Mybatis引出的一系列问题-spring多数据源配置
在日常开发中我们都是以单个数据库进行开发,在小型项目中是完全能够满足需求的。但是,当我们牵扯到像淘宝、京东这样的大型项目的时候,单个数据库就难以承受用户的CRUD操作。那么此时,我们就需要使用多个数据源进行读写分离的操作,这种方式也是目前一种流行的数据管理方式。
1 Spring Boot配置多数据源
在YAML文件中定义数据源所需的数据:
spring:datasource:type: com.alibaba.druid.pool.DruidDataSourcedefault-datasource:username: rootpassword: rooturl: jdbc:mysql://localhost:3306/default?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8driver-class-name: com.mysql.jdbc.Driverinspur-zs-datasource:username: rootpassword: rooturl: jdbc:mysql://localhost:3306/inspur-zs?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8driver-class-name: com.mysql.jdbc.Driverdruid:initial-size: 5min-idle: 1max-active: 20profiles:active: devmybatis:mapper-locations: classpath:/mapper/*.xmltype-aliases-package: com.inspur.pojoconfiguration:mapUnderscoreToCamelCase: truelog-impl: org.apache.ibatis.logging.slf4j.Slf4jImpl
定义多个数据源:
package com.inspur.spring.config.datasource;import com.alibaba.druid.spring.boot.autoconfigure.DruidDataSourceBuilder;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;import javax.sql.DataSource;/*** 数据源配置* ConfigurationProperties注解用于将YAML中指定的数据创建成指定的对象,* 但是,YAML中的数据必须要与对象对象中的属性同名,不然无法由Spring Boot完成赋值。** @author zhaoshuai-lc* @date 2023/07/11*/
@Configuration
public class DataSourceConfig {@Bean(name = "defaultDatasource")@ConfigurationProperties(prefix = "spring.datasource.default-datasource")public DataSource defaultDatasource() {return DruidDataSourceBuilder.create().build();}@Bean(name = "inspurZsDatasource")@ConfigurationProperties(prefix = "spring.datasource.inspur-zs-datasource")public DataSource inspurZsDatasource() {return DruidDataSourceBuilder.create().build();}
}
由于我们要定义多个数据源,所以在Spring Boot数据源自动配置类中就无法确定导入哪个数据源来完成初始化,所以我们就需要禁用掉Spring Boot的数据源自动配置类,然后使用我们自定义的数据源配置类来完成数据源的初始化与管理。
package com.inspur;import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;/*** WtMybatisStudyApplication* 由于我们要定义多个数据源,所以在Spring Boot数据源自动配置类中就无法确定导入哪个数据源来完成初始化,* 所以我们就需要禁用掉Spring Boot的数据源自动配置类,然后使用我们自定义的数据源配置类来完成数据源的初始化与管理。** @author zhaoshuai-lc* @date 2023/07/11*/
@SpringBootApplication(exclude = {DataSourceAutoConfiguration.class})
@EnableAspectJAutoProxy
public class WtMybatisStudyApplication {public static void main(String[] args) {SpringApplication.run(WtMybatisStudyApplication.class, args);}
}
1.1 指定数据源-实现DataSource接口
缺点:产生大量的代码冗余,在代码中存在硬编码。
package com.inspur.spring.config.datasource;import org.springframework.context.annotation.Primary;
import org.springframework.stereotype.Component;import javax.annotation.Resource;
import javax.sql.DataSource;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
import java.util.logging.Logger;/*** 方式一* DynamicDataSource1* 实现DataSource接口我们本质上只使用了一个方法就是getConnection()这个无参的方法** @author zhaoshuai-lc* @date 2023/07/11*/
@Component
@Primary
public class DynamicDataSource1 implements DataSource {public static ThreadLocal<String> flag = new ThreadLocal<>();@Resourceprivate DataSource defaultDatasource;@Resourceprivate DataSource inspurZsDatasource;public DynamicDataSource1() {flag.set("defaultDatasource");}@Overridepublic Connection getConnection() throws SQLException {if (flag.get().equals("defaultDatasource")) {return defaultDatasource.getConnection();} else if (flag.get().equals("inspurZsDatasource")) {return inspurZsDatasource.getConnection();}return defaultDatasource.getConnection();}@Overridepublic Connection getConnection(String username, String password) throws SQLException {return null;}@Overridepublic <T> T unwrap(Class<T> iface) throws SQLException {return null;}@Overridepublic boolean isWrapperFor(Class<?> iface) throws SQLException {return false;}@Overridepublic PrintWriter getLogWriter() throws SQLException {return null;}@Overridepublic void setLogWriter(PrintWriter out) throws SQLException {}@Overridepublic void setLoginTimeout(int seconds) throws SQLException {}@Overridepublic int getLoginTimeout() throws SQLException {return 0;}@Overridepublic Logger getParentLogger() throws SQLFeatureNotSupportedException {return null;}
}
实现DataSource接口我们本质上只使用了一个方法,就是getConnection()这个无参的方法,但是DataSource接口中所有的方法我们也都需要实现,只是不用写方法体而已,也就是存在了很多的 “废方法” 。
@Primary注解 == @Order(1),用于设置此类的注入顺序。
使用:
@Overridepublic PageData<BsFactoryCalendar> selectByExample(BsFactoryCalendarExample example) {PageData<BsFactoryCalendar> pageData = new PageData<>();DynamicDataSource1.flag.set("default-datasource");List<BsFactoryCalendar> bsFactoryCalendars = bsFactoryCalendarMapper.selectByExample(example);PageInfo<BsFactoryCalendar> pageInfo = new PageInfo<>(bsFactoryCalendars);pageData.setRows(bsFactoryCalendars);pageData.setTotal(pageInfo.getTotal());return pageData;}
1.2 指定数据源-继承AbstrictRoutingDataSource类
减少了代码的冗余,但是还是会存在硬编码。
package com.inspur.spring.config.datasource;import cn.hutool.core.map.MapUtil;
import org.springframework.context.annotation.Primary;
import org.springframework.jdbc.datasource.lookup.AbstractRoutingDataSource;
import org.springframework.stereotype.Component;import javax.annotation.Resource;
import javax.sql.DataSource;
import java.util.Map;/*** DynamicDataSource2* AbstrictRoutingDataSource的本质就是利用一个Map将数据源存储起来,然后通过Key来得到Value来修改数据源。** @author zhaoshuai-lc* @date 2023/07/11*/@Component
@Primary
public class DynamicDataSource2 extends AbstractRoutingDataSource {public static ThreadLocal<String> flag = new ThreadLocal<>();@Resourceprivate DataSource defaultDatasource;@Resourceprivate DataSource inspurZsDatasource;public DynamicDataSource2() {flag.set("defaultDatasource");}@Overrideprotected Object determineCurrentLookupKey() {return flag.get();}@Overridepublic void afterPropertiesSet() {Map<Object, Object> targetDataSource = MapUtil.newConcurrentHashMap();// 将第一个数据源设置为默认的数据源super.setDefaultTargetDataSource(defaultDatasource);targetDataSource.put("defaultDatasource", defaultDatasource);targetDataSource.put("inspurZsDatasource", inspurZsDatasource);super.setTargetDataSources(targetDataSource);super.afterPropertiesSet();}
}
AbstrictRoutingDataSource的本质就是利用一个Map将数据源存储起来,然后通过Key来得到Value来修改数据源。
使用:
@Overridepublic PageData<BsFactoryCalendar> selectByExample(BsFactoryCalendarExample example) {PageData<BsFactoryCalendar> pageData = new PageData<>();DynamicDataSource2.flag.set("default-datasource");List<BsFactoryCalendar> bsFactoryCalendars = bsFactoryCalendarMapper.selectByExample(example);PageInfo<BsFactoryCalendar> pageInfo = new PageInfo<>(bsFactoryCalendars);pageData.setRows(bsFactoryCalendars);pageData.setTotal(pageInfo.getTotal());return pageData;}
1.3 指定数据源-使用Spring AOP + 自定义注解的形式
Spring AOP + 自定义注解的形式是一种推荐的写法,减少代码的冗余且不存在硬编码。此方法适合对指定功能操作指定数据库的模式。
导入依赖:
<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-aop</artifactId>
</dependency>
开启AOP支持:
package com.inspur;import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;@SpringBootApplication(exclude = {DataSourceAutoConfiguration.class})
@EnableAspectJAutoProxy
public class WtMybatisStudyApplication {public static void main(String[] args) {SpringApplication.run(WtMybatisStudyApplication.class, args);}}
定义枚举来表示数据源的标识:
package com.inspur.spring.config.datasource.enums;public enum DataSourceType {DEFAULT_DATASOURCE,INSPURZS_DATASOURCE,
}
继承AbstractRoutingDataSource类:
package com.inspur.spring.config.datasource;import cn.hutool.core.map.MapUtil;
import com.inspur.spring.config.datasource.enums.DataSourceType;
import org.springframework.context.annotation.Primary;
import org.springframework.jdbc.datasource.lookup.AbstractRoutingDataSource;
import org.springframework.stereotype.Component;import javax.annotation.Resource;
import javax.sql.DataSource;
import java.util.Map;@Primary
@Component
public class DynamicDataSource3 extends AbstractRoutingDataSource {public static ThreadLocal<String> flag = new ThreadLocal<>();@Resourceprivate DataSource defaultDatasource;@Resourceprivate DataSource inspurZsDatasource;public DynamicDataSource3() {flag.set(DataSourceType.DEFAULT_DATASOURCE.name());}@Overrideprotected Object determineCurrentLookupKey() {return flag.get();}@Overridepublic void afterPropertiesSet() {Map<Object, Object> targetDataSource = MapUtil.newConcurrentHashMap();// 将第一个数据源设置为默认的数据源super.setDefaultTargetDataSource(defaultDatasource);targetDataSource.put(DataSourceType.DEFAULT_DATASOURCE.name(), defaultDatasource);targetDataSource.put(DataSourceType.INSPURZS_DATASOURCE.name(), inspurZsDatasource);super.setTargetDataSources(targetDataSource);super.afterPropertiesSet();}
}
自定义注解:
package com.inspur.spring.config.datasource.annotation;import com.inspur.spring.config.datasource.enums.DataSourceType;import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;@Target({ElementType.TYPE, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface TargetDataSource {DataSourceType value() default DataSourceType.DEFAULT_DATASOURCE;
}
定义注解的实现类:
package com.inspur.spring.config.datasource.annotation;import com.inspur.spring.config.datasource.DynamicDataSource3;
import lombok.extern.slf4j.Slf4j;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.stereotype.Component;import java.lang.reflect.Method;/*** TargetDataSourceAspect** @author zhaoshuai-lc* @date 2023/07/11*/
@Component
@Aspect
@Slf4j
public class TargetDataSourceAspect {@Before("@within(com.inspur.spring.config.datasource.annotation.TargetDataSource) || " +"@annotation(com.inspur.spring.config.datasource.annotation.TargetDataSource)")public void beforeNoticeUpdateDataSource(JoinPoint joinPoint) {TargetDataSource annotation = null;Class<? extends Object> target = joinPoint.getTarget().getClass();if (target.isAnnotationPresent(TargetDataSource.class)) {// 判断类上是否标注着注解annotation = target.getAnnotation(TargetDataSource.class);log.info("类: {}, 标注了注解@TargetDataSource", target);} else {Method method = ((MethodSignature) joinPoint.getSignature()).getMethod();if (method.isAnnotationPresent(TargetDataSource.class)) {// 判断方法上是否标注着注解,如果类和方法上都没有标注,则报错annotation = method.getAnnotation(TargetDataSource.class);log.info("方法: {}, 标注了注解@TargetDataSource", method);} else {log.error("注解@TargetDataSource只能用于类或者方法上, error: {} {}", target, method);throw new RuntimeException("注解@TargetDataSource使用错误");}}// 切换数据源DynamicDataSource3.flag.set(annotation.value().name());}
}
使用:
package com.inspur.spring.service;import com.github.pagehelper.PageInfo;
import com.inspur.spring.common.interfaceResult.PageData;
import com.inspur.spring.config.datasource.annotation.TargetDataSource;
import com.inspur.spring.config.datasource.enums.DataSourceType;
import com.inspur.spring.dao.BsFactoryCalendarMapper;
import com.inspur.spring.pojo.BsFactoryCalendar;
import com.inspur.spring.pojo.BsFactoryCalendarExample;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;import javax.annotation.Resource;
import java.util.List;@Service
@TargetDataSource(value = DataSourceType.DEFAULT_DATASOURCE) // 方式三 多数据源设置
public class BsFactoryCalendarServiceImpl implements BsFactoryCalendarService {@Resourceprivate BsFactoryCalendarMapper bsFactoryCalendarMapper;@Override@Transactionalpublic PageData<BsFactoryCalendar> selectByExample(BsFactoryCalendarExample example) {PageData<BsFactoryCalendar> pageData = new PageData<>();List<BsFactoryCalendar> bsFactoryCalendars = bsFactoryCalendarMapper.selectByExample(example);PageInfo<BsFactoryCalendar> pageInfo = new PageInfo<>(bsFactoryCalendars);pageData.setRows(bsFactoryCalendars);pageData.setTotal(pageInfo.getTotal());return pageData;}
}
1.4 通过SqlSessionFactory指定的数据源来操作指定目录的XML文件
使用此方法则不会与上面所述的类有任何关系,本方法会重新定义类。本方法也是一种推荐的方法,适用于对指定数据库的操作,也就是适合读写分离。不会存在代码冗余和存在硬编码。
配置YAML文件:
spring:datasource:type: com.alibaba.druid.pool.DruidDataSourcedefault-datasource:username: rootpassword: rootjdbc-url: jdbc:mysql://localhost:3306/default?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8driver-class-name: com.mysql.jdbc.Driverinspur-zs-datasource:username: rootpassword: rootjdbc-url: jdbc:mysql://localhost:3306/inspur-zs?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8driver-class-name: com.mysql.jdbc.Drivermain:allow-bean-definition-overriding : truedruid:initial-size: 5min-idle: 1max-active: 20profiles:active: devmybatis:mapper-locations: classpath:/mapper/*.xmltype-aliases-package: com.inspur.pojoconfiguration:mapUnderscoreToCamelCase: truelog-impl: org.apache.ibatis.logging.slf4j.Slf4jImpl
针对Mapper层通过SqlSessionFactory指定数据源来操作:
package com.inspur.spring.config.datasource;import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.mybatis.spring.SqlSessionTemplate;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.jdbc.DataSourceBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import org.springframework.jdbc.datasource.DataSourceTransactionManager;
import org.springframework.transaction.PlatformTransactionManager;import javax.sql.DataSource;@Configuration
@MapperScan(basePackages = "com.inspur.spring.dao.defaultzs", sqlSessionFactoryRef = "DefaultSqlSessionFactory")
public class DefaultDatasourceConfig {@Primary@Bean(name = "DefaultDatasource")@ConfigurationProperties(prefix = "spring.datasource.default-datasource")public DataSource getDateSource1() {return DataSourceBuilder.create().build();}@Primary@Bean(name = "DefaultSqlSessionFactory")public SqlSessionFactory sqlSessionFactory(@Qualifier("DefaultDatasource") DataSource datasource) throws Exception {SqlSessionFactoryBean bean = new SqlSessionFactoryBean();bean.setDataSource(datasource);// 设置mybatis的xml所在位置bean.setMapperLocations(new PathMatchingResourcePatternResolver().getResources("classpath*:mapper/defaultzs/*.xml"));return bean.getObject();}@Bean("DefaultSqlSessionTemplate")@Primarypublic SqlSessionTemplate sqlSessionTemplate(@Qualifier("DefaultSqlSessionFactory") SqlSessionFactory factory) {return new SqlSessionTemplate(factory);}@Beanpublic PlatformTransactionManager transactionManager(@Qualifier("DefaultDatasource") DataSource dataSource) {return new DataSourceTransactionManager(dataSource);}
}
package com.inspur.spring.config.datasource;import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.mybatis.spring.SqlSessionTemplate;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.jdbc.DataSourceBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import org.springframework.jdbc.datasource.DataSourceTransactionManager;
import org.springframework.transaction.PlatformTransactionManager;import javax.sql.DataSource;@Configuration
@MapperScan(basePackages = "com.inspur.spring.dao.inspurzs", sqlSessionFactoryRef = "InspurZsSqlSessionFactory")
public class InspurZsDatasourceConfig {@Primary@Bean(value = "InspurZsDatasource")@ConfigurationProperties(prefix = "spring.datasource.inspur-zs-datasource")public DataSource getDateSource1() {return DataSourceBuilder.create().build();}@Primary@Bean(value = "InspurZsSqlSessionFactory")public SqlSessionFactory sqlSessionFactory(@Qualifier("InspurZsDatasource") DataSource datasource) throws Exception {SqlSessionFactoryBean bean = new SqlSessionFactoryBean();bean.setDataSource(datasource);// 设置mybatis的xml所在位置bean.setMapperLocations(new PathMatchingResourcePatternResolver().getResources("classpath*:mapper/inspurzs/*.xml"));return bean.getObject();}@Bean(value = "InspurZsSqlSessionTemplate")@Primarypublic SqlSessionTemplate sqlSessionTemplate(@Qualifier("InspurZsSqlSessionFactory") SqlSessionFactory factory) {return new SqlSessionTemplate(factory);}@Beanpublic PlatformTransactionManager transactionManager(@Qualifier("InspurZsDatasource") DataSource dataSource) {return new DataSourceTransactionManager(dataSource);}
}
@MapperScan注解中的basePackages指向的是指定的Dao层。
@MapperScan注解中sqlSessionFactoryRef 用来指定使用某个SqlSessionFactory来操作数据源。
bean.setMapperLocations(new PathMatchingResourcePatternResolver().getResources("classpath*:mapper/inspurzs/*.xml"));
使用此种方法不会存在任何代码的冗余以及硬编码的存在,但是需要分层明确。唯一的不足就是添加一个数据源就需要重新写一个类,而这个类中的代码大部分又是相同的。
相关文章:
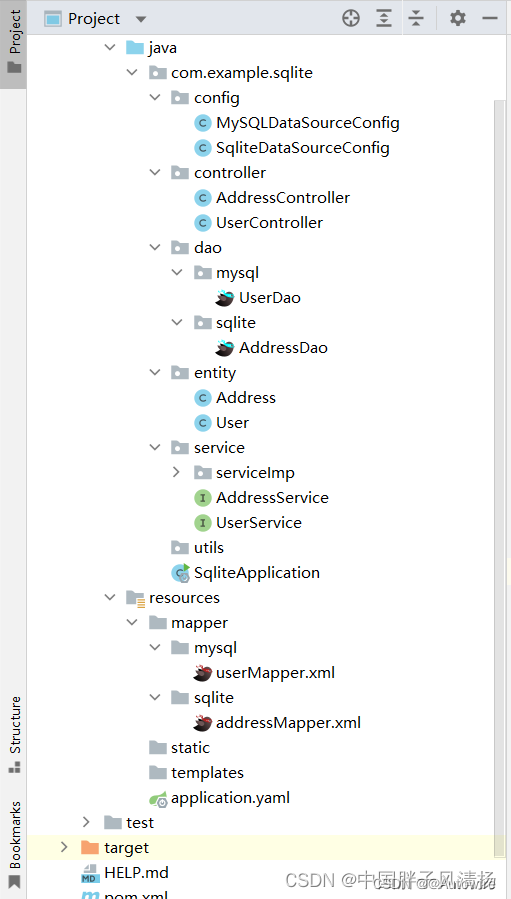
Mybatis引出的一系列问题-spring多数据源配置
在日常开发中我们都是以单个数据库进行开发,在小型项目中是完全能够满足需求的。但是,当我们牵扯到像淘宝、京东这样的大型项目的时候,单个数据库就难以承受用户的CRUD操作。那么此时,我们就需要使用多个数据源进行读写分离的操作…...
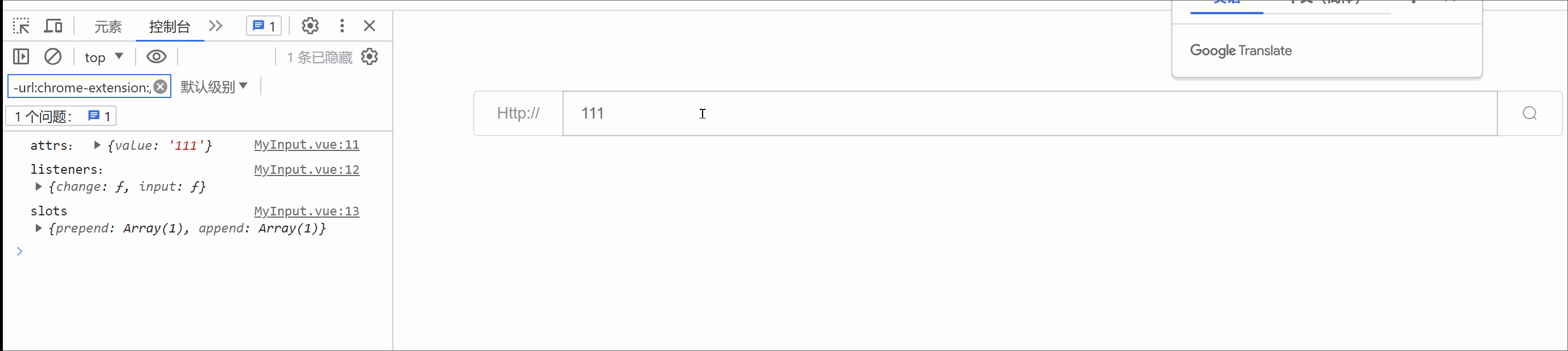
Vue-组件二次封装
本次对el-input进行简单封装进行演示 封装很简单,就给激活样式的边框(主要是功能) 本次封装主要使用到vue自带的几个对象 $attrs:获取绑定在组件上的所有属性$listeners: 获取绑定在组件上的所有函数方法$slots: 获取应用在组件内的所有插槽 …...
[C++]02.选择结构与循环结构
02.选择结构与循环结构 一.程序流程结构1.选择结构1.1.if语句1.2.三目运算符1.3.switch语句 2.循环结构2.1.while语句2.2.do-while语句2.3.for语句2.4.break语句2.5.continue语句2.6.goto语句 一.程序流程结构 C/C支持的最基本的运行结构: 顺序结构, 选择结构, 循环结构顺序结…...
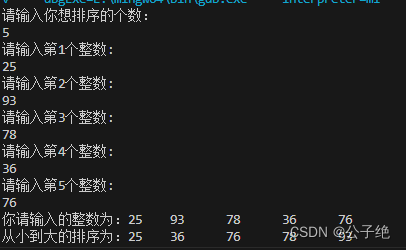
C语言案例 按序输出多个整数-03
难度2复杂度3 题目:输入多个整数,按从小到大的顺序输出 步骤一:定义程序的目标 编写一个C程序,随机输入整数,按照从小到大的顺序输出 步骤二:程序设计 整个C程序由三大模块组成,第一个模块使…...
如何获取vivado IP列表
TCL命令如下: set fid [open "vivado_included_ip_[version -short].csv" w] puts $fid "Name;Version" set ip_catalog [get_ipdefs *] foreach ip $ip_catalog{ set ipname [get_property DISPLAY_NAME [get_ipdefs $ip]]set iplib [get_p…...
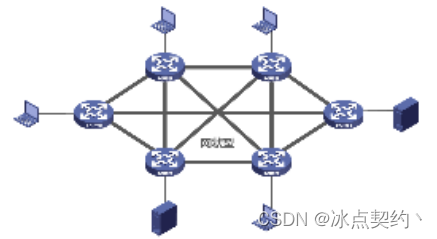
计算机网络的定义和分类
计算机网络的定义和分类 计算机网络的定义 计算机网络的精确定义并未统一计算机网络最简单的定义是:一些互相连接的、自治的计算机的集合 互连:指计算机之间可以通过有线或无线的方式进行数据通信自治:是指独立的计算机,它有自己的硬件和软件ÿ…...
【css】超过文本显示省略号
显示省略号的前提:必须有指定宽度 一、单行文本超出部分显示省略号 属性取值解释overflowhidden当内容超过盒子宽度, 隐藏溢出部分white-spacenowrap让文字在一行内显示, 不换行text-overflowellipsis如果溢出的内容是文字, 就用省略号代替 .one-line{overflow:h…...
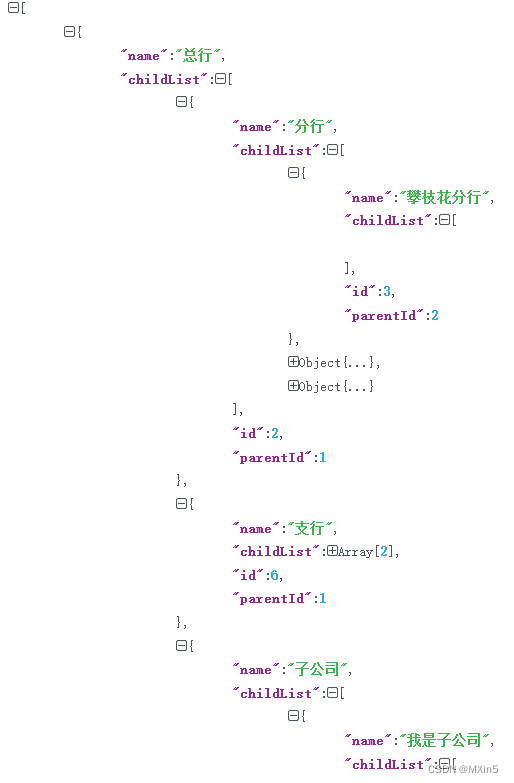
Java 8 中使用 Stream 遍历树形结构
在实际开发中,我们经常会开发菜单,树形结构,数据库一般就使用父id来表示,为了降低数据库的查询压力,我们可以使用Java8中的Stream流一次性把数据查出来,然后通过流式处理,我们一起来看看&#x…...
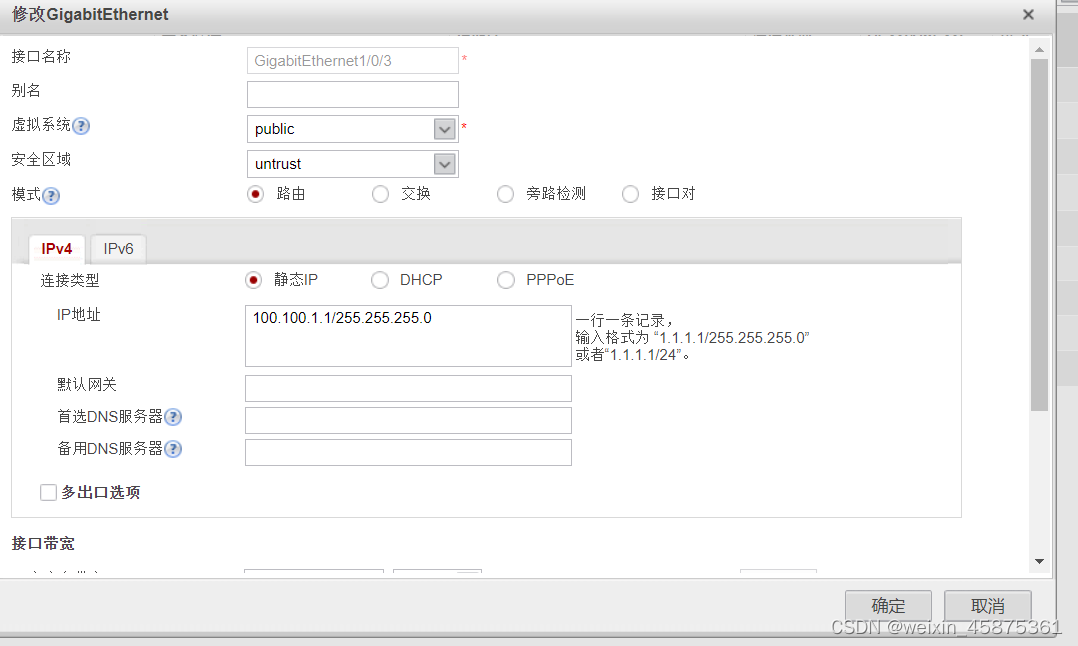
网络安全防火墙体验实验
网络拓扑 实验操作: 1、cloud配置 2、防火墙配置 [USG6000V1]int GigabitEthernet 0/0/0 [USG6000V1-GigabitEthernet0/0/0]ip add 192.168.200.100 24 打开防火墙的所有服务 [USG6000V1-GigabitEthernet0/0/0]service-manage all permit 3、进入图形化界面配置…...

YOLOv5引入FasterNet主干网络,目标检测速度提升明显
目录 一、背景介绍1.1 目标检测算法简介1.2 YOLOv5简介及发展历程 二、主干网络选择的重要性2.1 主干网络在目标检测中的作用2.2 YOLOv5使用的默认主干网络 三、FasterNet简介与原理解析3.1 FasterNet概述3.2 FasterNet的网络结构3.2.1 基础网络模块3.2.2 快速特征融合模块3.2.…...
SpringBoot运行时注入一个Bean
描述 使用GenericApplicationContext类的registerBean方法可以在项目运行时注入一个bean,获取GenericApplicationContext可以继承ApplicationContextAware,重写setApplicationContext,里面的参数就是ApplicationContext。 继承ApplicationC…...
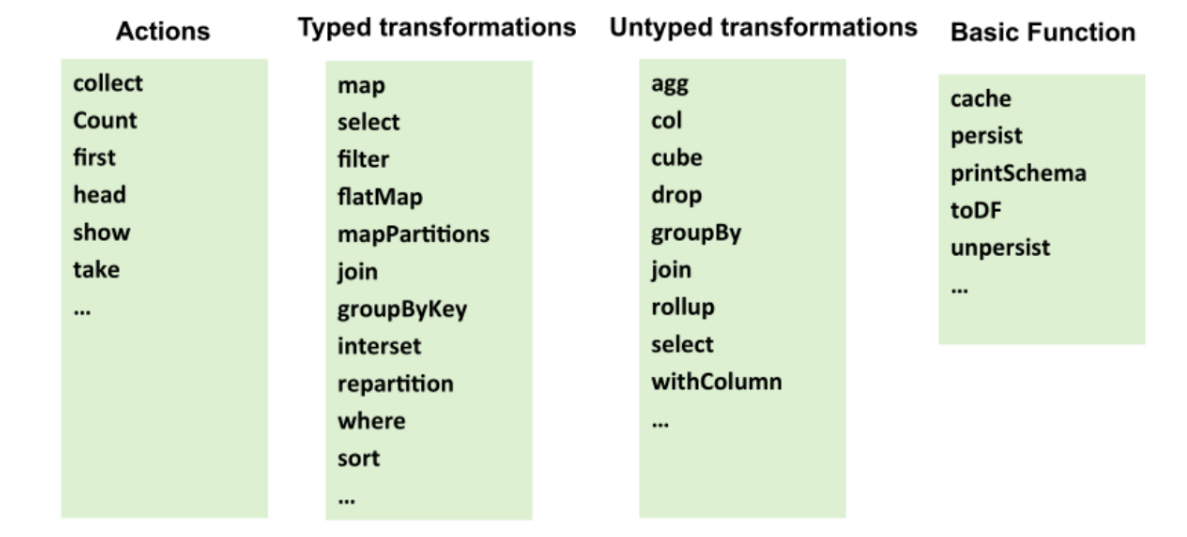
Pyspark
2、DataFrame 2.1 介绍 在Spark语义中,DataFrame是一个分布式的行集合,可以想象为一个关系型数据库的表,或者一个带有列名的Excel表格。它和RDD一样,有这样一些特点: Immuatable:一旦RDD、DataFrame被创…...
Spring Boot 项目五维度九层次分层架构实现实践研究——持续更新中
说明:本博文主要参考来自 https://blog.csdn.net/BASK2311/article/details/128198005 据实践内容及代码持续总结更新中。 五个分层维度:SpringBoot工程分层实战 1 分层思想 计算机领域有一句话:计算机中任何问题都可通过增加一个虚拟层解…...
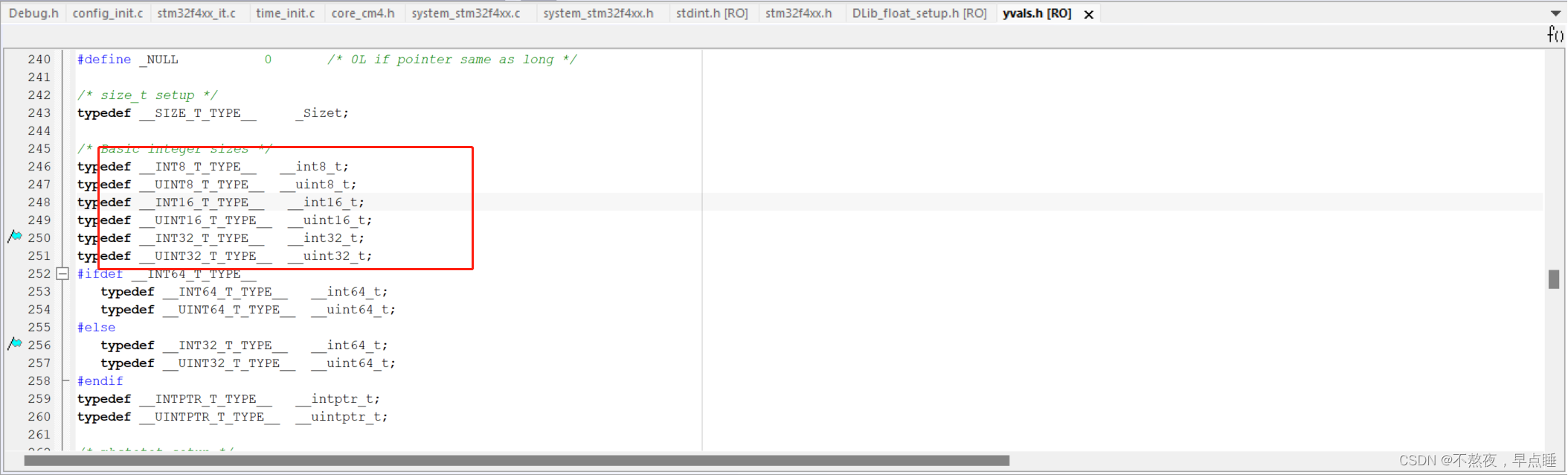
stm32常见数据类型
stm32的数据类型的字节长度 s8 占用1个byte,数据范围 -2^7 到 (2^7-1) s16 占用2个byte,数据范围 -2^15 到 (2^15-1) s32 占用 4个byte,数据范围 -2^31 到 (231-1)231 2147483647 int64_t占用8个byte,数据范围 -2^63 到 (2^63-1)…...
mac m1使用docker安装kafka
1.拉取镜像 docker pull zookeeper docker pull wurstmeister/kafka 2.启动zookeeper docker run -d --name zookeeper -p 2181:2181 zookeeper 3.设置zookeeper容器对外服务的ip Zookeeper_Server_IP$(docker inspect zookeeper --format{{ .NetworkSettings.IPAddress }}…...
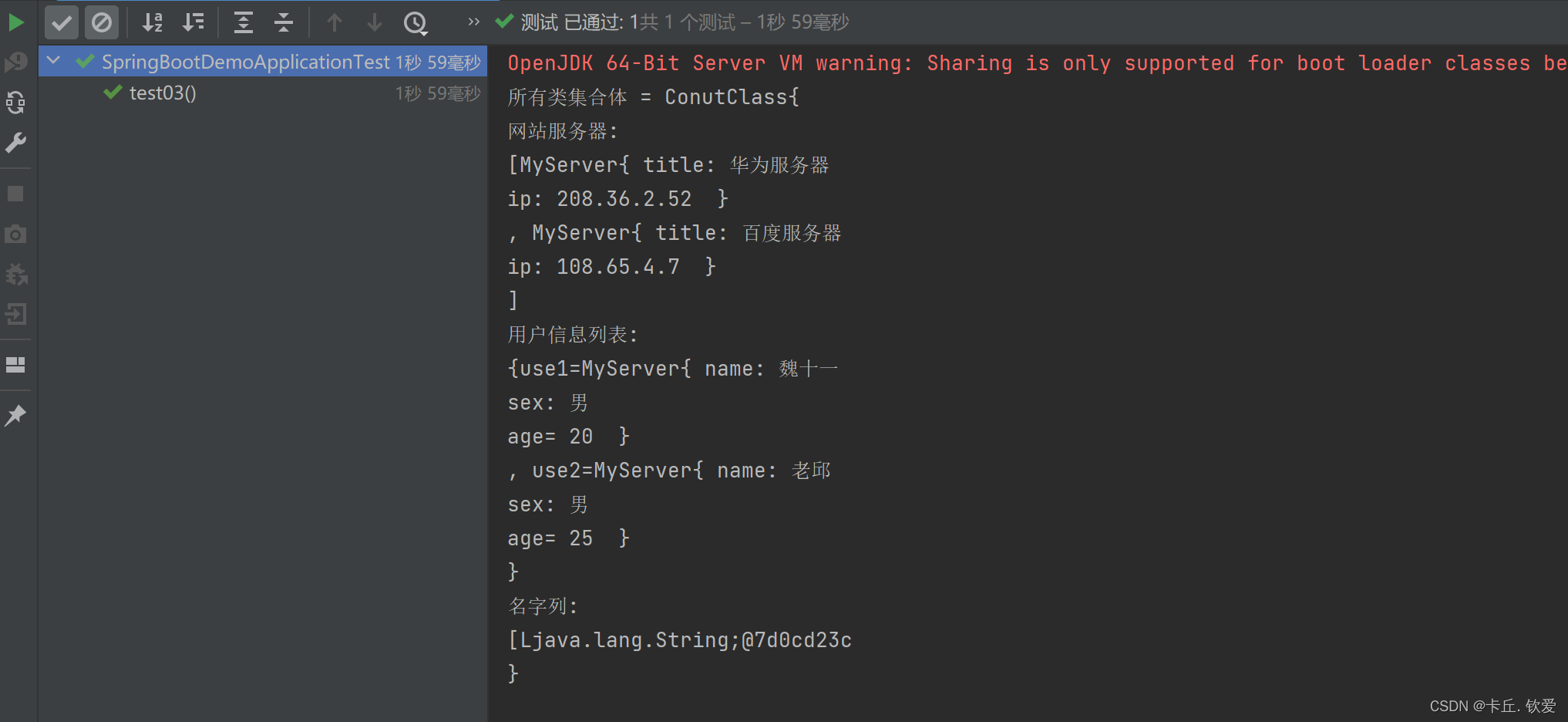
SpringBoot核心配置和注解
目录 一、注解 元注解 基本注解 启动注解 二、配置 格式介绍 读取配置文件信息 案例演示1 嵌套读取bean信息 案例演示2 读取Map,List 以及 Array 类型配置数据 案例演示3 三、总结 一、注解 之前我们了解了SpringBoot基础和AOP简单应用,这期来讲…...
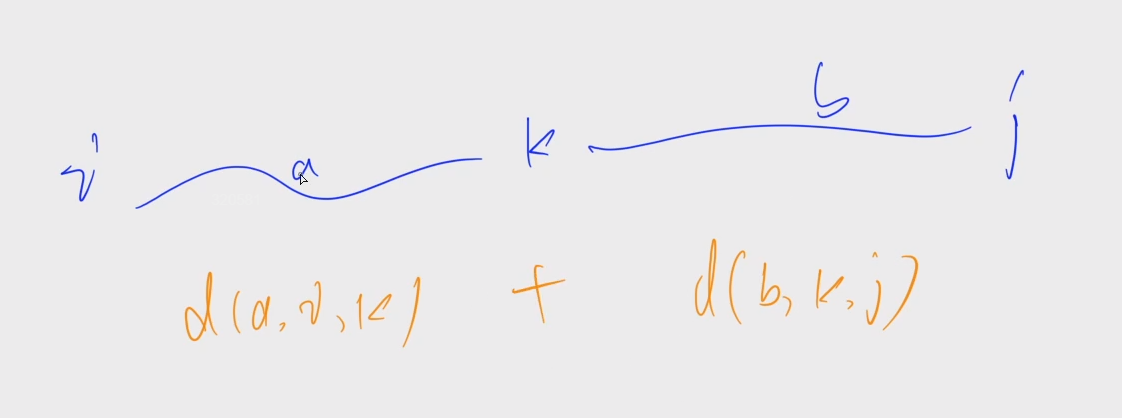
第三章 图论 No.3 flody之多源汇最短路,传递闭包,最小环与倍增
文章目录 多源汇最短路:1125. 牛的旅行传递闭包:343. 排序最小环:344. 观光之旅345. 牛站 flody的四个应用: 多源汇最短路传递闭包找最小环恰好经过k条边的最短路 倍增 多源汇最短路:1125. 牛的旅行 1125. 牛的旅行 …...
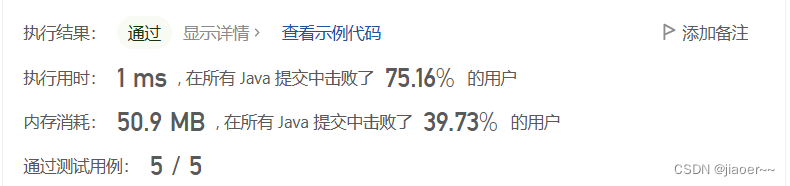
Leetcode-每日一题【剑指 Offer 17. 打印从1到最大的n位数】
题目 输入数字 n,按顺序打印出从 1 到最大的 n 位十进制数。比如输入 3,则打印出 1、2、3 一直到最大的 3 位数 999。 示例 1: 输入: n 1输出: [1,2,3,4,5,6,7,8,9] 说明: 用返回一个整数列表来代替打印 n 为正整数 解题思路 前置知识 M…...
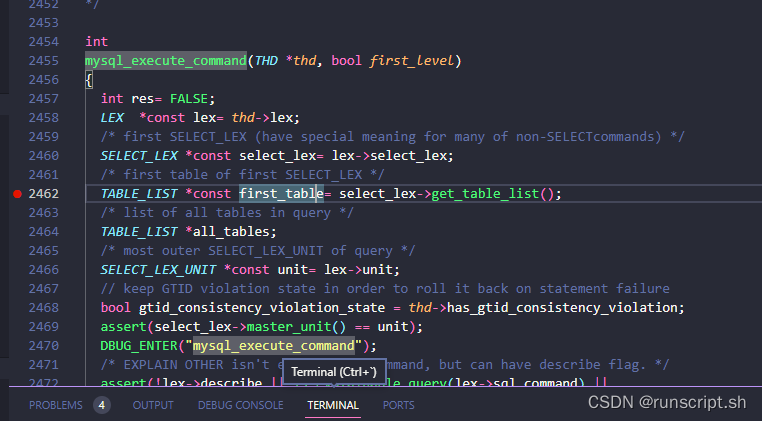
远程调试MySQL内核
1 vscode 需要安装remote-ssh插件 安装成功后,登录: 默认远程服务器的登录 ssh rootip注意,Linux需要设置root远程登录; 2 安装debug扩展 C\C extemsion Pack C\C3 设置Attach进程 {// Use IntelliSense to learn about poss…...
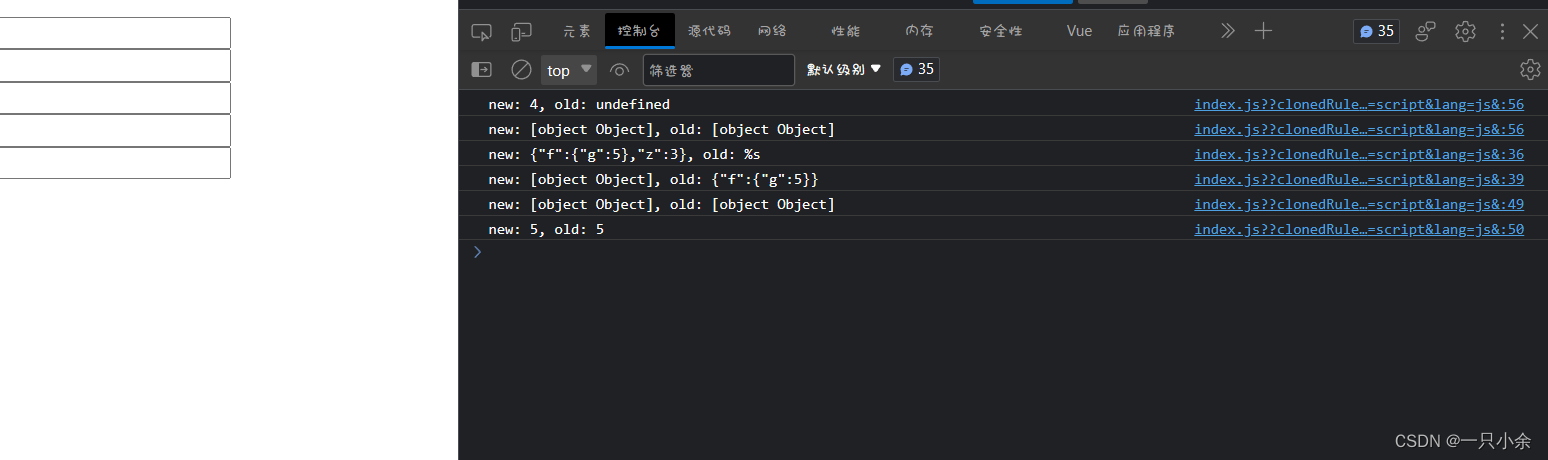
前端学习---vue2--选项/数据--data-computed-watch-methods-props
写在前面: vue提供了很多数据相关的。 文章目录 data 动态绑定介绍使用使用数据 computed 计算属性介绍基础使用计算属性缓存 vs 方法完整使用 watch 监听属性介绍使用 methodspropspropsData data 动态绑定 介绍 简单的说就是进行双向绑定的区域。 vue实例的数…...
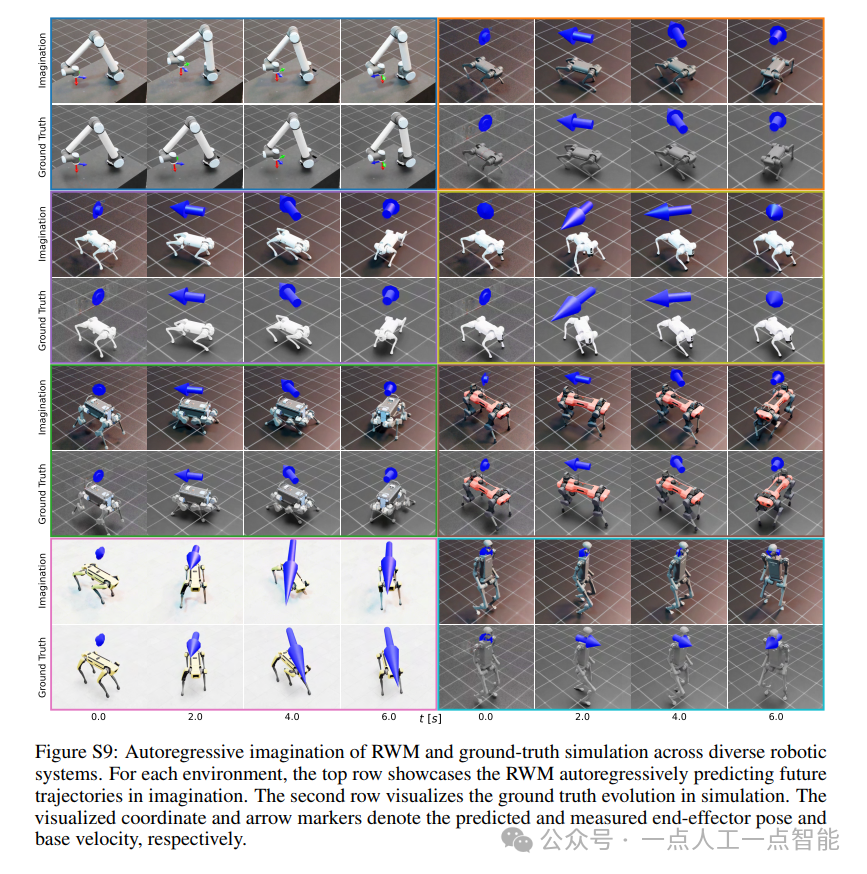
未来机器人的大脑:如何用神经网络模拟器实现更智能的决策?
编辑:陈萍萍的公主一点人工一点智能 未来机器人的大脑:如何用神经网络模拟器实现更智能的决策?RWM通过双自回归机制有效解决了复合误差、部分可观测性和随机动力学等关键挑战,在不依赖领域特定归纳偏见的条件下实现了卓越的预测准…...
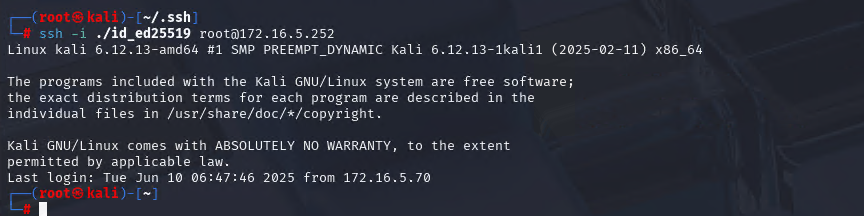
Xshell远程连接Kali(默认 | 私钥)Note版
前言:xshell远程连接,私钥连接和常规默认连接 任务一 开启ssh服务 service ssh status //查看ssh服务状态 service ssh start //开启ssh服务 update-rc.d ssh enable //开启自启动ssh服务 任务二 修改配置文件 vi /etc/ssh/ssh_config //第一…...
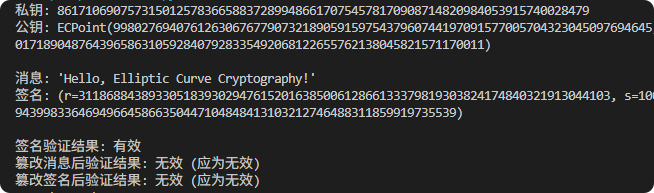
现代密码学 | 椭圆曲线密码学—附py代码
Elliptic Curve Cryptography 椭圆曲线密码学(ECC)是一种基于有限域上椭圆曲线数学特性的公钥加密技术。其核心原理涉及椭圆曲线的代数性质、离散对数问题以及有限域上的运算。 椭圆曲线密码学是多种数字签名算法的基础,例如椭圆曲线数字签…...
rnn判断string中第一次出现a的下标
# coding:utf8 import torch import torch.nn as nn import numpy as np import random import json""" 基于pytorch的网络编写 实现一个RNN网络完成多分类任务 判断字符 a 第一次出现在字符串中的位置 """class TorchModel(nn.Module):def __in…...
【Java学习笔记】BigInteger 和 BigDecimal 类
BigInteger 和 BigDecimal 类 二者共有的常见方法 方法功能add加subtract减multiply乘divide除 注意点:传参类型必须是类对象 一、BigInteger 1. 作用:适合保存比较大的整型数 2. 使用说明 创建BigInteger对象 传入字符串 3. 代码示例 import j…...
Caliper 负载(Workload)详细解析
Caliper 负载(Workload)详细解析 负载(Workload)是 Caliper 性能测试的核心部分,它定义了测试期间要执行的具体合约调用行为和交易模式。下面我将全面深入地讲解负载的各个方面。 一、负载模块基本结构 一个典型的负载模块(如 workload.js)包含以下基本结构: use strict;/…...
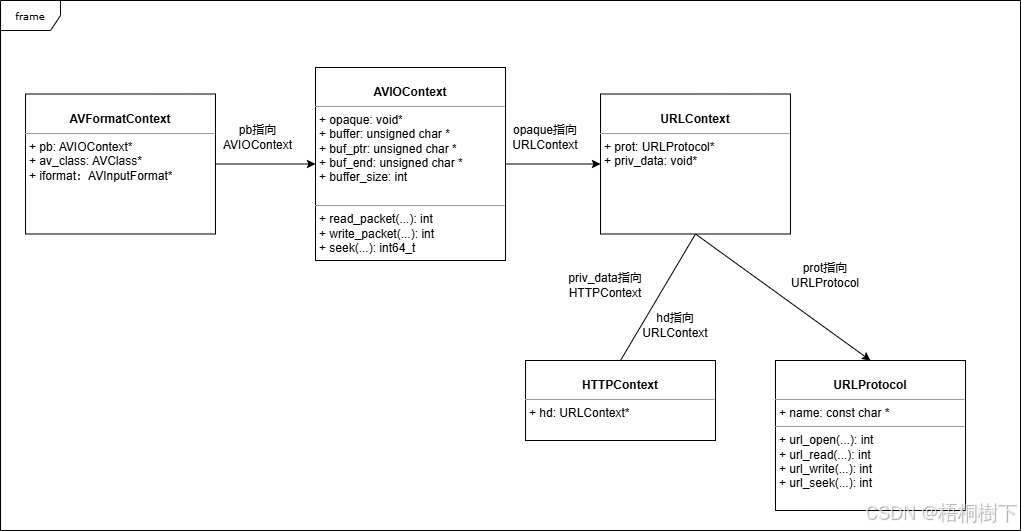
FFmpeg avformat_open_input函数分析
函数内部的总体流程如下: avformat_open_input 精简后的代码如下: int avformat_open_input(AVFormatContext **ps, const char *filename,ff_const59 AVInputFormat *fmt, AVDictionary **options) {AVFormatContext *s *ps;int i, ret 0;AVDictio…...
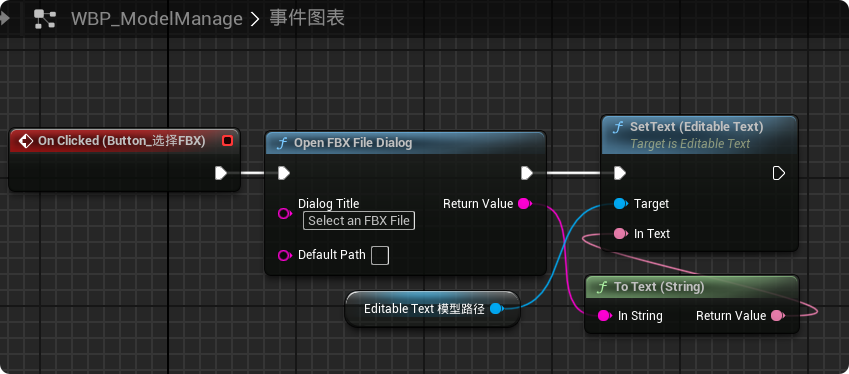
【UE5 C++】通过文件对话框获取选择文件的路径
目录 效果 步骤 源码 效果 步骤 1. 在“xxx.Build.cs”中添加需要使用的模块 ,这里主要使用“DesktopPlatform”模块 2. 添加后闭UE编辑器,右键点击 .uproject 文件,选择 "Generate Visual Studio project files",重…...
机器学习的数学基础:线性模型
线性模型 线性模型的基本形式为: f ( x ) ω T x b f\left(\boldsymbol{x}\right)\boldsymbol{\omega}^\text{T}\boldsymbol{x}b f(x)ωTxb 回归问题 利用最小二乘法,得到 ω \boldsymbol{\omega} ω和 b b b的参数估计$ \boldsymbol{\hat{\omega}}…...
前端调试HTTP状态码
1xx(信息类状态码) 这类状态码表示临时响应,需要客户端继续处理请求。 100 Continue 服务器已收到请求的初始部分,客户端应继续发送剩余部分。 2xx(成功类状态码) 表示请求已成功被服务器接收、理解并处…...