C++详解
//7.用new建立一个动态一维数组,并初始化int[10]={1,2,3,4,5,6,7,8,9,10},用指针输出,最后销毁数组所占空间。
#include<iostream>
#include<string>
using namespace std;
int main()
{
int *p;
p=new int[10];
for(int i=1;i<=10;i++)
{
*(p+i-1)=i;
cout<<*(p+i-1)<<" ";
}
delete []p;
return 0;
}
//21.某商店经销一种货物,货物成箱购进,成箱卖出,购进和卖出时以重量为单位,各箱的重量不一样,
//因此,商店需要记下目前库存货物的总量,要求把商店货物购进和卖出的情况模拟出来。
#include<iostream>
using namespace std;
class Goods
{
public:
Goods ( int w)
{
weight = w;
totalWeight += w;
}
~Goods ( )
{
totalWeight -= weight;
}
int Weight()
{
return weight;
}
static int TotalWeight ( )
{
return totalWeight;
}
private:
int weight;
static int totalWeight;
};
int Goods::totalWeight=0;
int main ( )
{
int w ;
cin >>w ;
Goods *g1=new Goods(w) ;
cin >> w ;
Goods *g2=new Goods(w) ;
cout << Goods::TotalWeight() << endl ;
delete g2;
cout << Goods::TotalWeight() << endl ;
return 0;
}
虚函数
//19.写一个程序,定义抽象类型Shape,由他派生三个类:Circle(圆形),Rectangle(矩形),Trapezoid(梯形),
//用一个函数printArea分别输出三者的面积,3个图形的数据在定义对象是给定。
#include <iostream>
using namespace std;
class Shape
{
public:
virtual double area() const =0;
};
class Circle:public Shape
{
public:
Circle(double r):radius(r){}
virtual double area() const {return 3.14159*radius*radius;};
protected:
double radius;
};
class Rectangle:public Shape
{
public:
Rectangle(double w,double h):width(w),height(h){}
virtual double area() const {return width*height;}
protected:
double width,height;
};
class Trapezoid:public Shape
{
public:
Trapezoid(double w,double h,double len):width(w),height(h),length(len){}
virtual double area() const {return 0.5*height*(width+length);}
protected:
double width,height,length;
};
void printArea(const Shape &s)
{
cout<<s.area()<<endl;
}
int main()
{
Circle circle(12.6);
cout<<"area of circle =";
printArea(circle);
Rectangle rectangle(4.5,8.4);
cout<<"area of rectangle =";
printArea(rectangle);
Trapezoid trapezoid(4.5,8.4,8.0);
cout<<"area of trapezoid =";
printArea(trapezoid);
return 0;
}
//4.声明一个类模板,利用它分别实现两个整数、浮点数和字符的比较,求出大数和小数。
#include <iostream>
using namespace std;
template<class numtype>
class Compare
{
public:
Compare(numtype a,numtype b)
{
x=a;
y=b;
}
numtype max()
{
return (x>y)?x:y;
}
numtype min()
{
return (x<y)?x:y;
}
private:
numtype x,y;
};
int main()
{
Compare<int> cmp1(3,7);
cout<<cmp1.max()<<" is the Maximum of two inteder numbers."<<endl;
cout<<cmp1.min()<<" is the Minimum of two inteder numbers."<<endl<<endl;
Compare<float> cmp2(45.78,93.6);
cout<<cmp2.max()<<" is the Maximum of two float numbers."<<endl;
cout<<cmp2.min()<<" is the Minimum of two float numbers."<<endl<<endl;
Compare<char> cmp3('a','A');
cout<<cmp3.max()<<" is the Maximum of two characters."<<endl;
cout<<cmp3.min()<<" is the Minimum of two characters."<<endl;
return 0;
}
//6.实现重载函数Double(x),返回值为输人参数的两倍;参数分别为整型、浮点型、双精度型,返回值类型与参数一样。(用类模板实现)
#include<iostream>
using namespace std;
template<class numtype>
class Double
{
public:
Double(numtype a)
{
x=a;
}
numtype bei()
{
return 2*x;
}
private:
numtype x;
};
int main()
{
Double<int>dou1(3);
cout<<dou1.bei()<<endl;
Double<float>dou2(12.36);
cout<<dou2.bei()<<endl;
Double<double>dou3(25.33333);
cout<<dou3.bei()<<endl;
return 0;
}
//5.建立一个对象数组,内放5个学生的数据(学号、成绩),用指针指向数组首元素,输出第1,3,5个学生的数据。初值自拟。
#include <iostream>
using namespace std;
class Student
{
public:
Student(int n,float s):num(n),score(s){}
void display();
private:
int num;
float score;
};
void Student::display()
{
cout<<num<<" "<<score<<endl;
}
int main()
{
Student stud[5]=
{
Student(101,78.5),
Student(102,85.5),
Student(103,98.5),
Student(104,100.0),
Student(105,95.5)
};
Student *p=stud;
for(int i=0;i<=4;p=p+1,i++)
p->display();
return 0;
}
//21.某商店经销一种货物,货物成箱购进,成箱卖出,购进和卖出时以重量为单位,各箱的重量不一样,
//因此,商店需要记下目前库存货物的总量,要求把商店货物购进和卖出的情况模拟出来。
#include<iostream>
using namespace std;
class Goods
{
public:
Goods ( int w)
{
weight = w;
totalWeight += w;
}
~Goods ( )
{
totalWeight -= weight;
}
int Weight()
{
return weight;
}
static int TotalWeight ( )
{
return totalWeight;
}
private:
int weight;
static int totalWeight;
};
int Goods::totalWeight=0;
int main ( )
{
int w ;
cin >>w ;
Goods *g1=new Goods(w) ;
cin >> w ;
Goods *g2=new Goods(w) ;
cout << Goods::TotalWeight() << endl ;
delete g2;
cout << Goods::TotalWeight() << endl ;
return 0;
}
文件的输入输出/*编程序实现以下功能:
(1)按职工号由小到大的顺序将5个员工的数据(包括号码,姓名,年龄,工资)输出到磁盘文件中保存
(2)从键盘输入两个员工的数据(职工号大于已有的职工号),增加到文件的末尾。
(3)输入文件中全部职工的数据
(4)从键盘输入一个号码,从文件中查找有无此职工号,如有则显示此职工是第几个职工,以及此职工的全部数据。如没有,就输出"无此人"。可以反复多次查询,如果输入查找的职工号是0,就结束查询。
*/
#include <iostream>
#include <fstream>
using namespace std;
struct staff
{
int num;
char name[20];
int age;
double pay;
};
int main()
{
staff staf[7]={2101,"Li",34,1203,
2104,"Wang",23,674.5,
2108,"Fun",54,778,
3006,"Xue",45,476.5,
5101,"Ling",39,656.6
}
,staf1;
fstream iofile("staff.dat",ios::in|ios::out|ios::binary);
if(!iofile)
{
cerr<<"open error!"<<endl;
abort();
}
int i,m,num;
cout<<"Five staff :"<<endl;
for(i=0;i<5;i++)
{
cout<<staf[i].num<<" "<<staf[i].name<<" "<<staf[i].age<<" "<<staf[i].pay<<endl;
iofile.write((char *)&staf[i],sizeof(staf[i]));
}
cout<<"please input data you want insert:"<<endl;
for(i=0;i<2;i++)
{
cin>>staf1.num>>staf1.name>>staf1.age>>staf1.pay;
iofile.seekp(0,ios::end);
iofile.write((char *)&staf1,sizeof(staf1));
}
iofile.seekg(0,ios::beg);
for(i=0;i<7;i++)
{
iofile.read((char *)&staf[i],sizeof(staf[i]));
cout<<staf[i].num<<" "<<staf[i].name<<" "<<staf[i].age<<" "<<staf[i].pay<<endl;
}
bool find;
cout<<"enter number you want search,enter 0 to stop.";
cin>>num;
while(num)
{
find=false;
iofile.seekg(0,ios::beg);
for(i=0;i<7;i++)
{
iofile.read((char *)&staf[i],sizeof(staf[i]));
if(num==staf[i].num)
{
m=iofile.tellg();
cout<<num<<" is No."<<m/sizeof(staf1)<<endl;
cout<<staf[i].num<<" "<<staf[i].name<<" "<<staf[i].age<<" "<<staf[i].pay<<endl;
find=true;
break;
}
}
if(!find)
cout<<"can't find "<<num<<endl;
cout<<"enter number you want search,enter 0 to stop.";
cin>>num;
}
iofile.close();
return 0;}
运算符重载
//8.定义一个复数类Complex,重载运算符"+",使之能用于复数的加法运算。
//将运算符函数重载为非成员、非友元的普通函数。编写程序,求两个复数之和。初值自拟。
#include <iostream>
using namespace std;
class Complex
{
public:
Complex()
{
real=0;
imag=0;
}
Complex(double r,double i)
{
real=r;
imag=i;
}
double get_real();
double get_imag();
void display();
private:
double real;
double imag;
};
double Complex::get_real()
{
return real;
}
double Complex::get_imag()
{
return imag;
}
void Complex::display()
{
cout<<"("<<real<<","<<imag<<"i)"<<endl;
}
Complex operator+(Complex &c1,Complex &c2)
{
return Complex(c1.get_real()+c2.get_real(),c1.get_imag()+c2.get_imag());
}
int main()
{
Complex c1(3,4),c2(5,-10),c3;
c3=c1+c2;
cout<<"c3=";
c3.display();
return 0;
}
//9.定义一个复数类 Complex,重载运算符"+","-",使之能用于复数的加,减运算,
//运算符重载函数作为 Complex类的成员函数。编程序,分别求出两个复数之和,差。初值自拟。
#include<iostream>
using namespace std;
class Complex
{
public:
Complex()
{
real=0;
imag=0;
}
Complex(double r,double i)
{
real=r;
imag=i;
}
Complex operator+(Complex &c2);
Complex operator-(Complex &c2);
void display();
private:
double real;
double imag;
};
Complex Complex::operator+(Complex &c2)
{
Complex c;
c.real=real+c2.real;
c.imag=imag+c2.imag;
return c;
}
Complex Complex::operator-(Complex &c2)
{
Complex c;
c.real=real-c2.real;
c.imag=imag-c2.imag;
return c;
}
void Complex::display()
{
cout<<real<<","<<imag<<"i"<<endl;
}
int main()
{
Complex c1(3,4),c2(5,-10),c3;
c3=c1+c2;//隐式调用,实际上为c1.operator+(c2)
cout<<"c1+c2=";
c3.display();
c3=c1-c2;//隐式调用
cout<<"c1-c2=";
c3.display();
return 0;
}
//12.有两个矩阵a和b,均为2行3列。求两个矩阵之和。
//重载运算符"+",使之能用于矩阵相加。如c=a+b。初值自拟。
#include<iostream>
using namespace std;
class Matrix
{
public:
Matrix();
friend Matrix operator+(Matrix &,Matrix &);
void input();
void display();
private:
int mat[2][3];
};
Matrix::Matrix()
{
for(int i=0;i<2;i++)
for(int j=0;j<3;j++)
mat[i][j]=0;
}
void Matrix::input()
{
cout<<"input value of matrix:"<<endl;
for(int i=0;i<2;i++)
for(int j=0;j<3;j++)
cin>>mat[i][j];
}
Matrix operator+(Matrix &a,Matrix &b)
{
Matrix c;
for(int i=0;i<2;i++)
for(int j=0;j<3;j++)
{
c.mat[i][j]=a.mat[i][j]+b.mat[i][j];
}
return c;
}
void Matrix::display()
{
for(int i=0;i<2;i++)
{
for(int j=0;j<3;j++)
{
cout<<mat[i][j]<<" ";
}
cout<<endl;
}
}
int main()
{
Matrix a,b,c;
a.input();
b.input();
cout<<endl<<"Matrix a:"<<endl;
a.display();
cout<<endl<<"Matrix b:"<<endl;
b.display();
c=a+b;
cout<<endl<<"Matrix c = Matrix a + Matrix b :"<<endl;
c.display();
return 0;
}
//14. 定义一个字符串类String,用来存放不定长的字符串,重载运算符"=="
//用于两个字符串的等于比较运算。初值自拟。
#include<iostream>
#include<string.h>
using namespace std;
class String
{
public:
String()
{
p=NULL;
}
String(char *str);
friend bool operator==(String &string1,String &string2);
void display();
private:
char* p;
};
String::String(char *str)
{
p=str;
}
void String::display()
{
cout<<p;
}
bool operator==(String &string1,String &string2)
{
if(strcmp(string1.p,string2.p)==0)
return true;
else
return false;
}
void compare(String &string1,String &string2)
{
if(operator==(string1,string2)==1)
{
string1.display();
cout<<"=";
string2.display();
}
else
{
cout<<"not equal"<<endl;
}
}
int main()
{
String string1("Hell"),string2("Hello");
compare(string1,string2);
return 0;
}
//7.有一个Time类,包含数据成员minute(分)和sec(秒),模拟秒表,每次走一秒,满60秒进一分钟,此时秒又从0开始算。
//要求输出分和秒的值。初值自拟。
#include <iostream>
using namespace std;
class Time
{
public:
Time()
{
minute=0;
sec=0;
}
Time(int m,int s):minute(m),sec(s){}
Time operator++();
void display()
{
cout<<minute<<":"<<sec<<endl;
}
private:
int minute;
int sec;
};
Time Time::operator++()
{
if(++sec>=60)
{
sec-=60;
++minute;
}
return *this;
}
int main()
{
Time time1(34,0);
for (int i=0;i<61;i++)
{
++time1;
time1.display();
}
return 0;
}
//12.分别用成员函数和友元函数重载运算符,使对实型的运算符"="适用于复数运算。
#include <iostream>
using namespace std;
class complex
{
private:
double real;
double imag;
public:
complex(double r=0.0,double i=0.0){ real=r; imag=i ;}
friend complex operator-(complex c1,complex c2);
void display();
};
complex operator - (complex c1,complex c2)
{
return complex( c1.real-c2.real,c1.imag-c2.imag );
}
void complex::display()
{
cout<<"("<<real<<","<<imag<<"i)"<<endl;
}
int main()
{
complex c1(5,4),c2(2,10),c3,;
cout<<"cl=";
c1.display();
cout<<"c2=";
c2.display();
c3=c1-c2;
cout<<"c3=c1-c2=";
c3.display();
}
//13.分别用成员函数和友元函数重载运算符,使对实型的运算符"+" 适用于复数运算。
#include <iostream.h>
class complex
{
private:
double real;
double imag;
public:
complex(double r=0.0,double i=0.0)
{
real=r;
imag=i;
}
friend complex operator +(complex c1,complex c2);
void display();
};
complex operator + (complex c1,complex c2)
{
return complex(c1.real+c2.real,c1.imag+c2.imag );
}
void complex::display()
{
cout<<"("<<real<<","<<imag<<"i)"<<endl;
}
void main()
{
complex c1(5,4),c2(2,10),c3,;
cout<<"cl=";
c1.display();
cout<<"c2=";
c2.display();
c3=c1+c2;
cout<<"c3=c1+c2=";
c3.display();
}
//2.请编写程序,处理一个复数与一个double数相加的运算,结果存放在一个double型的变量d1中,输出d1的值,再以复数形式输出此值。
//定义Complex(复数)类,在成员函数中包含重载类型转换运算符:operator double(){ return real;}。初值自拟。
#include <iostream>
using namespace std;
class Complex
{
public:
Complex(){real=0;imag=0;}
Complex(double r){real=r;imag=0;}
Complex(double r,double i){real=r;imag=i;}
operator double(){return real;}
void display();
private:
double real;
double imag;
};
void Complex::display()
{
cout<<"("<<real<<", "<<imag<<")"<<endl;
}
int main()
{
Complex c1(3,4),c2;
double d1;
d1=2.5+c1;
cout<<"d1="<<d1<<endl;
c2=Complex(d1);
cout<<"c2=";
c2.display();
return 0;
}
//4.有一个Time类,包含数据成员minute(分)和sec(秒),模拟秒表,每次走一秒,满60秒进一分钟,此时秒又从0开始算。
//要求输出分和秒的值,且对后置自增运算符的重载。
#include <iostream>
using namespace std;
class Time
{
public:
Time(){minute=0;sec=0;}
Time(int m,int s):minute(m),sec(s){}
Time operator++();
Time operator++(int);
void display(){cout<<minute<<":"<<sec<<endl;}
private:
int minute;
int sec;
};
Time Time::operator++()
{
if(++sec>=60)
{
sec-=60;
++minute;
}
return *this;
}
Time Time::operator++(int)
{
Time temp(*this);
sec++;
if(sec>=60)
{
sec-=60;
++minute;
}
return temp;
}
int main()
{
Time time1(34,0),time2(35,0);
for(int i=0;i<61;i++)
{
++time1;
time1.display();
}
for(int j=0;j<61;j++)
{
time2++;
time2.display();
}
return 0;
}
组合、继承
//18.先建立一个Point(点)类,包含数据成员x,y(坐标点)。
//以它为基类,派生出一个Circle(圆)类,增加数据成员r(半径),
//再以Circle类为直接基类,派生出一个Cylinder(圆柱体)类,在增加数据成员h(高)。
//编写程序,重载运算符"<<"和">>",使之能够用于输出以上类对象。
#include <iostream>
using namespace std;
class Point
{
public:
Point(float=0,float=0);
void setPoint(float,float);
float getX() const {return x;}
float getY() const {return y;}
friend ostream & operator<<(ostream &,const Point &);
protected:
float x,y;
};
Point::Point(float a,float b)
{
x=a;
y=b;
}
void Point::setPoint(float a,float b)
{
x=a;
y=b;
}
ostream &operator<<(ostream &output,const Point &p)
{
output<<"["<<p.x<<","<<p.y<<"]"<<endl;
return output;
}
class Circle:public Point
{
public:
Circle(float x=0,float y=0,float r=0);
void setRadius(float);
float getRadius() const;
float area () const;
friend ostream &operator<<(ostream &,const Circle &);
protected:
float radius;
};
Circle::Circle(float a,float b,float r):Point(a,b),radius(r){}
void Circle::setRadius(float r)
{
radius=r;
}
float Circle::getRadius() const
{
return radius;
}
float Circle::area() const
{
return 3.14159*radius*radius;
}
ostream &operator<<(ostream &output,const Circle &c)
{
output<<"Center=["<<c.x<<","<<c.y<<"], r="<<c.radius<<", area="<<c.area()<<endl;
return output;
}
class Cylinder:public Circle
{
public:
Cylinder (float x=0,float y=0,float r=0,float h=0);
void setHeight(float);
float getHeight() const;
float area() const;
float volume() const;
friend ostream& operator<<(ostream&,const Cylinder&);
protected:
float height;
};
Cylinder::Cylinder(float a,float b,float r,float h)
:Circle(a,b,r),height(h){}
void Cylinder::setHeight(float h){height=h;}
float Cylinder::getHeight() const
{
return height;
}
float Cylinder::area() const
{
return 2*Circle::area()+2*3.14159*radius*height;
}
float Cylinder::volume() const
{
return Circle::area()*height;
}
ostream &operator<<(ostream &output,const Cylinder& cy)
{
output<<"Center=["<<cy.x<<","<<cy.y<<"], r="<<cy.radius<<", h="<<cy.height
<<"\narea="<<cy.area()<<", volume="<<cy.volume()<<endl;
return output;
}
int main()
{
Cylinder cy1(3.5,6.4,5.2,10);
cout<<"\noriginal cylinder:\nx="<<cy1.getX()<<", y="<<cy1.getY()<<", r=";
cout<<cy1.getRadius()<<", h="<<cy1.getHeight()<<"\narea="<<cy1.area();
cout<<", volume="<<cy1.volume()<<endl;
cy1.setHeight(15);
cy1.setRadius(7.5);
cy1.setPoint(5,5);
cout<<"\nnew cylinder:\n"<<cy1;
Point &pRef=cy1;
cout<<"\npRef as a point:"<<pRef;
Circle &cRef=cy1;
cout<<"\ncRef as a Circle:"<<cRef;
return 0;
}
//20.定义一个人员类Cperson,包括数据成员:姓名、编号、性别和用于输入输出的成员函数。
//在此基础上派生出学生类CStudent(增加成绩)和老师类Cteacher(增加教龄),并实现对学生和教师信息的输入输出。
#include<iostream>
#include<string.h>
using namespace std;
class CPerson
{
public:
void SetData(char *name, char *id, bool isman=1)
{
int n=strlen(name);
strncpy(pName, name, n);
pName[n]='\0';
n=strlen(id);
strncpy(pID,id,n);
pID[n]='\0';
bMan=isman;
}
void Output()
{
cout<<"姓名:"<<pName<<endl;
cout<<"编号:"<<pID<<endl;
char *str=bMan?"男":"女";
cout<<"性别:"<<str<<endl;
}
private:
char pName[20];
char pID[20];
bool bMan;
};
class CStudent:public CPerson
{
public:
void InputScore(double score1, double score2, double score3)
{
dbScore[0] = score1;
dbScore[1] = score2;
dbScore[2] = score3;
}
void Print()
{
Output();
for (int i=0; i<3; i++)
cout<<"成绩"<<i+1<<":"<<dbScore[i]<<endl;
}
private:
double dbScore[3];
};
class Cteacher:public CPerson
{
public:
void Inputage(double age)
{
tage = age;
}
void Print()
{
Output();
cout<<"教龄:"<<tage<<endl;
}
private:
double tage;
};
int main()
{
CStudent stu;
Cteacher tea;
stu.SetData("LiMing", "21010211");
stu.InputScore( 80, 76, 91 );
stu.Print();
tea.SetData("zhangli","001");
tea.Inputage(12);
tea.Print();
return 0;
}
//8.声明一个教师(Teacher)类和一个学生(Student)类,用多重继承的方式声明一个研究生(Graduate)派生类。
//教师类中包括数据成员name(姓名),age(年龄),title(职称)。
//学生类中包括数据成员name(姓名),age(年龄),score(成绩)。
//在定义派生类对象时给出初始化的数据(自已定),然后输出这些数据。初值自拟。
#include<iostream>
#include<string.h>
using namespace std;
class Teacher
{
public:
Teacher(string nam,int a,string t)
{
name=nam;
age=a;
title=t;
}
void display()
{
cout<<"name:"<<name<<endl;
cout<<"age"<<age<<endl;
cout<<"title:"<<title<<endl;
}
protected:
string name;
int age;
string title;
};
class Student
{
public:
Student(string nam,char s,float sco)
{
name1=nam;
sex=s;
score=sco;
}
void display1()
{
cout<<"name:"<<name1<<endl;
cout<<"sex:"<<sex<<endl;
cout<<"score:"<<score<<endl;
}
protected:
string name1;
char sex;
float score;
};
class Graduate:public Teacher,public Student
{
public:
Graduate(string nam,int a,char s,string t,float sco,float w):
Teacher(nam,a,t),Student(nam,s,sco),wage(w) {}
void show( )
{
cout<<"name:"<<name<<endl;
cout<<"age:"<<age<<endl;
cout<<"sex:"<<sex<<endl;
cout<<"score:"<<score<<endl;
cout<<"title:"<<title<<endl;
cout<<"wages:"<<wage<<endl;
}
private:
float wage;
};
int main( )
{
Graduate grad1("Wang-li",24,'f',"assistant",89.5,1234.5);
grad1.show( );
return 0;
}
//16.定义一个国家基类Country,包含国名、首都、人口等属性,派生出省类Province,增加省会城市、人口数量属性。
#include<iostream>
#include<string>
using namespace std;
class Country
{public:
Country(string nam,string c,long int cp)
{name=nam;capital=c;country_population=cp;}
protected:
string name;
string capital;
long int country_population;
};
class Province:public Country
{public:
Province(string nam,string c,long int cp,string pc,long int pp):Country(nam,c,cp)
{
Province_capital=pc;
Province_population=pp;
};
void show()
{
cout<<"name:"<<name<<endl;
cout<<"capital:"<<capital<<endl;
cout<<"country_population:"<<country_population<<endl;
cout<<"Province_capital:"<<Province_capital<<endl;
cout<<"Province_population:"<<Province_population<<endl;
}
private:
string Province_capital;
long int Province_population;
};
int main()
{
Province prov1("China","Beijing",1300000000,"Nanchang",45000000);
prov1.show();
return 0;
}
//17.定义一个车基类Vehicle,含私有成员speed,weight。
//派生出自行车类Bicycle,增加high成员;汽车类Car,增加seatnum(座位数)成员。
//从bicycle和car中派生出摩托车类Motocycle。
#include<iostream>
using namespace std;
class Vehicle
{
public:
Vehicle(float sp,float w){speed=sp;weight=w;}
void display()
{
cout<<"speed:"<<speed<<" weight"<<weight<<endl;
}
private:
float speed;
float weight;
};
class Bicycle:virtual public Vehicle
{
public:
Bicycle(float sp,float w,float h):Vehicle(sp,w){high = h;}
float high;
};
class Car:virtual public Vehicle
{
public:
Car(float sp,float w,int num):Vehicle(sp,w)
{
seatnum = num;
}
protected:
int seatnum;
};
class Motorcycle:public Bicycle,public Car
{
public:
Motorcycle(float sp,float w,float h,int num):Vehicle(sp,w),Bicycle(sp,w,h),Car(sp,w,num){}
void display()
{
Vehicle::display();
cout<<" high:"<<high<<" seatnum:"<<seatnum<<endl;
}
};
int main()
{
Motorcycle m(120,120,120,1);
m.display();
return 0;
}
//18.把定义平面直角坐标系上的一个点的类Cpoint作为基类,派生出描述一条直线的类Cline,再派生出一个矩形类Crect。
//要求成员函数能求出两点间的距离、矩形的周长和面积
#include<iostream>
#include<math.h>
using namespace std;
class Cpoint{
public:
void setX(float x){X=x;}
void setY(float y){Y=y;}
float getX()
{
return X;
}
float getY()
{
return Y;
}
protected:
float X,Y;
};
class Cline:public Cpoint
{
protected:
float d,X1,Y1;
public:
void input()
{
cout <<"请输入一个点的坐标值X1,Y1:" <<endl;
cin>>X1>>Y1;
}
float getX1()
{
return X1;
}
float getY1()
{
return Y1;
}
float dis()
{
d=sqrt((X-X1)*(X-X1)+(Y-Y1)*(Y-Y1));
return d;
}
void show()
{
cout <<"直线长度为: "<<d <<endl;
}
};
class Crect:public Cline
{
public:
void input1()
{
cout <<"请输入矩形另外一条边的长度d1:" <<endl;
cin>>d1;
}
float getd1()
{
return d1;
}
float getd()
{
return d;
}
float area()
{
a = d*d1;
return a;
}
float zhou()
{
z=2*(d+d1);
return z;
}
void show1()
{
cout <<"此矩形的面积和周长分别是 :" <<a <<" " <<z <<endl;
}
protected:
float a, z,d1;
};
int main()
{
Cline l1;
Crect r1;
l1.setX(2);
l1.setY(2);
l1.input();
l1.dis();
l1.show();
r1.setX(2);
r1.setY(2);
r1.input();
r1.input1();
r1.getd1();
r1.dis();
r1.area();
r1.zhou();
r1.show1();
return 0;
}
//20.定义一个抽象类Cshape,包含纯虚函数Area(用来计算面积)和SetData(用来重设形状大小)。
//然后派生出三角形Ctriangle类、矩形Crect类、圆Ccircle类,分别求其面积。
//最后定义一个CArea类,计算这几个形状的面积之和,各形状的数据通过Carea类构造函数或成员函数来设置。编写一个完整程序。
#include<iostream>
using namespace std;
class Cshape
{
public:
virtual float Area()=0;
};
class CTriangle :public Cshape
{
int vect;
public:
CTriangle(int v):vect(v) {}
float Area()
{
return vect*1.732/4;
}
};
class CRect:public Cshape
{
int length,height;
public:
CRect(int l,int h):length(l),height(h) {}
float Area()
{
return length*height;
}
};
class CCircle:public Cshape
{
int radius;
public:
CCircle(int r):radius(r) {}
float Area()
{
return 3.14*radius*radius;
}
};
class Area
{
CTriangle t;
CRect r;
CCircle c;
public:
Area(int v,int l,int h,int r):t(v),r(l,h),c(r) {}
float sum() {return t.Area()+r.Area()+c.Area();}
};
int main()
{
Area a(10,20,30,5);
cout<<a.sum()<<endl;
return 0;
}
//3.定义一个Teacher(教师)类和一个Student(学生)类,
//二者有一部分数据成员是相同的,例如num(号码),name(姓名),sex(性别)。
//编写程序,将一个Student对象(学生)转换为Teacher(教师)类,只将以上3个相同的数据成员移植过去。
//可以设想为:一位学生大学毕业了,留校担任教师,他原有的部分数据对现在的教师身份来说仍然是有用的,
//应当保留并成为其教师的数据的一部分。
#include <iostream>
using namespace std;
class Student
{
public:
Student(int,char[],char,float);
int get_num(){return num;}
char * get_name(){return name;}
char get_sex(){return sex;}
void display()
{
cout<<"num:"<<num<<"\nname:"<<name<<"\nsex:"<<sex<<"\nscore:"<<score<<"\n\n";
}
private:
int num;
char name[20];
char sex;
float score;
};
Student::Student(int n,char nam[],char s,float so)
{
num=n;
strcpy(name,nam);
sex=s;
score=so;
}
class Teacher
{
public:
Teacher(){}
Teacher(Student&);
Teacher(int n,char nam[],char sex,float pay);
void display();
private:
int num;
char name[20];
char sex;
float pay;
};
Teacher::Teacher(int n,char nam[],char s,float p)
{
num=n;
strcpy(name,nam);
sex=s;
pay=p;
}
Teacher::Teacher(Student& stud)
{
num=stud.get_num();
strcpy(name,stud.get_name());
sex=stud.get_sex();
pay=1500;
}
void Teacher::display()
{
cout<<"num:"<<num<<"\nname:"<<name<<"\nsex:"<<sex<<"\npay:"<<pay<<"\n\n";
}
int main()
{
Teacher teacher1(10001,"Li",'f',1234.5),teacher2;
Student student1(20010,"Wang",'m',89.5);
cout<<"student1:"<<endl;
student1.display();
teacher2=Teacher(student1);
cout<<"teacher2:"<<endl;
teacher2.display();
return 0;
}
/*分别定义Teacher(教师)类和Cadre(干部)类,采用多重继承方式有这两个类派生出新类Teacher_Cadre(教师兼干部)。要求:
(1)、在两个基类中的包含姓名、年龄、性别、地址、电话、等数据成员。
(2)、在Teacher类中包含数据成员title(职称),在Cadre类中还包含数据成员post(职务),在Teacher_Cadre类中还包含数据成员wages(工资)。
(3)、对两个基类中的姓名、年龄、性别、职称、地址、电话等数据成员用相同的名字,在引用数据成员时制定作用域。
(4)、在类中声明成员函数,在类外定义成员函数 。
(5)、在派生类Teacher_cadre的成员函数show中调用Teacher类中的display函数。输出姓名,年龄,性别,职称,地址,电话,然后再用cout语句输出职务与工资。
*/
#include<string.h>
#include<iostream>
using namespace std;
class Teacher
{
public:
Teacher(string nam,int a,char s,string tit,string ad,string t);
void display();
protected:
string name;
int age;
char sex;
string title;
string addr;
string tel;
};
Teacher::Teacher(string nam,int a,char s,string tit,string ad,string t):
name(nam),age(a),sex(s),title(tit),addr(ad),tel(t){ }
void Teacher::display()
{
cout<<"name:"<<name<<endl;
cout<<"age"<<age<<endl;
cout<<"sex:"<<sex<<endl;
cout<<"title:"<<title<<endl;
cout<<"address:"<<addr<<endl;
cout<<"tel:"<<tel<<endl;
}
class Cadre
{
public:
Cadre(string nam,int a,char s,string p,string ad,string t);
void display();
protected:
string name;
int age;
char sex;
string post;
string addr;
string tel;
};
Cadre::Cadre(string nam,int a,char s,string p,string ad,string t):
name(nam),age(a),sex(s),post(p),addr(ad),tel(t){}
void Cadre::display()
{
cout<<"name:"<<name<<endl;
cout<<"age:"<<age<<endl;
cout<<"sex:"<<sex<<endl;
cout<<"post:"<<post<<endl;
cout<<"address:"<<addr<<endl;
cout<<"tel:"<<tel<<endl;
}
class Teacher_Cadre:public Teacher,public Cadre
{
public:
Teacher_Cadre(string nam,int a,char s,string tit,string p,string ad,string t,float w);
void show( );
private:
float wage;
};
Teacher_Cadre::Teacher_Cadre(string nam,int a,char s,string t,string p,string ad,string tel,float w):
Teacher(nam,a,s,t,ad,tel),Cadre(nam,a,s,p,ad,tel),wage(w) {}
void Teacher_Cadre::show( )
{
Teacher::display();
cout<<"post:"<<Cadre::post<<endl;
cout<<"wages:"<<wage<<endl;
}
int main( )
{
Teacher_Cadre te_ca("Wang-li",50,'f',"prof.","president","135 Beijing Road,Shanghai","(021)61234567",1534.5);
te_ca.show( );
return 0;
}
//写一个程序,定义抽象类型Shape,
//由他派生五个类:Circle(圆形),Square(正方形),Rectangle(矩形),Trapezoid(梯形),Triangle(三角形)。
//用虚函数分别计算几种图形的面积,并求它们的和。要求用基类指针数组,使它的每一个元素指向一个派生类的对象。
#include <iostream>
using namespace std;
class Shape
{
public:
virtual double area() const =0;
};
//定义Circle类
class Circle:public Shape
{
public:
Circle(double r):radius(r){}
virtual double area() const
{
return 3.14159*radius*radius;
};
protected:
double radius; };
//定义Rectangle类
class Rectangle:public Shape
{
public:
Rectangle(double w,double h):width(w),height(h){}
virtual double area() const {return width*height;}
protected:
double width,height;
};
class Triangle:public Shape
{
public:
Triangle(double w,double h):width(w),height(h){}
virtual double area() const {return 0.5*width*height;}
protected:
double width,height;
};
void printArea(const Shape &s)
{
cout<<s.area()<<endl;
}
int main()
{
Circle circle(12.6);
cout<<"area of circle =";
printArea(circle);
Rectangle rectangle(4.5,8.4);
cout<<"area of rectangle =";
printArea(rectangle);
Triangle triangle(4.5,8.4);
cout<<"area of triangle =";
printArea(triangle);
return 0;
}
相关文章:
C++详解
//7.用new建立一个动态一维数组,并初始化int[10]{1,2,3,4,5,6,7,8,9,10},用指针输出,最后销毁数组所占空间。 #include<iostream> #include<string> using namespace std; int main() { int *p; pnew int[10]; for(i…...
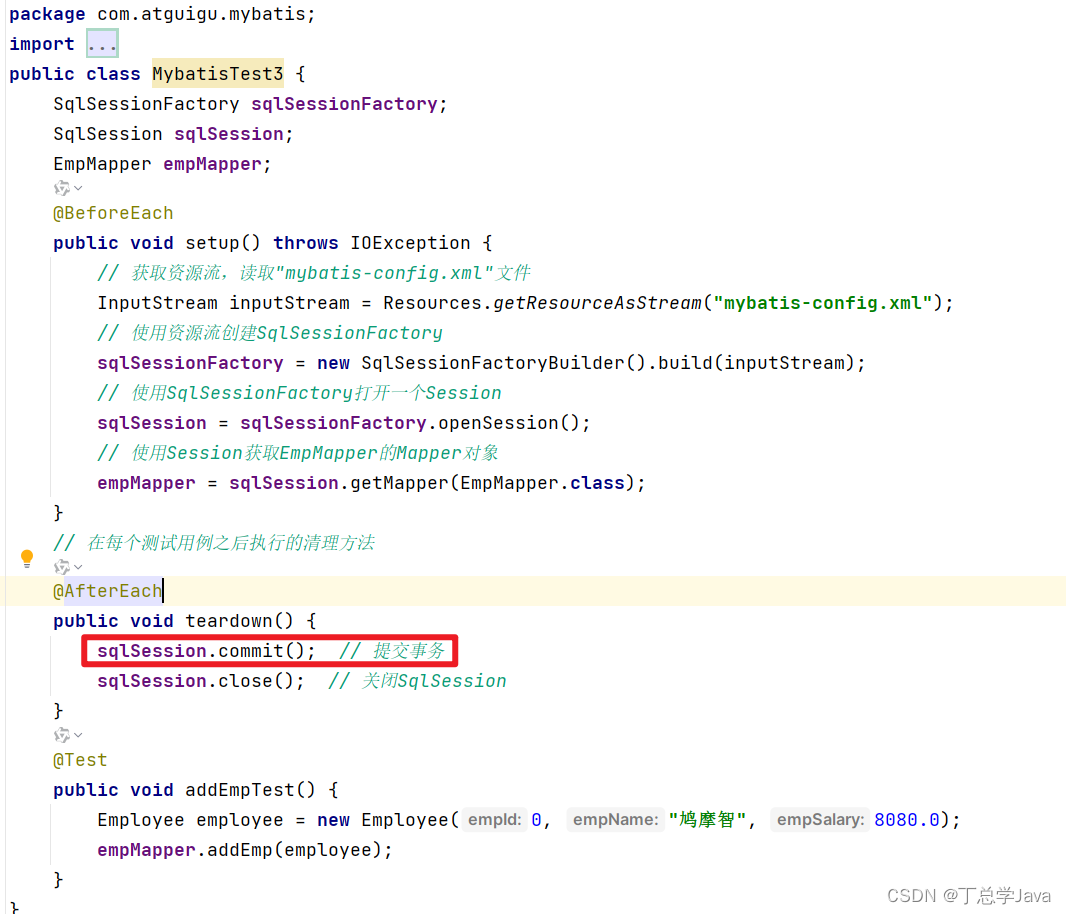
mybatis数据输入-实体类型的参数
1、建库建表 CREATE DATABASE mybatis-example;USE mybatis-example;CREATE TABLE t_emp(emp_id INT AUTO_INCREMENT,emp_name CHAR(100),emp_salary DOUBLE(10,5),PRIMARY KEY(emp_id) );INSERT INTO t_emp(emp_name,emp_salary) VALUES("tom",200.33); INSERT INTO…...
Java-接口
目录 定义 格式 使用 接口中成员的特点 成员变量 构造方法 成员方法 JDK8新特性:可以定义有方法体的方法 默认方法 作用 定义格式 注意事项 静态方法 定义格式 注意事项 JDK9新特性:可以定义私有方法 私有方法的定义格式 接口和接口之…...
mysql常用命令行代码
连接到 MySQL 服务器: mysql -u your_username -p替换 your_username 为你的 MySQL 用户名。系统会提示你输入密码。 退出 MySQL 命令行: EXIT;或者按 Ctrl D。 显示所有数据库: SHOW DATABASES;选择数据库: USE your_database…...
Python压缩、解压文件
#!/usr/bin/python3 # -*- coding:utf-8 -*- """ author: JHC file: util_compress.py time: 2023/5/28 14:58 desc: rarfile 使用需要安装 rarfile 和 unrar 并且将 unrar.exe 复制到venv/Scrpits目录下 (从WinRar安装目录下白嫖的) 下载…...
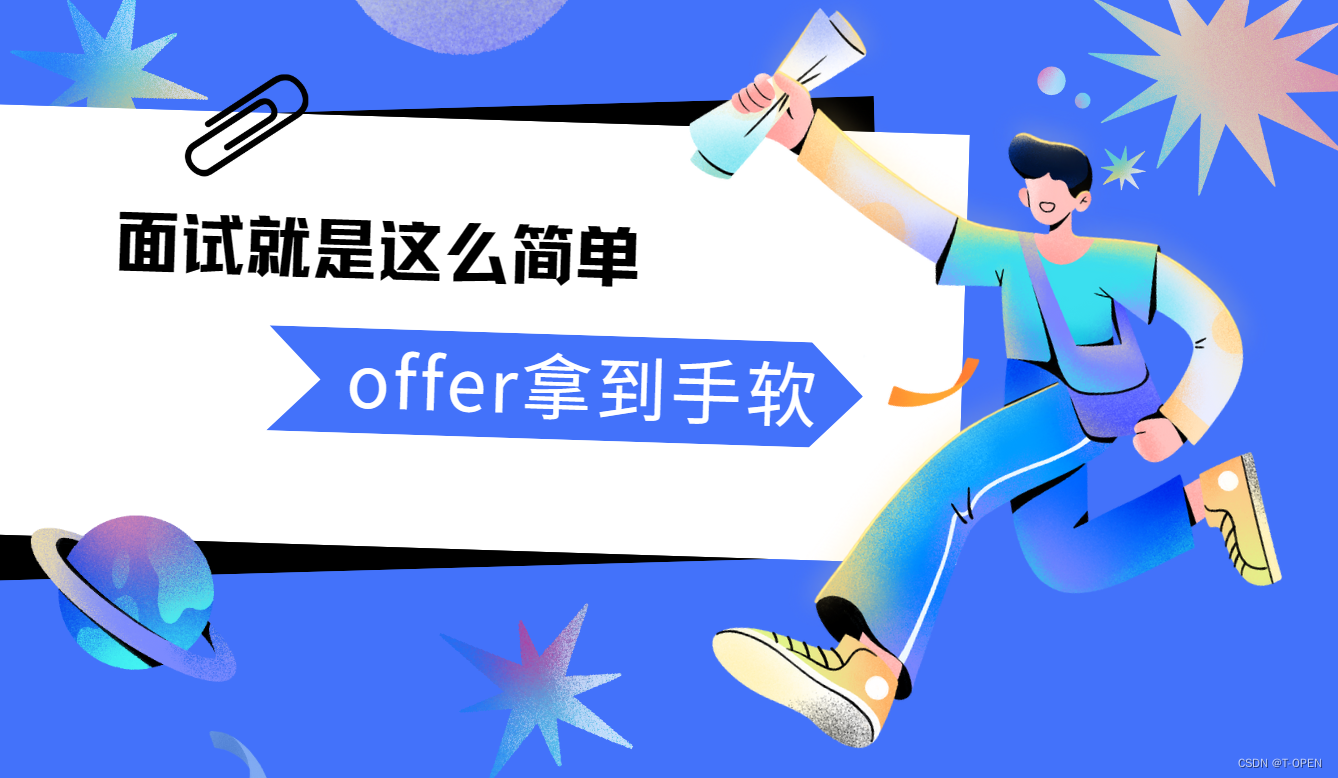
面试就是这么简单,offer拿到手软(一)—— 常见非技术问题回答思路
面试系列: 面试就是这么简单,offer拿到手软(一)—— 常见非技术问题回答思路 面试就是这么简单,offer拿到手软(二)—— 常见65道非技术面试问题 文章目录 一、前言二、常见面试问题回答思路问…...
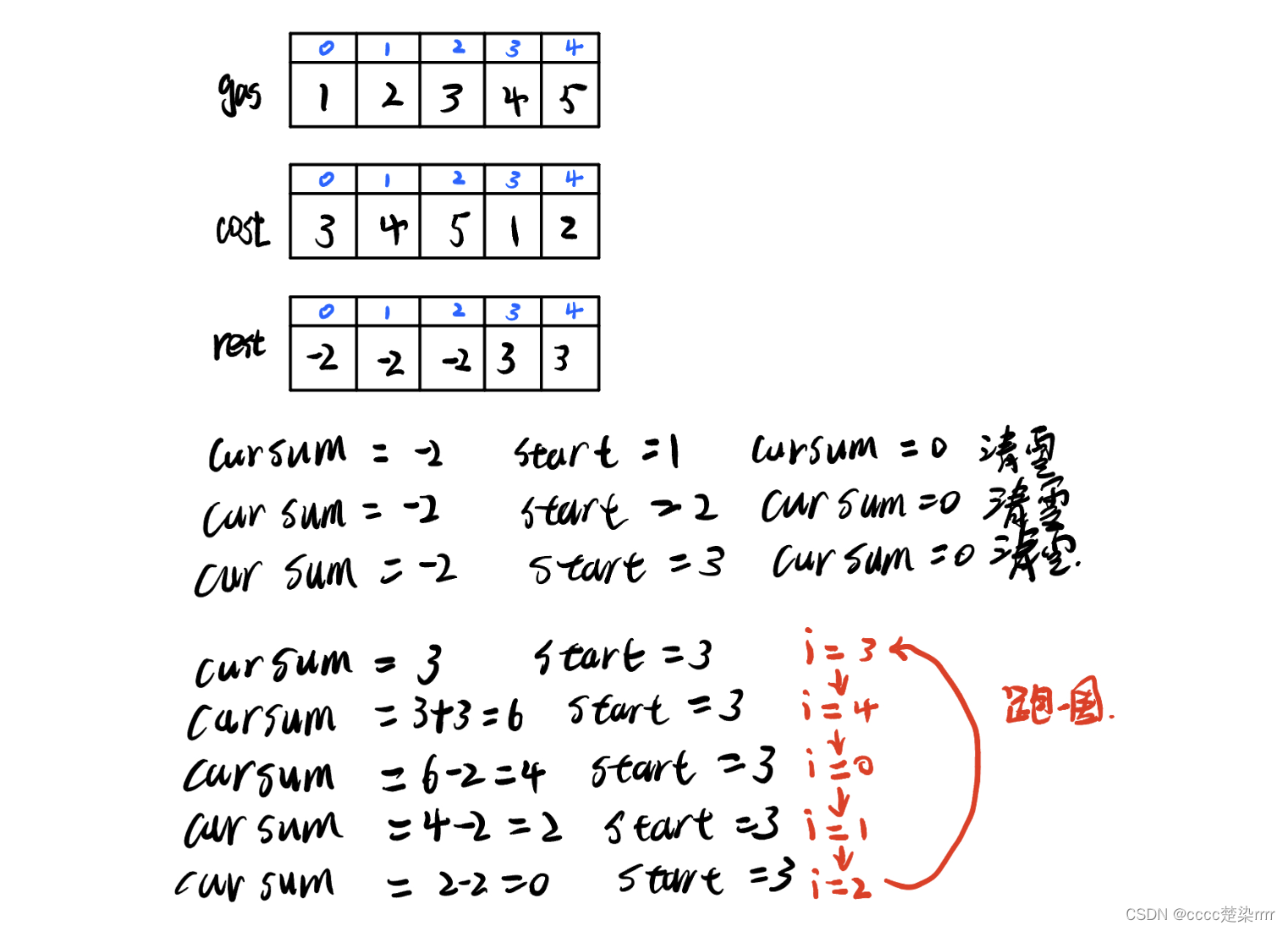
134. 加油站(贪心算法)
根据题解 这道题使用贪心算法,找到当前可解决问题的状态即可 「贪心算法」的问题需要满足的条件: 最优子结构:规模较大的问题的解由规模较小的子问题的解组成,规模较大的问题的解只由其中一个规模较小的子问题的解决定ÿ…...
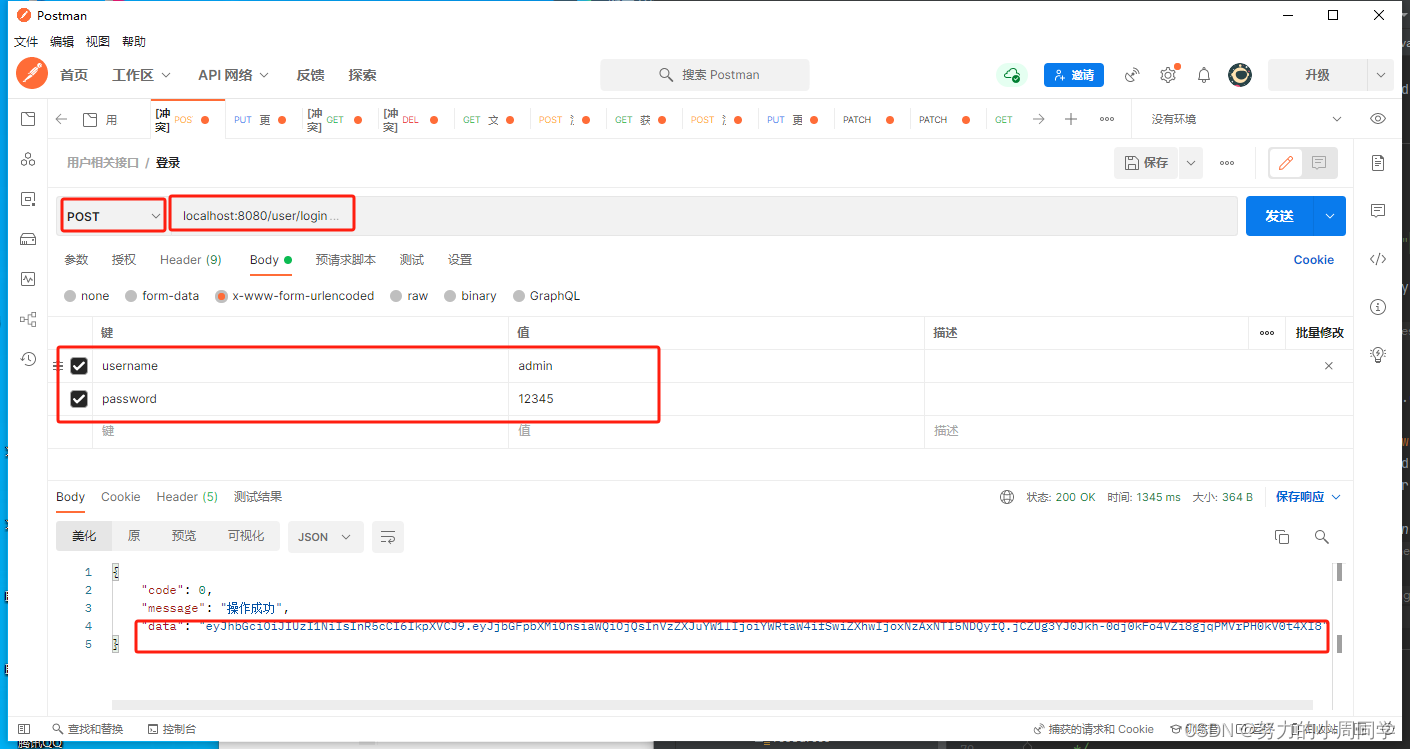
Springboot3+vue3从0到1开发实战项目(二)
前面完成了注册功能这次就来写登录功能, 还是按照这个方式来 明确需求: 登录接口 前置工作 : 想象一下登录界面(随便在百度上找一张) 看前端的能力咋样了, 现在我们不管后端看要什么参数就好 阅读接口文档…...

Spring中Bean的生命周期
1.生命周期 Spring应用中容器管理了我们每一个bean的生命周期,为了保证系统的可扩展性,同时为用户提供自定义的能力,Spring提供了大量的扩展点。完整的Spring生命周期如下图所示,绿色背景的节点是ApplictionContext生命周期特有的…...
IndexOutOfBoundsException: Index: 2048, Size: 2048] Controller接收对象集合长度超过2048错误
完整异常信息: org.apache.catalina.core.StandardWrapperValve.invoke Servlet.service() for servlet [spring] in context with path [/jsgc] threw exception [Request processing failed; nested exception is org.springframework.beans.InvalidPropertyExce…...
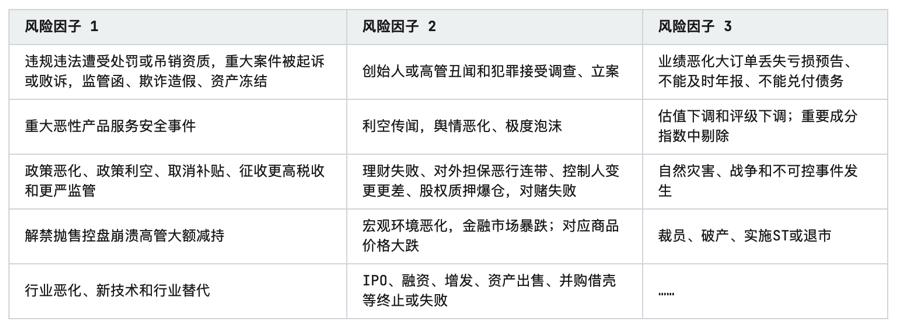
2023年中国消费金融行业研究报告
第一章 行业概况 1.1 定义 中国消费金融行业,作为国家金融体系的重要组成部分,旨在为消费者提供多样化的金融产品和服务,以满足其消费需求。这一行业包括银行、消费金融公司、小额贷款公司等多种金融机构,涵盖了包括消费贷款在内…...
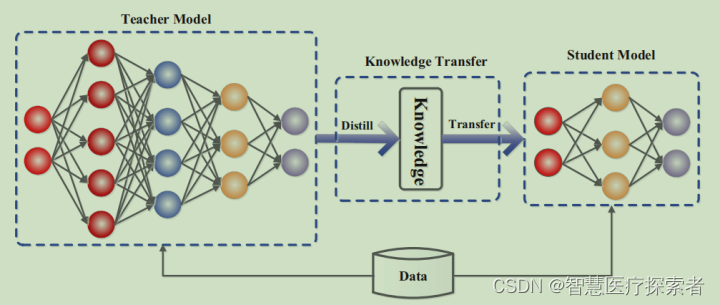
深度学习:什么是知识蒸馏(Knowledge Distillation)
1 概况 1.1 定义 知识蒸馏(Knowledge Distillation)是一种深度学习技术,旨在将一个复杂模型(通常称为“教师模型”)的知识转移到一个更简单、更小的模型(称为“学生模型”)中。这一技术由Hint…...
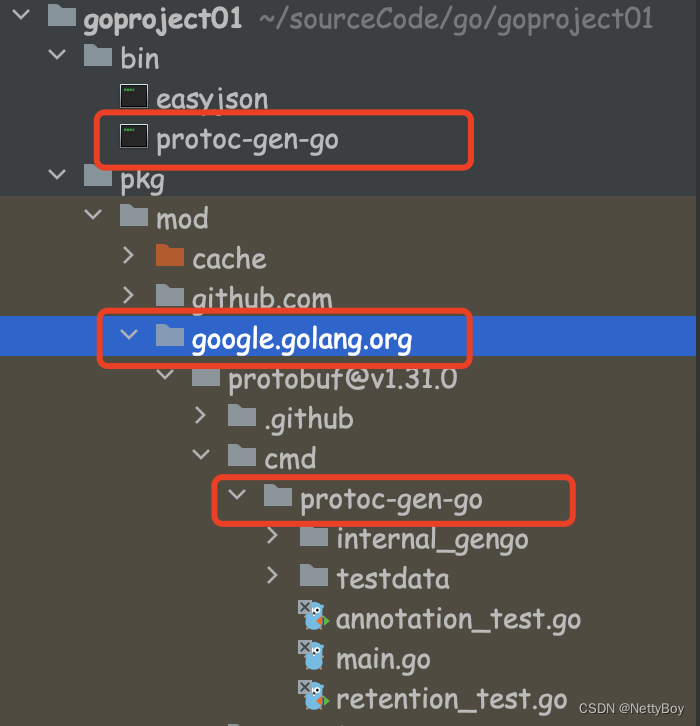
【Go】protobuf介绍及安装
目录 一、Protobuf介绍 1.Protobuf用来做什么 2. Protobuf的序列化与反序列化 3. Protobuf的优点和缺点 4. RPC介绍 <1>文档规范 <2>消息编码 <3>传输协议 <4>传输性能 <5>传输形式 <6>浏览器的支持度 <7>消息的可读性和…...
c语言编程题经典100例——(41~45例)
1,实现动态内存分配。 在C语言中,动态内存分配使用malloc、calloc、realloc和free函数。以下是一个示例: #include <stdio.h> #include <stdlib.h> int main() { int *ptr NULL; // 初始化为空 int n 5; // 假设我们想要分配5个整数…...
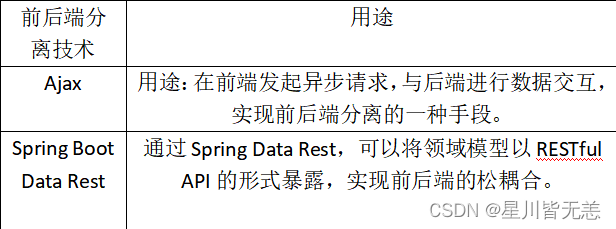
计算机毕业设计|基于SpringBoot+MyBatis框架健身房管理系统的设计与实现
计算机毕业设计|基于SpringBootMyBatis框架的健身房管理系统的设计与实现 摘 要:本文基于Spring Boot和MyBatis框架,设计并实现了一款综合功能强大的健身房管理系统。该系统涵盖了会员卡查询、会员管理、员工管理、器材管理以及课程管理等核心功能,并且…...
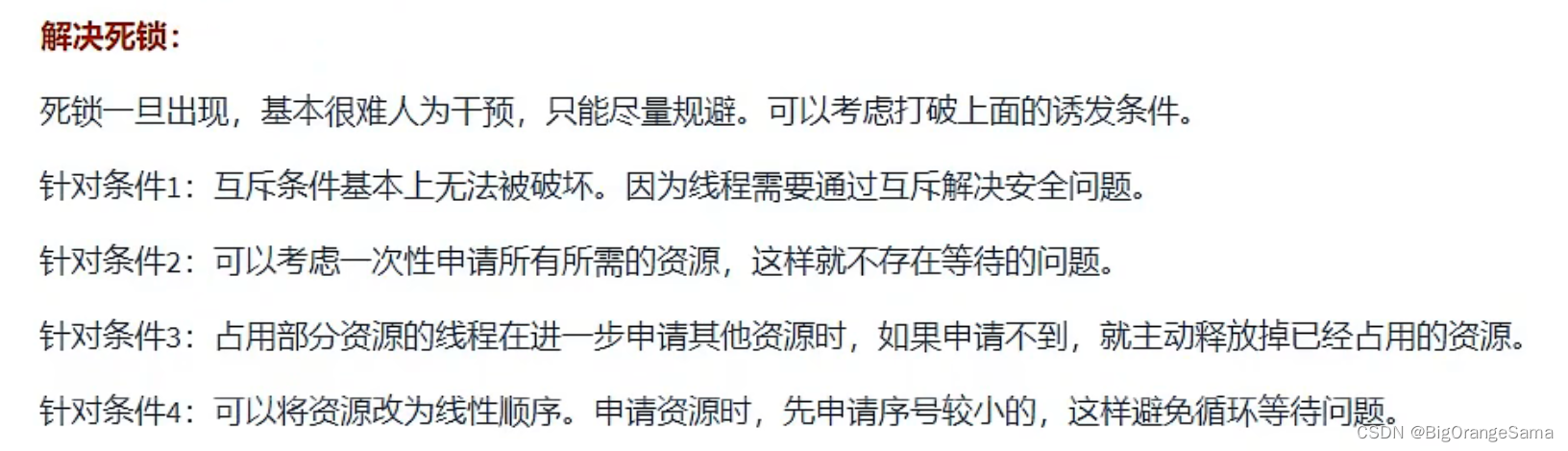
java学习part27线程死锁
基本就是操作系统的内容 138-多线程-线程安全的懒汉式_死锁_ReentrantLock的使用_哔哩哔哩_bilibili...
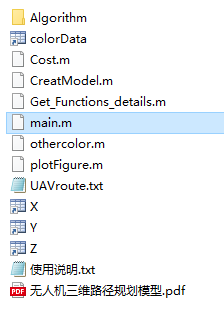
(二)Tiki-taka算法(TTA)求解无人机三维路径规划研究(MATLAB)
一、无人机模型简介: 单个无人机三维路径规划问题及其建模_IT猿手的博客-CSDN博客 参考文献: [1]胡观凯,钟建华,李永正,黎万洪.基于IPSO-GA算法的无人机三维路径规划[J].现代电子技术,2023,46(07):115-120 二、Tiki-taka算法(TTA…...
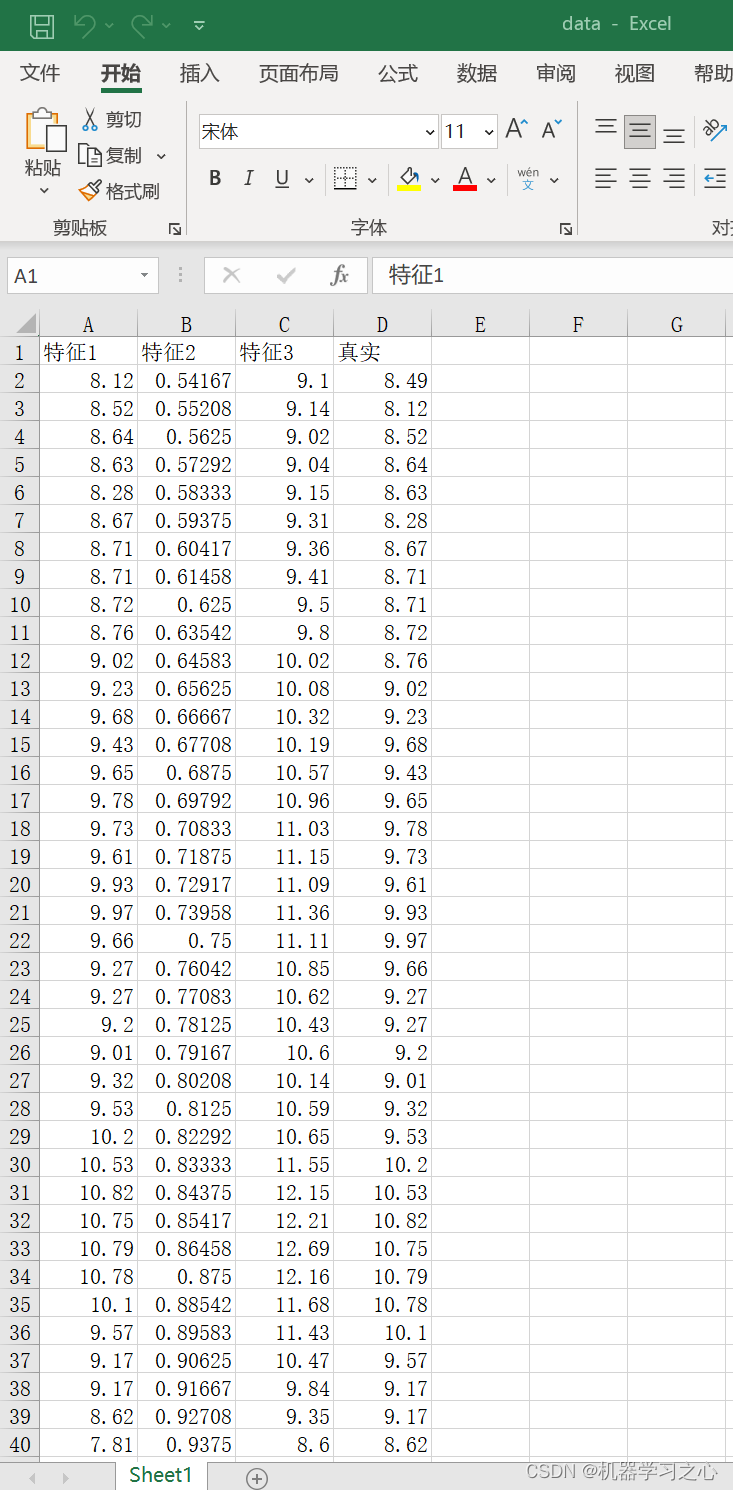
区间预测 | Matlab实现BP-KDE的BP神经网络结合核密度估计多变量时序区间预测
区间预测 | Matlab实现BP-KDE的BP神经网络结合核密度估计多变量时序区间预测 目录 区间预测 | Matlab实现BP-KDE的BP神经网络结合核密度估计多变量时序区间预测效果一览基本介绍程序设计参考资料 效果一览 基本介绍 1.BP-KDE多变量时间序列区间预测,基于BP神经网络多…...

LD_PRELOAD劫持、ngixn临时文件、无需临时文件rce
LD_PRELOAD劫持 <1> LD_PRELOAD简介 LD_PRELOAD 是linux下的一个环境变量。用于动态链接库的加载,在动态链接库的过程中他的优先级是最高的。类似于 .user.ini 中的 auto_prepend_file,那么我们就可以在自己定义的动态链接库中装入恶意函数。 也…...
循环神经网络训练情感分析
文章目录 1 循环神经网络训练情感分析2 完整代码3 代码详解 1 循环神经网络训练情感分析 下面介绍如何使用长短记忆模型(LSTM)处理情感分类LSTM模型是循环神经网络的一种,按照时间顺序,把信息进行有效的整合,有的信息…...
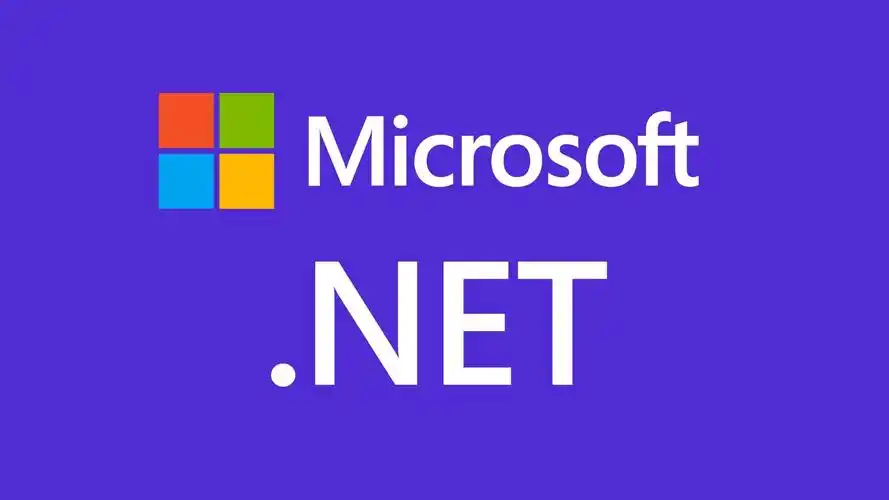
.Net框架,除了EF还有很多很多......
文章目录 1. 引言2. Dapper2.1 概述与设计原理2.2 核心功能与代码示例基本查询多映射查询存储过程调用 2.3 性能优化原理2.4 适用场景 3. NHibernate3.1 概述与架构设计3.2 映射配置示例Fluent映射XML映射 3.3 查询示例HQL查询Criteria APILINQ提供程序 3.4 高级特性3.5 适用场…...
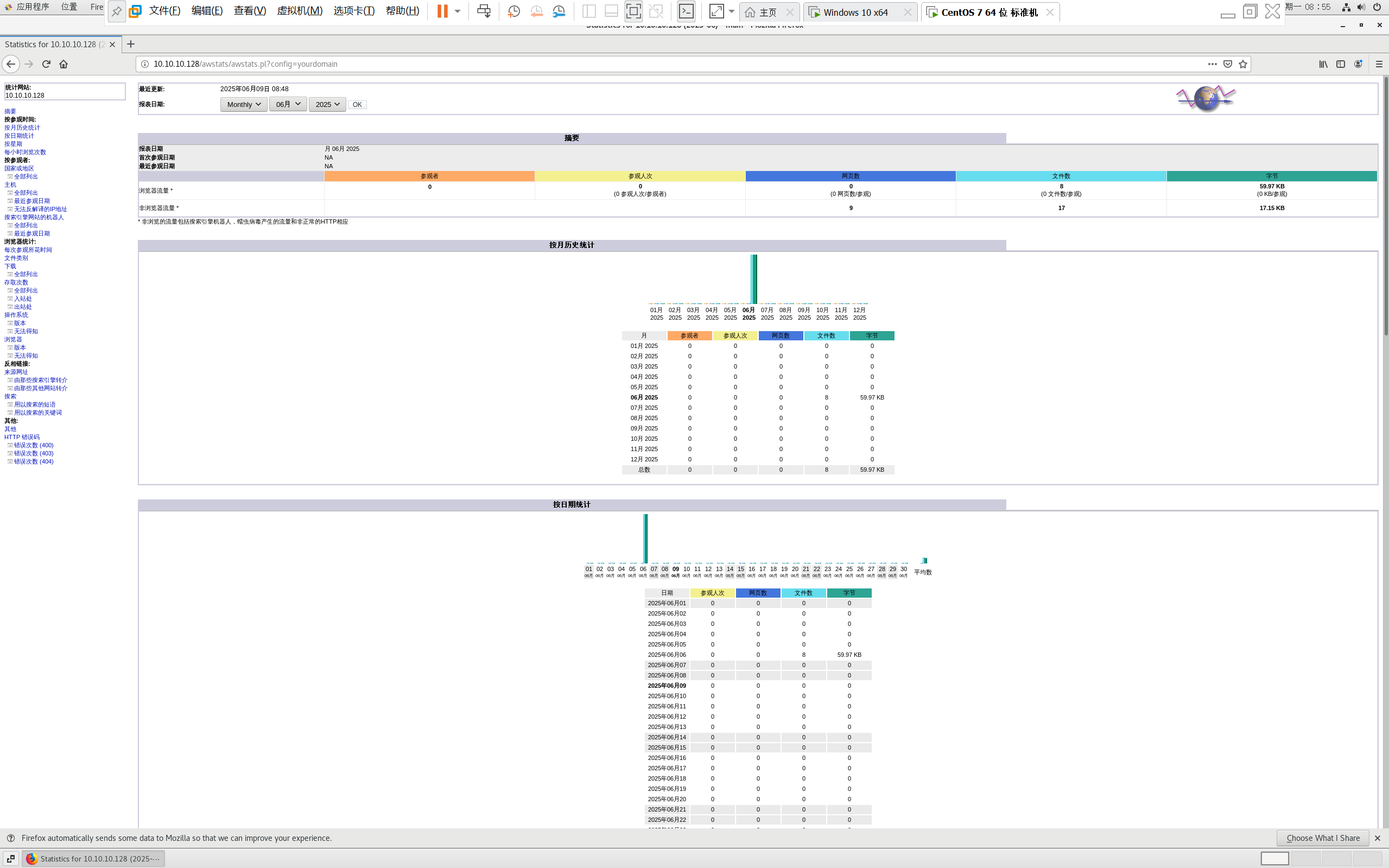
centos 7 部署awstats 网站访问检测
一、基础环境准备(两种安装方式都要做) bash # 安装必要依赖 yum install -y httpd perl mod_perl perl-Time-HiRes perl-DateTime systemctl enable httpd # 设置 Apache 开机自启 systemctl start httpd # 启动 Apache二、安装 AWStats࿰…...
1688商品列表API与其他数据源的对接思路
将1688商品列表API与其他数据源对接时,需结合业务场景设计数据流转链路,重点关注数据格式兼容性、接口调用频率控制及数据一致性维护。以下是具体对接思路及关键技术点: 一、核心对接场景与目标 商品数据同步 场景:将1688商品信息…...
解决本地部署 SmolVLM2 大语言模型运行 flash-attn 报错
出现的问题 安装 flash-attn 会一直卡在 build 那一步或者运行报错 解决办法 是因为你安装的 flash-attn 版本没有对应上,所以报错,到 https://github.com/Dao-AILab/flash-attention/releases 下载对应版本,cu、torch、cp 的版本一定要对…...
聊一聊接口测试的意义有哪些?
目录 一、隔离性 & 早期测试 二、保障系统集成质量 三、验证业务逻辑的核心层 四、提升测试效率与覆盖度 五、系统稳定性的守护者 六、驱动团队协作与契约管理 七、性能与扩展性的前置评估 八、持续交付的核心支撑 接口测试的意义可以从四个维度展开,首…...
LRU 缓存机制详解与实现(Java版) + 力扣解决
📌 LRU 缓存机制详解与实现(Java版) 一、📖 问题背景 在日常开发中,我们经常会使用 缓存(Cache) 来提升性能。但由于内存有限,缓存不可能无限增长,于是需要策略决定&am…...
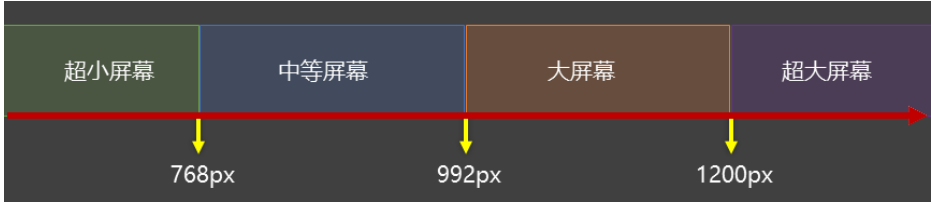
CSS3相关知识点
CSS3相关知识点 CSS3私有前缀私有前缀私有前缀存在的意义常见浏览器的私有前缀 CSS3基本语法CSS3 新增长度单位CSS3 新增颜色设置方式CSS3 新增选择器CSS3 新增盒模型相关属性box-sizing 怪异盒模型resize调整盒子大小box-shadow 盒子阴影opacity 不透明度 CSS3 新增背景属性ba…...
Java中栈的多种实现类详解
Java中栈的多种实现类详解:Stack、LinkedList与ArrayDeque全方位对比 前言一、Stack类——Java最早的栈实现1.1 Stack类简介1.2 常用方法1.3 优缺点分析 二、LinkedList类——灵活的双端链表2.1 LinkedList类简介2.2 常用方法2.3 优缺点分析 三、ArrayDeque类——高…...

[10-1]I2C通信协议 江协科技学习笔记(17个知识点)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17...
npm install 相关命令
npm install 相关命令 基本安装命令 # 安装 package.json 中列出的所有依赖 npm install npm i # 简写形式# 安装特定包 npm install <package-name># 安装特定版本 npm install <package-name><version>依赖类型选项 # 安装为生产依赖(默认&…...