Java 基础知识九(网络编程)
UDP
DatagramSocket:通讯的数据管道
-send 和receive方法
-(可选,多网卡)绑定一个IP和Port
DatagramPacket
-集装箱:封装数据
-地址标签:目的地IP+Port
package org.example.net;import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;public class UdpRecv {public static void main(String[] args) throws Exception {DatagramSocket ds = new DatagramSocket(3000);byte[] buf = new byte[1024];DatagramPacket dp = new DatagramPacket(buf, 1024);System.out.println("UdpRecv:我在等待信息");ds.receive(dp);System.out.println("UdpRecv:我接收到信息");String strRecv = new String(dp.getData(), 0, dp.getLength()) + " from " + dp.getAddress().getHostAddress() + ":" + dp.getPort();System.out.println(strRecv);Thread.sleep(1000);System.out.println("UdpRecv:我要发送信息");String str = "hello world 222";DatagramPacket dp2 = new DatagramPacket(str.getBytes(), str.length(), InetAddress.getByName("127.0.0.1"), dp.getPort());ds.send(dp2);System.out.println("UdpRecv:我发送信息结束");ds.close();}
}
package org.example.net;import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;public class UdpSend {public static void main(String[] args) throws Exception {DatagramSocket ds = new DatagramSocket();String str = "hello world";DatagramPacket dp = new DatagramPacket(str.getBytes(), str.length(), InetAddress.getByName("127.0.0.1"), 3000);System.out.println("UdpSend:我要发送信息");ds.send(dp);System.out.println("UdpSend:我发送信息结束");Thread.sleep(1000);byte[] buf = new byte[1024];DatagramPacket dp2 = new DatagramPacket(buf, 1024);System.out.println("UdpSend:我在等待信息");ds.receive(dp2);System.out.println("UdpSend:我接收到信息");String str2 = new String(dp2.getData(), 0, dp2.getLength()) + " from " + dp2.getAddress().getHostAddress() + ":" + dp2.getPort();System.out.println(str2);ds.close();}
}
TCP
TCP协议:有链接、保证可靠的无误差通讯
-1)服务器:创建一个ServerSocket,等待连接
-2)客户机:创建一个Socket,连接到服务器-
3)服务器:ServerSocket接收到连接,创建一个Socket和客户的Socket建立专线连接,后续服务器和客户机的对话(这一对Sock会在一个单独的线程(服务器端)上运行
4)服务器的ServerSocket继续等待连接,返回1
ServerSocket: 服务器码头
需要绑定port
如果有多块网卡,需要绑定一个IP地址
Socket: 运输通道
-客户端需要绑定服务器的地址和Port
-客户端往Socket输入流写入数据,送到服务端-客户端从Socket输出流取服务器端过来的数据
-服务端反之亦然
服务端等待响应时,处于阻塞状态
服务端可以同时响应多个客户端
服务端每接受一个客户端,就启动一个独立的线程与之对应
客户端或者服务端都可以选择关闭这对Socket的通道
实例
-服务端先启动,且一直保留
-客户端后启动,可以先退出
package org.example.net;import java.io.*;
import java.net.InetAddress;
import java.net.Socket;public class TcpClient {public static void main(String[] args) {try {Socket s = new Socket(InetAddress.getByName("127.0.0.1"),8001);//需要服务端先开启//同一个通道,服务端的输出流就是客户端的输入流;服务端的输入流就是客户端的输出流// 开启通道的输入流InputStream ips =s.getInputStream();BufferedReader brNet = new BufferedReader(new InputStreamReader(ips));OutputStream ops = s.getOutputStream();//开启通道的输出流DataOutputStream dos = new DataOutputStream(ops);BufferedReader brKey = new BufferedReader(new InputStreamReader(System.in));while (true) {String strWord = brKey.readLine();if (strWord.equalsIgnoreCase("quit")) {break;}else{System.out.println("I want to send:" + strWord);dos.writeBytes(strWord + System.getProperty("line.separator") );System.out.println("Server said :" + brNet.readLine());}}}catch (IOException e){e.printStackTrace();}}
}
package org.example.net;import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;public class TcpServer {public static void main(String[] args) {try {ServerSocket ss = new ServerSocket(8001);//驻守在8001端口Socket s = ss.accept();//阻寒,等到有客户端连接上来System.out.println("welcome to the java world");InputStream ips = s.getInputStream();//有人连上来,打开输入流OutputStream ops = s.getOutputStream();//打开输出流// 同一个通道,服务端的输出流就是客户端的输入流;服务端的输入流就是客户端的输出流ops.write("Hello,client!".getBytes()); //输出一句话给客户端BufferedReader br = new BufferedReader(new InputStreamReader(ips));//从客户端读取一句话System.out.println("client said:" + br.readLine());ips.close();ops.close();s.close();ss.close();}catch (IOException e){e.printStackTrace();}}
}
server2 处理多客户端
package org.example.net;import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;public class TcpServer2 {public static void main(String[] args) {try {ServerSocket ss = new ServerSocket(8001);//驻守在8001端口while (true){Socket s = ss.accept();//阻寒,等到有客户端连接上来System.out.println("a new client coming");new Thread(new Worker(s)).start();}}catch (IOException e){e.printStackTrace();}}
}
package org.example.net;import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;public class Worker implements Runnable {Socket s;public Worker(Socket s) {this.s = s;}@Overridepublic void run() {try {System.out.println("server has started");InputStream ips = s.getInputStream();//有人连上来,打开输入流OutputStream ops = s.getOutputStream();//打开输出流BufferedReader br = new BufferedReader(new InputStreamReader(ips));DataOutputStream dos = new DataOutputStream(ops);while (true) {String strWord = br.readLine();System.out.println("client said:" + strWord + ":" + strWord.length());if (strWord.equalsIgnoreCase("quit")) {break;}String strEcho = strWord + " echo";// dos.writeBytes(strWord + System.getProperty("line.separator"));System.out.println("Server said :" + strWord + "---->" + strEcho);dos.writeBytes(strWord + "---->" + strEcho + System.getProperty("line.separator"));}br.close();//关闭包装类,会自动关闭包装类中所包装过的底层类。所以不用调用ips.close()dos.close();s.close();} catch (Exception e) {e.printStackTrace();}}
}
HTTP
Java HTTP编程(java.net包)
-支持模拟成浏览器的方式去访问网页
-URL,Uniform Resource Locator,代表一个资源http://www.ecnu.edu.cn/index.html?a=1&b=2&c=3
-URLConnection
获取资源的连接器
根据URL的openConnection(方法获得URLConnection
connect方法,建立和资源的联系通道
getInputStream方法,获取资源的内容
package org.example.net;import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.URL;
import java.net.URLConnection;
import java.util.List;
import java.util.Map;public class URLConnectionGetTest {public static void main(String[] args) {try {String urlName = "https://www.baidu.com";URL url = new URL(urlName);URLConnection connection = url.openConnection();connection.connect();//打印http的头部信息Map<String, List<String>> headers = connection.getHeaderFields();for (Map.Entry<String, List<String>> entry : headers.entrySet()) {String key = entry.getKey();for (String value : entry.getValue()) {System.out.println(key + ":" + value);}}//输出将要收到的内容属性信息System.out.println("-----------------------");System.out.println("getcontentType:"+connection.getContentType());System.out.println("getcontentLength:"+ connection.getContentLength());System.out.println("getcontentEncoding:?"+ connection.getContentEncoding());System.out.println("getDate:"+ connection.getDate());System.out.println("getExpiration:"+ connection.getExpiration());System.out.println("getLastModifed:"+ connection.getLastModified());System.out.println("----------");BufferedReader br = new BufferedReader(new InputStreamReader(connection.getInputStream(),"UTF-8"));//输出收到的内容String line ="";while ((line= br.readLine())!= null){System.out.println(line);}} catch (Exception e) {e.printStackTrace();}}
}
package org.example.net;import java.io.*;
import java.net.*;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;public class URLConnectionPostTest {public static void main(String[] args) throws IOException {String urlstring = "https://tools.usps.com/go/zipLookupAction.action";Object userAgent = "HTTPie/0.9.2";Object redirects = "1";CookieHandler.setDefault(new CookieManager(null, CookiePolicy.ACCEPT_ALL));Map<String, String> params = new HashMap<String, String>();params.put("tAddress", "1 Market street");params.put("tcity", "San Francisco");params.put("sstate", "CA");String result = doPost(new URL(urlstring), params,userAgent == null ? null : userAgent.toString(),redirects == null ? -1 : Integer.parseInt(redirects.toString()));System.out.println(result);}public static String doPost(URL url, Map<String, String> nameValuePairs, String userAgent, int redirects) throws IOException {HttpURLConnection connection = (HttpURLConnection) url.openConnection();if (userAgent != null)connection.setRequestProperty("User-Agent", userAgent);if (redirects >= 0)connection.setInstanceFollowRedirects(false);connection.setDoOutput(true);//输出请求的参数try (PrintWriter out = new PrintWriter(connection.getOutputStream())) {boolean first = true;for (Map.Entry<String, String> pair : nameValuePairs.entrySet()) {//参数必须这样拼接a = 1 & b = 2 & c = 3if (first) {first = false;} else {out.print('&');}String name = pair.getKey();String value = pair.getValue();out.print(name);out.print('=');out.print(URLEncoder.encode(value, "UTF-8"));}}String encoding = connection.getContentEncoding();if (encoding == null){encoding = "UTF-8";}if (redirects > 0) {int responseCode = connection.getResponseCode();System.out.println("responsecode:" + responseCode);if (responseCode == HttpURLConnection.HTTP_MOVED_PERM|| responseCode == HttpURLConnection.HTTP_MOVED_TEMP|| responseCode == HttpURLConnection.HTTP_SEE_OTHER) {String location = connection.getHeaderField("Location");if (location != null) {URL base = connection.getURL();connection.disconnect();return doPost(new URL(base, location), nameValuePairs, userAgent, redirects - 1);}}} else if (redirects == 0) {throw new IOException("Too many redirects");}StringBuilder response = new StringBuilder();try (Scanner in = new Scanner(connection.getInputStream(),encoding)){while(in.hasNextLine()){response.append(in.nextLine());response.append("\n");}} catch (IOException e) {InputStream err = connection.getErrorStream();if (err == null) throw e;try (Scanner in = new Scanner(err)) {response.append(in.nextLine());response.append("\n");}}return response.toString();}
}
HTTPClient
java.net.http包
取代URLConnection 支持HTTP/1.1和HTTP/2 实现大部分HTTP方法主要类
-HttpClient -HttpRequest- HttpResponse
package org.example.net;import java.io.IOException;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;public class JDKHttpClientGetTest {public static void main(String[] args) throws IOException, InterruptedException {doGet();}public static void doGet() {try {HttpClient client = HttpClient.newHttpClient();HttpRequest request = HttpRequest.newBuilder(URI.create("http://www.baidu.com")).build();HttpResponse response = client.send(request, HttpResponse.BodyHandlers.ofString());System.out.println(response.body());} catch (Exception e) {e.printStackTrace();}}
}
Httpcomponents
hc.apache.org,Apache出品从HttpClient进化而来,是一个集成的Java HTIP工具包
-实现所有HTTP方法:get/post/put/delete
-支持自动转向
-支持https协议
-支持代理服务器等
NIO
·Buffer 缓冲区,一个可以读写的内存区域-ByteBuffer, CharBuffer, DoubleBuffer, IntBuffer, LongBufferShortBuffer (StringBuffer 不是Buffer缓冲区)
四个主要属性
capacity 容量,position 读写位置
limit 界限,mark标记,用于重复一个读/写操作
Channel 通道
全双工的,支持读/写(而Stream流是单向的)
-支持异步读写
-和Buffer配合,提高效率
-ServerSocketChannel 服务器TCP Socket 接入通道,接收客户端-SocketChannel TCP Socket通道,可支持阻寒/非阻塞通讯-DatagramChannel UDp 通道
-FileChannel 文件通道
elector多路选择器
-每隔一段时间,不断轮询注册在其上的Channel-如果有一个Channel有接入、读、写操作,就会被轮询出来进行后续IO操作
-根据SelectionKey可以获取相应的Channel,
-避免过多的线程
-SelectionKey四种类型
·OP_CONNECT
·OP_ACCEPT
·OP READ
·OP WRITE
package org.example.NIO;import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.SocketChannel;
import java.util.Iterator;
import java.util.Set;
import java.util.UUID;public class NioClient {public static void main(String[] args) {String host = "127.0.0.1";int port = 8001;Selector selector = null;SocketChannel socketchannel = null;try {selector = Selector.open();socketchannel = SocketChannel.open();socketchannel.configureBlocking(false);//非阻塞//如果直接连接成功,则注册到多路复用器上,发送请求消息,读应答if (socketchannel.connect(new InetSocketAddress(host, port))) {socketchannel.register(selector, SelectionKey.OP_READ);doWrite(socketchannel);} else {socketchannel.register(selector, SelectionKey.OP_CONNECT);}} catch (IOException e) {e.printStackTrace();}while (true) {try {selector.select(1000);Set<SelectionKey> selectedKeys = selector.selectedKeys();Iterator<SelectionKey> it = selectedKeys.iterator();SelectionKey key = null;while (it.hasNext()) {key = it.next();it.remove();try {handleInput(selector, key);} catch (Exception e) {if (key != null) {key.cancel();if (key.channel() != null)key.channel().close();}}}} catch (Exception ex) {ex.printStackTrace();}}}public static void doWrite(SocketChannel sc)throws IOException {byte[] str = UUID.randomUUID().toString().getBytes();ByteBuffer writeBuffer = ByteBuffer.allocate(str.length);writeBuffer.put(str);writeBuffer.flip();sc.write(writeBuffer);}public static void handleInput(Selector selector, SelectionKey key) throws IOException, InterruptedException {if(key.isValid()) {//判断是否连接成功SocketChannel sc = (SocketChannel) key.channel();if (key.isConnectable()) {if (sc.finishConnect()) {sc.register(selector, SelectionKey.OP_READ);}}if (key.isReadable()) {ByteBuffer readBuffer = ByteBuffer.allocate(1024);int readBytes = sc.read(readBuffer);if (readBytes > 0) {readBuffer.flip();byte[] bytes = new byte[readBuffer.remaining()];readBuffer.get(bytes);String body = new String(bytes, "UTF-8");System.out.println("server said :" + body);} else if (readBytes < 0) {//对端链路关闭key.cancel();sc.close();} else; // 读到字节,忽略}Thread.sleep(5000);doWrite(sc);}}
}
package org.example.NIO;import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.util.Iterator;
import java.util.Set;public class NioServer {public static void main(String[] args) throws IOException {int port = 8001;Selector selector = null;ServerSocketChannel servChannel = null;try {selector = selector.open();servChannel = ServerSocketChannel.open();servChannel.configureBlocking(false);servChannel.socket().bind(new InetSocketAddress(port), 1024);servChannel.register(selector, SelectionKey.OP_ACCEPT);System.out.println("服务器在8001端口守候");} catch (IOException e) {e.printStackTrace();System.exit(1);}while (true) {try {selector.select(1000);Set<SelectionKey> selectedKeys = selector.selectedKeys();Iterator<SelectionKey> it = selectedKeys.iterator();SelectionKey key = null;while (it.hasNext()) {key = it.next();it.remove();try {handleInput(selector, key);} catch (Exception e) {if (key != null) {key.cancel();if (key.channel() != null)key.channel().close();}}}} catch (Exception ex) {ex.printStackTrace();}try {Thread.sleep(500);} catch (Exception ex) {ex.printStackTrace();}}}public static void handleInput(Selector selector, SelectionKey key) throws IOException {if (key.isValid()) {//判断是否连接成功if (key.isAcceptable()) {ServerSocketChannel ssc = (ServerSocketChannel) key.channel();SocketChannel sc = ssc.accept();sc.configureBlocking(false);sc.register(selector, SelectionKey.OP_READ);}if (key.isReadable()) {SocketChannel sc = (SocketChannel) key.channel();ByteBuffer readBuffer = ByteBuffer.allocate(1024);int readBytes = sc.read(readBuffer);if (readBytes > 0) {readBuffer.flip();byte[] bytes = new byte[readBuffer.remaining()];readBuffer.get(bytes);String request = new String(bytes, "UTF-8");System.out.println("server said :" + request);String response = request + " 666";doWrite(sc,response);} else if (readBytes < 0) {//对端链路关闭key.cancel();sc.close();} else; // 读到字节,忽略}}}public static void doWrite(SocketChannel channel,String response) throws IOException {if (response != null && response.trim().length() > 0) {byte[] bytes = response.getBytes();ByteBuffer writeBuffer = ByteBuffer.allocate(bytes.length);writeBuffer.put(bytes);writeBuffer.flip();channel.write(writeBuffer);}}
}
AIO
package org.example.Aio;import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.CharBuffer;
import java.nio.channels.AsynchronousSocketChannel;
import java.nio.channels.CompletionHandler;
import java.nio.charset.Charset;
import java.nio.charset.CharsetDecoder;
import java.util.UUID;public class AioClient {public static void main(String[] a){try {AsynchronousSocketChannel channel = AsynchronousSocketChannel.open();channel.connect(new InetSocketAddress("localhost", 8001), null, new CompletionHandler<Void, Void>() {public void completed(Void result, Void attachment){String str = UUID.randomUUID().toString();channel.write(ByteBuffer.wrap(str.getBytes()), null, new CompletionHandler<Integer, Object>() {@Overridepublic void completed(Integer result, Object attachment){try {System.out.println("write " +str + " , and wait response");ByteBuffer buffer = ByteBuffer.allocate(1024);channel.read(buffer, buffer, new CompletionHandler<Integer, ByteBuffer>() {@Overridepublic void completed(Integer result_num, ByteBuffer attachment) {attachment.flip();CharBuffer charBuffer = CharBuffer.allocate(1024);CharsetDecoder decoder = Charset.defaultCharset().newDecoder();decoder.decode(attachment, charBuffer, false);charBuffer.flip();String data = new String(charBuffer.array(), 0, charBuffer.limit());System.out.println("server said: " + data);try {channel.close();}catch (Exception e){e.printStackTrace();}}@Overridepublic void failed(Throwable exc, ByteBuffer attachment) {System.out.println("read error "+ exc.getMessage());}});channel.close();}catch (Exception e){e.printStackTrace();}}public void failed(Throwable exc, Object attachment){System.out.println("write error ");}});}public void failed(Throwable exc, Void attachment){System.out.println("faild ");}});Thread.sleep(10000);}catch (Exception e){e.printStackTrace();}}
}
package org.example.Aio;import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.CharBuffer;
import java.nio.channels.AsynchronousByteChannel;
import java.nio.channels.AsynchronousServerSocketChannel;
import java.nio.channels.AsynchronousSocketChannel;
import java.nio.channels.CompletionHandler;
import java.nio.charset.Charset;
import java.nio.charset.CharsetDecoder;public class AioServer {public static void main(String[] args) throws IOException {AsynchronousServerSocketChannel server = AsynchronousServerSocketChannel.open();server.bind(new InetSocketAddress("localhost", 8001));System.out.println(" server is witing at port 8001");server.accept(null, new CompletionHandler<AsynchronousSocketChannel, Object>() {@Overridepublic void completed(AsynchronousSocketChannel channel, Object attchment){server.accept(null, this);ByteBuffer buffer = ByteBuffer.allocate(1024);channel.read(buffer, buffer, new CompletionHandler<Integer, ByteBuffer>() {@Overridepublic void completed(Integer result_num, ByteBuffer attachment) {attachment.flip();CharBuffer charBuffer = CharBuffer.allocate(1024);CharsetDecoder decoder = Charset.defaultCharset().newDecoder();decoder.decode(attachment, charBuffer, false);charBuffer.flip();String data = new String(charBuffer.array(),0,charBuffer.limit());System.out.println("client said: "+data);channel.write(ByteBuffer.wrap((data + " 666").getBytes()));try {channel.close();}catch (Exception e){e.printStackTrace();}};@Overridepublic void failed(Throwable exc, ByteBuffer attachment) {System.out.println("read error "+ exc.getMessage());}});}public void failed(Throwable exc, Object attachment){System.out.println("failed "+ exc.getMessage());}});while (true){try {Thread.sleep(5000);}catch (InterruptedException e){e.printStackTrace();}}}}
相关文章:
Java 基础知识九(网络编程)
UDP DatagramSocket:通讯的数据管道 -send 和receive方法 -(可选,多网卡)绑定一个IP和Port DatagramPacket -集装箱:封装数据 -地址标签:目的地IPPort package org.example.net;import java.net.DatagramPacket; import java.net.DatagramSocket; import java.n…...
深入解析Go语言的类型方法、接口与反射
解锁Python编程的无限可能:《奇妙的Python》带你漫游代码世界 Go语言作为一门现代编程语言,以其简洁高效的特性受到广大开发者的喜爱。在本文中,我们将深入探讨Go语言中的类型方法、接口和反射机制。通过丰富的代码示例和详尽的解释,帮助您全面理解这些关键概念,并在实际…...
C#中线程池【异步】
在 WinForm 项目中,线程池中的线程主要用于执行异步和并发任务。当你调用某些异步方法或使用并行编程时,线程池中的线程就会被使用。 在以下场景中,线程池的线程会被使用: 使用场景 异步任务执行 当你使用 Task.Run() 或 TaskF…...
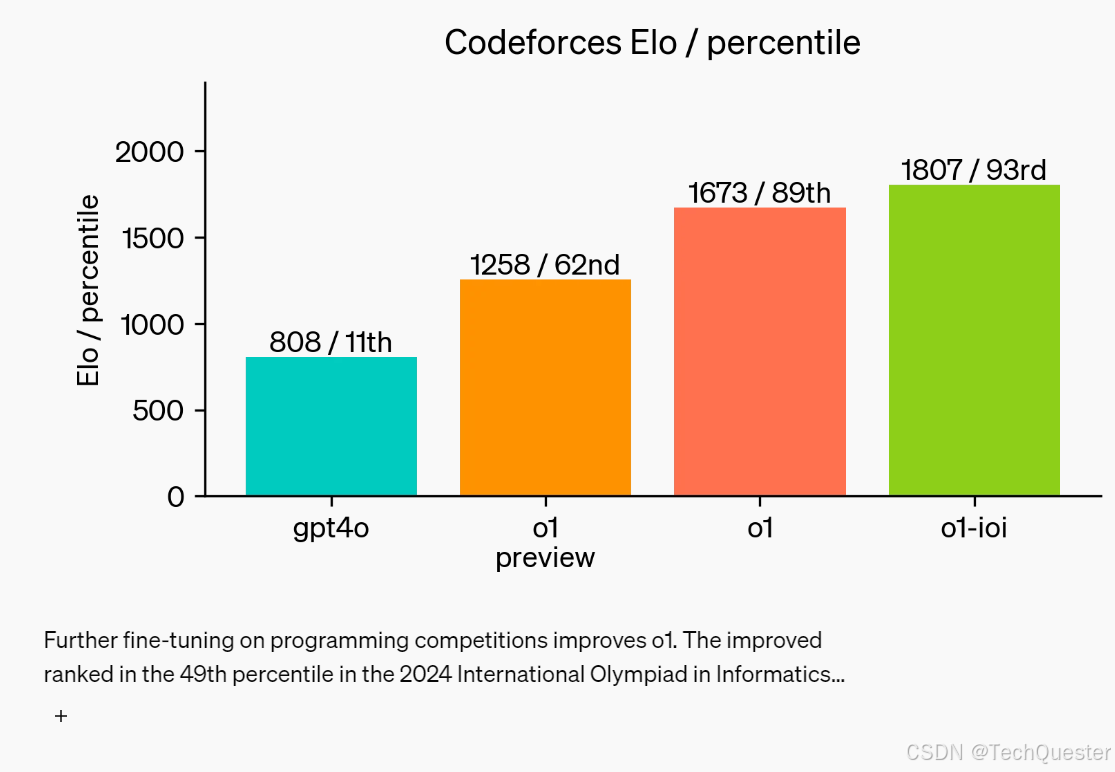
OpenAI 刚刚推出 o1 大模型!!突破LLM极限
北京时间 9 月 13 日午夜,OpenAI 正式发布了一系列全新的 AI 大模型,专门用于应对复杂问题。 这一新模型的出现代表了一个重要突破,其具备的复杂推理能力远远超过了以往用于科学、代码和数学等领域的通用模型,能够解决比之前更难的…...
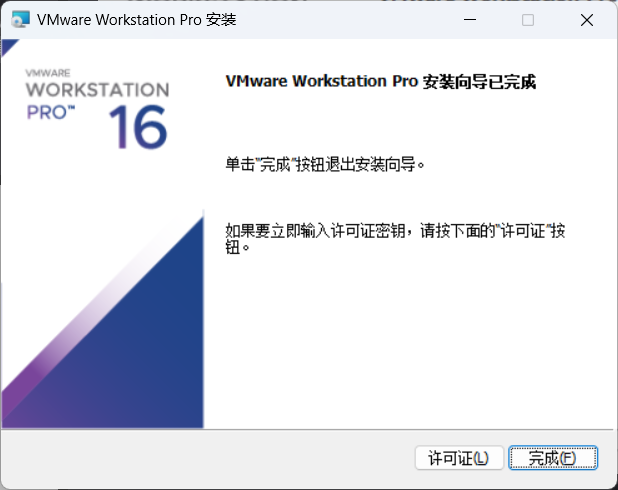
【Vmware16安装教程】
📖Vmware16安装教程 ✅1.下载✅2.安装 ✅1.下载 官网地址:https://www.vmware.com/ 百度云盘:Vmware16下载 123云盘:Vmware16下载 ✅2.安装 1.双击安装包VMware-workstation-full-16.1.0-LinuxProbe.Com.exe,点击…...
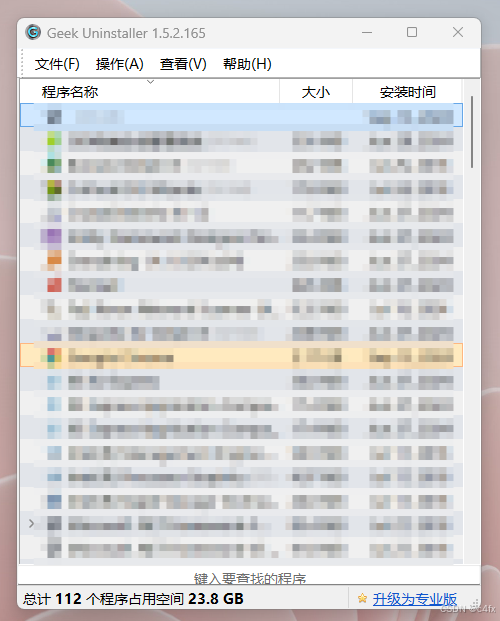
Delphi5利用DLL实现窗体的重用
文章目录 效果图参考利用DLL实现窗体的重用步骤1 设计出理想窗体步骤2 编写一个用户输出的函数或过程,在其中对窗体进行创建使它实例化步骤3 对工程文件进行相应的修改以适应DLL格式的需要步骤4 编译工程文件生成DLL文件步骤5 在需要该窗体的其他应用程序中重用该窗…...
使用JavaWeb开发注册功能时,校验用户名是否已存在的一个思路(附代码)
在开发 Web 应用程序时,用户注册是一个常见的功能。为了确保每个用户都有一个唯一的用户名,我们需要在用户注册时检查数据库中是否已经存在该用户名。本文将详细介绍如何在 Servlet 中使用 JDBC 技术来检查用户名是否存在。 1. JDBC 简介 Java Databas…...
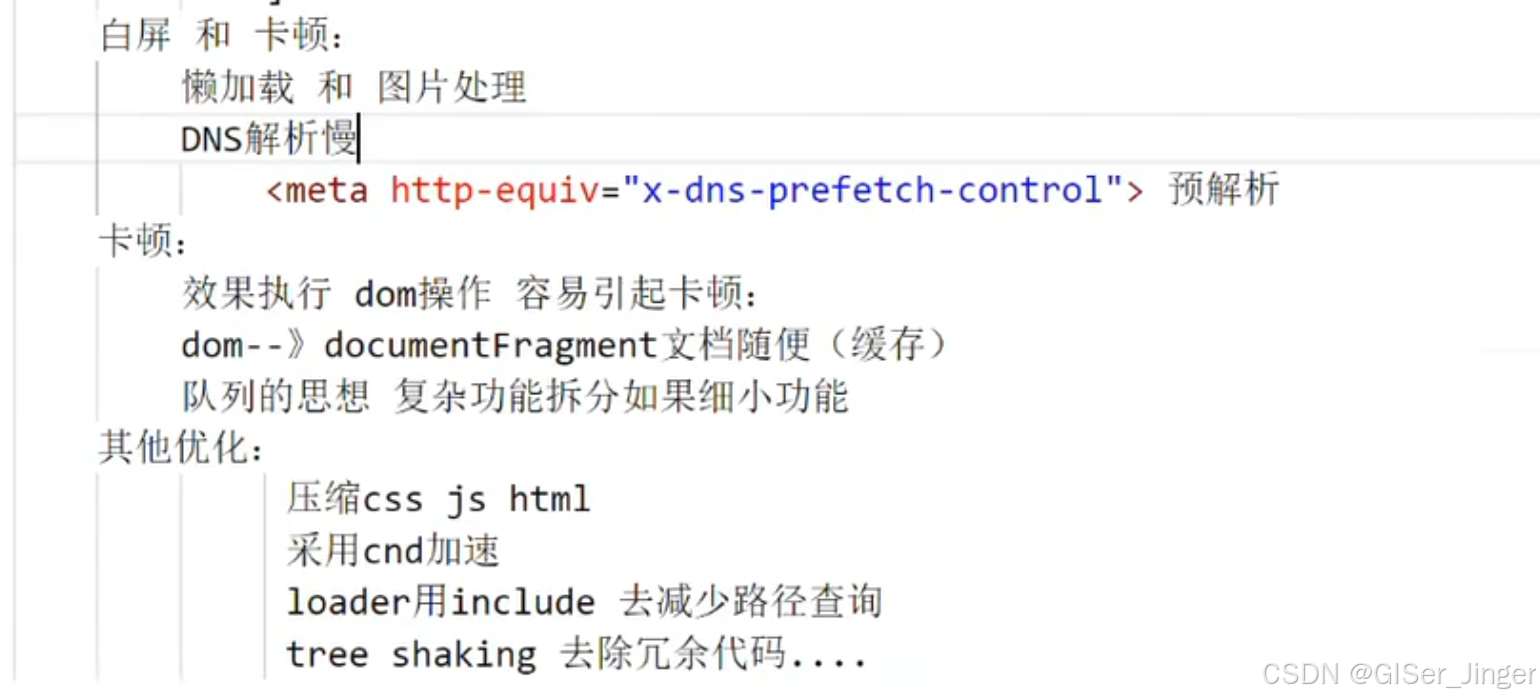
前端常见面试-首页性能提升、项目优化
首页性能提升 Vue 首页性能提升是Vue应用开发中非常重要的一环,它直接影响用户体验和应用的加载速度。以下是一些关键的Vue首页性能提升策略: 1. 代码分割与懒加载 路由懒加载:利用Webpack的动态导入(import())特性…...
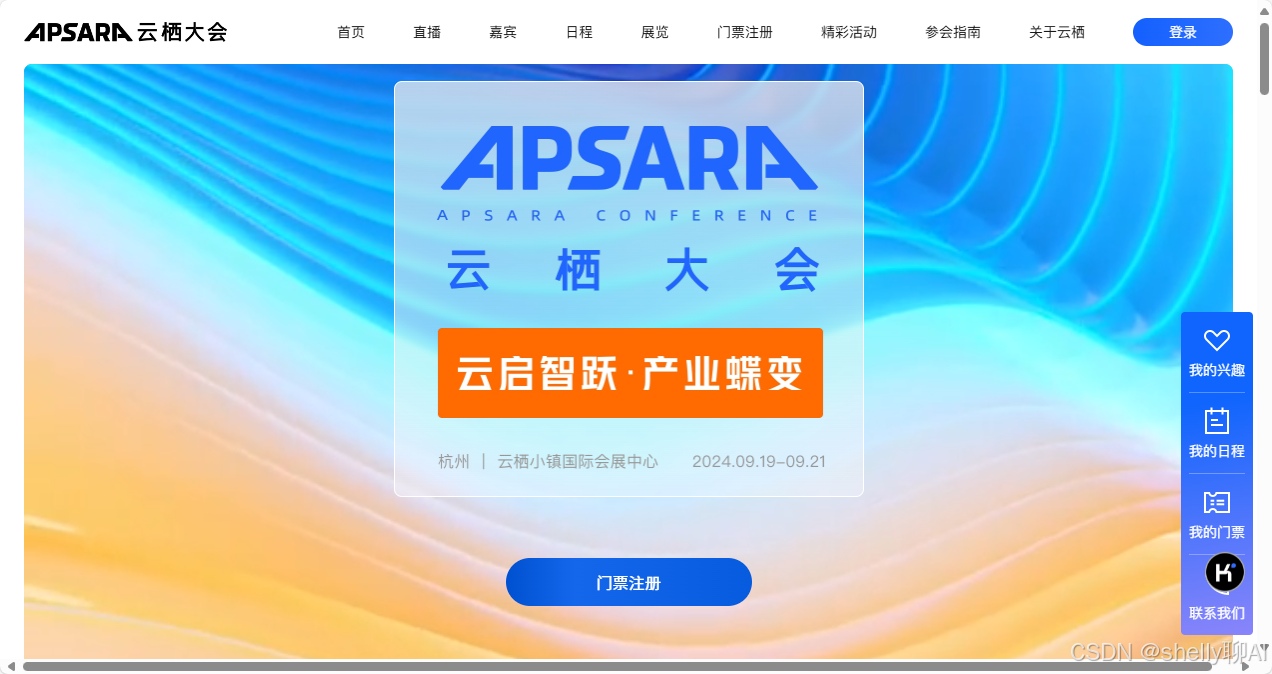
卷王阿里又开启价格战,大模型价格降价85%!
我是Shelly,一个专注于输出AI工具和科技前沿内容的AI应用教练,体验过300款以上的AI应用工具。关注科技及大模型领域对社会的影响10年。关注我一起驾驭AI工具,拥抱AI时代的到来。 9月19日,就是昨天,一年一度的云计算盛…...
Java中的异步编程模式:CompletableFuture与Reactive Programming的实战
Java中的异步编程模式:CompletableFuture与Reactive Programming的实战 大家好,我是微赚淘客返利系统3.0的小编,是个冬天不穿秋裤,天冷也要风度的程序猿!在现代Java开发中,异步编程已经成为提高应用性能和…...
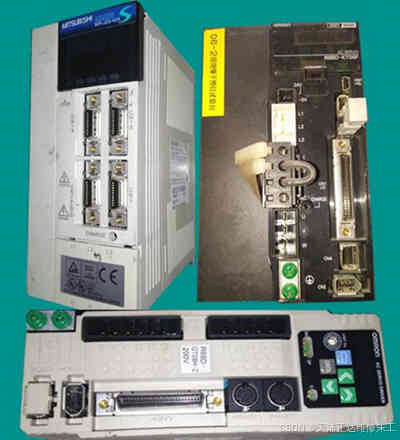
7iDU AMP田岛绣花机驱动器维修0J2100400022
7iDU AMP神州田岛绣花机驱动器维修0J2101300000绣花机控制器等全系列型号均可处理。 田岛7iDU AMP是田岛绣花机中使用很广的一种5相驱动器,在田岛平绣车TMEF-H,TMFD中应用,在链条车TMCE112S,和盘带车TMLG中大量使用。其采用的东芝…...
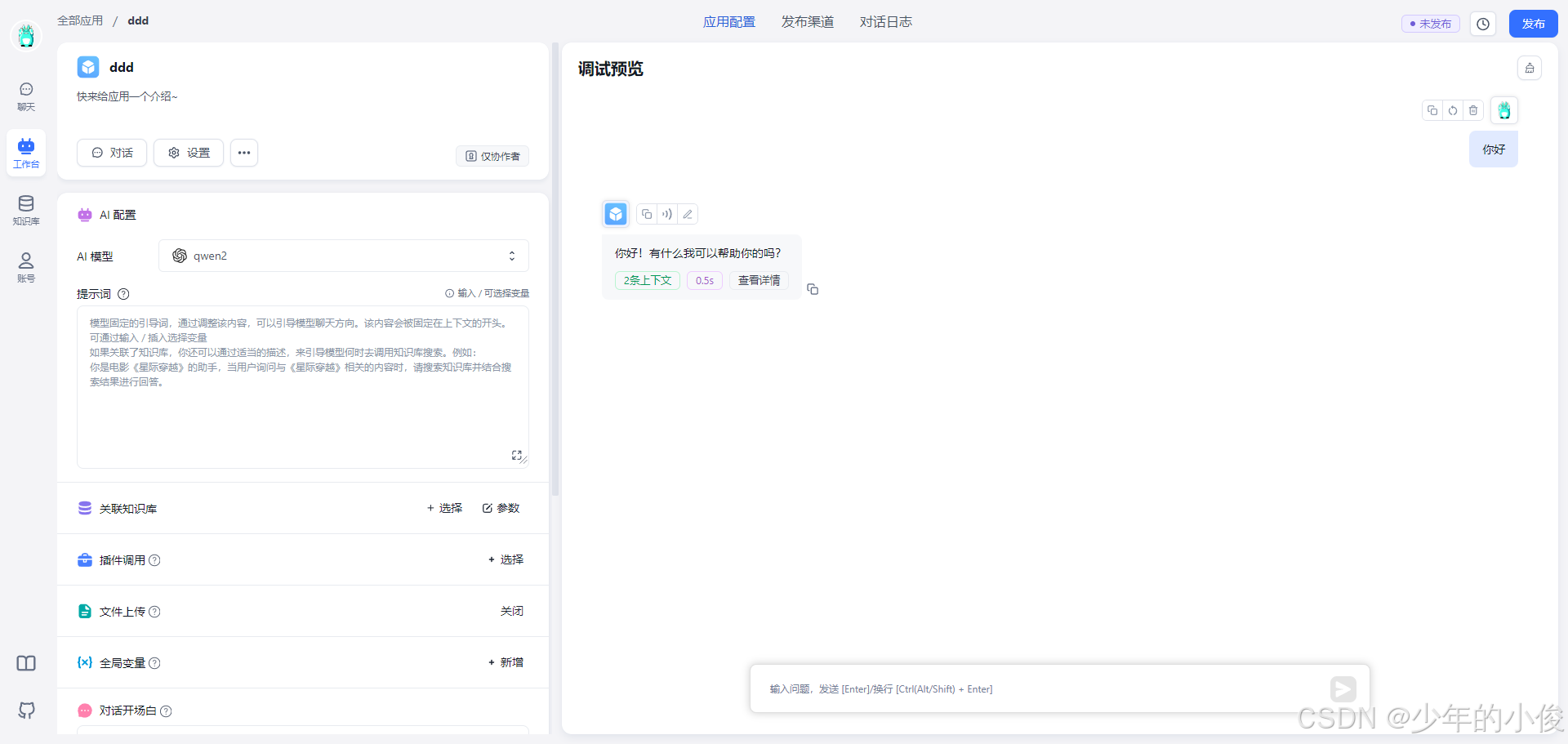
部署自己的对话大模型,使用Ollama + Qwen2 +FastGPT 实现
部署资源 AUTODL 使用最小3080Ti 资源,cuda > 12.0使用云服务器,部署fastGPT oneAPI,M3E 模型 操作步骤 配置代理 export HF_ENDPOINThttps://hf-mirror.com下载qwen2模型 - 如何下载huggingface huggingface-cli download Qwen/Qwen2-…...
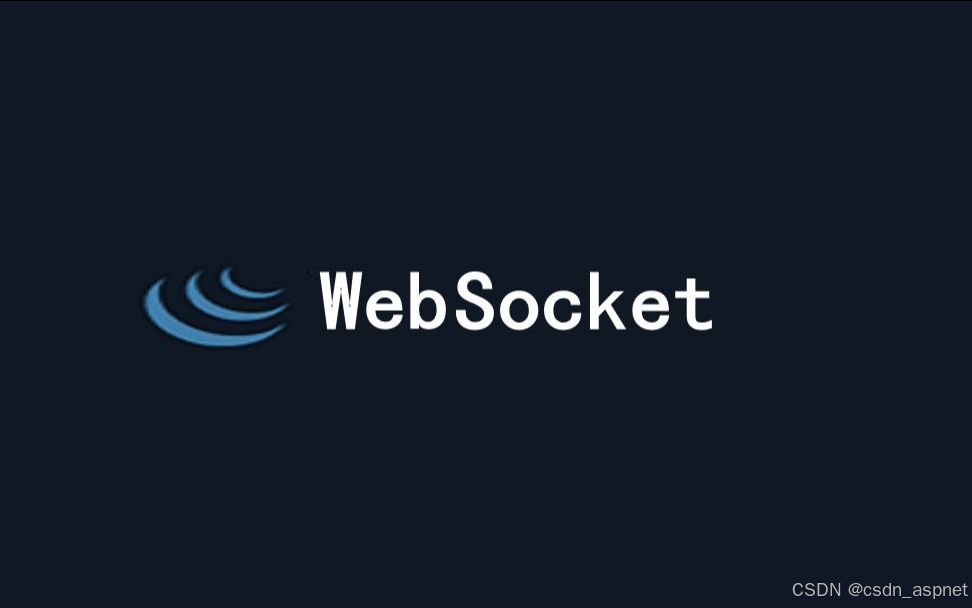
vue websocket 使用
基于webSocket通信的库主要有 socket.io,SockJS 关于SockJS的使用 先安装 sockjs-client 和 stompjs npm install sockjs-client npm install stompjs import SockJS from sockjs-client; import Stomp from stompjs; export default { data () { …...
Spring Boot 入门面试五道题
在准备Spring Boot面试时,从简单到困难设计面试题可以帮助你系统地复习和评估自己的掌握程度。以下是五个不同难度的Spring Boot面试题: 1. 简单题:什么是Spring Boot?它主要解决了什么问题? 答案: Sprin…...
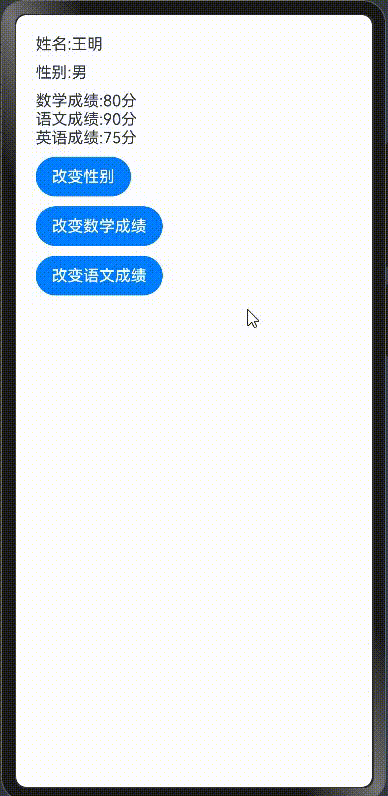
【鸿蒙】HarmonyOS NEXT开发快速入门教程之ArkTS语法装饰器(上)
文章目录 前言一、ArkTS基本介绍1、 ArkTS组成2、组件参数和属性2.1、区分参数和属性的含义2.2、父子组件嵌套 二、装饰器语法1.State2.Prop3.Link4.Watch5.Provide和Consume6.Observed和ObjectLink代码示例:示例1:(不使用Observed和ObjectLi…...
国产品牌 KTH1701系列 高性能、低功耗、全极磁场检测霍尔开关传感器
国产品牌 KTH1701系列 高性能、低功耗、全极磁场检测霍尔开关传感器 概述: KTH1701 是一款低功耗霍尔开关传感器,专为空间紧凑系统和电池电量敏感系统而设计。该芯片可以提供多种磁场阈值、开关工作频率和封装形式以适配各种应用。 当施加的S 极或 N 极…...
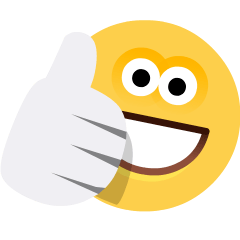
如何不终止容器退出Docker Bash会话
如何不终止容器退出Docker Bash会话 💖The Begin💖点点关注,收藏不迷路💖 当通过docker exec进入Docker容器的bash会话后,如果想退出但不停止容器,可以使用快捷键组合: 按下Ctrl+P然后紧接着按下Ctrl+Q。 这个操作会让你从bash会话中“分离”出来,但容器会继续运行…...
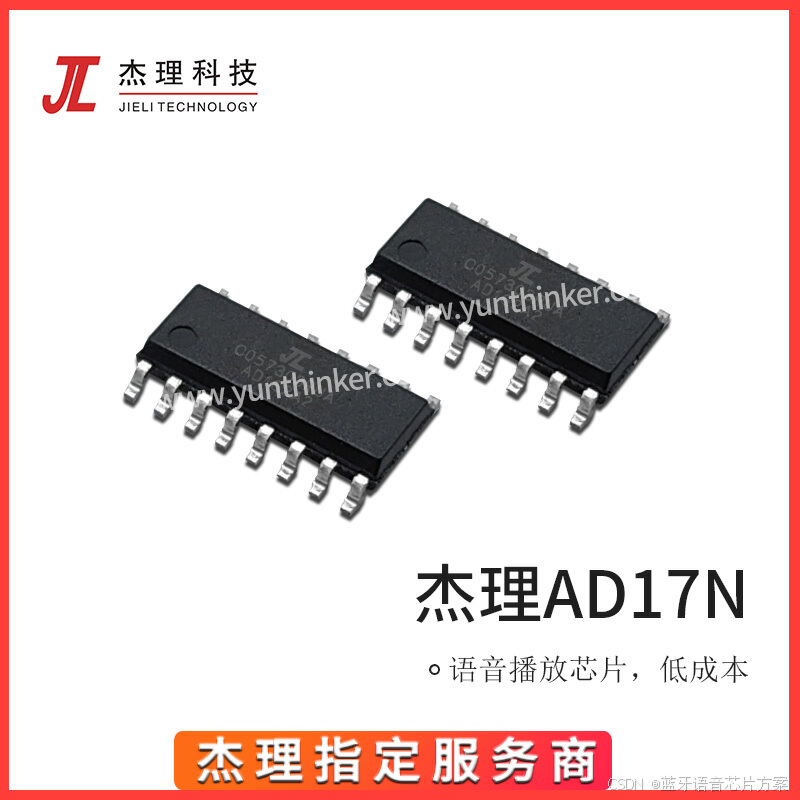
杰理芯片各型号大全,方案芯片推荐—云信通讯
29₤vFG537sTUWr《 https://s.tb.cn/h.gJ4LjAH CZ0016 杰理芯片 杰理芯片各型号大全,方案芯片推荐 https://shop.m.taobao.com/shop/shopIndex.htm?shop_id498574364&bc_fl_srctbsms_crm_3928605685_deliver$2553947245685_10973444242...
解决服务器首次请求异常耗时问题
1. 问题描述 在我们的图像识别API服务中,我们遇到了一个棘手的问题:服务器在首次接收请求时,响应时间异常地长,经常导致超时错误。这不仅影响了用户体验,还可能导致系统不稳定。 现象: 测试接口在首次调用时出现超时后续请求则能正常响应复现方法: 只需在服务重启后或长时间…...
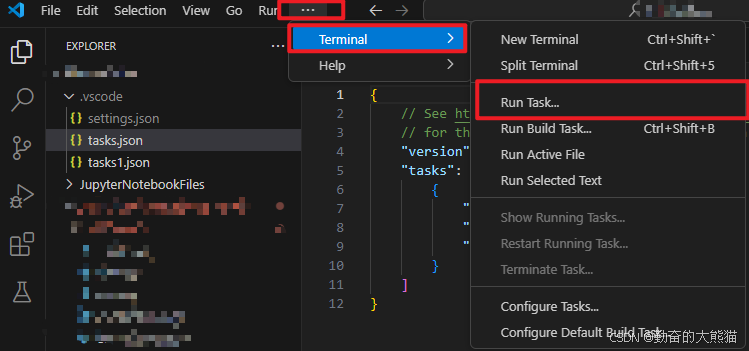
VS code 创建与运行 task.json 文件
VS code 创建与运行 task.json 文件 引言正文创建 .json 文件第一步第二步第三步 运行 .json 文件 引言 之前在 VS code EXPLORER 中不显示指定文件及文件夹设置(如.pyc, pycache, .vscode 文件) 一文中我们介绍了 settings.json 文件,这里我…...
浅谈 React Hooks
React Hooks 是 React 16.8 引入的一组 API,用于在函数组件中使用 state 和其他 React 特性(例如生命周期方法、context 等)。Hooks 通过简洁的函数接口,解决了状态与 UI 的高度解耦,通过函数式编程范式实现更灵活 Rea…...
挑战杯推荐项目
“人工智能”创意赛 - 智能艺术创作助手:借助大模型技术,开发能根据用户输入的主题、风格等要求,生成绘画、音乐、文学作品等多种形式艺术创作灵感或初稿的应用,帮助艺术家和创意爱好者激发创意、提高创作效率。 - 个性化梦境…...
日语学习-日语知识点小记-构建基础-JLPT-N4阶段(33):にする
日语学习-日语知识点小记-构建基础-JLPT-N4阶段(33):にする 1、前言(1)情况说明(2)工程师的信仰2、知识点(1) にする1,接续:名词+にする2,接续:疑问词+にする3,(A)は(B)にする。(2)復習:(1)复习句子(2)ために & ように(3)そう(4)にする3、…...
【SSH疑难排查】轻松解决新版OpenSSH连接旧服务器的“no matching...“系列算法协商失败问题
【SSH疑难排查】轻松解决新版OpenSSH连接旧服务器的"no matching..."系列算法协商失败问题 摘要: 近期,在使用较新版本的OpenSSH客户端连接老旧SSH服务器时,会遇到 "no matching key exchange method found", "n…...

Golang——6、指针和结构体
指针和结构体 1、指针1.1、指针地址和指针类型1.2、指针取值1.3、new和make 2、结构体2.1、type关键字的使用2.2、结构体的定义和初始化2.3、结构体方法和接收者2.4、给任意类型添加方法2.5、结构体的匿名字段2.6、嵌套结构体2.7、嵌套匿名结构体2.8、结构体的继承 3、结构体与…...
华为OD最新机试真题-数组组成的最小数字-OD统一考试(B卷)
题目描述 给定一个整型数组,请从该数组中选择3个元素 组成最小数字并输出 (如果数组长度小于3,则选择数组中所有元素来组成最小数字)。 输入描述 行用半角逗号分割的字符串记录的整型数组,0<数组长度<= 100,0<整数的取值范围<= 10000。 输出描述 由3个元素组成…...
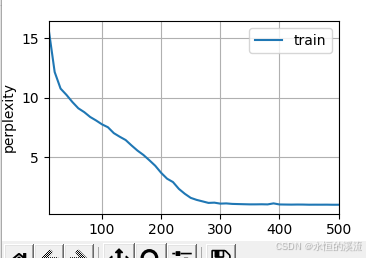
李沐--动手学深度学习--GRU
1.GRU从零开始实现 #9.1.2GRU从零开始实现 import torch from torch import nn from d2l import torch as d2l#首先读取 8.5节中使用的时间机器数据集 batch_size,num_steps 32,35 train_iter,vocab d2l.load_data_time_machine(batch_size,num_steps) #初始化模型参数 def …...
基于Java项目的Karate API测试
Karate 实现了可以只编写Feature 文件进行测试,但是对于熟悉Java语言的开发或是测试人员,可以通过编程方式集成 Karate 丰富的自动化和数据断言功能。 本篇快速介绍在Java Maven项目中编写和运行测试的示例。 创建Maven项目 最简单的创建项目的方式就是创建一个目录,里面…...
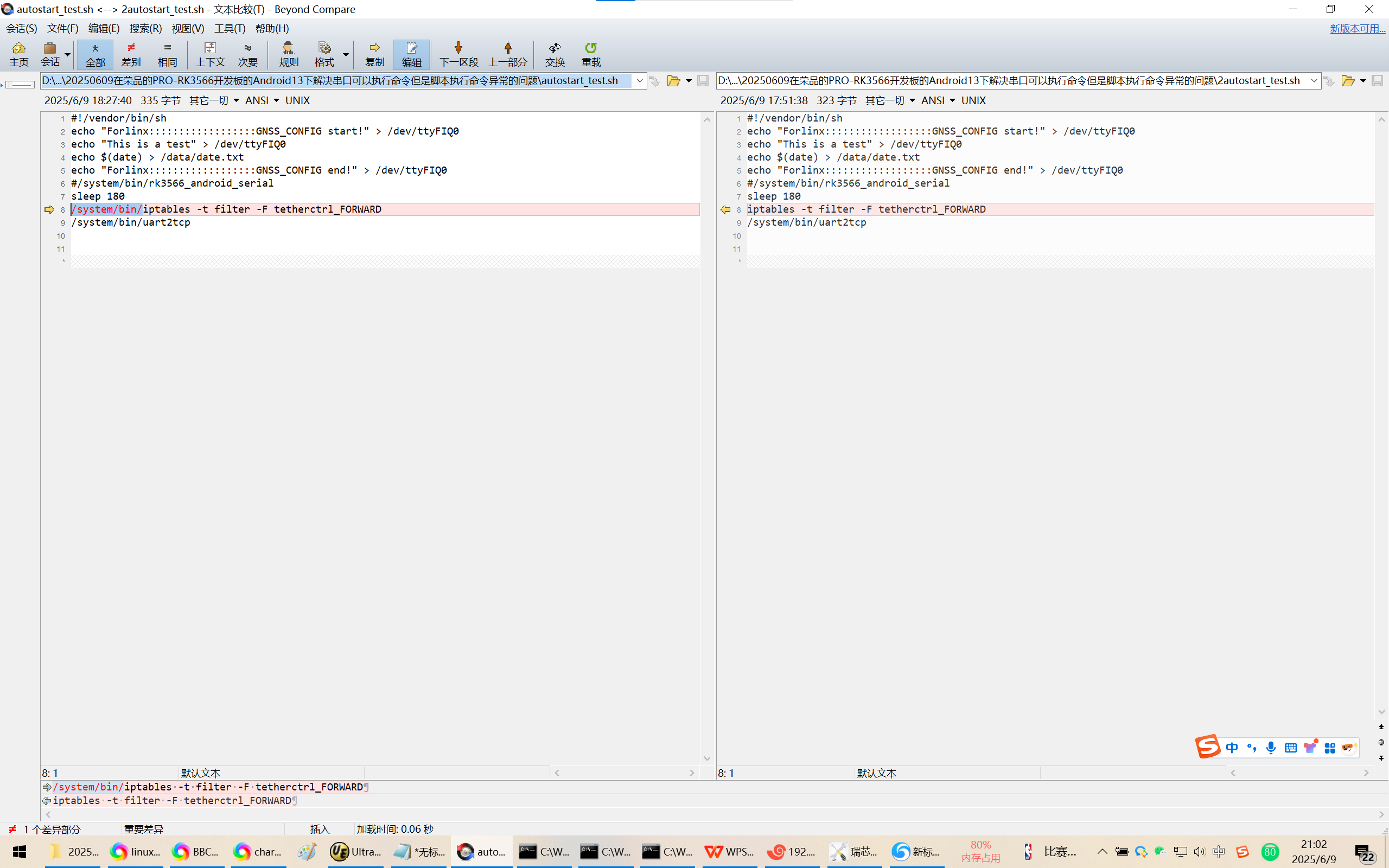
20250609在荣品的PRO-RK3566开发板的Android13下解决串口可以执行命令但是脚本执行命令异常的问题
20250609在荣品的PRO-RK3566开发板的Android13下解决串口可以执行命令但是脚本执行命令异常的问题 2025/6/9 20:54 缘起,为了跨网段推流,千辛万苦配置好了网络参数。 但是命令iptables -t filter -F tetherctrl_FORWARD可以在调试串口/DEBUG口正确执行。…...
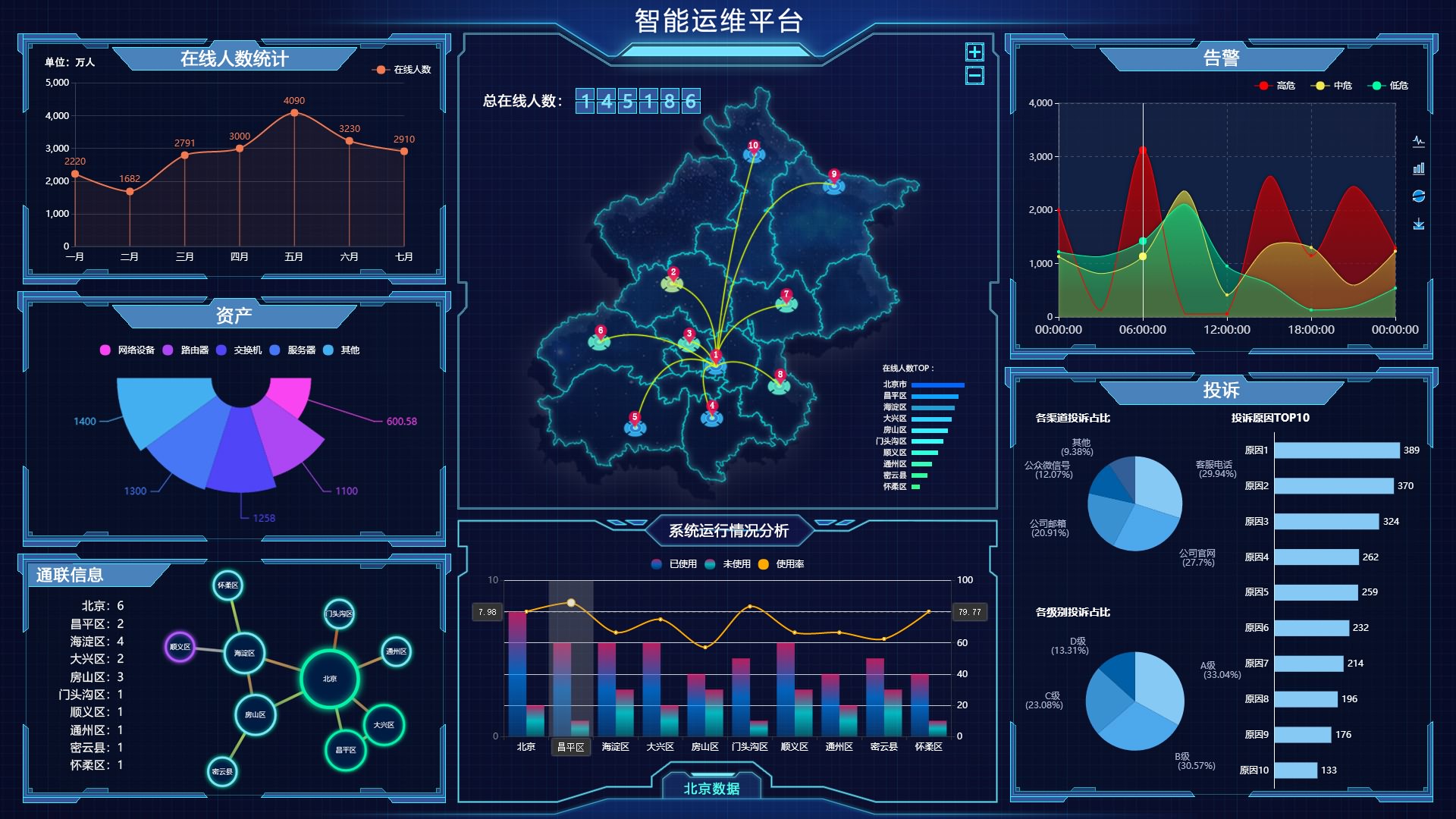
可视化预警系统:如何实现生产风险的实时监控?
在生产环境中,风险无处不在,而传统的监控方式往往只能事后补救,难以做到提前预警。但如今,可视化预警系统正在改变这一切!它能够实时收集和分析生产数据,通过直观的图表和警报,让管理者第一时间…...