深入理解Spring框架几个重要扩展接口
本文介绍Spring框架的几个日常开发重要扩展接口,方便日常项目中按需扩展使用。
一、Processor 系列接口
用途: Processor 系列接口包括 BeanPostProcessor 和 BeanFactoryPostProcessor,它们的设计目的是在 Spring 容器启动过程中对 Bean 和 BeanFactory 进行自定义处理,实现一些额外的逻辑。加深理解SpringBean的生命周期理解,以及扩展更多自定义实现。
BeanPostProcessor该接口目前有两个方法:
-
postProcessBeforeInitialization 该在初始化方法之前调用。
-
postProcessAfterInitialization 该方法再初始化方法之后调用。
源码如下:
public interface BeanPostProcessor {/*** Apply this {@code BeanPostProcessor} to the given new bean instance <i>before</i> any bean* initialization callbacks (like InitializingBean's {@code afterPropertiesSet}* or a custom init-method). The bean will already be populated with property values.* The returned bean instance may be a wrapper around the original.* <p>The default implementation returns the given {@code bean} as-is.* @param bean the new bean instance* @param beanName the name of the bean* @return the bean instance to use, either the original or a wrapped one;* if {@code null}, no subsequent BeanPostProcessors will be invoked* @throws org.springframework.beans.BeansException in case of errors* @see org.springframework.beans.factory.InitializingBean#afterPropertiesSet*/@Nullabledefault Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {return bean;}/*** Apply this {@code BeanPostProcessor} to the given new bean instance <i>after</i> any bean* initialization callbacks (like InitializingBean's {@code afterPropertiesSet}* or a custom init-method). The bean will already be populated with property values.* The returned bean instance may be a wrapper around the original.* <p>In case of a FactoryBean, this callback will be invoked for both the FactoryBean* instance and the objects created by the FactoryBean (as of Spring 2.0). The* post-processor can decide whether to apply to either the FactoryBean or created* objects or both through corresponding {@code bean instanceof FactoryBean} checks.* <p>This callback will also be invoked after a short-circuiting triggered by a* {@link InstantiationAwareBeanPostProcessor#postProcessBeforeInstantiation} method,* in contrast to all other {@code BeanPostProcessor} callbacks.* <p>The default implementation returns the given {@code bean} as-is.* @param bean the new bean instance* @param beanName the name of the bean* @return the bean instance to use, either the original or a wrapped one;* if {@code null}, no subsequent BeanPostProcessors will be invoked* @throws org.springframework.beans.BeansException in case of errors* @see org.springframework.beans.factory.InitializingBean#afterPropertiesSet* @see org.springframework.beans.factory.FactoryBean*/@Nullabledefault Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {return bean;}}
public interface InstantiationAwareBeanPostProcessor extends BeanPostProcessor {/*** Apply this BeanPostProcessor <i>before the target bean gets instantiated</i>.* The returned bean object may be a proxy to use instead of the target bean,* effectively suppressing default instantiation of the target bean.* <p>If a non-null object is returned by this method, the bean creation process* will be short-circuited. The only further processing applied is the* {@link #postProcessAfterInitialization} callback from the configured* {@link BeanPostProcessor BeanPostProcessors}.* <p>This callback will be applied to bean definitions with their bean class,* as well as to factory-method definitions in which case the returned bean type* will be passed in here.* <p>Post-processors may implement the extended* {@link SmartInstantiationAwareBeanPostProcessor} interface in order* to predict the type of the bean object that they are going to return here.* <p>The default implementation returns {@code null}.* @param beanClass the class of the bean to be instantiated* @param beanName the name of the bean* @return the bean object to expose instead of a default instance of the target bean,* or {@code null} to proceed with default instantiation* @throws org.springframework.beans.BeansException in case of errors* @see #postProcessAfterInstantiation* @see org.springframework.beans.factory.support.AbstractBeanDefinition#getBeanClass()* @see org.springframework.beans.factory.support.AbstractBeanDefinition#getFactoryMethodName()*/@Nullabledefault Object postProcessBeforeInstantiation(Class<?> beanClass, String beanName) throws BeansException {return null;}/*** Perform operations after the bean has been instantiated, via a constructor or factory method,* but before Spring property population (from explicit properties or autowiring) occurs.* <p>This is the ideal callback for performing custom field injection on the given bean* instance, right before Spring's autowiring kicks in.* <p>The default implementation returns {@code true}.* @param bean the bean instance created, with properties not having been set yet* @param beanName the name of the bean* @return {@code true} if properties should be set on the bean; {@code false}* if property population should be skipped. Normal implementations should return {@code true}.* Returning {@code false} will also prevent any subsequent InstantiationAwareBeanPostProcessor* instances being invoked on this bean instance.* @throws org.springframework.beans.BeansException in case of errors* @see #postProcessBeforeInstantiation*/default boolean postProcessAfterInstantiation(Object bean, String beanName) throws BeansException {return true;}/*** Post-process the given property values before the factory applies them* to the given bean, without any need for property descriptors.* <p>Implementations should return {@code null} (the default) if they provide a custom* {@link #postProcessPropertyValues} implementation, and {@code pvs} otherwise.* In a future version of this interface (with {@link #postProcessPropertyValues} removed),* the default implementation will return the given {@code pvs} as-is directly.* @param pvs the property values that the factory is about to apply (never {@code null})* @param bean the bean instance created, but whose properties have not yet been set* @param beanName the name of the bean* @return the actual property values to apply to the given bean (can be the passed-in* PropertyValues instance), or {@code null} which proceeds with the existing properties* but specifically continues with a call to {@link #postProcessPropertyValues}* (requiring initialized {@code PropertyDescriptor}s for the current bean class)* @throws org.springframework.beans.BeansException in case of errors* @since 5.1* @see #postProcessPropertyValues*/@Nullabledefault PropertyValues postProcessProperties(PropertyValues pvs, Object bean, String beanName)throws BeansException {return null;}/*** Post-process the given property values before the factory applies them* to the given bean. Allows for checking whether all dependencies have been* satisfied, for example based on a "Required" annotation on bean property setters.* <p>Also allows for replacing the property values to apply, typically through* creating a new MutablePropertyValues instance based on the original PropertyValues,* adding or removing specific values.* <p>The default implementation returns the given {@code pvs} as-is.* @param pvs the property values that the factory is about to apply (never {@code null})* @param pds the relevant property descriptors for the target bean (with ignored* dependency types - which the factory handles specifically - already filtered out)* @param bean the bean instance created, but whose properties have not yet been set* @param beanName the name of the bean* @return the actual property values to apply to the given bean (can be the passed-in* PropertyValues instance), or {@code null} to skip property population* @throws org.springframework.beans.BeansException in case of errors* @see #postProcessProperties* @see org.springframework.beans.MutablePropertyValues* @deprecated as of 5.1, in favor of {@link #postProcessProperties(PropertyValues, Object, String)}*/@Deprecated@Nullabledefault PropertyValues postProcessPropertyValues(PropertyValues pvs, PropertyDescriptor[] pds, Object bean, String beanName) throws BeansException {return pvs;}}
案例一:获取当前项目启动过程中所有的实现扩展实现类。
ConfigurableApplicationContext context = SpringApplication.run(SkywalkApplication.class, args);
String[] beanNames = context.getBeanNamesForType(BeanPostProcessor.class, true, false);
for(String bean:beanNames){System.out.println("实现后置处理器:"+bean);
}
运行如下:
案例二:监控Bean的初始化信息例如最耗时的Bean。
package com.boot.skywalk.processor;import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.BeanPostProcessor;
import org.springframework.stereotype.Component;import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.stream.Collectors;/*** 通用的Bean的后置处理器统计最耗时的Bean*/
@Component
public class CommonBeanPostProcessor implements BeanPostProcessor {/*** 初始化前的Map,Bean->时间点*/private static final Map<String,Long> BEGIN_INIT_MAP=new ConcurrentHashMap<>();/*** 初始化后的Map,Bean->时间点*/private static final Map<String,Long> INIT_COST_MAP=new ConcurrentHashMap<>();/*** 最耗时的Bean*/private static final int TOP_COST_INIT_BEAN=3;/*** Bean初始化之前* @param bean* @param beanName* @return* @throws BeansException*/@Overridepublic Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {BEGIN_INIT_MAP.putIfAbsent(beanName, System.currentTimeMillis());return bean;}@Overridepublic Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {Long initPoint = BEGIN_INIT_MAP.get(beanName);INIT_COST_MAP.put(beanName, System.currentTimeMillis()-initPoint);return bean;}/*** 流式获取3,List返回Map.collect(Collectors.toMap(p -> p.getKey(), p -> p.getValue())* @return*/public Map<String,Long> getTopCostInitBean(){Map<String, Long> result = INIT_COST_MAP.entrySet()// 降序排列.stream().sorted((a,b)-> (int) (b.getValue()-a.getValue()))// 转换Map或者是List<Object[]>.limit(TOP_COST_INIT_BEAN).collect(Collectors.toMap(p -> p.getKey(), p -> p.getValue()));return result;}
CommonBeanPostProcessor beanPostProcessor= context.getBean(CommonBeanPostProcessor.class);Map<String, Long> topCostInitBean = beanPostProcessor.getTopCostInitBean();topCostInitBean.forEach((key,value)->{System.out.println(key+":"+value+"ms");});
运行截图,可以据此数据来分析项目中哪些Bean可以设置为延迟加载,提高项目启动速度。
BeanFactoryPostProcessor:
Spring 在容器启动时,会检测容器中是否存在实现了 BeanFactoryPostProcessor 接口的 Bean,并在 BeanFactory 实例化之后、Bean 实例化之前调用其相应的方法。通过实现 BeanFactoryPostProcessor 接口,我们可以在容器启动时对 BeanFactory 进行配置,如修改 Bean 的定义、添加 Bean 的属性值等。
@FunctionalInterface
public interface BeanFactoryPostProcessor {/*** Modify the application context's internal bean factory after its standard* initialization. All bean definitions will have been loaded, but no beans* will have been instantiated yet. This allows for overriding or adding* properties even to eager-initializing beans.* @param beanFactory the bean factory used by the application context* @throws org.springframework.beans.BeansException in case of errors*/void postProcessBeanFactory(ConfigurableListableBeanFactory beanFactory) throws BeansException;}
二、Aware 接口
Aware 接口的设计目的是增强 Bean 对容器的感知能力,使 Bean 能够更方便地与容器进行交互,获取容器中的特定资源或实例。
Spring提供了大量以 Aware 命名的接口,如BeanNameAware、BeanFactoryAware、ApplicationContextAware等。
这些接口定义了回调方法,通过这些回调方法,Spring容器可以将容器中的一些资源、状态、环境信息注入到Bean中。
例如:ApplicationContextAware
org.springframework.context.support.ApplicationContextAwareProcessor#invokeAwareInterfaces
首先会判断对象是否属于 Aware接口类型,接着根据不同的Aware接口实现类,调用不同的实现类的逻辑。
private void invokeAwareInterfaces(Object bean) {if (bean instanceof EnvironmentAware) {((EnvironmentAware) bean).setEnvironment(this.applicationContext.getEnvironment());}if (bean instanceof EmbeddedValueResolverAware) {((EmbeddedValueResolverAware) bean).setEmbeddedValueResolver(this.embeddedValueResolver);}if (bean instanceof ResourceLoaderAware) {((ResourceLoaderAware) bean).setResourceLoader(this.applicationContext);}if (bean instanceof ApplicationEventPublisherAware) {((ApplicationEventPublisherAware) bean).setApplicationEventPublisher(this.applicationContext);}if (bean instanceof MessageSourceAware) {((MessageSourceAware) bean).setMessageSource(this.applicationContext);}if (bean instanceof ApplicationStartupAware) {((ApplicationStartupAware) bean).setApplicationStartup(this.applicationContext.getApplicationStartup());}if (bean instanceof ApplicationContextAware) {((ApplicationContextAware) bean).setApplicationContext(this.applicationContext);}}
案例一:通过Aware接口获取ApplicationContext和BeanFactory访问容器中其他Bean。
先看相关接口源码,比较简单。
public interface ApplicationContextAware extends Aware {/*** Set the ApplicationContext that this object runs in.* Normally this call will be used to initialize the object.* <p>Invoked after population of normal bean properties but before an init callback such* as {@link org.springframework.beans.factory.InitializingBean#afterPropertiesSet()}* or a custom init-method. Invoked after {@link ResourceLoaderAware#setResourceLoader},* {@link ApplicationEventPublisherAware#setApplicationEventPublisher} and* {@link MessageSourceAware}, if applicable.* @param applicationContext the ApplicationContext object to be used by this object* @throws ApplicationContextException in case of context initialization errors* @throws BeansException if thrown by application context methods* @see org.springframework.beans.factory.BeanInitializationException*/void setApplicationContext(ApplicationContext applicationContext) throws BeansException;}
public interface BeanFactoryAware extends Aware {/*** Callback that supplies the owning factory to a bean instance.* <p>Invoked after the population of normal bean properties* but before an initialization callback such as* {@link InitializingBean#afterPropertiesSet()} or a custom init-method.* @param beanFactory owning BeanFactory (never {@code null}).* The bean can immediately call methods on the factory.* @throws BeansException in case of initialization errors* @see BeanInitializationException*/void setBeanFactory(BeanFactory beanFactory) throws BeansException;}
@Service
public class HomeApplicationContextAwareService implements ApplicationContextAware {private ApplicationContext applicationContext;@Overridepublic void setApplicationContext(ApplicationContext applicationContext) throws BeansException {this.applicationContext=applicationContext;}public void getBean(){Home home = (Home)applicationContext.getBean("home");home.test();}
}
@Service
public class HomeBeanFactoryAwareService implements BeanFactoryAware {private BeanFactory beanFactory;@Overridepublic void setBeanFactory(BeanFactory beanFactory) throws BeansException {this.beanFactory=beanFactory;}public void getBean(){Home bean = (Home) beanFactory.getBean("home");bean.test();}
}
三、ImportSelector 接口
用途: ImportSelector 接口的设计目的是允许在配置类中根据条件动态选择需要导入的其他配置类,以实现模块化和条件化配置。
常见应用场景:
- 根据不同的环境条件选择性地导入不同的配置类。
- 实现特定模块的自动配置功能,根据用户的配置情况动态加载相应的配置类。
源码如下:
public interface ImportSelector {/*** Select and return the names of which class(es) should be imported based on* the {@link AnnotationMetadata} of the importing @{@link Configuration} class.* @return the class names, or an empty array if none*/String[] selectImports(AnnotationMetadata importingClassMetadata);/*** Return a predicate for excluding classes from the import candidates, to be* transitively applied to all classes found through this selector's imports.* <p>If this predicate returns {@code true} for a given fully-qualified* class name, said class will not be considered as an imported configuration* class, bypassing class file loading as well as metadata introspection.* @return the filter predicate for fully-qualified candidate class names* of transitively imported configuration classes, or {@code null} if none* @since 5.2.4*/@Nullabledefault Predicate<String> getExclusionFilter() {return null;}}
案例一:注入指定的Bean
public class ConfigurationImportSelector implements ImportSelector {@Overridepublic String[] selectImports(AnnotationMetadata importingClassMetadata) {return new String[]{EmailService.class.getName(),MessageService.class.getName(),PhoneService.class.getName()};}
}
@Import(ConfigurationImportSelector.class)
@Configuration
public class ServiceConfiguration {
}
public interface ConfigurationService {void doService();
}
public class EmailService implements ConfigurationService{@Overridepublic void doService() {System.out.println("Email Service");}
}
public class MessageService implements ConfigurationService{@Overridepublic void doService() {System.out.println("Message Service");}
}
public class EmailService implements ConfigurationService{@Overridepublic void doService() {System.out.println("Email Service");}
}
案例二:SpringBoot的底层Import注解实现的自动配置扫描实现
跟进入。
点进去,这些自动配置的类都要注入到Spring容器中。
相关文章:
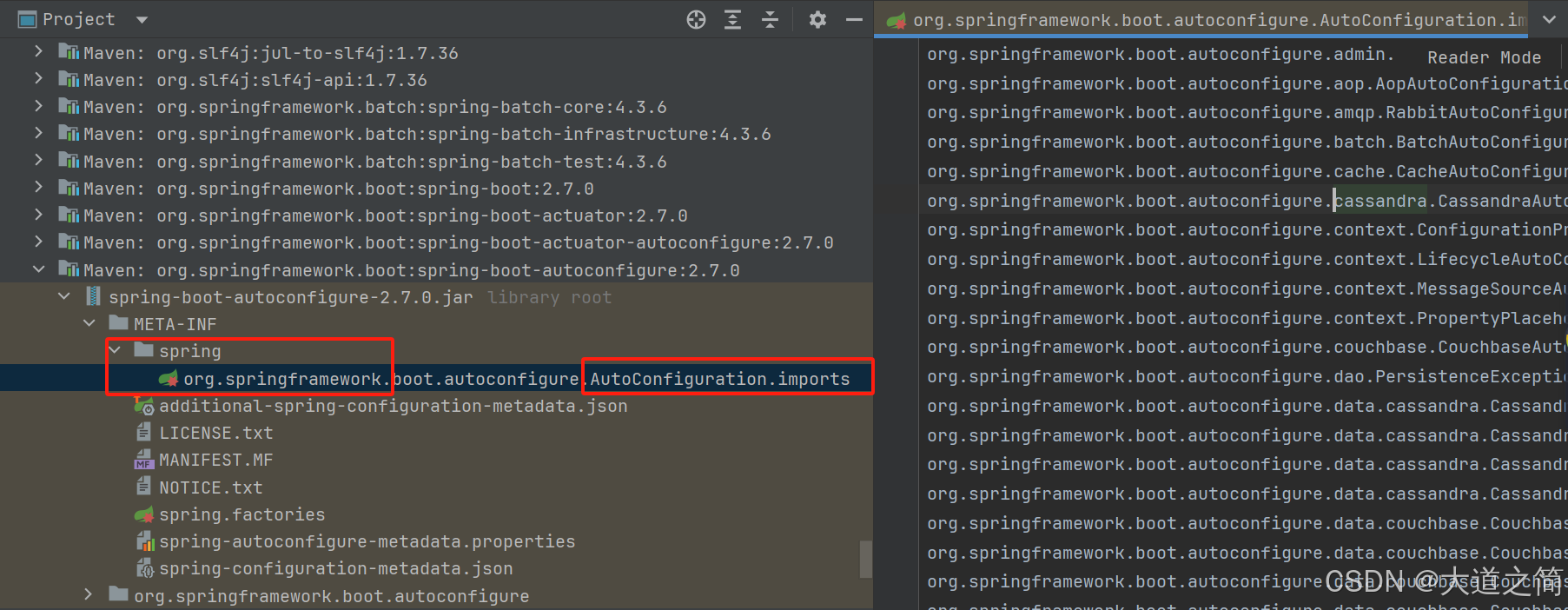
深入理解Spring框架几个重要扩展接口
本文介绍Spring框架的几个日常开发重要扩展接口,方便日常项目中按需扩展使用。 一、Processor 系列接口 用途: Processor 系列接口包括 BeanPostProcessor 和 BeanFactoryPostProcessor,它们的设计目的是在 Spring 容器启动过程中对 Bean 和…...

使用dotnet-counters和dotnet-dump 分析.NET Core 项目内存占用问题
在.NET Core 项目部署后,我们往往会遇到内存占用越来越高的问题,但是由于项目部署在Linux上,因此无法使用VS的远程调试工具来排查内存占用问题。那么这篇文章我们大家一起来学习一下如何排查内存占用问题。 首先,我们来看一下应用…...
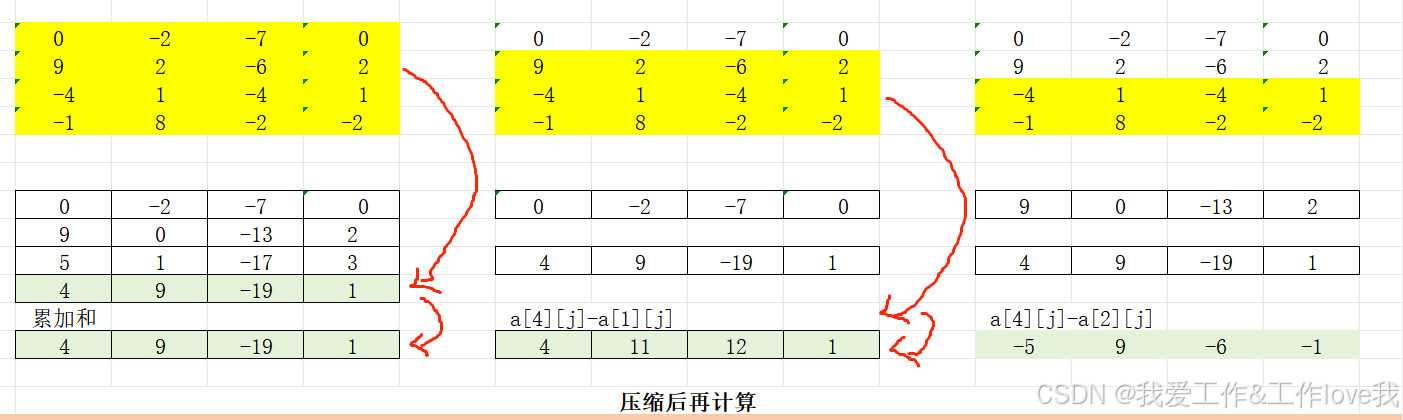
1282:最大子矩阵
题目: 已知矩阵的大小定义为矩阵中所有元素的和。给定一个矩阵,你的任务是找到最大的非空(大小至少是1 1)子矩阵。 比如,如下4 4的矩阵 0 -2 -7 0 9 2 -6 2 -4 1 -4 1 -1 8 0 -2 的最大子矩阵是 9 2 -4 1 -1 8 这个子矩阵的大小是15。 …...
C++编程语言:抽象机制:特殊运算符(Bjarne Stroustrup)
第19章 特殊运算符(Special Operators) 目录 19.1 引言 19.2 特殊运算符(Special Operators) 19.2.1 下标运算符(Subscripting) 19.2.2 函数调用运算符(Function Call) 19.2.3 解引用(Dereferencing) 19.2.4 递增和递减(Increment and Decrement) 19…...
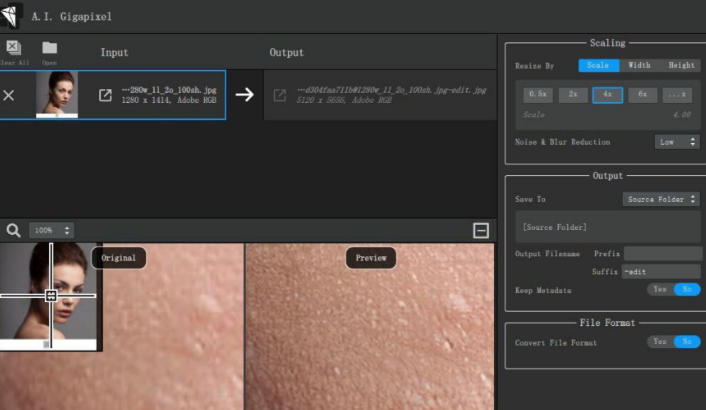
图片无损放大工具Topaz Gigapixel AI v7.4.4 绿色版
Topaz A.I. Gigapixel是这款功能齐全的图象无损变大运用,应用可将智能机拍摄的图象也可以有着专业相机的高质量大尺寸作用。你可以完美地放大你的小照片并大规模打印,它根本不会粘贴。它具有清晰的效果和完美的品质。 借助AIGigapixel,您可以…...
Vue中计算属性computed—(详解计算属性vs方法Methods,包括案例+代码)
文章目录 计算属性computed3.1 概述3.2 使用3.3 计算属性vs方法Methods3.4 计算属性的完整写法 计算属性computed 3.1 概述 基于现有的数据,计算出来的新属性。 依赖的数据变化,自动重新计算 语法: 声明在 computed 配置项中,…...
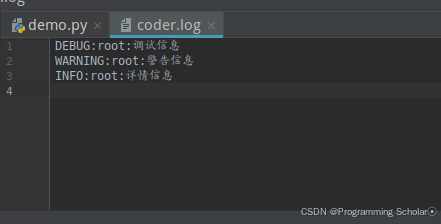
Python程序设计 内置函数 日志模块
logging(日志) 日志记录是程序员工具箱中非常有用的工具。它可以帮助您更好地理解程序的流程,并发现您在开发过程中可能没有想到的场景。 日志为开发人员提供了额外的一组眼睛,这些眼睛不断关注应用程序正在经历的流程。它们可以存储信息,例…...

中标麒麟v5安装qt512.12开发软件
注意 需要联网操作 遇到问题1:yum提示没有可用软件包问题 终端执行如下命令 CentOS7将yum源更换为国内源保姆级教程 中标麒麟V7-yum源的更换(阿里云源) wget -O /etc/yum.repos.d/CentOS-Base.repo http://mirrors.aliyun.com/repo/Cento…...
每日算法一练:剑指offer——数组篇(3)
1.报数 实现一个十进制数字报数程序,请按照数字从小到大的顺序返回一个整数数列,该数列从数字 1 开始,到最大的正整数 cnt 位数字结束。 示例 1: 输入:cnt 2 输出:[1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,1…...
Java代码说明设计模式
以下是使用 Java 代码分别说明设计模式中的工厂模式、抽象工厂模式(这里推测你可能想说的是抽象工厂模式而非虚拟工厂模式)、建造者模式和观察者模式。 一、工厂模式 工厂模式是一种创建对象的设计模式,它提供了一种创建对象的方式…...
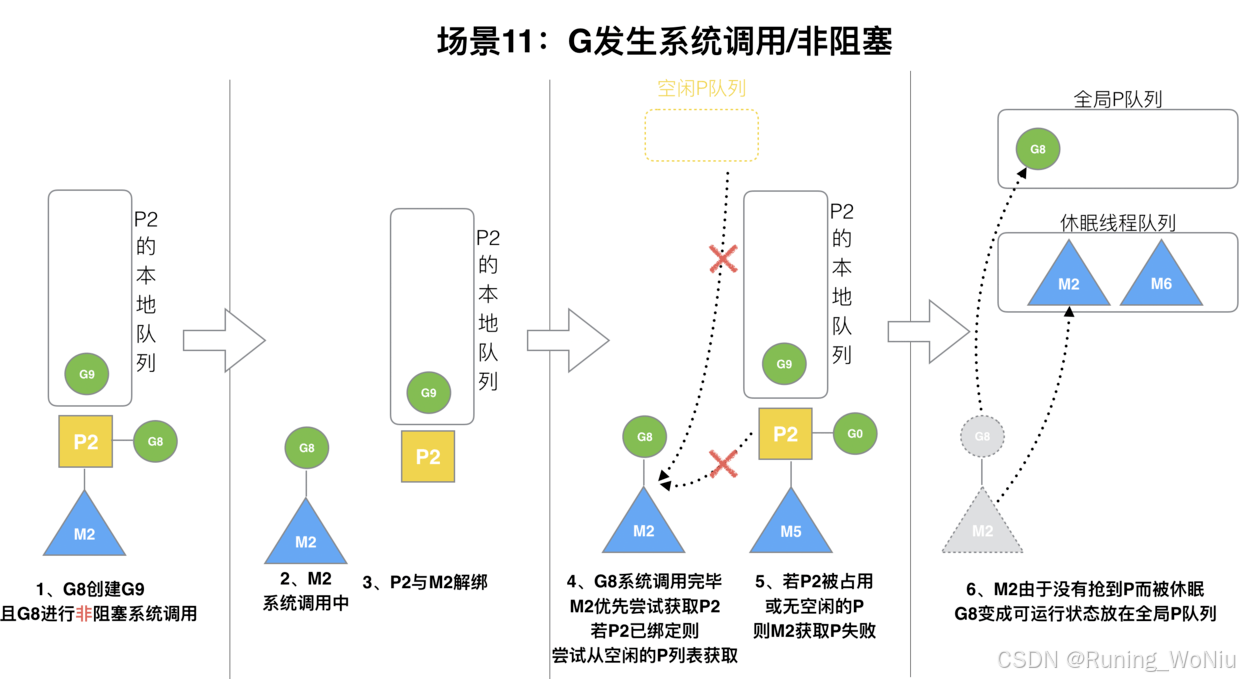
Golang笔记_day06
一、GMP 调度器 1、调度器理解思路 理解golang的调度器要从进程到协程演进来说明: 进程--->线程--->协程---> golang的协程(goroutine) 从上图可以看出,进程到多线程到协程,最终目的就是为了提高CPU的利用率…...
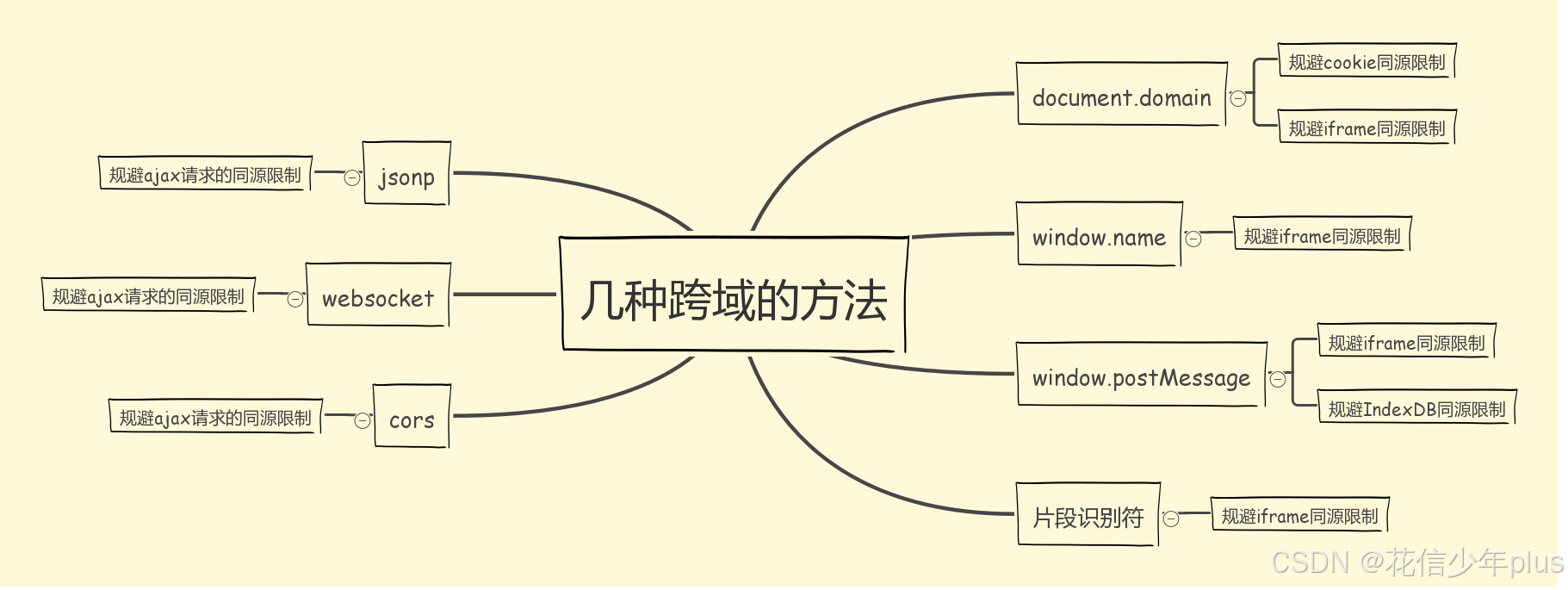
「从零开始的 Vue 3 系列」:第十一章——跨域问题解决方案全解析
前言 本系列将从零开始,系统性地介绍 Vue 3 的常用 API,逐步深入每个核心概念与功能模块。通过详尽的讲解与实战演示,帮助大家掌握 Vue 3 的基础与进阶知识,最终具备独立搭建完整 Vue 3 项目的能力。 第十一章:跨域问…...
C语言结构体数组 java静动数组及问题
1. (1)先声明,后定义:如上一天 //(2).声明时直接定义 #define N 5 typedef struct student { int num; int score; }STU; int main(void) { STU class3[N] { {10,90},{14,70},{8,95} }; …...
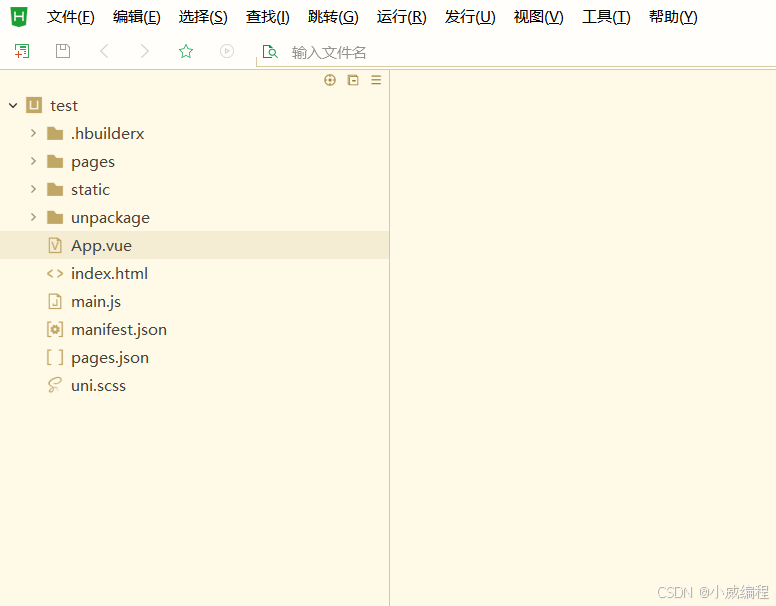
uniapp项目结构基本了解
基本结构的解释 App.vue:应用的根组件,定义全局布局和逻辑。pages/:存放各个页面的 .vue 文件,定义应用的具体页面和功能模块。main.js:应用入口文件,初始化应用,挂载 App.vue。manifest.json&…...
常见Web知识1
List item 常见Web知识1 JSON: JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,易于人类阅读和编写,同时也易于机器解析和生成。它通常用于客户端和服务器之间的数据传输。 JSON 结构 JSON 主要由两…...
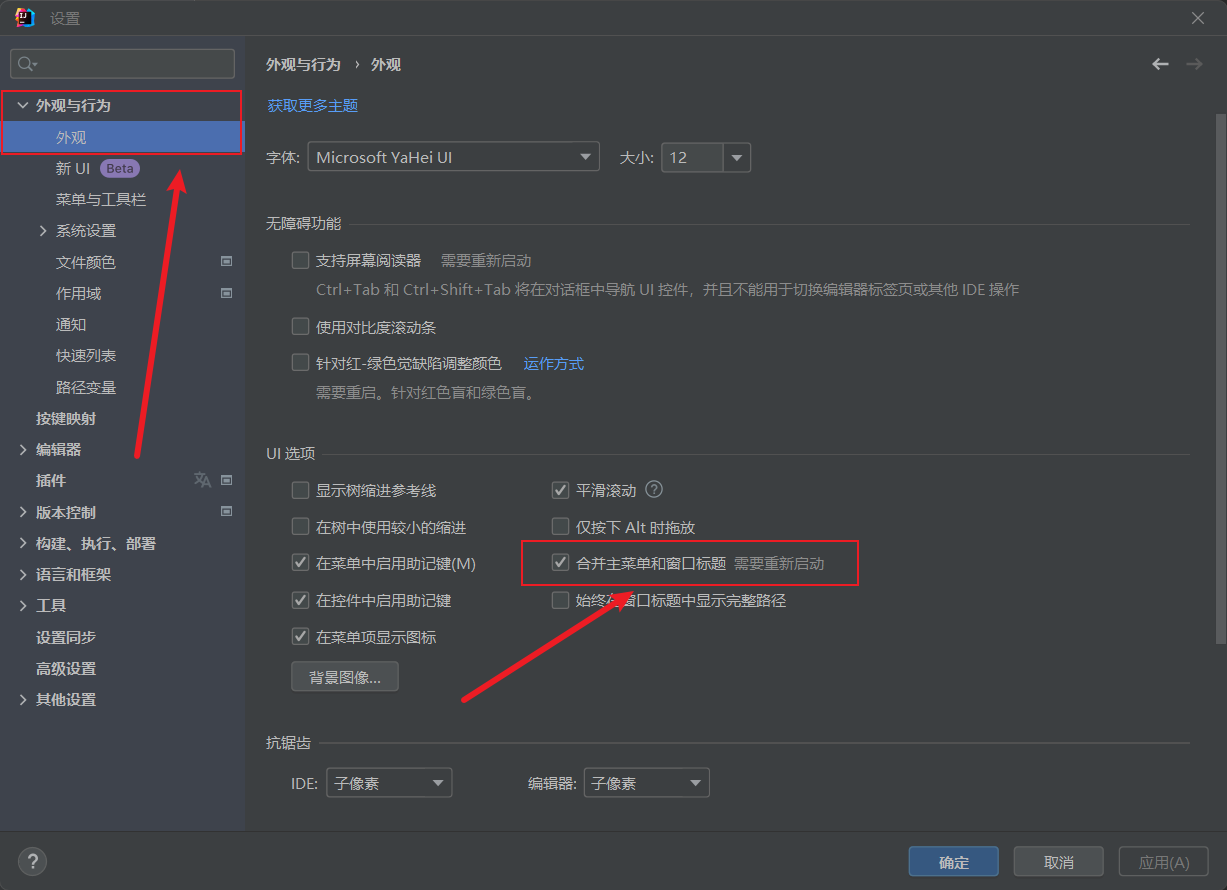
新版idea菜单栏展开与合并
新版idea把菜单栏合并了看着很是不习惯,找了半天原来在这里展开 ① 点击文件 -> 设置 ② 点击外观与行为 -> 外观 -> 合并主菜单和窗口标题 然后确定,重启即可...
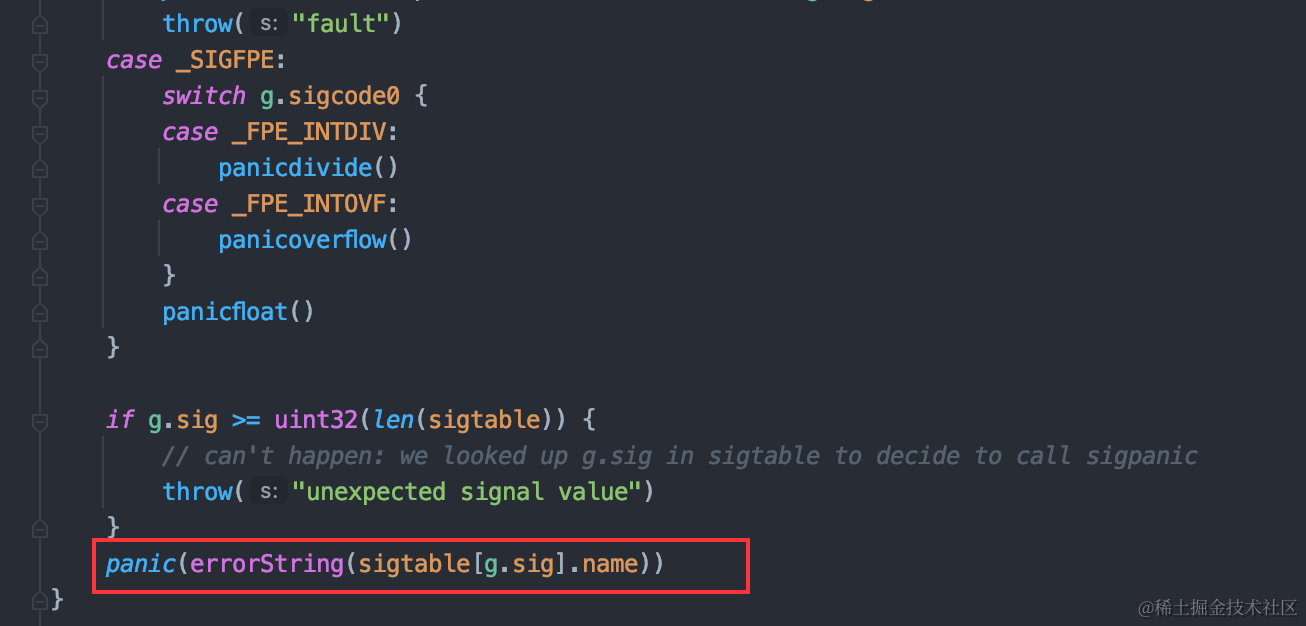
聊聊Go语言的异常处理机制
背景 最近因为遇到了一个panic问题,加上之前零零散散看了些关于程序异常处理相关的东西,对这块有点兴趣,于是整理了一下golang对于异常处理的机制。 名词介绍 Painc golang的内置方法,能够改变程序的控制流。 当函数调用了pan…...
复习:如何理解 React 中的 fiber
React 中的 Fiber 可以理解为 React 16 引入的一种新的协调(reconciliation)引擎,旨在提高 React 应用的性能和响应性。以下是对 React Fiber 的详细解释: 一、Fiber 的定义与背景 Fiber 是对 React 核心算法的一次重新实现,它将渲染工作分解成一系列小的任务单元,这些任…...

10分钟了解腾讯云混元大模型AIGC系列产品
前言 其实说到AIGC,作为开发者,大家其实已经见怪不怪了,那么AIGC是什么,这里我再简单科普一下。 AIGC的全称是Artificial Intelligence Generated Content (人工智能生成内容)或者说叫生成式人工智能&…...
Unity发送Http
本篇实现在Unity中发送Http请求。 讲解Get,Post,用于在Unity中进行数据对接。 一、Get IEnumerator Get() {string url "";//链接UnityWebRequest request UnityWebRequest.Get(url);//创建UnityWebRequest实例并设置请求方式为Getyield …...
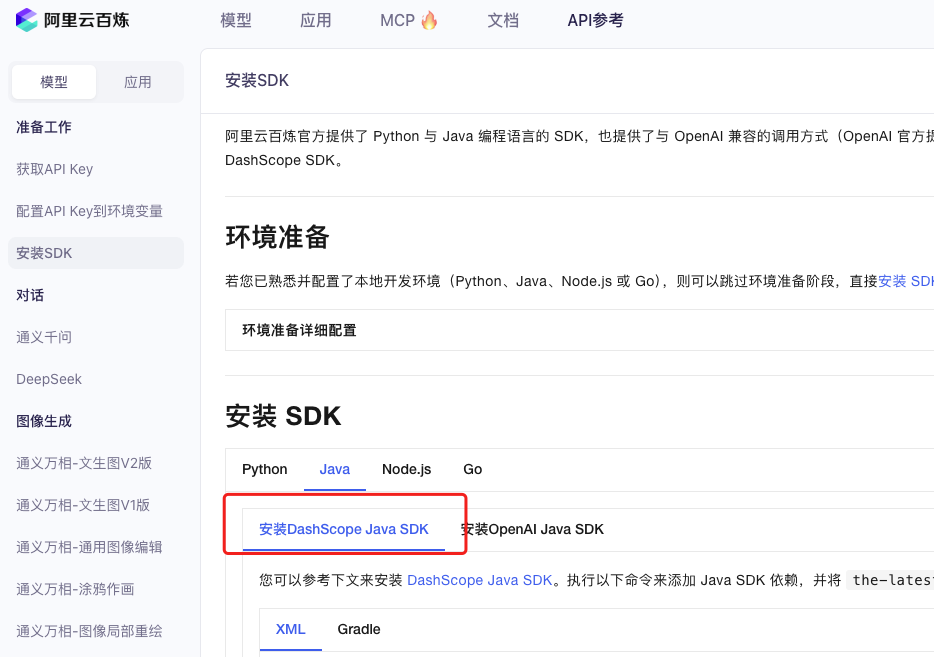
业务系统对接大模型的基础方案:架构设计与关键步骤
业务系统对接大模型:架构设计与关键步骤 在当今数字化转型的浪潮中,大语言模型(LLM)已成为企业提升业务效率和创新能力的关键技术之一。将大模型集成到业务系统中,不仅可以优化用户体验,还能为业务决策提供…...
R语言AI模型部署方案:精准离线运行详解
R语言AI模型部署方案:精准离线运行详解 一、项目概述 本文将构建一个完整的R语言AI部署解决方案,实现鸢尾花分类模型的训练、保存、离线部署和预测功能。核心特点: 100%离线运行能力自包含环境依赖生产级错误处理跨平台兼容性模型版本管理# 文件结构说明 Iris_AI_Deployme…...
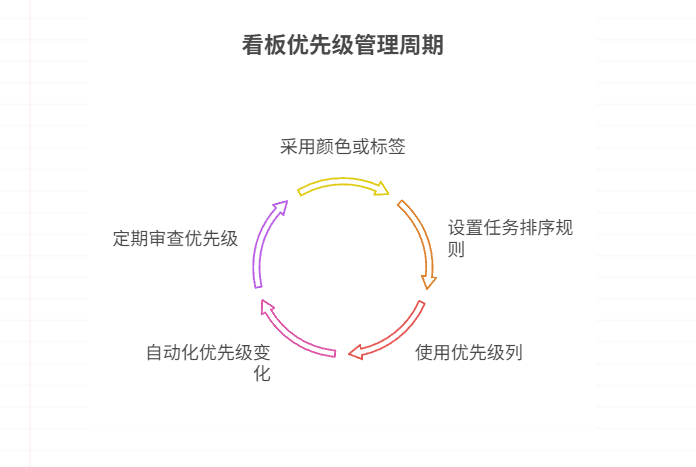
如何在看板中体现优先级变化
在看板中有效体现优先级变化的关键措施包括:采用颜色或标签标识优先级、设置任务排序规则、使用独立的优先级列或泳道、结合自动化规则同步优先级变化、建立定期的优先级审查流程。其中,设置任务排序规则尤其重要,因为它让看板视觉上直观地体…...
基于服务器使用 apt 安装、配置 Nginx
🧾 一、查看可安装的 Nginx 版本 首先,你可以运行以下命令查看可用版本: apt-cache madison nginx-core输出示例: nginx-core | 1.18.0-6ubuntu14.6 | http://archive.ubuntu.com/ubuntu focal-updates/main amd64 Packages ng…...
Swagger和OpenApi的前世今生
Swagger与OpenAPI的关系演进是API标准化进程中的重要篇章,二者共同塑造了现代RESTful API的开发范式。 本期就扒一扒其技术演进的关键节点与核心逻辑: 🔄 一、起源与初创期:Swagger的诞生(2010-2014) 核心…...
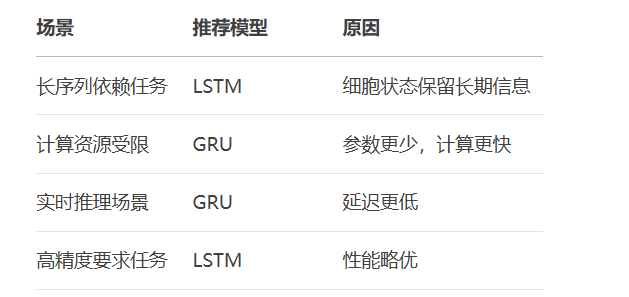
RNN避坑指南:从数学推导到LSTM/GRU工业级部署实战流程
本文较长,建议点赞收藏,以免遗失。更多AI大模型应用开发学习视频及资料,尽在聚客AI学院。 本文全面剖析RNN核心原理,深入讲解梯度消失/爆炸问题,并通过LSTM/GRU结构实现解决方案,提供时间序列预测和文本生成…...
React---day11
14.4 react-redux第三方库 提供connect、thunk之类的函数 以获取一个banner数据为例子 store: 我们在使用异步的时候理应是要使用中间件的,但是configureStore 已经自动集成了 redux-thunk,注意action里面要返回函数 import { configureS…...
蓝桥杯 冶炼金属
原题目链接 🔧 冶炼金属转换率推测题解 📜 原题描述 小蓝有一个神奇的炉子用于将普通金属 O O O 冶炼成为一种特殊金属 X X X。这个炉子有一个属性叫转换率 V V V,是一个正整数,表示每 V V V 个普通金属 O O O 可以冶炼出 …...
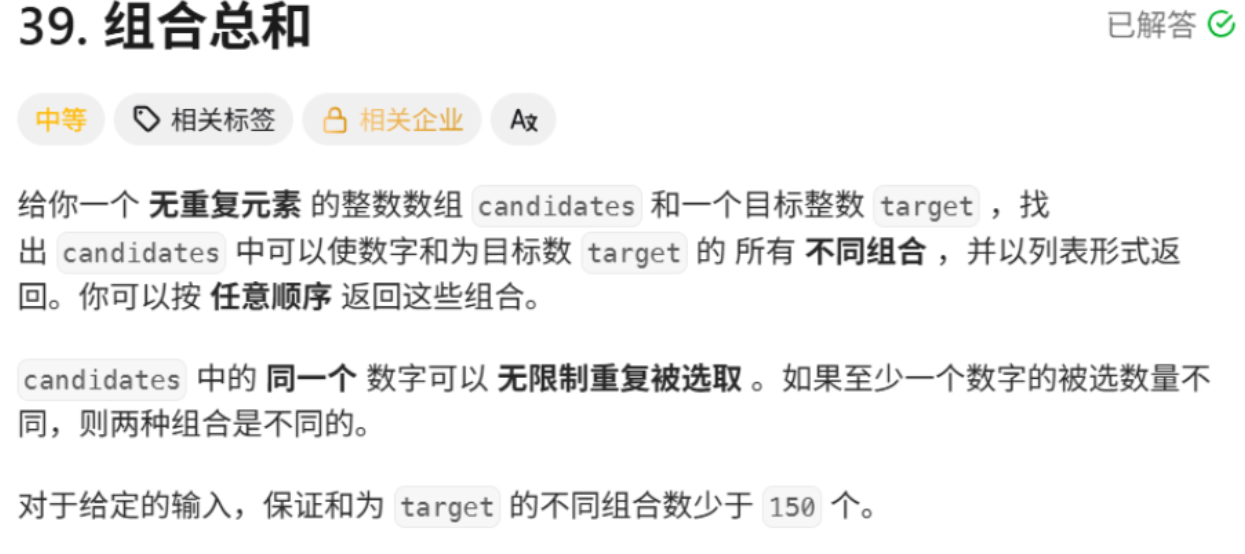
回溯算法学习
一、电话号码的字母组合 import java.util.ArrayList; import java.util.List;import javax.management.loading.PrivateClassLoader;public class letterCombinations {private static final String[] KEYPAD {"", //0"", //1"abc", //2"…...
JavaScript 数据类型详解
JavaScript 数据类型详解 JavaScript 数据类型分为 原始类型(Primitive) 和 对象类型(Object) 两大类,共 8 种(ES11): 一、原始类型(7种) 1. undefined 定…...