【Node.js】http 模块
1. http 模块
import http from 'http'
// 创建本地服务器接收数据
const server = http.createServer((req, res) => {console.log(req.url)res.writeHead(200, { 'Content-Type': 'application/json' // 'Content-Type': 'text/html;charset=utf-8' // 将内容以 html 标签和 utf-8 的形式展示到网页上 })// write 中的内容直接展示到网页上// res.write('hello')res.end(JSON.stringify({data: "hello"}))
})
server.listen(8000,()=> {console.log("server is running")
})
1.1 解决跨域问题
接口 jsonp 解决跨域
// server.js
const http = require('http')
const url = require('url')const app = http.createServer((req, res) => {let urlObj = url.parse(req.url, true)console.log(urlObj.query.callback)switch (urlObj.pathname) {case '/api/user':res.end(`${urlObj.query.callback}(${JSON.stringify({name:'xxx',age:18})})`)breakdefault:res.end('404.')break}
})app.listen(3000, () => {console.log('localhost:3000')
})
<!-- index.html -->
<!DOCTYPE html>
<html lang="en"><head><meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><title>Document</title>
</head><body><script>const oscript = document.createElement('script');oscript.src = 'http://localhost:3000/api/user?callback=test';document.body.appendChild(oscript);function test(obj) {console.log(obj)}</script></body></html>
CORS 解决跨域
// server.js
const http = require('http')
const url = require('url')const app = http.createServer((req, res) => {let urlObj = url.parse(req.url, true)// console.log(urlObj.query.callback)res.writeHead(200, {'Content-Type': 'application/json; charset=utf-8',// CORS 头'Access-Control-Allow-Origin': '*'})switch (urlObj.pathname) {case '/api/user':res.end(`${JSON.stringify({ name: 'xxx', age: 18 })}`)breakdefault:res.end('404.')break}
})app.listen(3000, () => {console.log('localhost:3000')
})
<!-- index.html -->
<!DOCTYPE html>
<html lang="en"><head><meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><title>Document</title>
</head><body><script>fetch('http://localhost:3000/api/user').then(res=>res.json()).then(res=>console.log(res))</script></body></html>
1.2 作为客户端
Node.js 既可以做服务端开发,又可以做客户端开发。
get
<!-- index.html -->
<!DOCTYPE html>
<html lang="en"><head><meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><title>Document</title>
</head><body><script>fetch('http://localhost:3000/api/user').then(res=>res.json()).then(res=>console.log(res))</script>
</body></html>
// get.js
const http = require('http')
const https = require('https')
const url = require('url')const app = http.createServer((req, res) => {let urlObj = url.parse(req.url, true)// console.log(urlObj.query.callback)res.writeHead(200, {'Content-Type': 'application/json; charset=utf-8',// CORS 头'Access-Control-Allow-Origin': '*'})switch (urlObj.pathname) {case '/api/user':// 现在作为客户端 去猫眼api请求数据// 注意协议要统一:https还是httphttpget(res)breakdefault:res.end('404.')break}
})
app.listen(3000, () => {console.log('localhost:3000')
})
function httpget(response) {let data = ''https.get(`https://i.maoyan.com/api/mmdb/movie/v3/list/hot.json?ct=%E7%9F%B3%E5%AE%B6%E5%BA%84&ci=76&channelId=4`,res => {// data 是一份一份的数据收集,end 是最终收集到的所有数据res.on('data', chunk => {data += chunk})res.on('end', () => {console.log(data)response.end(data)})})
}
另一种写法:
// get.js
const http = require('http')
const https = require('https')
const url = require('url')const app = http.createServer((req, res) => {let urlObj = url.parse(req.url, true)// console.log(urlObj.query.callback)res.writeHead(200, {'Content-Type': 'application/json; charset=utf-8',// CORS 头'Access-Control-Allow-Origin': '*'})switch (urlObj.pathname) {case '/api/user':// 现在作为客户端 去猫眼api请求数据// 注意协议要统一:https还是http// data 收集好的时候调用内部传入的 cb 函数httpget((data)=> {res.end(data)})breakdefault:res.end('404.')break}
})
app.listen(3000, () => {console.log('localhost:3000')
})
function httpget(cb) {let data = ''https.get(`https://i.maoyan.com/api/mmdb/movie/v3/list/hot.json?ct=%E7%9F%B3%E5%AE%B6%E5%BA%84&ci=76&channelId=4`,res => {// data 是一份一份的数据收集,end 是最终收集到的所有数据res.on('data', chunk => {data += chunk})res.on('end', () => {console.log(data)cb(data)})})
}
post
<!-- index.html -->
<!DOCTYPE html>
<html lang="en"><head><meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><title>Document</title>
</head><body><script>fetch('http://localhost:3000/api/user').then(res=>res.json()).then(res=>console.log(res))</script></body></html>
// post.js
const http = require('http')
const https = require('https')
const url = require('url')const app = http.createServer((req, res) => {let urlObj = url.parse(req.url, true)// console.log(urlObj.query.callback)res.writeHead(200, {'Content-Type': 'application/json; charset=utf-8',// CORS 头'Access-Control-Allow-Origin': '*'})switch (urlObj.pathname) {case '/api/user':// 现在作为客户端 去小米优品 api 请求数据// 注意协议要统一:https还是httphttpPost((data) => {res.end(data)})breakdefault:res.end('404.')break}
})
app.listen(3000, () => {console.log('localhost:3000')
})
function httpPost(cb) {let data = ''const options = {hostname: 'm.xiaomiyoupin.com',port: '443', // 80 是 http 默认端口号,443 是 https 默认端口号path: '/mtop/market/search/placeHolder',methods: "POST",headers: {"Content-Type": "application/json",}}const req = https.request(options, (res) => {res.on('data', (chunk) => {data += chunk})res.on('end', () => {cb(data)})})req.write(JSON.stringify([{}, { baseParam: { ypClient: 1 } }]))req.end()
}
1.3 爬虫
相关文章:

【Node.js】http 模块
1. http 模块 import http from http // 创建本地服务器接收数据 const server http.createServer((req, res) > {console.log(req.url)res.writeHead(200, { Content-Type: application/json // Content-Type: text/html;charsetutf-8 // 将内容以 html 标签和 utf-8 的…...
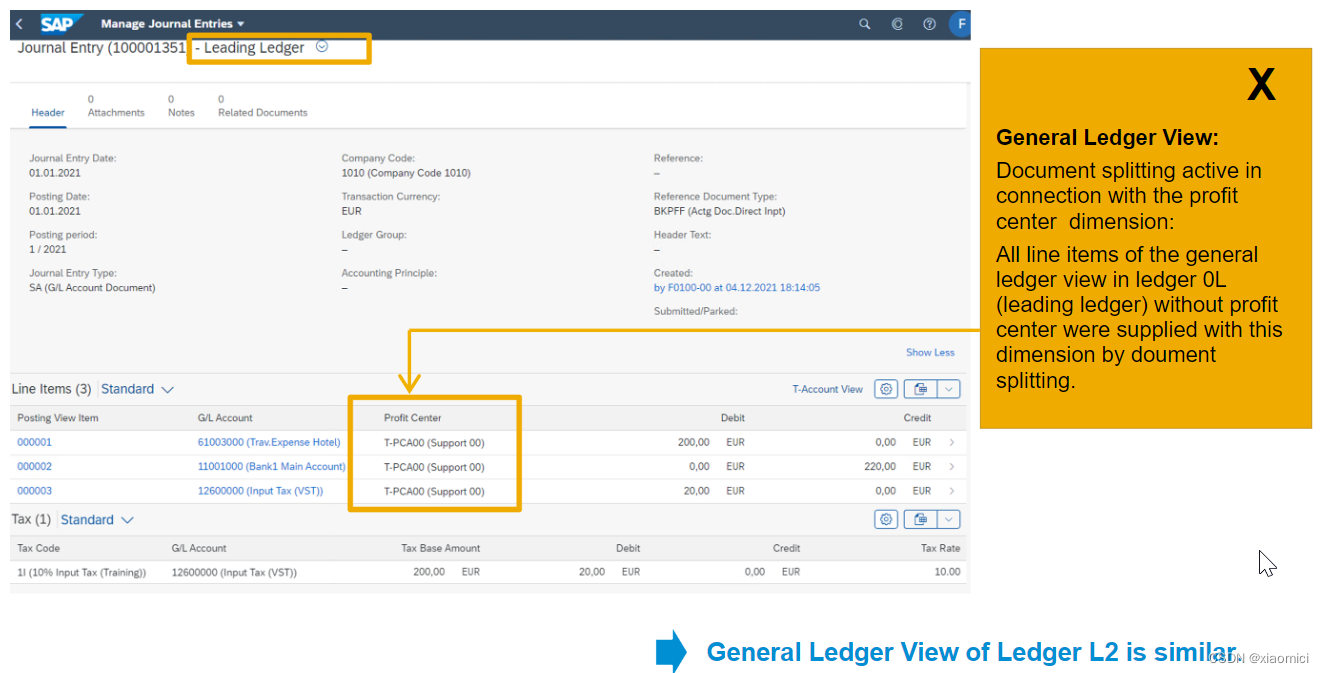
S/4 HANA 大白话 - 财务会计-2 总账主数据
接下来看看财务模块的一些具体操作。 总账相关主数据 公司每天运转,每天办公室有租金,有水电费,有桌椅板凳损坏,鼠标损坏要换,有产品买卖,有收入。那么所有这些都得记下来。记哪里?记在总账里…...
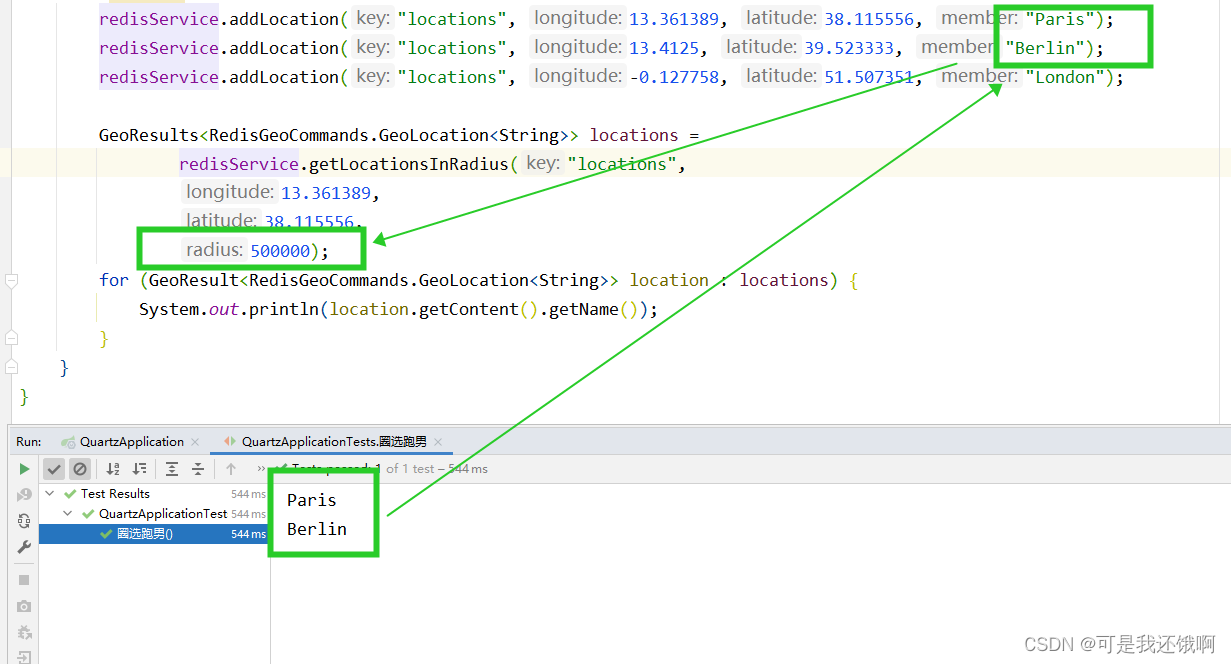
Redis根据中心点坐标和半径筛选符合的数据
目录 1.启动Redis编辑 2.导入maven依赖 3.添加redis配置 4.编写RedisService 5.使用 6.验证 1.启动Redis 2.导入maven依赖 <dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-data-redis</artifac…...

springboot 集成 zookeeper 问题记录
springboot 集成 zookeeper 问题记录 环境 springboot - 2.7.8 dubbo - 3.1.11 dubbo-dependencies-zookeeper-curator5 - 3.1.11 模拟真实环境,将 windows 上的 zookeeper 迁移到虚拟机 linux 的 docker 环境 failed to connect to zookeeper server 迁移到…...
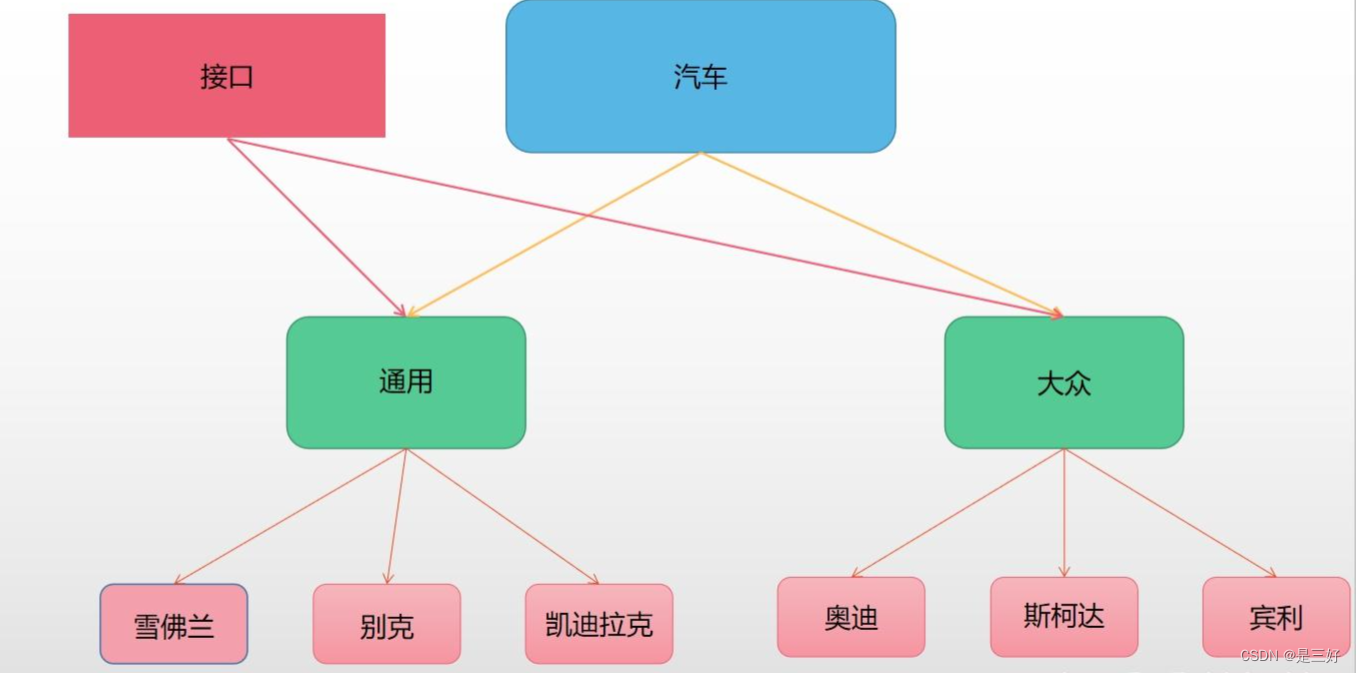
java中的接口interface
一、面向对象基本概念 Java是一种面向对象的语言,其中「对象」就相当于是现实世界中的一个个具体的例子,而「类」就相当于是一个抽象的模板,将抽象的概念模板转化为具体的例子的过程就叫做「实例化」。 比如说人这个概念就是一个抽象化的「…...

多个git提交,只推送其中一个到远程该如何处理
用新分支去拉取当前分支的指定commit记录,之后推送到当前分支远程仓库实现推送指定历史提交的功能 1.查看当前分支最近五次提交日志 git log --oneline -5 2.拉取远程分支创建临时本地分支 localbranch 为本地分支名 origin/dev 为远程目标分支 git checkout …...

uniapp中input的disabled属性
uniapp中input的disabled属性: 小程序中兼容性好; 在H5中兼容性差; 在H5中使用uniapp的input的disabled属性,属性值只能是true或false,如果为0, "都会为true; <input class"in…...
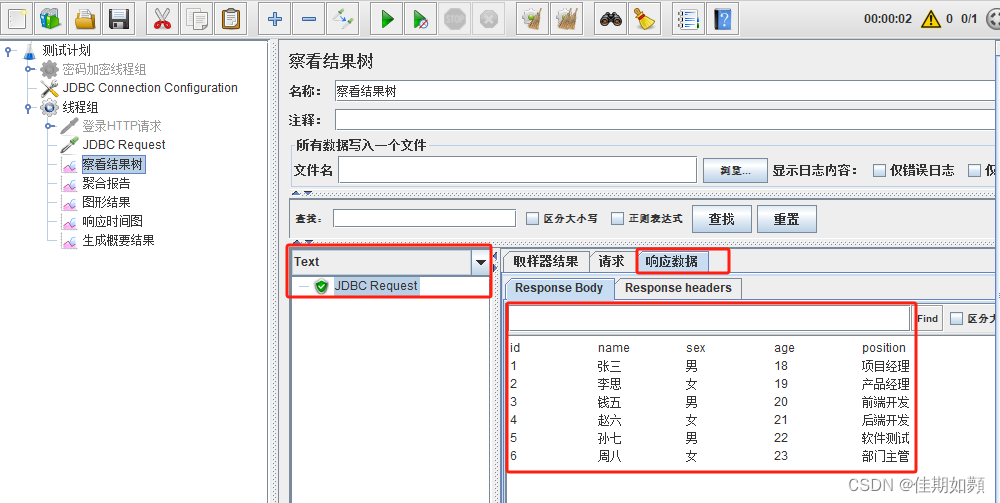
Jmeter连接mysql数据库详细步骤
一、一般平常工作中使用jmeter 连接数据库的作用 主要包括: 1、本身对数据库进行测试(功能、性能测试)时会需要使用jmeter连接数据库 2、功能测试时,测试出来的结果需要和数据库中的数据进行对比是否正确一致。这时候可以通过j…...
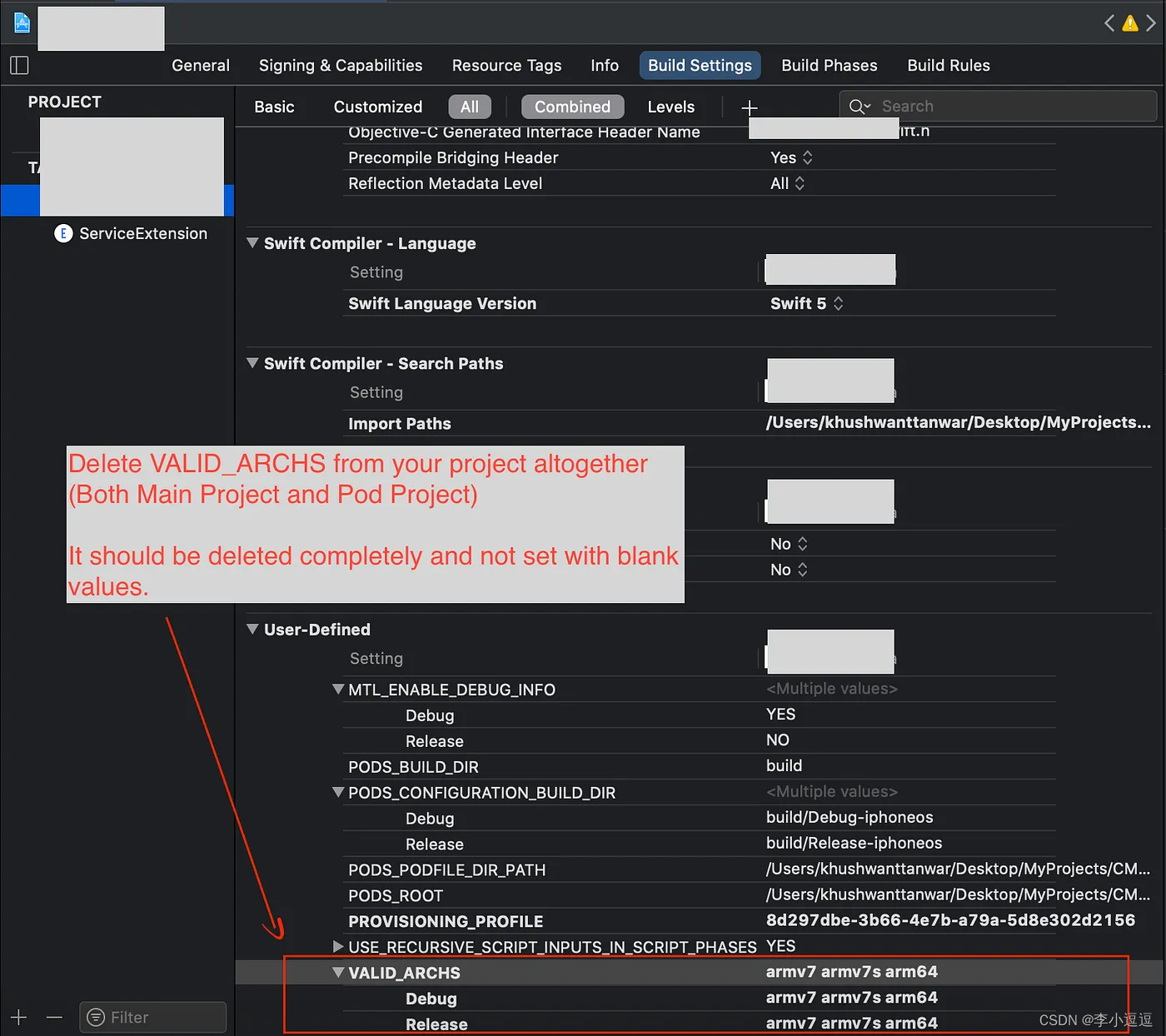
Xcode 14.3.1build 报错整理
1、Command PhaseScriptExecution failed with a nonzero exit code 2、In /Users/XX/XX/XX/fayuan-mediator-app-rn/ios/Pods/CocoaLibEvent/lib/libevent.a(buffer.o), building for iOS Simulator, but linking in object file built for iOS, file /Users/XX/XX/XX/fayuan…...
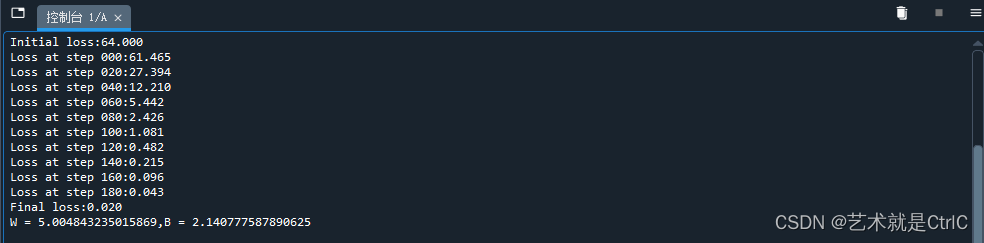
TensorFlow入门(十三、动态图Eager)
一个图(Graph)代表一个计算任务,且在模型运行时,需要把图放入会话(session)里被启动。一旦模型开始运行,图就无法修改了。TensorFlow把这种图一般称为静态图。 动态图是指在Python中代码被调用后,其操作立即被执行的计算。 它与静态图最大的区别是不需要使用session来建立会话…...
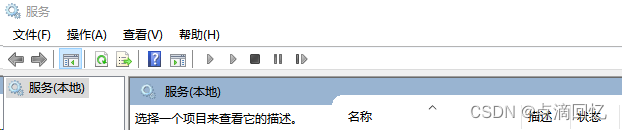
批量执行insert into 的脚本报2006 - MySQL server has gone away
数据库执行批量数据导入是报“2006 - MySQL server has gone away”错误,脚本并没有问题,只是insert into 的批量操作语句过长导致。 解决办法: Navicat ->工具 ->服务器监控->mysql ——》变量 修改max_allowed_packet大小为512…...
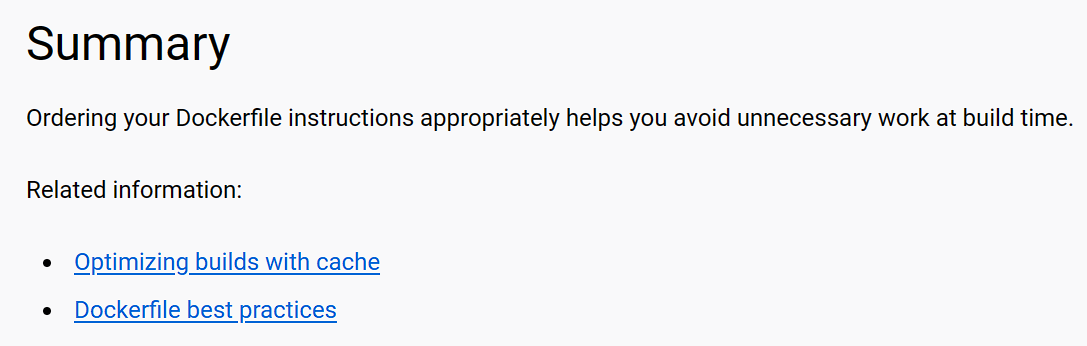
翻译docker官方文档(残缺版)
Build with docker(使用 Docker 技术构建应用程序或系统镜像) Overview (概述) 介绍(instruction) 层次结构(Layers) The order of Dockerfile instructions matters. A Docker build consists of a series of ordered build ins…...
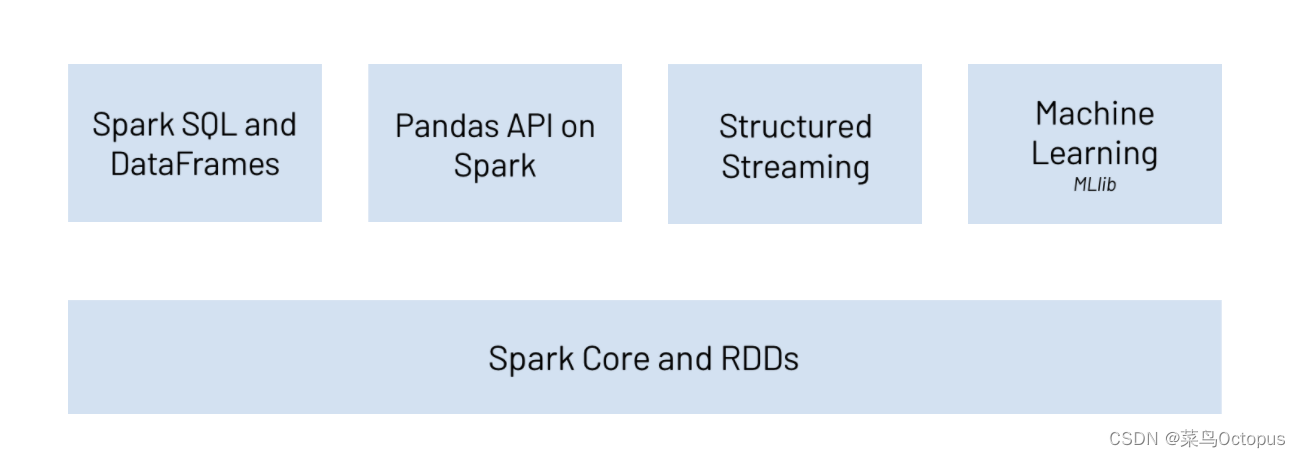
PySpark 概述
文章最前: 我是Octopus,这个名字来源于我的中文名--章鱼;我热爱编程、热爱算法、热爱开源。所有源码在我的个人github ;这博客是记录我学习的点点滴滴,如果您对 Python、Java、AI、算法有兴趣,可以关注我的…...

『heqingchun-ubuntu系统下Qt报错connot find -lGL解决方法』
ubuntu系统下Qt报错connot find -lGL解决方法 问题: Qt报错 connot find -lGL collect2:error:ld returned 1 exit status 解决方式: cd /usr/lib/x86_64-linux-gnu查看一下 ls | grep libGLlibGLdispatch.so.0 libGLdispatch.so.0.0.0 libGLESv2.so.…...

代码整洁之道:程序员的职业素养(十六)
辅导、学徒期与技艺 导师的重要性在职业发展中是不可低估的。尽管最好的计算机科学学位教学计划可以提供坚实的理论基础,但面对实际工作中的挑战,年轻毕业生往往需要更多指导。幸运的是,有许多优秀的年轻人可以通过观察和模仿他们的导师来快…...
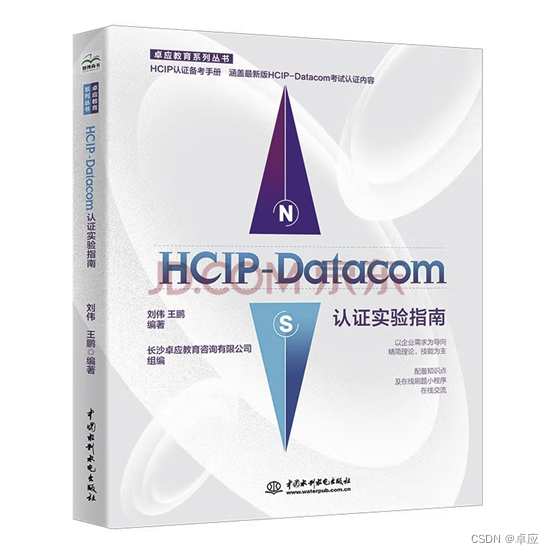
OSPF的原理与配置
第1章 OSPF[1] 本章阐述了OSPF协议的特征、术语,OSPF的路由器类型、网络类型、区域类型、LSA类型,OSPF报文的具体内容及作用,描述了OSPF的邻居关系,通过实例让读者掌握OSPF在各种场景中的配置。 本章包含以下内容: …...
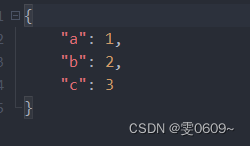
uni-app : 生成三位随机数、自定义全局变量、自定义全局函数、传参、多参数返回值
核心代码 function generateRandomNumber() {const min 100;const max 999;// 生成 min 到 max 之间的随机整数// Math.random() 函数返回一个大于等于 0 且小于 1 的随机浮点数。通过将其乘以 (max - min 1),我们得到一个大于等于 0 且小于等于 (max - min 1…...

EF core 如何撤销对对象的更改
一般情况下 DB.SaveChanges() 就可以正常提交更改了. 但是如何撤销更改, 可以使用下面的代码. //撤销更改 //放弃更改. 防止后面的finally出错 DB.ChangeTracker.Entries().Where(e > e.Entity ! null).ToList().ForEach(e > e.State EntityState.Detached);...

以字符串mark作为分隔符,对字符串s进行分割
int main() {string s "How are you?";string mark " ";string tmp;int cur 0, first 0;//找到第一个标记while ((cur s.find_first_of(mark, cur)) ! string::npos){//获取第一个标记前的子串tmp s.substr(first, cur - first);cout << tmp …...
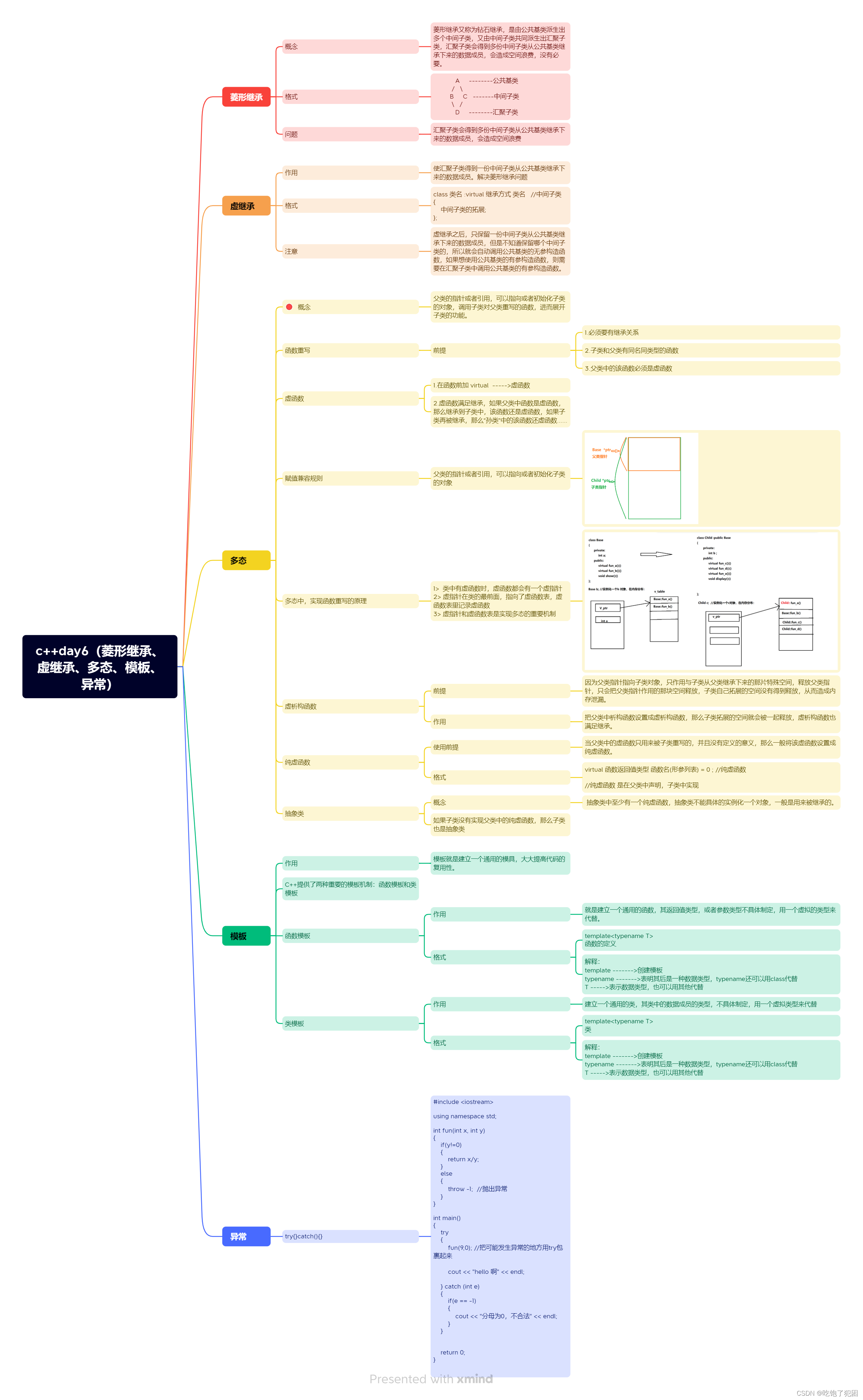
c++day6(菱形继承、虚继承、多态、模板、异常)
今日任务 1.思维导图 2.编程题: 代码: #include <iostream>using namespace std; /*以下是一个简单的比喻,将多态概念与生活中的实际情况相联系: 比喻:动物园的讲解员和动物表演 想象一下你去了一家动物园&a…...
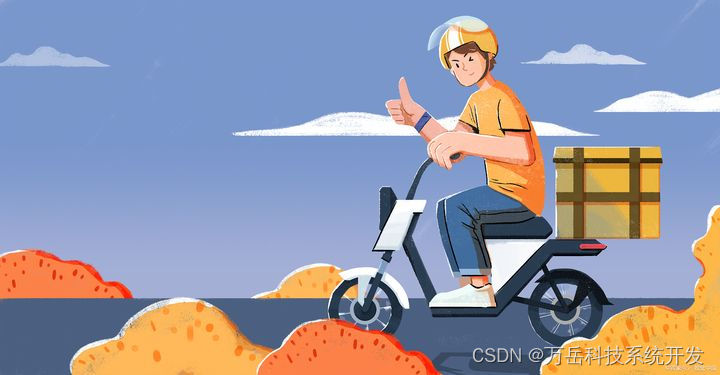
外卖跑腿系统开发的最佳实践和成功案例
外卖跑腿系统的开发既涉及技术实现,也需要考虑用户体验、运营策略和合规性。以下是一些最佳实践和一些成功的案例,以帮助您更好地理解这个领域的要点。 1. 技术框架的选择 选择适合的技术框架是外卖跑腿系统成功的关键。您可以考虑使用以下技术&#…...

python中的range()函数详解
range() 是 Python 内置的一个函数,用于生成一个整数序列。 range([start], [stop], [step])start、stop、step 分别表示序列的起始值、终止值和步长。start 和 step 是可选参数,如果不指定则默认为 0 和 1。 一、range()传递不…...

【taro react】 ---- 常用自定义 React Hooks 的实现【四】之遮罩层
1. 问题场景 在实际开发中我们会遇到一个遮罩层会受到多个组件的操作影响,如果我们不采用 redux 之类的全局状态管理,而是选择组件之间的值传递,我们就会发现使用组件的变量来控制组件的显示和隐藏很不方便,更不要说像遮罩层这样一个项目多处使用的公共组件,他的隐藏和显示…...

【git】git命令行
首先要了解git整个流程的一个分类: workspace:工作区staging area:暂存区/缓存区local repository:版本库或本地仓库remote repository:远程仓库 创建仓库 git clone gitgithub.comxxxxxxxxxxxx//拷贝一份远程仓库 …...

centos8 jenkins 搭建和使用
一、安装jenkins 直接war包搭建下载地址:https://get.jenkins.io/war-stable/ 下载稳定长期版本 二、jenkins 启动依赖java, 安装java sdk ,好像支持java 11和17版本,21版本不支持会报错 下载sdk地址,https://www.oracle.com/j…...

Hive实战(03)-深入了解Hive JDBC:在大数据世界中实现数据交互
在大数据领域,Hive作为一种数据仓库解决方案,为用户提供了一种SQL接口来查询和分析存储在Hadoop集群中的数据。为了更灵活地与Hive进行交互,我们可以使用Hive JDBC(Java Database Connectivity)驱动程序。本文将深入探…...
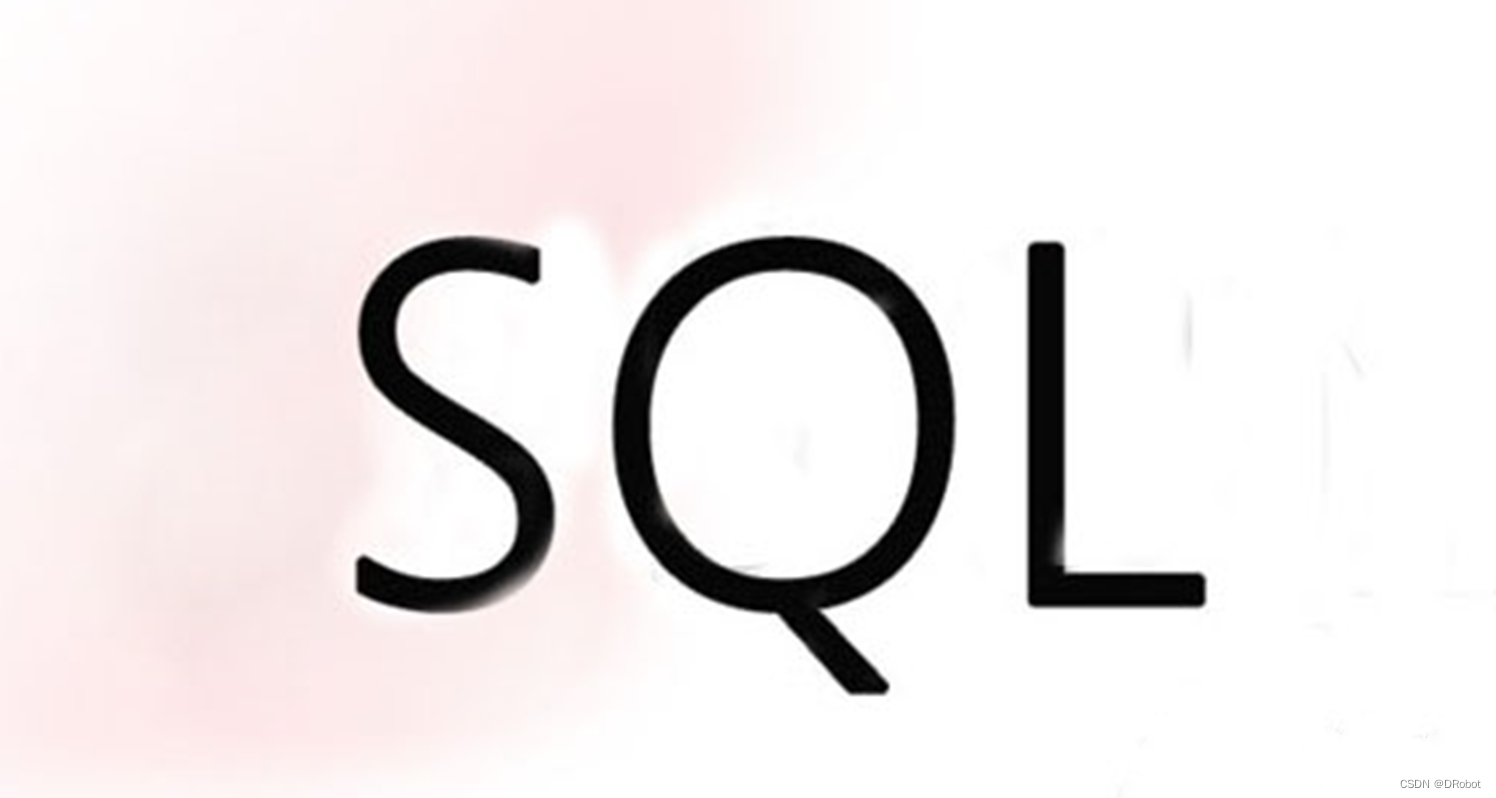
SQL开发笔记之专栏介绍
Sql是用于访问和处理数据库的标准计算机语言,使用SQL访问和处理数据系统中的数据,这类数据库包括:Mysql、PostgresSql、Oracle、Sybase、DB2等等,数据库无非围绕着“增删改查”的核心业务进行开发。并且目前绝大多数的后端程序开发…...
华为OD机考算法题:找终点
目录 题目部分 解读与分析 代码实现 题目部分 题目找终点难度易题目说明给定一个正整数数组,设为nums,最大为100个成员,求从第一个成员开始,正好走到数组最后一个成员,所使用的最少步骤数。 要求: 1.第…...

el-table通过scope.row获取表格每列的值,以及scope.$index
<el-table-column type"selection" width"55"></el-table-column><el-table-column prop"id" label"ID" width"80"></el-table-column><el-table-column prop"name" label"文件名…...

uni-app:本地缓存的使用
uni-app 提供了多种方法用于本地缓存的操作。下面是一些常用的 uni-app 本地缓存方法: uni.setStorageSync(key, data): 同步方式将数据存储到本地缓存中,可以使用对应的 key 来获取该数据。 uni.setStorage({key, data}): 异步方式将数据存储到本地缓存…...