redis数据类型:list
list 的相关命令配合使用的应用场景:
- 栈和队列:插入和弹出命令的配合,亦可实现栈和队列的功能
实现哪种数据结构,取决于插入和弹出
命令的配合,如 左插右出
或右插左出
:这两种种方式实现先进先出的数据结构,即异侧配合
可以实现队列结构。左插左出
或者右插右出
:这两种方式可以实现先进后出的数据结构,即同侧配合
可以实现栈结构。- 消息队列:实现
发布与订阅
模型。- 生产者使用尾部插入命令
RPUSH
将消息插入 list; 消费者使用LPOP
命令从 list 的左边消费消息 - 生产者使用尾部插入命令
LPUSH
将消息插入 list; 消费者使用RPOP
命令从 list 的左边消费消息
- 生产者使用尾部插入命令
- 限流:
- 每次请求时向 List 添加时间戳,通过检查 List 长度来决定是否允许新的请求,实现 API 请求频率控制。
- 缓存记录:聊天记录、文章推送,热点数据等。
简介
Redis lists are linked lists of string values. Redis lists are frequently used to:
- Implement stacks and queues.
- Build queue management for background worker systems.
list 结构示意图,可参考 python 中的 list,特别是范围查找逻辑
list 类型支持双向查找:
- 正序查找:如 0 到 5,从头开始算起,
- 负序查找:如 0 到 -5,
通常用于实现栈和队列:
- 栈:先进后出,使用 list 的 lpush 可以实现栈的功能,依次向前插入数据,按正序索引取值的时候,可以实现栈的功能。
- 队列:先进先出,使用 rpush可以实现队列的功能,依次向后插入数据,按正序索引取值的时候,可以实现队列的功能。
The max length of a Redis list is 2 32 − 1 2^{32} - 1 232−1 (4,294,967,295) elements.
命令
插入元素
- 头部插入:
LPUSH
adds a new element to the head of a list;
LPUSH key element [element ...]
该命令会向list 的头部依次插入给定的元素,在读取该命令插入数据的时候,就像在使用栈一样,元素先进后出。
- 逐次插入元素示意图,这种方式可以将 list 当栈来使用
127.0.0.1:6379> lpush block jj1
(integer) 1
127.0.0.1:6379> lindex block 0
"jj1"
127.0.0.1:6379> lpush block jj2
(integer) 2
127.0.0.1:6379> lindex block 0
"jj2"
127.0.0.1:6379>
- 批量插入元示意图,取出元素的时候,可以发现符合栈的结构特点:先进后出。
127.0.0.1:6379> lpush block jj1 jj2 jj3 jj4 jj5
(integer) 5
上述命令执行逻辑是,元素依次入栈。jj1
将位于栈低,jj5
将位于栈顶。
127.0.0.1:6379> lrange block 0 5
1) "jj5"
2) "jj4"
3) "jj3"
4) "jj2"
5) "jj1"
上述命令执行结果存储示意图
- 尾部插入:
RPUSH
adds to the tail.
RPUSH key element [element ...]
该命令会向list 的尾部依次插入给定的元素,在读取该命令插入数据的时候,就像在使用队列一样,元素先进先出。
127.0.0.1:6379> rpush block1 jj1 jj2 jj3 jj4 jj5
(integer) 5
127.0.0.1:6379> lindex block1 0
"jj1"
127.0.0.1:6379>
查找元素的索引
LPOS key element [RANK rank] [COUNT num-matches] [MAXLEN len]
RANK rank
: 排名,次序- 假设 list 中有多个 a,则
rank 2
表示查找 第二个 a 出现的位置,可参考案例 rank
:可以是赋值,表示倒数第一个,rank -1
表示倒数第一个
- 假设 list 中有多个 a,则
The command returns the index of matching elements inside a Redis list. By default, when no options are given, it will scan the list from head to tail, looking for the first match of “element”. If the element is found, its index (the zero-based position in the list) is returned. Otherwise, if no match is found,
nil
is returned.
- 该命令返回 Redis 列表中匹配元素的索引。
- 默认情况下,如果没有给出任何选项,它将从头到尾扫描列表,寻找第一个匹配的“元素”。
- 如果找到元素,则返回其索引(列表中从零开始的位置)。否则,如果未找到匹配项,则返回 nil。
- 查看现有数据
127.0.0.1:6379> lrange block 0 8
1) "jj5"
2) "a"
3) "jj4"
4) "a"
5) "a"
6) "jj3"
7) "jj2"
8) "jj1"
127.0.0.1:6379>
- 查找第一个 a 所在的索引(位置): rank n 表示第一个,
- n为正序:表示正数第几个
- n为负数:表示倒数第几个:
127.0.0.1:6379> lpos block a
(integer) 1
127.0.0.1:6379> lpos block a rank 1
(integer) 1
127.0.0.1:6379> lpos block a rank -1 # 倒数第1个 a的位置
(integer) 4
127.0.0.1:6379> lpos block a rank -2 # 倒数第2个 a的位置
(integer) 3
127.0.0.1:6379>
- 返回前n 个 指定元素所在的位置,如返回 list 中前两个 a 的位置:
127.0.0.1:6379> lpos block a count 2 # 前2 个所在的位置
1) (integer) 1
2) (integer) 3
127.0.0.1:6379>
元素的个数
LLEN
returns the length of a list.
Returns the length of the list stored at
key
. Ifkey
does not exist, it is interpreted as an empty list and0
is returned. An error is returned when the value stored atkey
is not a list.
返回按键存储的列表的长度。
- 如果键不存在,则将其解释为空列表并返回0。
- 如果键上存储的值不是列表,则返回错误。
- 查看 block元素的个数
127.0.0.1:6379> llen block
(integer) 5
127.0.0.1:6379>
- 查看所有的键
127.0.0.1:6379> keys *
1) "coinmarketapikey"
2) "block"
3) "exchangerate"
4) "apikeyexceeded"
5) "mysite"
127.0.0.1:6379>
- 查看不存在的键的元素个数,返回 0
127.0.0.1:6379> llen block1
(integer) 0
127.0.0.1:6379>
- 使用此命令查看 hash 类型:命令错误的使用方式
127.0.0.1:6379> llen mysite
(error) WRONGTYPE Operation against a key holding the wrong kind of value
127.0.0.1:6379>
删除元素
- 头部移除:
LPOP
removes and returns an element from the head of a list;
Removes and returns the first elements of the list stored at
key
.By default, the command pops a single element from the beginning of the list. When provided with the optional
count
argument, the reply will consist of up tocount
elements, depending on the list’s length.
- 移除并返回 list 的第一个元素。
- 如果给定了个数 count,则会从 list前面移除指定个数的元素。
LPOP key [count]
- 尾部移除:
RPOP
does the same but from the tails of a list.
LPOP key [count]
Removes and returns the last elements of the list stored at
key
.By default, the command pops a single element from the end of the list. When provided with the optional
count
argument, the reply will consist of up tocount
elements, depending on the list’s length.
- 移除并返回 list 的最后一个元素。
- 如果给定了个数 count,则会从 list后面移除指定个数的元素。
移走元素
LMOVE
atomically moves elements from one list to another.
LMOVE source destination <LEFT | RIGHT> <LEFT | RIGHT>
<LEFT | RIGHT>
- 第一个选项,指要移出位于source 的
头部|尾部
的元素 - 第二个选项,从source 移除的元素要插入 destination 的位置
头部|尾部
- 第一个选项,指要移出位于source 的
Atomically returns and removes the first/last element (head/tail depending on the
wherefrom
argument) of the list stored atsource
, and pushes the element at the first/last element (head/tail depending on thewhereto
argument) of the list stored atdestination
.
原子地返回并删除存储在源中的列表的第一个/最后一个元素(head/tail 取决于 where 参数) ,并将元素推送到存储在目的地的列表的第一个/最后一个元素(head/tail 取决于 where 参数)。
For example: consider
source
holding the lista,b,c
, anddestination
holding the listx,y,z
. ExecutingLMOVE source destination RIGHT LEFT
results insource
holdinga,b
anddestination
holdingc,x,y,z
.
举例说明:假设现有两个 list:
- key 为 source 值为
a,b,c
, - key 为 destination 值为
x,y,z
执行移走命令:
LMOVE source destination RIGHT LEFT
结果为:
- source 值为
a,b
, - destination 值为
c,x,y,z
获取元素
按下表(索引)检索
LINDEX key index
Returns the element at index
index
in the list stored atkey
. The index is zero-based, so0
means the first element,1
the second element and so on. Negative indices can be used to designate elements starting at the tail of the list. Here,-1
means the last element,-2
means the penultimate and so forth.When the value at
key
is not a list, an error is returned.
- 返回键存储的列表中索引位置的元素。
- 索引是从零开始的,所以0表示第一个元素,1表示第二个元素,依此类推。
- 负索引可用于指定从列表尾部开始的元素。在这里,-1表示最后一个元素,-2表示倒数第二个元素,依此类推。
- 当 key 值不是列表时,将返回错误。
按范围检索
可参考 python 中的 list
if __name__ == '__main__':list_ = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]print(list_[0:-1])print(list_[-5:-3])
LRANGE
extracts a range of elements from a list,与 python 中的 list 有着同样的结构。
LRANGE key start stop
- start:包含此位置的元素
- stop:包含此位置的元素
Returns the specified elements of the list stored at
key
. The offsetsstart
andstop
are zero-based indexes, with0
being the first element of the list (the head of the list),1
being the next element and so on.These offsets can also be negative numbers indicating offsets starting at the end of the list. For example,
-1
is the last element of the list,-2
the penultimate, and so on.Out of range indexes will not produce an error. If
start
is larger than the end of the list, an empty list is returned. Ifstop
is larger than the actual end of the list, Redis will treat it like the last element of the list.
- 返回按键存储的列表的指定元素。
- 偏移量 start 和 stop 是从零开始的索引,0是列表的第一个元素(列表的头) ,1是下一个元素,依此类推。
- 这些偏移量也可以是负数,表示从列表末尾开始的偏移量。例如,-1是列表的最后一个元素,-2是倒数第二个元素,依此类推。
- 索引越界:不存在这种异常,redis 会自动处理
127.0.0.1:6379> lrange block -8 -3
1) "jj5"
2) "a"
3) "jj4"
4) "a"
5) "a"
6) "jj3"
127.0.0.1:6379>
插入元素
LINSERT key <BEFORE | AFTER> pivot element
<BEFORE | AFTER>
:要在指定值pivot
前|后
插入element
element
:要插入的元素pivot
:list 中已存在的数据
Inserts
element
in the list stored atkey
either before or after the reference valuepivot
.When
key
does not exist, it is considered an empty list and no operation is performed.An error is returned when
key
exists but does not hold a list value.
- 在引用值 pivot 之前或之后按键存储的列表中插入元素。
- 如果键不存在,则将其视为空列表,不执行任何操作。
- 如果键存在但不包含列表值,则返回错误。
- 插入一个元素
127.0.0.1:6379> linsert block before jj3 a
(integer) 6
127.0.0.1:6379> lrange block 0 8
1) "jj5"
2) "jj4"
3) "a"
4) "jj3"
5) "jj2"
6) "jj1"
127.0.0.1:6379>
- 在不存在的值前后插入元素
127.0.0.1:6379> linsert block before love me
(integer) -1
127.0.0.1:6379>
减少元素
LTRIM
reduces a list to the specified range of elements.
Trim an existing list so that it will contain only the specified range of elements specified. Both
start
andstop
are zero-based indexes, where0
is the first element of the list (the head),1
the next element and so on.For example:
LTRIM foobar 0 2
will modify the list stored atfoobar
so that only the first three elements of the list will remain.
start
andend
can also be negative numbers indicating offsets from the end of the list, where-1
is the last element of the list,-2
the penultimate element and so on.Out of range indexes will not produce an error: if
start
is larger than the end of the list, orstart > end
, the result will be an empty list (which causeskey
to be removed). Ifend
is larger than the end of the list, Redis will treat it like the last element of the list.
- 修剪现有列表,使其仅包含指定的元素范围。
- Start 和 stop 都是从零开始的索引,其中0是列表的第一个元素(head) ,1是下一个元素,依此类推。
- 例如: LTRIM foobar 02将修改存储在 foobar 中的列表,以便只保留列表的前三个元素。
- Start 和 end 也可以是负数,表示从列表末尾开始的偏移量,其中 -1是列表的最后一个元素,-2是倒数第二个元素,依此类推。
- 超出范围的索引不会产生错误:
- 如果 start 大于列表的末尾,或 start > end,结果将是一个空列表(这将导致删除键)。
- 如果 end 大于列表的末尾,Redis 会将其视为列表的最后一个元素。
127.0.0.1:6379> lrange block 0 100
1) "jj5" # 0
2) "a" # 1
3) "jj4" # 2
4) "a"
5) "a"
6) "jj3" # -3
7) "jj2" #-2
8) "jj1" #-1
127.0.0.1:6379> ltrim block 2 -3
OK
127.0.0.1:6379> lrange block 0 100
1) "jj4"
2) "a"
3) "a"
4) "jj3"
127.0.0.1:6379>
相关文章:
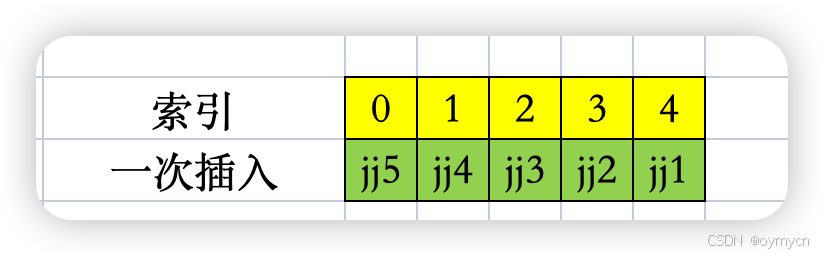
redis数据类型:list
list 的相关命令配合使用的应用场景: 栈和队列:插入和弹出命令的配合,亦可实现栈和队列的功能 实现哪种数据结构,取决于插入和弹出命令的配合,如左插右出或右插左出:这两种种方式实现先进先出的数据结构&a…...

.NET周刊【12月第2期 2024-12-08】
国内文章 终于解决了.net在线客服系统总是被360误报的问题(对软件进行数字签名) https://www.cnblogs.com/sheng_chao/p/18581139 升讯威在线客服与营销系统由.net core和WPF开发,旨在开放、开源、共享。开发者为解决360与其他国产管家的误…...

C#—扩展方法
扩展方法 扩展方法是C#中一种特殊的静态方法,它定义在一个静态类中,但是可以像实例方法一样被调用,使得代码看起来更为直观和易于阅读。扩展方法允许你在不修改原始类的情况下,添加新的方法到现有的类型中。 有↓箭头的是扩展方…...
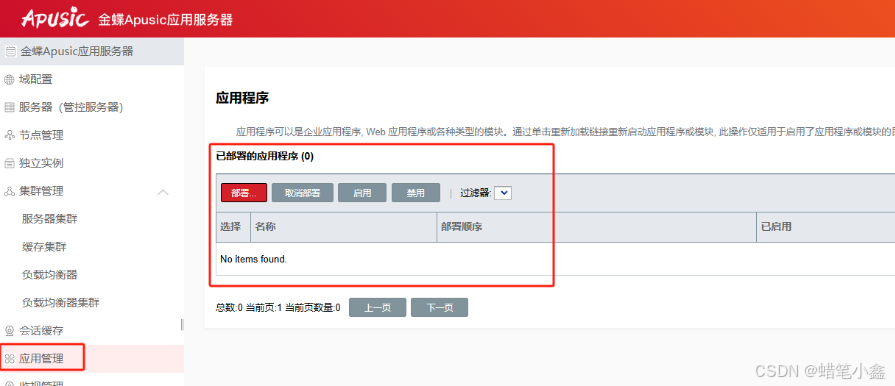
金碟中间件-AAS-V10.0安装
金蝶中间件AAS-V10.0 AAS-V10.0安装 1.解压AAS-v10.0安装包 unzip AAS-V10.zip2.更新license.xml cd /root/ApusicAS/aas# 这里要将license复制到该路径 [rootvdb1 aas]# ls bin docs jmods lib modules templates config domains …...

sql server 查询对象的修改时间
sql server 不能查询索引的最后修改时间,可以查询表,存储过程,函数,pk 的最后修改时间使用以下语句 select * from sys.all_objects ob order by ob.modify_date desc 但可以参考一下统计信息的最后修改时间,因为索…...
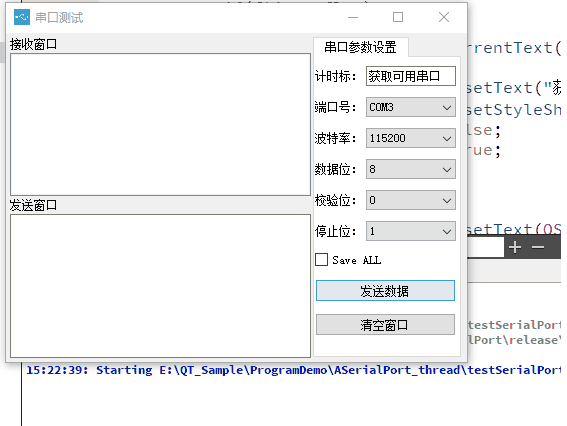
Qt之串口设计-线程实现(十二)
Qt开发 系列文章 - Serial-port(十二) 目录 前言 一、SerialPort 二、实现方式 1.创建类 2.相关功能函数 3.用户使用 4.效果演示 5.拓展应用-实时刷新 总结 前言 Qt作为一个跨平台的应用程序开发框架,在串口编程方面提供了方便易用…...

探索 Seaborn Palette 的奥秘:为数据可视化增色添彩
一、引言 在数据科学的世界里,视觉传达是不可或缺的一环。一个好的数据可视化不仅能传递信息,还能引发共鸣。Seaborn 是 Python 中一款广受欢迎的可视化库,而它的调色板(palette)功能,则为我们提供了调配绚…...

Linux创建普通用户和修改主机名
创建修改用户名和用户组 工作组相关命令 功能命令说明切换用户su username注销用户logout新建用户adduser username 创建用户并分配到用户组useradd -g test username 设置用户密码passwd username查看某一用户w username查看登录用户w查看登陆用户并显示IPwho查看登录历史…...
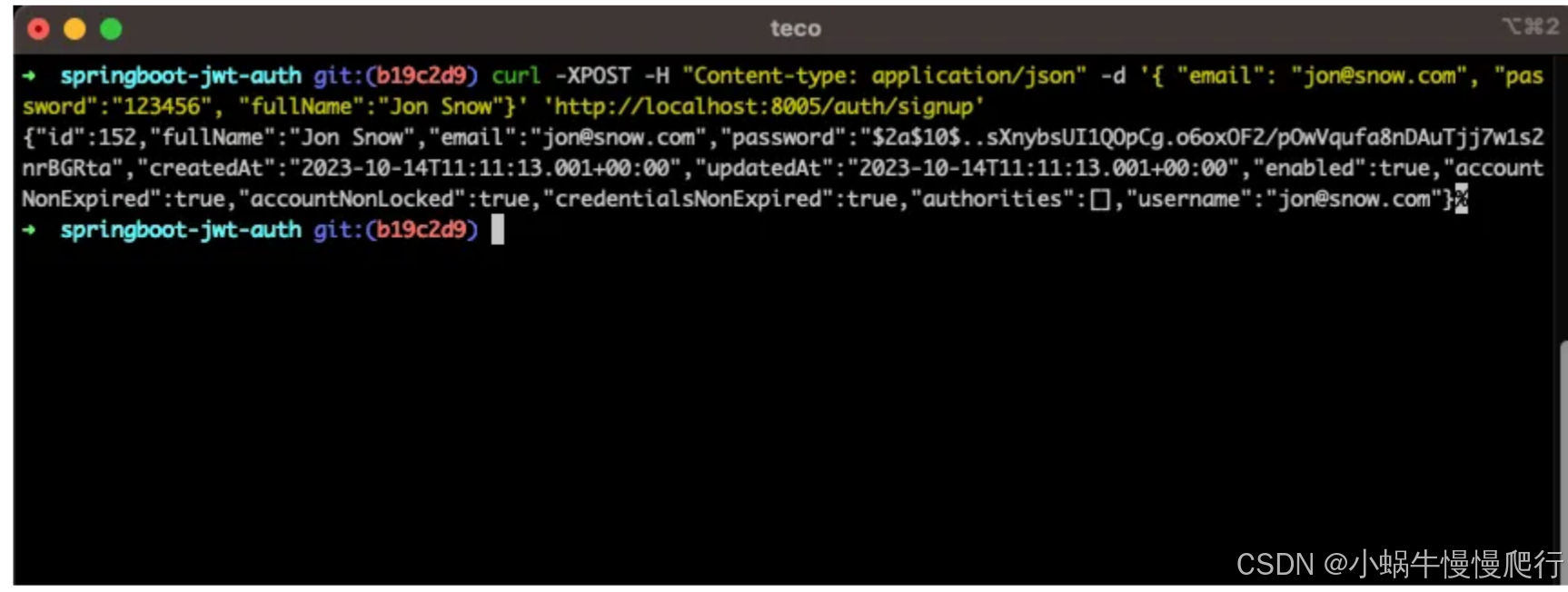
在 Spring Boot 3 中实现基于角色的访问控制
基于角色的访问控制 (RBAC) 是一种有价值的访问控制模型,可增强安全性、简化访问管理并提高效率。它在管理资源访问对安全和运营至关重要的复杂环境中尤其有益。 我们将做什么 我们有一个包含公共路由和受限路由的 Web API。受限路由需要数据库中用户的有效 JWT。 现在用户…...
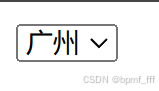
二八(vue2-04)、scoped、data函数、父子通信、props校验、非父子通信(EventBus、provideinject)、v-model进阶
1. 组件的三大组成部分(结构/样式/逻辑) 1.1 scoped 样式冲突 App.vue <template><!-- template 只能有一个根元素 --><div id"app"><BaseOne></BaseOne><BaseTwo></BaseTwo></div> </template><script…...

配置PostgreSQL用于集成测试的步骤
在进行软件开发时,集成测试是确保各个组件能够协同工作的关键环节。PostgreSQL作为一种强大的开源数据库系统,常被用于集成测试中。下面将详细介绍如何在不同的环境中配置PostgreSQL以支持集成测试。 1. 选择并安装PostgreSQL 首先,你需要根…...
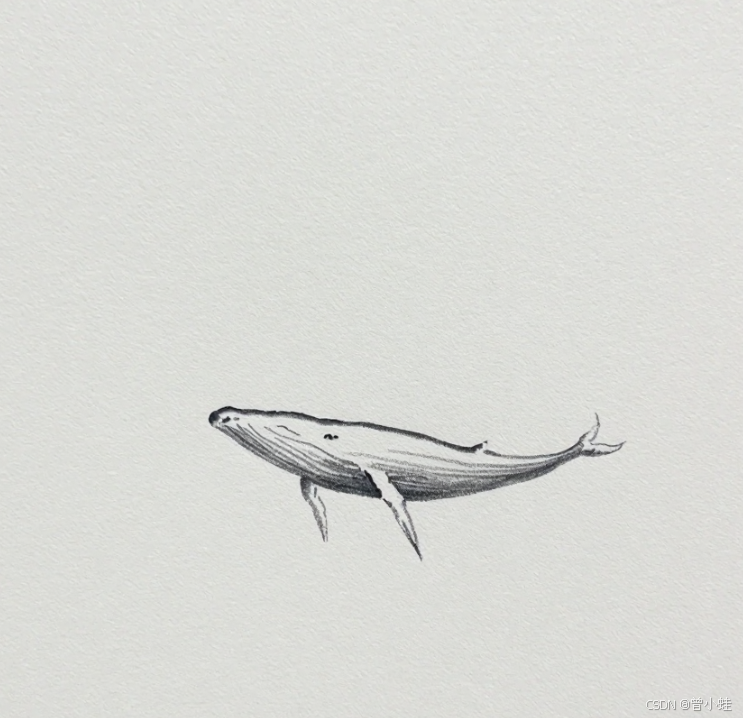
【ComfyUI + 铅笔素描画风】艺术家DaTou发布了的彩色铅笔素描风格生成(真实感超强)
发布时间:2024年12月09日 项目主页:https://hf-mirror.com/Datou1111/shou_xin 基础模型:flux.1-dev comfyui工作流下载:https://pan.baidu.com/s/1FrLQ4o8ldckKwhIrN1Pv7g?pwd1220 自己测试 官方效果 生成猫猫 shou_xin, a m…...
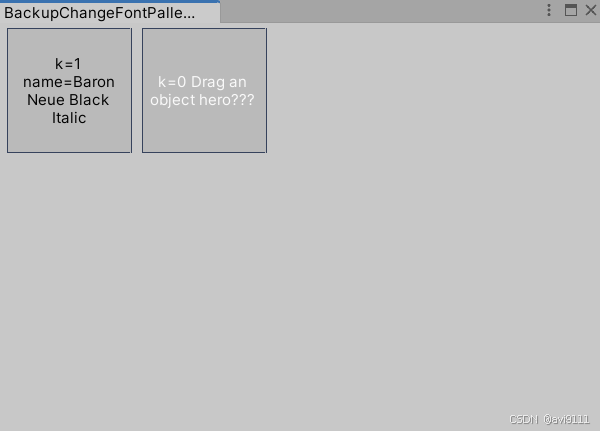
Unity-Editor扩展GUI基本实现一个可拖拉放的格子列表
短短几百行代码,好吧,又是“参考”了国外的月亮 操作,还真地挺自然的。。。。。。国外的实现有点小牛 拖拉,增加+ 一个Element 鼠标左键长按,可以出提示 鼠标右键,清除Element, 有点小bug,不是很自然地完全清除, using System.Collections; using System.Collecti…...
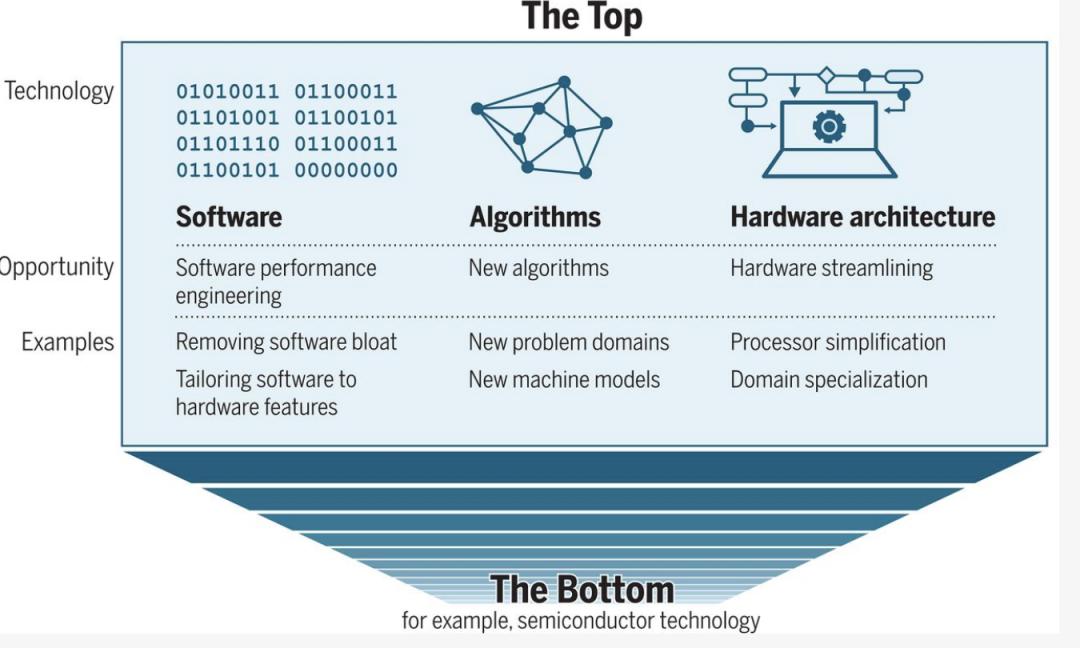
后摩尔定律时代,什么将推动计算机性能优化的发展?
在摩尔定律时代,每两年芯片上的晶体管数量就会翻一番,这一看似不可避免的趋势被称为摩尔定律,它极大地促进了计算机性能的提高。然而,硅基晶体管不可能一直小下去,半导体晶体管的微型化推动了计算机性能的提升…...
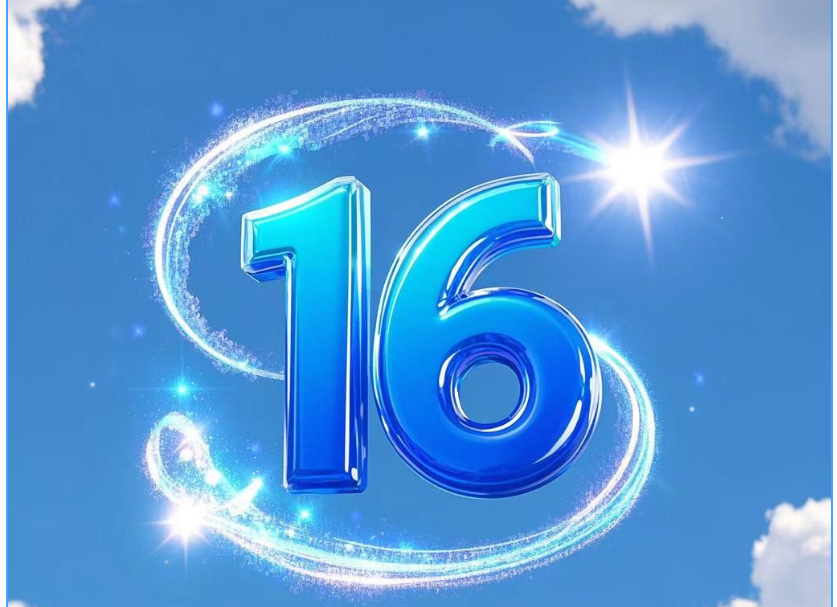
SQL进阶技巧:如何计算商品需求与到货队列表进出计划?
目录 0 需求描述 1 数据准备 2 问题分析 3 小结 累计到货数量计算 出货数量计算 剩余数量计算 0 需求描述 假设现有多种商品的订单需求表 DEMO_REQUIREMENT,以及商品的到货队列表 DEMO_ARR_QUEUE,要求按照业务需要,设计一个报表&#…...
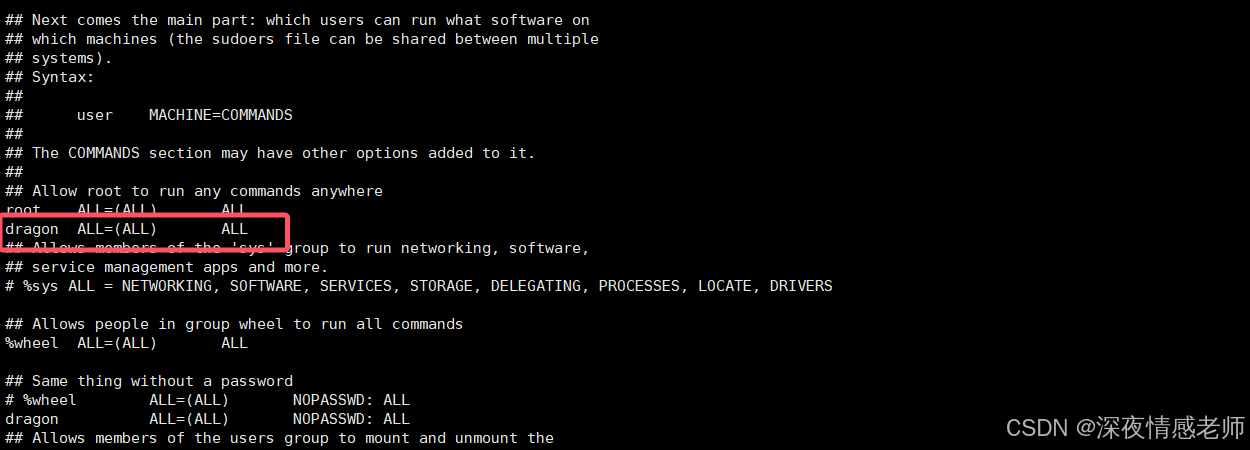
linux普通用户使用sudo不需要输密码
1.root用户如果没有密码,先给root用户设置密码 sudo passwd root #设置密码 2.修改visudo配置 su #切换到root用户下 sudo visudo #修改visudo配置文件 用户名 ALL(ALL) NOPASSWD: ALL #下图所示处新增一行配置 用户名需要输入自己当前主机的用户名...
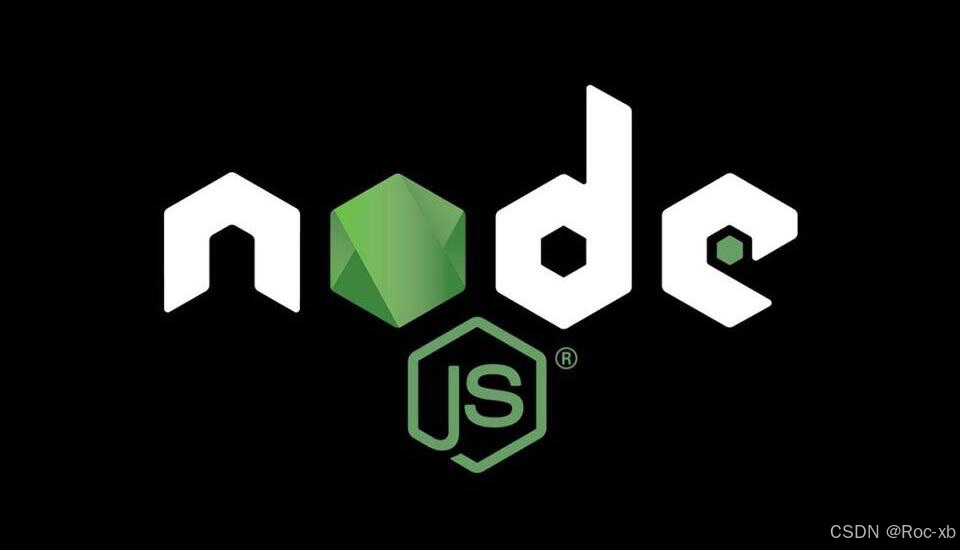
Mac配置 Node镜像源的时候报错解决办法
在Mac电脑中配置国内镜像源的时候报错,提示权限问题,无法写入配置文件。本文提供解决方法,青测有效。 一、原因分析 遇到的错误是由于 .npm 目录下的文件被 root 用户所拥有,导致当前用户无法写入相关配置文件。 二、解决办法 在终端输入以下命令,输入管理员密码即可。 su…...
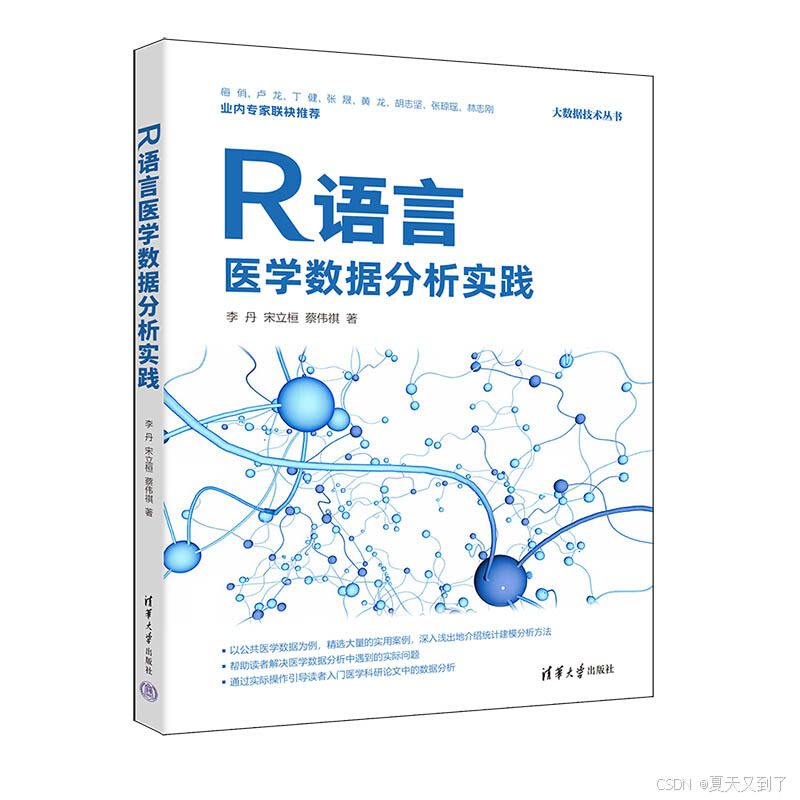
R语言的数据结构-数据框
【图书推荐】《R语言医学数据分析实践》-CSDN博客 《R语言医学数据分析实践 李丹 宋立桓 蔡伟祺 清华大学出版社9787302673484》【摘要 书评 试读】- 京东图书 (jd.com) R语言医学数据分析实践-R语言的数据结构-CSDN博客 在医学领域中,R语言的数据框(…...
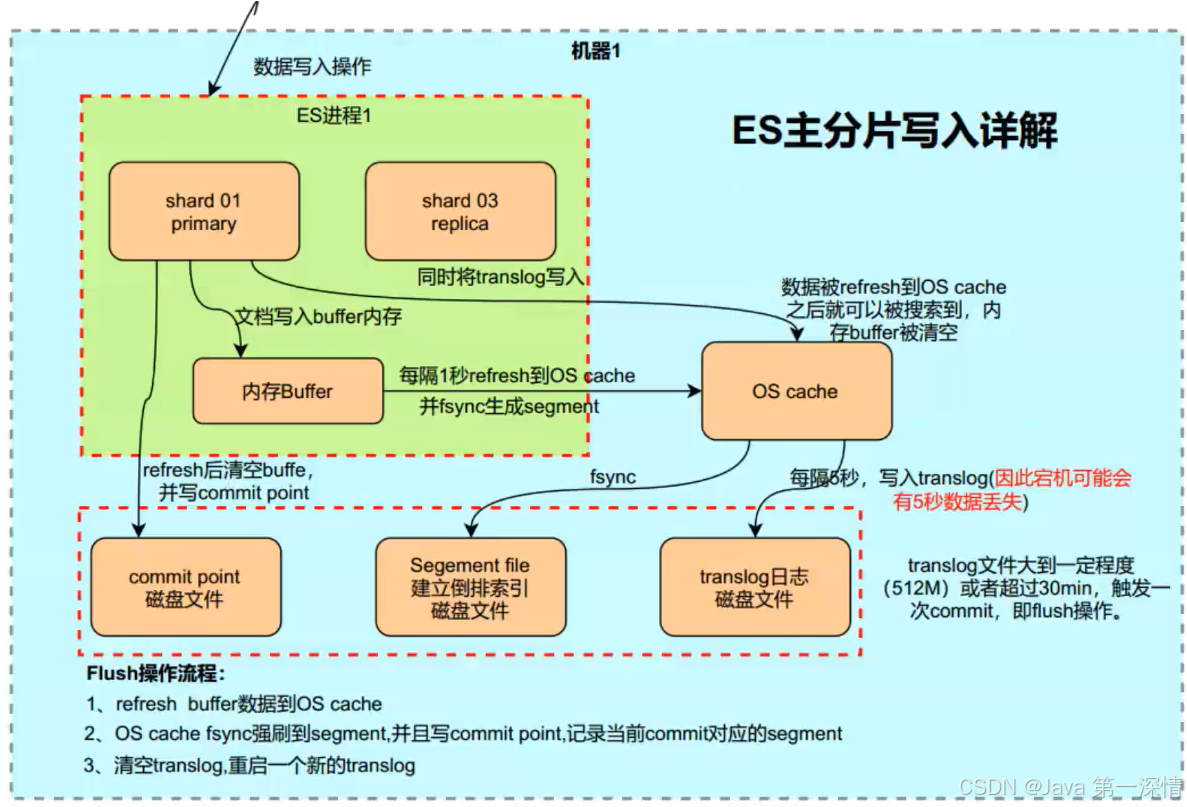
分布式全文检索引擎ElasticSearch-数据的写入存储底层原理
一、数据写入的核心流程 当向 ES 索引写入数据时,整体流程如下: 1、客户端发送写入请求 客户端向 ES 集群的任意节点(称为协调节点,Coordinating Node)发送一个写入请求,比如 index(插入或更…...
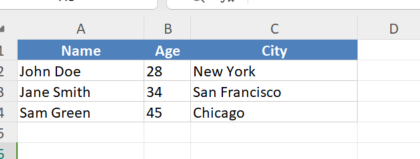
react中实现导出excel文件
react中实现导出excel文件 一、安装依赖二、实现导出功能三、自定义列标题四、设置列宽度五、样式优化1、安装扩展库2、设置样式3、扩展样式功能 在 React 项目中实现点击按钮后导出数据为 Excel 文件,可以使用 xlsx 和 file-saver 这两个库。 一、安装依赖 在项目…...
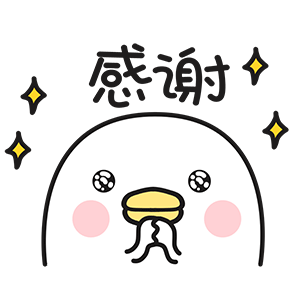
有监督学习 vs 无监督学习:机器学习的两大支柱
有监督学习 vs 无监督学习:机器学习的两大支柱 有监督学习 vs 无监督学习:机器学习的两大支柱一、有无“老师”来指导二、解决的问题类型不同三、模型的输出不同 有监督学习 vs 无监督学习:机器学习的两大支柱 在机器学习的奇妙世界里&#…...
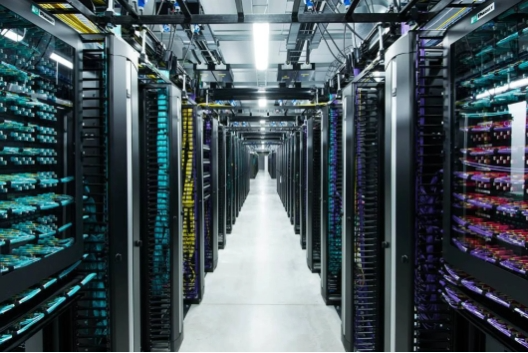
c4d动画怎么导出mp4视频,c4d动画视频格式设置
宝子们,今天来给大家讲讲 C4D 咋导出mp4视频的方法。通过用图文教程的形式给大家展示得明明白白的,让你能轻松理解和掌握,不管是理论基础,还是实际操作和技能技巧,都能学到,快速入门然后提升自己哦。 c4d动…...
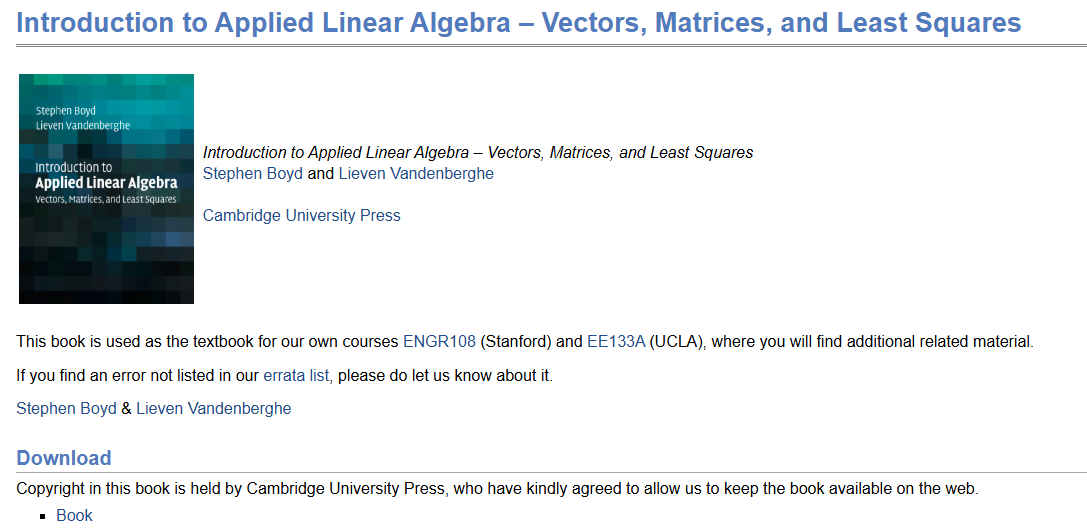
差分矩阵(Difference Matrix)与累计和矩阵(Running Sum Matrix)的概念与应用:中英双语
本文是学习这本书的笔记: https://web.stanford.edu/~boyd/vmls/ 差分矩阵(Difference Matrix)与累计和矩阵(Running Sum Matrix)的概念与应用 在线性代数和信号处理等领域中,矩阵运算常被用来表示和计算各种数据变换…...

全面解析 Golang Gin 框架
1. 引言 在现代 Web 开发中,随着需求日益增加,开发者需要选择合适的工具来高效地构建应用程序。对于 Go 语言(Golang)开发者来说,Gin 是一个备受青睐的 Web 框架。它轻量、性能高、易于使用,并且具备丰富的…...

全脐点曲面当且仅当平面或者球面的一部分
S 是全脐点曲面当且仅当 S 是平面或者球面的一部分。 S_\text{ 是全脐点曲面当且仅当 }{S_\text{ 是平面或者球面的一部分。}} S 是全脐点曲面当且仅当 S 是平面或者球面的一部分。 证: 充分性显然,下证必要性。 若 r ( u , v ) r(u,v) r(u,v)是…...
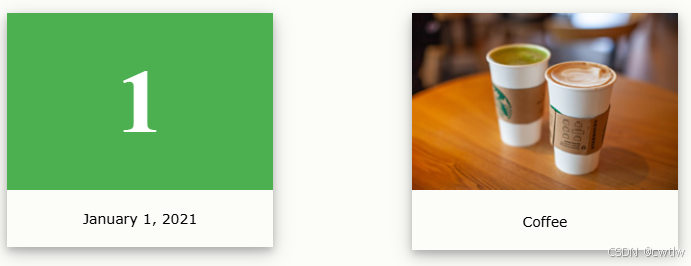
CSS学习记录18
CSS渐变 CSS渐变您可以显示两种或多种指定颜色之间的平滑过渡。 CSS定义了两种渐变类型: 线性渐变(向下/向上/向左/向右/对角线)径向渐变(由其中心定义) CSS线性渐变 如需创建线性渐变,您必须至少两个色…...

实验13 C语言连接和操作MySQL数据库
一、安装MySQL 1、使用包管理器安装MySQL sudo apt update sudo apt install mysql-server2、启动MySQL服务: sudo systemctl start mysql3、检查MySQL服务状态: sudo systemctl status mysql二、安装MySQL开发库 sudo apt-get install libmysqlcli…...
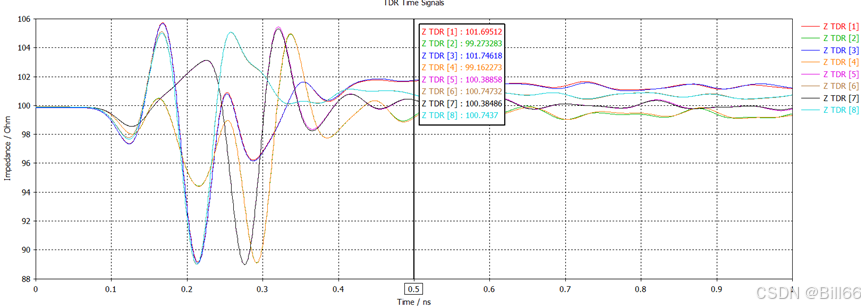
90度Floating B to B 高速连接器信号完整性仿真
在180度 B to B Connector 信号完整性仿真时,不会碰到端口设置不方便问题,但在做90度B to B Connector信号完整性仿真时就会碰到端口设置问题。如下面的90度B to B Connector。 公座 母座 公母对插后如下: 客户要求改Connector需符合PCI-E3.…...
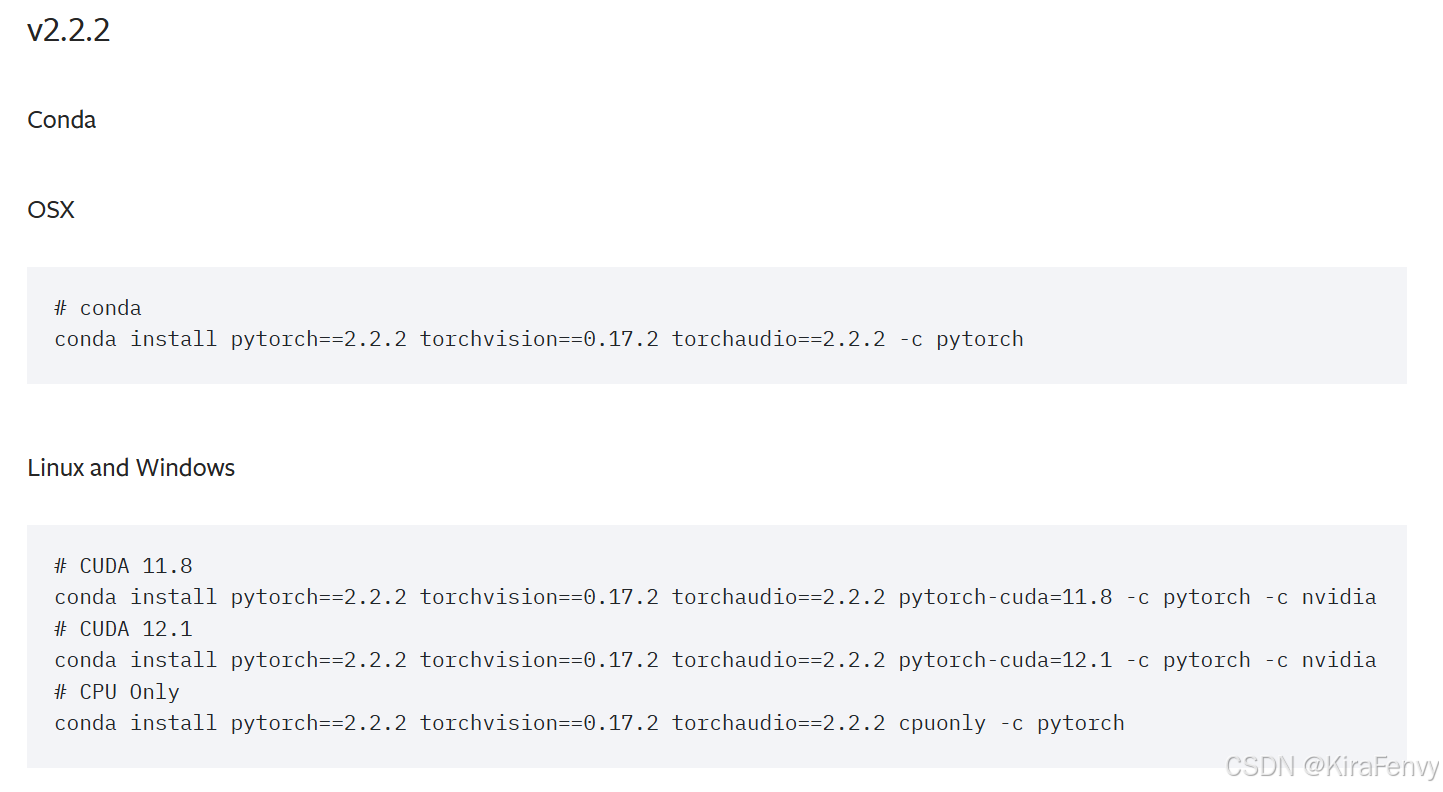
【踩坑】Pytorch与CUDA版本的关系及安装
Pytorch、CUDA和CUDA Toolkit区分 查看当前环境常用shell命令python脚本 Driver API CUDA(nvidia-smi)Runtime API CUDA(nvcc --version)pytorch选择CUDA版本的顺序安装需要的CUDA,多版本共存和自由切换 本文参考 http…...

信息隐藏 数字图像空域隐写与分析技术的实现
数字图像隐写与分析 摘要 随着信息技术的发展,隐写术作为一种信息隐藏技术,越来越受到关注。本文介绍了一种基于最低有效位(LSB)方法的数字图像隐写技术,并实现了隐写数据的嵌入与提取。通过卡方检验分析隐写图像的统计特性,评估隐写数据对图像的影响。实验结果表明,该…...