Spring Profiles and @Profile
1. Overview
In this tutorial, we’ll focus on introducing Profiles in Spring.
Profiles are a core feature of the framework — allowing us to map our beans to different profiles — for example, dev
, test
, and prod
.
We can then activate different profiles in different environments to bootstrap only the beans we need.
2. Use @Profile on a Bean
Let’s start simple and look at how we can make a bean belong to a particular profile. We use the @Profile annotation — we are mapping the bean to that particular profile
; the annotation simply takes the names of one (or multiple) profiles.
Consider a basic scenario: We have a bean that should only be active during development but not deployed in production
.
We annotate that bean with a dev profile, and it will only be present in the container during development. In production, the dev simply won’t be active:
@Component
@Profile("dev")
public class DevDatasourceConfig
As a quick sidenote, profile names can also be prefixed with a NOT operator, e.g., !dev
, to exclude them from a profile.
In the example, the component is activated only if dev profile is not active:
@Component
@Profile("!dev")
public class DevDatasourceConfig
3. Declare Profiles in XML
Profiles can also be configured in XML. The tag has a profile attribute, which takes comma-separated values of the applicable profiles:
<beans profile="dev"><bean id="devDatasourceConfig" class="org.baeldung.profiles.DevDatasourceConfig" />
</beans>
4. Set Profiles
The next step is to activate and set the profiles so that the respective beans are registered in the container.
This can be done in a variety of ways, which we’ll explore in the following sections.
4.1. Programmatically via WebApplicationInitializer Interface
In web applications, WebApplicationInitializer
can be used to configure the ServletContext
programmatically.
It’s also a very handy location to set our active profiles programmatically:
@Configuration
public class MyWebApplicationInitializer implements WebApplicationInitializer {@Overridepublic void onStartup(ServletContext servletContext) throws ServletException {servletContext.setInitParameter("spring.profiles.active", "dev");}
}
4.2. Programmatically via ConfigurableEnvironment
We can also set profiles directly on the environment:
@Autowired
private ConfigurableEnvironment env;
...
env.setActiveProfiles("dev");
4.3. Context Parameter in web.xml
Similarly, we can define the active profiles in the web.xml file of the web application, using a context parameter:
<context-param><param-name>contextConfigLocation</param-name><param-value>/WEB-INF/app-config.xml</param-value>
</context-param>
<context-param><param-name>spring.profiles.active</param-name><param-value>dev</param-value>
</context-param>
4.4. JVM System Parameter
The profile names can also be passed in via a JVM system parameter. These profiles will be activated during application startup:
-Dspring.profiles.active=dev
4.5. Environment Variable
In a Unix environment, profiles can also be activated via the environment variable
:
export spring_profiles_active=dev
4.6. Maven Profile
Spring profiles can also be activated via Maven profiles, by specifying the spring.profiles.active
configuration property.
In every Maven profile, we can set a spring.profiles.active property
:
<profiles><profile><id>dev</id><activation><activeByDefault>true</activeByDefault></activation><properties><spring.profiles.active>dev</spring.profiles.active></properties></profile><profile><id>prod</id><properties><spring.profiles.active>prod</spring.profiles.active></properties></profile>
</profiles>
Its value will be used to replace the @spring.profiles.active@
placeholder in application.properties
:
spring.profiles.active=@spring.profiles.active@
Now we need to enable resource filtering in pom.xml
:
<build><resources><resource><directory>src/main/resources</directory><filtering>true</filtering></resource></resources>...
</build>
and append a -P
parameter to switch which Maven profile will be applied:
mvn clean package -Pprod
This command will package the application for prod profile. It also applies the spring.profiles.active
value prod for this application when it is running.
4.7. @ActiveProfile in Tests
Tests make it very easy to specify what profiles are active using the @ActiveProfile annotation to enable specific profiles:
@ActiveProfiles("dev")
So far, we’ve looked at multiple ways of activating profiles. Let’s now see which one has priority over the other and what happens if we use more than one, from highest to lowest priority:
- Context parameter in web.xml
- WebApplicationInitializer
- JVM System parameter
- Environment variable
- Maven profile
5. The Default Profile
Any bean that does not specify a profile belongs to the default profile.
Spring also provides a way to set the default profile when no other profile is active — by using the spring.profiles.default
property.
6. Get Active Profiles
Spring’s active profiles drive the behavior of the @Profile annotation for enabling/disabling beans. However, we may also wish to access the list of active profiles programmatically.
We have two ways to do it, using Environment or spring.profiles.active
.
6.1. Using Environment
We can access the active profiles from the Environment object by injecting it:
public class ProfileManager {@Autowiredprivate Environment environment;public void getActiveProfiles() {for (String profileName : environment.getActiveProfiles()) {System.out.println("Currently active profile - " + profileName);} }
}
6.2. Using spring.profiles.active
Alternatively, we could access the profiles by injecting the property spring.profiles.active
:
@Value("${spring.profiles.active}")
private String activeProfile;
Here, our activeProfile variable will contain the name of the profile that is currently active
,
-And if there are several, it’ll contain their names separated by a comma
.
And if we want to access the list of them just like in the previous example, we can do it by splitting the activeProfile variable:
public class ProfileManager {@Value("${spring.profiles.active:}")private String activeProfiles;public String getActiveProfiles() {for (String profileName : activeProfiles.split(",")) {System.out.println("Currently active profile - " + profileName);}}
}
However, we should consider what would happen if there is no active profile at all
. With our code above, the absence of an active profile would prevent the application context from being created. This would result in an IllegalArgumentException
owing to the missing placeholder for injecting into the variable.
In order to avoid this, we can define a default value
:
@Value("${spring.profiles.active:}")
private String activeProfile;
Now, if no profiles are active, our activeProfile will just contain an empty string.
7. Example: Separate Data Source Configurations Using Profiles
Now that the basics are out of the way, let’s take a look at a real example.
Consider a scenario where we have to maintain the data source configuration for both the development and production environments
.
Let’s create a common interface DatasourceConfig that needs to be implemented by both data source implementations:
public interface DatasourceConfig {public void setup();
}
Following is the configuration for the development environment:
@Component
@Profile("dev")
public class DevDatasourceConfig implements DatasourceConfig {@Overridepublic void setup() {System.out.println("Setting up datasource for DEV environment. ");}
}
And configuration for the production environment:
@Component
@Profile("production")
public class ProductionDatasourceConfig implements DatasourceConfig {@Overridepublic void setup() {System.out.println("Setting up datasource for PRODUCTION environment. ");}
}
Now let’s create a test and inject our DatasourceConfig interface; depending on the active profile, Spring will inject DevDatasourceConfig or ProductionDatasourceConfig bean:
public class SpringProfilesWithMavenPropertiesIntegrationTest {@AutowiredDatasourceConfig datasourceConfig;public void setupDatasource() {datasourceConfig.setup();}
}
When the dev profile is active, Spring injects DevDatasourceConfig object, and when calling then setup() method, the following is the output:
Setting up datasource for DEV environment.
8. Profiles in Spring Boot
Spring Boot supports all the profile configuration outlined so far, with a few additional features.
8.1. Activating or Setting a Profile
The initialization parameter spring.profiles.active
, introduced in Section 4, can also be set up as a property in Spring Boot to define currently active profiles. This is a standard property that Spring Boot will pick up automatically:
spring.profiles.active=dev
However, starting Spring Boot 2.4, this property cannot be used in conjunction with spring.config.activate.on-profile
, as this could raise a ConfigDataException
(i.e. an InvalidConfigDataPropertyException
or an InactiveConfigDataAccessException
).
To set profiles programmatically, we can also use the SpringApplication class:
SpringApplication.setAdditionalProfiles("dev");
To set profiles using Maven in Spring Boot, we can specify profile names under spring-boot-maven-plugin
in pom.xml
:
<plugins><plugin><groupId>org.springframework.boot</groupId><artifactId>spring-boot-maven-plugin</artifactId><configuration><profiles><profile>dev</profile></profiles></configuration></plugin>...
</plugins>
and execute the Spring Boot-specific Maven goal:
mvn spring-boot:run
8.2. Profile-specific Properties Files
However, the most important profiles-related feature that Spring Boot brings is profile-specific properties files. These have to be named in the format application-{profile}.properties
.
Spring Boot will automatically load the properties in an application.properties file for all profiles
, and the ones in profile-specific.properties
files only for the specified profile.
For example, we can configure different data sources for dev and production profiles by using two files named application-dev.properties
and application-production.properties
:
In the application-production.properties
file, we can set up a MySql data source:
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/db
spring.datasource.username=root
spring.datasource.password=root
Then we can configure the same properties for the dev profile in the application-dev.properties
file, to use an in-memory H2 database:
spring.datasource.driver-class-name=org.h2.Driver
spring.datasource.url=jdbc:h2:mem:db;DB_CLOSE_DELAY=-1
spring.datasource.username=sa
spring.datasource.password=sa
In this way, we can easily provide different configurations for different environments.
Prior to Spring Boot 2.4, it was possible to activate a profile from profile-specific documents. But that is no longer the case; with later versions, the framework will throw – again – an InvalidConfigDataPropertyException
or an InactiveConfigDataAccessException
in these circumstances.
8.3. Multi-Document Files
To further simplify defining properties for separate environments, we can even club all the properties in the same file and use a separator to indicate the profile
.
Starting version 2.4, Spring Boot has extended its support for multi-document files for properties files in addition to previously supported YAML
. So now, we can specify the dev and production properties in the same application.properties
:
my.prop=used-always-in-all-profiles
#---
spring.config.activate.on-profile=dev
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/db
spring.datasource.username=root
spring.datasource.password=root
#---
spring.config.activate.on-profile=production
spring.datasource.driver-class-name=org.h2.Driver
spring.datasource.url=jdbc:h2:mem:db;DB_CLOSE_DELAY=-1
spring.datasource.username=sa
spring.datasource.password=sa
This file is read by Spring Boot in top to bottom order
. That is, if some property, say my.prop
, occurs once more at the end in the above example, the endmost value will be considered.
8.4. Profile Groups
Another feature added in Boot 2.4 is Profile Groups. As the name suggests, it allows us to group similar profiles together.
Let’s consider a use case where we'd have multiple configuration profiles for the production environment
. Say, a proddb for the database and prodquartz for the scheduler in the production environment.
To enable these profiles all at once via our application.properties file, we can specify:
spring.profiles.group.production=proddb,prodquartz
Consequently, activating the production profile will activate proddb and prodquartz as well.
参考:
Spring Profiles
相关文章:

Spring Profiles and @Profile
1. Overview In this tutorial, we’ll focus on introducing Profiles in Spring. Profiles are a core feature of the framework — allowing us to map our beans to different profiles — for example, dev, test, and prod. We can then activate different profiles…...

数据分析-数据探索
文章目录前言主要内容总结更多宝藏前言 😎🥳😎🤠😮🤖🙈💭🍳🍱 随着大数据和人工智能技术的不断发展,数据分析已经成为了一种非常重要的技能和工…...
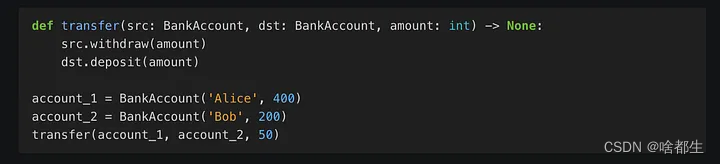
7个最受欢迎的Python库,大大提高开发效率
当第三方库可以帮我们完成需求时,就不要重复造轮子了 整理了GitHub上7个最受好评的Python库,将在你的开发之旅中提供帮助 PySnooper 很多时候时间都花在了Debug上,大多数人呢会在出错位置的附近使用print,打印某些变量的值 这个…...
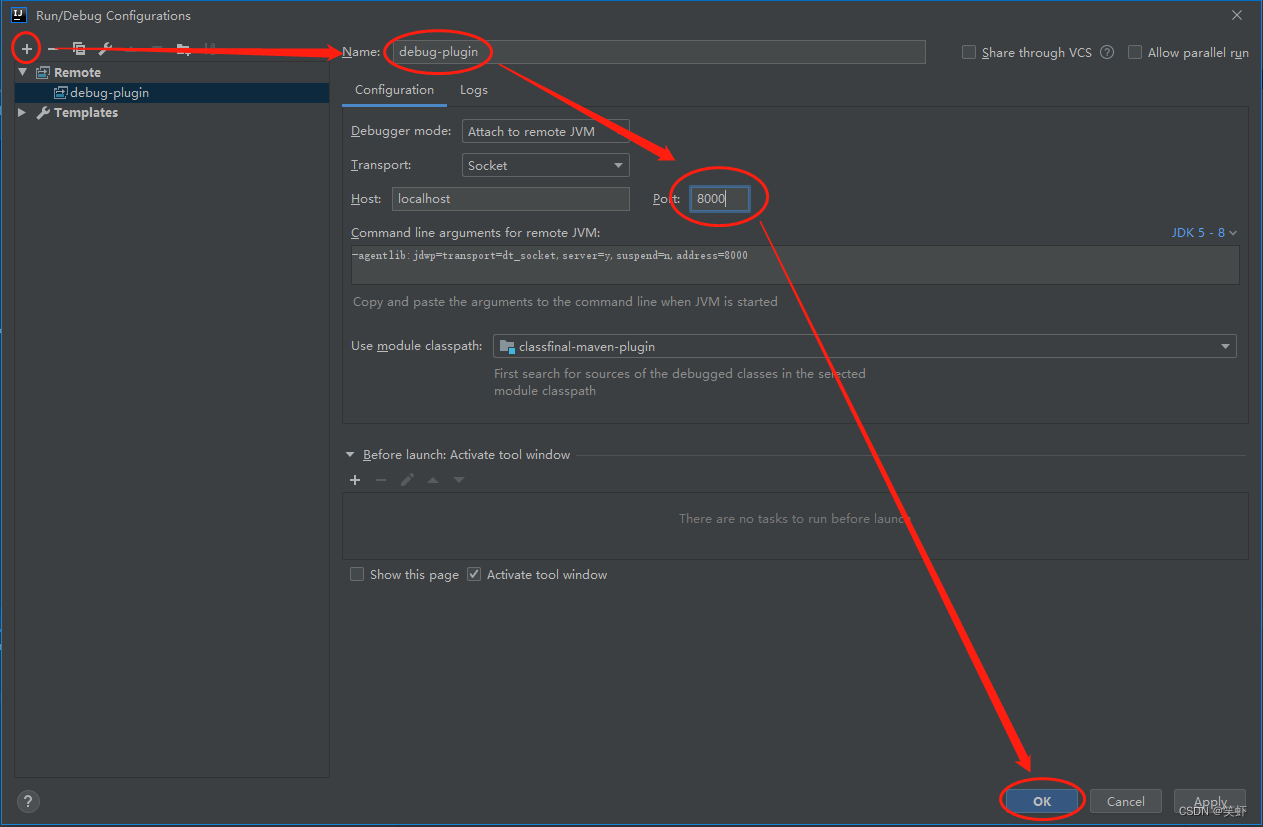
Intellij IDEA 中调试 maven 插件
Intellij IDEA 中调试 maven 插件话痨一下步骤1. classfinal-demo 项目部分2. ClassFinal 部分参考资料话痨一下 目前有两个项目: ClassFinal 是一款java class文件安全加密工具。classfinal-demo 是我建的一个Demo,用来测试ClassFinal的加密效果。 目…...
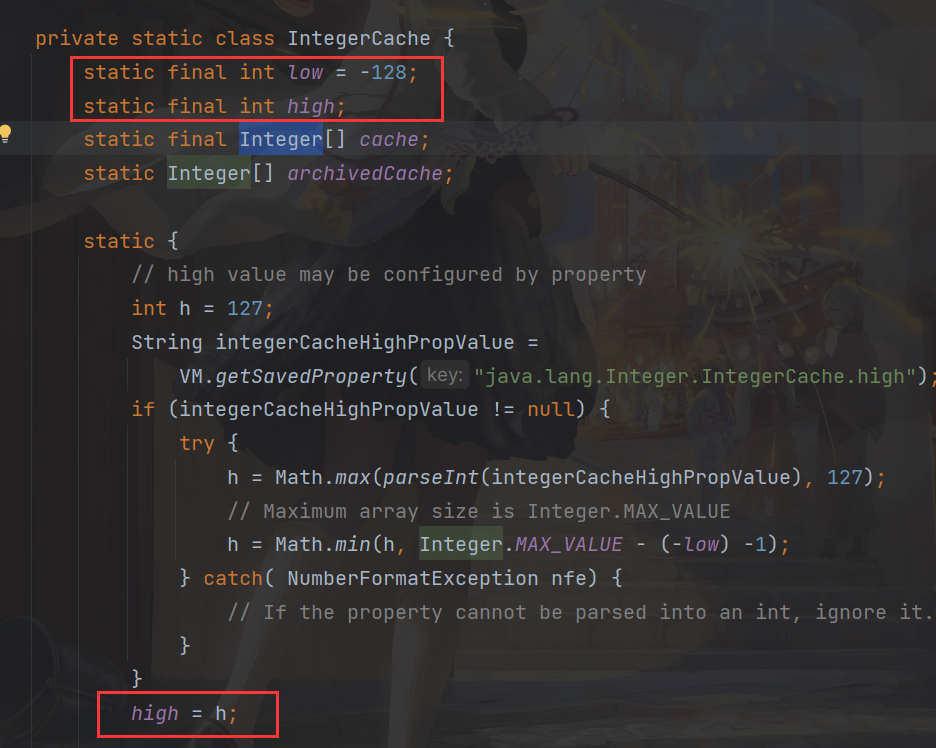
Java全栈知识(1)缓存池
我们先看这么一道题 Integer x new Integer(123); Integer y new Integer(123); System.out.println(x y); // false Integer z 123; Integer k 123; System.out.println(z k); // true Integer a 200; Integer b 200; System.out.println(z k); //false 我们…...
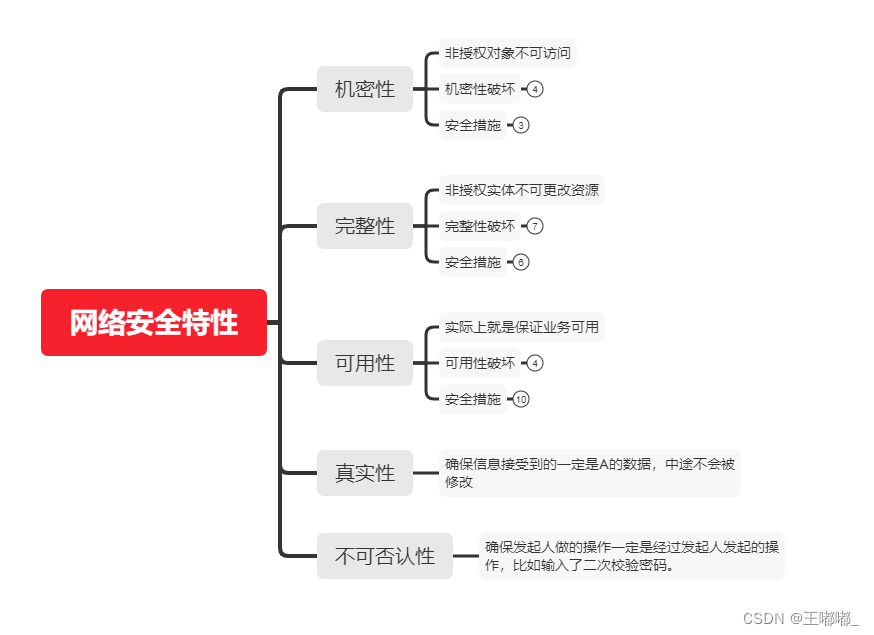
网络安全的特性
0x00 前言 网络安全的特性包括,机密性,完整性,可用性,真实性和不可否认性。详细的内容可以参考如下的内容。 Xmind资源请下载~ 0x01 机密性 机密性(Confidentiality) 意味着阻止未经授权的实体&#x…...
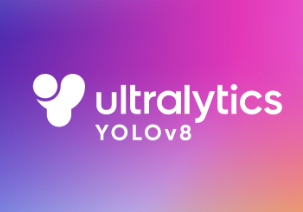
YOLOv8 多目标跟踪
文章大纲 简介环境搭建代码样例跟踪原理代码分析原始老版实现新版本封装代码实现追踪与计数奇奇怪怪错误汇总lap 安装过程报错推理过程报错参考文献与学习路径简介 使用yolov8 做多目标跟踪 文档地址: https://docs.ultralytics.com/modes/track/https://github.com/ultralyt…...
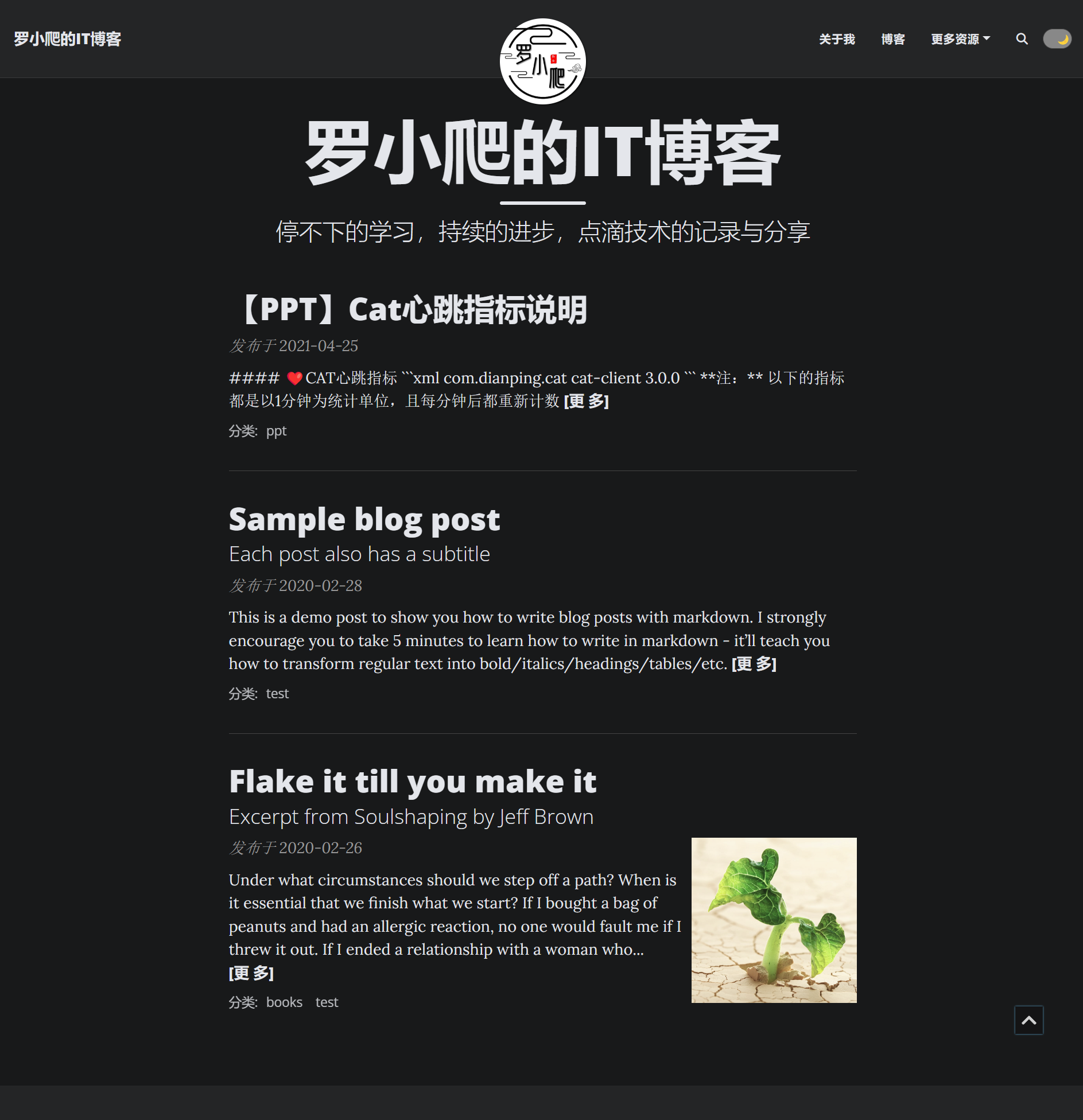
Gitee搭建个人博客(Beautiful Jekyll)
目录一、引言二、博客模板选型 - Jekyll三、安装Jekyll环境3.1 安装Ruby3.2 安装Jekyll3.3 下载Jekyll主题四、搭建我的Gitee博客4.1 选择主题 - Beautiful Jekyll4.2 创建Gitee账号同名代码库4.3 写博客4.4 开通Gitee Pages服务五、对Beautifu Jekyll的相关优化一、引言 之前…...

图形视图框架 事件处理(item)
在图形界面框架中的事件都是先由视图进行接收,然后传递给场景,再由场景传递给图形项。通过键盘处理的话,需要设置焦点,在QGraphicsScene中使用setFoucesItem()函数可以设置焦点,或者图形项使用s…...
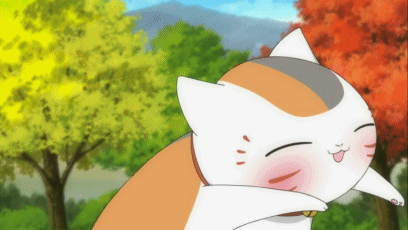
PTA第六章作业详解
🚀write in front🚀 📝个人主页:认真写博客的夏目浅石. 🎁欢迎各位→点赞👍 收藏⭐️ 留言📝 📣系列专栏:夏目的作业 💬总结:希望你看完之后&am…...
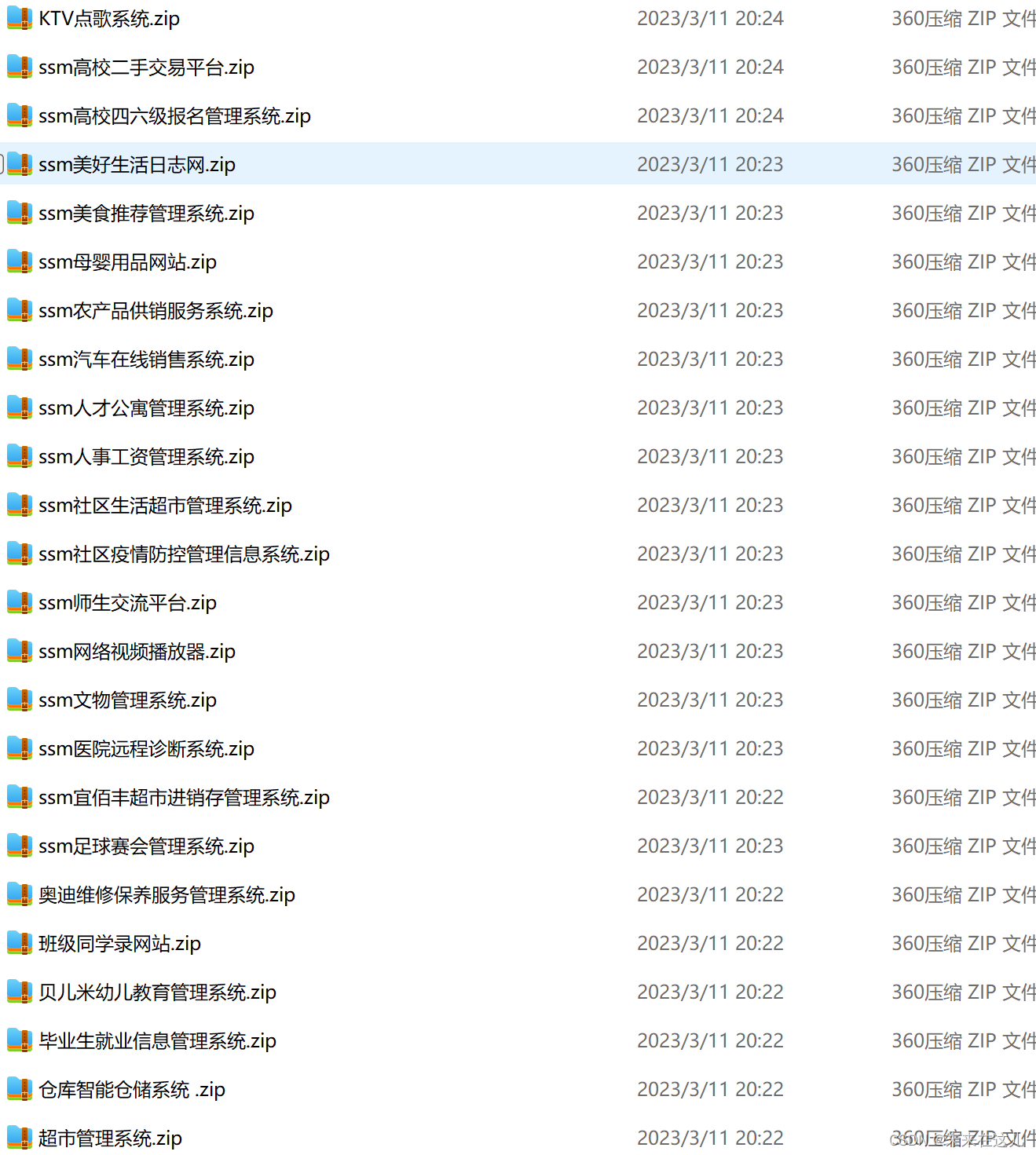
Java课程设计项目--音乐视频网站系统
一、功能介绍 随着社会的快速发展,计算机的影响是全面且深入的。人们生活水平的不断提高,日常生活中人们对音乐方面的要求也在不断提高,听歌的人数更是不断增加,使得音乐网站的设计的开发成为必需而且紧迫的事情。音乐网站的设计主…...
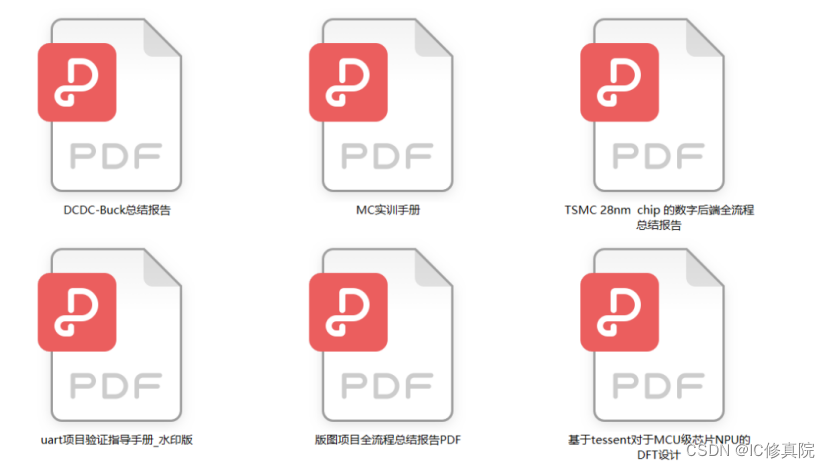
FPGA可以转IC设计吗?需要学习哪些技能?
曾经在知乎上看到一个回答“入职做FPGA,后续是否还可以转数字IC设计?” 从下面图内薪资就可以对比出来,对比FPGA的行业薪资水平,IC行业中的一些基础性岗位薪资比很多FPGA大多数岗位薪资都要高。 除了薪资之外更多FPGA转IC设计的有…...
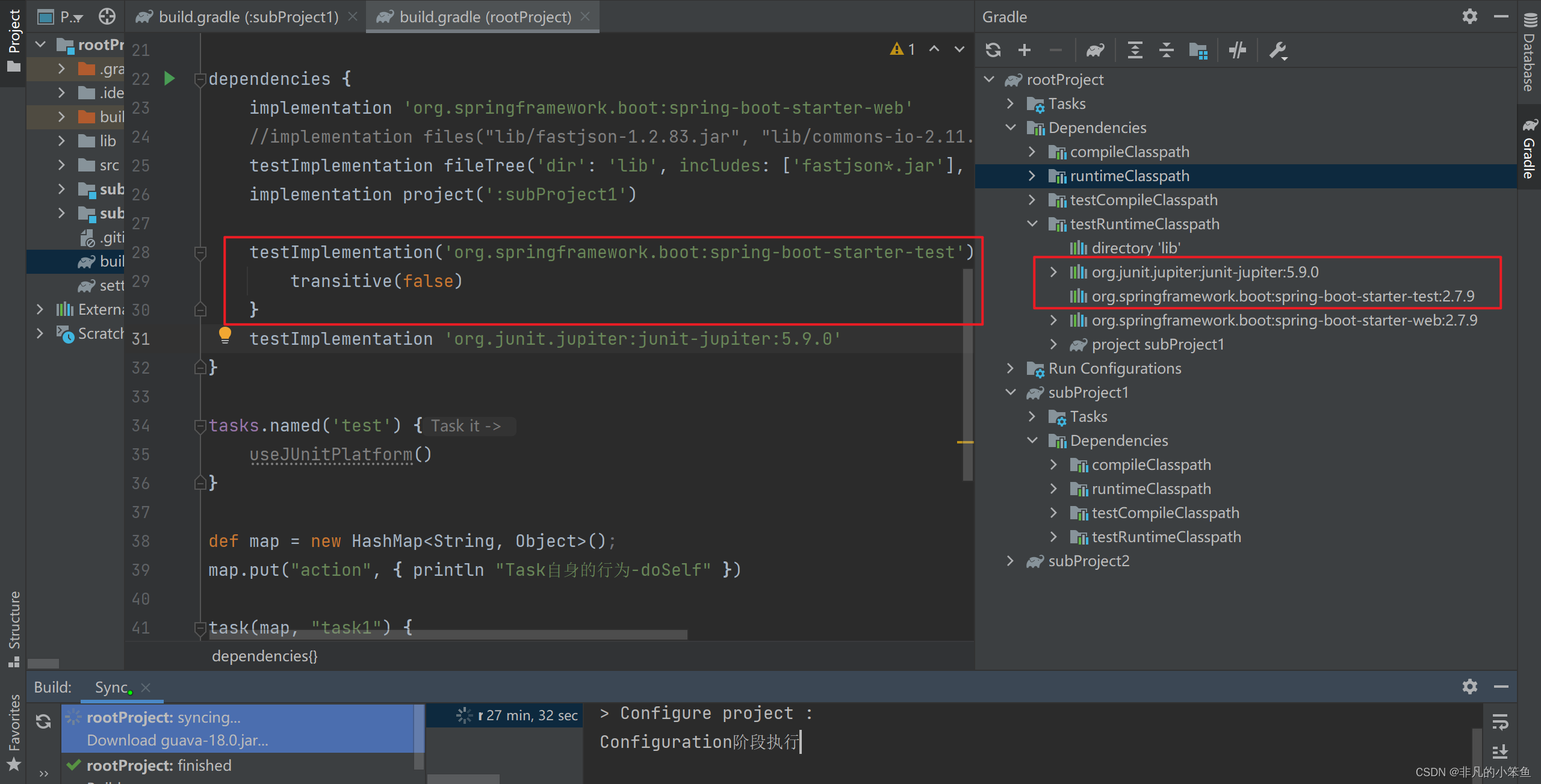
初探Gradle
目录一.概述二.优点三.安装与配置1. 官网下载2. 配置环境变量3. 检验4. 配置国内镜像(可选)5. IDEA配置三.工程结构四.生命周期1.Initialization阶段2.Configuration阶段3.Execution阶段五.Task六.常用任务指令七.引入依赖1.本地依赖2.项目依赖3.直接依赖八.依赖类型九.插件十.…...

国产数据库介绍
人大金仓 Kingbase 北京人大金仓信息技术股份有限公司于1999年由中共人民大学专家创立,自成立以来,始终立足自主研发,专注数据管理领域,先后承担了国家“863”、“核高基”等重大专项,研发出了具有国际先进水平的大型…...

Java OpenJudge-test3
目录 1:明明的随机数 2:合影效果 3:不重复的单词 4:和为给定数 5:字符串数组排序问题 6:字符串排序 7:求序列中的众数 1:明明的随机数 总时间限制: 1000ms 内存限制: 65536kB 描述 明明想在学校中请一些同学一起做一项问卷调查,为了实验的客观性ÿ…...
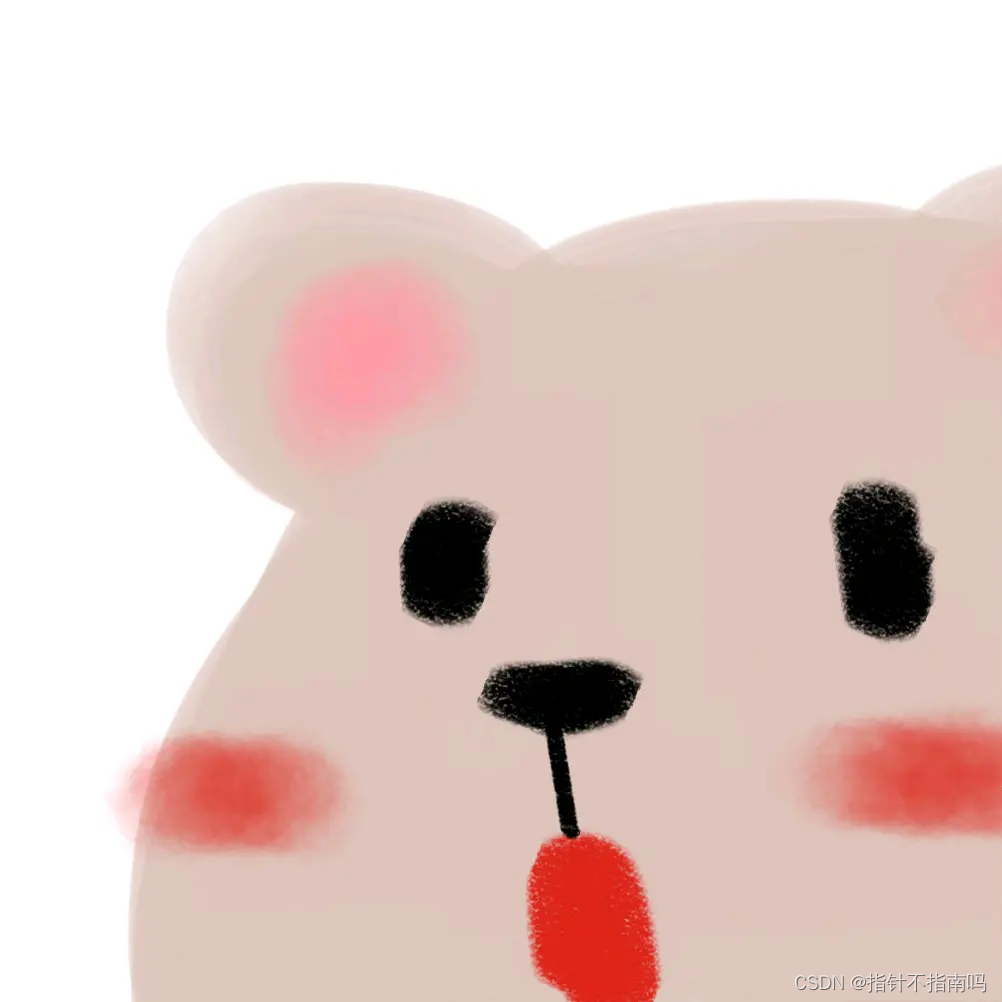
蓝桥杯刷题冲刺 | 倒计时22天
作者:指针不指南吗 专栏:蓝桥杯倒计时冲刺 🐾马上就要蓝桥杯了,最后的这几天尤为重要,不可懈怠哦🐾 文章目录1.选数异或2.特殊年份1.选数异或 题目 链接: 选数异或 - 蓝桥云课 (lanqiao.cn) 给定…...
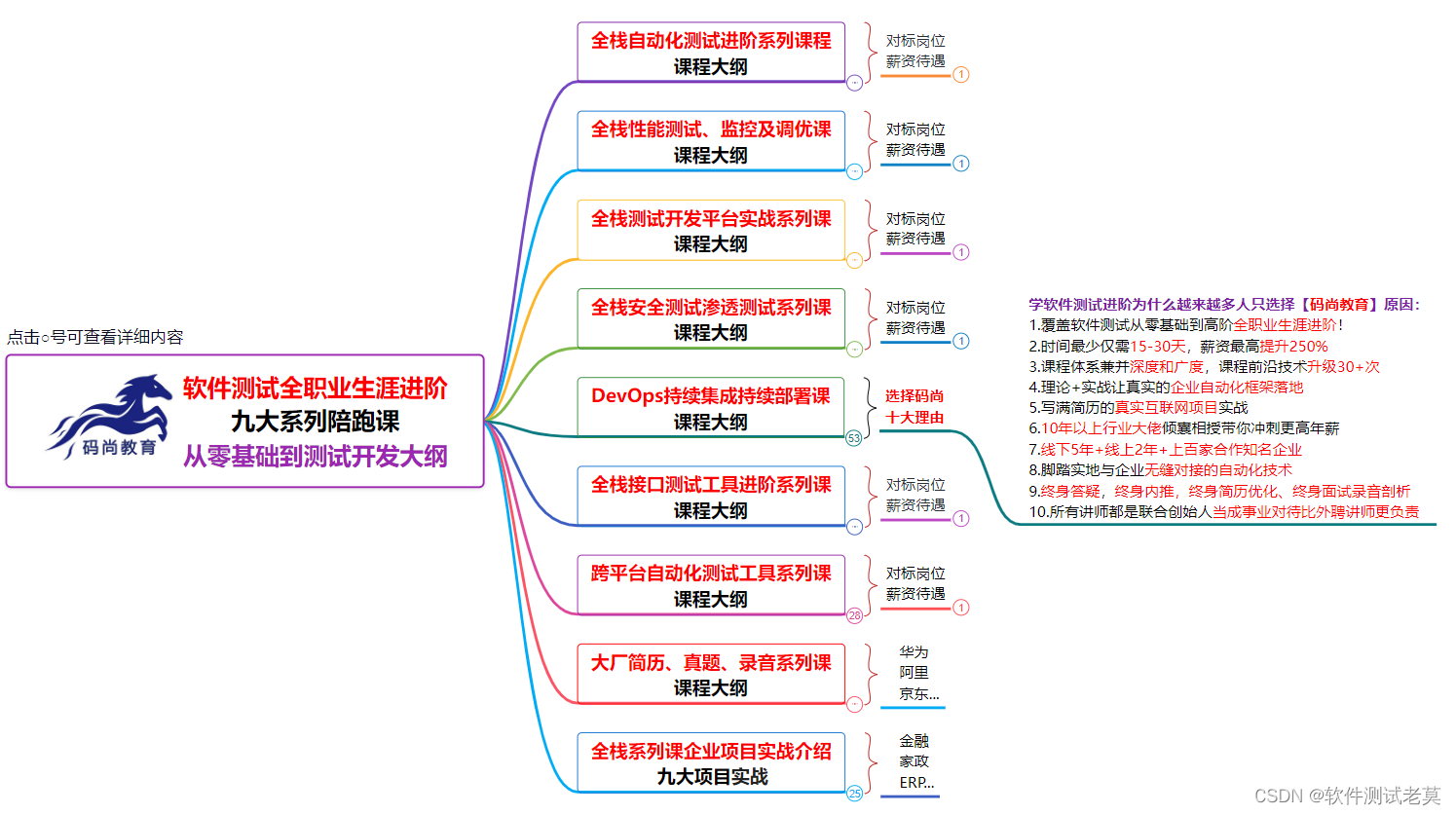
入行 5年,跳槽 3次,我终于摸透了软件测试这行(来自过来人的忠告)
目录 前言 第一年 第二年 第三年 第四年 作为过来人的一些忠告 前言 最近几年行业在如火如荼的发展壮大,以及其他传统公司都需要大批量的软件测试人员,但是20年的疫情导致大规模裁员,让人觉得行业寒冬已来,软件测试人员的职…...

开源时序数据库学习
计划学习使用QuestDB解决大数据日志存储场景。以下是常见引擎比较 比较项目 InfluxDB TimescaleDB OpenTSDB QuestDB 数据模型 Key-Value Relational Key-Value Relational 存储引擎 自主开发的TSI PostgreSQL扩展程序 Apache HBase 自主开发 查询语言 InfluxQ…...
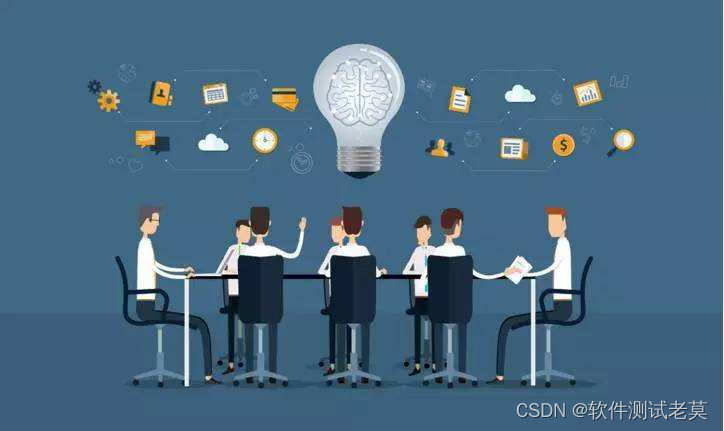
字节测试工程师悄悄告诉我的软件测试、测试开发常用的测试策略与测试手段
目录 前言 测试策略的关注重点 测试策略主要内容 总体测试策略 初级版本测试策略 跟踪测试执行 版本质量评估 后续版本测试策略 发布质量评估 测试手段 前言 测试策略是指在特定环境约束之下,描述软件开发周期中关于测试原则、方法、方式的纲要ÿ…...

我常用的shell 进制转换工具
一、进制的一些基础知识 1. 二进制(binary) 二进制的取值是0和1; 前缀是 0b 2. 八进制(Octal) 八进制的取值是0-7;前缀是 O 3. 十进制(decimal) 十进制的取值是0-9;没有前缀 …...
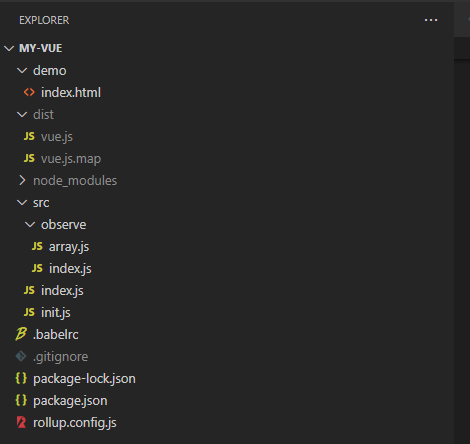
手写vue(二)响应式实现
名词解释:vm:指Vue实例一、目标效果vue定义(1)新建vm时,可以通过一个data对象,或者data函数,其属性可以通过vm直接访问,而data对象可以通过vm._data获取(2)修…...

mysql数据库常问面试题
1、NOW()和CURRENT_DATE()有什么区别? NOW()命令用于显示当前年份,月份,日期,小时,分钟和秒。 CURRENT_DATE()仅显示当前年份,月份和日期。 2、CHAR和VARCHAR的区别? (1)…...
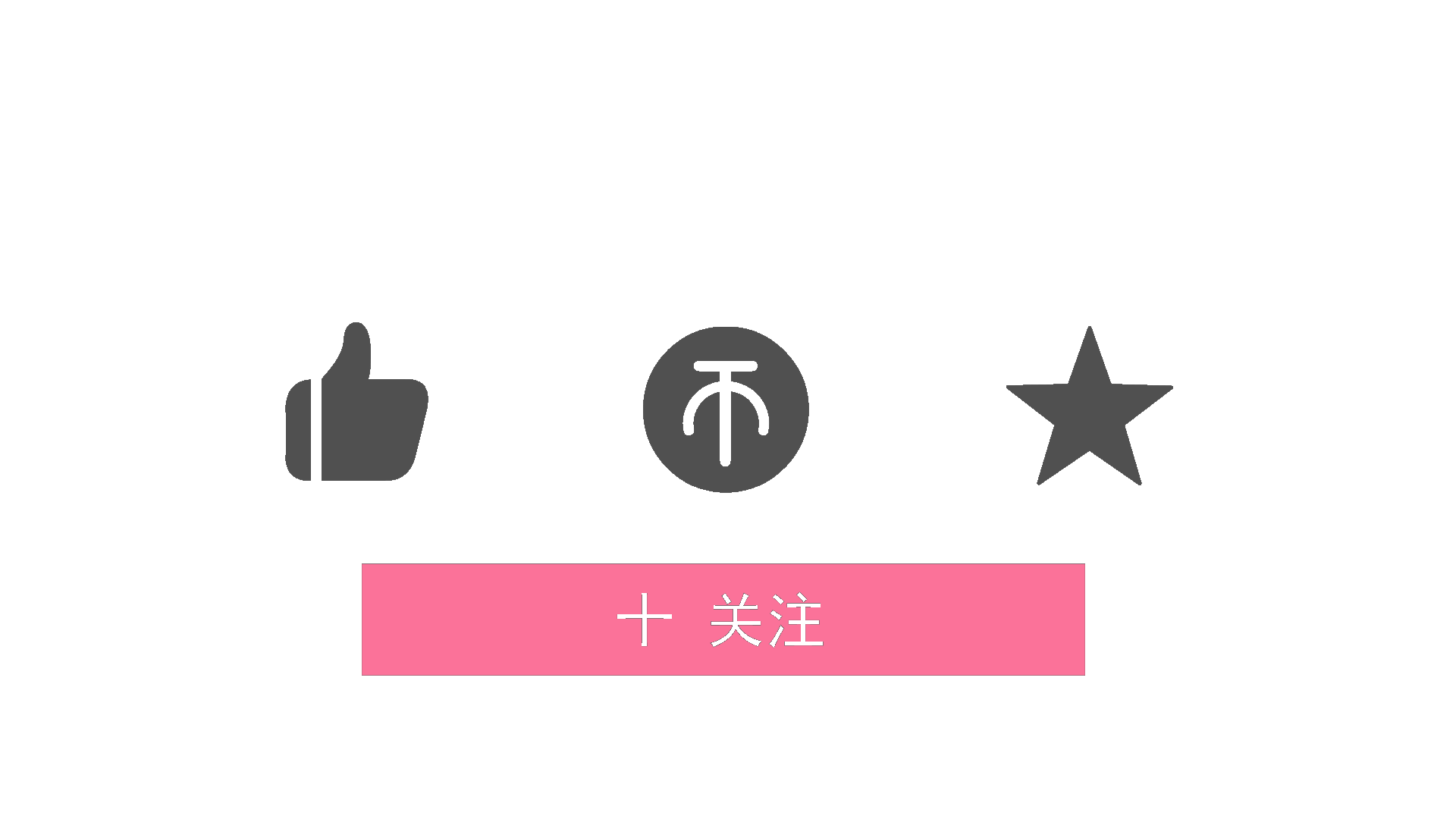
AI风暴 :文心一言 VS GPT-4
💗wei_shuo的个人主页 💫wei_shuo的学习社区 🌐Hello World ! 文心一言 VS GPT-4 文心一言:知识增强大语言模型百度全新一代知识增强大语言模型,文心大模型家族的新成员,能够与人对话互动&#…...
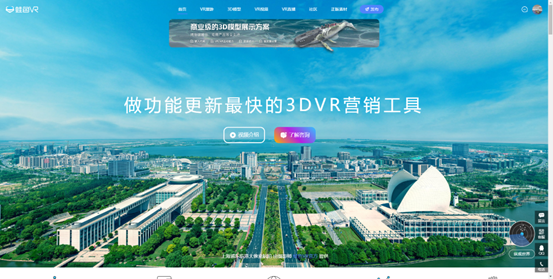
VR全景城市,用720全景树立城市形象,打造3D可视化智慧城市
随着城市化进程的加速,城市之间的竞争也日益激烈。城市管理者们需要寻求新的方式来提升城市的品牌形象和吸引力。在这个过程中,VR全景营销为城市提供了一种全新的营销手段,可以帮助提升城市的价值和吸引力。一、城市宣传新方式VR全景营销是一…...
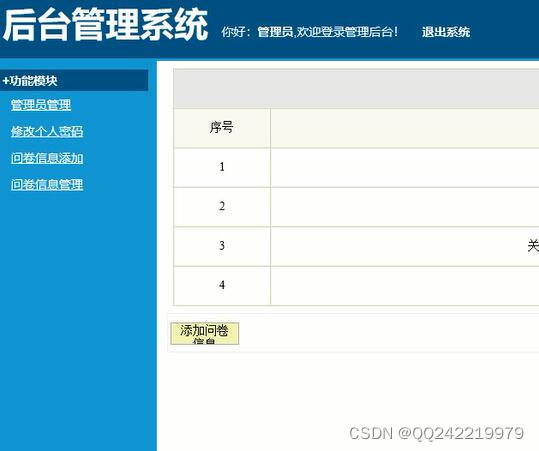
javaweb窗口服务人员分析评价系统ssh
A)后台管理员模块:通过该功能模块,管理员可以修改自己的密码,并对管理员进行添加和删除操作。 B)注册用户模块:通过该功能模块,管理员可以查看注册用户的基本信息,对存在问题的用户进…...
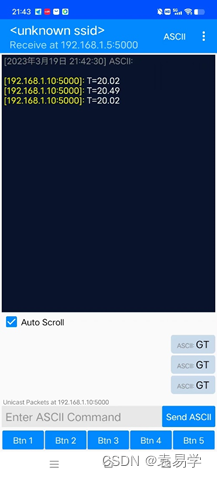
树莓派Pico W无线开发板UDP协议MicroPython网络编程实践
树莓派Pico W无线开发板(简称Pico W)是树莓派基金会于2022年6月底推出的搭载无线通信芯片的树莓派Pico开发板。本文在介绍树莓派Pico W无线开发板接口信号和TCP/IP和UDP通信协议基础上,给出Pico W无线开发板的UDP协议MicroPython网络编程实例…...
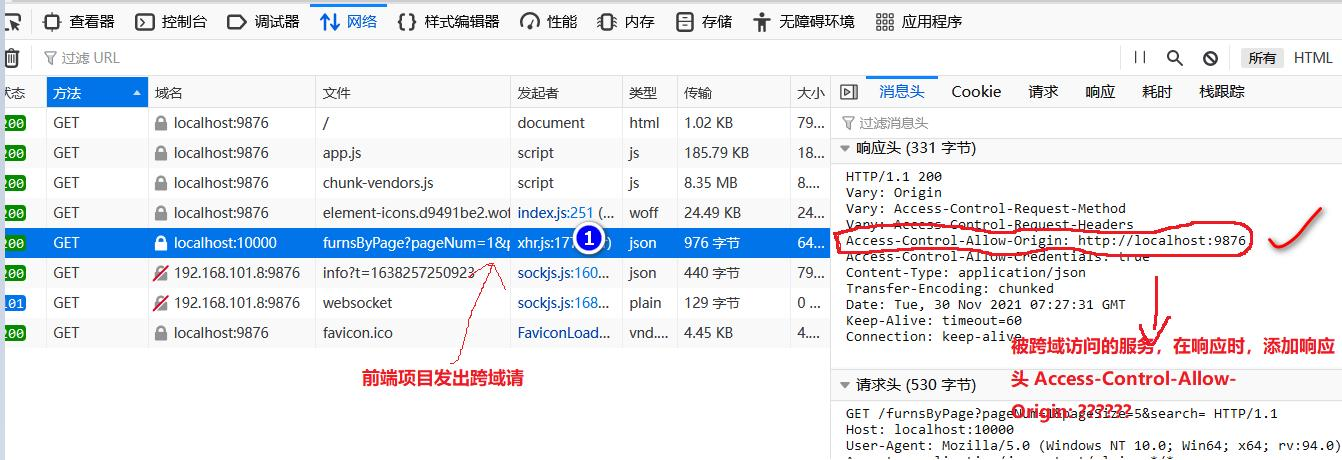
跨域解决方案
跨域解决方案 1.跨域基本介绍 文档:https://developer.mozilla.org/zh-CN/docs/Web/HTTP/CORS 跨域问题是什么? 一句话:跨域指的是浏览器不能执行其他网站的脚本,它是由浏览器的同源策略造成的,是浏览器对 javascr…...

springboot的统一处理
在处理网络请求时,有一部分功能是需要抽出来统一处理的,与业务隔开。 登录校验 可以利用spring mvc的拦截器Interceptor,实现HandlerInterceptor接口即可。实现该接口后,会在把请求发给Controller之前进行拦截处理。 拦截器的实…...
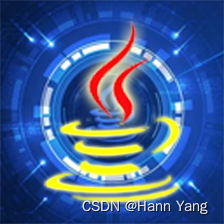
C/C++每日一练(20230319)
目录 1. 反转链表 II 🌟🌟 2. 解码方法 🌟🌟 3. 擅长编码的小k 🌟🌟🌟 🌟 每日一练刷题专栏 🌟 Golang每日一练 专栏 Python每日一练 专栏 C/C每日一练 专栏 …...
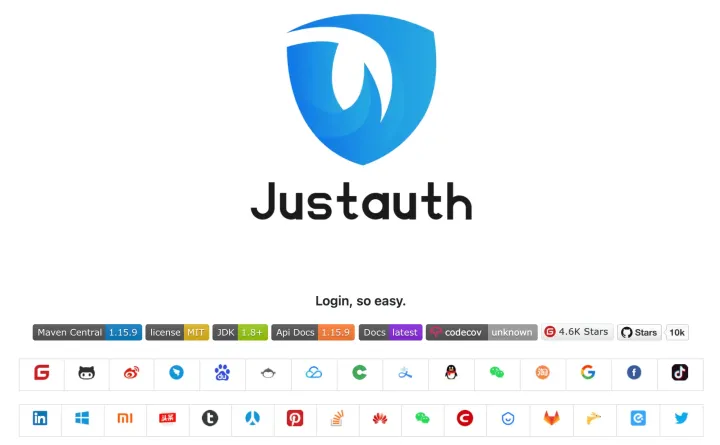
GitHub 上有些什么好玩的项目?
前言 各个领域模块的都整理了一下,包含游戏、一些沙雕的工具、实用正经的工具以及一些相关的电商项目,希望他们可以给你学习的路上增加几分的乐趣,我们直接进入正题~ 游戏 1.吃豆人 一款经典的游戏开发案例,包括地图绘制、玩家控…...