Elasticsearch封装公共索引增删改查
什么是索引?
- 定义:索引是 Elasticsearch 中用于存储数据的逻辑命名空间。它由多个文档组成,每个文档是一个 JSON 格式的结构化数据
- 对应关系:在关系数据库中,索引类似于表;而在 Elasticsearch 中,索引则相当于数据库的集合或目录。
依赖
选择方案一
使用这个依赖的话必须搭配配置类去使用
<!-- elasticsearch --><dependency><groupId>org.elasticsearch</groupId><artifactId>elasticsearch</artifactId><version>7.7.0</version></dependency><dependency><groupId>org.elasticsearch.client</groupId><artifactId>elasticsearch-rest-high-level-client</artifactId><version>7.7.0</version></dependency>
选择方案二
使用这个依赖的话配置类可写可不写,因为springboot工程已经帮我们自动的去完成配置了,不需要我们自己写了
<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-data-elasticsearch</artifactId></dependency>
配置
es:host: 111.229.0.43port: 9200scheme: http
配置类
package com.macro.mall.demo.config;import lombok.Data;
import org.apache.http.HttpHost;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestHighLevelClient;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.stereotype.Component;@Data
@Component
public class InitEsRes {@Value("${es.host}")private String host;@Value("${es.port}")private int port;@Value("${es.scheme}")private String scheme;@Beanpublic RestHighLevelClient restHighLevelClient(){return new RestHighLevelClient(RestClient.builder(new HttpHost(host,port,scheme)));}
}
dto
package com.macro.mall.demo.dto;import io.swagger.annotations.ApiModelProperty;
import jakarta.validation.constraints.NotEmpty;
import jakarta.validation.constraints.NotNull;
import lombok.Data;/*** @Author:xsp* @Description: es索引传输对象* @name:EsIndexDto* @Date:2024/10/16 15:30*/
@Data
public class EsIndexDto {/*** 索引名称*/@NotEmpty(message = "索引名称不能为空")@ApiModelProperty(value = "索引名称", required = true, example = "。。。。")private String indexName;/*** 索引映射*/@ApiModelProperty(value = "索引映射", required = true, example = "。。。。")private String indexMappings;/*** 索引配置*/@ApiModelProperty(value = "索引配置", required = true, example = "。。。。")private String indexSettings;}
controller
package com.macro.mall.demo.controller;import com.macro.mall.common.api.CommonResult;
import com.macro.mall.demo.dto.EsIndexDto;
import com.macro.mall.demo.service.EsIndexService;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import jakarta.validation.constraints.NotEmpty;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.*;import java.util.Map;/*** @Author:xsp* @Description: es索引管理* @name:EsController* @Date:2024/10/15 20:38*/
@RestController
@RequestMapping("/index")
@Validated
@Api(tags = "es索引管理")
public class EsIndexController {@Autowiredprivate EsIndexService esIndexService;/*** 创建索引的接口* @param esIndexDto 索引信息* @return*/@ApiOperation(value = "创建索引")@PostMapping("/create")public CommonResult createIndex(@Validated @RequestBody EsIndexDto esIndexDto) {esIndexService.createIndex(esIndexDto);return CommonResult.successMessage("索引创建成功"); // 调用服务方法创建索引}/*** 删除索引的接口* @param indexName 索引名称* @return*/@ApiOperation(value = "删除索引")@DeleteMapping("/delete")public CommonResult deleteIndex(@RequestParam @NotEmpty(message = "索引名称不能为空") String indexName) {esIndexService.deleteIndex(indexName); // 调用服务方法删除索引return CommonResult.successMessage("索引删除成功");}/*** 获取索引的接口* @param indexName 索引名称* @return*/@ApiOperation(value = "获取索引映射")@GetMapping("/get")public CommonResult<Map<String, Object>> getIndex(@RequestParam @NotEmpty(message = "索引名称不能为空") String indexName) {Map<String, Object> indexMappings = esIndexService.getIndex(indexName);return CommonResult.success(indexMappings); // 调用服务方法获取索引}/*** 根据索引名称修改索引配置* @param esIndexDto 索引信息* @return*/@ApiOperation(value = "修改索引配置")@PutMapping("/update")public CommonResult updateIndex(@Validated @RequestBody EsIndexDto esIndexDto) {esIndexService.updateIndex(esIndexDto);return CommonResult.successMessage("索引更新成功"); // 调用服务方法更新索引}/*** 判断索引是否存在* @param indexName 索引名称* @return*/@ApiOperation(value = "判断索引是否存在")@GetMapping("/exists")public CommonResult exists(@RequestParam @NotEmpty(message = "索引名称不能为空") String indexName) {boolean exists =esIndexService.exists(indexName);return CommonResult.success(exists);}
}
serveice
package com.macro.mall.demo.service;import com.macro.mall.demo.dto.EsDocDto;
import com.macro.mall.demo.dto.EsIndexDto;import java.util.List;
import java.util.Map;/*** @Author:xsp* @Description:* @name:EsService* @Date:2024/10/15 20:39*/
public interface EsDocService {/*** 批量添加* @param esDocDto 文档信息*/void batchAdd(EsDocDto esDocDto);/*** 批量删除* @param indexName 索引名称* @param ids 多个id*/void batchDelete(String indexName, List<String> ids);
}
impl
package com.macro.mall.demo.service.impl;import com.macro.mall.demo.dto.EsDocDto;
import com.macro.mall.demo.dto.EsIndexDto;
import com.macro.mall.demo.service.EsIndexService;
import lombok.extern.log4j.Log4j2;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.elasticsearch.action.admin.indices.create.CreateIndexRequest;
import org.elasticsearch.action.admin.indices.delete.DeleteIndexRequest;
import org.elasticsearch.action.admin.indices.settings.put.UpdateSettingsRequest;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.client.indices.GetIndexRequest;
import org.elasticsearch.client.indices.GetIndexResponse;
import org.elasticsearch.common.xcontent.XContentType;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;import java.util.Map;/*** @Author:xsp* @Description:* @name:EsServiceImpl* @Date:2024/10/15 20:39*/
@Service
@Slf4j
public class EsIndexServiceImpl implements EsIndexService {@Autowiredprivate RestHighLevelClient restHighLevelClient;/*** 创建索引** @param esIndexDto 索引信息*/@Overridepublic void createIndex(EsIndexDto esIndexDto) {// 检查索引是否已存在if (exists(esIndexDto.getIndexName())) {throw new RuntimeException("索引已经存在: " + esIndexDto.getIndexName());}// 创建索引请求CreateIndexRequest request = new CreateIndexRequest(esIndexDto.getIndexName());// 设置索引配置if (StringUtils.isNotBlank(esIndexDto.getIndexMappings())) {request.settings("_doc",esIndexDto.getIndexMappings(), XContentType.JSON);}// 执行创建索引操作try {restHighLevelClient.indices().create(request, RequestOptions.DEFAULT);log.info("索引创建成功: {}", esIndexDto.getIndexName());} catch (Exception e) {log.error("创建索引失败, 错误信息: {}", e.getMessage());throw new RuntimeException("创建索引失败: " + esIndexDto.getIndexName(), e);}}/*** 删除索引** @param indexName 索引名称*/@Overridepublic void deleteIndex(String indexName) {// 检查索引是否存在if (!exists(indexName)) {throw new RuntimeException("索引不存在: " + indexName);}// 创建删除索引请求DeleteIndexRequest request = new DeleteIndexRequest(indexName);// 执行删除索引操作try {restHighLevelClient.indices().delete(request, RequestOptions.DEFAULT);log.info("索引删除成功: {}", indexName);} catch (Exception e) {log.error("删除索引失败, 错误信息: {}", e.getMessage());throw new RuntimeException("删除索引失败: " + indexName, e);}}/*** 获取索引映射** @param indexName 索引名称* @return 索引映射信息*/@Overridepublic Map<String, Object> getIndex(String indexName) {// 检查索引是否存在if (!exists(indexName)) {throw new RuntimeException("索引不存在: " + indexName);}// 创建获取索引请求GetIndexRequest request = new GetIndexRequest(indexName);// 执行获取索引映射操作try {GetIndexResponse response = restHighLevelClient.indices().get(request, RequestOptions.DEFAULT);log.info("获取索引映射成功: {}", indexName);return response.getMappings().get(indexName).sourceAsMap(); // 返回索引映射} catch (Exception e) {log.error("获取索引映射失败, 错误信息: {}", e.getMessage());throw new RuntimeException("获取索引映射失败: " + indexName, e);}}/*** 更新索引配置** @param esIndexDto 索引信息*/@Overridepublic void updateIndex(EsIndexDto esIndexDto) {// 检查索引是否存在if (!exists(esIndexDto.getIndexName())) {throw new RuntimeException("索引不存在: " + esIndexDto.getIndexName());}// 创建更新索引设置请求UpdateSettingsRequest request = new UpdateSettingsRequest(esIndexDto.getIndexName());// 更新索引映射if (StringUtils.isNotBlank(esIndexDto.getIndexMappings())) {request.settings(esIndexDto.getIndexMappings(), XContentType.JSON);}// 执行更新索引设置操作try {boolean acknowledged = restHighLevelClient.indices().putSettings(request, RequestOptions.DEFAULT).isAcknowledged();if (acknowledged) {log.info("索引设置更新成功: {}", esIndexDto.getIndexName());} else {log.warn("索引设置更新未被确认: {}", esIndexDto.getIndexName());}} catch (Exception e) {log.error("更新索引设置失败, 错误信息: {}", e.getMessage());throw new RuntimeException("更新索引设置失败: " + esIndexDto.getIndexName(), e);}}/*** 判断索引是否存在** @param indexName 索引名称* @return 索引是否存在*/@Overridepublic boolean exists(String indexName) {// 创建获取索引请求GetIndexRequest request = new GetIndexRequest(indexName);try {// 执行获取索引操作并返回索引是否存在boolean exists = restHighLevelClient.indices().exists(request, RequestOptions.DEFAULT);log.info("判断索引是否存在: {}, 结果: {}", indexName, exists);return exists;} catch (Exception e) {log.error("判断索引是否存在失败, 错误信息: {}", e.getMessage());return false; // 返回判断失败}}}
统一结果集
package com.macro.mall.common.api;import cn.hutool.json.JSONUtil;/*** 通用返回对象* Created by 9a8204a7-f77d-4ab8-ae70-b4721fef2f95 on 2019/4/19.*/
public class CommonResult<T> {private long code;private String message;private T data;protected CommonResult() {}protected CommonResult(long code, String message, T data) {this.code = code;this.message = message;this.data = data;}/*** 成功返回信息* @param message 提示信息*/public static <T> CommonResult<T> successMessage(String message) {return new CommonResult<T>(ResultCode.SUCCESS.getCode(), message, null);}/*** 成功返回结果** @param data 获取的数据*/public static <T> CommonResult<T> success(T data) {return new CommonResult<T>(ResultCode.SUCCESS.getCode(), ResultCode.SUCCESS.getMessage(), data);}/*** 成功返回结果** @param data 获取的数据* @param message 提示信息*/public static <T> CommonResult<T> success(T data, String message) {return new CommonResult<T>(ResultCode.SUCCESS.getCode(), message, data);}/*** 失败返回结果* @param errorCode 错误码*/public static <T> CommonResult<T> failed(IErrorCode errorCode) {return new CommonResult<T>(errorCode.getCode(), errorCode.getMessage(), null);}/*** 失败返回结果* @param errorCode 错误码* @param message 错误信息*/public static <T> CommonResult<T> failed(IErrorCode errorCode,String message) {return new CommonResult<T>(errorCode.getCode(), message, null);}/*** 失败返回结果* @param message 提示信息*/public static <T> CommonResult<T> failed(String message) {return new CommonResult<T>(ResultCode.FAILED.getCode(), message, null);}/*** 失败返回结果*/public static <T> CommonResult<T> failed() {return failed(ResultCode.FAILED);}/*** 参数验证失败返回结果*/public static <T> CommonResult<T> validateFailed() {return failed(ResultCode.VALIDATE_FAILED);}/*** 参数验证失败返回结果* @param message 提示信息*/public static <T> CommonResult<T> validateFailed(String message) {return new CommonResult<T>(ResultCode.VALIDATE_FAILED.getCode(), message, null);}/*** 未登录返回结果*/public static <T> CommonResult<T> unauthorized(T data) {return new CommonResult<T>(ResultCode.UNAUTHORIZED.getCode(), ResultCode.UNAUTHORIZED.getMessage(), data);}/*** 未授权返回结果*/public static <T> CommonResult<T> forbidden(T data) {return new CommonResult<T>(ResultCode.FORBIDDEN.getCode(), ResultCode.FORBIDDEN.getMessage(), data);}public long getCode() {return code;}public void setCode(long code) {this.code = code;}public String getMessage() {return message;}public void setMessage(String message) {this.message = message;}public T getData() {return data;}public void setData(T data) {this.data = data;}@Overridepublic String toString() {return JSONUtil.toJsonStr(this);}
}
这里我用的是这个统一结果集,结合自己实际情况去使用相对应的统一结果集
Spring原生效验异常
@ResponseBody@ExceptionHandler(value = ApiException.class)public CommonResult handle(ApiException e) {if (e.getErrorCode() != null) {return CommonResult.failed(e.getErrorCode());}return CommonResult.failed(e.getMessage());}@ResponseBody@ExceptionHandler(value = MethodArgumentNotValidException.class)public CommonResult handleValidException(MethodArgumentNotValidException e) {BindingResult bindingResult = e.getBindingResult();String message = null;if (bindingResult.hasErrors()) {FieldError fieldError = bindingResult.getFieldError();if (fieldError != null) {message = fieldError.getField()+fieldError.getDefaultMessage();}}return CommonResult.validateFailed(message);}@ResponseBody@ExceptionHandler(value = BindException.class)public CommonResult handleValidException(BindException e) {BindingResult bindingResult = e.getBindingResult();String message = null;if (bindingResult.hasErrors()) {FieldError fieldError = bindingResult.getFieldError();if (fieldError != null) {message = fieldError.getField()+fieldError.getDefaultMessage();}}return CommonResult.validateFailed(message);}/*** 最大异常* @param e* @return*/@ResponseBody@ExceptionHandler(value = Exception.class)public CommonResult handle(Exception e) {e.printStackTrace();return CommonResult.validateFailed(e.getMessage());}
这里我是用的这几个写的异常捕获器,结合自己实际情况去使用相对应的异常捕获
目前先更新到这里,后期补发
相关文章:
Elasticsearch封装公共索引增删改查
什么是索引? 定义:索引是 Elasticsearch 中用于存储数据的逻辑命名空间。它由多个文档组成,每个文档是一个 JSON 格式的结构化数据对应关系:在关系数据库中,索引类似于表;而在 Elasticsearch 中࿰…...
Python异常检测:Isolation Forest与局部异常因子(LOF)详解
这里写目录标题 Python异常检测:Isolation Forest与局部异常因子(LOF)详解引言一、异常检测的基本原理1.1 什么是异常检测?1.2 异常检测的应用场景 二、Isolation Forest2.1 Isolation Forest的原理2.1.1 算法步骤 2.2 Python实现…...
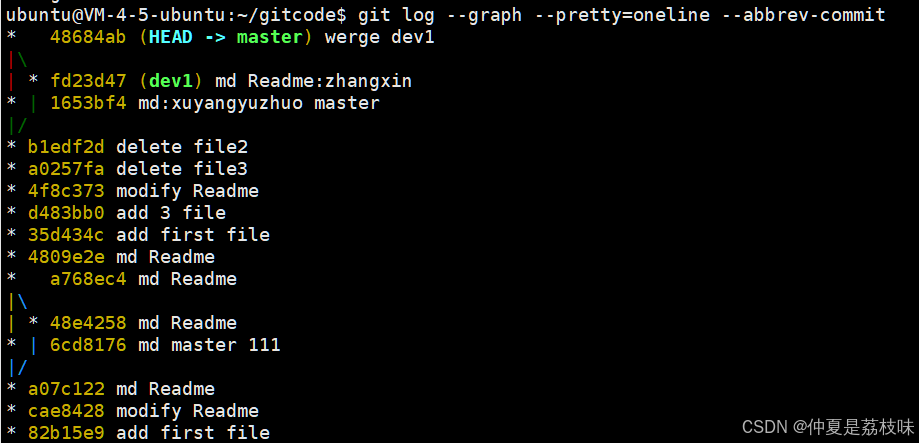
Git的原理和使用(二)
1. git的版本回退 之前我们也提到过,Git 能够管理⽂件的历史版本,这也是版本控制器重要的能⼒。如果有⼀天你发现 之前前的⼯作做的出现了很⼤的问题,需要在某个特定的历史版本重新开始,这个时候,就需要版本 回退的功能…...
docker 发布镜像
如果要推广自己的软件,势必要自己制作 image 文件。 1 制作自己的 Docker 容器 基于 centos 镜像构建自己的 centos 镜像,可以在 centos 镜像基础上,安装相关的软件,之后进行构建新的镜像。 1.1 dockerfile 文件编写 首先&…...
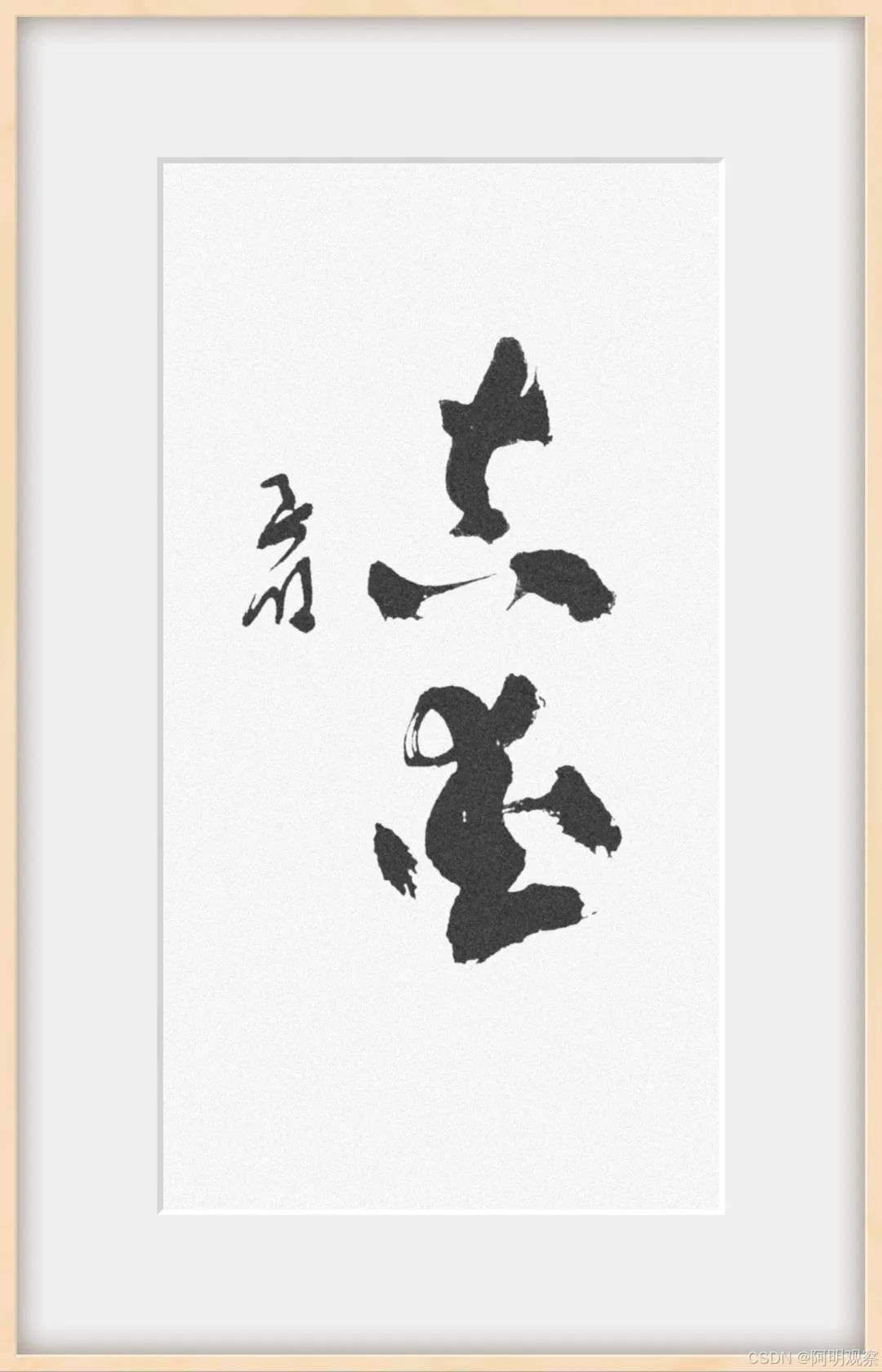
投了15亿美元,芯片创新公司Ampere为何成了Oracle真爱?
【科技明说 | 科技热点关注】 一个数据库软件公司却想要操控一家芯片厂商,这样的想法不错。也真大胆。 目前,全球数据库巨头甲骨文Oracle已经持有Ampere Computing LLC 29%的股份,并有可能通过未来的投资选择权获得对这家芯片制造…...
vue 报告标题时间来自 elementUI的 el-date-picker 有开始时间和结束时间
要在Vue中使用 Element UI 的 el-date-picker 来选择开始时间和结束时间,并将其展示在报告中,以下是详细的实现步骤。 实现思路: 使用 Element UI 的 el-date-picker 组件,让用户选择时间范围(开始时间和结束时间&am…...
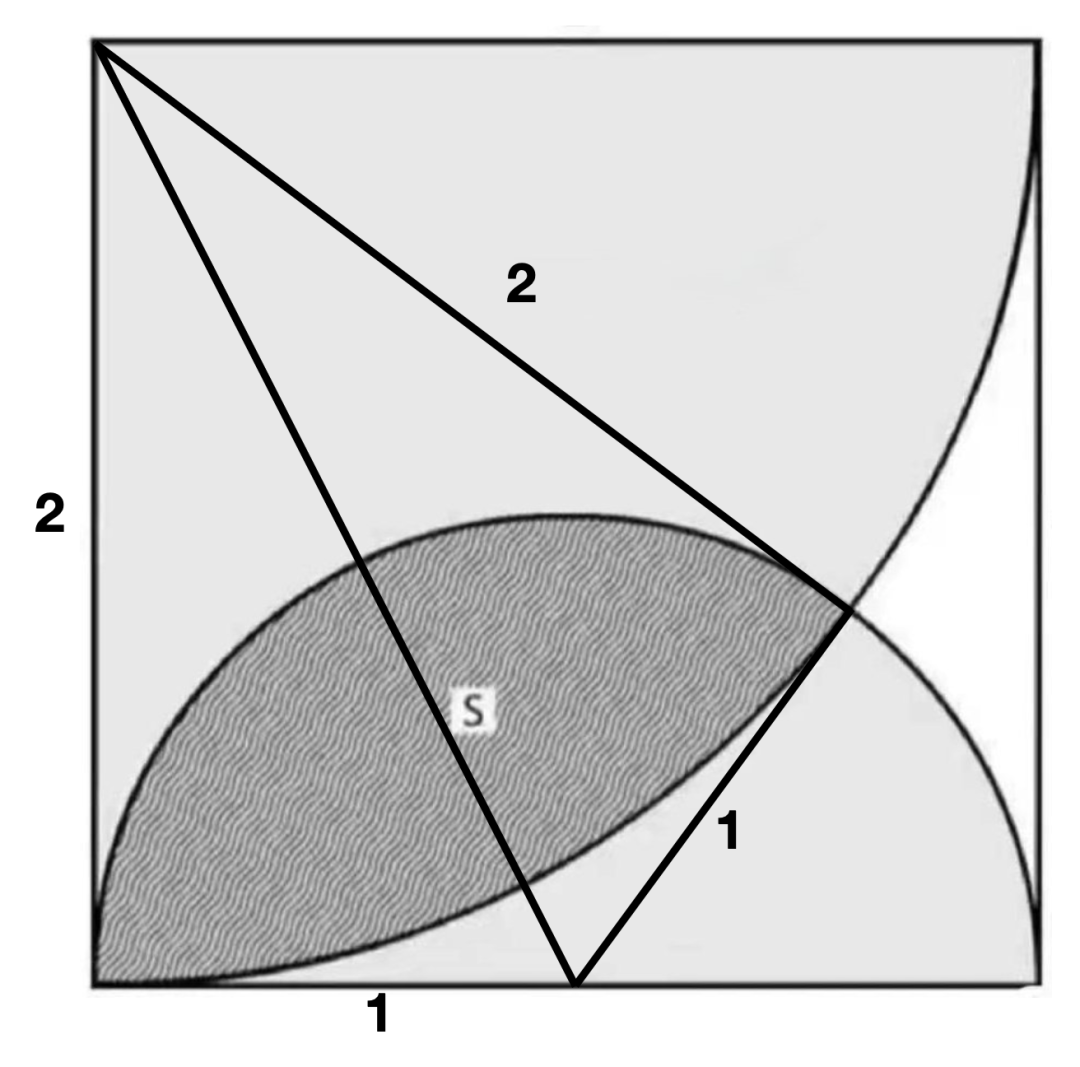
简单几何问题的通解
来,这道题怎么做?边长为2的正方形内,2个扇形的交集面积是多少?这道题一定要画辅助线,因为要用到两个扇形的交点,如果不画辅助线,这个交点相关的4个子图一个都无法求出面积,只能求出子…...
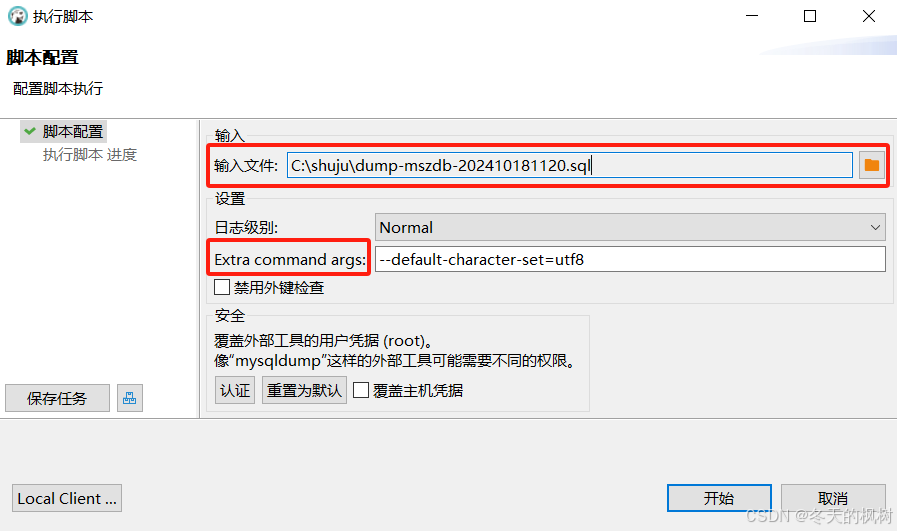
DBeaver导出数据表结构和数据,导入到另一个环境数据库进行数据更新
在工作中,我们会进行不同环境之间数据库的数据更新,这里使用DBeaver导出新的数据表结构和数据,并执行脚本,覆盖另一个环境的数据库中对应数据表,完成数据表的更新。 一、导出 右键点击选中想要导出的数据表࿰…...
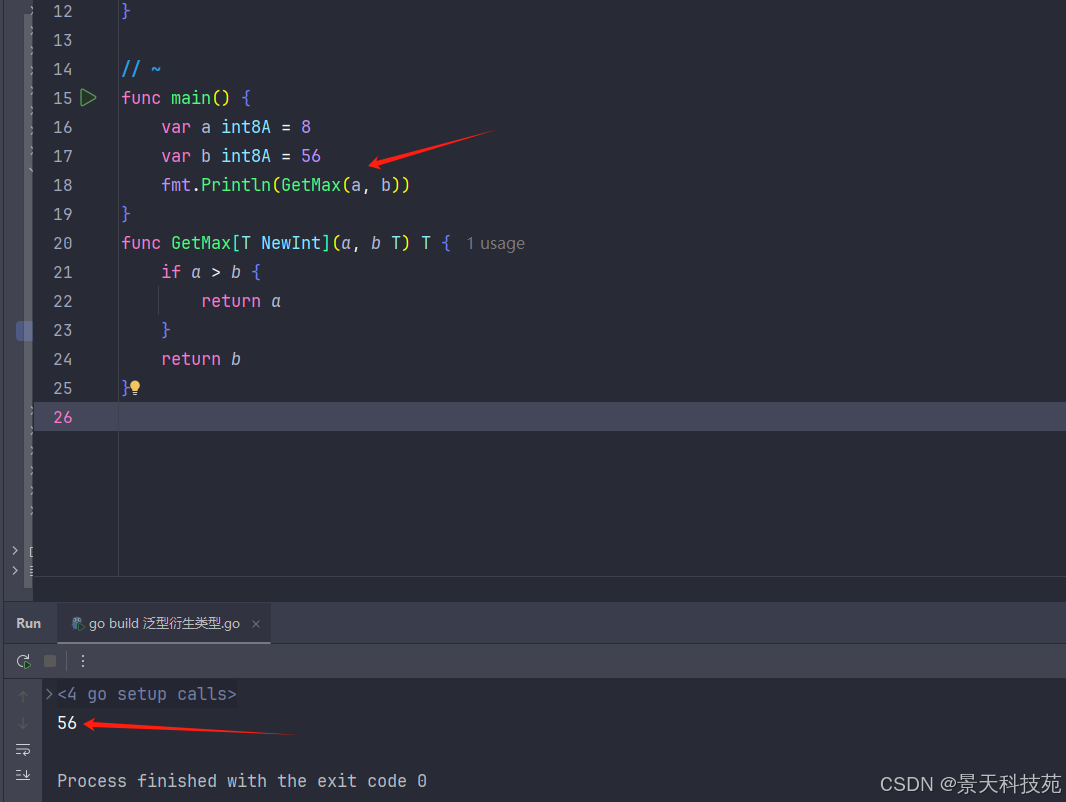
【Golang】合理运用泛型,简化开发流程
✨✨ 欢迎大家来到景天科技苑✨✨ 🎈🎈 养成好习惯,先赞后看哦~🎈🎈 🏆 作者简介:景天科技苑 🏆《头衔》:大厂架构师,华为云开发者社区专家博主,…...
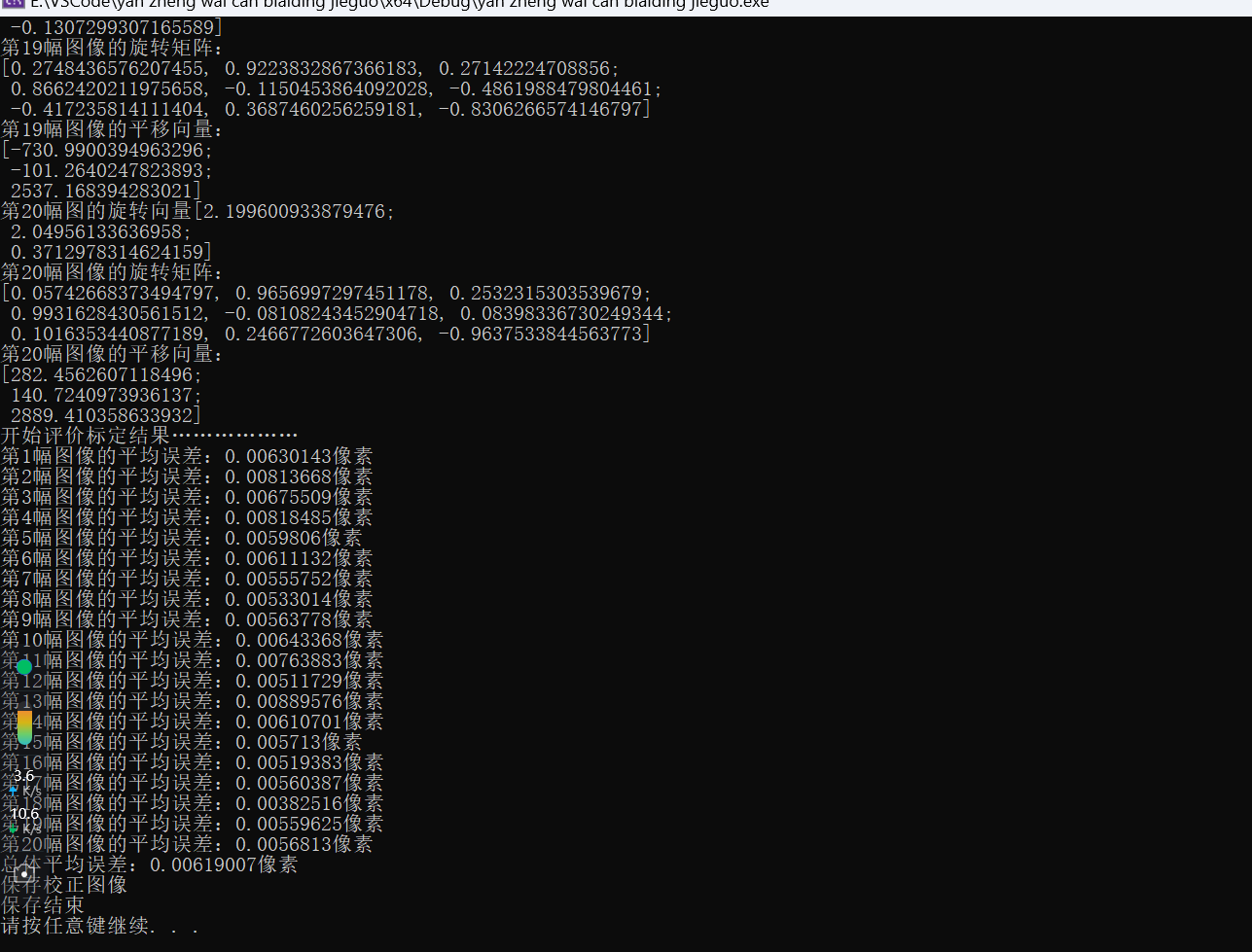
OpenCV单目相机内参标定C++
基于OpenCV 实现单目相机内参标定: a.使用OpenCV库实现内参标定过程。通过角点检测、亚像素角点定位、角点存储与三维坐标生成和摄像机标定分别获取左右相机的内参。 b.具体地,使用库函数检测两组图像(左右相机拍摄图像)中棋盘格…...
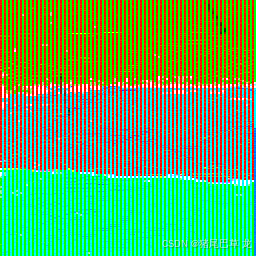
基于MATLAB(DCT DWT)
第三章 图像数字水印的方案 3.1 图像数字水印的技术方案 在数据库中存储在国际互联网上传输的水印图像一般会被压缩,有时达到很高的压缩比。因此,数字水印算法所面临的第一个考验就是压缩。JPEG和EZW(Embedded Zero-Tree Wavelet࿰…...
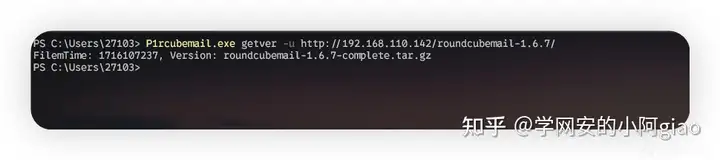
渗透基础-rcube_webmail版本探测
简介 本文介绍了开源产品RoundCube webmail邮件系统的版本探测思路,并用go语言实现工具化、自动化探测。 正文 0x01 探测思路研究 探测系统版本,最理想的方法就是系统主页html代码中有特定的字符串,比如特定版本对应的hash在主页的html代…...
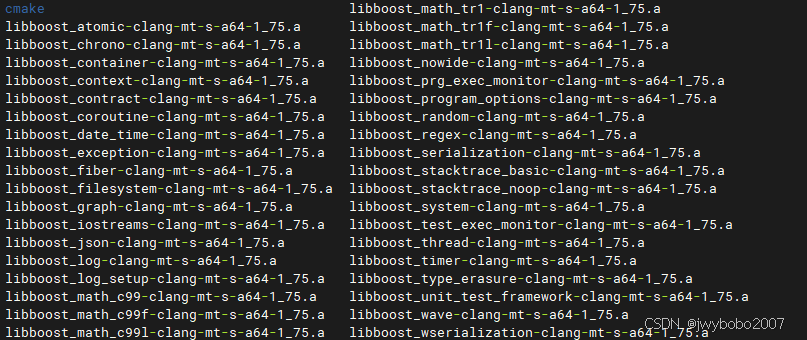
linux下编译鸿蒙版boost库
我在上一篇文章中介绍了curl和openssl的编译方式(linux下编译鸿蒙版curl、openssl-CSDN博客),这篇再介绍一下boost库的编译。 未经许可,请勿转载! 一.环境准备 1.鸿蒙NDK 下载安装方式可以参考上篇文章,…...
滚雪球学Redis[6.3讲]:Redis分布式锁的实战指南:从基础到Redlock算法
全文目录: 🎉前言🚦Redis分布式锁的概念与应用场景🍃1.1 什么是分布式锁?🍂1.2 应用场景 ⚙️使用Redis实现分布式锁🌼2.1 基本思路🌻2.2 示例代码🥀2.3 代码解析 &#…...
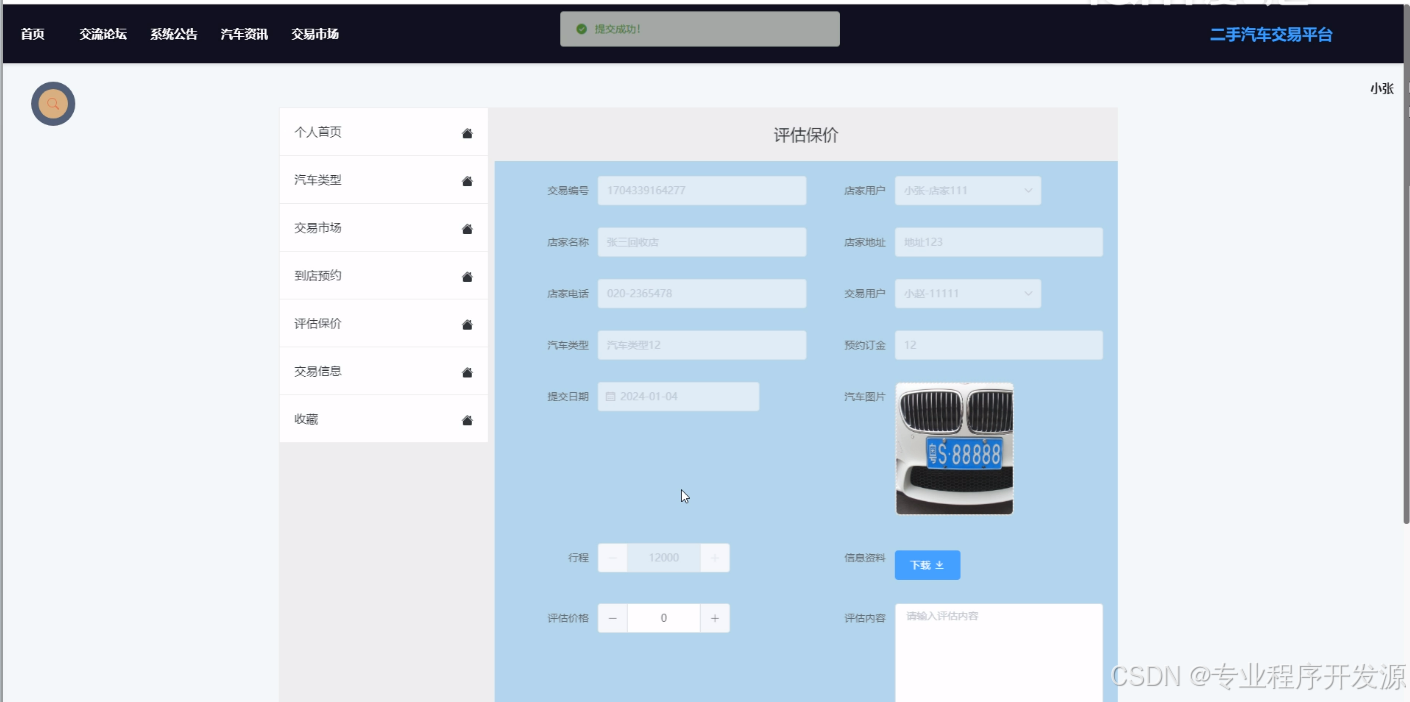
springboot二手汽车交易平台-计算机毕业设计源码82053
目录 1 绪论 1.1研究背景 1.2研究意义 1.3国内外研究现状 2 二手汽车交易平台系统分析 2.1 可行性分析 2.2 系统流程分析 2.3 功能需求分析 2.4 性能需求分析 3 二手汽车交易平台概要设计 3.1 系统体系结构设计 3.2总体功设计 3.3子模块设计设计 3.4 数据库设计 …...
typescript 中的类型推断
在 TypeScript 中,类型推断(Type Inference)是一种编译器自动确定变量或表达式类型的能力。这大大减少了需要显式声明类型的代码量,使得代码更加简洁和易读。TypeScript 的类型推断机制非常强大,可以在很多情况下自动推…...
linux 隐藏文件
在Linux中,隐藏文件以点(.)开头的文件或文件夹被认为是隐藏文件。隐藏文件通常用于存储系统配置文件或敏感文件。 以下是几种不同的方法来隐藏文件或文件夹: 方法1:在文件或文件夹名字前面加上点(.&#…...
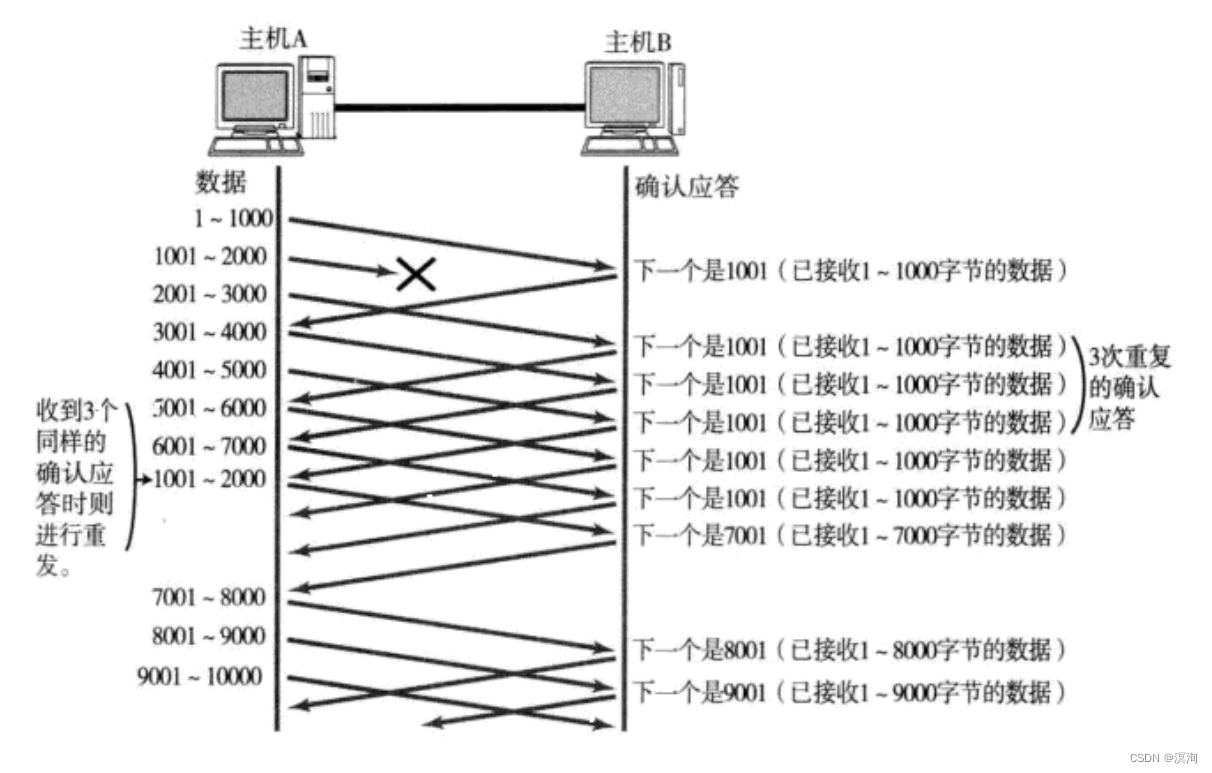
【网络协议栈】Tcp协议(上)结构的解析 和 Tcp中的滑动窗口(32位确认序号、32位序号、4位首部长度、6位标记位、16为窗口大小、16位紧急指针)
绪论 “没有那么多天赋异禀,优秀的人总是努力翻山越岭。”本章主要讲到了再五层网络协议从上到下的第二层传输层中使用非常广泛的Tcp协议他的协议字段结构,通过这些字段去认识其Tcp协议运行的原理底层逻辑和基础。后面将会再写一篇Tcp到底是通过什么调…...
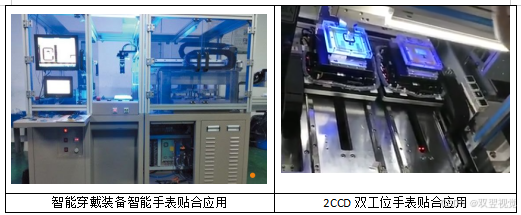
手表玻璃盖板视觉贴合
在手表生产过程中,贴合加工是一个至关重要的环节,它涉及将手表的盖板与LCM模组或各种功能片进行精准贴合。这一过程不仅要求高度的精度,还追求效率与稳定性,以满足现代可穿戴设备日益增长的市场需求。然而,当前行业在这…...
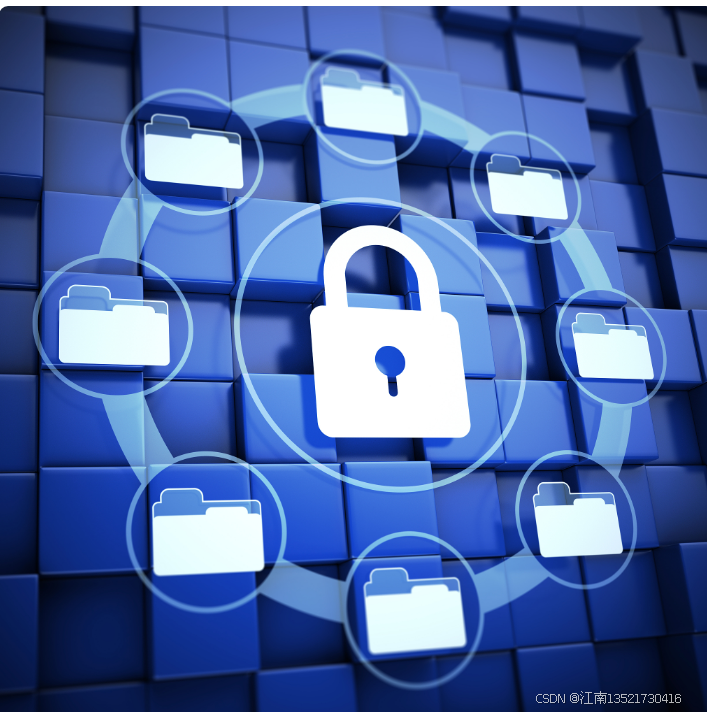
电信和互联网行业数据安全评估师CCRC-DSA人才强基计划
“电信和互联网行业数据安全人才强基计划”(以下简称“强基计划”)自 2022 年 4 月启动伊始,始终秉持以人才需求为导向,以体系化能力建设为重点,扎实铸就数据安全人才培养品牌,力促行业数据安全人才培养工作…...
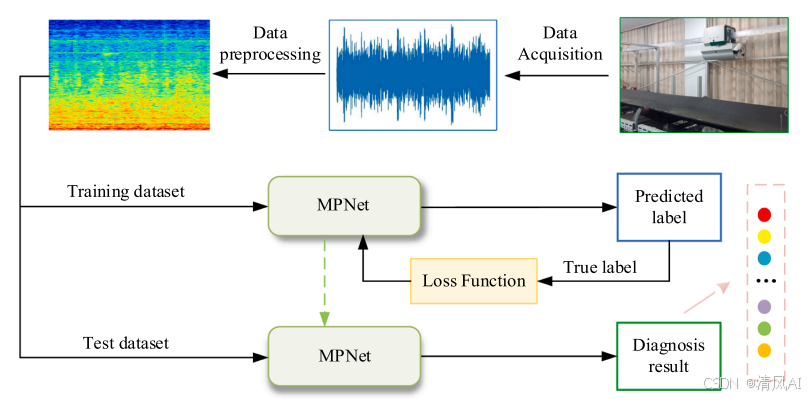
MPNet:旋转机械轻量化故障诊断模型详解python代码复现
目录 一、问题背景与挑战 二、MPNet核心架构 2.1 多分支特征融合模块(MBFM) 2.2 残差注意力金字塔模块(RAPM) 2.2.1 空间金字塔注意力(SPA) 2.2.2 金字塔残差块(PRBlock) 2.3 分类器设计 三、关键技术突破 3.1 多尺度特征融合 3.2 轻量化设计策略 3.3 抗噪声…...
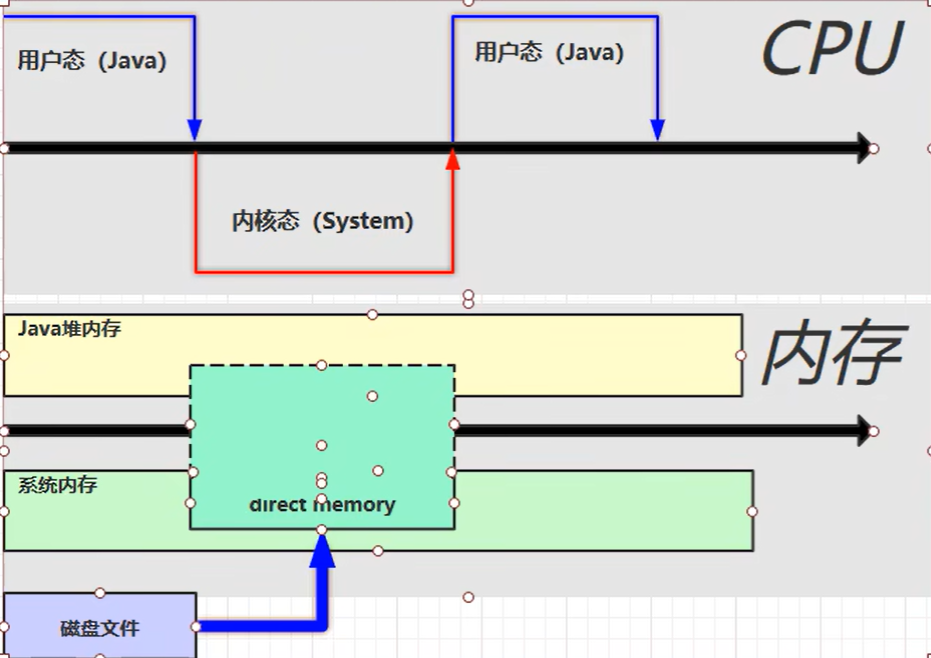
【JVM】- 内存结构
引言 JVM:Java Virtual Machine 定义:Java虚拟机,Java二进制字节码的运行环境好处: 一次编写,到处运行自动内存管理,垃圾回收的功能数组下标越界检查(会抛异常,不会覆盖到其他代码…...
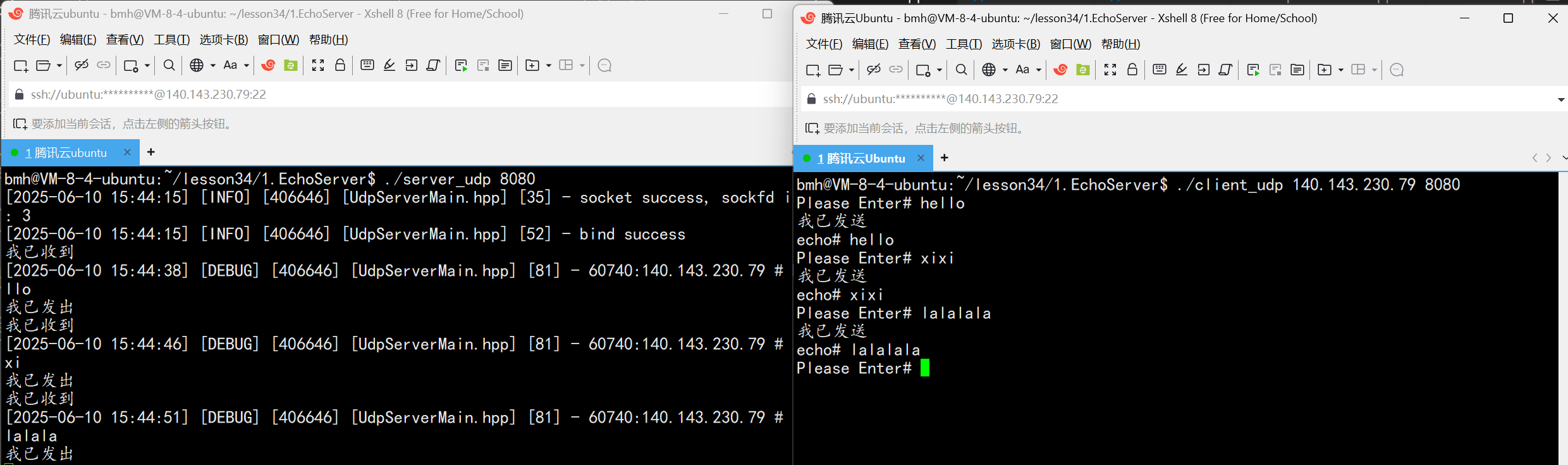
UDP(Echoserver)
网络命令 Ping 命令 检测网络是否连通 使用方法: ping -c 次数 网址ping -c 3 www.baidu.comnetstat 命令 netstat 是一个用来查看网络状态的重要工具. 语法:netstat [选项] 功能:查看网络状态 常用选项: n 拒绝显示别名&#…...
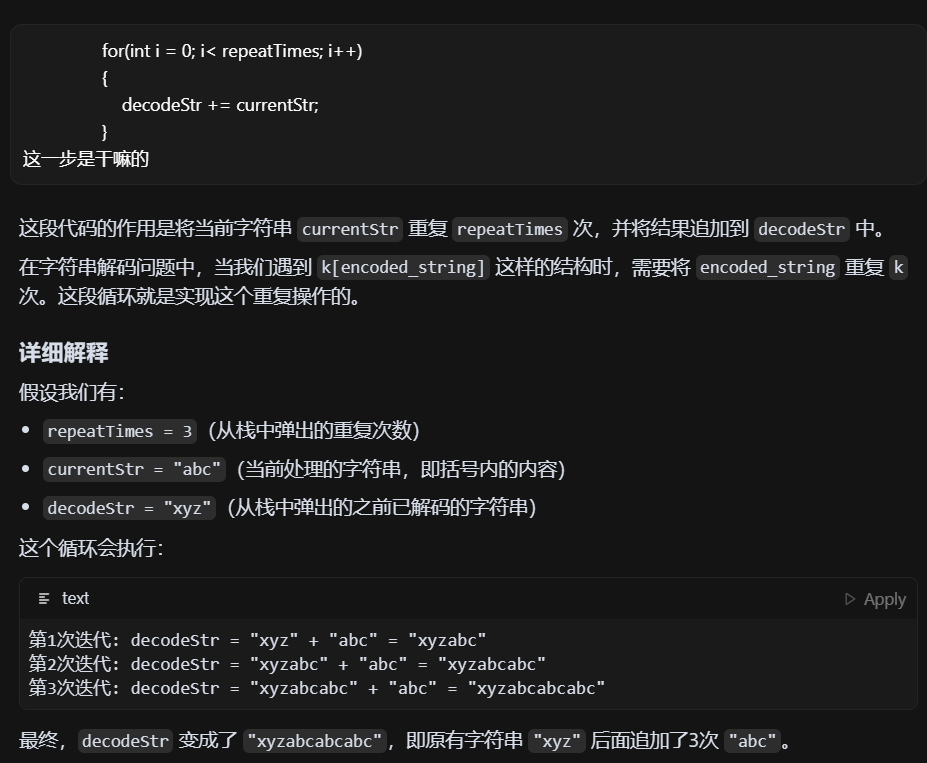
LeetCode - 394. 字符串解码
题目 394. 字符串解码 - 力扣(LeetCode) 思路 使用两个栈:一个存储重复次数,一个存储字符串 遍历输入字符串: 数字处理:遇到数字时,累积计算重复次数左括号处理:保存当前状态&a…...
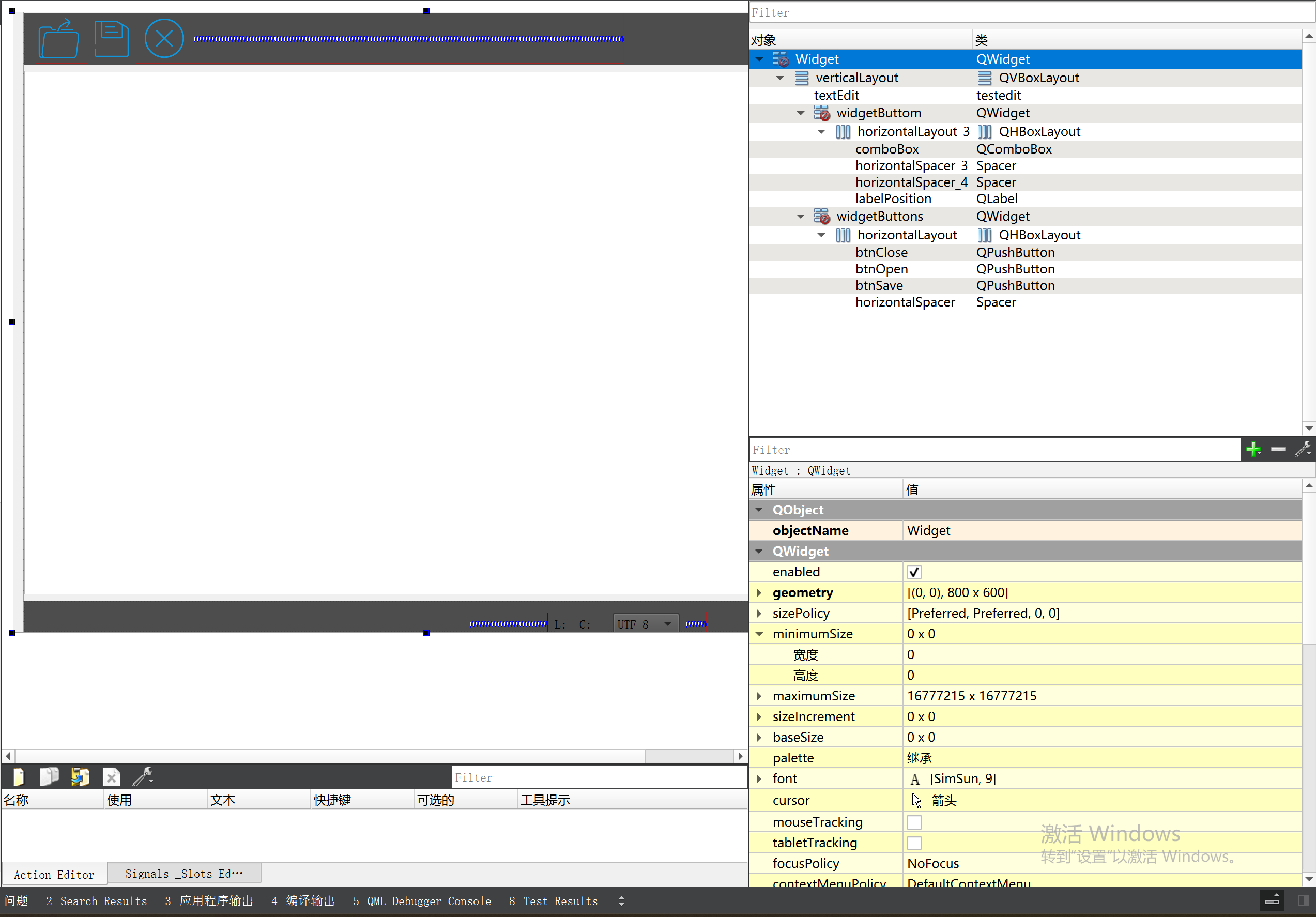
P3 QT项目----记事本(3.8)
3.8 记事本项目总结 项目源码 1.main.cpp #include "widget.h" #include <QApplication> int main(int argc, char *argv[]) {QApplication a(argc, argv);Widget w;w.show();return a.exec(); } 2.widget.cpp #include "widget.h" #include &q…...
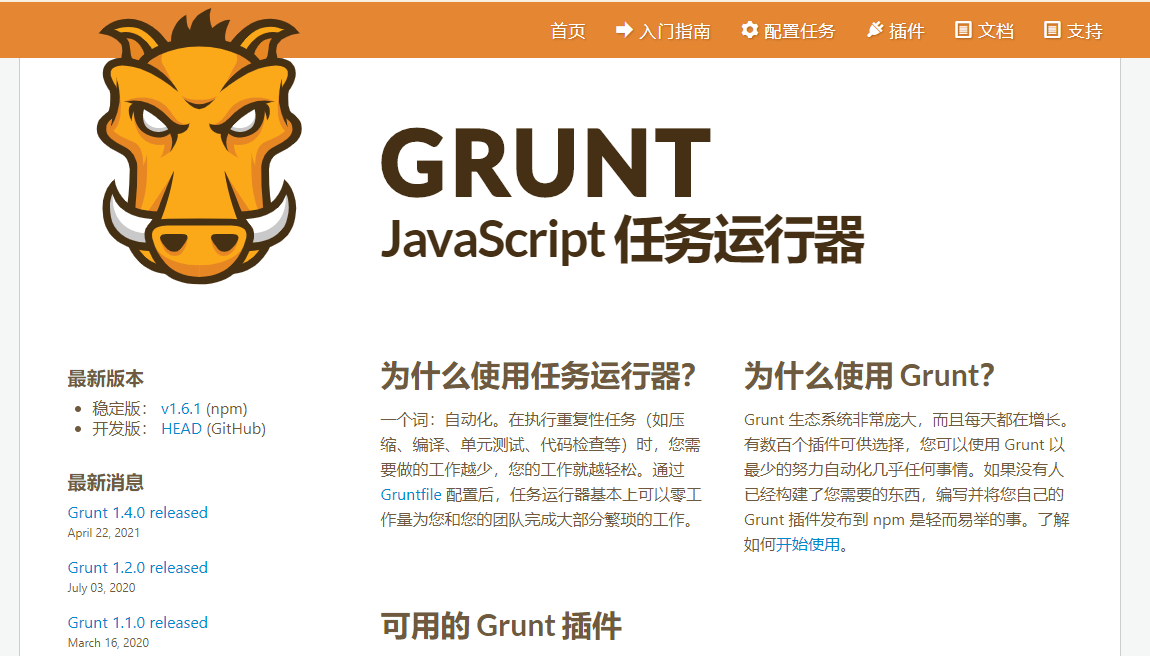
GruntJS-前端自动化任务运行器从入门到实战
Grunt 完全指南:从入门到实战 一、Grunt 是什么? Grunt是一个基于 Node.js 的前端自动化任务运行器,主要用于自动化执行项目开发中重复性高的任务,例如文件压缩、代码编译、语法检查、单元测试、文件合并等。通过配置简洁的任务…...
JS手写代码篇----使用Promise封装AJAX请求
15、使用Promise封装AJAX请求 promise就有reject和resolve了,就不必写成功和失败的回调函数了 const BASEURL ./手写ajax/test.jsonfunction promiseAjax() {return new Promise((resolve, reject) > {const xhr new XMLHttpRequest();xhr.open("get&quo…...
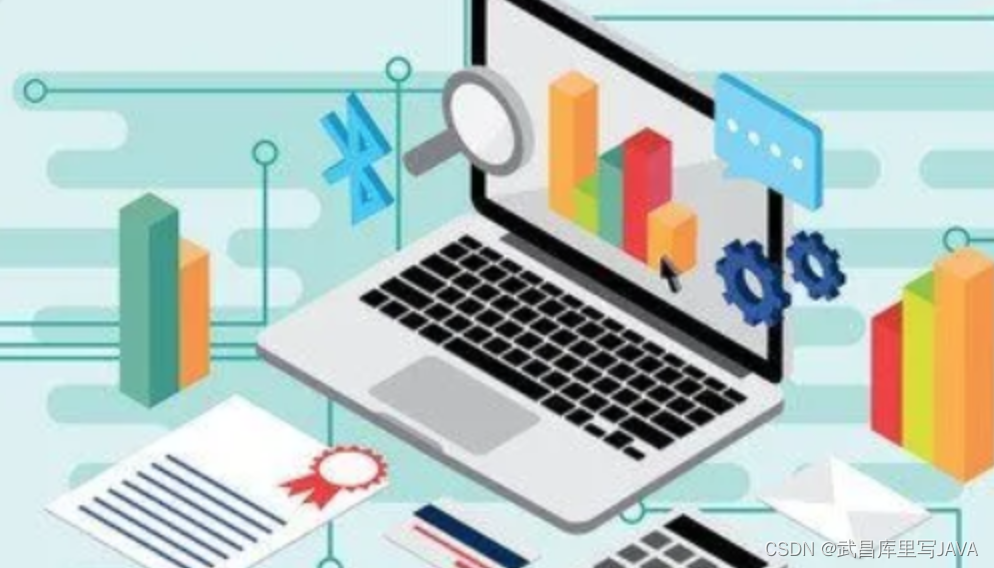
iview框架主题色的应用
1.下载 less要使用3.0.0以下的版本 npm install less2.7.3 npm install less-loader4.0.52./src/config/theme.js文件 module.exports {yellow: {theme-color: #FDCE04},blue: {theme-color: #547CE7} }在sass中使用theme配置的颜色主题,无需引入,直接可…...
MySQL 部分重点知识篇
一、数据库对象 1. 主键 定义 :主键是用于唯一标识表中每一行记录的字段或字段组合。它具有唯一性和非空性特点。 作用 :确保数据的完整性,便于数据的查询和管理。 示例 :在学生信息表中,学号可以作为主键ÿ…...
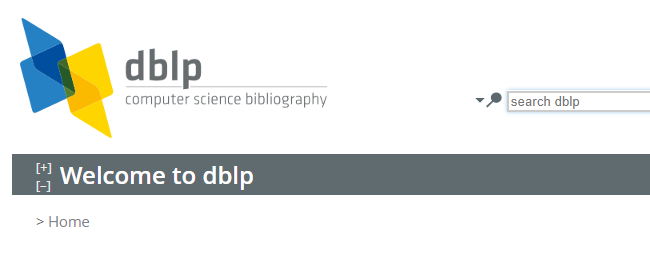
DBLP数据库是什么?
DBLP(Digital Bibliography & Library Project)Computer Science Bibliography是全球著名的计算机科学出版物的开放书目数据库。DBLP所收录的期刊和会议论文质量较高,数据库文献更新速度很快,很好地反映了国际计算机科学学术研…...