springboot3 集成spring-authorization-server (一 基础篇)
官方文档
Spring Authorization Server
环境介绍
java:17
SpringBoot:3.2.0
SpringCloud:2023.0.0
引入maven配置
<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-oauth2-authorization-server</artifactId>
</dependency>
<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
AuthorizationServerConfig认证中心配置类
package com.auth.config;import com.jilianyun.exception.ServiceException;
import com.nimbusds.jose.jwk.JWKSet;
import com.nimbusds.jose.jwk.RSAKey;
import com.nimbusds.jose.jwk.source.ImmutableJWKSet;
import com.nimbusds.jose.jwk.source.JWKSource;
import com.nimbusds.jose.proc.SecurityContext;
import lombok.extern.slf4j.Slf4j;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.MediaType;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.security.config.Customizer;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.oauth2.core.AuthorizationGrantType;
import org.springframework.security.oauth2.core.ClientAuthenticationMethod;
import org.springframework.security.oauth2.jwt.JwtDecoder;
import org.springframework.security.oauth2.server.authorization.client.JdbcRegisteredClientRepository;
import org.springframework.security.oauth2.server.authorization.client.RegisteredClient;
import org.springframework.security.oauth2.server.authorization.client.RegisteredClientRepository;
import org.springframework.security.oauth2.server.authorization.config.annotation.web.configuration.OAuth2AuthorizationServerConfiguration;
import org.springframework.security.oauth2.server.authorization.config.annotation.web.configurers.OAuth2AuthorizationServerConfigurer;
import org.springframework.security.oauth2.server.authorization.settings.AuthorizationServerSettings;
import org.springframework.security.oauth2.server.authorization.settings.ClientSettings;
import org.springframework.security.provisioning.InMemoryUserDetailsManager;
import org.springframework.security.web.SecurityFilterChain;
import org.springframework.security.web.authentication.LoginUrlAuthenticationEntryPoint;
import org.springframework.security.web.util.matcher.AntPathRequestMatcher;
import org.springframework.security.web.util.matcher.MediaTypeRequestMatcher;import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.interfaces.RSAPrivateKey;
import java.security.interfaces.RSAPublicKey;
import java.util.UUID;/*** <p>认证中心配置类</p>** @author By: chengxuyuanshitang* Ceate Time 2024-05-07 11:42*/@Slf4j
@EnableWebSecurity
@Configuration(proxyBeanMethods = false)
public class AuthorizationServerConfig {/*** Security过滤器链,用于协议端点** @param http HttpSecurity* @return SecurityFilterChain*/@Beanpublic SecurityFilterChain authorizationServerSecurityFilterChain (HttpSecurity http) throws Exception {OAuth2AuthorizationServerConfiguration.applyDefaultSecurity (http);http.getConfigurer (OAuth2AuthorizationServerConfigurer.class)//启用OpenID Connect 1.0.oidc (Customizer.withDefaults ());http// 未从授权端点进行身份验证时重定向到登录页面.exceptionHandling ((exceptions) -> exceptions.defaultAuthenticationEntryPointFor (new LoginUrlAuthenticationEntryPoint ("/login"),new MediaTypeRequestMatcher (MediaType.TEXT_HTML)))//接受用户信息和/或客户端注册的访问令牌.oauth2ResourceServer ((resourceServer) -> resourceServer.jwt (Customizer.withDefaults ()));return http.build ();}/*** 用于认证的Spring Security过滤器链。** @param http HttpSecurity* @return SecurityFilterChain*/@Beanpublic SecurityFilterChain defaultSecurityFilterChain (HttpSecurity http) throws Exception {http.authorizeHttpRequests ((authorize) -> authorize.requestMatchers (new AntPathRequestMatcher ("/actuator/**"),new AntPathRequestMatcher ("/oauth2/**"),new AntPathRequestMatcher ("/**/*.json"),new AntPathRequestMatcher ("/**/*.css"),new AntPathRequestMatcher ("/**/*.html")).permitAll ().anyRequest ().authenticated ()).formLogin (Customizer.withDefaults ());return http.build ();}/*** 配置密码解析器,使用BCrypt的方式对密码进行加密和验证** @return BCryptPasswordEncoder*/@Beanpublic PasswordEncoder passwordEncoder () {return new BCryptPasswordEncoder ();}@Beanpublic UserDetailsService userDetailsService () {UserDetails userDetails = User.withUsername ("chengxuyuanshitang").password (passwordEncoder ().encode ("chengxuyuanshitang")).roles ("admin").build ();return new InMemoryUserDetailsManager (userDetails);}/*** RegisteredClientRepository 的一个实例,用于管理客户端** @param jdbcTemplate jdbcTemplate* @param passwordEncoder passwordEncoder* @return RegisteredClientRepository*/@Beanpublic RegisteredClientRepository registeredClientRepository (JdbcTemplate jdbcTemplate, PasswordEncoder passwordEncoder) {RegisteredClient registeredClient = RegisteredClient.withId (UUID.randomUUID ().toString ()).clientId ("oauth2-client").clientSecret (passwordEncoder.encode ("123456"))// 客户端认证基于请求头.clientAuthenticationMethod (ClientAuthenticationMethod.CLIENT_SECRET_BASIC)// 配置授权的支持方式.authorizationGrantType (AuthorizationGrantType.AUTHORIZATION_CODE).authorizationGrantType (AuthorizationGrantType.REFRESH_TOKEN).authorizationGrantType (AuthorizationGrantType.CLIENT_CREDENTIALS).redirectUri ("https://www.baidu.com").scope ("user").scope ("admin")// 客户端设置,设置用户需要确认授权.clientSettings (ClientSettings.builder ().requireAuthorizationConsent (true).build ()).build ();JdbcRegisteredClientRepository registeredClientRepository = new JdbcRegisteredClientRepository (jdbcTemplate);RegisteredClient repositoryByClientId = registeredClientRepository.findByClientId (registeredClient.getClientId ());if (repositoryByClientId == null) {registeredClientRepository.save (registeredClient);}return registeredClientRepository;}/*** 用于签署访问令牌** @return JWKSource*/@Beanpublic JWKSource<SecurityContext> jwkSource () {KeyPair keyPair = generateRsaKey ();RSAPublicKey publicKey = (RSAPublicKey) keyPair.getPublic ();RSAPrivateKey privateKey = (RSAPrivateKey) keyPair.getPrivate ();RSAKey rsaKey = new RSAKey.Builder (publicKey).privateKey (privateKey).keyID (UUID.randomUUID ().toString ()).build ();JWKSet jwkSet = new JWKSet (rsaKey);return new ImmutableJWKSet<> (jwkSet);}/*** 创建RsaKey** @return KeyPair*/private static KeyPair generateRsaKey () {KeyPair keyPair;try {KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance ("RSA");keyPairGenerator.initialize (2048);keyPair = keyPairGenerator.generateKeyPair ();} catch (Exception e) {log.error ("generateRsaKey Exception", e);throw new ServiceException ("generateRsaKey Exception");}return keyPair;}/*** 解码签名访问令牌** @param jwkSource jwkSource* @return JwtDecoder*/@Beanpublic JwtDecoder jwtDecoder (JWKSource<SecurityContext> jwkSource) {return OAuth2AuthorizationServerConfiguration.jwtDecoder (jwkSource);}@Beanpublic AuthorizationServerSettings authorizationServerSettings () {return AuthorizationServerSettings.builder ().build ();}}
详细介绍
SecurityFilterChain authorizationServerSecurityFilterChain (HttpSecurity http)
Spring Security的过滤器链,用于协议端点
SecurityFilterChain defaultSecurityFilterChain (HttpSecurity http)
Security的过滤器链,用于Security的身份认证
PasswordEncoder passwordEncoder ()
配置密码解析器,使用BCrypt的方式对密码进行加密和验证
UserDetailsService userDetailsService ()
用于进行用户身份验证
RegisteredClientRepository registeredClientRepository (JdbcTemplate jdbcTemplate, PasswordEncoder passwordEncoder)
用于管理客户端
JWKSource<SecurityContext> jwkSource ()
用于签署访问令牌
KeyPair generateRsaKey ()
创建RsaKey
JwtDecoder jwtDecoder (JWKSource<SecurityContext> jwkSource)
解码签名访问令牌
AuthorizationServerSettings authorizationServerSettings ()
配置Spring Authorization Server的AuthorizationServerSettings实例
初始化自带的数据表
自带的表在spring-security-oauth2-authorization-server-1.2.0.jar 中 下面是对应的截图
对应的SQL
-- 已注册的客户端信息表
CREATE TABLE oauth2_registered_client
(id varchar(100) NOT NULL,client_id varchar(100) NOT NULL,client_id_issued_at timestamp DEFAULT CURRENT_TIMESTAMP NOT NULL,client_secret varchar(200) DEFAULT NULL,client_secret_expires_at timestamp DEFAULT NULL,client_name varchar(200) NOT NULL,client_authentication_methods varchar(1000) NOT NULL,authorization_grant_types varchar(1000) NOT NULL,redirect_uris varchar(1000) DEFAULT NULL,post_logout_redirect_uris varchar(1000) DEFAULT NULL,scopes varchar(1000) NOT NULL,client_settings varchar(2000) NOT NULL,token_settings varchar(2000) NOT NULL,PRIMARY KEY (id)
);-- 认证授权表
CREATE TABLE oauth2_authorization_consent
(registered_client_id varchar(100) NOT NULL,principal_name varchar(200) NOT NULL,authorities varchar(1000) NOT NULL,PRIMARY KEY (registered_client_id, principal_name)
);
/*
IMPORTANT:If using PostgreSQL, update ALL columns defined with 'blob' to 'text',as PostgreSQL does not support the 'blob' data type.
*/
-- 认证信息表
CREATE TABLE oauth2_authorization
(id varchar(100) NOT NULL,registered_client_id varchar(100) NOT NULL,principal_name varchar(200) NOT NULL,authorization_grant_type varchar(100) NOT NULL,authorized_scopes varchar(1000) DEFAULT NULL,attributes blob DEFAULT NULL,state varchar(500) DEFAULT NULL,authorization_code_value blob DEFAULT NULL,authorization_code_issued_at timestamp DEFAULT NULL,authorization_code_expires_at timestamp DEFAULT NULL,authorization_code_metadata blob DEFAULT NULL,access_token_value blob DEFAULT NULL,access_token_issued_at timestamp DEFAULT NULL,access_token_expires_at timestamp DEFAULT NULL,access_token_metadata blob DEFAULT NULL,access_token_type varchar(100) DEFAULT NULL,access_token_scopes varchar(1000) DEFAULT NULL,oidc_id_token_value blob DEFAULT NULL,oidc_id_token_issued_at timestamp DEFAULT NULL,oidc_id_token_expires_at timestamp DEFAULT NULL,oidc_id_token_metadata blob DEFAULT NULL,refresh_token_value blob DEFAULT NULL,refresh_token_issued_at timestamp DEFAULT NULL,refresh_token_expires_at timestamp DEFAULT NULL,refresh_token_metadata blob DEFAULT NULL,user_code_value blob DEFAULT NULL,user_code_issued_at timestamp DEFAULT NULL,user_code_expires_at timestamp DEFAULT NULL,user_code_metadata blob DEFAULT NULL,device_code_value blob DEFAULT NULL,device_code_issued_at timestamp DEFAULT NULL,device_code_expires_at timestamp DEFAULT NULL,device_code_metadata blob DEFAULT NULL,PRIMARY KEY (id)
);
application.yml中数据库配置
spring:profiles:active: devdatasource:type: com.alibaba.druid.pool.DruidDataSourcedriver-class-name: com.mysql.cj.jdbc.Driverurl: jdbc:mysql://192.168.0.1:3306/auth?characterEncoding=utf8&useSSL=false&serverTimezone=GMT%2B8&rewriteBatchedStatements=true&allowPublicKeyRetrieval=trueusername: authpassword: 12345
启动AuthServerApplication
启动完成后查看数据库的oauth2_registered_client表中有一条数据;
查看授权服务配置
地址:http://127.0.0.1:8801/.well-known/openid-configuration
访问/oauth2/authorize前往登录页面
地址:ip/端口/oauth2/authorize?client_id=app-client&response_type=code&scope=user&redirect_uri=https://www.baidu.com
实例:
http://127.0.0.1:8801/oauth2/authorize?client_id=app-client&response_type=code&scope=user&redirect_uri=https://www.baidu.com
浏览器跳转到:http://127.0.0.1:8801/login
输入上面配置的密码和账号。我这里都是:chengxuyuanshitang 点击提交。跳转
跳转到
网址栏的地址:https://www.baidu.com/?code=S73PUvl26OSxBc-yBbRRPJMTzcvE2x-VFZGXFPjpvOXHrecbY3Thsj6aOxPdN31H4a6GUgujSc1D4lj9D1ApIUAfZi55YJLqiRLpCivb-Is_4h3grILgR8H8M9UWyhJt
code的值就是=后面的
code=S73PUvl26OSxBc-yBbRRPJMTzcvE2x-VFZGXFPjpvOXHrecbY3Thsj6aOxPdN31H4a6GUgujSc1D4lj9D1ApIUAfZi55YJLqiRLpCivb-Is_4h3grILgR8H8M9UWyhJt
获取token
请求地址:http://127.0.0.1:8801/oauth2/token?grant_type=authorization_code&redirect_uri=https://www.baidu.com&code=S73PUvl26OSxBc-yBbRRPJMTzcvE2x-VFZGXFPjpvOXHrecbY3Thsj6aOxPdN31H4a6GUgujSc1D4lj9D1ApIUAfZi55YJLqiRLpCivb-Is_4h3grILgR8H8M9UWyhJt
用postman请求
添加header参数
header中的 Authorization参数:因为我们用的客户端认证方式 为 client_secret_basic ,这个需要传参,还有一些其他的认证方式,
client_secret_basic: 将 clientId 和 clientSecret 通过 : 号拼接,并使用 Base64 进行编码得到一串字符,再在前面加个 注意有个 Basic 前缀(Basic后有一个空格), 即得到上面参数中的 Basic 。
我的是:app-client:123456
Base64 进行编码:YXBwLWNsaWVudDoxMjM0NTY=
返回:
{"access_token": "eyJraWQiOiI2ZTJmYTA5ZS0zMmYzLTQ0MmQtOTM4Zi0yMzJjNDViYTM1YmMiLCJhbGciOiJSUzI1NiJ9.eyJzdWIiOiJjaGVuZ3h1eXVhbnNoaXRhbmciLCJhdWQiOiJhcHAtY2xpZW50IiwibmJmIjoxNzE1MDcxOTczLCJzY29wZSI6WyJ1c2VyIl0sImlzcyI6Imh0dHA6Ly8xMjcuMC4wLjE6ODgwMSIsImV4cCI6MTcxNTA3MjI3MywiaWF0IjoxNzE1MDcxOTczLCJqdGkiOiI0MWI4ZGZmZS03MTI2LTQ4NWYtODRmYy00Yjk4OGE0N2ZlMzUifQ.VxP2mLHt-eyXHZOI36yhVlwC2UQEdAtaRBKTWwJn1bFup0ZjGbZfgxENUb1c03yjcki2H-gCW4Jgef11BMNtjyWSnwMHVWLB9fcT3rRKDQWwoWqBYAcULS8oC5n8qTZwffDSrnjepMEbw4CblL3oH7T9nLProTXQP326RIE1RczsUYkteUCkyIvKTSs3ezOjIVR1GyCs_Cl1A_3OllmkGnSO2q-NKkwasrQjMuuPTY3nhDyDGiefYlfDEcmzz1Yk_FE42P7PEeyqmZwAj7vUnE4brQuNqipaMsS7INe_wTE1kJv-arfbnUo_zQdipHxIhsDgoLaPlSSecQ31QgwEHA","refresh_token": "TqJyWbLWe5Yww6dOV89zDbO0C3YEBA__0TJU_GclmQTAH92SSQ2OvdMChIdln97u1WsA7G7n3BqzNZBjPRU7xmkRooa5ifsMBJ-d3C4kPmuPQI-Bmbq20pck-QEk0Dqt","scope": "user","token_type": "Bearer","expires_in": 300
}
访问接口/userinfo
请求地址:http://127.0.0.1:8801/userinfo
添加header参数:Authorization: Bearer +空格+ ${access_token}
相关文章:
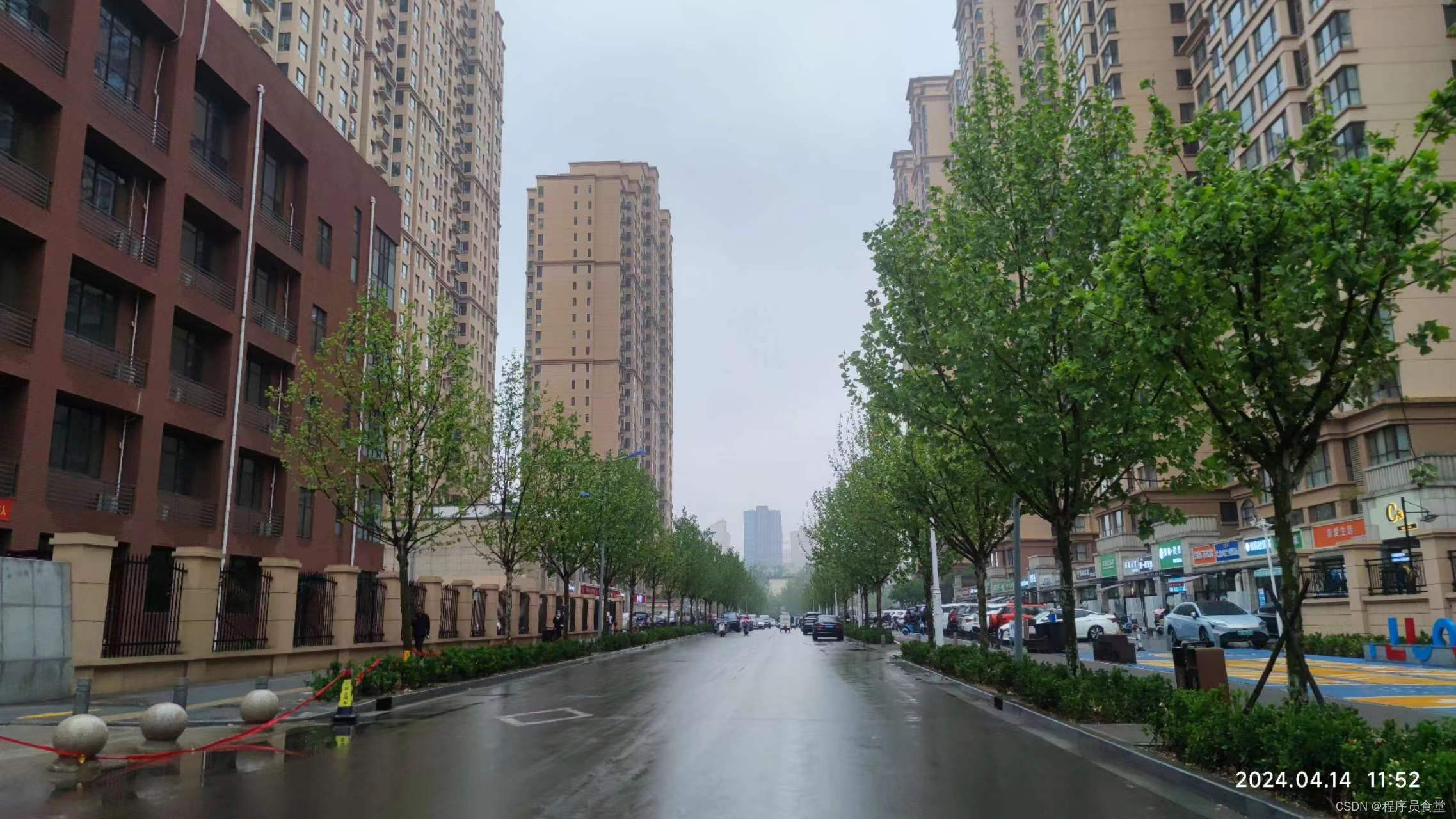
springboot3 集成spring-authorization-server (一 基础篇)
官方文档 Spring Authorization Server 环境介绍 java:17 SpringBoot:3.2.0 SpringCloud:2023.0.0 引入maven配置 <dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter…...
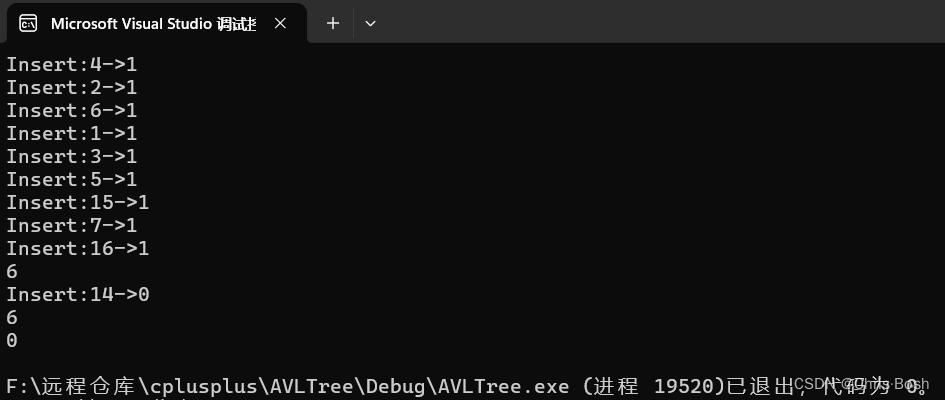
AVL树!
文章目录 1.AVL树的概念2.AVL树的插入和旋转3.AVL树的旋转3.1旋转的底层:3.2 右旋转3.3 左旋转3.4 双旋 4.AVL树的底层 1.AVL树的概念 当向二叉搜索树中插入新结点后,如果能保证每个结点的左右子树高度之差的绝对值不超过1(需要对树中的结点进行调整)&a…...
知识付费系统怎么安装教程,教师课堂教学该掌握哪些表达技巧?
课堂教学语言表达是教学艺术的一个基本且重要的组成部分。教师向学生传道、授业、解惑以及师生之间信息的传递和情感的交流,都离不开运用教学语言这一有力的工具,在课堂上,教师通过情趣盎然的表述,鞭辟入里的分析,恰到…...
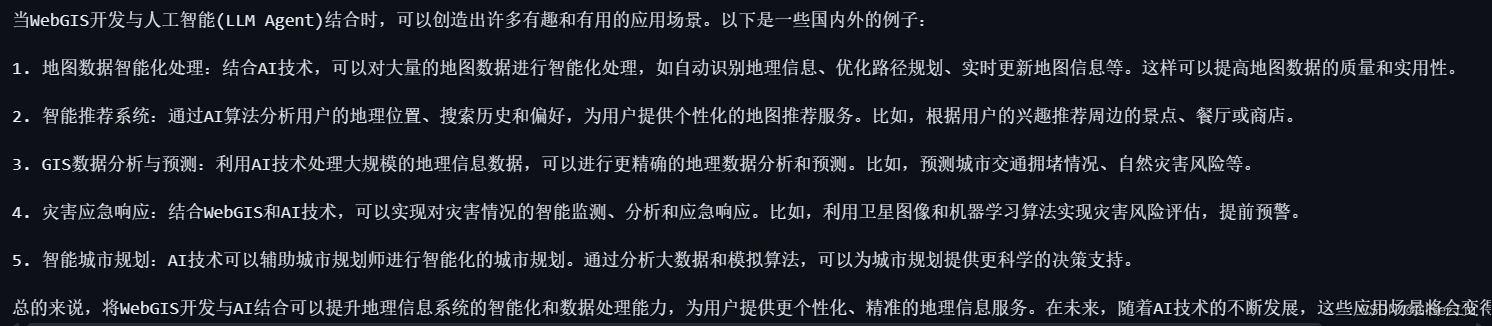
基于MetaGPT的LLM Agent学习实战(一)
前言 我最近一直在做基于AI Agent 的个人项目, 因为工作加班较多,设计思考时间不足,这里借着Datawhale的开源学习课程《MetaGPT智能体理论与实战》课程,来完善自己的思路,抛砖引玉,和各位开发者一起学习&am…...
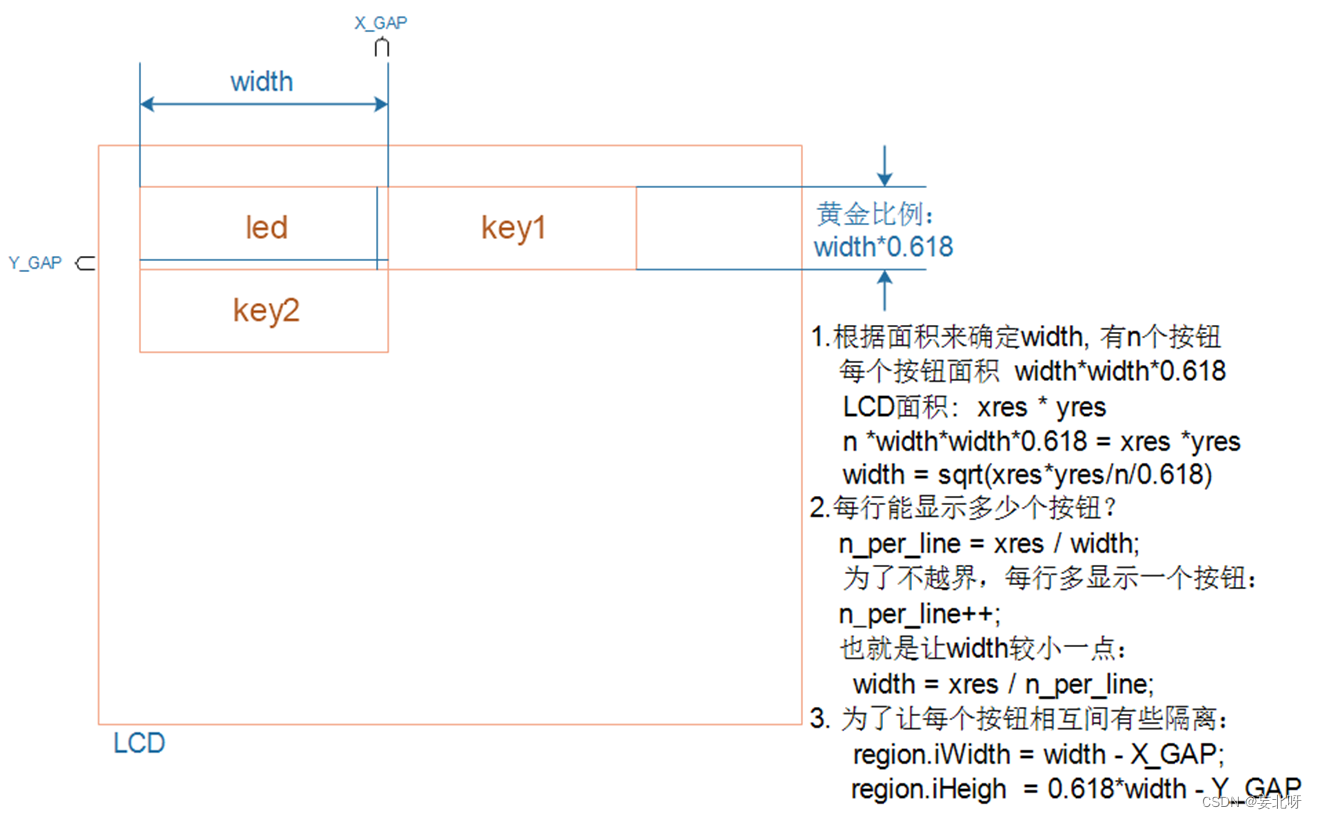
【IMX6ULL项目】IMX6ULL上Linux系统实现产测工具框架
电子产品量产测试与烧写工具。这是一套软件,用在我们的实际生产中, 有如下特点: 1.简单易用: 把这套软件烧写在 SD 卡上,插到 IMX6ULL 板子里并启动,它就会自动测试各个模块、烧写 EMMC 系统。 工人只要按…...
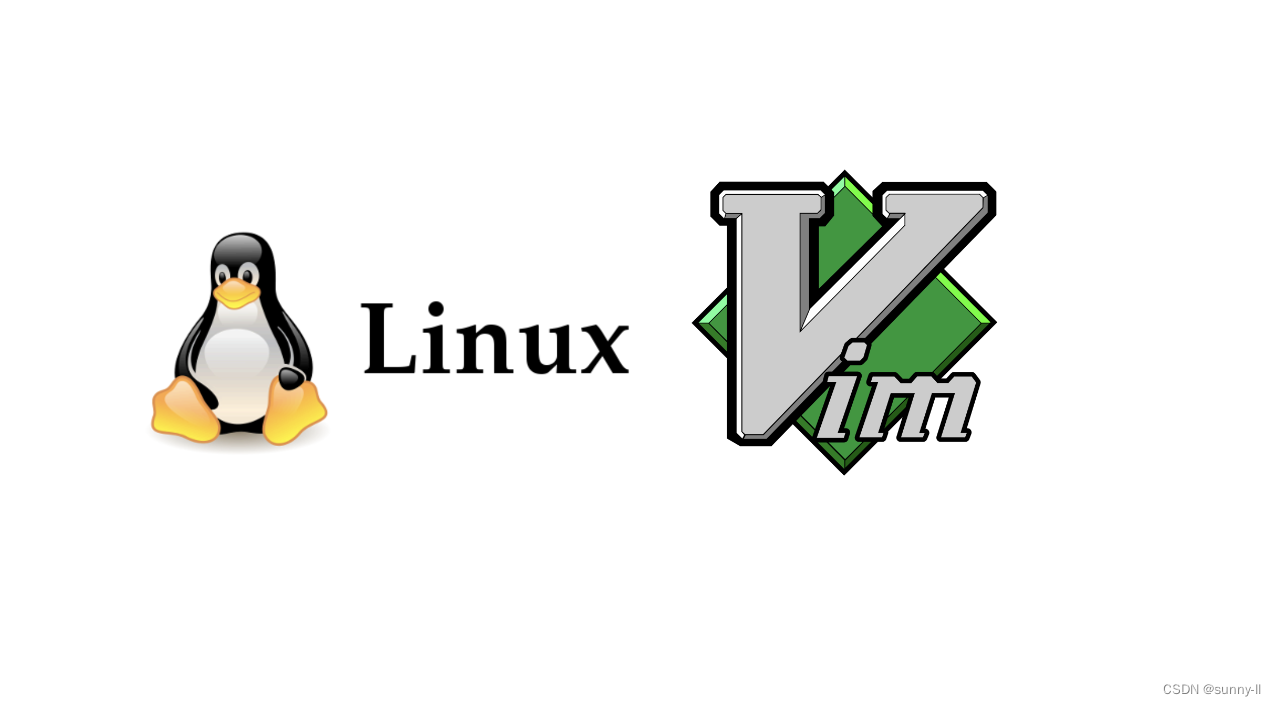
【Linux基础】Vim保姆级一键配置教程(手把手教你把Vim打造成高效率C++开发环境)
目录 一、前言 二、安装Vim 三、原始Vim编译器的缺陷分析 四、Vim配置 🥝预备知识----.vimrc 隐藏文件 🍋手动配置 Vim --- (不推荐) 🍇自动化一键配置 Vim --- (强烈推荐) ✨功能演示 五、共勉 一、前言 Vim作为…...
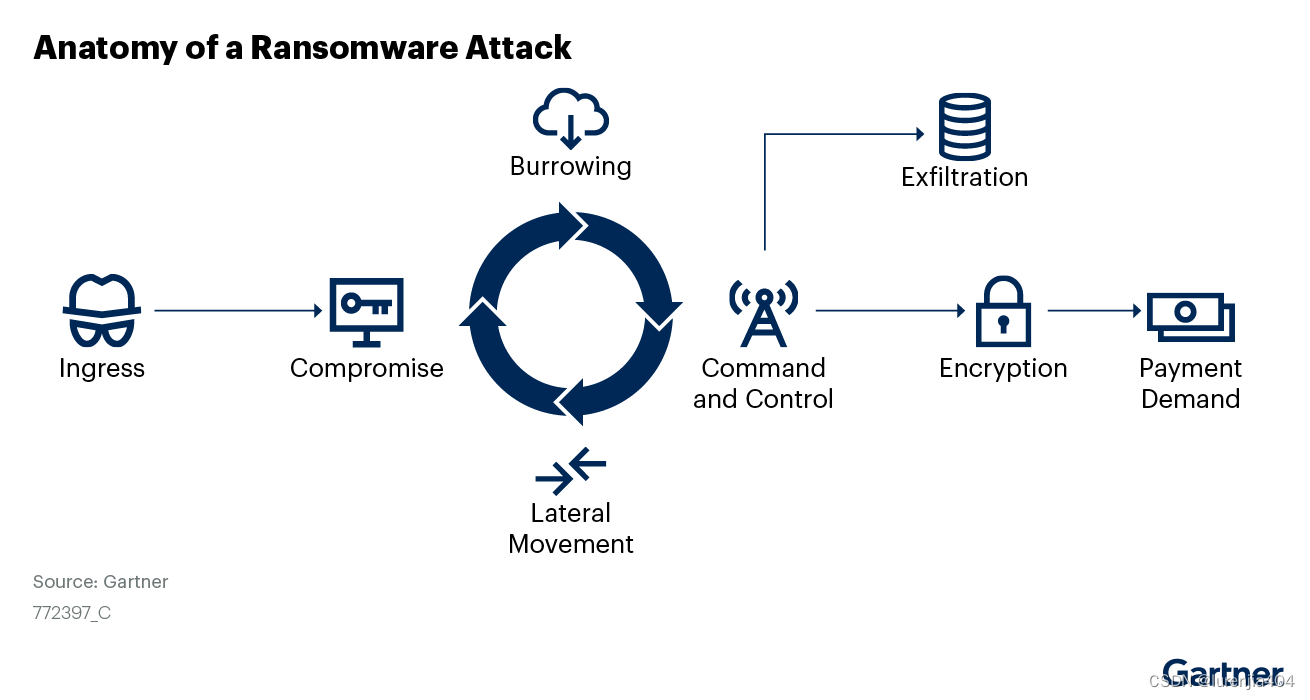
Gartner发布准备应对勒索软件攻击指南:勒索软件攻击的三个阶段及其防御生命周期
攻击者改变了策略,在某些情况下转向勒索软件。安全和风险管理领导者必须通过提高检测和预防能力来为勒索软件攻击做好准备,同时还要改进其事后应对策略。 主要发现 勒索软件(无加密的数据盗窃攻击)是攻击者越来越多地使用的策略。…...
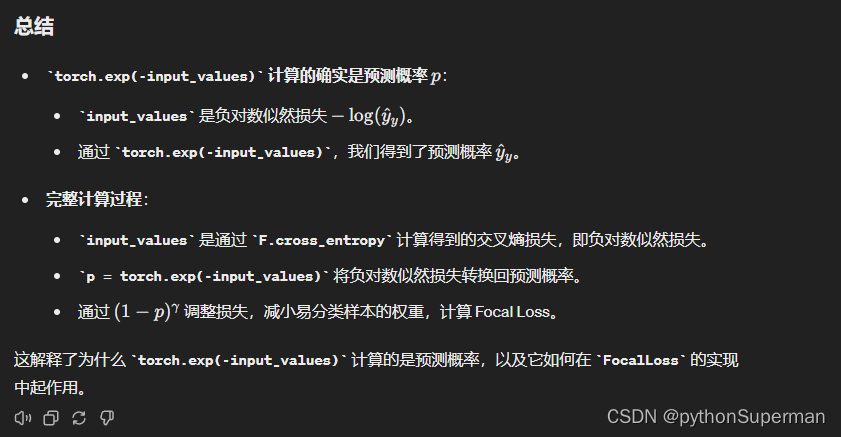
IB 公式解析
IB损失 自我感悟 根据对决策边界的影响程度来分配权重。影响程度越大,分配到的权重越小;影响程度越小,分配到的权重越大。 最后其实就是平衡因子和交叉熵损失的输出的乘积 公式 3.2. Influence Function 影响函数允许我们在移除样本时估…...
开发辅助工具的缩写
开发辅助工具的缩写有很多,这些工具通常是为了提高软件开发效率、代码质量和团队协作效率而设计的。以下是一些常见的开发辅助工具及其缩写: IDE - Integrated Development Environment(集成开发环境) VCS - Version Control Sys…...
linux程序分析命令(一)
linux程序分析命令(一) **ldd:**用于打印共享库依赖。这个命令会显示出一个可执行文件所依赖的所有共享库(动态链接库),这对于解决运行时库依赖问题非常有用。**nm:**用于列出对象文件的符号表。这个命令可以显示出定…...
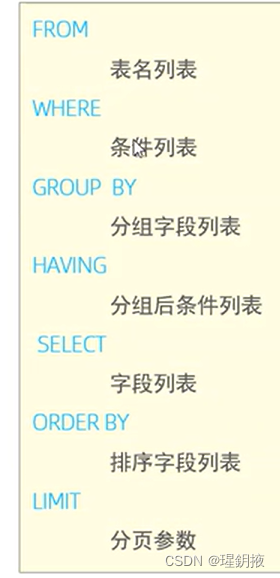
MYSQL数据库-SQL语句
数据库相关概念 名称全称简称数据库存储数据的仓库,数据是有组织的进行存储DataBase(DB)数据库管理系统操纵和管理数据库的大型软件DataBase Management System(DBMS)SQL操作关系型数据库的编程语言,定义了一套操作关系型数据库统一标准Structured Quer…...

MyBatis认识
一、定义 MyBatis是一款优秀的持久层框架,它支持自定义 SQL、存储过程以及高级映射。MyBatis 免除了几乎所有的 JDBC 代码以及设置参数和获取结果集的工作。MyBatis 可以通过简单的 XML 或注解来配置和映射原始类型、接口和 Java POJO(Plain Old Java O…...
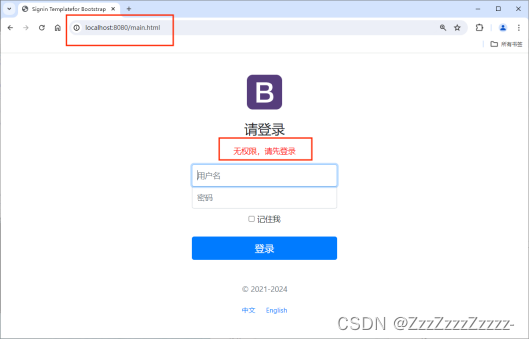
【WEEK11】 【DAY6】Employee Management System Part 7【English Version】
2024.5.11 Saturday Continued from 【WEEK11】 【DAY5】Employee Management System Part 6【English Version】 Contents 10.8. Delete and 404 Handling10.8.1. Modify list.html10.8.2. Modify EmployeeController.java10.8.3. Restart10.8.4. 404 Page Handling10.8.4.1. …...
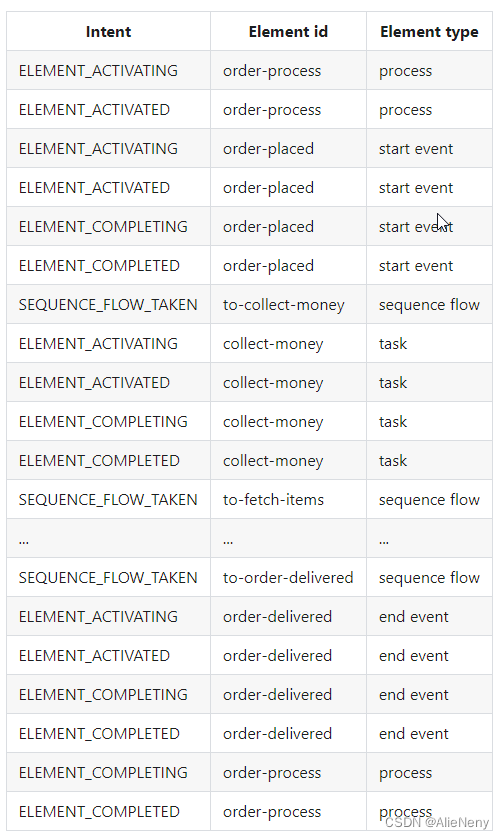
【52】Camunda8-Zeebe核心引擎-Clustering与流程生命周期
Clustering集群 Zeebe本质是作为一个brokers集群运行,形成一个点对点网络。在这个网络中,所有brokers的功能与服务都相同,没有单点故障。 Gossip协议 Zeebe实现了gossip协议,并借此知悉哪些broker当前是集群的一部分。 集群使用…...
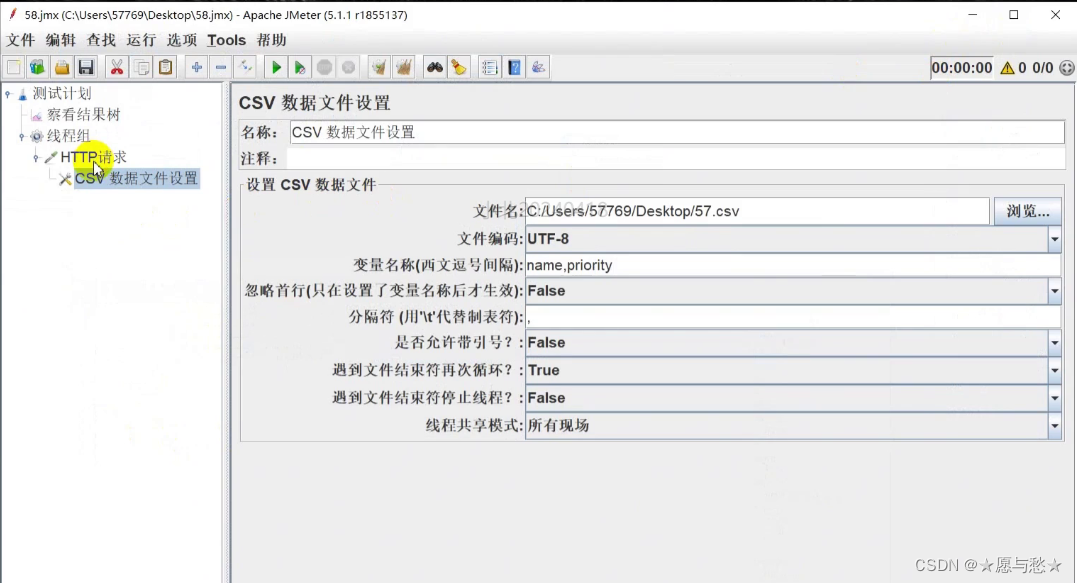
从零开始的软件测试学习之旅(八)jmeter线程组参数化及函数学习
jmeter线程组参数化及函数学习 Jmeter基础基本使用流程组件与元件 线程组线程的执行方式Jmeter组件执行顺序 常见属性设置查看结果数的作用域举例 Jmeter参数化实现方式1.用户定义参数2.用户参数3.函数4.csv数据文件设置 每日复习 Jmeter基础 基本使用流程 启动项目案例 启动…...
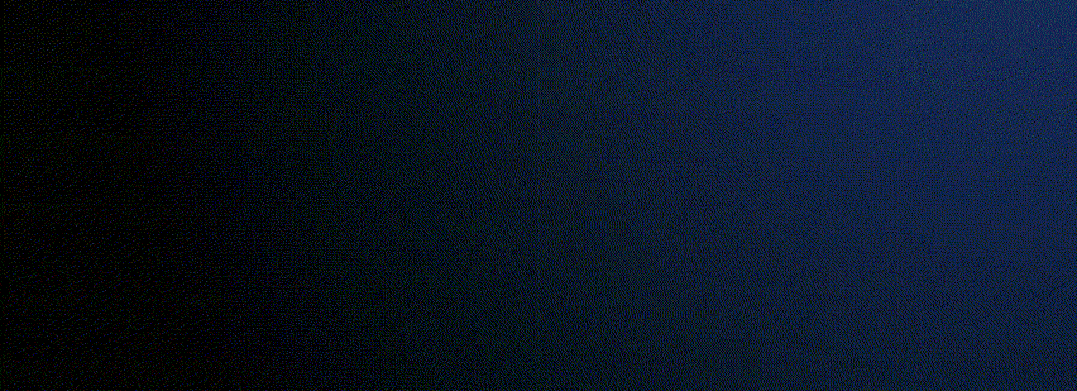
图文并茂:解析Spring Boot Controller返回图片的三种方式
欢迎来到我的博客,代码的世界里,每一行都是一个故事 图文并茂:解析Spring Boot Controller返回图片的三种方式 前言使用Base64编码返回图片使用byte数组返回图片使用Resource对象返回图片图片格式转换与性能对比 前言 在互联网的世界里&…...
问题处理记录 | 表输出报错 Packet for query is too large (5,214,153 > 4,194,304).
这个错误是由于MySQL服务器接收到的查询数据包太大而引起的。具体来说,错误消息中提到的数据包大小为5,214,153字节,而MySQL服务器默认只接受最大为4,194,304字节的数据包。 要解决这个问题,你可以尝试通过修改MySQL服务器的配置来增大max_a…...
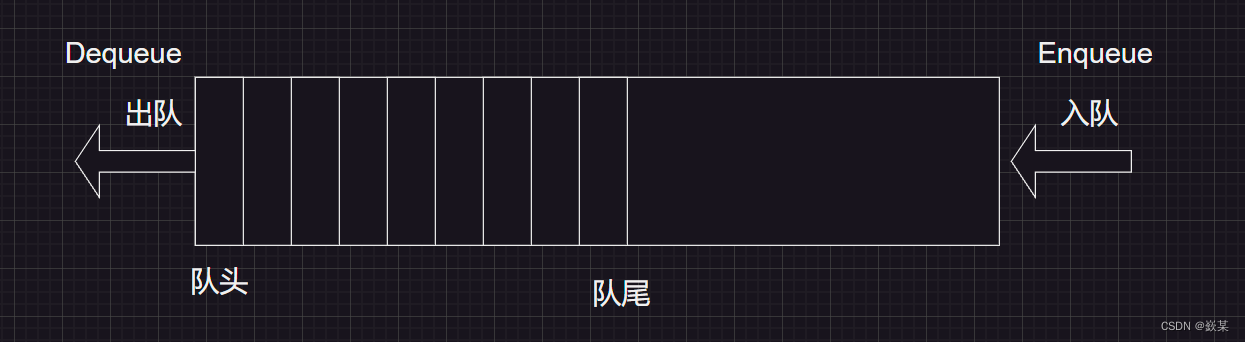
数据结构_栈和队列(Stack Queue)
✨✨所属专栏:数据结构✨✨ ✨✨作者主页:嶔某✨✨ 栈: 代码:function/数据结构_栈/stack.c 钦某/c-language-learning - 码云 - 开源中国 (gitee.com)https://gitee.com/wang-qin928/c-language-learning/blob/master/function/…...
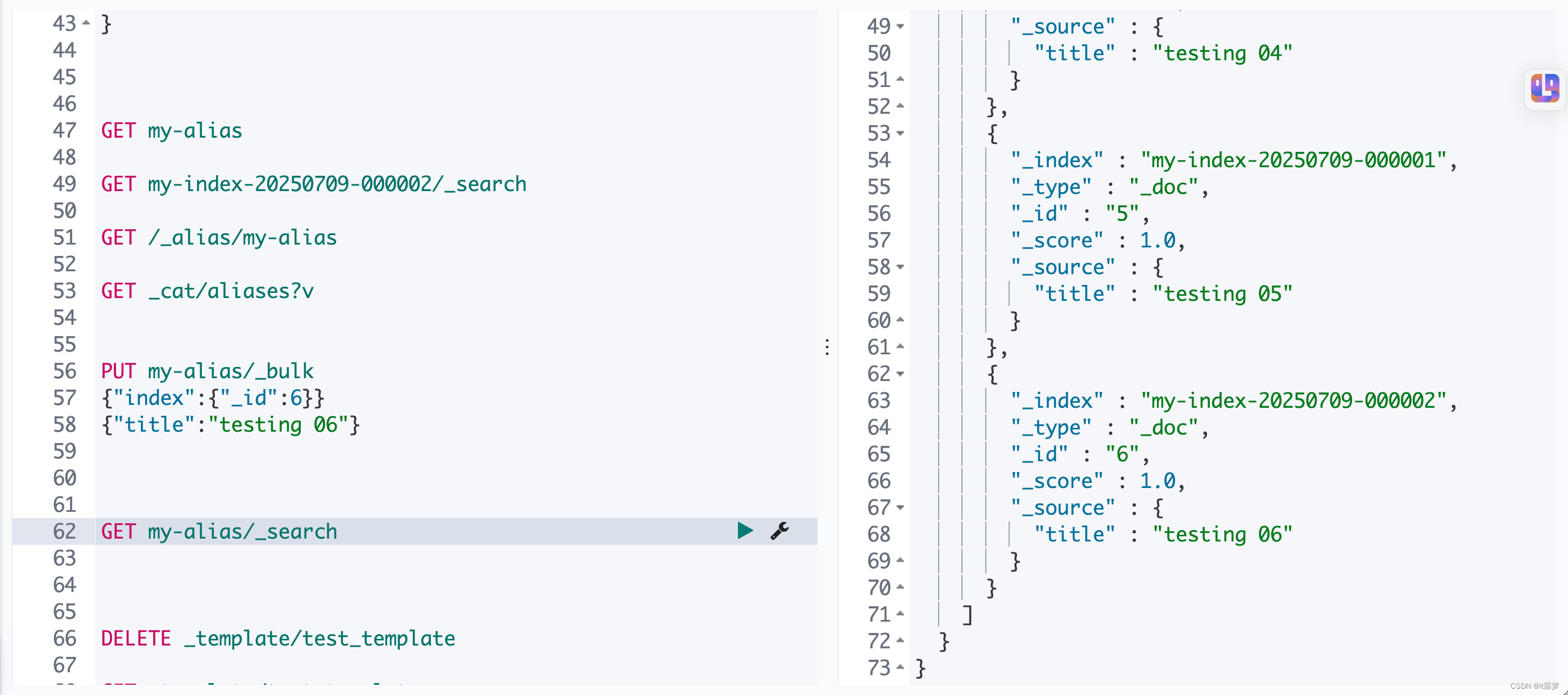
基于docker 的elasticsearch冷热分离及生命周期管理
文章目录 冷热集群架构的应用场景冷热集群架构的优势冷热集群架构实战搭建集群 索引生命周期管理认识索引生命周期索引生命周期管理的历史演变索引生命周期管理的基础知识Rollover:滚动索引 冷热集群架构的应用场景 某客户的线上业务场景如下:系统每天增…...
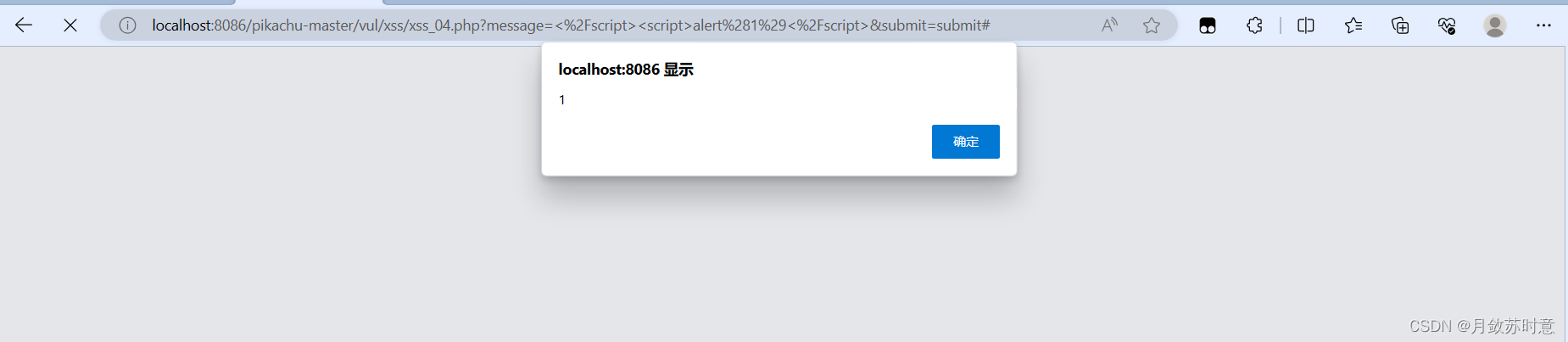
pikachu靶场(xss通关教程)
(注:若复制注入代码攻击无效,请手动输入注入语句,在英文输入法下) 反射型xss(get型) 1.打开网站 发现有个框,然后我们在框中输入一个“1”进行测试, 可以看到提交的数据在url处有显示…...
Leetcode 3577. Count the Number of Computer Unlocking Permutations
Leetcode 3577. Count the Number of Computer Unlocking Permutations 1. 解题思路2. 代码实现 题目链接:3577. Count the Number of Computer Unlocking Permutations 1. 解题思路 这一题其实就是一个脑筋急转弯,要想要能够将所有的电脑解锁&#x…...
Java多线程实现之Callable接口深度解析
Java多线程实现之Callable接口深度解析 一、Callable接口概述1.1 接口定义1.2 与Runnable接口的对比1.3 Future接口与FutureTask类 二、Callable接口的基本使用方法2.1 传统方式实现Callable接口2.2 使用Lambda表达式简化Callable实现2.3 使用FutureTask类执行Callable任务 三、…...
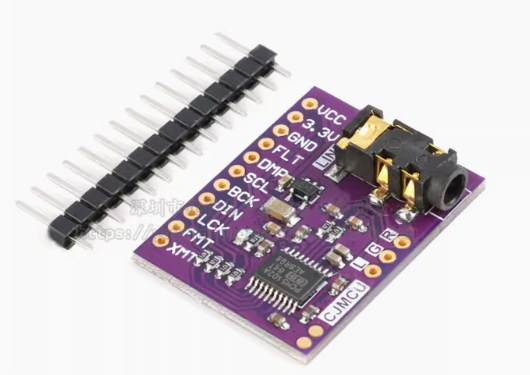
ESP32 I2S音频总线学习笔记(四): INMP441采集音频并实时播放
简介 前面两期文章我们介绍了I2S的读取和写入,一个是通过INMP441麦克风模块采集音频,一个是通过PCM5102A模块播放音频,那如果我们将两者结合起来,将麦克风采集到的音频通过PCM5102A播放,是不是就可以做一个扩音器了呢…...
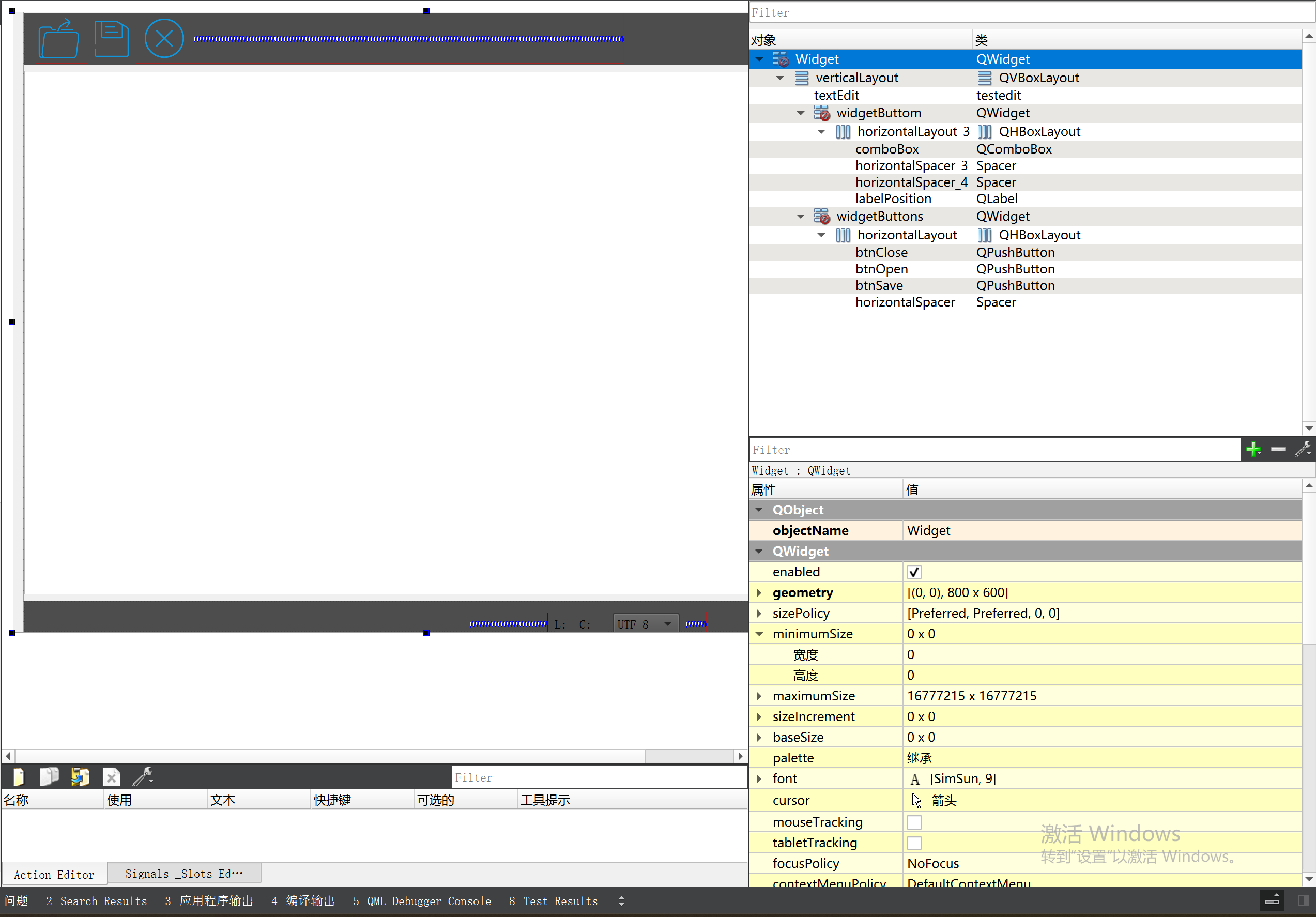
P3 QT项目----记事本(3.8)
3.8 记事本项目总结 项目源码 1.main.cpp #include "widget.h" #include <QApplication> int main(int argc, char *argv[]) {QApplication a(argc, argv);Widget w;w.show();return a.exec(); } 2.widget.cpp #include "widget.h" #include &q…...
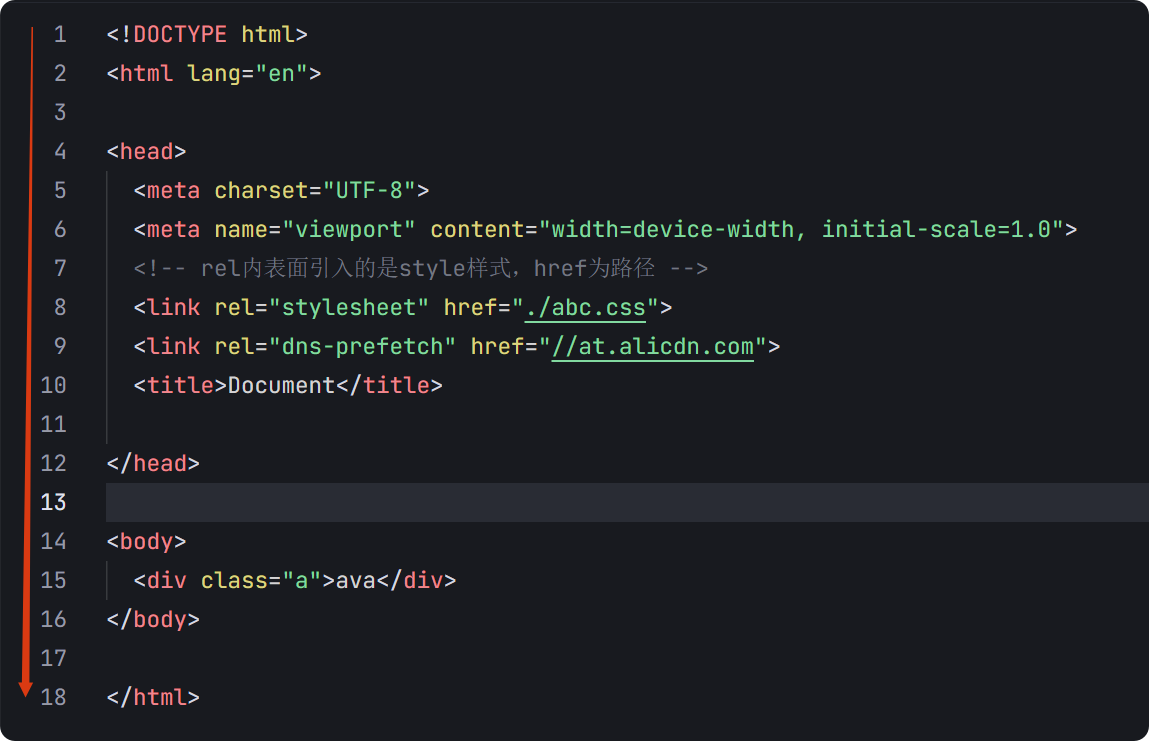
04-初识css
一、css样式引入 1.1.内部样式 <div style"width: 100px;"></div>1.2.外部样式 1.2.1.外部样式1 <style>.aa {width: 100px;} </style> <div class"aa"></div>1.2.2.外部样式2 <!-- rel内表面引入的是style样…...
浅谈不同二分算法的查找情况
二分算法原理比较简单,但是实际的算法模板却有很多,这一切都源于二分查找问题中的复杂情况和二分算法的边界处理,以下是博主对一些二分算法查找的情况分析。 需要说明的是,以下二分算法都是基于有序序列为升序有序的情况…...
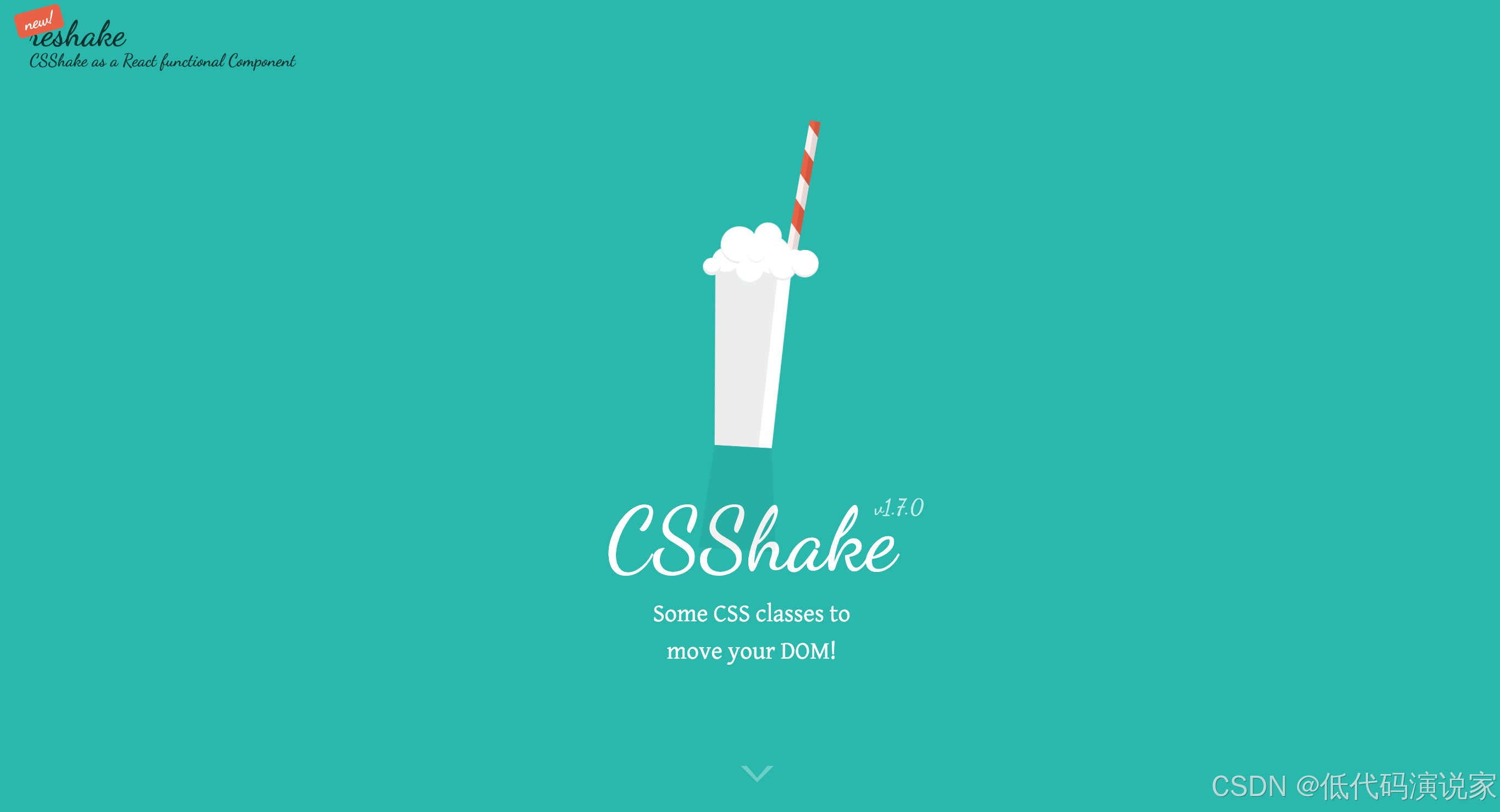
20个超级好用的 CSS 动画库
分享 20 个最佳 CSS 动画库。 它们中的大多数将生成纯 CSS 代码,而不需要任何外部库。 1.Animate.css 一个开箱即用型的跨浏览器动画库,可供你在项目中使用。 2.Magic Animations CSS3 一组简单的动画,可以包含在你的网页或应用项目中。 3.An…...
python爬虫——气象数据爬取
一、导入库与全局配置 python 运行 import json import datetime import time import requests from sqlalchemy import create_engine import csv import pandas as pd作用: 引入数据解析、网络请求、时间处理、数据库操作等所需库。requests:发送 …...
0x-3-Oracle 23 ai-sqlcl 25.1 集成安装-配置和优化
是不是受够了安装了oracle database之后sqlplus的简陋,无法删除无法上下翻页的苦恼。 可以安装readline和rlwrap插件的话,配置.bahs_profile后也能解决上下翻页这些,但是很多生产环境无法安装rpm包。 oracle提供了sqlcl免费许可,…...
k8s从入门到放弃之HPA控制器
k8s从入门到放弃之HPA控制器 Kubernetes中的Horizontal Pod Autoscaler (HPA)控制器是一种用于自动扩展部署、副本集或复制控制器中Pod数量的机制。它可以根据观察到的CPU利用率(或其他自定义指标)来调整这些对象的规模,从而帮助应用程序在负…...