boost beast http server 测试
boost beast http client
boost http server
boost beast 是一个非常好用的库,带上boost的协程,好很多东西是比较好用的,以下程序使用四个线程开启协程处理进入http协议处理。协议支持http get 和 http post
#include <boost/beast/core.hpp>
#include <boost/beast/http.hpp>
#include <boost/beast/version.hpp>
#include <boost/asio/ip/tcp.hpp>
#include <boost/asio/spawn.hpp>
#include <boost/config.hpp>
#include <algorithm>
#include <cstdlib>
#include <iostream>
#include <memory>
#include <string>
#include <thread>
#include <vector>namespace beast = boost::beast; // from <boost/beast.hpp>
namespace http = beast::http; // from <boost/beast/http.hpp>
namespace net = boost::asio; // from <boost/asio.hpp>
using tcp = boost::asio::ip::tcp; // from <boost/asio/ip/tcp.hpp>
#undef BOOST_BEAST_VERSION_STRING
#define BOOST_BEAST_VERSION_STRING "qbversion1.1"
// Return a reasonable mime type based on the extension of a file.
beast::string_view
mime_type(beast::string_view path)
{using beast::iequals;auto const ext = [&path]{auto const pos = path.rfind(".");if(pos == beast::string_view::npos)return beast::string_view{};return path.substr(pos);}();if(iequals(ext, ".htm")) return "text/html";if(iequals(ext, ".html")) return "text/html";if(iequals(ext, ".php")) return "text/html";if(iequals(ext, ".css")) return "text/css";if(iequals(ext, ".txt")) return "text/plain";if(iequals(ext, ".js")) return "application/javascript";if(iequals(ext, ".json")) return "application/json";if(iequals(ext, ".xml")) return "application/xml";if(iequals(ext, ".swf")) return "application/x-shockwave-flash";if(iequals(ext, ".flv")) return "video/x-flv";if(iequals(ext, ".png")) return "image/png";if(iequals(ext, ".jpe")) return "image/jpeg";if(iequals(ext, ".jpeg")) return "image/jpeg";if(iequals(ext, ".jpg")) return "image/jpeg";if(iequals(ext, ".gif")) return "image/gif";if(iequals(ext, ".bmp")) return "image/bmp";if(iequals(ext, ".ico")) return "image/vnd.microsoft.icon";if(iequals(ext, ".tiff")) return "image/tiff";if(iequals(ext, ".tif")) return "image/tiff";if(iequals(ext, ".svg")) return "image/svg+xml";if(iequals(ext, ".svgz")) return "image/svg+xml";return "application/text";
}// Append an HTTP rel-path to a local filesystem path.
// The returned path is normalized for the platform.
std::string
path_cat(beast::string_view base,beast::string_view path)
{if(base.empty())return std::string(path);std::string result(base);
#ifdef BOOST_MSVCchar constexpr path_separator = '\\';if(result.back() == path_separator)result.resize(result.size() - 1);result.append(path.data(), path.size());for(auto& c : result)if(c == '/')c = path_separator;
#elsechar constexpr path_separator = '/';if(result.back() == path_separator)result.resize(result.size() - 1);result.append(path.data(), path.size());
#endifreturn result;
}// This function produces an HTTP response for the given
// request. The type of the response object depends on the
// contents of the request, so the interface requires the
// caller to pass a generic lambda for receiving the response.
template<class Body, class Allocator,class Send>
void
handle_request(beast::string_view doc_root,http::request<Body, http::basic_fields<Allocator>>&& req,Send&& send)
{// Returns a bad request responseauto const bad_request =[&req](beast::string_view why){http::response<http::string_body> res{http::status::bad_request, req.version()};res.set(http::field::server, BOOST_BEAST_VERSION_STRING);res.set(http::field::content_type, "text/html");res.keep_alive(req.keep_alive());res.body() = std::string(why);res.prepare_payload();return res;};// Returns a not found responseauto const not_found =[&req](beast::string_view target){http::response<http::string_body> res{http::status::not_found, req.version()};res.set(http::field::server, BOOST_BEAST_VERSION_STRING);res.set(http::field::content_type, "text/html");res.keep_alive(req.keep_alive());res.body() = "The resource '" + std::string(target) + "' was not found.";res.prepare_payload();return res;};// Returns a server error responseauto const server_error =[&req](beast::string_view what){http::response<http::string_body> res{http::status::internal_server_error, req.version()};res.set(http::field::server, BOOST_BEAST_VERSION_STRING);res.set(http::field::content_type, "text/html");res.keep_alive(req.keep_alive());res.body() = "An error occurred: '" + std::string(what) + "'";res.prepare_payload();return res;};// Make sure we can handle the methodif (req.method() != http::verb::get &&req.method() != http::verb::head){if (req.method() == http::verb::post){std::cout << req.target() << std::endl;/* string body = req.body();std::cout << "the body is " << body << std::endl;*/std::string lines = req.body();std::cout << lines << std::endl;http::response<http::empty_body> res{ http::status::ok, req.version() };res.set(http::field::server, BOOST_BEAST_VERSION_STRING);res.set(http::field::content_type, mime_type("./"));res.content_length(0);res.keep_alive(req.keep_alive());return send(std::move(res));//return send(bad_request("Unknown HTTP-method"));}else{std::cerr << "unknown http method\n";return send(bad_request("Unknown HTTP-method"));}}// Request path must be absolute and not contain "..".if( req.target().empty() ||req.target()[0] != '/' ||req.target().find("..") != beast::string_view::npos)return send(bad_request("Illegal request-target"));// Build the path to the requested filestd::string path = path_cat(doc_root, req.target());if(req.target().back() == '/')path.append("index.html");// Attempt to open the filebeast::error_code ec;http::file_body::value_type body;body.open(path.c_str(), beast::file_mode::scan, ec);// Handle the case where the file doesn't existif(ec == beast::errc::no_such_file_or_directory)return send(not_found(req.target()));// Handle an unknown errorif(ec)return send(server_error(ec.message()));// Cache the size since we need it after the moveauto const size = body.size();// Respond to HEAD requestif(req.method() == http::verb::head){http::response<http::empty_body> res{http::status::ok, req.version()};res.set(http::field::server, BOOST_BEAST_VERSION_STRING);res.set(http::field::content_type, mime_type(path));res.content_length(size);res.keep_alive(req.keep_alive());return send(std::move(res));}// Respond to GET requesthttp::response<http::file_body> res{std::piecewise_construct,std::make_tuple(std::move(body)),std::make_tuple(http::status::ok, req.version())};res.set(http::field::server, BOOST_BEAST_VERSION_STRING);res.set(http::field::content_type, mime_type(path));res.content_length(size);res.keep_alive(req.keep_alive());return send(std::move(res));
}//------------------------------------------------------------------------------// Report a failure
void
fail(beast::error_code ec, char const* what)
{std::cerr << what << ": " << ec.message() << "\n";
}// This is the C++11 equivalent of a generic lambda.
// The function object is used to send an HTTP message.
struct send_lambda
{beast::tcp_stream& stream_;bool& close_;beast::error_code& ec_;net::yield_context yield_;send_lambda(beast::tcp_stream& stream,bool& close,beast::error_code& ec,net::yield_context yield): stream_(stream), close_(close), ec_(ec), yield_(yield){}template<bool isRequest, class Body, class Fields>voidoperator()(http::message<isRequest, Body, Fields>&& msg) const{// Determine if we should close the connection afterclose_ = msg.need_eof();// We need the serializer here because the serializer requires// a non-const file_body, and the message oriented version of// http::write only works with const messages.http::serializer<isRequest, Body, Fields> sr{msg};http::async_write(stream_, sr, yield_[ec_]);}
};// Handles an HTTP server connection
void
do_session(beast::tcp_stream& stream,std::shared_ptr<std::string const> const& doc_root,net::yield_context yield)
{bool close = false;beast::error_code ec;// This buffer is required to persist across readsbeast::flat_buffer buffer;// This lambda is used to send messagessend_lambda lambda{stream, close, ec, yield};for(;;){// Set the timeout.stream.expires_after(std::chrono::seconds(30));// Read a requesthttp::request<http::string_body> req;http::async_read(stream, buffer, req, yield[ec]);if(ec == http::error::end_of_stream)break;if(ec)return fail(ec, "read");// Send the responsehandle_request(*doc_root, std::move(req), lambda);if(ec)return fail(ec, "write");if(close){// This means we should close the connection, usually because// the response indicated the "Connection: close" semantic.break;}}// Send a TCP shutdownstream.socket().shutdown(tcp::socket::shutdown_send, ec);// At this point the connection is closed gracefully
}//------------------------------------------------------------------------------// Accepts incoming connections and launches the sessions
void
do_listen(net::io_context& ioc,tcp::endpoint endpoint,std::shared_ptr<std::string const> const& doc_root,net::yield_context yield)
{beast::error_code ec;// Open the acceptortcp::acceptor acceptor(ioc);acceptor.open(endpoint.protocol(), ec);if(ec)return fail(ec, "open");// Allow address reuseacceptor.set_option(net::socket_base::reuse_address(true), ec);if(ec)return fail(ec, "set_option");// Bind to the server addressacceptor.bind(endpoint, ec);if(ec)return fail(ec, "bind");// Start listening for connectionsacceptor.listen(net::socket_base::max_listen_connections, ec);if(ec)return fail(ec, "listen");for(;;){tcp::socket socket(ioc);acceptor.async_accept(socket, yield[ec]);if(ec)fail(ec, "accept");elsenet::spawn(acceptor.get_executor(),std::bind(&do_session,beast::tcp_stream(std::move(socket)),doc_root,std::placeholders::_1));}
}int main(int argc, char* argv[])
{auto const address = net::ip::make_address("0.0.0.0");auto const port = static_cast<unsigned short>(std::atoi("8080"));auto const doc_root = std::make_shared<std::string>("./");auto const threads = std::max<int>(1, std::atoi("4"));// The io_context is required for all I/Onet::io_context ioc{threads};// Spawn a listening portnet::spawn(ioc,std::bind(&do_listen,std::ref(ioc),tcp::endpoint{address, port},doc_root,std::placeholders::_1));// Run the I/O service on the requested number of threadsstd::vector<std::thread> v;v.reserve(threads - 1);for(auto i = threads - 1; i > 0; --i)v.emplace_back([&ioc]{ioc.run();});ioc.run();return EXIT_SUCCESS;
}
python post 测试
post 客户端
import json
import requests
import time
headers = {'Content-Type': 'application/json'}
data = {"projectname":"four screen","picnum":8,"memo":"test"
}
try:r = requests.post("http://127.0.0.1:8080/test", json=data, headers=headers)print(r.text)
except requests.exceptions.ConnectionError:print('connectionError')
time.sleep(1)
get
用浏览器就行,写一个http index.html
浏览器打开8080 端口
下载文件
直接可以下载文件,比如放入一个rar压缩文件,也就是完成了一个http file server,也是可以接收post 数据,后面可以自行扩展
websocket
可以看我另外一个文章boost bease websocket协议
相关文章:
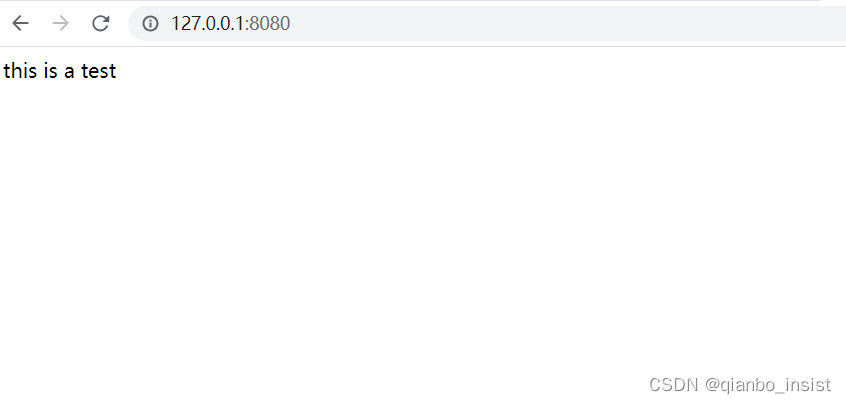
boost beast http server 测试
boost beast http client boost http server boost beast 是一个非常好用的库,带上boost的协程,好很多东西是比较好用的,以下程序使用四个线程开启协程处理进入http协议处理。协议支持http get 和 http post #include <boost/beast/cor…...

Android 10.0 系统开启禁用adb push和adb pull传输文件功能
1.使用场景 在进行10.0的系统开发中,在一些产品中由于一些开发的功能比较重要,防止技术点外泄在出货产品中,禁用 adb pull 和adb push等命令 来获取系统system下的jar 和apk 等文件,所以需要禁用这些命令 2.系统开启禁用adb push和adb pull传输文件功能的分析 看了下系统…...

浙大数据结构第七周之07-图4 哈利·波特的考试
基础知识:(最短路的前提都是在图中两条边之间的权值非定值) (一)Dijkstra方法 算法实现: …...
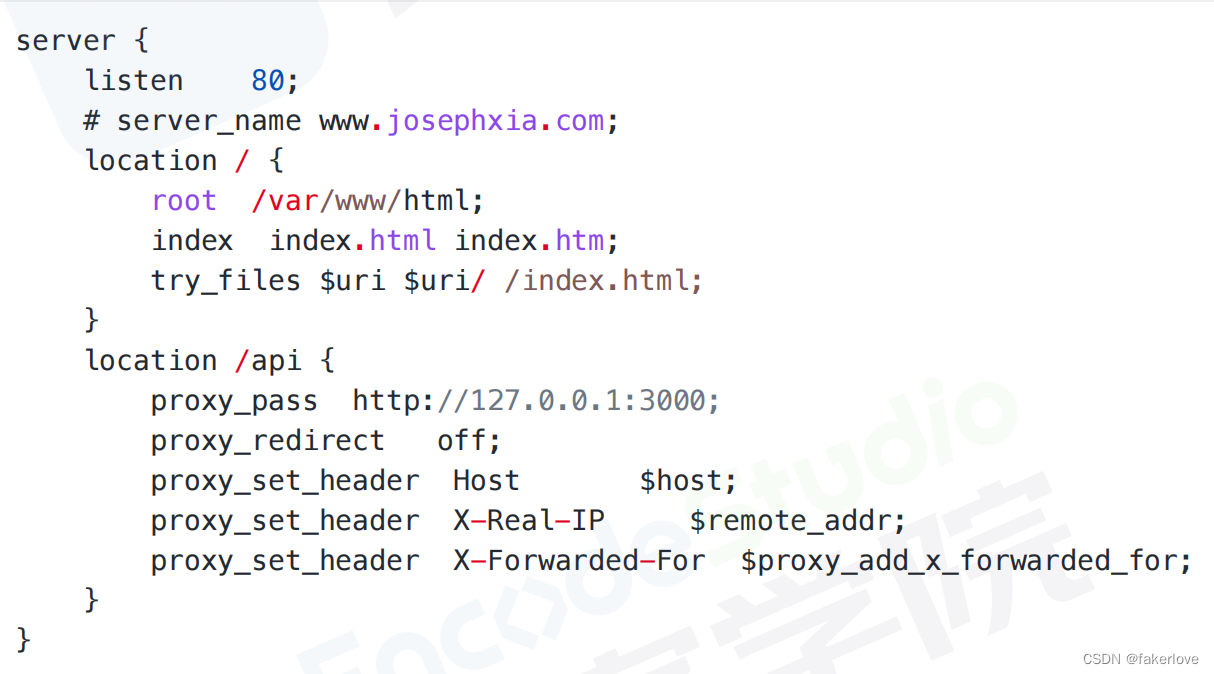
vue2-vue项目中你是如何解决跨域的?
1、跨域是什么? 跨域本质是浏览器基于同源策略的一种安全手段。 同源策略(sameoriginpolicy),是一种约定,它是浏览器最核心也是最基本的安全功能。 所谓同源(即指在同一个域)具有以下三个相同点…...
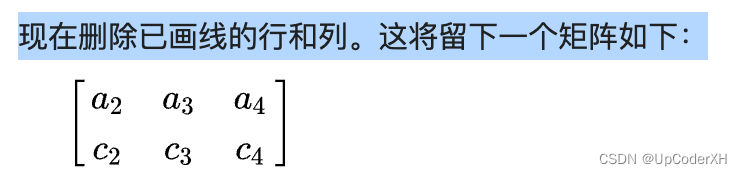
【Paper Reading】DETR:End-to-End Object Detection with Transformers
背景 Transformer已经在NLP领域大展拳脚,逐步替代了LSTM/GRU等相关的Recurrent Neural Networks,相比于传统的RNN,Transformer主要具有以下几点优势 可解决长时序依赖问题,因为Transformer在计算attention的时候是在全局维度进行…...
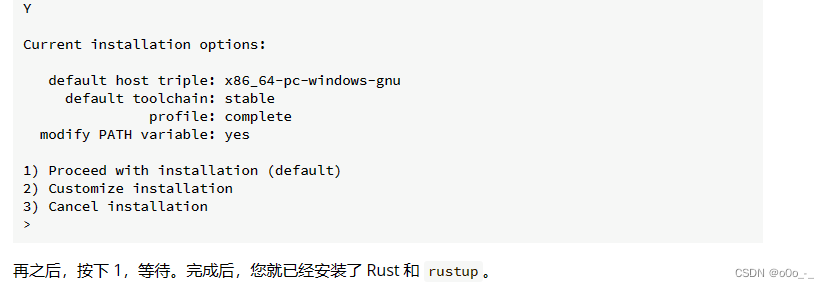
【rust/入门】windows安装rust gnu环境(折腾)
说在前面 首先说明,我是rust入门选手,之前都是在wsl写rust,突然想在windows下装下rust。windows版本:windows11 22H2原文换源 心路历程 看到教程我陷入了沉默,(官方推荐) 打开Microsoft C Build Tools我开始不解&…...

java面试---字符串相关内容
字符串 1. 什么是Java中的字符串池(String Pool)?2. String、StringBuilder和StringBuffer之间的区别是什么?3. 如何比较两个字符串的内容是否相等?4、equals和的区别5. String类有哪些常用的方法? 1. 什么…...
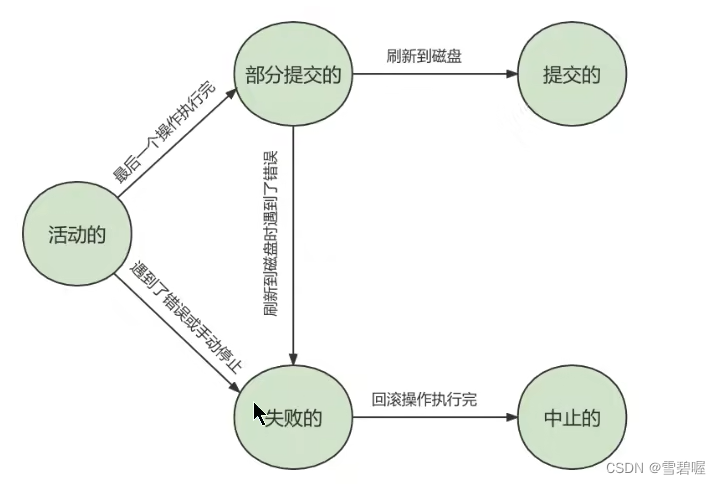
MYSQL进阶-事务的基础知识
1.什么是数据库事务? 就是把好几个sql语句打包成一个整体执行,要么全部成功,要么全部失败!!! 事务是一个不可分割的数据库操作序列,也是数据库并发控制的基本单位,其执 行的结果必…...
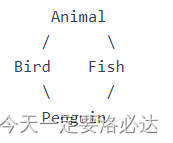
【C++】C++面向对象,泛型编程总结篇(封装,继承,多态,模板)|(秋招篇)
文章目录 前言如何理解面向对象?如何理解泛型编程?C面向对象的三大特性是什么构造函数有哪几种?讲一下移动构造函数当我们定义一个类 系统会自动帮我们生成哪些函数?标题讲一下类中三类成员(公有私有保护)三…...
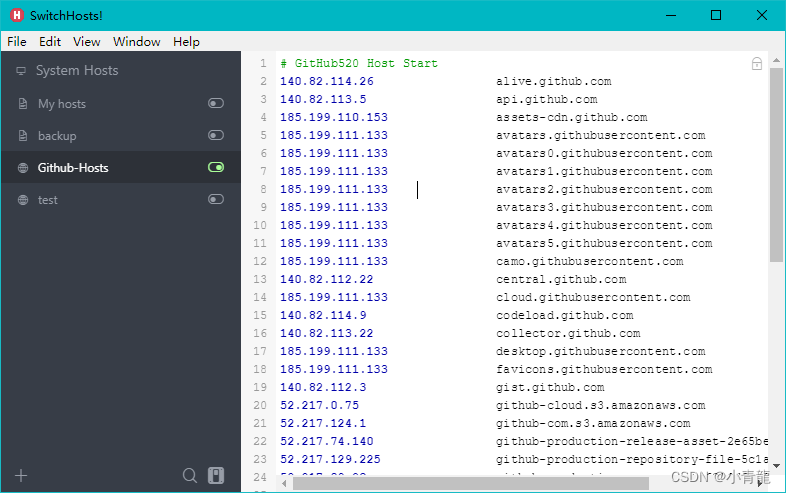
【Github】作为程序员不得不知道的几款Github加速神器
文章目录 背景推荐1:FastGithub推荐2:dev-sidecar推荐3:Watt Toolkit推荐4:篡改猴插件用户脚本1)下载安装-->篡改猴 Tampermonkey 插件2)下载安装-->Github 增强 - 高速下载 用户脚本 推荐5ÿ…...

react18之08自定义hook (简单的axios-get、修改浏览器title、localStorage、获取滚动条位置、img转换为base64)
目录 react18之自定义hook ()01:自定义一个 简单的axios hook 发起get请求useHttp.jsx使用useHttp hook效果 02:自定义一个 修改浏览器title hook03:自定义一个 localStorage(获取、存储、移除) hookuseLocalStorage.jsx使用hook效果 04&…...

对CommonJS、AMD、CMD、ES Module的理解
CommonJS 常用于:服务器端,node,webpack 特点:同步/运行时加载,磁盘读取速度快 语法: // 1. 导出:通过module.exports或exports来暴露模块 module.exports { attr1, attr2 } ex…...

JVM之类加载与字节码(二)
3. 编译期处理 什么是语法糖 所谓的 语法糖 ,其实就是指 java 编译器把 *.java 源码编译为 *.class 字节码的过程中,自动生成 和转换的一些代码,主要是为了减轻程序员的负担,算是 java 编译器给我们的一个额外福利(给…...
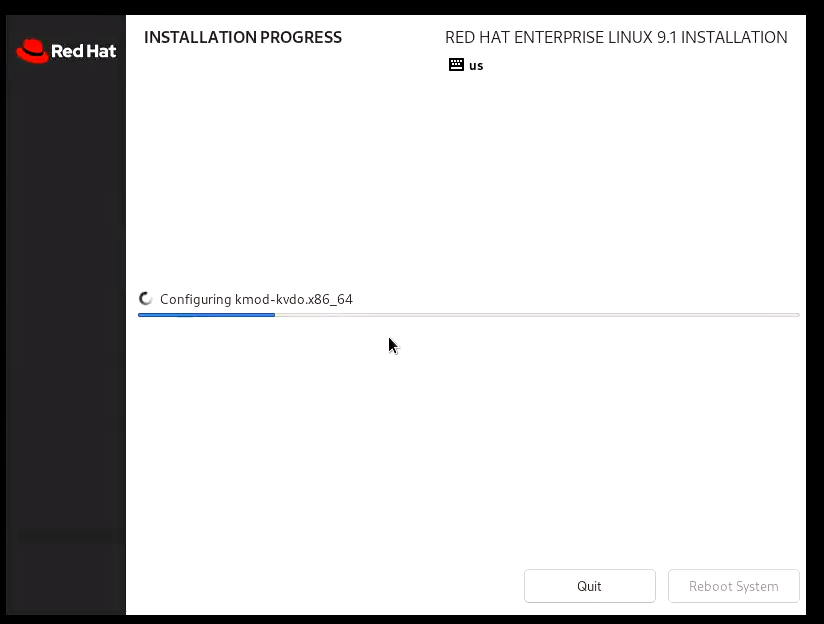
安装linux操作系统
安装虚拟机的步骤: 安装linux系统 之后开启虚拟机 之后重启,打开虚拟机,登录root账号...
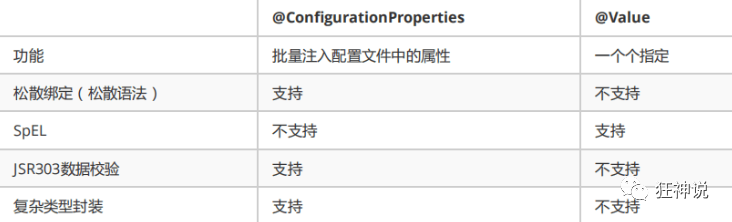
【SpringBoot】知识
.第一个程序HelloWorld 项目创建方式:使用 IDEA 直接创建项目 1、创建一个新项目 2、选择spring initalizr , 可以看到默认就是去官网的快速构建工具那里实现 3、填写项目信息 4、选择初始化的组件(初学勾选 Web 即可) 5、填…...
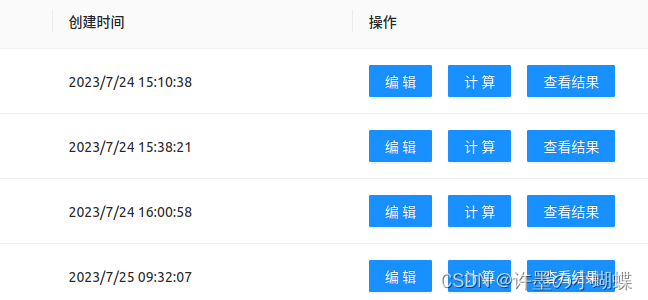
react ant add/change created_at
1.引入ant的 Table import { Table, Space, Button, message } from antd; 2.获得接口的数据的时候增加上创建时间 const response await axios.get(${Config.BASE_URL}/api/v1/calculation_plans?token${getToken()});if (response.data.message ok) {const data respon…...
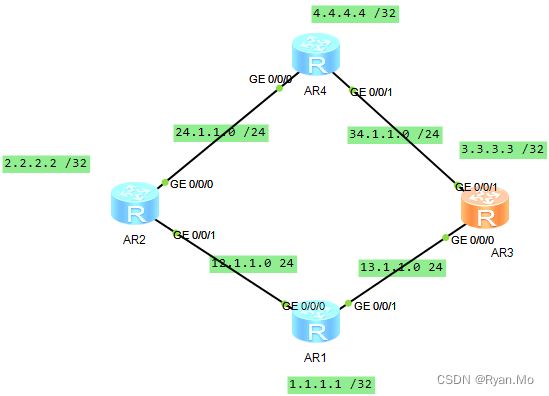
OSPF 动态路由协议 路由传递
影响OSPF路由选择的因素: 1.OSPF路由的开销值:宽带参考值默认为100. COST1000/接口带宽。此时接口 带宽的值可更改,更改后只改变参考数值,带宽仍然为初始值。 注意:更改COST需要 在路由的入方向,数据的出方…...

5.kubeadm安装
文章目录 kubeadm部署环境初始化所有的节点安装Docker所有节点安装kubeadm,kubelet和kubectl初始化方法一,配置文件初始化方法二,命令初始化 网络插件node节点总结 证书过期方法一方法二总结 部署Dashboard kubeadm部署 环境初始化 ###所有…...
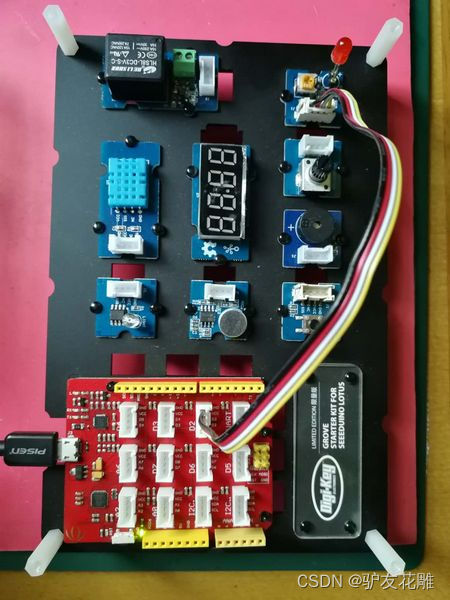
【雕爷学编程】Arduino动手做(180)---Seeeduino Lotus开发板2
37款传感器与执行器的提法,在网络上广泛流传,其实Arduino能够兼容的传感器模块肯定是不止这37种的。鉴于本人手头积累了一些传感器和执行器模块,依照实践出真知(一定要动手做)的理念,以学习和交流为目的&am…...

6.5 池化层
是什么:池化层跟卷积层类似有个滑动窗口,用来取一个区域内的最大值或者平均值。 作用:卷积神经网络的最后的部分应该要看到整个图像的全局,通过池化(汇聚)操作,逐渐汇聚要取的像素,最终实现学习全局表示的…...
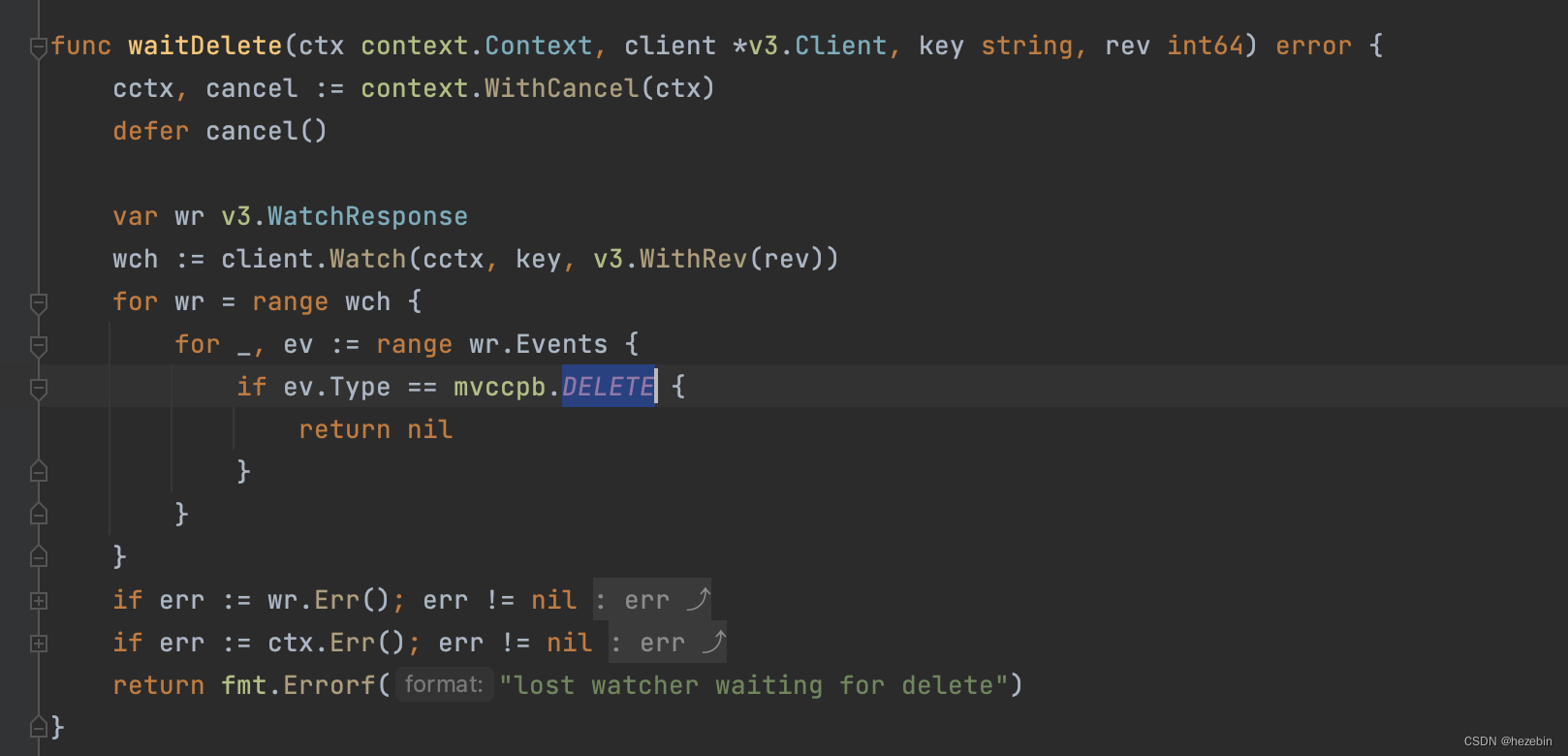
etcd
文章目录 etcd单机安装设置键值对watch操作读取键过往版本的值压缩修订版本lease租约(过期机制)授予租约撤销租约keepAlive续约获取租约信息 事务基于etcd实现分布式锁原生实现官方 concurrency 包实现 服务注册与发现Go 操作 Etcd 参考 etcd etcd 是一…...

W5500-EVB-PICO做DNS Client进行域名解析(四)
前言 在上一章节中我们用W5500-EVB-PICO通过dhcp获取ip地址(网关,子网掩码,dns服务器)等信息,给我们的开发板配置网络信息,成功的接入网络中,那么本章将教大家如何让我们的开发板进行DNS域名解析…...
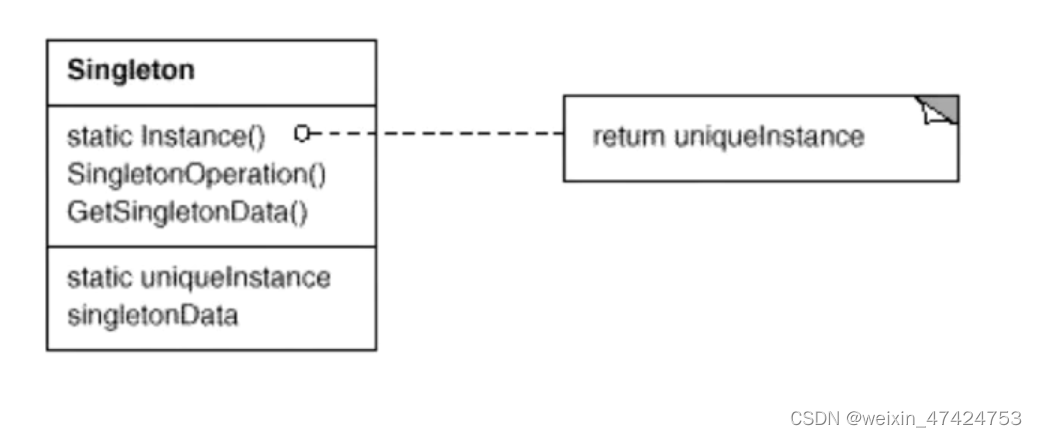
单例模式(C++)
定义 保证一个类仅有一个实例,并提供一个该实例的全局访问点。 应用场景 在软件系统中,经常有这样一些特殊的类,必须保证它们在系统中只存在一个实例,才能确保它们的逻辑正确性、以及良好的效率。如何绕过常规的构造器,提供一种…...
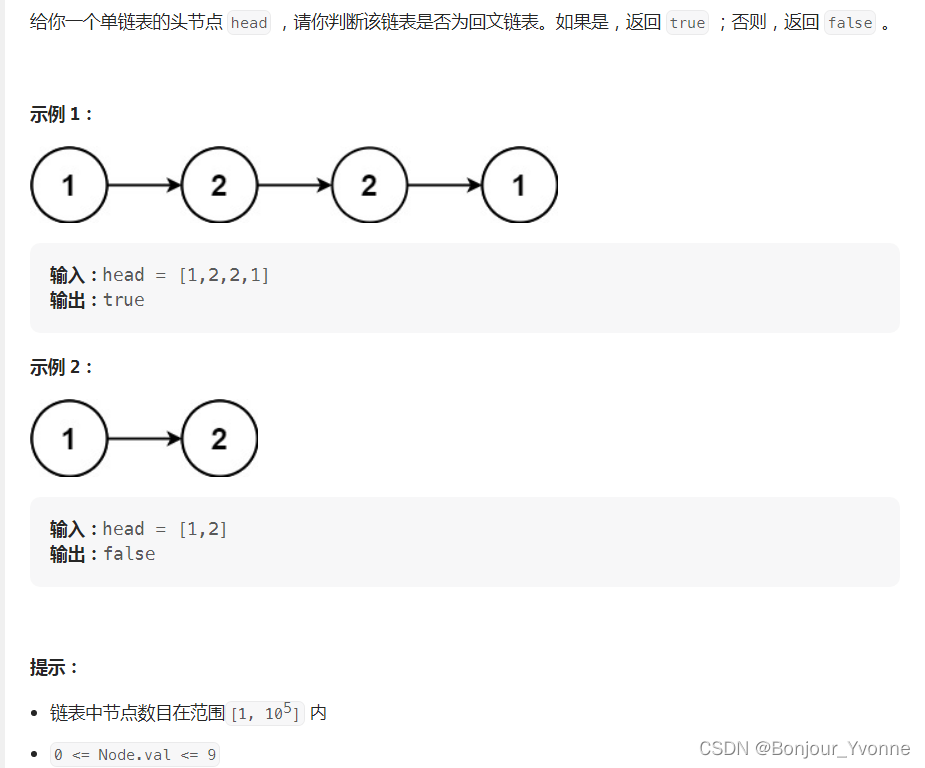
LeetCode 热题 100 JavaScript--234. 回文链表
function ListNode(val, next) {this.val val undefined ? 0 : val;this.next next undefined ? null : next; }var isPalindrome function (head) {if (!head || !head.next) {return true; }// 使用快慢指针法找到链表的中间节点let slow head;let fast head;while …...
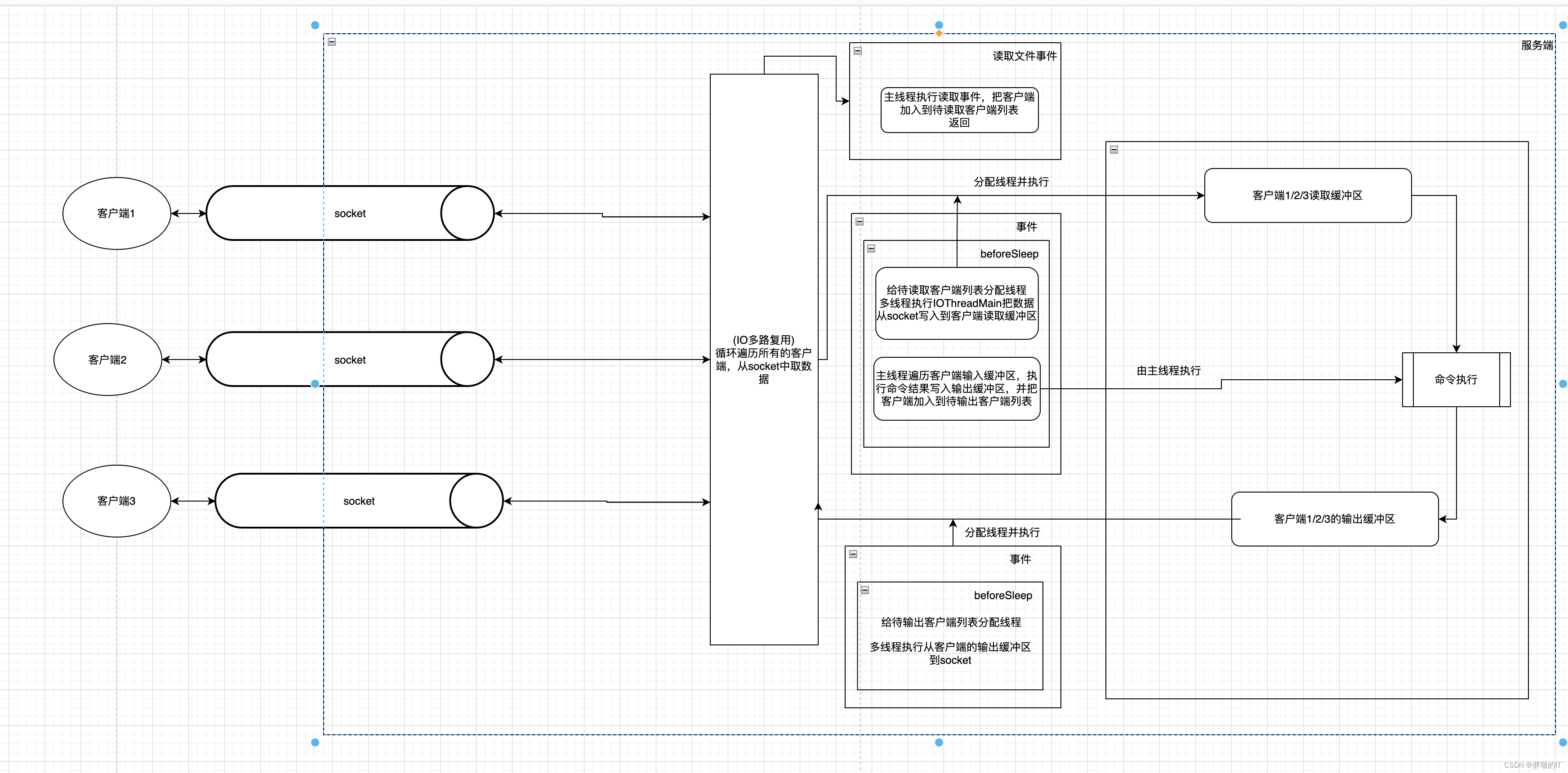
Redis 6.5 服务端开启多线程源码
redis支持开启多线程,只有从socket到读取缓冲区和从输出缓冲区到socket这两段过程是多线程,而命令的执行还是单线程,并且是由主线程执行 借鉴:【Redis】事件驱动框架源码分析(多线程) 一、main启动时初始化…...

嵌入式面试笔试刷题(day6)
文章目录 前言一、进程和线程的区别二、共享内存的原理三、中断有传参和返回值吗四、串口数据帧格式五、进程通信有几种,哪几种需要借助内核1.方式2.需要借助内核的 六、flash有哪几种类型七、指针的本质是什么八、指针和数组的区别九、使用宏定义交换变量不能使用中…...
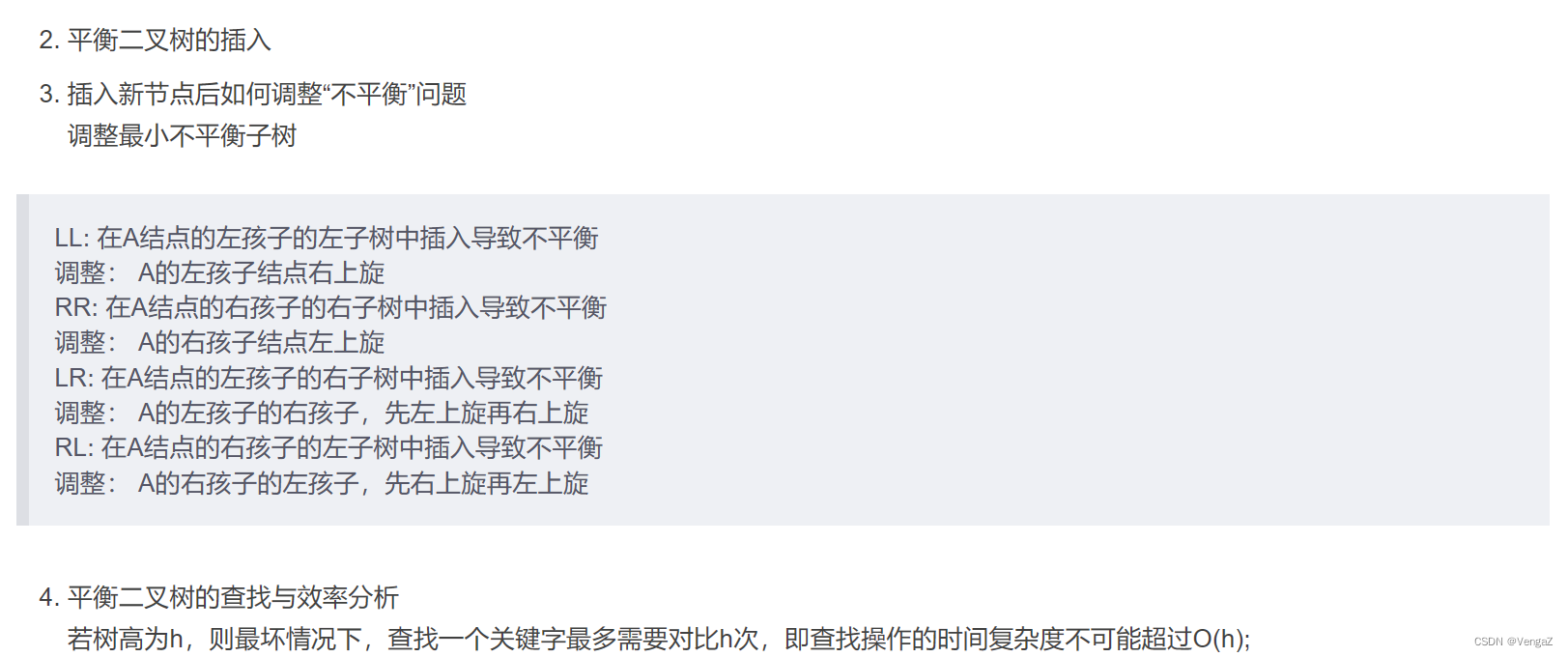
24考研数据结构-第五章:树与二叉树
目录 第五章:树5.1树的基本概念5.1.1树的定义5.1.2 基本术语5.1.3 树的性质 5.2二叉树的概念5.2.1 二叉树的定义与特性5.2.2 几种特殊的二叉树5.2.3 二叉树的性质5.2.4 完全二叉树的性质5.2.5 二叉树的存储结构1. 顺序存储重要的基本操作非完全二叉树2. 链式存储逆向…...

构建稳健的微服务架构:关键的微服务设计原则和最佳实践
在现代软件开发中,微服务架构正逐渐成为构建复杂应用程序的首选方法之一。微服务架构的核心理念是将应用程序划分为一系列小型、自治的服务,每个服务专注于一个特定的业务功能。然而,要实现一个稳健的微服务架构并不仅仅是将功能拆分成微服务…...
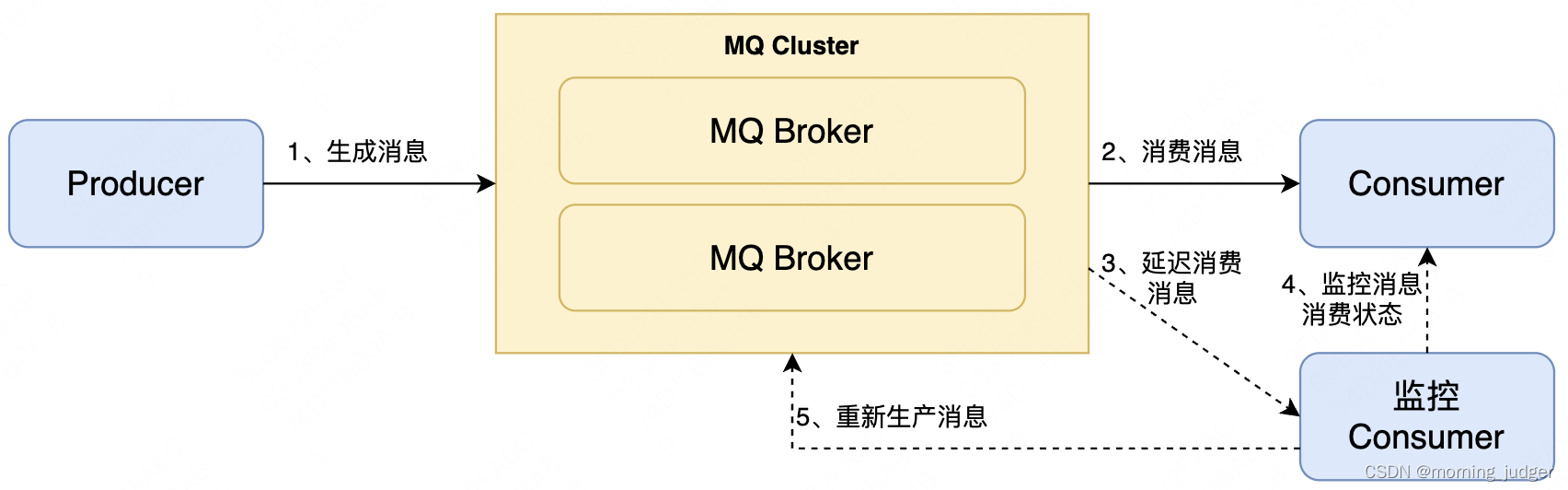
消息队列常见问题(1)-如何保障不丢消息
目录 1. 为什么消息队列会丢消息? 2. 怎么保障消息可靠传递? 2.1 生产者不丢消息 2.2 服务端不丢消息 2.3 消费者不丢消息 3. 消息丢失如何快速止损? 3.1 完善监控 3.2 完善止损工具 1. 为什么消息队列会丢消息? 现在主流…...

Circle of Mistery 2023牛客暑期多校训练营5 B
登录—专业IT笔试面试备考平台_牛客网 题目大意:给出一个n个数的数组a,求一个排列,使其形成的其中一个置换环上的数的和>k,并使产生的逆序对数量最少 1<n<1e3;-1e6<k<1e6;-1e6<ai<1e6 tips:关于置换环是什…...