空间 R通道:红色通道 G通道&…...
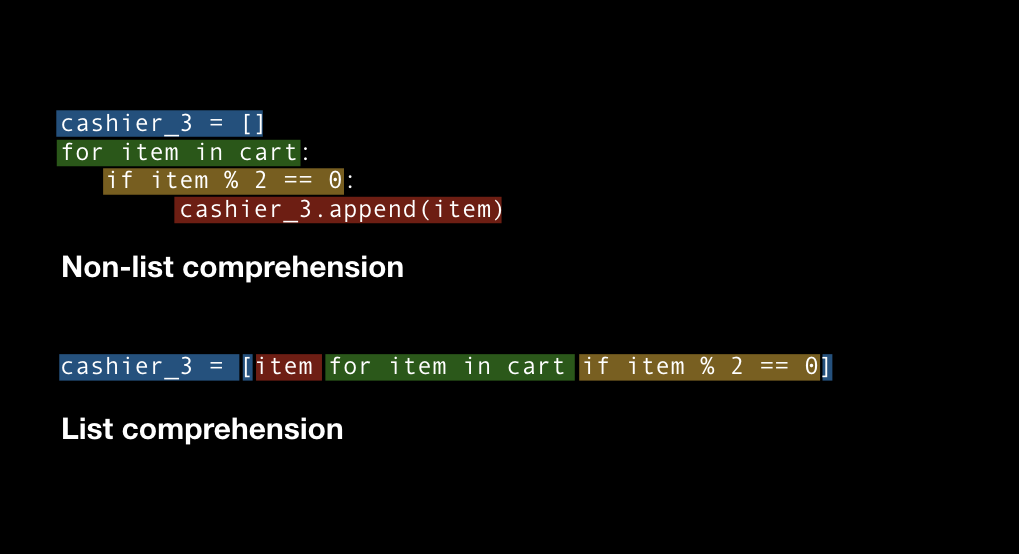
让你的Python代码更简洁:一篇文章带你了解Python列表推导式
文章目录 📖 介绍 📖🏡 演示环境 🏡📒 列表推导式 📒📝 语法📝 条件筛选📝 多重循环📝 列表推导式的优点📝 使用场景📝 示例代码🎯 示例1🎯 示例2⚓️ 相关链接 ⚓️📖 介绍 📖 在Python编程中,列表推导式是一种强大且高效的语法,它允许你用…...
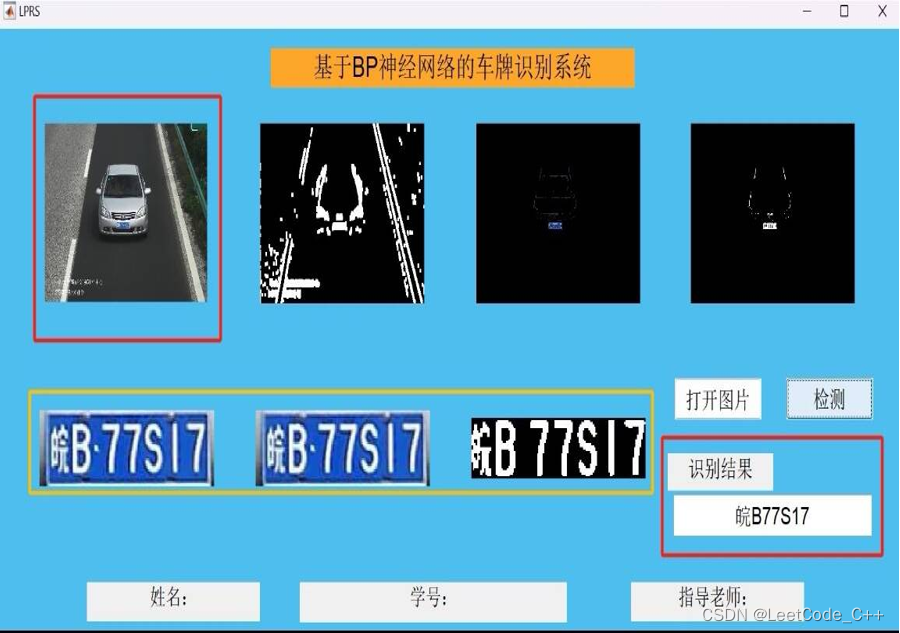
基于Matlab的BP神经网络的车牌识别系统(含GUI界面)【W7】
简介: 本系统结合了图像处理技术和机器学习方法(BP神经网络),能够有效地实现车牌的自动识别。通过预处理、精确定位、字符分割和神经网络识别,系统能够准确地识别各种车牌图像,并在智能交通管理、安防监控等…...

jetpack compose的@Preview和自定义主题
1.Preview Preview可以在 Android Studio 的预览窗口中实时查看和调试 UI 组件。 基本使用 import androidx.compose.foundation.layout.fillMaxSize import androidx.compose.material.MaterialTheme import androidx.compose.material.Surface import androidx.compose.ma…...

Temu(拼多多跨境电商) API接口:获取商品详情
核心功能介绍——获取商品详情 在竞争激烈的电商市场中,快速、准确地获取商品数据详情对于电商业务的成功至关重要。此Temu接口的核心功能在于其能够实时、全面地获取平台上的商品数据详情。商家通过接入Temu接口,可以轻松获取商品的标题、价格、库存、…...

ArcGIS Pro SDK (五)内容 2 工程项
ArcGIS Pro SDK (五)内容 2 地图工程 目录 ArcGIS Pro SDK (五)内容 2 地图工程1 将文件夹连接项添加到当前工程2.2 获取所有工程项2.3 获取工程的所有“MapProjectItems”2.4 获取特定的“MapProjectItem”2.5 获取所有“样式工程…...

【ai】初识pytorch
初识PyTorch 大神的例子运行: 【ai】openai-quickstart 配置pycharm工程 简单例子初识一下Pytorch 好像直接点击下载比较慢? 大神的代码 在这个例子中,首先定义一个线性模型,该模型有一个输入特征和一个输出特征。然后定义一个损失函数和一个优化器,接着生成一些简单的线性…...
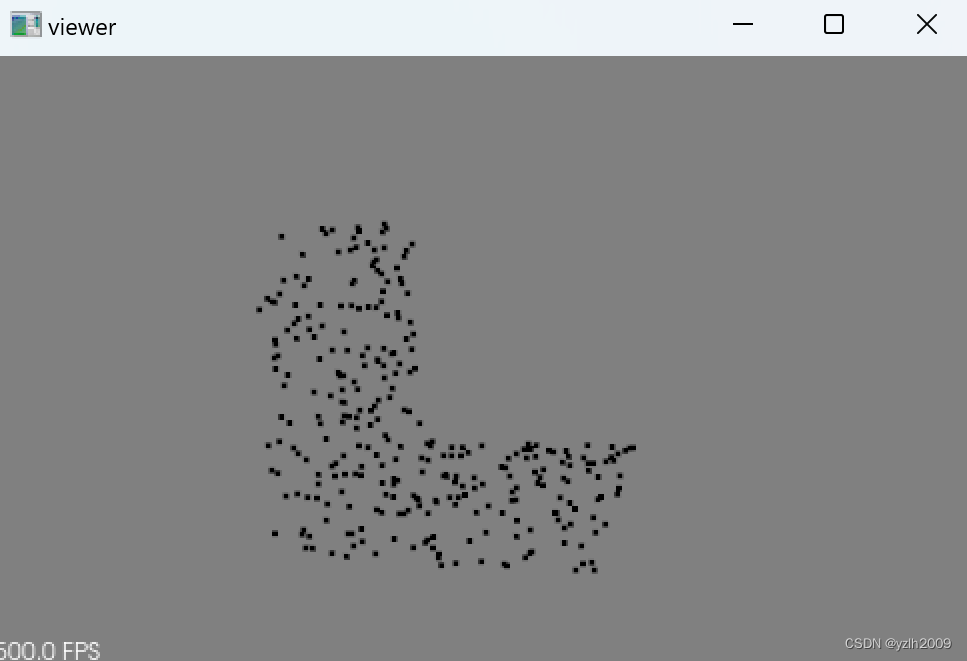
pcl::PointXYZRGBA造成点云无法显示
如果pcd文件没有rgba信息,使用pcl::PointXYZRGBA类型打开会提示以下信息: Failed to find match for field rgba另外,显示出来的点云是黑色,如果使用默认背景色为黑色,就无法显示点云了。 如果设置其它背景色…...
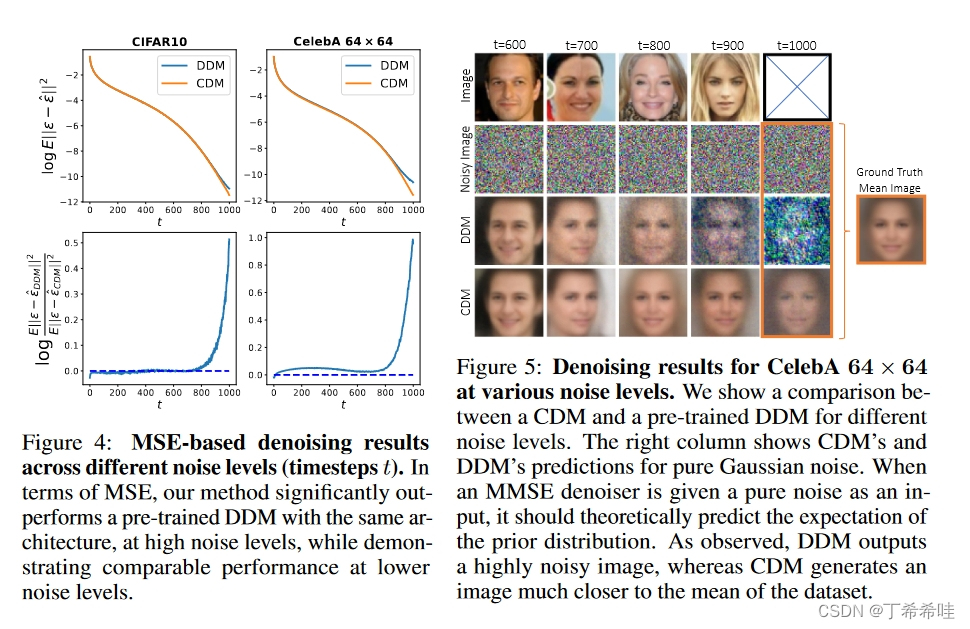
【论文精读】分类扩散模型:重振密度比估计(Revitalizing Density Ratio Estimation)
文章目录 一、文章概览(一)问题的提出(二)文章工作 二、理论背景(一)密度比估计DRE(二)去噪扩散模型 三、方法(一)推导分类和去噪之间的关系(二&a…...
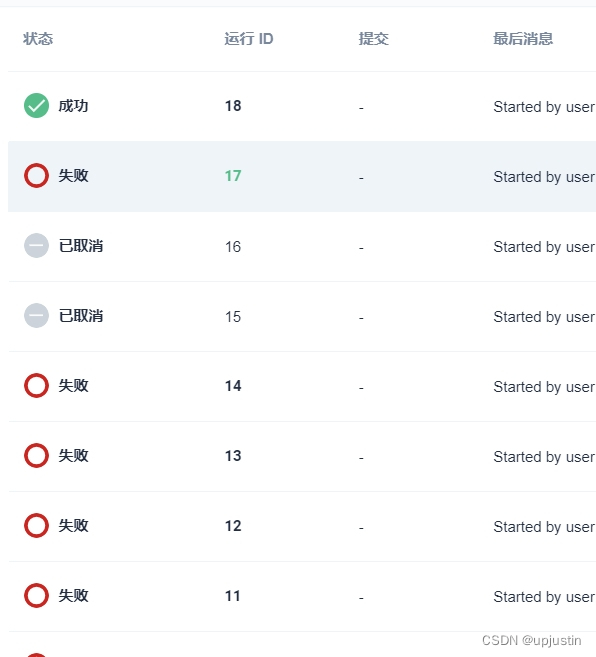
kubesphere踩过的坑,持续更新....
踩过的坑 The connection to the server lb.kubesphere.local:6443 was refused - did you specify the right host… 另一篇文档中 dashboard 安装 需要在浏览器中输入thisisunsafe,即可进入登录页面 ingress 安装的问题 问题描述: 安装后通过命令 kubectl g…...
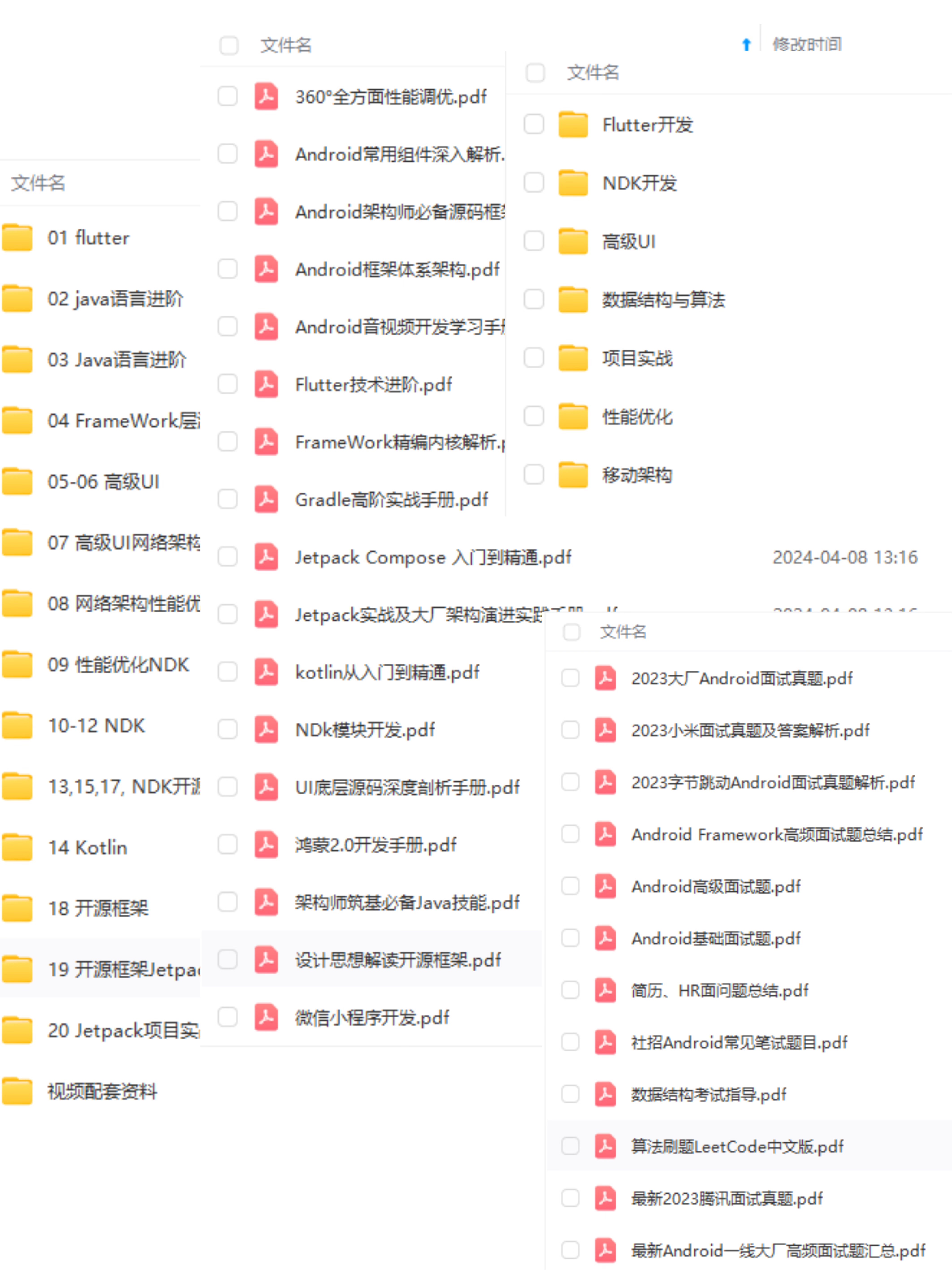
做Android开发怎么才能不被淘汰?
多学一项技能,可能就会成为你升职加薪的利器。经常混迹于各复杂业务线的人,才能跳出重复工作、不断踩坑的怪圈。而一个成熟的码农在于技术过关后,更突出其他技能对专业技术的附加值。 毋须讳言的是,35岁以后你的一线coding能力一…...
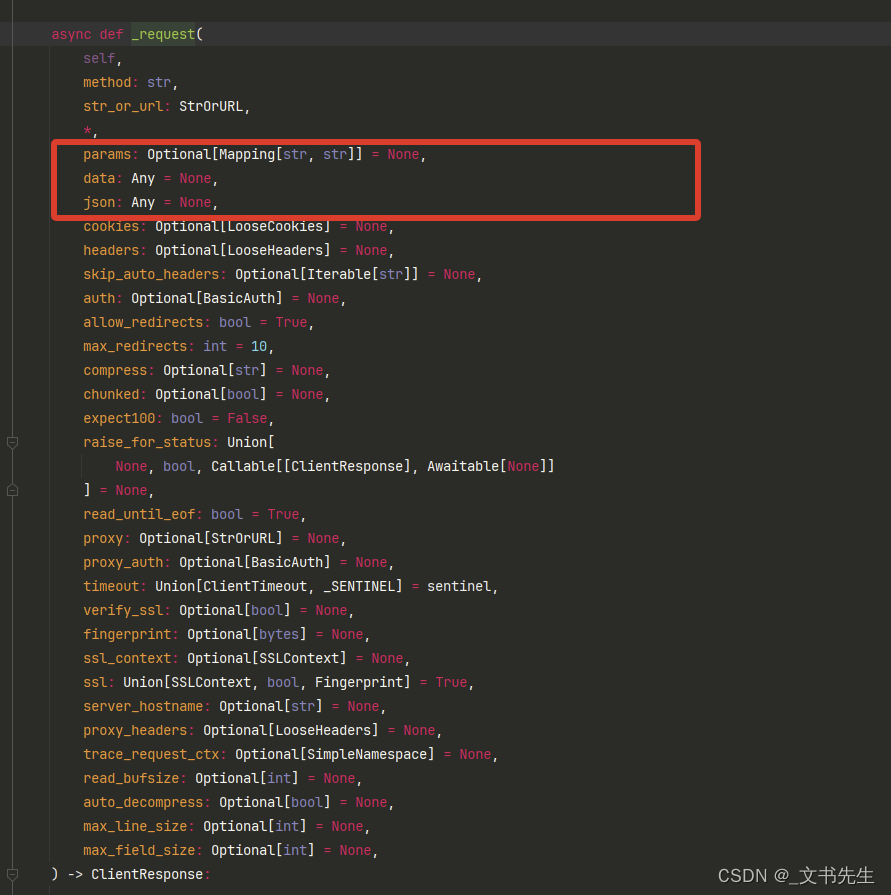
异步爬虫:aiohttp 异步请求库使用:
使用requests 请求库虽然可以完成爬虫业务,但是对于异步任务来说,它是做不到的, 这时候我们需要借助 aiohttp 异步请求库来完成异步爬虫的编写: 话不多说,直接看示例: 注意:楼主使用的python版…...

代码随想录算法训练营第四十七天|LeetCode123 买卖股票的最佳时机Ⅲ
题1: 指路:123. 买卖股票的最佳时机 III - 力扣(LeetCode) 思路与代码: 买卖股票专题中三者不同的是Ⅰ为只买卖一次,Ⅱ可多次买卖,Ⅲ最多可买卖两次。那么我们将买买卖行为分为五个状态部分(…...
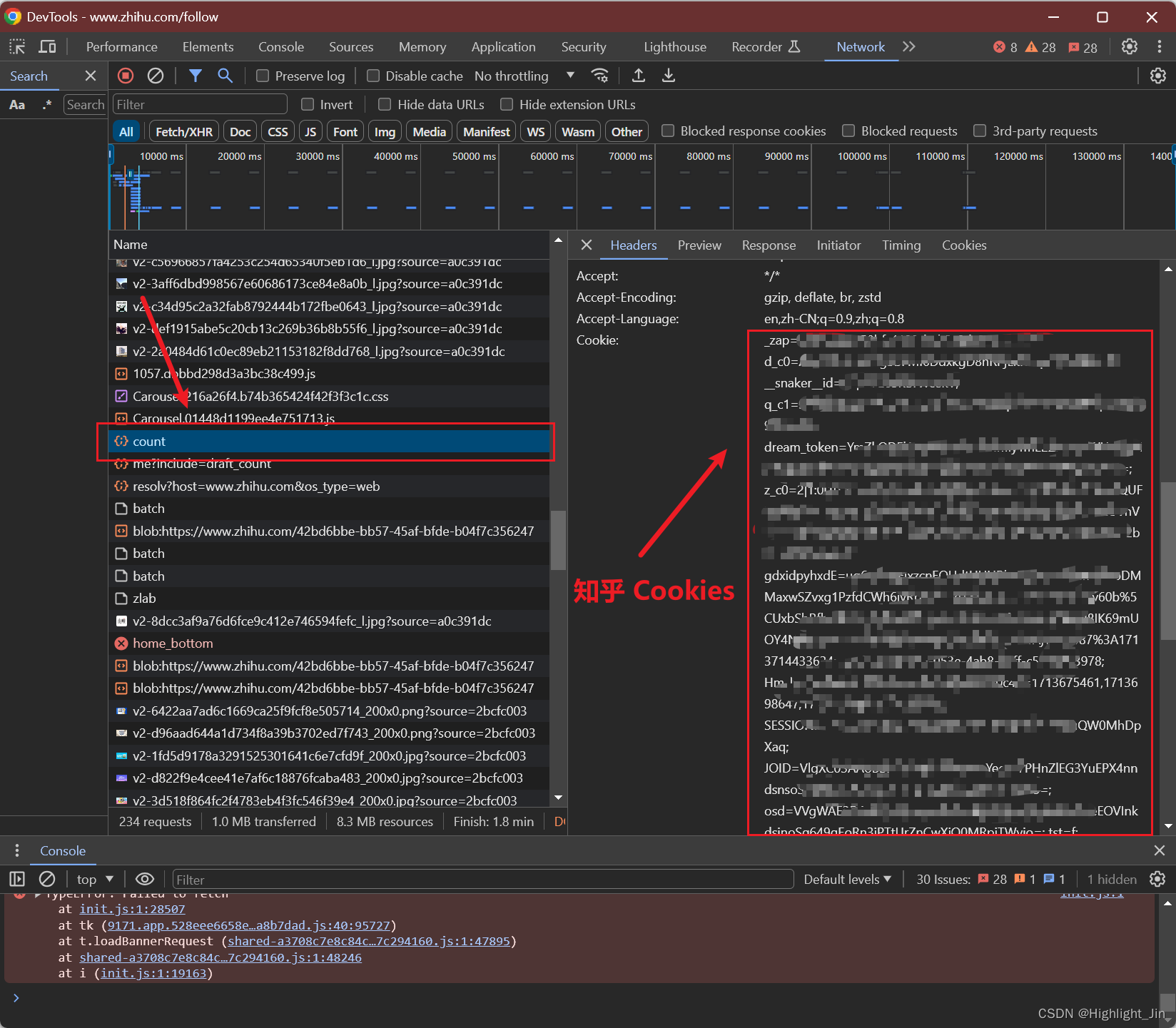
将知乎专栏文章转换为 Markdown 文件保存到本地
一、参考内容 参考知乎文章代码 | 将知乎专栏文章转换为 Markdown 文件保存到本地,利用代码为GitHub:https://github.com/chenluda/zhihu-download。 二、步骤 1.首先安装包flask、flask-cors、markdownify 2. 运行app.py 3.在浏览器中打开链接&…...
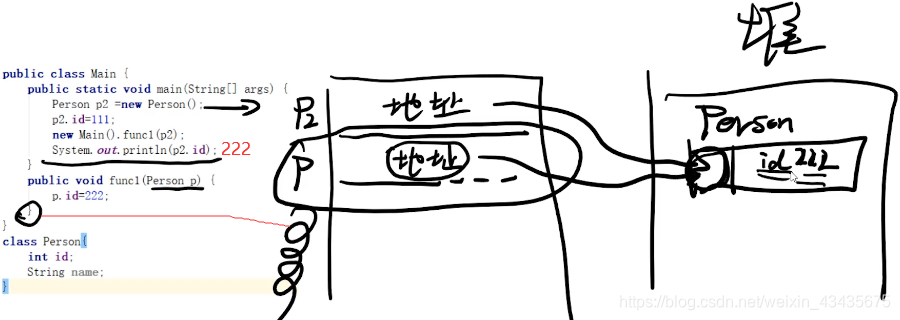
【notes2】并发,IO,内存
文章目录 1.线程/协程/异步:并发对应硬件资源是cpu,线程是操作系统如何利用cpu资源的一种抽象2.并发:cpu,线程2.1 可见性:volatile2.2 原子性(读写原子):AtomicInteger/synchronized…...
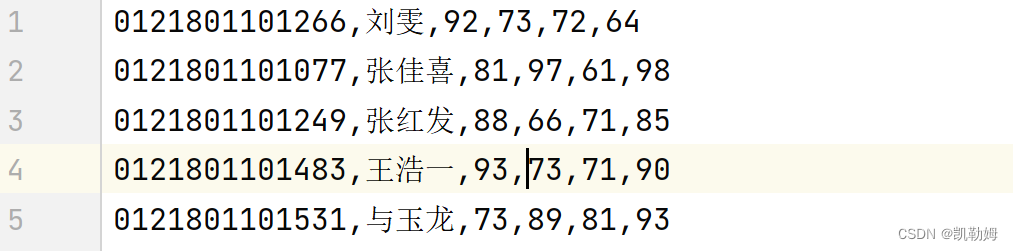
Python题目
实例 3.1 兔子繁殖问题(斐波那契数列) 兔子从出生后的第三个月开始,每月都会生一对兔子,小兔子成长到第三个月后也会生一对独自。初始有一对兔子,假如兔子都不死,那么计算并输出1-n个月兔子的数量 n int…...

Hive怎么调整优化Tez引擎的查询?在Tez上优化Hive查询的指南
文章目录 在Tez上优化Hive查询的指南调优指南理解Tez中的并行化理解mapper数量理解reducer数量 并发案例1:未指定队列名称案例2:指定队列名称并发的指南/建议 容器复用和预热容器容器复用预热容器 一般Tez调优参数 在Tez上优化Hive查询的指南 在Tez上优…...
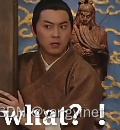
关于小程序内嵌H5页面交互的问题?
有木有遇到?有木有遇到。 小程序内嵌了H5,然后H5某个按钮,需要打开小程序某个页面进行信息完善或登记,登记后要返回H5页面,而H5页面要动态显示刚才在小程序页面登记的信息。 操作流程是这样: 方案1&#…...

Linux下手动查杀木马与Rootkit的实战指南
模拟木马程序的自动运行 黑客可以通过多种方式让木马程序自动运行,包括: 计划任务 (crontab):通过设置定时任务来周期性地执行木马脚本。开机启动:在系统的启动脚本中添加木马程序,确保系统启动时木马也随之运行。替…...
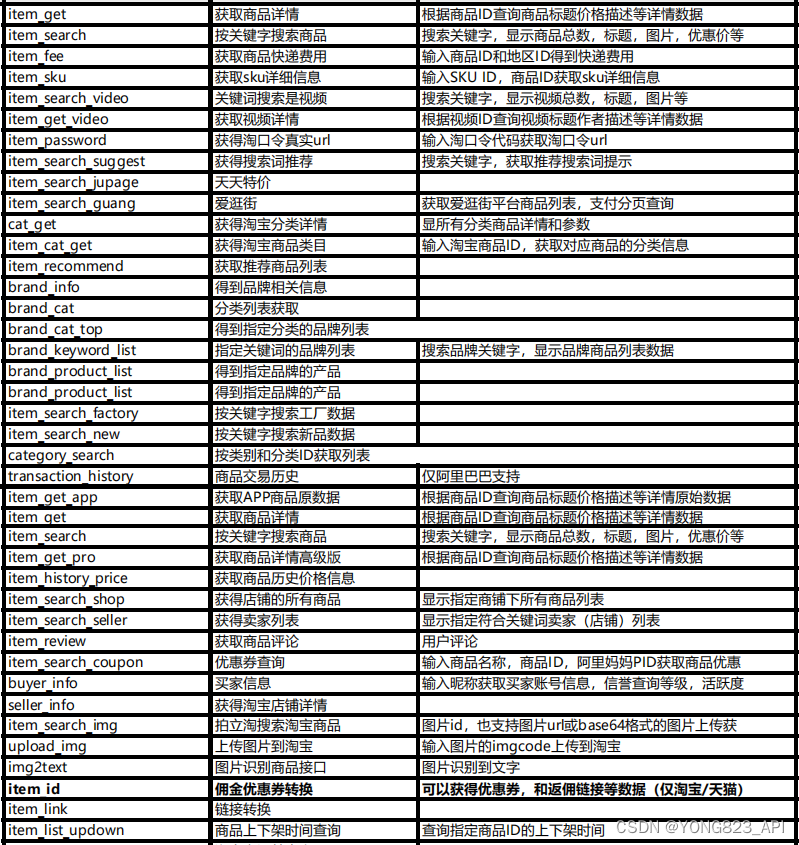
电商爬虫API的定制开发:满足个性化需求的解决方案
一、引言 随着电子商务的蓬勃发展,电商数据成为了企业决策的重要依据。然而,电商数据的获取并非易事,特别是对于拥有个性化需求的企业来说,更是面临诸多挑战。为了满足这些个性化需求,电商爬虫API的定制开发成为了解决…...

nuc马原复习资料
哲学:世界观的理论形态,或者说是系统化、理论化的世界观;世界观和方法论的统一。马克思主义哲学:辩证唯物主义和历史唯物主义,关于自然。社会和思维发展的普遍规律的学说,无产阶级世界观的理论体系。世界观…...