langchain agent
zero-shot-react-description
代码
from langchain.agents import initialize_agent
from langchain.llms import OpenAI
from langchain.tools import BaseTool
import os
os.environ['OPENAI_API_KEY']="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"# 搜索工具
class SearchTool(BaseTool):name = "Search"description = "如果我想知道天气,'鸡你太美'这两个问题时,请使用它"return_direct = True # 直接返回结果def _run(self, query: str) -> str:print("\nSearchTool query: " + query)return "这个是一个通用的返回"async def _arun(self, query: str) -> str:raise NotImplementedError("暂时不支持异步")# 计算工具
class CalculatorTool(BaseTool):name = "Calculator"description = "如果是关于数学计算的问题,请使用它"def _run(self, query: str) -> str:print("\nCalculatorTool query: " + query)return "100"async def _arun(self, query: str) -> str:raise NotImplementedError("暂时不支持异步")llm = OpenAI(temperature=0.5)
tools = [SearchTool(), CalculatorTool()]
agent = initialize_agent(tools, llm, agent="zero-shot-react-description", verbose=True)print("问题:")
print("答案:" + agent.run("查询这周天气"))
print("问题:")
print("答案:" + agent.run("告诉我'鸡你太美'是什么意思"))
print("问题:")
print("答案:" + agent.run("告诉我'hello world'是什么意思"))
print("问题:")
print("答案:" + agent.run("告诉我10的3次方是多少?"))
以上代码构造如下prompt
Answer the following questions as best you can. You have access to the following tools:Search: 如果我想知道天气,'鸡你太美'这两个问题时,请使用它
Calculator: 如果是关于数学计算的问题,请使用它Use the following format:Question: the input question you must answer
Thought: you should always think about what to do
Action: the action to take, should be one of [Search, Calculator]
Action Input: the input to the action
Observation: the result of the action
... (this Thought/Action/Action Input/Observation can repeat N times)
Thought: I now know the final answer
Final Answer: the final answer to the original input questionBegin!Question: 查询这周天气
Thought:
prompt模板:
PREFIX = """Answer the following questions as best you can. You have access to the following tools:"""
FORMAT_INSTRUCTIONS = """Use the following format:Question: the input question you must answer
Thought: you should always think about what to do
Action: the action to take, should be one of [{tool_names}]
Action Input: the input to the action
Observation: the result of the action
... (this Thought/Action/Action Input/Observation can repeat N times)
Thought: I now know the final answer
Final Answer: the final answer to the original input question"""
SUFFIX = """Begin!Question: {input}
Thought:{agent_scratchpad}"""
示例二
代码
import os
os.environ['SERPAPI_API_KEY']="xxx"from langchain.agents import Tool
from langchain.agents import AgentType
from langchain.memory import ConversationBufferMemory
from langchain import OpenAI
from langchain.utilities import SerpAPIWrapper
from langchain.agents import initialize_agent
import os
os.environ['OPENAI_API_KEY']="sk-xxx"
search = SerpAPIWrapper()
tools = [Tool(name = "Current Search",func=search.run,description="useful for when you need to answer questions about current events or the current state of the world"),
]memory = ConversationBufferMemory(memory_key="chat_history")llm=OpenAI(temperature=0)
agent_chain = initialize_agent(tools, llm, agent=AgentType.CONVERSATIONAL_REACT_DESCRIPTION, verbose=True, memory=memory)
agent_chain.run(input="hi, i am bob")
agent_chain.run(input="what's my name?")
agent_chain.run("what are some good dinners to make this week, if i like thai food?")
agent_chain.run(input="tell me the last letter in my name, and also tell me who won the world cup in 1978?")
agent_chain.run(input="whats the current temperature in pomfret?")
以上代码使用如下的prompt
Assistant is a large language model trained by OpenAI.Assistant is designed to be able to assist with a wide range of tasks, from answering simple questions to providing in-depth explanations and discussions on a wide range of topics. As a language model, Assistant is able to generate human-like text based on the input it receives, allowing it to engage in natural-sounding conversations and provide responses that are coherent and relevant to the topic at hand.Assistant is constantly learning and improving, and its capabilities are constantly evolving. It is able to process and understand large amounts of text, and can use this knowledge to provide accurate and informative responses to a wide range of questions. Additionally, Assistant is able to generate its own text based on the input it receives, allowing it to engage in discussions and provide explanations and descriptions on a wide range of topics.Overall, Assistant is a powerful tool that can help with a wide range of tasks and provide valuable insights and information on a wide range of topics. Whether you need help with a specific question or just want to have a conversation about a particular topic, Assistant is here to assist.TOOLS:
------Assistant has access to the following tools:> Current Search: useful for when you need to answer questions about current events or the current state of the worldTo use a tool, please use the following format:```
Thought: Do I need to use a tool? Yes
Action: the action to take, should be one of [Current Search]
Action Input: the input to the action
Observation: the result of the action
```When you have a response to say to the Human, or if you do not need to use a tool, you MUST use the format:```
Thought: Do I need to use a tool? No
AI: [your response here]
```Begin!Previous conversation history:
Human: hi, i am bob
AI: Hi Bob, nice to meet you! How can I help you today?
Human: what's my name?
AI: Your name is Bob!New input: what are some good dinners to make this week, if i like thai food?
prompt 模板:
# flake8: noqa
PREFIX = """Assistant is a large language model trained by OpenAI.Assistant is designed to be able to assist with a wide range of tasks, from answering simple questions to providing in-depth explanations and discussions on a wide range of topics. As a language model, Assistant is able to generate human-like text based on the input it receives, allowing it to engage in natural-sounding conversations and provide responses that are coherent and relevant to the topic at hand.Assistant is constantly learning and improving, and its capabilities are constantly evolving. It is able to process and understand large amounts of text, and can use this knowledge to provide accurate and informative responses to a wide range of questions. Additionally, Assistant is able to generate its own text based on the input it receives, allowing it to engage in discussions and provide explanations and descriptions on a wide range of topics.Overall, Assistant is a powerful tool that can help with a wide range of tasks and provide valuable insights and information on a wide range of topics. Whether you need help with a specific question or just want to have a conversation about a particular topic, Assistant is here to assist.TOOLS:
------Assistant has access to the following tools:"""
FORMAT_INSTRUCTIONS = """To use a tool, please use the following format:```
Thought: Do I need to use a tool? Yes
Action: the action to take, should be one of [{tool_names}]
Action Input: the input to the action
Observation: the result of the action
```When you have a response to say to the Human, or if you do not need to use a tool, you MUST use the format:```
Thought: Do I need to use a tool? No
{ai_prefix}: [your response here]
```"""SUFFIX = """Begin!Previous conversation history:
{chat_history}New input: {input}
{agent_scratchpad}"""
llm_math_chain
每一步action,可以是另外一个chain,
代码示例:
# Import things that are needed generically
import os
os.environ['SERPAPI_API_KEY']="111xxxx"
os.environ['OPENAI_API_KEY']="sk-xxxxxx"
from langchain import LLMMathChain, SerpAPIWrapper
from langchain.agents import AgentType, initialize_agent
from langchain.chat_models import ChatOpenAI
from langchain.tools import BaseTool, StructuredTool, Tool, tool
from pydantic import BaseModel, Field
llm = ChatOpenAI(temperature=0)# Load the tool configs that are needed.
search = SerpAPIWrapper()
llm_math_chain = LLMMathChain(llm=llm, verbose=True)from typing import Optional, Typefrom langchain.callbacks.manager import (AsyncCallbackManagerForToolRun,CallbackManagerForToolRun,
)class CustomSearchTool(BaseTool):name = "custom_search"description = "useful for when you need to answer questions about current events"def _run(self, query: str, run_manager: Optional[CallbackManagerForToolRun] = None) -> str:"""Use the tool."""return search.run(query)async def _arun(self, query: str, run_manager: Optional[AsyncCallbackManagerForToolRun] = None) -> str:"""Use the tool asynchronously."""raise NotImplementedError("custom_search does not support async")class CalculatorInput(BaseModel):question: str = Field()class CustomCalculatorTool(BaseTool):name = "Calculator"description = "useful for when you need to answer questions about math"args_schema: Type[BaseModel] = CalculatorInputdef _run(self, query: str, run_manager: Optional[CallbackManagerForToolRun] = None) -> str:"""Use the tool."""return llm_math_chain.run(query)async def _arun(self, query: str, run_manager: Optional[AsyncCallbackManagerForToolRun] = None) -> str:"""Use the tool asynchronously."""raise NotImplementedError("Calculator does not support async")
tools = [CustomSearchTool(), CustomCalculatorTool()]
agent = initialize_agent(tools, llm, agent=AgentType.ZERO_SHOT_REACT_DESCRIPTION, verbose=True
)agent.run("Who is Leo DiCaprio's girlfriend? What is her current age raised to the 0.43 power?"
)
prompt
Translate a math problem into a expression that can be executed using Python's numexpr library. Use the output of running this code to answer the question.Question: ${Question with math problem.}
```text
${single line mathematical expression that solves the problem}
```
...numexpr.evaluate(text)...
```output
${Output of running the code}
```
Answer: ${Answer}Begin.Question: What is 37593 * 67?
```text
37593 * 67
```
...numexpr.evaluate("37593 * 67")...
```output
2518731
```
Answer: 2518731Question: 37593^(1/5)
```text
37593**(1/5)
```
...numexpr.evaluate("37593**(1/5)")...
```output
8.222831614237718
```
Answer: 8.222831614237718Question: 19 ^ 0.43
prompt模板:
"""Translate a math problem into a expression that can be executed using Python's numexpr library. Use the output of running this code to answer the question.Question: ${{Question with math problem.}}
```text
${{single line mathematical expression that solves the problem}}
```
...numexpr.evaluate(text)...
```output
${{Output of running the code}}
```
Answer: ${{Answer}}Begin.Question: What is 37593 * 67?
```text
37593 * 67
```
...numexpr.evaluate("37593 * 67")...
```output
2518731
```
Answer: 2518731Question: 37593^(1/5)
```text
37593**(1/5)
```
...numexpr.evaluate("37593**(1/5)")...
```output
8.222831614237718
```
Answer: 8.222831614237718Question: {question}
"""
STRUCTURED_CHAT_ZERO_SHOT_REACT_DESCRIPTION
核心思想:工具如果需要多种输入,也需要将参数一起构建进prompt当中
代码示例
import os
os.environ['SERPAPI_API_KEY']="23123"
os.environ['OPENAI_API_KEY']="sk-232323"
os.environ["LANGCHAIN_TRACING"] = "true"
from langchain import OpenAI
from langchain.agents import initialize_agent, AgentTypellm = OpenAI(temperature=0)
from langchain.tools import StructuredTooldef multiplier(a: float, b: float) -> float:"""Multiply the provided floats."""return a * btool = StructuredTool.from_function(multiplier)
# Structured tools are compatible with the STRUCTURED_CHAT_ZERO_SHOT_REACT_DESCRIPTION agent type.
agent_executor = initialize_agent([tool],llm,agent=AgentType.STRUCTURED_CHAT_ZERO_SHOT_REACT_DESCRIPTION,verbose=True,
)agent_executor.run("What is 3 times 4")
prompt
System: Respond to the human as helpfully and accurately as possible. You have access to the following tools:multiplier: multiplier(a: float, b: float) -> float - Multiply the provided floats., args: {{'a': {{'title': 'A', 'type': 'number'}}, 'b': {{'title': 'B', 'type': 'number'}}}}Use a json blob to specify a tool by providing an action key (tool name) and an action_input key (tool input).Valid "action" values: "Final Answer" or multiplierProvide only ONE action per $JSON_BLOB, as shown:```
{"action": $TOOL_NAME,"action_input": $INPUT
}
```Follow this format:Question: input question to answer
Thought: consider previous and subsequent steps
Action:
```
$JSON_BLOB
```
Observation: action result
... (repeat Thought/Action/Observation N times)
Thought: I know what to respond
Action:
```
{"action": "Final Answer","action_input": "Final response to human"
}
```Begin! Reminder to ALWAYS respond with a valid json blob of a single action. Use tools if necessary. Respond directly if appropriate. Format is Action:```$JSON_BLOB```then Observation:.
Thought:
Human: What is 3 times 4This was your previous work (but I haven't seen any of it! I only see what you return as final answer):
Action:
```
{"action": "multiplier","action_input": {"a": 3, "b": 4}
}
```Observation: 12.0
Thought:
相关文章:

langchain agent
zero-shot-react-description 代码 from langchain.agents import initialize_agent from langchain.llms import OpenAI from langchain.tools import BaseTool import os os.environ[OPENAI_API_KEY]"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"# 搜索…...
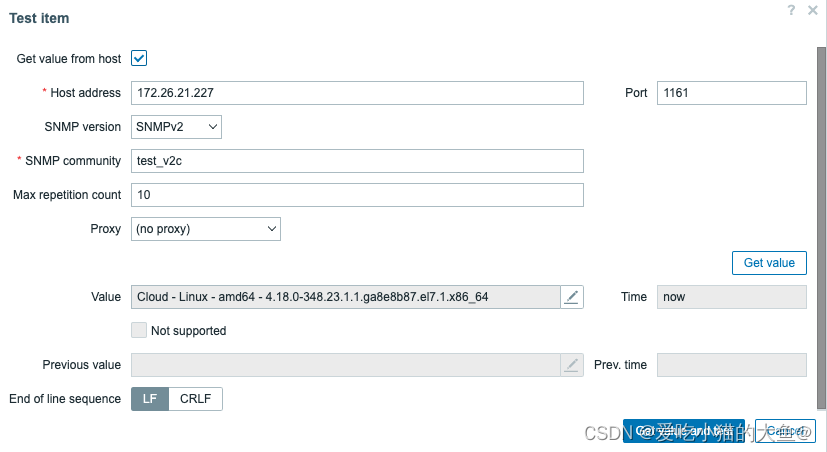
Zabbix下载安装及SNMP Get使用
帮助文档:6. Zabbix Appliance 一、zabbix下载安装 1、获取Zabbix Appliance镜像 Download Zabbix appliance 2、使用该镜像创建虚拟机 3、打开虚拟机控制台自动安装,等待安装完成即可 默认配置 系统/数据库:root:zabbix Zabbix 前端&am…...
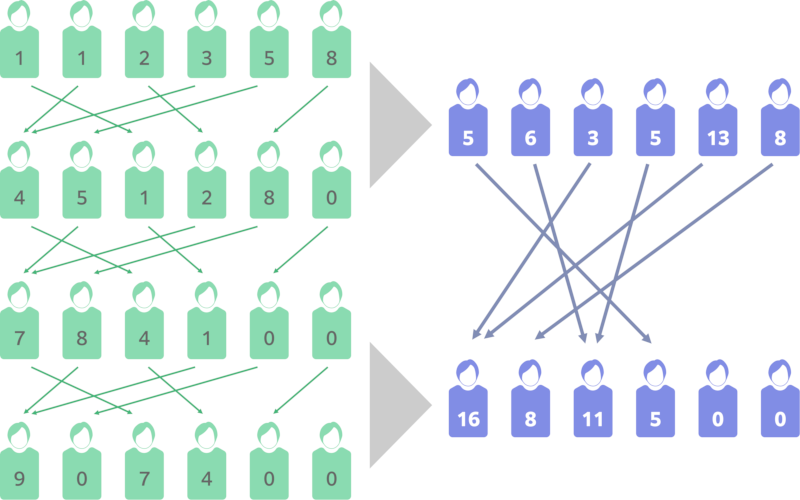
freee Programming Contest 2023(AtCoder Beginner Contest 310)
文章目录 A - Order Something Else(模拟)B - Strictly Superior(模拟)C - Reversible(模拟)D - Peaceful Teams(DFS状压)E - NAND repeatedly(普通dp)F - Make 10 Again(状态压缩概率dp&#x…...
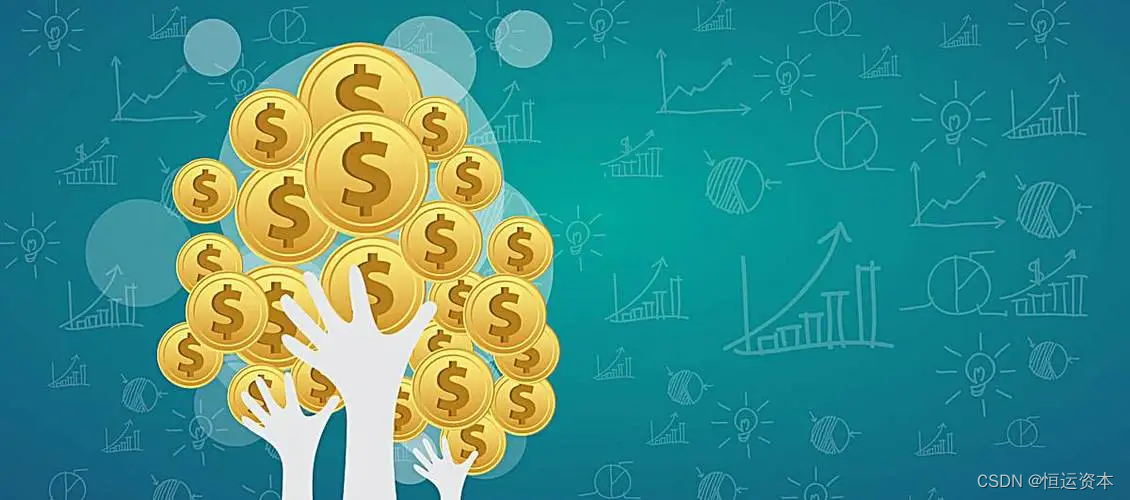
恒运资本:概念股是什么意思
概念股是指在特定的经济布景、方针环境、职业远景或社会热点等方面具有某种特别的发展远景和投资价值的股票。在投资者心目中,概念股的危险较大,可是或许带来高于商场平均水平的收益率。那么,概念股到底是什么意思?在本文中&#…...
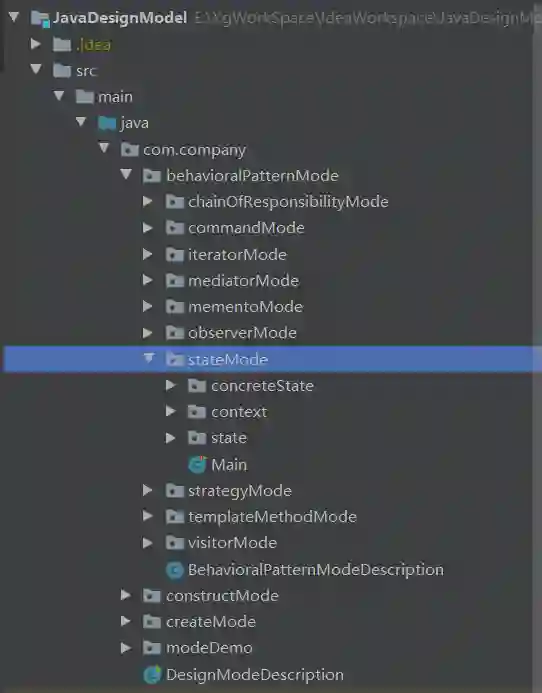
十九、状态模式
一、什么是状态模式 状态(State)模式的定义:对有状态的对象,把复杂的“判断逻辑”提取到不同的状态对象中,允许状态对象在其内部状态发生改变时改变其行为。 状态模式包含以下主要角色: 环境类(…...
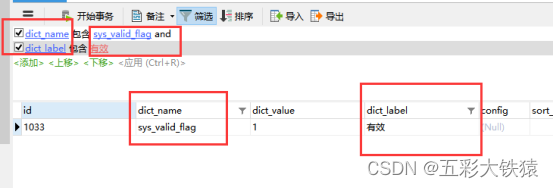
MySQL用navicat工具对表进行筛选查找
这个操作其实很简单,但是对于没操作的人来说,就是不会呀。所以小编出这一个详细图解,希望能够帮助到大家。话不多说看图。 第一步: 点进一张表,点击筛选。 第二步: 点击添加 第三步: 选择要…...

音视频 ffplay简单过滤器
视频旋转 ffplay -i test.mp4 -vf transpose1视频反转 ffplay test.mp4 -vf hflip ffplay test.mp4 -vf vflip视频旋转和反转 ffplay test.mp4 -vf hflip,transpose1音频变速播放 ffplay -i test.mp4 -af atempo2视频变速播放 ffplay -i test.mp4 -vf setptsPTS/2音视频同…...

索引 事务 存储引擎
################索引##################### 一、索引的概念 ●索引是一个排序的列表,在这个列表中存储着索引的值和包含这个值的数据所在行的物理地址(类似于C语言的链表通过指针指向数据记录的内存地址)。 ●使用索引后可以不用扫描全表来…...
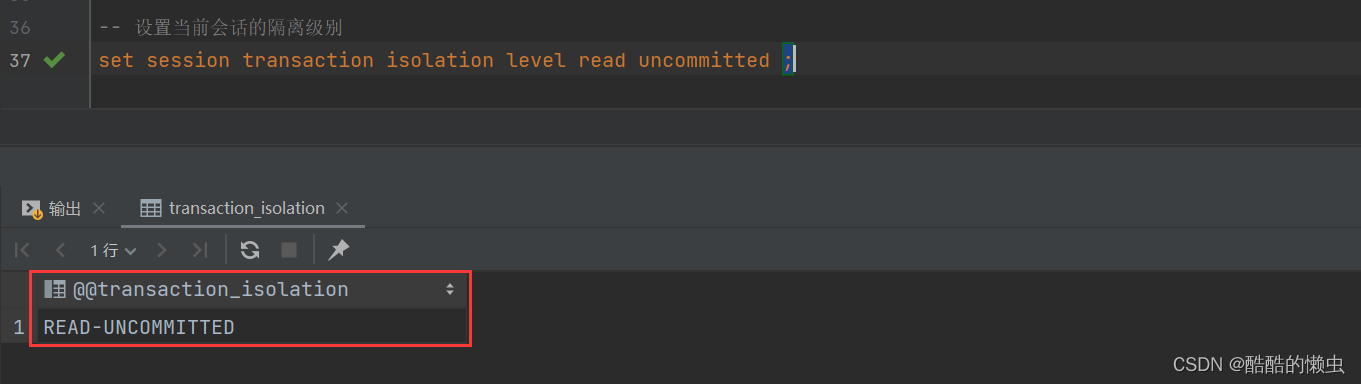
MySQL— 基础语法大全及操作演示!!!(事务)
MySQL—— 基础语法大全及操作演示(事务) 六、事务6.1 事务简介6.2 事务操作6.2.1 未控制事务6.2.2 控制事务一6.2.3 控制事务二 6.3 事务四大特性6.4 并发事务问题6.5 事务隔离级别 MySQL— 基础语法大全及操作演示!!!…...
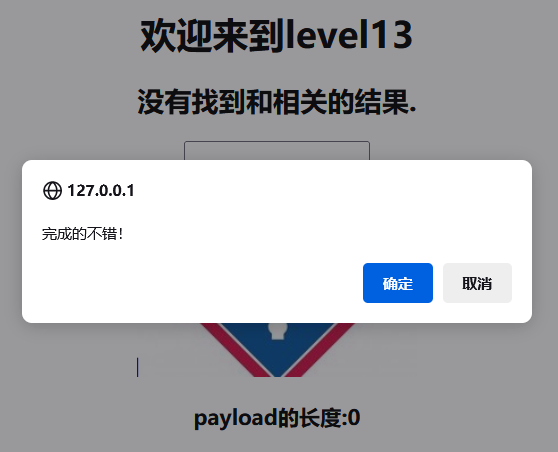
xsschallenge1~13通关详细教程
文章目录 XSS 挑战靶场通关level1level2level3level4level5level6level7level8level9level10level11level12level13 XSS 挑战靶场通关 level1 通过观察发现这个用户信息可以修改 那么我们直接输入攻击代码 <script>alert(/wuhu/)</script>弹框如下: …...
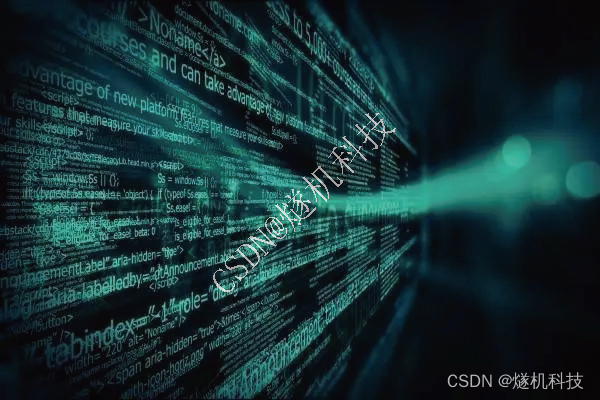
考生作弊行为分析算法
考生作弊行为分析系统利用pythonyolo系列网络模型算法框架,考生作弊行为分析算法利用图像处理和智能算法对考生的行为进行分析和识别,经过算法服务器的复杂计算和逻辑判断,算法将根据考生行为的特征和规律,判定是否存在作弊行为。…...

Python 操作 Redis 数据库介绍
Redis 作为常用的 NoSql 数据库,主要用于缓存数据,提高数据读取效率,那在 Python 中应该如果连接和操作 Redis 呢?今天就为大概简单介绍下,在 Python 中操作 Redis 常用命令。 安装 redis 首先还是需要先安装 redis …...

十年JAVA搬砖路——软件工程概述
软件工程是一门关注软件开发过程的学科,它涉及到软件的设计、开发、测试、部署和维护等方面。软件工程的目标是通过系统化的方法和工具,以确保软件项目能够按时、按预算和按要求完成。 • 软件工程的7个基本概念: 软件生命周期:软…...
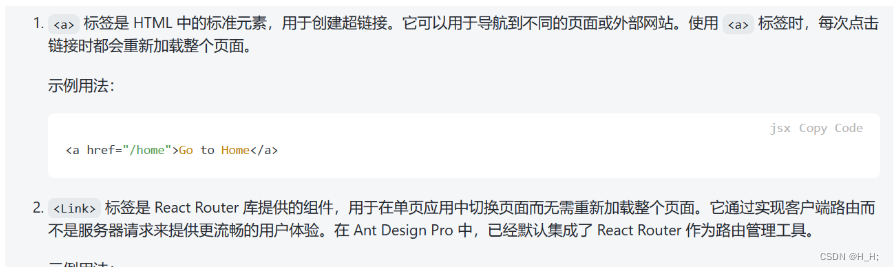
前后端项目部署上线详细笔记
部署 参考文章:如何部署网站?来比比谁的方法多 - 哔哩哔哩大家好,我是鱼皮,不知道朋友们有没有试着部署过自己开发的网站呢?其实部署网站非常简单,而且有非常多的花样。这篇文章就给大家分享几种主流的前端…...
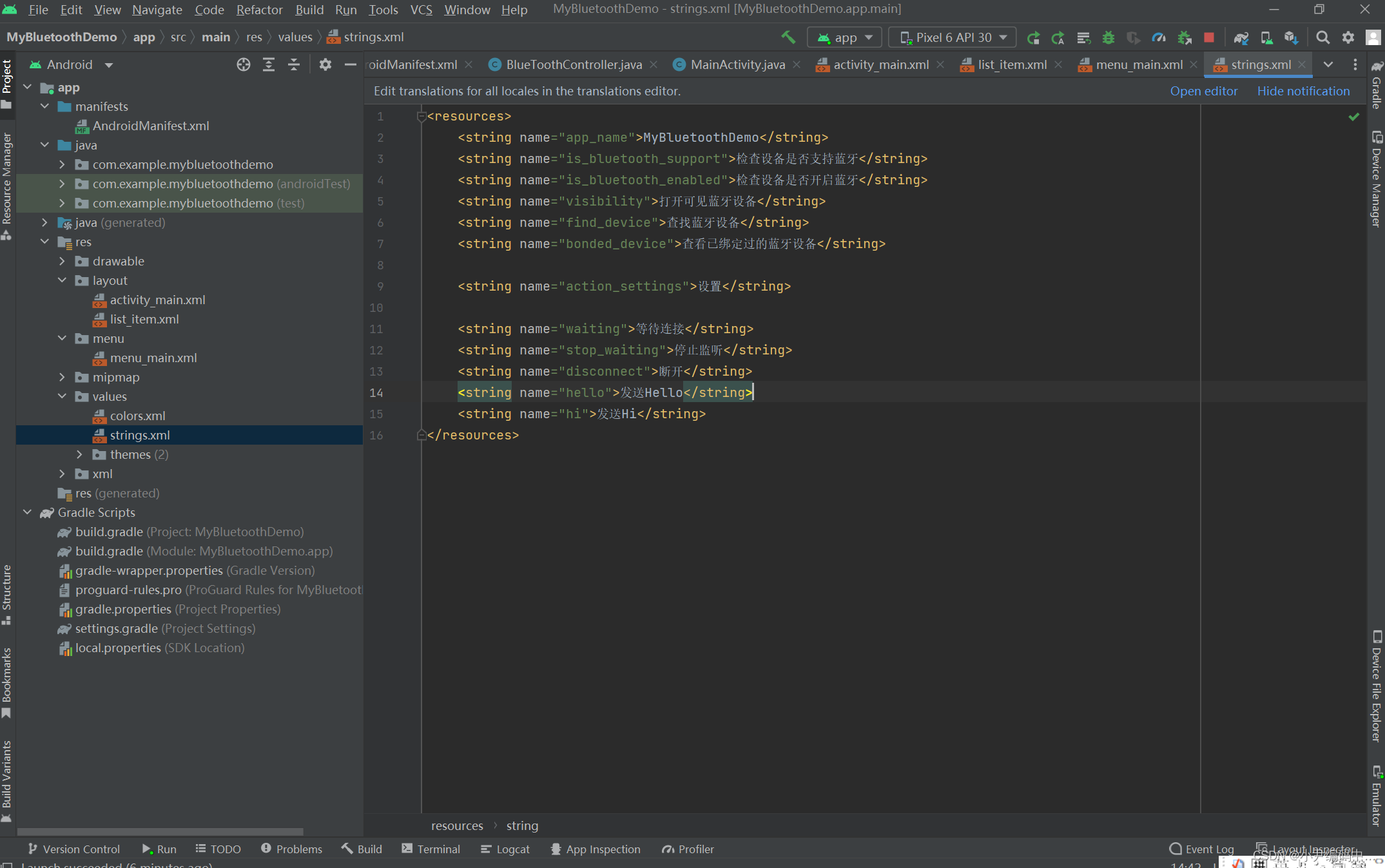
Android 蓝牙开发( 二 )
前言 上一篇文章给大家分享了Android蓝牙的基础知识和基础用法,不过上一篇都是一些零散碎片化的程序,这一篇给大家分享Android蓝牙开发实战项目的初步使用 效果演示 : Android蓝牙搜索,配对,连接,通信 Android蓝牙实…...
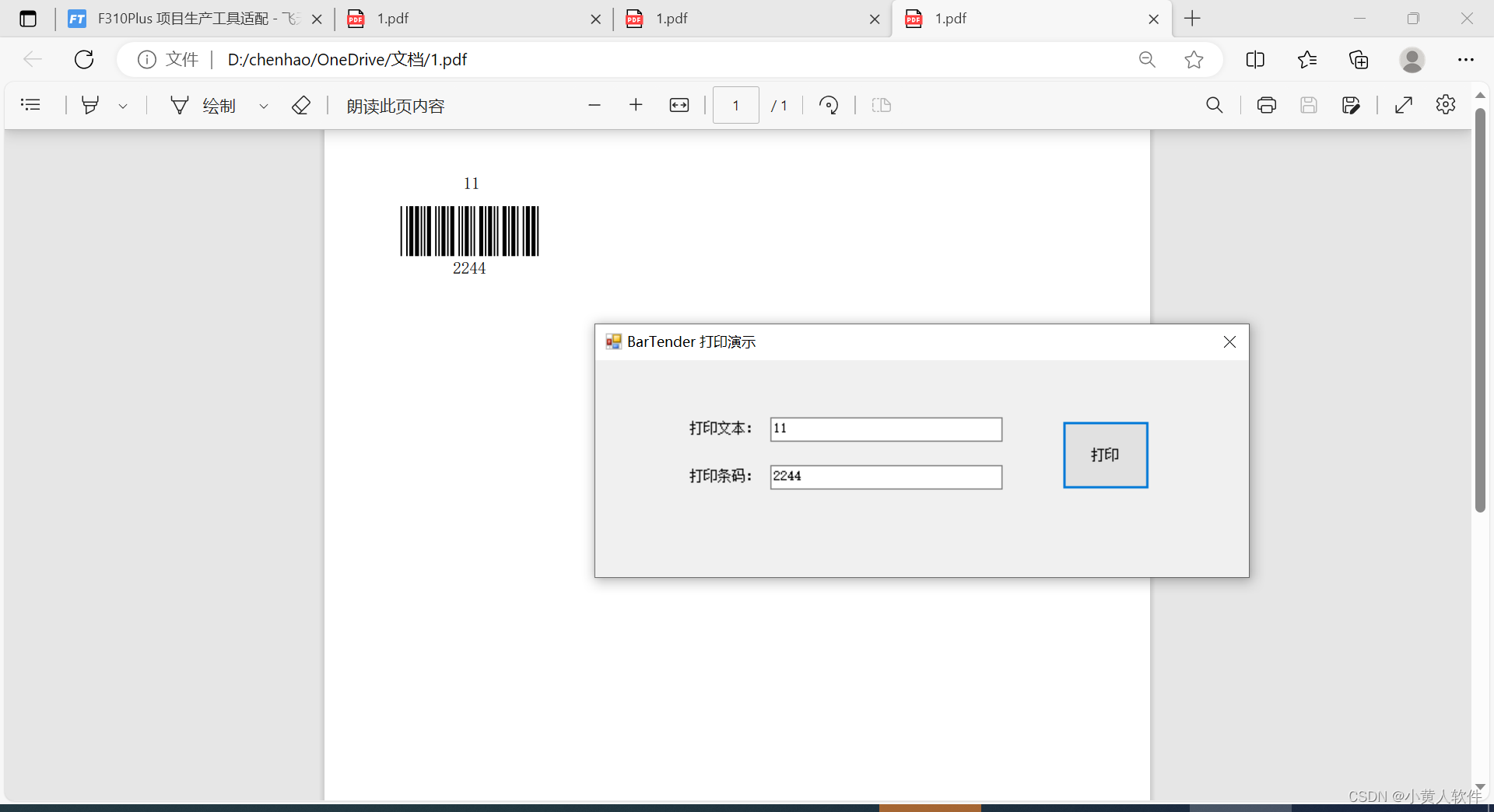
C#调用barTender打印标签示例
使用的电脑需要先安装BarTender 我封装成一个类 using System; using System.Windows.Forms;namespace FT_Tools {public class SysContext{public static BarTender.Application btapp new BarTender.Application();public static BarTender.Format btFormat;public void Q…...
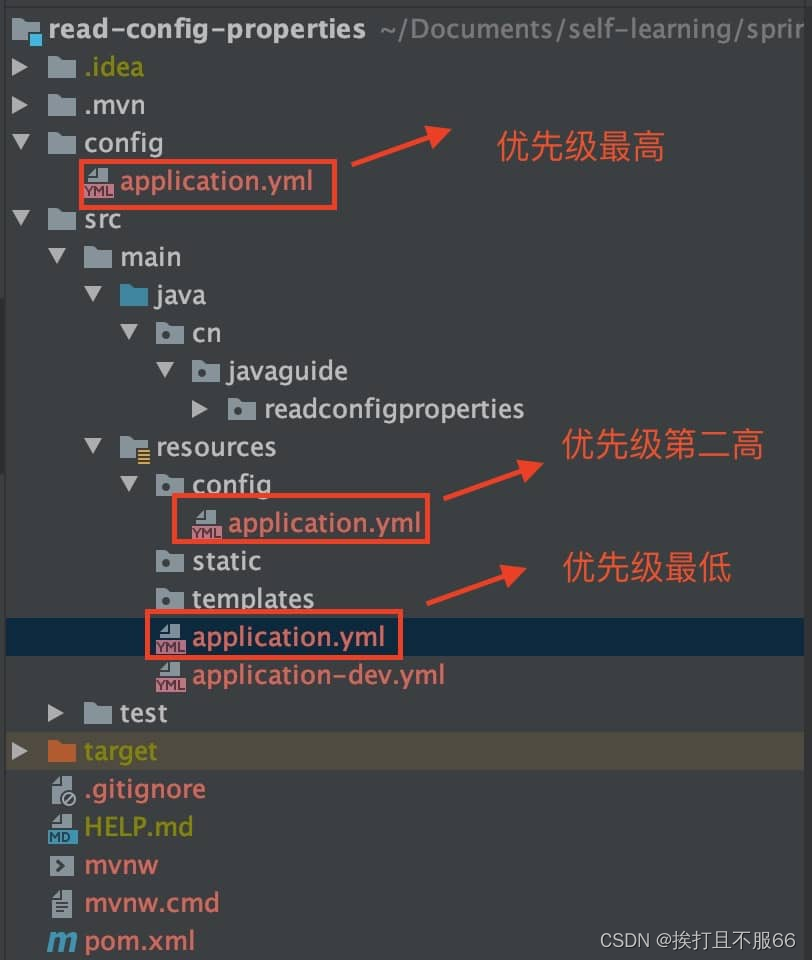
Spring——Spring读取文件
文章目录 1.通过 value 读取比较简单的配置信息2.通过ConfigurationProperties读取并与 bean 绑定3.通过ConfigurationProperties读取并校验4. PropertySource 读取指定 properties 文件5.题外话:Spring加载配置文件的优先级 很多时候我们需要将一些常用的配置信息比如阿里云os…...
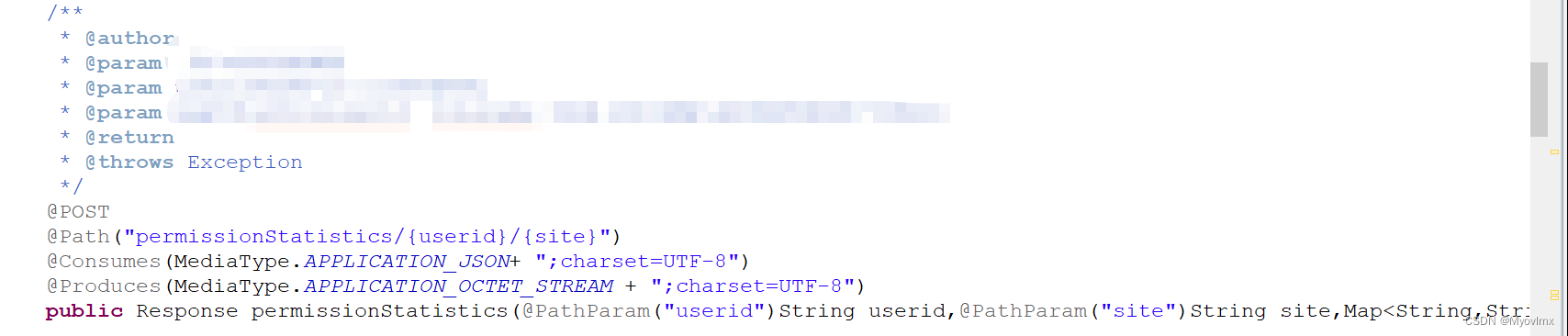
这是一条求助贴(postman测试的时候一直是404)
看到这个问题是404的时候总感觉不该求助大家,404多常见一看就是简单的路径问题,我的好像不是,我把我的问题奉上。 首先我先给出我的url http://10.3.22.195:8080/escloud/rest/escloud_contentws/permissionStatistics/jc-haojl/sz 这是我…...
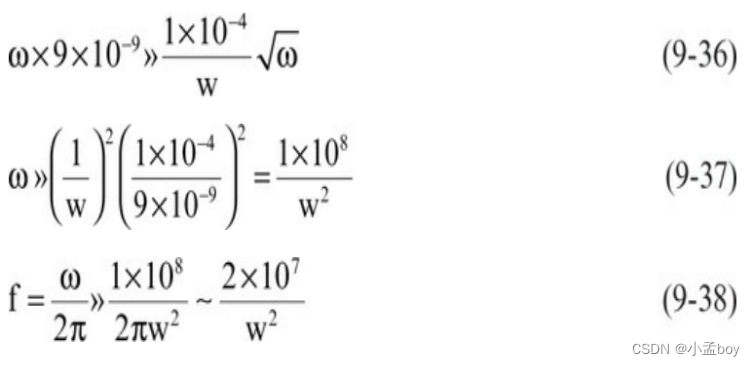
信号完整性分析基础知识之有损传输线、上升时间衰减和材料特性(四):有损传输线建模
传输线中信号衰减的两个损耗过程是通过信号和返回路径导体的串联电阻以及通过有损耗介电材料的分流电阻。这两个电阻器的电阻都与频率相关。 值得注意的是,理想电阻器的电阻随频率恒定。我们已经证明,在理想的有损传输线中,用于描述损耗的两个…...
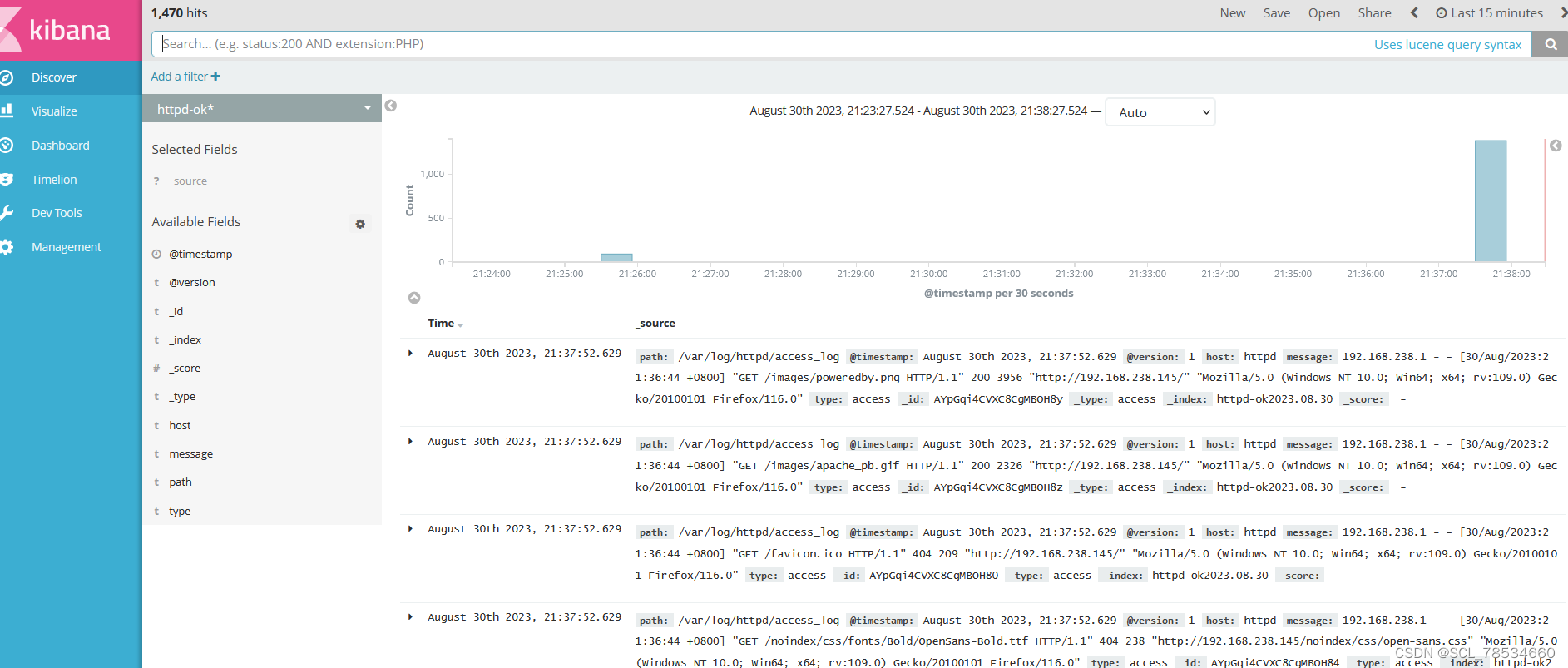
elk日志收集系统
目录 前言 一、概述 二、案例 (一)、环境配置 安装node1与node2节点的elasticsearch node1的elasticsearch-head插件 (二)、node1服务器安装logstash 测试1: 标准输入与输出 测试2:使用rubydebug解…...

perl 语言中 AUTOLOAD 的用法
这里的 AUTOLOAD可以理解为自动加载。具体来说就是,在正常情况下,我们不能调用一个尚未定义的函数(子例程)。不过,如果在未定义函数的包中有一个名为 AUTOLOAD的函数,那么对未定义函数的调用都会路由至这个…...
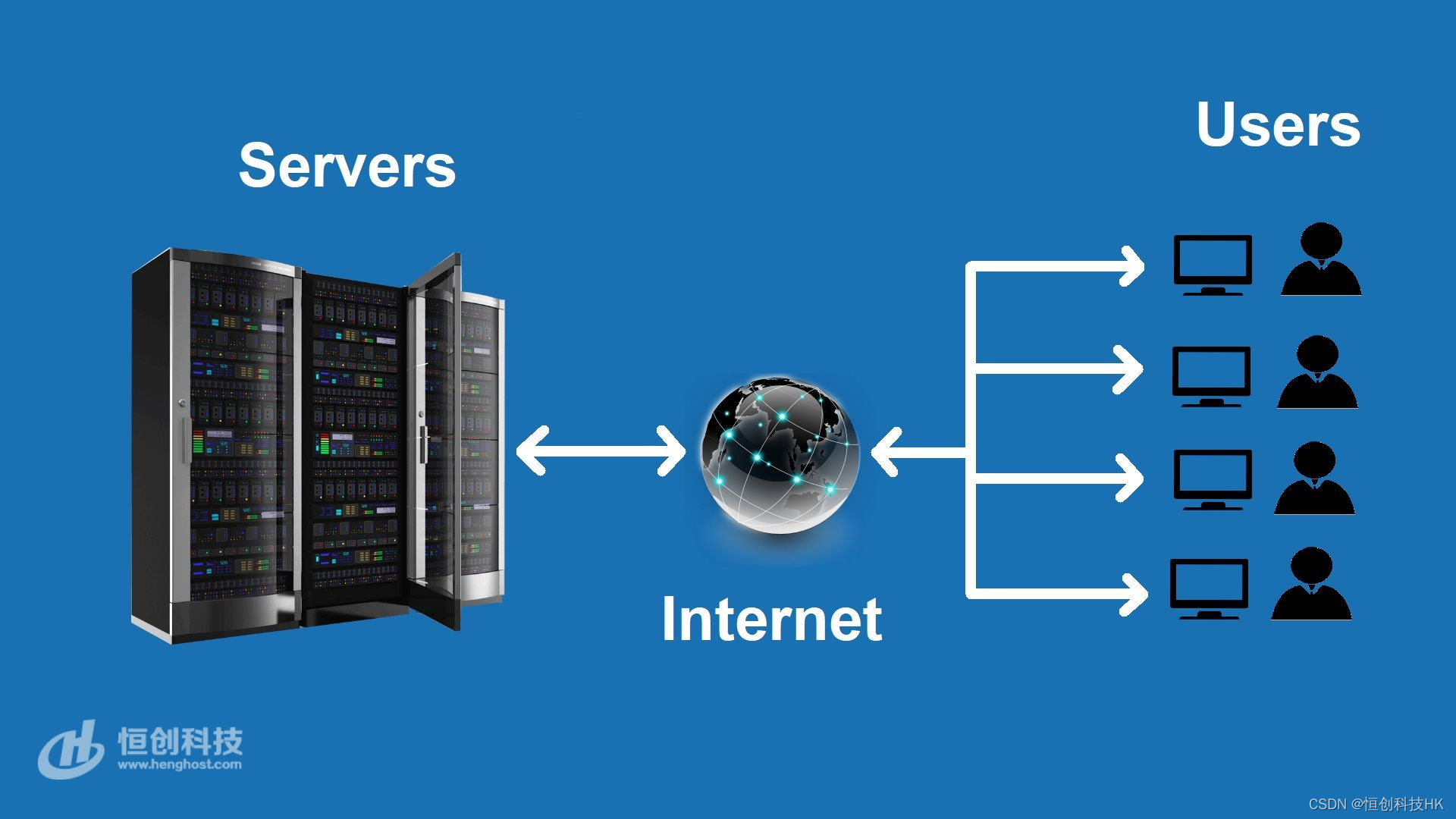
服务器放在香港好用吗?
相较于国内服务器,将网站托管在香港服务器上最直观的好处是备案层面上的。香港服务器上的网站无需备案,因此更无备案时限,购买之后即可使用。 带宽优势 香港服务器的带宽一般分为香港本地带宽和国际带宽、直连中国骨干网 CN2三种。香港…...
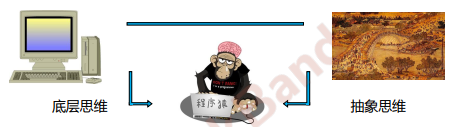
C++设计模式_01_设计模式简介(多态带来的便利;软件设计的目标:复用)
文章目录 本栏简介1. 什么是设计模式2. GOF 设计模式3. 从面向对象谈起4. 深入理解面向对象5. 软件设计固有的复杂性5.1 软件设计复杂性的根本原因5.2 如何解决复杂性 ? 6. 结构化 VS. 面向对象6.1 同一需求的分解写法6.1.1 Shape1.h6.1.2 MainForm1.cpp 6.2 同一需求的抽象的…...

Docker技术--WordPress博客系统部署初体验
如果使用的是传统的项目部署方式,你要部署WordPress博客系统,那么你需要装备一下的环境,才可以部署使用。 -1:操作系统linux -2:PHP5.6或者是更高版本环境 -3:MySQL数据环境 -4:Apache环境 但是如果使用Docker技术,那么就只需要进行如下的几行简单的指令: docker run …...

提高代码可读性和可维护性的命名建议
当进行接口自动化测试时,良好的命名可以提高代码的可读性和可维护性。以下是一些常用的命名建议: 变量和函数命名: 使用具有描述性的名称,清晰地表达变量或函数的用途和含义。使用小写字母和下划线来分隔单词,例如 log…...
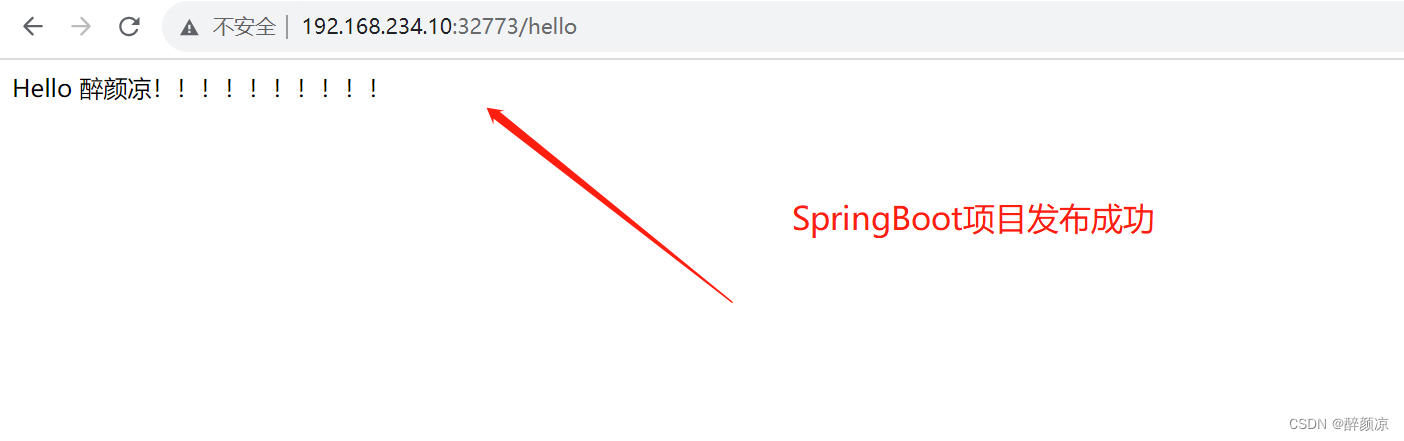
Docker基础入门:Docker网络与微服务项目发布
Docker基础入门:Docker网络与微服务项目发布 一、前言二、Docker0理解2.1 ip a查看当前网络环境2.2 实战--启动一个tomact01容器(查看网络环境)2.3 实战--启动一个tomact02容器(查看网络环境)2.4 容器与容器之间的通信…...
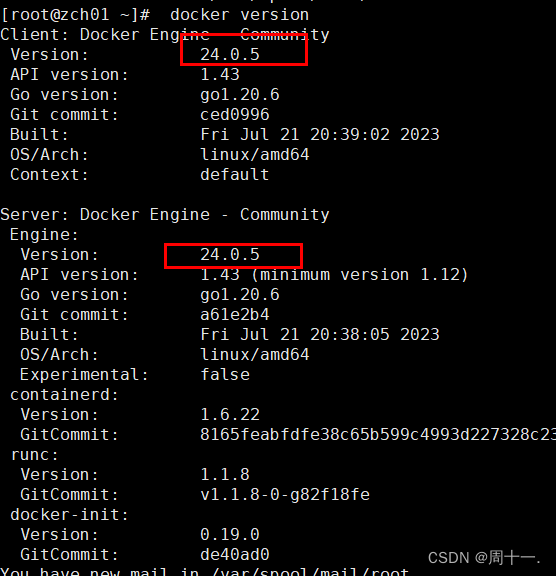
Docker安装详细步骤
Docker安装详细步骤 1、安装环境准备 主机:192.168.40.5 zch01 设置主机名 # hostnamectl set-hostname zch01 && bash 配置hosts文件 [root ~]# vi /etc/hosts 添加如下内容: 192.168.40.5 zch01 关闭防火墙 [rootzch01 ~]# systemct…...
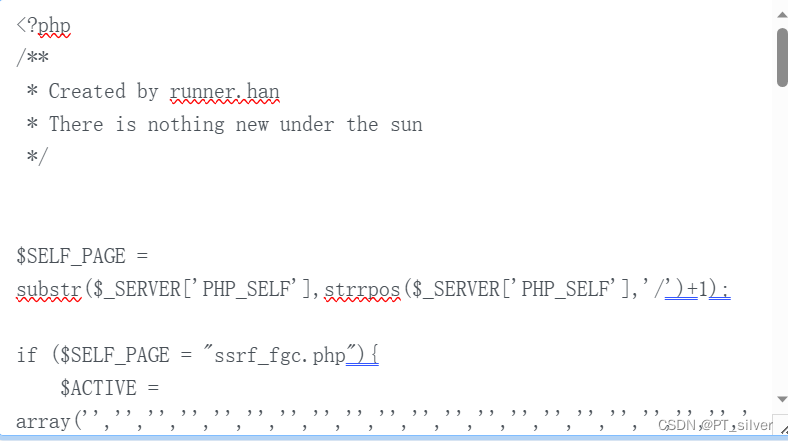
十六、pikachu之SSRF
文章目录 1、SSRF概述2、SSRF(URL)3、SSRF(file_get_content) 1、SSRF概述 SSRF(Server-Side Request Forgery:服务器端请求伪造):其形成的原因大都是由于服务端提供了从其他服务器应用获取数据的功能&…...
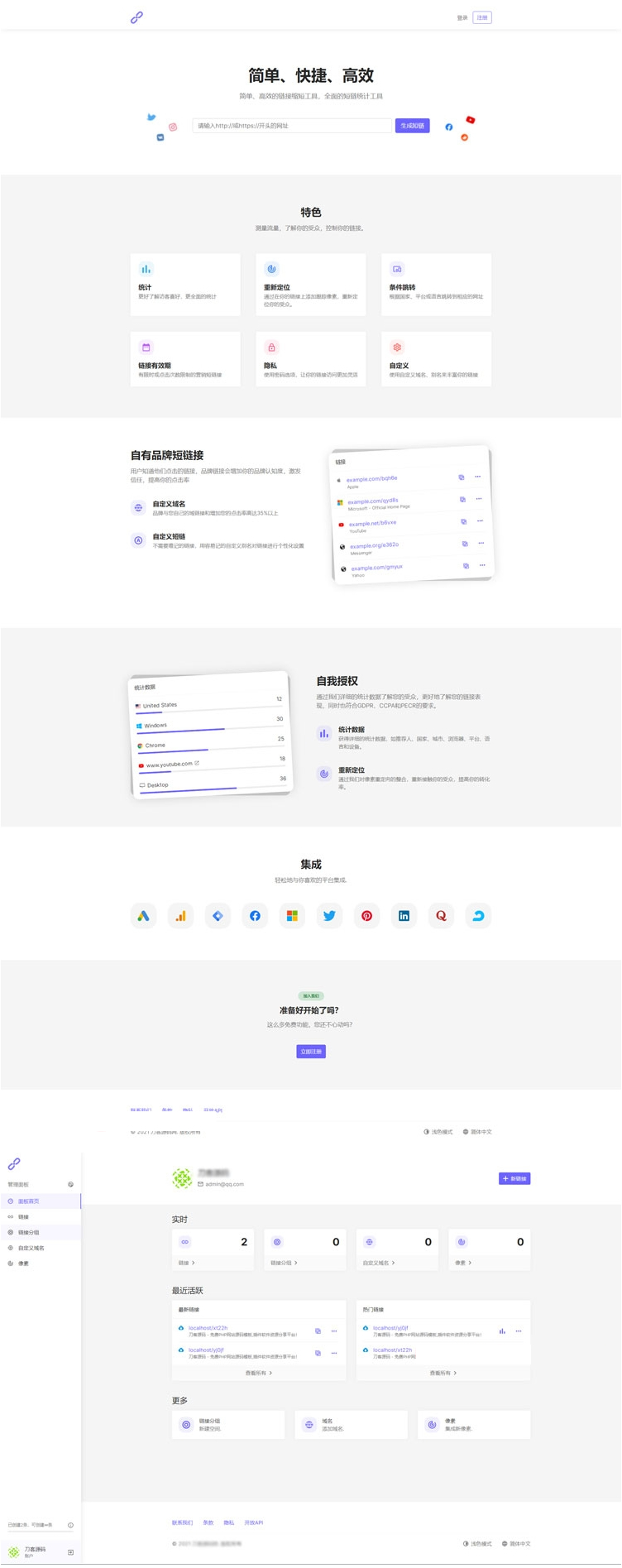
最新PHP短网址生成系统/短链接生成系统/URL缩短器系统源码
全新PHP短网址系统URL缩短器平台,它使您可以轻松地缩短链接,根据受众群体的位置或平台来定位受众,并为缩短的链接提供分析见解。 系统使用了Laravel框架编写,前后台双语言使用,可以设置多域名,还可以开设套…...
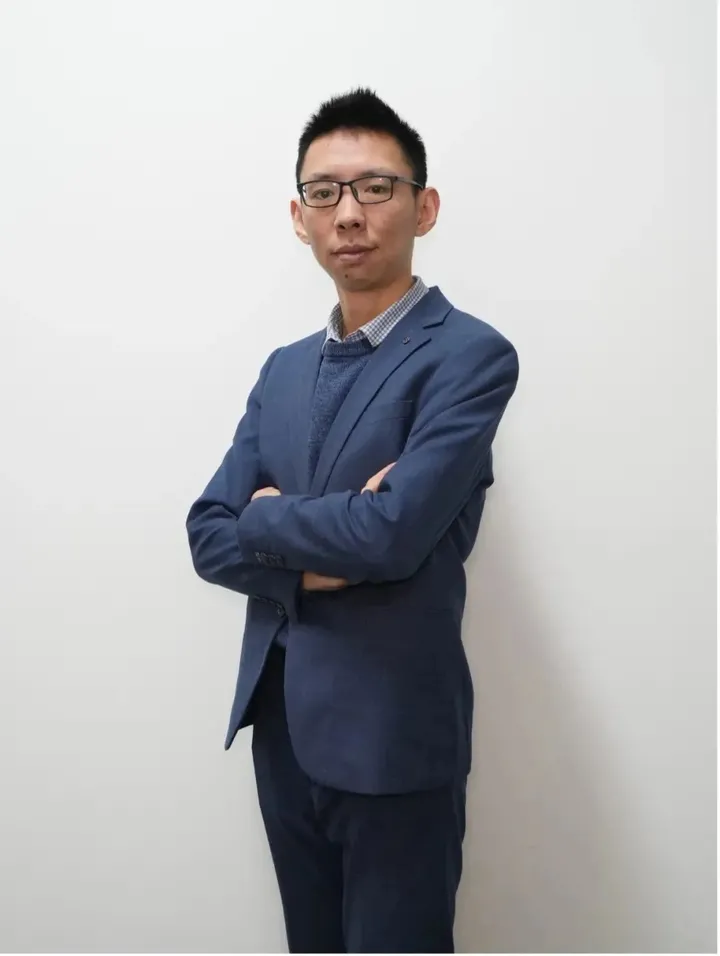
漱玉平民大药房:多元化药店变革的前夜
作者 | 王聪彬 编辑 | 舞春秋 来源 | 至顶网 本文介绍了漱玉平民大药房在药品零售领域的数字化转型和发展历程。通过技术创新, 漱玉平民 建设了覆盖医药全生命周期的大健康生态圈,采用混合云架构和国产分布式数据库 TiDB,应对庞大的会员数据处…...