Netty中用到了哪些设计模式
Netty作为一个高性能的网络通信框架,里面有很多优秀的代码值得我们学习,今天我们一起看下Netty中用到了哪些设计模式。
一、单例模式
Netty通过 NioEventLoop 将通道注册到选择器,并在事件循环中多路复用它们。其中提供了一个选择策略对象 SelectStrategy,它只有一个默认实现:DefaultSelectStrategy。
/*** Default select strategy.*/
final class DefaultSelectStrategy implements SelectStrategy {static final SelectStrategy INSTANCE = new DefaultSelectStrategy();private DefaultSelectStrategy() { }@Overridepublic int calculateStrategy(IntSupplier selectSupplier, boolean hasTasks) throws Exception {return hasTasks ? selectSupplier.get() : SelectStrategy.SELECT;}
}
还有 ReadTimeoutException 和 WriteTimeoutException
/*** A {@link TimeoutException} raised by {@link ReadTimeoutHandler} when no data* was read within a certain period of time.*/
public final class ReadTimeoutException extends TimeoutException {private static final long serialVersionUID = 169287984113283421L;public static final ReadTimeoutException INSTANCE = PlatformDependent.javaVersion() >= 7 ?new ReadTimeoutException(true) : new ReadTimeoutException();ReadTimeoutException() { }private ReadTimeoutException(boolean shared) {super(shared);}
}
/*** A {@link TimeoutException} raised by {@link WriteTimeoutHandler} when a write operation* cannot finish in a certain period of time.*/
public final class WriteTimeoutException extends TimeoutException {private static final long serialVersionUID = -144786655770296065L;public static final WriteTimeoutException INSTANCE = PlatformDependent.javaVersion() >= 7 ?new WriteTimeoutException(true) : new WriteTimeoutException();private WriteTimeoutException() { }private WriteTimeoutException(boolean shared) {super(shared);}
}
二、工厂模式
工厂模式是非常常见的一种模式,Netty中也使用到,比如 上面提到的选择策略工厂: DefaultSelectStrategyFactory
/*** Factory which uses the default select strategy.*/
public final class DefaultSelectStrategyFactory implements SelectStrategyFactory {public static final SelectStrategyFactory INSTANCE = new DefaultSelectStrategyFactory();private DefaultSelectStrategyFactory() { }@Overridepublic SelectStrategy newSelectStrategy() {return DefaultSelectStrategy.INSTANCE;}
}
三、策略模式
在默认的事件执行选择工厂 DefaultEventExecutorChooserFactory 的 newChooser 方法中,根据数组参数的长度是否是2的幂 来选择不同的 EventExecutorChooser。两种方式都是简单的轮询方式,只是方式不同。
@Overridepublic EventExecutorChooser newChooser(EventExecutor[] executors) {if (isPowerOfTwo(executors.length)) {return new PowerOfTwoEventExecutorChooser(executors);} else {return new GenericEventExecutorChooser(executors);}}
private static final class PowerOfTwoEventExecutorChooser implements EventExecutorChooser {private final AtomicInteger idx = new AtomicInteger();private final EventExecutor[] executors;PowerOfTwoEventExecutorChooser(EventExecutor[] executors) {this.executors = executors;}@Overridepublic EventExecutor next() {return executors[idx.getAndIncrement() & executors.length - 1];}}private static final class GenericEventExecutorChooser implements EventExecutorChooser {// Use a 'long' counter to avoid non-round-robin behaviour at the 32-bit overflow boundary.// The 64-bit long solves this by placing the overflow so far into the future, that no system// will encounter this in practice.private final AtomicLong idx = new AtomicLong();private final EventExecutor[] executors;GenericEventExecutorChooser(EventExecutor[] executors) {this.executors = executors;}@Overridepublic EventExecutor next() {return executors[(int) Math.abs(idx.getAndIncrement() % executors.length)];}}
四、装饰者模式
WrappedByteBuf 就是对 ByteBuf的装饰,来实现对它的增加。
class WrappedByteBuf extends ByteBuf {protected final ByteBuf buf;protected WrappedByteBuf(ByteBuf buf) {if (buf == null) {throw new NullPointerException("buf");}this.buf = buf;}......
}
五、责任链模式
ChannelPipeline 就是用到了责任链模式,所谓的责任链模式是指:它允许多个对象在处理请求时形成一条链,每个对象都有机会处理请求,将请求沿着链传递,直到有一个对象处理它为止。
/*** The default {@link ChannelPipeline} implementation. It is usually created* by a {@link Channel} implementation when the {@link Channel} is created.*/
public class DefaultChannelPipeline implements ChannelPipeline {static final InternalLogger logger = InternalLoggerFactory.getInstance(DefaultChannelPipeline.class);private static final String HEAD_NAME = generateName0(HeadContext.class);private static final String TAIL_NAME = generateName0(TailContext.class);private static final FastThreadLocal<Map<Class<?>, String>> nameCaches =new FastThreadLocal<Map<Class<?>, String>>() {@Overrideprotected Map<Class<?>, String> initialValue() {return new WeakHashMap<Class<?>, String>();}};private static final AtomicReferenceFieldUpdater<DefaultChannelPipeline, MessageSizeEstimator.Handle> ESTIMATOR =AtomicReferenceFieldUpdater.newUpdater(DefaultChannelPipeline.class, MessageSizeEstimator.Handle.class, "estimatorHandle");final HeadContext head;final TailContext tail;private final Channel channel;private final ChannelFuture succeededFuture;private final VoidChannelPromise voidPromise;private final boolean touch = ResourceLeakDetector.isEnabled();private Map<EventExecutorGroup, EventExecutor> childExecutors;private volatile MessageSizeEstimator.Handle estimatorHandle;private boolean firstRegistration = true;/*** This is the head of a linked list that is processed by {@link #callHandlerAddedForAllHandlers()} and so process* all the pending {@link #callHandlerAdded0(AbstractChannelHandlerContext)}.** We only keep the head because it is expected that the list is used infrequently and its size is small.* Thus full iterations to do insertions is assumed to be a good compromised to saving memory and tail management* complexity.*/private PendingHandlerCallback pendingHandlerCallbackHead;/*** Set to {@code true} once the {@link AbstractChannel} is registered.Once set to {@code true} the value will never* change.*/private boolean registered;protected DefaultChannelPipeline(Channel channel) {this.channel = ObjectUtil.checkNotNull(channel, "channel");succeededFuture = new SucceededChannelFuture(channel, null);voidPromise = new VoidChannelPromise(channel, true);tail = new TailContext(this);head = new HeadContext(this);head.next = tail;tail.prev = head;}
相关文章:
Netty中用到了哪些设计模式
Netty作为一个高性能的网络通信框架,里面有很多优秀的代码值得我们学习,今天我们一起看下Netty中用到了哪些设计模式。 一、单例模式 Netty通过 NioEventLoop 将通道注册到选择器,并在事件循环中多路复用它们。其中提供了一个选择策略对象 S…...
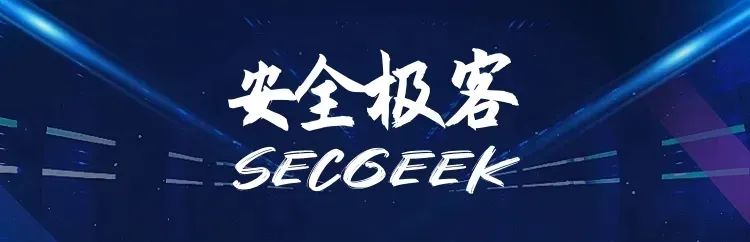
第67期 | GPTSecurity周报
GPTSecurity是一个涵盖了前沿学术研究和实践经验分享的社区,集成了生成预训练Transformer(GPT)、人工智能生成内容(AIGC)以及大语言模型(LLM)等安全领域应用的知识。在这里,您可以找…...

Chrome 浏览器插件获取网页 window 对象(方案三)
前言 最近有个需求,是在浏览器插件中获取 window 对象下的某个数据,当时觉得很简单,和 document 一样,直接通过嵌入 content_scripts 直接获取,然后使用 sendMessage 发送数据到插件就行了,结果发现不是这…...
动态规划-分割回文串ⅡⅣ
在本篇博客中将介绍分割回文串Ⅱ以及分割回文串Ⅳ这两个题目。 分割回文串Ⅱ 题目描述 给你一个字符串 s,请你将 s 分割成一些子串,使每个子串都是回文串。 返回符合要求的 最少分割次数 。 示例: 输入:s "aabac" 输…...
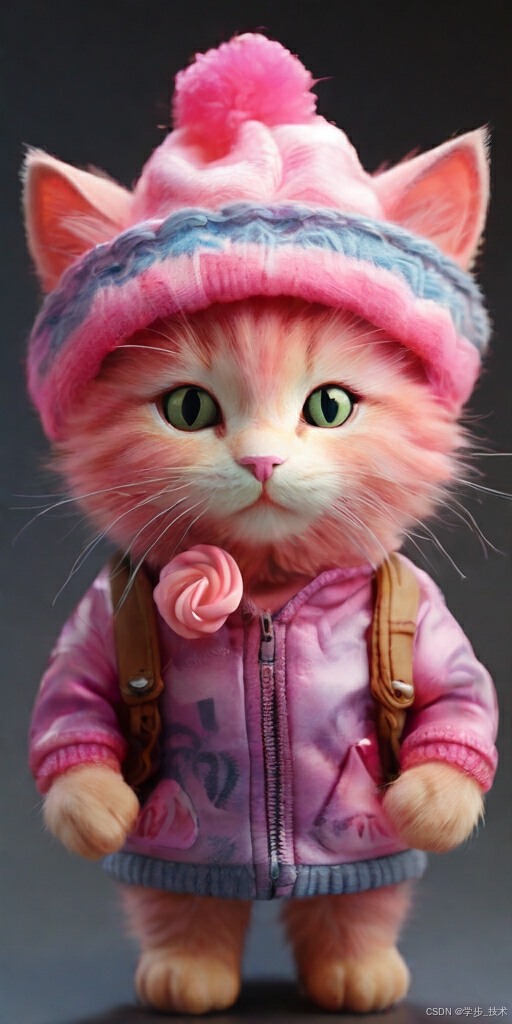
Python编码系列—Python项目维护与迭代:持续进化的艺术
🌟🌟 欢迎来到我的技术小筑,一个专为技术探索者打造的交流空间。在这里,我们不仅分享代码的智慧,还探讨技术的深度与广度。无论您是资深开发者还是技术新手,这里都有一片属于您的天空。让我们在知识的海洋中…...
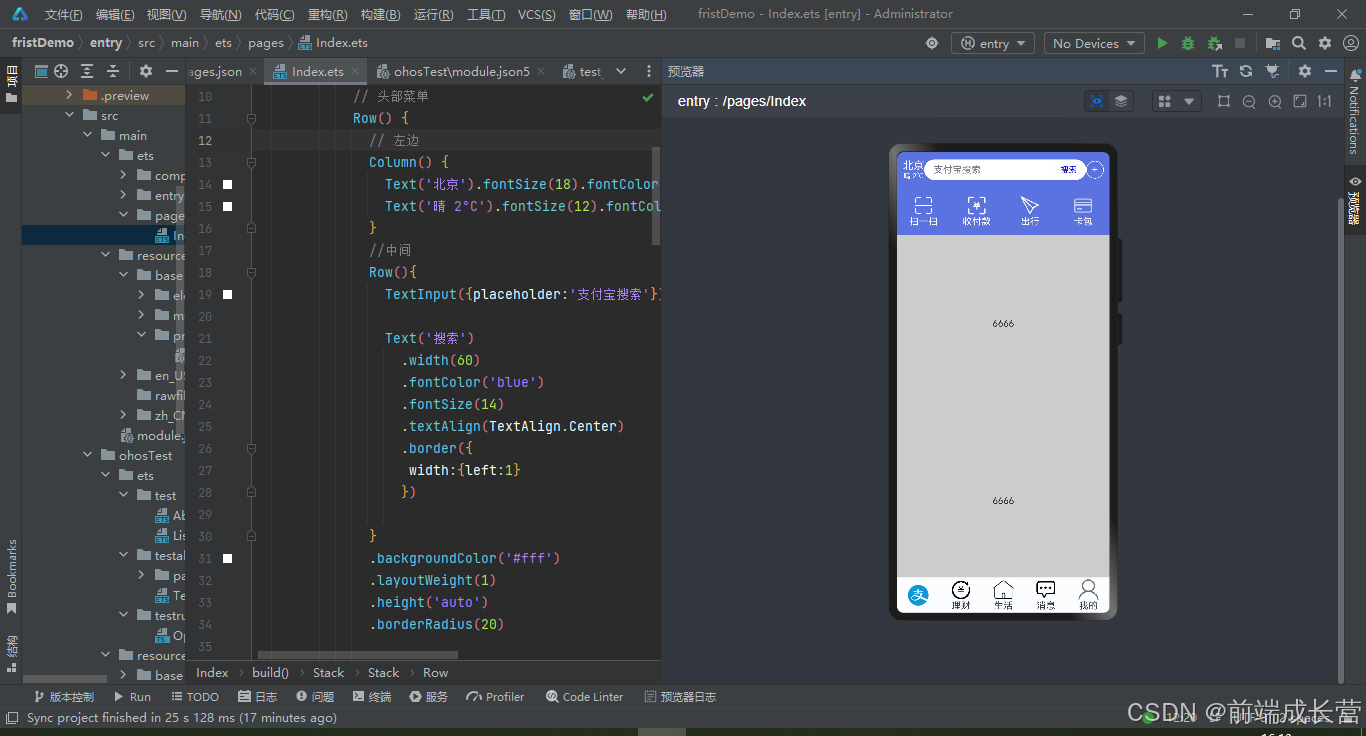
【鸿蒙开发工具报错】Build task failed. Open the Run window to view details.
Build task failed. Open the Run window to view details. 问题描述 在使用deveco-studio 开发工具进行HarmonyOS第一个应用构建开发时,通过Previewer预览页面时报错,报错信息为:Build task failed. Open the Run window to view details.…...
k8s集群部署:容器运行时
1. 卸载旧版本 Docker # 卸载旧版本的 Docker 组件 sudo yum remove docker \docker-client \docker-client-latest \docker-common \docker-latest \docker-latest-logrotate \docker-logrotate \docker-engine注释: 该命令会移除系统中现有的 Docker 及其相关组件࿰…...
PHP7 的内核结构
PHP7 是 PHP 语言的一个重要版本,带来了许多性能提升和语言特性改进。要深入了解 PHP7 的内核,我们需要探讨其设计和实现的关键方面,包括 PHP 的执行模型、内存管理、编译和优化过程等。 1. PHP7 的内核结构 1.1 执行模型 PHP 是一种解释型…...
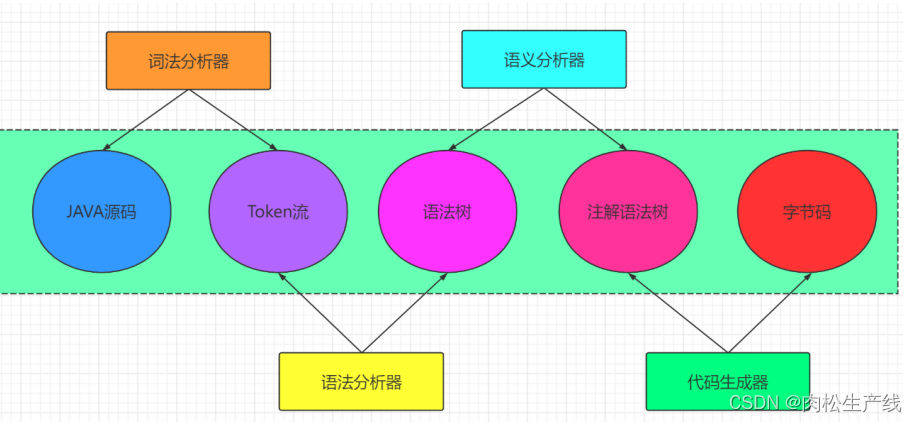
JVM合集
序言: 1.什么是JVM? JVM就是将javac编译后的.class字节码文件翻译为操作系统能执行的机器指令翻译过程: 前端编译:生成.class文件就是前端编译后端编译:通过jvm解释(或即时编译或AOT)执行.class文件时跨平台的,jvm并不是跨平台的通过javap进行反编译2.java文件是怎么变…...
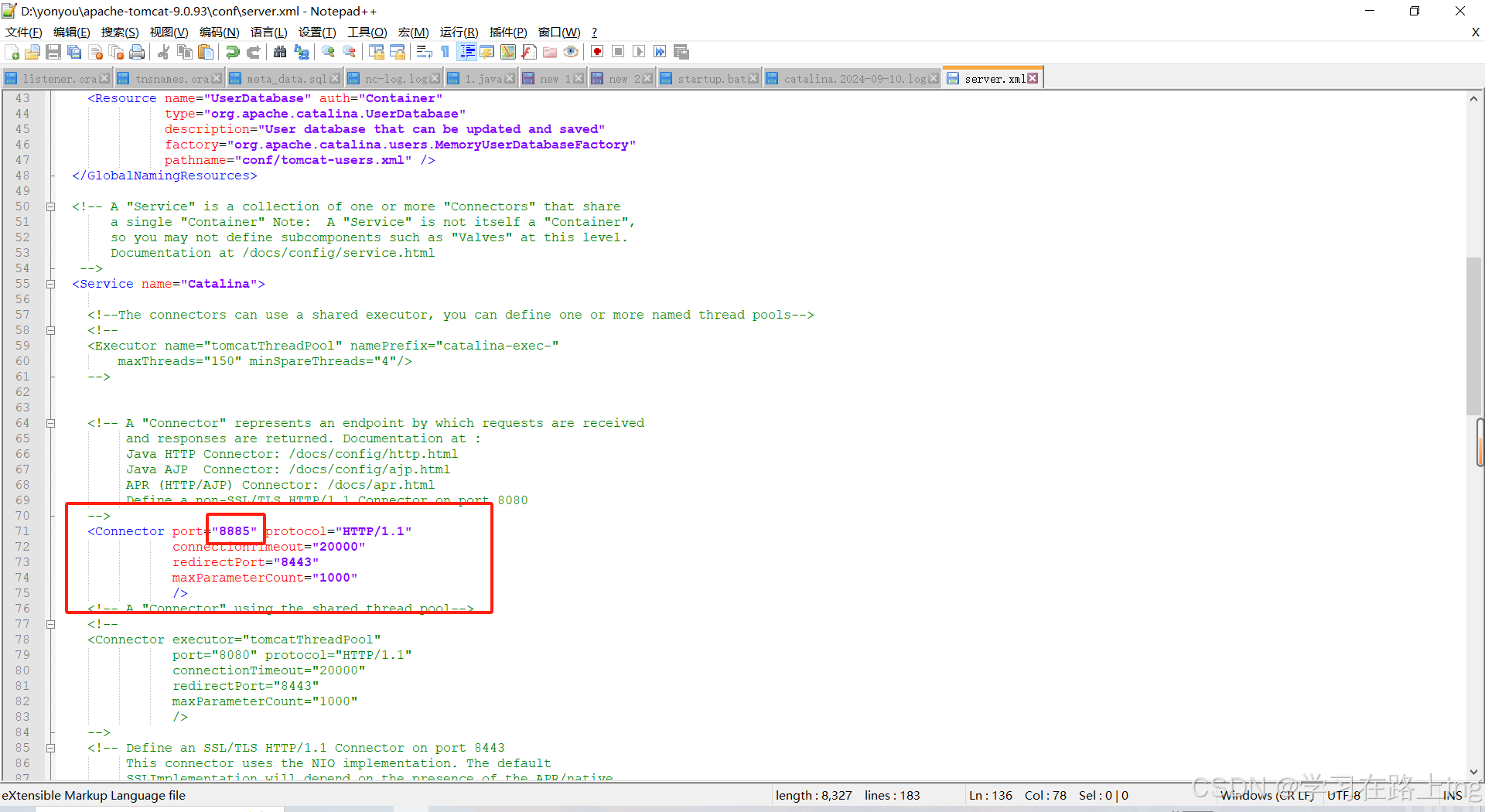
tomcat端口被占用解决方法
在安装目录的conf下修改server.xml文件,修改后保存重启即可...
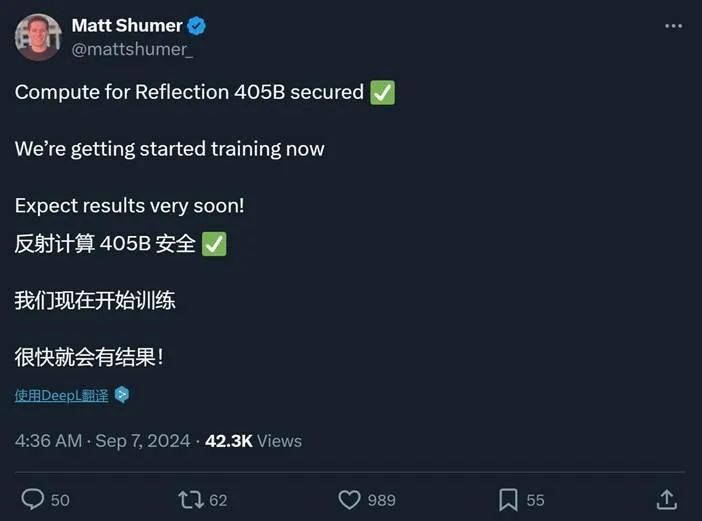
全新的训练算法:Reflection 70B进入大众的视野
在2024年9月6日,大模型的圈子迎来了一位新成员——Reflection 70B,它横扫了MMLU、MATH、IFEval、GSM8K等知名的模型基准测试,完美超越了GPT-4o,同时也超越了Claude3.5 Sonnet成为了新的大模型之王,Reflection 70B到底是…...
静态标注rtk文件参数解析
目录 在静态标注中,rtk(Real-Time Kinematic)文件的主要作用 rtk文件包含几种类型数据 具体作用 具体示例 %RAWIMUSA #INSPVAXA $GPRMC 背景: 最近工作中涉及到静态标注 slam相关,因为初入门,对于rtk文件中有很多参数&…...
TensorFlow和PyTorch小知识
TensorFlow和PyTorch是当前最流行的两个开源机器学习库,它们都广泛用于研究和工业界的深度学习项目。下面是对它们的介绍: 1,TensorFlow - **开发背景:** TensorFlow最初由Google Brain Team开发,并于2015年11月开源…...
Java证书信息收集
1.Java二级 【NCRE 二级Java语言程序设计02】考试流程及二级Java大纲_java语言程序设计计算机二级-CSDN博客...
flink写入hudi MOR表
第一步:创建flink内存表从kafka读取数据: DROP TABLE IF EXISTS HUDI_KAFKA_DEBEZIUM_ZHANG; CREATE TABLE IF NOT EXISTS HUDI_KAFKA_DEBEZIUM_ZHANG( ID STRING comment 编码 ,NAME STRING comment 名称 ,PRIMARY KEY(RCLNT,RLDNR,RRCTY,RVERS,RYEAR,…...
智能工厂程序设计 之-2 (Substrate) :三个世界--“存在的意义”-“‘我’的价值的实现” 之2
Q13、我刚看了一下前门前面的讨论。有一段文字您的重新 理解一下。那就是: 对题目 的另一角度( “智能工厂的程序设计”的三个层次词 分别关注的问题 及其 解决 思路的描述)的解释: 三个不同层次(深度)&…...
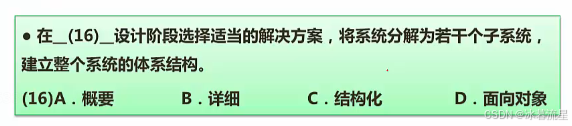
概要设计例题
答案:A 知识点: 概要设计 设计软件系统的总体结构:采用某种方法,将一个复杂的系统按照功能划分成模块;确定每个模块的功能;确定模块之间的调用关系;确定模块之间的接口,即模块之间…...
注册表模式:使用注册表和装饰器函数的模块化设计
在现代软件开发中,模块化设计是提高代码可维护性和可扩展性的关键技术之一。本文将探讨如何使用注册表(Registry)和装饰器函数(Decorator Function)来实现模块化设计,提升代码的灵活性和可扩展性。 什么是…...
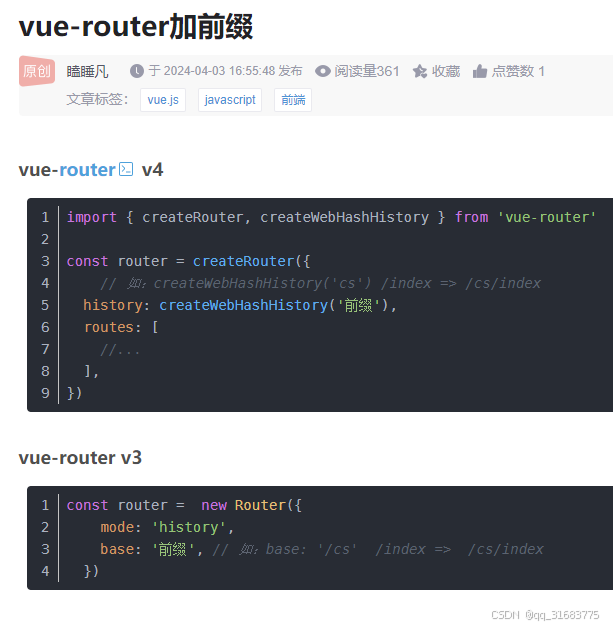
怎样将vue项目 部署在ngixn的子目录下
如果同一服务器的80端口下,需要部署两个或以上数量的vue项目,那么就需要将其中一个vue项目部署在根目录下,其他的项目部署在子目录下. 像这样的配置 访问根目录 / 访问灭火器后台管理,访问 /mall/ 访问商城的后台管理 那么商场的vue项目,这样配置,才能在/mall/下正常访问? 1…...
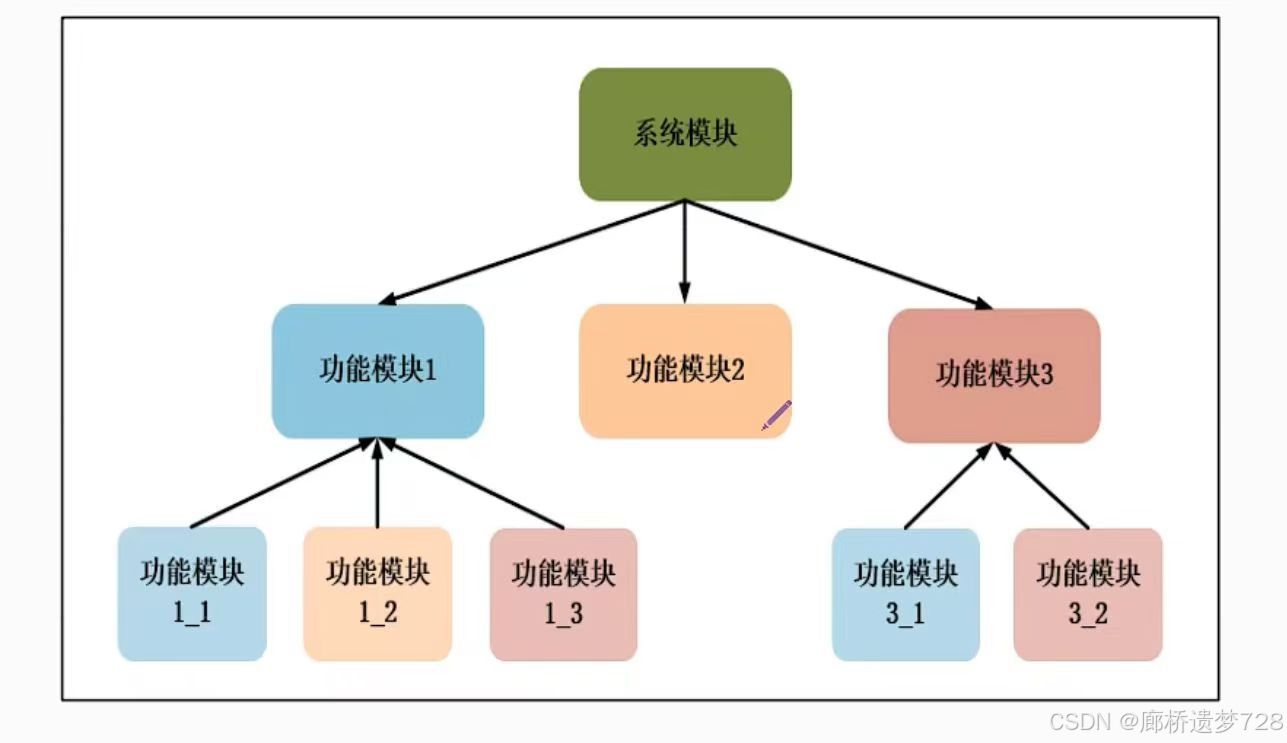
FPGA开发:Verilog数字设计基础
EDA技术 EDA指Electronic Design Automation,翻译为:电子设计自动化,最早发源于美国的影像技术,主要应用于集成电路设计、FPGA应用、IC设计制造、PCB设计上面。 而EDA技术就是指以计算机为工具,设计者在EDA软件平台上…...
【网络】每天掌握一个Linux命令 - iftop
在Linux系统中,iftop是网络管理的得力助手,能实时监控网络流量、连接情况等,帮助排查网络异常。接下来从多方面详细介绍它。 目录 【网络】每天掌握一个Linux命令 - iftop工具概述安装方式核心功能基础用法进阶操作实战案例面试题场景生产场景…...
ubuntu搭建nfs服务centos挂载访问
在Ubuntu上设置NFS服务器 在Ubuntu上,你可以使用apt包管理器来安装NFS服务器。打开终端并运行: sudo apt update sudo apt install nfs-kernel-server创建共享目录 创建一个目录用于共享,例如/shared: sudo mkdir /shared sud…...
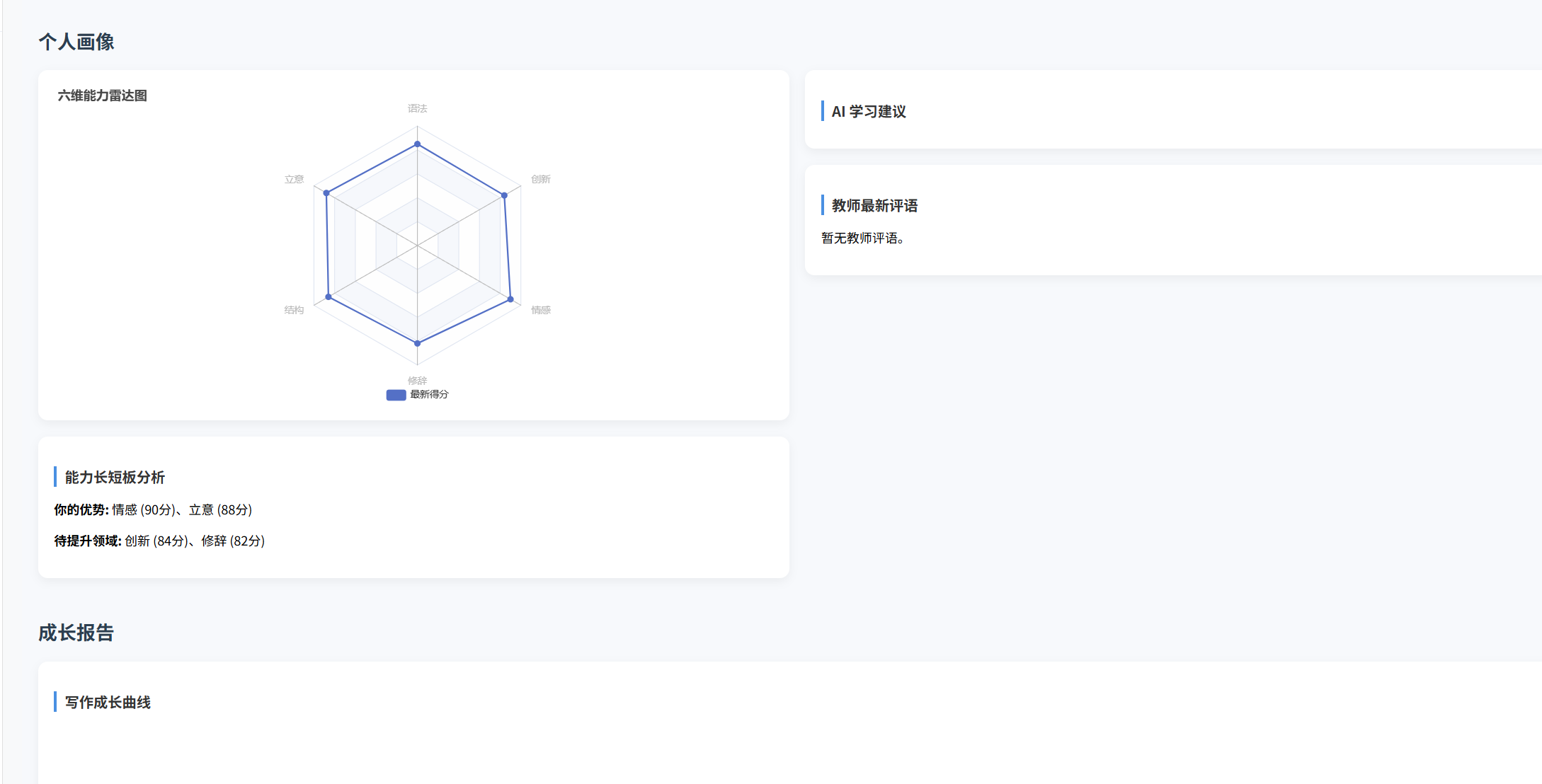
(十)学生端搭建
本次旨在将之前的已完成的部分功能进行拼装到学生端,同时完善学生端的构建。本次工作主要包括: 1.学生端整体界面布局 2.模拟考场与部分个人画像流程的串联 3.整体学生端逻辑 一、学生端 在主界面可以选择自己的用户角色 选择学生则进入学生登录界面…...
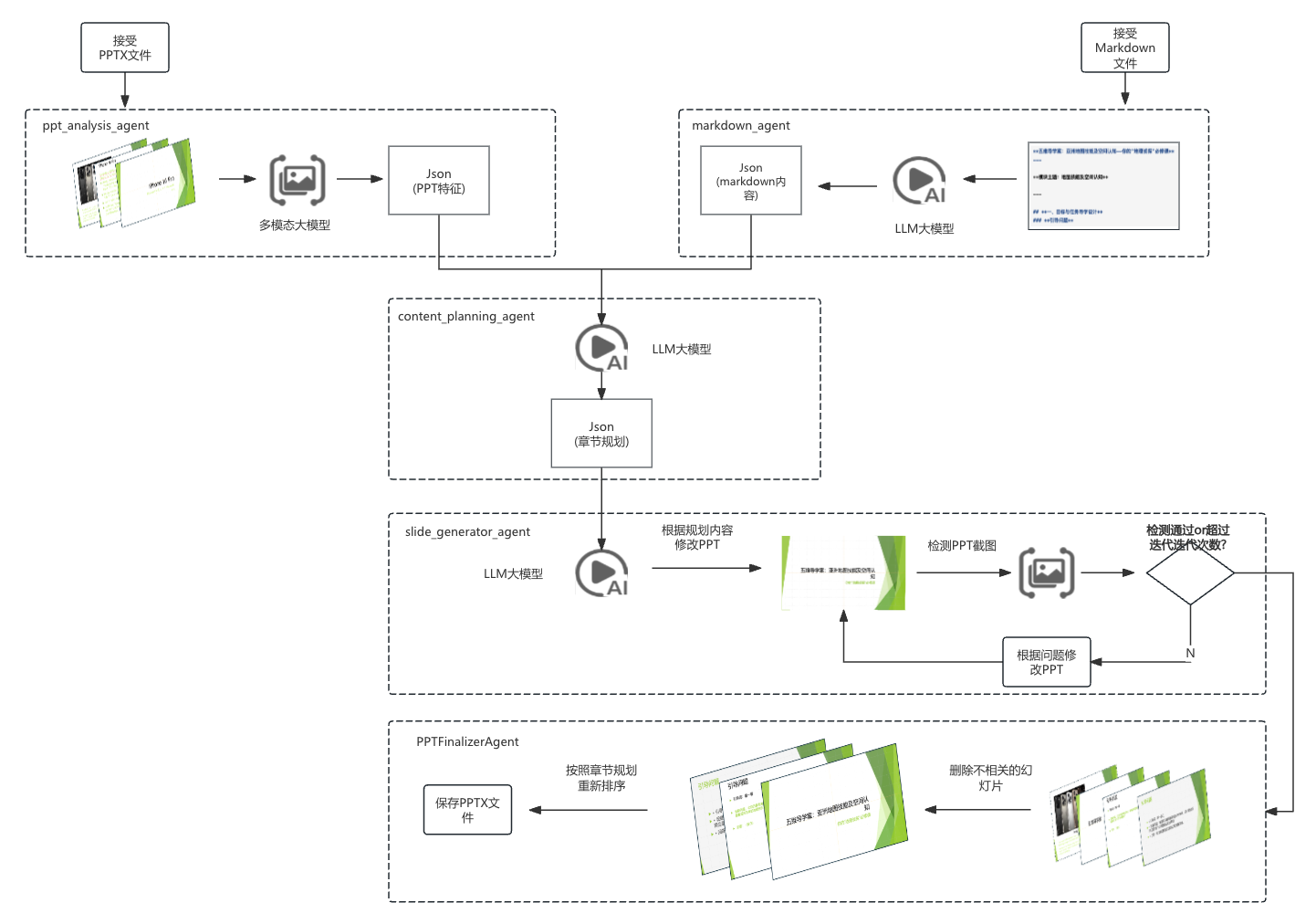
【项目实战】通过多模态+LangGraph实现PPT生成助手
PPT自动生成系统 基于LangGraph的PPT自动生成系统,可以将Markdown文档自动转换为PPT演示文稿。 功能特点 Markdown解析:自动解析Markdown文档结构PPT模板分析:分析PPT模板的布局和风格智能布局决策:匹配内容与合适的PPT布局自动…...
vue3 定时器-定义全局方法 vue+ts
1.创建ts文件 路径:src/utils/timer.ts 完整代码: import { onUnmounted } from vuetype TimerCallback (...args: any[]) > voidexport function useGlobalTimer() {const timers: Map<number, NodeJS.Timeout> new Map()// 创建定时器con…...
大学生职业发展与就业创业指导教学评价
这里是引用 作为软工2203/2204班的学生,我们非常感谢您在《大学生职业发展与就业创业指导》课程中的悉心教导。这门课程对我们即将面临实习和就业的工科学生来说至关重要,而您认真负责的教学态度,让课程的每一部分都充满了实用价值。 尤其让我…...
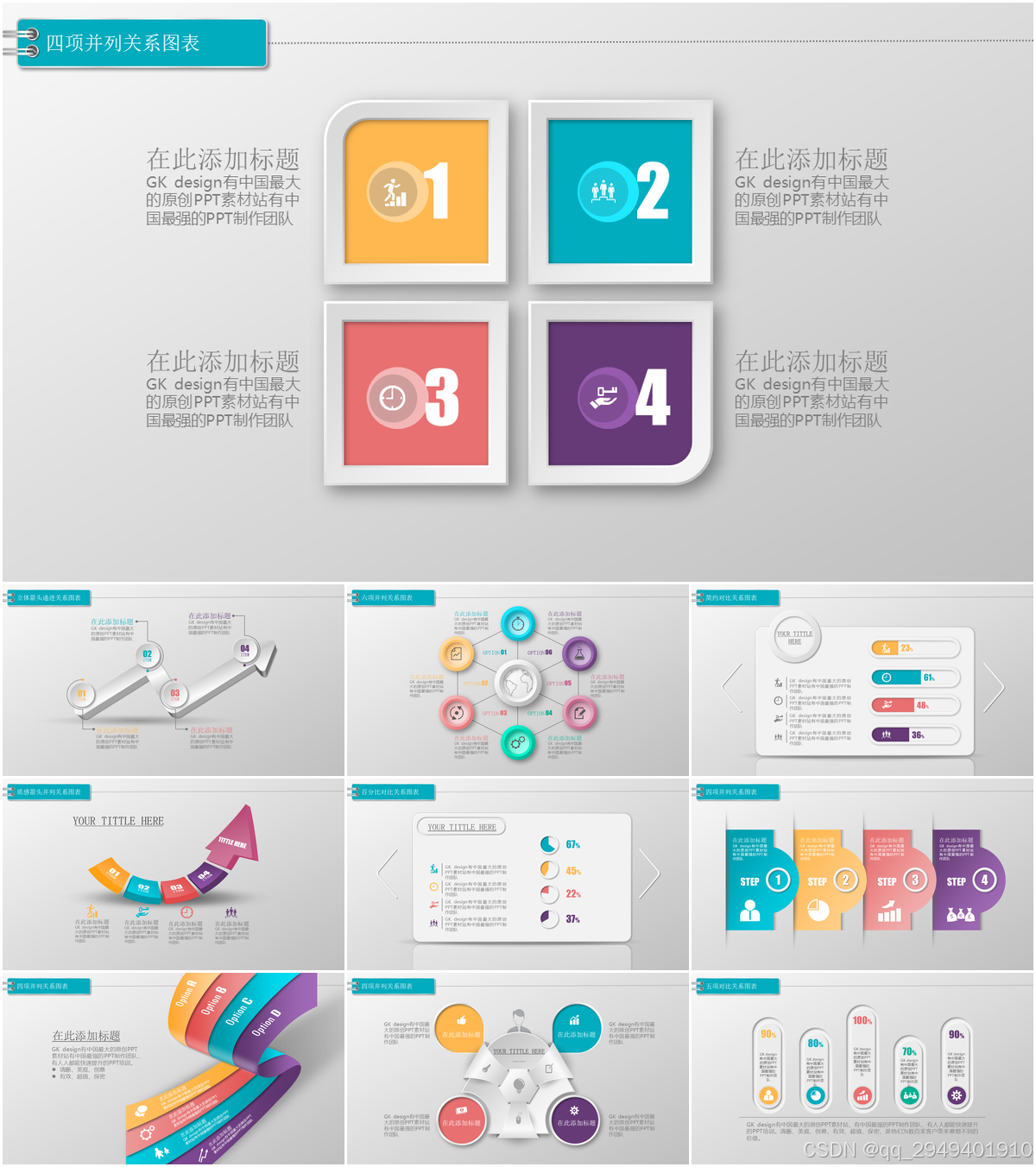
图表类系列各种样式PPT模版分享
图标图表系列PPT模版,柱状图PPT模版,线状图PPT模版,折线图PPT模版,饼状图PPT模版,雷达图PPT模版,树状图PPT模版 图表类系列各种样式PPT模版分享:图表系列PPT模板https://pan.quark.cn/s/20d40aa…...
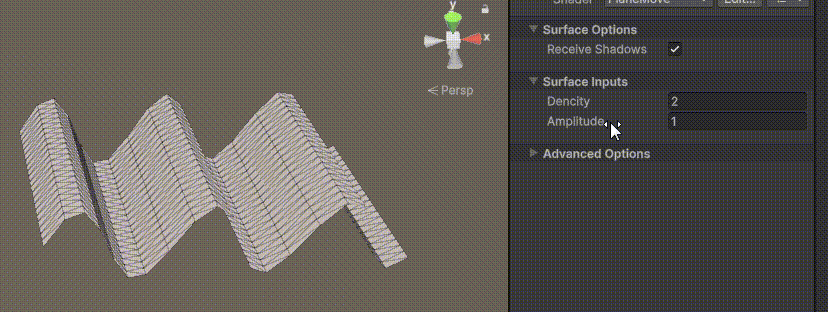
Unity | AmplifyShaderEditor插件基础(第七集:平面波动shader)
目录 一、👋🏻前言 二、😈sinx波动的基本原理 三、😈波动起来 1.sinx节点介绍 2.vertexPosition 3.集成Vector3 a.节点Append b.连起来 4.波动起来 a.波动的原理 b.时间节点 c.sinx的处理 四、🌊波动优化…...
Spring是如何解决Bean的循环依赖:三级缓存机制
1、什么是 Bean 的循环依赖 在 Spring框架中,Bean 的循环依赖是指多个 Bean 之间互相持有对方引用,形成闭环依赖关系的现象。 多个 Bean 的依赖关系构成环形链路,例如: 双向依赖:Bean A 依赖 Bean B,同时 Bean B 也依赖 Bean A(A↔B)。链条循环: Bean A → Bean…...
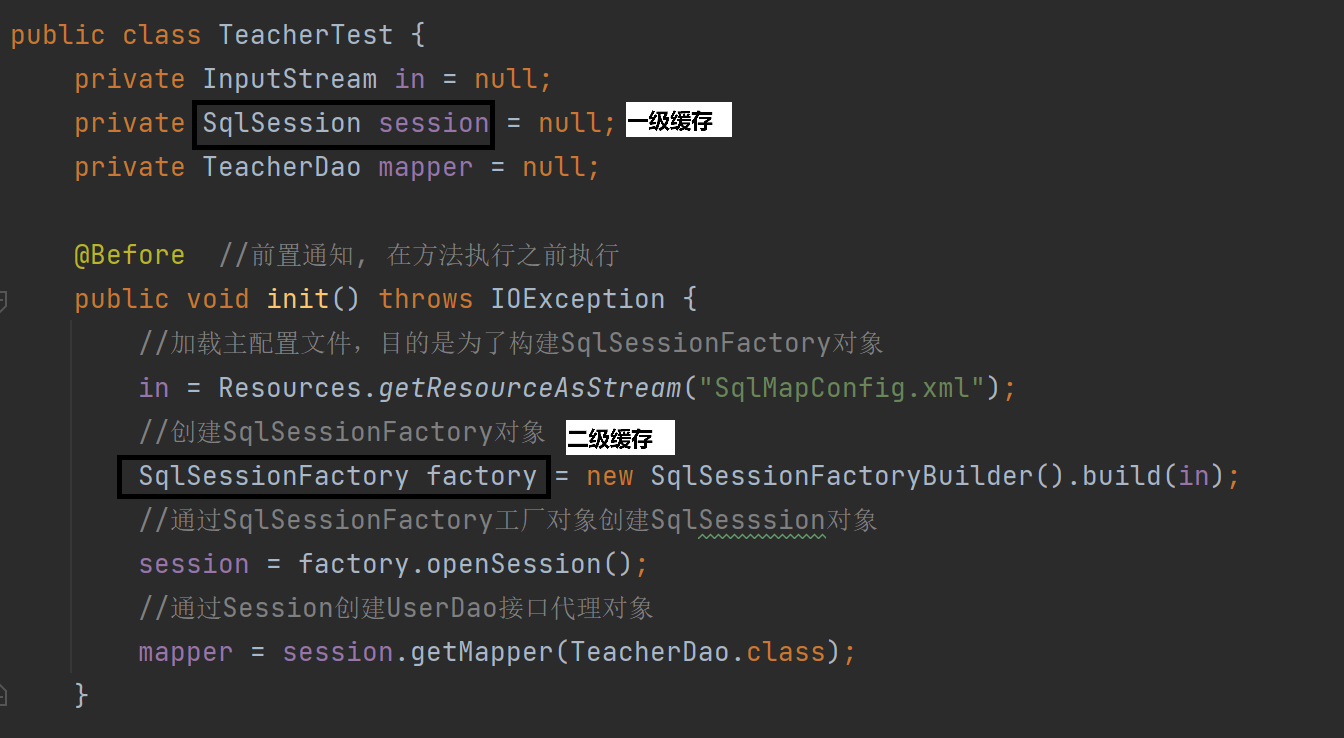
MyBatis中关于缓存的理解
MyBatis缓存 MyBatis系统当中默认定义两级缓存:一级缓存、二级缓存 默认情况下,只有一级缓存开启(sqlSession级别的缓存)二级缓存需要手动开启配置,需要局域namespace级别的缓存 一级缓存(本地缓存&#…...