Python 小型项目大全 16~20
#16 钻石
原文:http://inventwithpython.com/bigbookpython/project16.html
这个程序的特点是一个小算法,用于绘制各种尺寸的 ASCII 艺术画钻石。它包含绘制轮廓或你指定大小的填充式菱形的功能。这些功能对于初学者来说是很好的练习;试着理解钻石图背后的图案,因为它们的尺寸越来越大。
运行示例
当您运行diamonds.py
时,输出将如下所示:
Diamonds, by Al Sweigart email@protected/\
\//\
\//\
/ \
\ /\//\
//\\
\\//\//\/ \
/ \
\ /\ /\//\//\\
///\\\
\\\///\\//\/
`--snip--`
工作原理
自己创建这个程序的一个有用的方法是首先在你的编辑器中“画”几个大小的钻石,然后随着钻石变大,找出它们遵循的模式。这项技术将帮助您认识到菱形轮廓的每一行都有四个部分:前导空格数、外部正斜杠、内部空格数和外部反斜杠。实心钻石有几个内部正斜线和反斜线,而不是内部空间。破解这个模式就是我写diamonds.py
的方法。
r"""Diamonds, by Al Sweigart email@protected
Draws diamonds of various sizes.
View this code at https://nostarch.com/big-book-small-python-projects/\ /\/ \ //\\/\ /\ / \ ///\\\/ \ //\\ / \ \\\\
/\ /\ / \ ///\\\ \ / \\\\
/ \ //\\ \ / \\\/// \ / \\\///
\ / \\// \ / \\// \ / \\//\/ \/ \/ \/ \/ \/
Tags: tiny, beginner, artistic"""def main():print('Diamonds, by Al Sweigart email@protected')# Display diamonds of sizes 0 through 6:for diamondSize in range(0, 6):displayOutlineDiamond(diamondSize)print() # Print a newline.displayFilledDiamond(diamondSize)print() # Print a newline.def displayOutlineDiamond(size):# Display the top half of the diamond:for i in range(size):print(' ' * (size - i - 1), end='') # Left side space.print('/', end='') # Left side of diamond.print(' ' * (i * 2), end='') # Interior of diamond.print('\\') # Right side of diamond.# Display the bottom half of the diamond:for i in range(size):print(' ' * i, end='') # Left side space.print('\\', end='') # Left side of diamond.print(' ' * ((size - i - 1) * 2), end='') # Interior of diamond.print('/') # Right side of diamond.def displayFilledDiamond(size):# Display the top half of the diamond:for i in range(size):print(' ' * (size - i - 1), end='') # Left side space.print('/' * (i + 1), end='') # Left half of diamond.print('\\' * (i + 1)) # Right half of diamond.# Display the bottom half of the diamond:for i in range(size):print(' ' * i, end='') # Left side space.print('\\' * (size - i), end='') # Left side of diamond.print('/' * (size - i)) # Right side of diamond.# If this program was run (instead of imported), run the game:
if __name__ == '__main__':main()
在输入源代码并运行几次之后,尝试对其进行实验性的修改。你也可以自己想办法做到以下几点:
- 创建其他形状:三角形,矩形和菱形。
- 将形状输出到文本文件,而不是屏幕。
探索程序
试着找出下列问题的答案。尝试对代码进行一些修改,然后重新运行程序,看看这些修改有什么影响。
- 把第 31 行的
print('\\')
改成print('@')
会怎么样? - 把第 30 行的
print(' ' * (i * 2), end='')
改成print('@' * (i * 2), end='')
会怎么样? - 把第 18 行的
range(0, 6)
改成range(0, 30)
会怎么样? - 在第 34 行或第 49 行删除或注释掉
for i in range(size):
会发生什么?
#17 骰子数学
原文:http://inventwithpython.com/bigbookpython/project17.html
这个数学测验程序掷出两到六个骰子,你必须尽快把它们的边加起来。但是这个程序不仅仅是自动的闪存卡;它将骰子的正面画到屏幕上随机的地方。当你练习算术时,ASCII 艺术画方面增加了一个有趣的转折。
运行示例
当您运行dicemath.py
时,输出如下:
Dice Math, by Al Sweigart email@protectedAdd up the sides of all the dice displayed on the screen. You have
30 seconds to answer as many as possible. You get 4 points for each
correct answer and lose 1 point for each incorrect answer.Press Enter to begin...+-------+| O O || O || O O |+-------++-------++-------+ | O O | +-------+| O | | | | O || | | O O | | || O | +-------+ | O |+-------+ +-------+
Enter the sum: 13
`--snip--`
工作原理
屏幕上的骰子由存储在canvas
变量中的字典表示。在 Python 中,元组类似于列表,但是它们的内容不能改变。该字典的关键字是标记骰子左上角位置的(x, y)
元组,而值是ALL_DICE
中的“骰子元组”之一。您可以在第 28 到 80 行中看到,每个骰子元组包含一个字符串列表,它以图形方式表示一个可能的骰子面,以及骰子面上有多少点数的整数。该程序使用这些信息来显示骰子并计算它们的总和。
第 174 到 177 行将canvas
字典中的数据呈现在屏幕上,其方式类似于项目 13“康威的生命游戏”在屏幕上呈现单元格的方式。
"""Dice Math, by Al Sweigart email@protected
A flash card addition game where you sum the total on random dice rolls.
View this code at https://nostarch.com/big-book-small-python-projects
Tags: large, artistic, game, math"""import random, time# Set up the constants:
DICE_WIDTH = 9
DICE_HEIGHT = 5
CANVAS_WIDTH = 79
CANVAS_HEIGHT = 24 - 3 # -3 for room to enter the sum at the bottom.# The duration is in seconds:
QUIZ_DURATION = 30 # (!) Try changing this to 10 or 60.
MIN_DICE = 2 # (!) Try changing this to 1 or 5.
MAX_DICE = 6 # (!) Try changing this to 14.# (!) Try changing these to different numbers:
REWARD = 4 # (!) Points awarded for correct answers.
PENALTY = 1 # (!) Points removed for incorrect answers.
# (!) Try setting PENALTY to a negative number to give points for
# wrong answers!# The program hangs if all of the dice can't fit on the screen:
assert MAX_DICE <= 14D1 = (['+-------+','| |','| O |','| |','+-------+'], 1)D2a = (['+-------+','| O |','| |','| O |','+-------+'], 2)D2b = (['+-------+','| O |','| |','| O |','+-------+'], 2)D3a = (['+-------+','| O |','| O |','| O |','+-------+'], 3)D3b = (['+-------+','| O |','| O |','| O |','+-------+'], 3)D4 = (['+-------+','| O O |','| |','| O O |','+-------+'], 4)D5 = (['+-------+','| O O |','| O |','| O O |','+-------+'], 5)D6a = (['+-------+','| O O |','| O O |','| O O |','+-------+'], 6)D6b = (['+-------+','| O O O |','| |','| O O O |','+-------+'], 6)ALL_DICE = [D1, D2a, D2b, D3a, D3b, D4, D5, D6a, D6b]print('''Dice Math, by Al Sweigart email@protectedAdd up the sides of all the dice displayed on the screen. You have
{} seconds to answer as many as possible. You get {} points for each
correct answer and lose {} point for each incorrect answer.
'''.format(QUIZ_DURATION, REWARD, PENALTY))
input('Press Enter to begin...')# Keep track of how many answers were correct and incorrect:
correctAnswers = 0
incorrectAnswers = 0
startTime = time.time()
while time.time() < startTime + QUIZ_DURATION: # Main game loop.# Come up with the dice to display:sumAnswer = 0diceFaces = []for i in range(random.randint(MIN_DICE, MAX_DICE)):die = random.choice(ALL_DICE)# die[0] contains the list of strings of the die face:diceFaces.append(die[0])# die[1] contains the integer number of pips on the face:sumAnswer += die[1]# Contains (x, y) tuples of the top-left corner of each die.topLeftDiceCorners = []# Figure out where dice should go:for i in range(len(diceFaces)):while True:# Find a random place on the canvas to put the die:left = random.randint(0, CANVAS_WIDTH - 1 - DICE_WIDTH)top = random.randint(0, CANVAS_HEIGHT - 1 - DICE_HEIGHT)# Get the x, y coordinates for all four corners:# left# v#top > +-------+ ^# | O | |# | O | DICE_HEIGHT (5)# | O | |# +-------+ v# <-------># DICE_WIDTH (9)topLeftX = lefttopLeftY = toptopRightX = left + DICE_WIDTHtopRightY = topbottomLeftX = leftbottomLeftY = top + DICE_HEIGHTbottomRightX = left + DICE_WIDTHbottomRightY = top + DICE_HEIGHT# Check if this die overlaps with previous dice.overlaps = Falsefor prevDieLeft, prevDieTop in topLeftDiceCorners:prevDieRight = prevDieLeft + DICE_WIDTHprevDieBottom = prevDieTop + DICE_HEIGHT# Check each corner of this die to see if it is inside# of the area the previous die:for cornerX, cornerY in ((topLeftX, topLeftY),(topRightX, topRightY),(bottomLeftX, bottomLeftY),(bottomRightX, bottomRightY)):if (prevDieLeft <= cornerX < prevDieRightand prevDieTop <= cornerY < prevDieBottom):overlaps = Trueif not overlaps:# It doesn't overlap, so we can put it here:topLeftDiceCorners.append((left, top))break# Draw the dice on the canvas:# Keys are (x, y) tuples of ints, values the character at that# position on the canvas:canvas = {}# Loop over each die:for i, (dieLeft, dieTop) in enumerate(topLeftDiceCorners):# Loop over each character in the die's face:dieFace = diceFaces[i]for dx in range(DICE_WIDTH):for dy in range(DICE_HEIGHT):# Copy this character to the correct place on the canvas:canvasX = dieLeft + dxcanvasY = dieTop + dy# Note that in dieFace, a list of strings, the x and y# are swapped:canvas[(canvasX, canvasY)] = dieFace[dy][dx]# Display the canvas on the screen:for cy in range(CANVAS_HEIGHT):for cx in range(CANVAS_WIDTH):print(canvas.get((cx, cy), ' '), end='')print() # Print a newline.# Let the player enter their answer:response = input('Enter the sum: ').strip()if response.isdecimal() and int(response) == sumAnswer:correctAnswers += 1else:print('Incorrect, the answer is', sumAnswer)time.sleep(2)incorrectAnswers += 1# Display the final score:
score = (correctAnswers * REWARD) - (incorrectAnswers * PENALTY)
print('Correct: ', correctAnswers)
print('Incorrect:', incorrectAnswers)
print('Score: ', score)
在输入源代码并运行几次之后,尝试对其进行实验性的修改。标有(!)
的注释对你可以做的小改变有建议。你也可以自己想办法做到以下几点:
- 重新设计 ASCII 艺术画骰子面。
- 添加七点、八点或九点的骰子点数。
探索程序
试着找出下列问题的答案。尝试对代码进行一些修改,然后重新运行程序,看看这些修改有什么影响。
- 如果把 82 行改成
ALL_DICE = [D1]
会怎么样? - 如果把 176 行的
get((cx, cy), ' ')
改成get((cx, cy), '.')
会怎么样? - 如果把 182 行的
correctAnswers += 1
改成correctAnswers += 0
会怎么样? - 如果删除或注释掉第 93 行的
correctAnswers = 0
,会得到什么错误信息?
十八、滚动骰子
原文:http://inventwithpython.com/bigbookpython/project18.html
地下城&龙和其他桌面角色扮演游戏使用特殊的骰子,可以有 4、8、10、12 甚至 20 面。这些游戏也有一个特定的符号来指示掷哪个骰子。例如,3d6
是指掷出三个六面骰子,而1d10+2
是指掷出一个十面骰子,并在掷骰子时增加两点奖励。这个程序模拟掷骰子,以防你忘记带自己的。它还可以模拟物理上不存在的滚动骰子,如 38 面骰子。
运行示例
当您运行diceroller.py
时,输出将如下所示:
Dice Roller, by Al Sweigart email@protected
`--snip--`
> 3d6
7 (3, 2, 2)
> 1d10+2
9 (7, +2)
> 2d38-1
32 (20, 13, -1)
> 100d6
364 (3, 3, 2, 4, 2, 1, 4, 2, 4, 6, 4, 5, 4, 3, 3, 3, 2, 5, 1, 5, 6, 6, 6, 4, 5, 5, 1, 5, 2, 2, 2, 5, 1, 1, 2, 1, 4, 5, 6, 2, 4, 3, 4, 3, 5, 2, 2, 1, 1, 5, 1, 3, 6, 6, 6, 6, 5, 2, 6, 5, 4, 4, 5, 1, 6, 6, 6, 4, 2, 6, 2, 6, 2, 2, 4, 3, 6, 4, 6, 4, 2, 4, 3, 3, 1, 6, 3, 3, 4, 4, 5, 5, 5, 6, 2, 3, 6, 1, 1, 1)
`--snip--`
工作原理
这个程序中的大部分代码都致力于确保用户输入的内容格式正确。实际的随机掷骰子本身是对random.randint()
的简单调用。这个函数没有偏见:传递给它的范围内的每个整数都有可能被返回。这使得random.randint()
非常适合模拟掷骰子。
"""Dice Roller, by Al Sweigart email@protected
Simulates dice rolls using the Dungeons & Dragons dice roll notation.
This code is available at https://nostarch.com/big-book-small-python-programming
Tags: short, simulation"""import random, sysprint('''Dice Roller, by Al Sweigart email@protectedEnter what kind and how many dice to roll. The format is the number of
dice, followed by "d", followed by the number of sides the dice have.
You can also add a plus or minus adjustment.Examples:3d6 rolls three 6-sided dice1d10+2 rolls one 10-sided die, and adds 22d38-1 rolls two 38-sided die, and subtracts 1QUIT quits the program
''')while True: # Main program loop:try:diceStr = input('> ') # The prompt to enter the dice string.if diceStr.upper() == 'QUIT':print('Thanks for playing!')sys.exit()# Clean up the dice string:diceStr = diceStr.lower().replace(' ', '')# Find the "d" in the dice string input:dIndex = diceStr.find('d')if dIndex == -1:raise Exception('Missing the "d" character.')# Get the number of dice. (The "3" in "3d6+1"):numberOfDice = diceStr[:dIndex]if not numberOfDice.isdecimal():raise Exception('Missing the number of dice.')numberOfDice = int(numberOfDice)# Find if there is a plus or minus sign for a modifier:modIndex = diceStr.find('+')if modIndex == -1:modIndex = diceStr.find('-')# Find the number of sides. (The "6" in "3d6+1"):if modIndex == -1:numberOfSides = diceStr[dIndex + 1 :]else:numberOfSides = diceStr[dIndex + 1 : modIndex]if not numberOfSides.isdecimal():raise Exception('Missing the number of sides.')numberOfSides = int(numberOfSides)# Find the modifier amount. (The "1" in "3d6+1"):if modIndex == -1:modAmount = 0else:modAmount = int(diceStr[modIndex + 1 :])if diceStr[modIndex] == '-':# Change the modification amount to negative:modAmount = -modAmount# Simulate the dice rolls:rolls = []for i in range(numberOfDice):rollResult = random.randint(1, numberOfSides)rolls.append(rollResult)# Display the total:print('Total:', sum(rolls) + modAmount, '(Each die:', end='')# Display the individual rolls:for i, roll in enumerate(rolls):rolls[i] = str(roll)print(', '.join(rolls), end='')# Display the modifier amount:if modAmount != 0:modSign = diceStr[modIndex]print(', {}{}'.format(modSign, abs(modAmount)), end='')print(')')except Exception as exc:# Catch any exceptions and display the message to the user:print('Invalid input. Enter something like "3d6" or "1d10+2".')print('Input was invalid because: ' + str(exc))continue # Go back to the dice string prompt.
在输入源代码并运行几次之后,尝试对其进行实验性的修改。你也可以自己想办法做到以下几点:
- 添加一个乘法修饰符来补充加法和减法修饰符。
- 增加自动移除最低模具辊的能力。
探索程序
试着找出下列问题的答案。尝试对代码进行一些修改,然后重新运行程序,看看这些修改有什么影响。
- 如果删除或注释掉第 69 行的
rolls.append(rollResult)
会发生什么? - 如果把第 69 行的
rolls.append(rollResult)
改成rolls.append(-rollResult)
会怎么样? - 如果删除或注释掉第 77 行的
print(', '.join(rolls), end='')
会怎么样? - 如果不掷骰子而什么都不输入会怎么样?
十九、数字时钟
原文:http://inventwithpython.com/bigbookpython/project19.html
这个程序显示一个带有当前时间的数字时钟。第六十四个项目的sevseg.py
模块“七段显示模块”为每个数字生成图形,而不是直接呈现数字字符。这个项目类似于项目 14,“倒计时”
运行示例
当您运行digitalclock.py
时,输出将如下所示:
__ __ __ __ __ __
| | |__| * __| __| * __| |__
|__| __| * __| __| * __| |__|Press Ctrl-C to quit.
工作原理
数字时钟程序看起来类似于项目 14,“倒计时。”他们不仅都导入了sevseg.py
模块,还必须用splitlines()
方法拆分由sevseg.getSevSegStr()
返回的多行字符串。这允许我们在时钟的小时、分钟和秒部分的数字之间放置一个由星号组成的冒号。将这段代码与倒计时中的代码进行比较,看看有哪些相似之处,有哪些不同之处。
"""Digital Clock, by Al Sweigart email@protected
Displays a digital clock of the current time with a seven-segment
display. Press Ctrl-C to stop.
More info at https://en.wikipedia.org/wiki/Seven-segment_display
Requires sevseg.py to be in the same folder.
This code is available at https://nostarch.com/big-book-small-python-programming
Tags: tiny, artistic"""import sys, time
import sevseg # Imports our sevseg.py program.try:while True: # Main program loop.# Clear the screen by printing several newlines:print('\n' * 60)# Get the current time from the computer's clock:currentTime = time.localtime()# % 12 so we use a 12-hour clock, not 24:hours = str(currentTime.tm_hour % 12)if hours == '0':hours = '12' # 12-hour clocks show 12:00, not 00:00.minutes = str(currentTime.tm_min)seconds = str(currentTime.tm_sec)# Get the digit strings from the sevseg module:hDigits = sevseg.getSevSegStr(hours, 2)hTopRow, hMiddleRow, hBottomRow = hDigits.splitlines()mDigits = sevseg.getSevSegStr(minutes, 2)mTopRow, mMiddleRow, mBottomRow = mDigits.splitlines()sDigits = sevseg.getSevSegStr(seconds, 2)sTopRow, sMiddleRow, sBottomRow = sDigits.splitlines()# Display the digits:print(hTopRow + ' ' + mTopRow + ' ' + sTopRow)print(hMiddleRow + ' * ' + mMiddleRow + ' * ' + sMiddleRow)print(hBottomRow + ' * ' + mBottomRow + ' * ' + sBottomRow)print()print('Press Ctrl-C to quit.')# Keep looping until the second changes:while True:time.sleep(0.01)if time.localtime().tm_sec != currentTime.tm_sec:break
except KeyboardInterrupt:print('Digital Clock, by Al Sweigart email@protected')sys.exit() # When Ctrl-C is pressed, end the program.
探索程序
试着找出下列问题的答案。尝试对代码进行一些修改,然后重新运行程序,看看这些修改有什么影响。
- 如果把第 45 行的
time.sleep(0.01)
改成time.sleep(2)
会怎么样? - 如果把第 27、30、33 行的
2
改成1
会怎么样? - 如果删除或注释掉第 15 行的
print('\n' * 60)
会发生什么? - 如果删除或注释掉第 10 行的
import sevseg
,会得到什么错误信息?
二十、数字雨
原文:http://inventwithpython.com/bigbookpython/project20.html
这个程序模仿了科幻电影《黑客帝国》中的“数字雨”可视化效果。随机的二进制“雨”珠从屏幕底部流上来,创造了一个很酷的、黑客般的可视化效果。(不幸的是,由于文本随着屏幕向下滚动而移动的方式,如果不使用bext
这样的模块,就不可能让流向下移动。)
运行示例
当您运行digitalstream.py
时,输出将如下所示:
Digital Stream Screensaver, by Al Sweigart email@protected
Press Ctrl-C to quit.0 00 01 0 0 1 1 0 10 0 0 1 0 0 0 0 00 1 0 0 0 1 0 0 1 0 10 1 0 0 1 011 1 1 0 1 00 1 0 0 0 000 11 0 0 1 1 01 1 0 1 0 1 1 110 10 1 0 1 0 1 01 101 0 0 1 000 11 1 1 11 1 1 10 100 1 0 11 00 0 1 01 01 1 001 1 1 0 1 10 0 10 00 0 010 0 1 1 11 11 0 0
`--snip--`
工作原理
像项目 15,“深坑”,这个程序使用由print()
调用引起的滚动来创建动画。在列列表中,每一列都由一个整数表示:columns[0]
是最左边一列的整数,columns[1]
是右边一列的整数,依此类推。程序最初将这些整数设置为0
,这意味着它打印' '
(一个空格字符串)而不是该列中的流。随机地,它将每个整数改变为一个在MIN_STREAM_LENGTH
和MAX_STREAM_LENGTH
之间的值。每打印一行,该整数就减少1
。只要一列的整数大于0
,程序就会在该列中打印一个随机的1
或0
。这会产生您在屏幕上看到的“数字雨”效果。
"""Digital Stream, by Al Sweigart email@protected
A screensaver in the style of The Matrix movie's visuals.
This code is available at https://nostarch.com/big-book-small-python-programming
Tags: tiny, artistic, beginner, scrolling"""import random, shutil, sys, time# Set up the constants:
MIN_STREAM_LENGTH = 6 # (!) Try changing this to 1 or 50.
MAX_STREAM_LENGTH = 14 # (!) Try changing this to 100.
PAUSE = 0.1 # (!) Try changing this to 0.0 or 2.0.
STREAM_CHARS = ['0', '1'] # (!) Try changing this to other characters.# Density can range from 0.0 to 1.0:
DENSITY = 0.02 # (!) Try changing this to 0.10 or 0.30.# Get the size of the terminal window:
WIDTH = shutil.get_terminal_size()[0]
# We can't print to the last column on Windows without it adding a
# newline automatically, so reduce the width by one:
WIDTH -= 1print('Digital Stream, by Al Sweigart email@protected')
print('Press Ctrl-C to quit.')
time.sleep(2)try:# For each column, when the counter is 0, no stream is shown.# Otherwise, it acts as a counter for how many times a 1 or 0# should be displayed in that column.columns = [0] * WIDTHwhile True:# Set up the counter for each column:for i in range(WIDTH):if columns[i] == 0:if random.random() <= DENSITY:# Restart a stream on this column.columns[i] = random.randint(MIN_STREAM_LENGTH,MAX_STREAM_LENGTH)# Display an empty space or a 1/0 character.if columns[i] > 0:print(random.choice(STREAM_CHARS), end='')columns[i] -= 1else:print(' ', end='')print() # Print a newline at the end of the row of columns.sys.stdout.flush() # Make sure text appears on the screen.time.sleep(PAUSE)
except KeyboardInterrupt:sys.exit() # When Ctrl-C is pressed, end the program.
在输入源代码并运行几次之后,尝试对其进行实验性的修改。标有(!)
的注释对你可以做的小改变有建议。你也可以自己想办法做到以下几点:
- 包括除 1 和 0 之外的字符。
- 包括线以外的形状,包括矩形、三角形和菱形。
探索程序
试着找出下列问题的答案。尝试对代码进行一些修改,然后重新运行程序,看看这些修改有什么影响。
- 如果把第 46 行的
print(' ', end='')
改成print('.', end='')
会怎么样? - 如果将第 11 行的
PAUSE = 0.1
改为PAUSE = -0.1
,会得到什么错误信息? - 如果把第 42 行的
columns[i] > 0
改成columns[i] < 0
会怎么样? - 如果把第 42 行的
columns[i] > 0
改成columns[i] <= 0
会怎么样? - 如果把第 44 行的
columns[i] -= 1
改成columns[i] += 1
会怎么样?
相关文章:
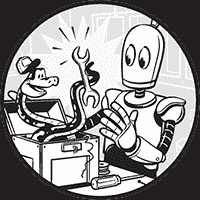
Python 小型项目大全 16~20
#16 钻石 原文:http://inventwithpython.com/bigbookpython/project16.html 这个程序的特点是一个小算法,用于绘制各种尺寸的 ASCII 艺术画钻石。它包含绘制轮廓或你指定大小的填充式菱形的功能。这些功能对于初学者来说是很好的练习;试着理解…...
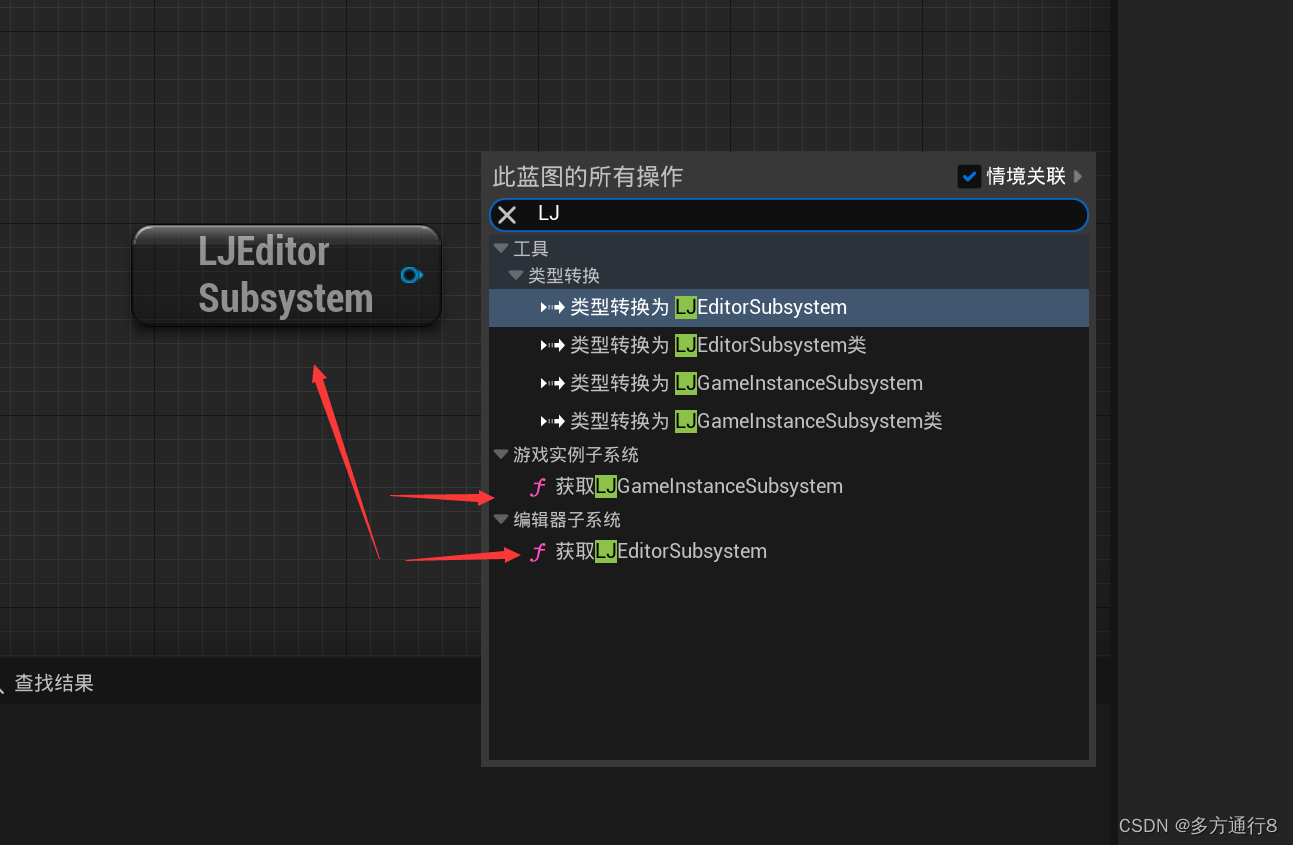
UE4/5C++之SubSystem的了解与创建
目录 了解生命周期 为什么用他,简单讲解? SubSystems创建和使用 创建SubSystems中的UGamelnstanceSubsystem类: 写基本的3个函数: 在蓝图中的样子: 创建SubSystems中的UEditorSubsystem类: SubSyste…...
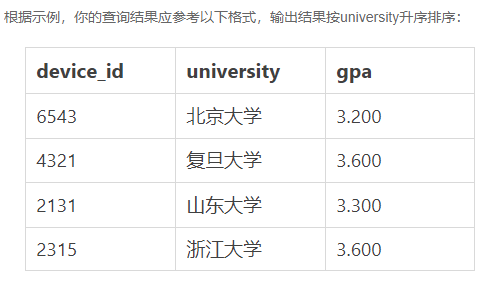
牛客网在线编程SQL篇非技术快速入门题解(二)
大家好,我是RecordLiu。 初学SQL,有哪些合适的练习网站推荐呢? 如果你有编程基础,那么我推荐你到Leetcode这样的专业算法刷题网站,如果没有,也不要紧,你也可以到像牛客网一样的编程网站去练习。 牛客网有很多面向非技…...

航天器轨道六要素和TLE两行轨道数据格式
航天器轨道要素 椭圆轨道六根数指的是:半长轴aaa,离心率e,轨道倾角iii、升交点赤经Ω\OmegaΩ、近地点辐角ω\omegaω、和过近地点时刻t0t_0t0(或真近点角φ)。 决定轨道形状: 轨道半长轴aaa࿱…...

【Spring Cloud Alibaba】第01节 - 课程介绍
一、Spring Cloud Alibaba 阿里巴巴公司 以Spring Cloud的衍生微服务一站式解决方案 二、学习Spring Cloud Alibaba的原因 Spring Cloud 多项组件宣布闭源或停止维护Spring Cloud Alibaba 性能优于Spring Cloud 三、适应群体 有Java编程和SpringBoot基础,最好有Sp…...

iOS和Android手机浏览器链接打开app store或应用市场下载软件讲解
引言当开发一个app出来后,通过分享引流用户去打开/下载该app软件,不同手机下载的地方不一样,比如:ios需要到苹果商店去下载,Android手机需要到各个不同的应用商店去下载(华为手机需要到华为应用商店下载,vi…...

2023第十四届蓝桥杯省赛java B组
试题 A: 阶乘求和 本题总分:5 分 【问题描述】 令 S 1! 2! 3! ... 202320232023!,求 S 的末尾 9 位数字。 提示:答案首位不为 0。 【答案提交】 这是一道结果填空的题,你只需要算出结果后提交即可。本题的结果为一 个整数…...
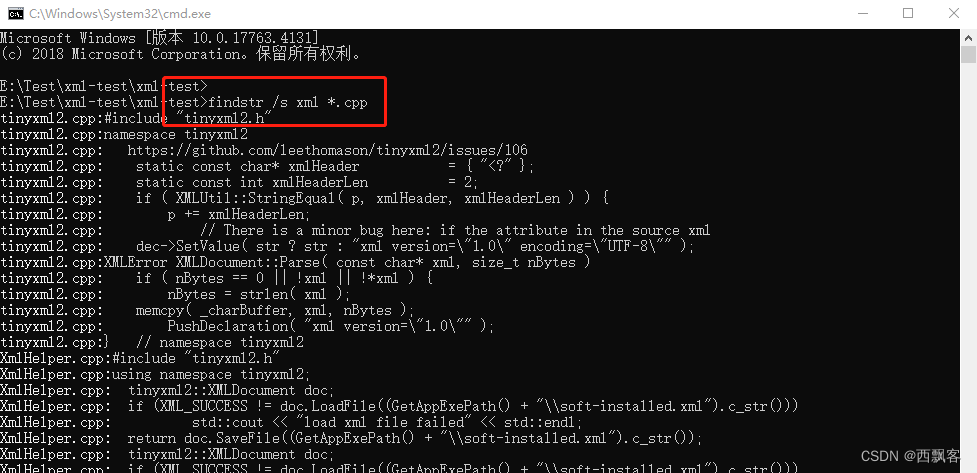
windows下如何快速搜索文件内容
安装git,使用linux命令 grep 这里不再多说 windows版本的命令 Windows提供find/findstr类似命令,其中findstr要比find功能更多一些,可以/?查看帮助。...

Redis集群分片
文章目录1、Redis集群的基本概念2、浅析集群算法-分片-槽位slot2.1 Redis集群的槽位slot2.2 Redis集群的分片2.3 两大优势2.4 如何进行slot槽位映射2.5 为什么redis集群的最大槽数是16384个?2.6 Redis集群不保证强一致性3、集群环境搭建3.1 主从容错切换迁移3.2 主从…...

ISP-AF相关-聚焦区域选择-清晰度评价-1(待补充)
1、镜头相关 镜头类型 变焦类型: 定焦、手动变焦、自动变焦 光圈: 固定光圈、手动光圈、自动光圈 视场角: 鱼眼镜头、超广角镜头、广角镜头、标准镜头、长焦镜头、超长焦镜头(由大至小) 光圈: 超星…...

[element-ui] el-table行添加阴影悬浮效果
问题: 在el-table每一行获得焦点与鼠标经过时,显示一个整行的阴影悬浮效果 /*其中,table-row-checkd是我自定义的焦点行添加类名,大家可以自己起名*/ .el-table tbody tr:hover,.el-table tbody tr.table-row-checked{box-shadow: 0px 3px …...
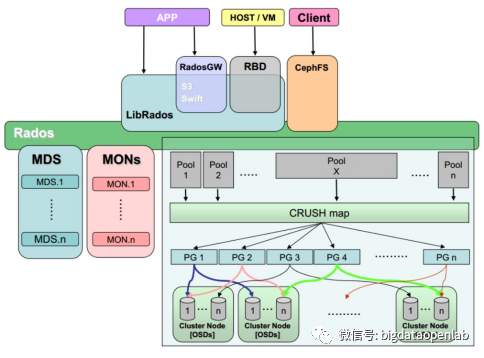
分布式存储技术(上):HDFS 与 Ceph的架构原理、特性、优缺点解析
面对企业级数据量,单机容量太小,无法存储海量的数据,这时候就需要用到多台机器存储,并统一管理分布在集群上的文件,这样就形成了分布式文件系统。HDFS是Hadoop下的分布式文件系统技术,Ceph是能处理海量非结…...

【python设计模式】20、解释器模式
哲学思想: 解释器模式(Interpreter Pattern)是一种行为型设计模式,它提供了一种方式来解释和执行特定语言的语法或表达式。该模式中,解释器通过将表达式转换为可以执行的对象来实现对表达式的解释和执行。通常…...
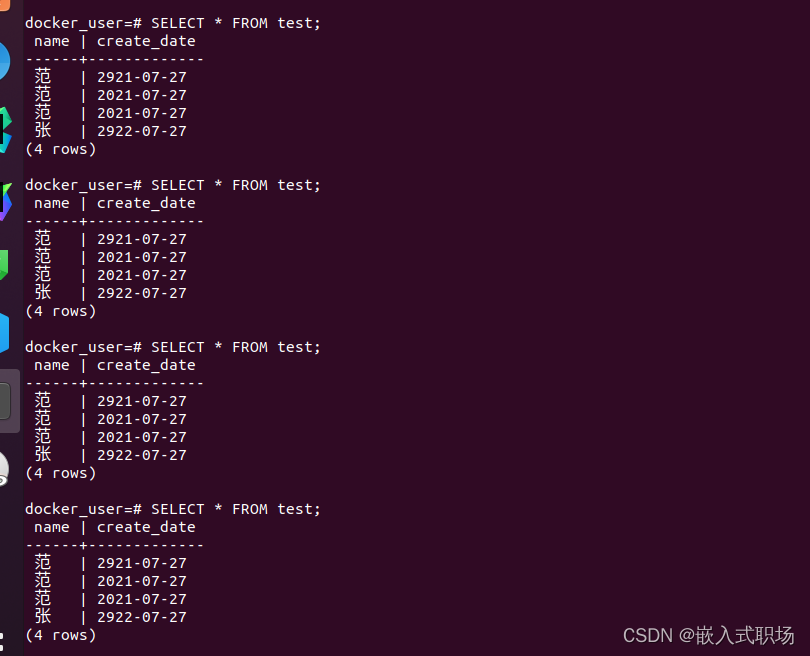
【PostgreSQL】通过docker的方式运行部署PostgreSQL与go操作数据库
目录 1、docker的方式运行部署PostgreSQL 2、控制台命令 3、go操作增删改查 1、docker的方式运行部署PostgreSQL docker pull postgres docker run --name learn_postgres -e POSTGRES_PASSWORDdocker_user -e POSTGRES_USERdocker_user -p 5433:5432 -d postgres进入容器&am…...

Kotlin协程序列:
1: 使用方式一 ,callback和coroutine相互转化。 import kotlinx.coroutines.* import java.lang.Exception class MyCallback {fun doSomething(callback: (String?, Exception?) -> Unit) {// 模拟异步操作GlobalScope.launch {try {delay(1000) // 延迟 1 秒…...

java获取视频时长
1、先导包 <dependency><groupId>ws.schild</groupId><artifactId>jave-all-deps</artifactId><version>2.6.0</version> </dependency>2、获取时长 Testpublic void test01() {long time 0;try {String url "http://…...

EDAS投稿系统的遇到的问题及解决办法
问题1: gutter: Upload failed: The gutter between columns is 0.2 inches wide (on page 1), but should be at least 0.2 inches 解决: 在\begin{document}前添加\columnsep 0.201 in(0.2in也会报错,建议填大一点点)…...
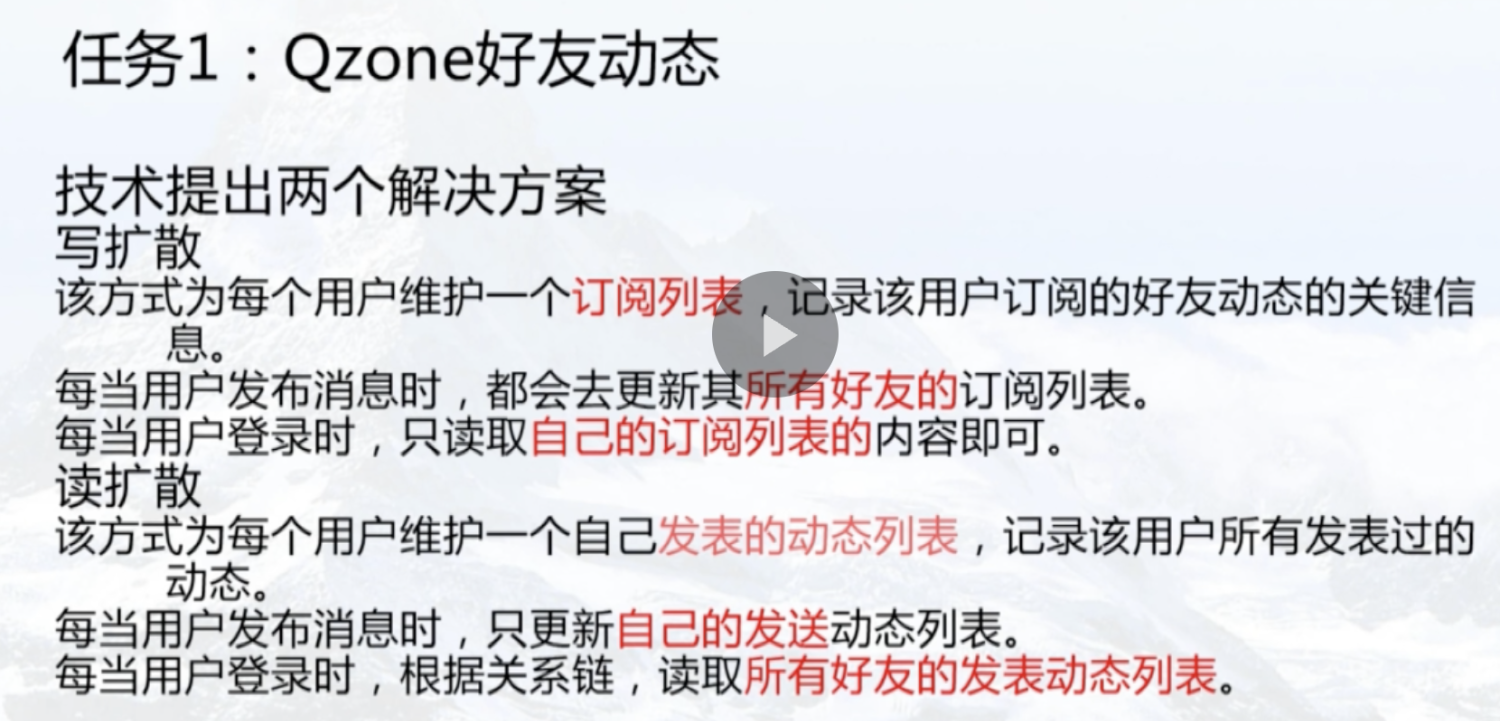
t-learning 产品经理课程笔记
t-learning 腾讯公开课——产品经理课程 第一课 化身用户研究员,张小龙《产品精讲》 1-3:执行 4-7:中坚力量 7:核心leader 能解决问题的,就是好的产品经理 如何储备产品知识与素养 (1)了解并…...

校招,从准备开始准备(持续更新ing...)
诸神缄默不语-个人CSDN博文目录 作者现在有科研任务在身(今天还在标数据哦),所以不能实习。 所以就是纯纯拉个表。 最近更新时间:2023.4.9 最早更新时间:2023.4.6 文章目录1. 学习资料和知识点清单1.1 机器学习1.2 深…...
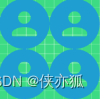
Android:使用LayerDrawable动态生成四宫格头像(包含双人、三人头像)
其实用自定义View也可以实现,我比较懒,就用LayerDrawable来创建一个新的Drawable资源实现。 举例4宫格,9宫格原理类似,每个图标的位置需要用边距慢慢调成预期的效果 效果如下: 双人头像: 三人头像&#x…...

Android Jetpack 从使用到源码深耕【数据库注解Room 从实践到原理 】(三)
前面两篇文章,我们一起学习了,Room引入的背景、Room的使用方式、Room的实现原理猜想验证、Room的源码原理探索总结。 本文,我们将其中牵扯到的课外知识点 or 过程中没有说到的知识点,进行一下单独的总结。 题外话:扩展知识点总结 1. 抽象工厂的设计模式应用 在源码探索…...
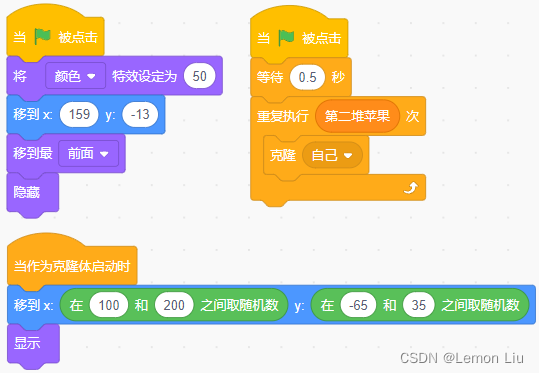
中国电子学会2023年03月份青少年软件编程Scratch图形化等级考试试卷三级真题(含答案)
2023-03 Scratch三级真题 分数:100 题数:38 测试时长:60min 一、单选题(共25题,共50分) 1.计算“248……128”,用变量n表示每项,根据变化规律,变量n的赋值用下列哪个最合适?&am…...

分布式事务培训
MQ发生成功 MQ响应失败 断网 DIY seary 不保证隔离性,扣账不成功,钱被花了。导致回滚不成功 超时处理。 超时处理机制 防悬挂, try 的 try catch 导致不报错。空提交 处理链,inputlog万一数据库出现问题。outlog 最终保证回滚。映…...
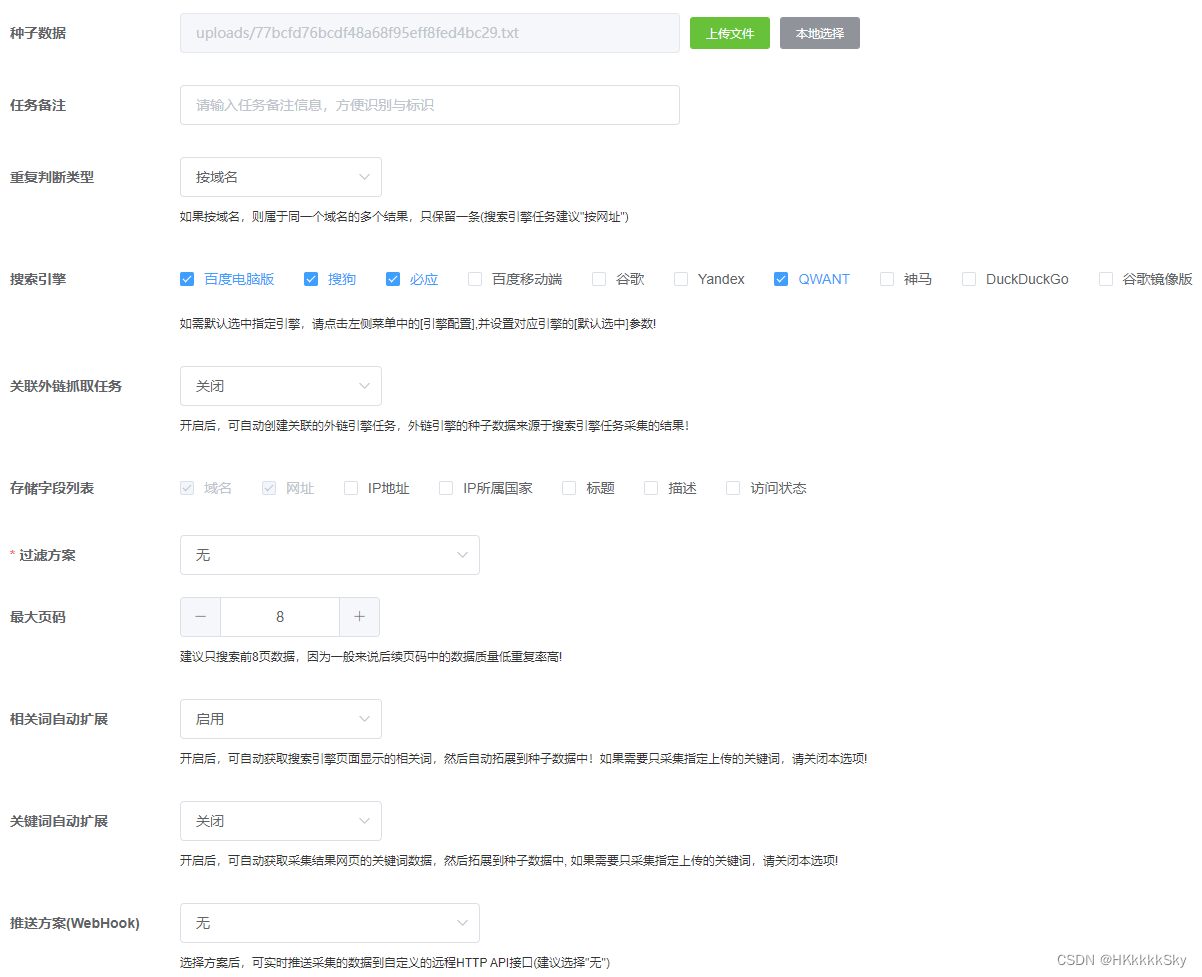
关键词采集工具可以帮助我们做那些方面的工作
针对搜索引擎的关键词采集工具可以帮助我们做那些方面的工作,至少从10个工作场景说明,并列举详细的使用场景 Msray-plus,是一款企业级综合性爬虫/采集软件。 支持亿级数据存储、导入、重复判断等。无需使用复杂的命令,提供本地W…...
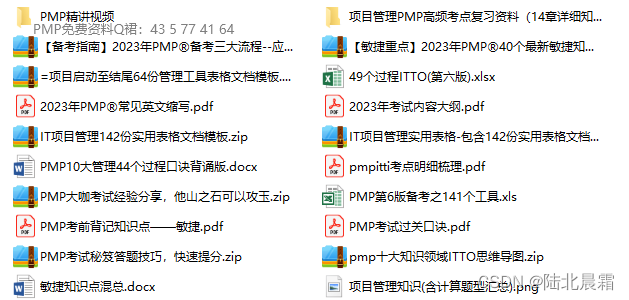
2023年5月PMP难考吗?
PMP考试难不难,还是因人而异的,对小白而言,肯定是难的,对项目管理老人而言,难度肯定是没那么高。 难点主要是非常多而难理解的知识点,以及答题时的知识点提取。经过系统的学习,分解知识点&…...

定语从句的省略
1. 关系代词的省略(即that which之类的) 条件:首先限制定语从句(即没有逗号的) 先行词在从句中作宾语成分 两个条件缺一不可,先行词中作主语成分是不可以的。(这就是形容词短语作定语后置和定…...
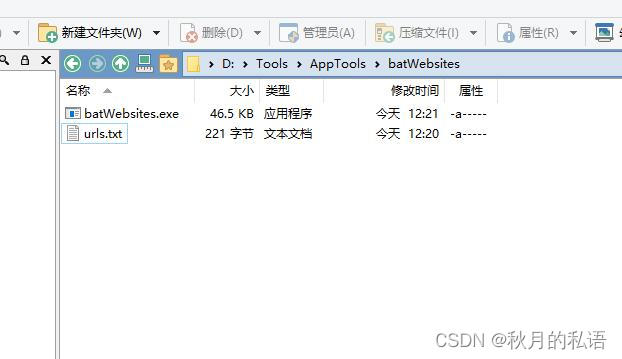
简易小工具实现批量打开多个网页
最近有个需求,希望一次性可以打开多个网页,网址自由指定,这个需求的实现非常简单,使用基本的c代码调用system函数即可,都不需要MFC相关的东西。 但是我实测一些工具后发现一个问题,当打开超过大约3个网址的…...

swiper 点击事件
点击swiper 获取当前下标 两种模式 "swiper": "^5.4.5", "vue-awesome-swiper": "^3.1.3",swiperOption: {autoplay: { delay: 3000 },loop: true, //循环slidesPerView: auto,direction: "vertical",disableOnInteraction:…...
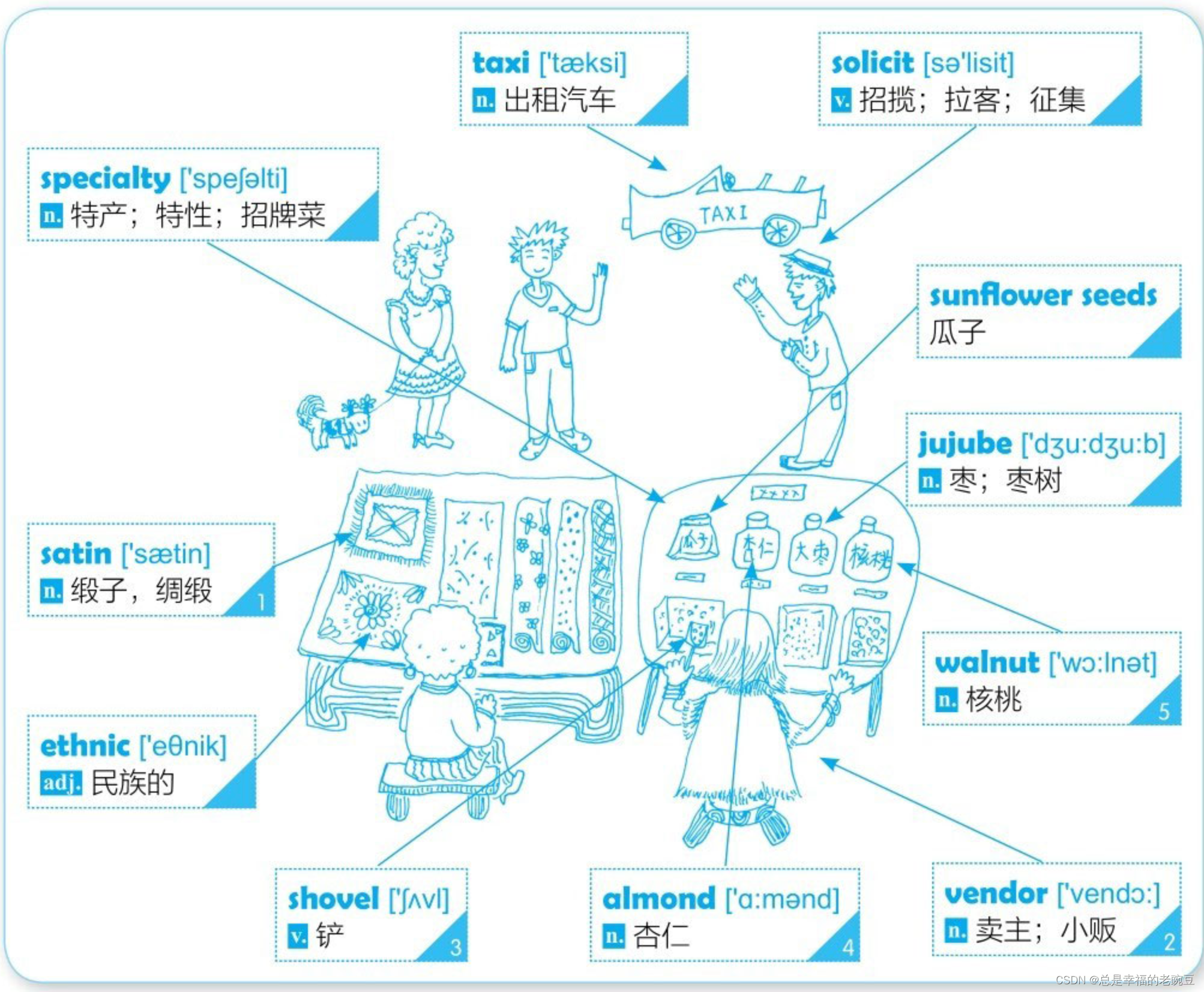
旅游心得Traveling Experience
前言 加油 原文 旅游心得常用会话 ❶ Share photos of the trip with friends. 与朋友分享旅游的照片。 ❷ We’ll go to the Great Wall, if you prefer. 你如果愿意的话,我们去长城。 ❸ Would you go to the church or the synagogue or the mosque? 你会去教堂,犹太…...
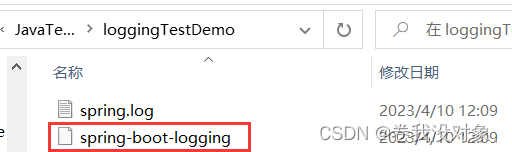
【 SpringBoot ⽇志⽂件 】
文章目录一、⽇志的作用二、认识⽇志三、⾃定义⽇志打印3.1 在程序中得到⽇志对象3.2 使⽤⽇志对象打印⽇志3.3 ⽇志格式说明四、⽇志级别4.1 ⽇志级别的作用4.2 ⽇志级别的分类与使⽤4.2.1 ⽇志级别的分类4.2.2 ⽇志使⽤4.2.2.1 配置全局日志级别4.2.2.2 配置局部文件夹的日志…...