第十五章 Nest Pipe(内置及自定义)
NestJS的Pipe是一个用于数据转换和验证的特殊装饰器。Pipe可以应用于控制器(Controller)的处理方法(Handler)和中间件(Middleware),用于处理传入的数据。它可以用来转换和验证数据,确保数据的有效性和一致性。
内置的 Pipe 是指在使用 NestJS 框架时,已经预定义好的一些管道,用于验证和转换传入的数据。
- ValidationPipe(验证管道):用于验证传入的数据是否符合指定的规则,可以使用 class-validator 和 class-transformer 库来实现验证和转换。
- ParseIntPipe(整数转换管道):用于将传入的字符串转换为整数。
- ParseBoolPipe(布尔值转换管道):用于将传入的字符串转换为布尔值。
- ParseArrayPipe(数组转换管道):用于将传入的字符串转换为数组。
- ParseUUIDPipe(UUID 转换管道):用于将传入的字符串转换为 UUID。
- DefaultValuePipe(默认值管道):用于在传入的参数不存在时,给参数设置一个默认值。
- ParseEnumPipe(枚举转换管道):用于将传入的字符串转换为枚举类型。
- ParseFloatPipe(浮点数转换管道):用于将传入的字符串转换为浮点数。
- ParseFilePipe(文件转换管道):用于将传入的文件转换为上传的文件对象。
接下来我们测试一下内置 Pipe 的功能:
创建项目
nest new pipe -p npm
修改 app.controller.ts 可以看到这个接口默认接收test的字符串参数
import { Controller, Get, Query } from '@nestjs/common';
import { AppService } from './app.service';@Controller()
export class AppController {constructor(private readonly appService: AppService) { }@Get()getHello(@Query('test') test: string): string {return test;}
}
1、ParseIntPipe
我们可以使用ParseIntPipe 将接收的字符串参数转为整数:
import { Controller, Get, ParseIntPipe, Query } from '@nestjs/common';
import { AppService } from './app.service';@Controller()
export class AppController {constructor(private readonly appService: AppService) { }@Get()getHello(@Query('test', ParseIntPipe) test: string): string {return test+10;}
}
访问接口 http://localhost:3000/?test=100 可以看到参数test传入 100 变成了 110
且当我们传入的参数不能转为init时 则会报错 例如下面 访问 http://localhost:3000/?test=ww
这个返回的报错 我们也可以自定义 但是这种方式需要使用new XxxPipe 的方式 例如指定状态码为404
import { Controller, Get, HttpStatus, ParseIntPipe, Query } from '@nestjs/common';
import { AppService } from './app.service';@Controller()
export class AppController {constructor(private readonly appService: AppService) { }@Get()getHello(@Query('test', new ParseIntPipe({errorHttpStatusCode: HttpStatus.NOT_FOUND,})) test: string): string {return test + 10;}
}
同时也可以抛出异常再进行处理
import { Controller, Get, HttpException, HttpStatus, ParseIntPipe, Query } from '@nestjs/common';
import { AppService } from './app.service';@Controller()
export class AppController {constructor(private readonly appService: AppService) { }@Get()getHello(@Query('test', new ParseIntPipe({errorHttpStatusCode: HttpStatus.NOT_FOUND,exceptionFactory(error) {console.log(error);throw new HttpException('测试报错' + error, HttpStatus.NOT_IMPLEMENTED)},})) test: string): string {return test + 10;}
}
2、ParseFloatPipe
ParseFloatPipe 的作用是将参数转为float 类型
我们新建一个接口: test
import { Controller, Get, HttpException, HttpStatus, ParseFloatPipe, ParseIntPipe, Query } from '@nestjs/common';
import { AppService } from './app.service';@Controller()
export class AppController {constructor(private readonly appService: AppService) { }@Get()getHello(@Query('test', new ParseIntPipe({errorHttpStatusCode: HttpStatus.NOT_FOUND,exceptionFactory(error) {console.log(error);throw new HttpException('测试报错' + error, HttpStatus.NOT_IMPLEMENTED)},})) test: string): string {return test + 10;}@Get('test')getTest(@Query('test', ParseFloatPipe) test: number) {return test + 10;}
}
访问 http://localhost:3000/test?test=10.4
同样可以new ParseFloatPipe 的形式,传入 errorHttpStatusCode 和 exceptionFactory
3、ParseBoolPipe
用于将传入的字符串转换为布尔值。
@Get('boolean')getBoolean(@Query('test') test: boolean) {return test;}
访问 http://localhost:3000/boolean?test=false
4、ParseArrayPipe
用于将传入的字符串转换为数组。
@Get('array')getArray(@Query('test', ParseArrayPipe) test: number[]) {return test.reduce((pre, cur) => pre + cur, 0);}
保存代码可以发现报错了 提示需要class-validator 这个包class-class-class-validator 允许使用基于装饰器和非装饰器的验证。 内部使用 validator.js 来执行验证。
pnpm install class-validator
安装之后启动 发现还是报错 缺少 class-transformer
class-transformer 允许您将普通对象转换为类的某个实例,反之亦然。 此外,它还允许根据条件序列化/反序列化对象。 这个工具在前端和后端都非常有用。
pnpm install class-transformer
访问 http://localhost:3000/array?test=1,2,3,4
可以发现 每一项都提取出来了但是类型还是string 没有转换成number类型
此时需要 new XxxPipe 的方式传入参数:
@Get('array')getArray(@Query('test', new ParseArrayPipe({items: Number,})) test: number[]) {return test.reduce((pre, cur) => pre + cur, 0);}
再次访问 http://localhost:3000/array?test=1,2,3,4
同时还可以指定传入时的分割符: separator: ‘/’,
@Get('array')getArray(@Query('test', new ParseArrayPipe({items: Number,separator: '/',})) test: number[]) {return test.reduce((pre, cur) => pre + cur, 0);}
访问 http://localhost:3000/array?test=1/2/3/4
当我们没有传入参数的时候会报错:
我们可以通过设置 optional 来实现不传参数不报错
@Get('array')getArray(@Query('test', new ParseArrayPipe({items: Number,separator: '/',optional: true,})) test: number[]) {return test}
5、ParseEnumPipe
用于将传入的字符串转换为枚举类型。
import { Controller, Get, HttpException, HttpStatus, ParseArrayPipe, ParseEnumPipe, ParseFloatPipe, ParseIntPipe, Query } from '@nestjs/common';
import { AppService } from './app.service';enum TestEnum {A = 'A111',B = 'B111',
}@Controller()
export class AppController {constructor(private readonly appService: AppService) { }@Get()getHello(@Query('test', new ParseIntPipe({errorHttpStatusCode: HttpStatus.NOT_FOUND,exceptionFactory(error) {console.log(error);throw new HttpException('测试报错' + error, HttpStatus.NOT_IMPLEMENTED)},})) test: string): string {return test + 10;}@Get('test')getTest(@Query('test', ParseFloatPipe) test: number) {return test + 10;}@Get('boolean')getBoolean(@Query('test') test: boolean) {return test;}@Get('array')getArray(@Query('test', new ParseArrayPipe({items: Number,separator: '/',optional: true,})) test: number[]) {return test}@Get('enum/:test')getEnum(@Query('test', new ParseEnumPipe(TestEnum)) test: TestEnum) {return test}
}
访问 http://localhost:3000/enum/111
当我们传的参数不是枚举定义的类型时会报错
访问 http://localhost:3000/enum/555
6、 ParseUUIDPipe
用于将传入的字符串转换为 UUID。在参数里,可以用 ParseUUIDPipe 来校验是否是 UUID
首先我们安装一下 uuid 用于生成uuid
pnpm install uuid
接着我们实现2个接口 getUuid getUuid2
import { Controller, Get, HttpException, HttpStatus, Param, ParseArrayPipe, ParseEnumPipe, ParseFloatPipe, ParseIntPipe, ParseUUIDPipe, Query } from '@nestjs/common';
import { AppService } from './app.service';const uuid = require('uuid');enum TestEnum {A = '111',B = '222',C = '333'
}@Controller()
export class AppController {constructor(private readonly appService: AppService) { }@Get()getHello(@Query('test', new ParseIntPipe({errorHttpStatusCode: HttpStatus.NOT_FOUND,exceptionFactory(error) {console.log(error);throw new HttpException('测试报错' + error, HttpStatus.NOT_IMPLEMENTED)},})) test: string): string {return test + 10;}@Get('test')getTest(@Query('test', ParseFloatPipe) test: number) {return test + 10;}@Get('boolean')getBoolean(@Query('test') test: boolean) {return test;}@Get('array')getArray(@Query('test', new ParseArrayPipe({items: Number,separator: '/',optional: true,})) test: number[]) {return test}@Get('enum/:test')getEnum(@Param('test', new ParseEnumPipe(TestEnum)) e: TestEnum) {return e}@Get('uuid')getUuid() {return uuid.v4()}@Get('uuid/:uuid')getUuid2(@Param('uuid', new ParseUUIDPipe) uuid: string) {return uuid}
}
访问 http://localhost:3000/uuid 获取uuid
接着访问 http://localhost:3000/uuid/0a5c5ce9-22ff-4091-85ef-523fcb64223c 可以看到正常访问
但是如果参数不是正常的uuid那么会报错
http://localhost:3000/uuid/xxx
7、DefaultValuePipe
用于在传入的参数不存在时,给参数设置一个默认值。
创建一个接口:
@Get('default')getDefault(@Query('test', new DefaultValuePipe('test')) test: string) {return test}
当我们没传参数的时候 会使用默认值test http://localhost:3000/default
当我们传参数之后将会返回参数 例如 http://localhost:3000/default?test=123456
8、自定义Pipe
首先创建一个 pipe:
nest g pipe www --flat --no-spec
www.pipe.ts:
import { ArgumentMetadata, Injectable, PipeTransform } from '@nestjs/common';@Injectable()
export class WwwPipe implements PipeTransform {transform(value: any, metadata: ArgumentMetadata) {console.log(value,metadata);return 'www';}
}
增加接口:
@Get('www')getWww(@Query('test', WwwPipe) test: string, @Query('test2', WwwPipe) test2: string) {return test + test2}
浏览器访问 http://localhost:3000/www?test=1234&test2=1
这就是Pipe返回的值
控制台打印了 value 就是 query、param 的值,而 metadata 里包含 type、metatype、data
type 就是 @Query、@Param、@Body 装饰器,或者自定义装饰器
metatype 是参数的 ts 类型
data 参数值
相关文章:
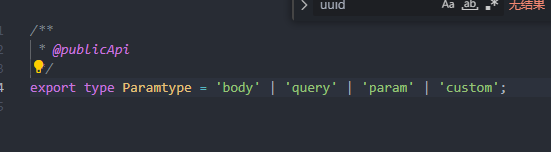
第十五章 Nest Pipe(内置及自定义)
NestJS的Pipe是一个用于数据转换和验证的特殊装饰器。Pipe可以应用于控制器(Controller)的处理方法(Handler)和中间件(Middleware),用于处理传入的数据。它可以用来转换和验证数据,确…...

实战篇(八):使用Processing创建动态图形:诡异八爪鱼
使用Processing创建动态图形:诡异八爪鱼 引言 在这个教程中,我们将深入探讨如何使用Processing编程语言创建一个动态的图形效果。我们将通过一个具体的例子,展示如何绘制一个跟随鼠标移动的“鱿鱼”图形,并使其颜色和形状动态变化。这个项目不仅适合初学者学习Processing…...
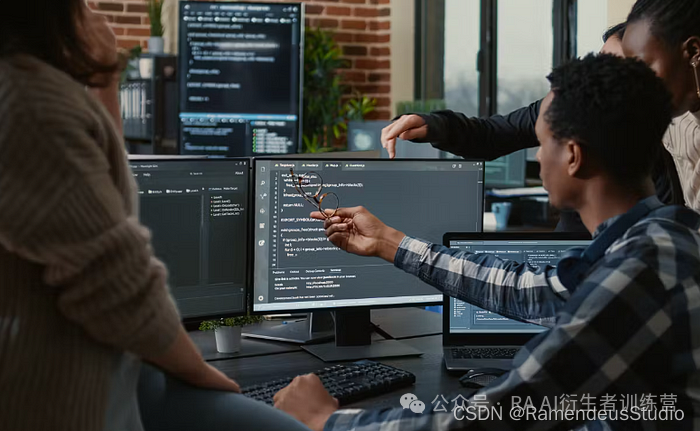
大模型成为软件和数据工程师
前言 想象一下这样一个世界:人工智能伙伴负责编码工作,让软件和数据工程师释放他们的创造天赋来应对未来的技术挑战! 想象一下:你是一名软件工程师,埋头于堆积如山的代码中,淹没在无数的错误中࿰…...
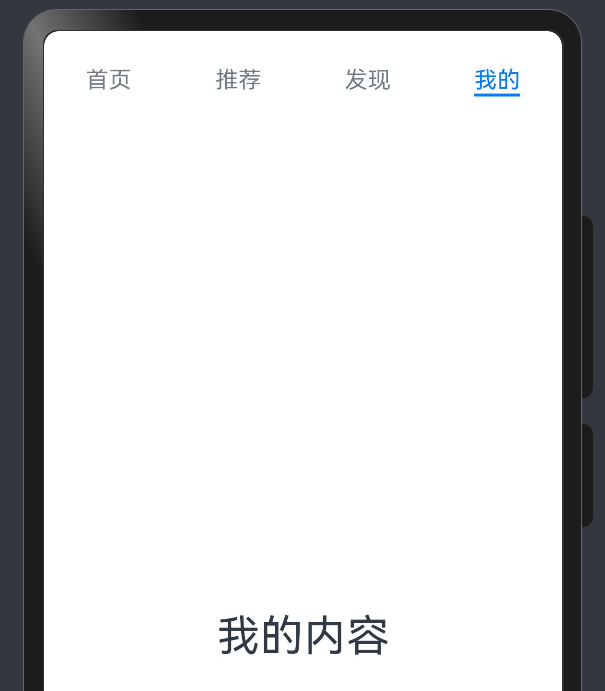
【鸿蒙学习笔记】页面布局
官方文档:布局概述 常见页面结构图 布局元素的组成 线性布局(Row、Column) 了解思路即可,更多样例去看官方文档 Entry Component struct PracExample {build() {Column() {Column({ space: 20 }) {Text(space: 20).fontSize(15)…...
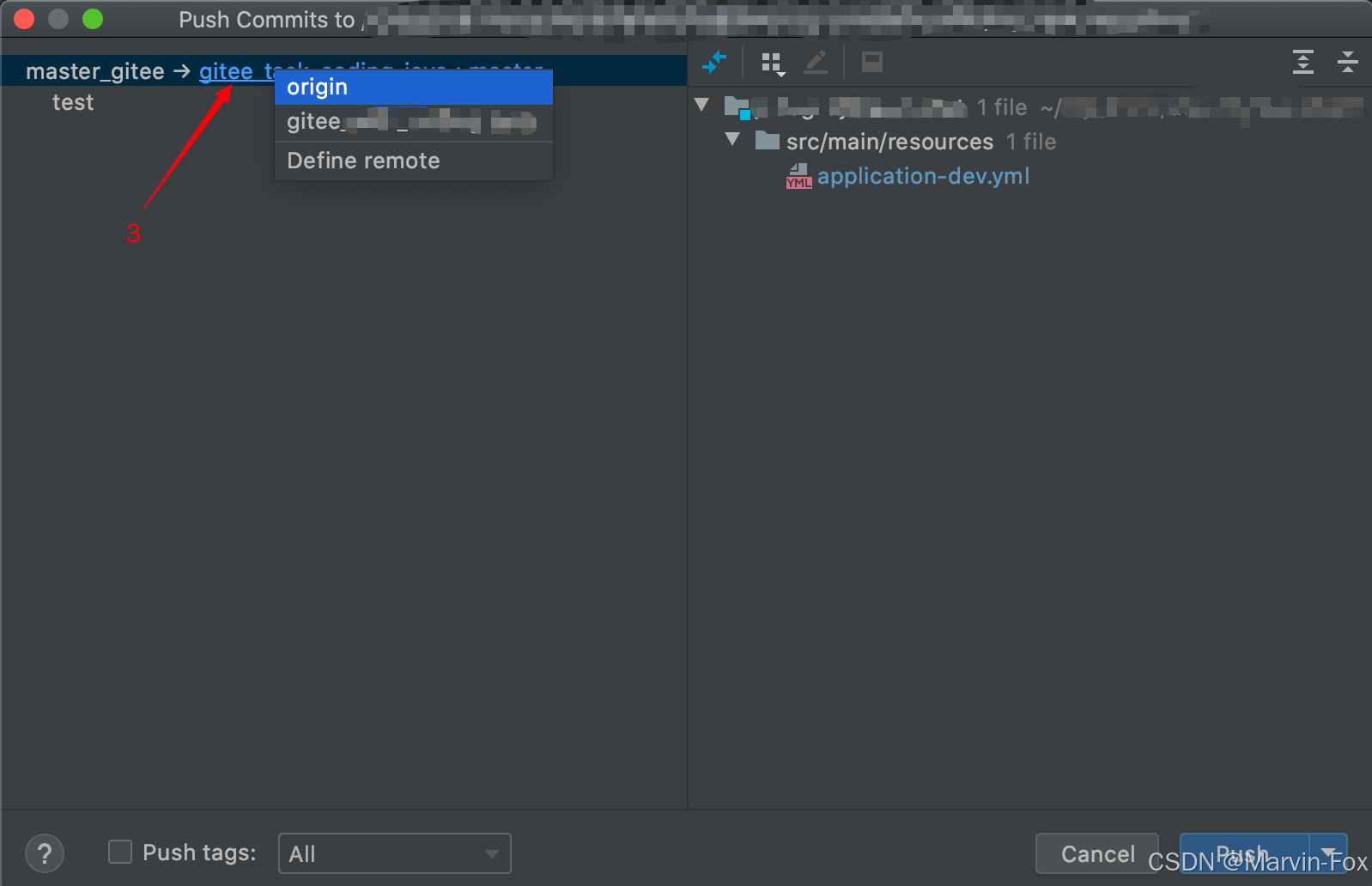
GIT 使用相关技巧记录
目录 1、commit 用户信息变更 全局用户信息(没有特殊配置的情况下默认直接用全局信息) 特定仓库用户信息(只针对于当前项目) 方法一:修改config文件 方法二:命令方式 2、idea同一代码推向多个远端仓库…...
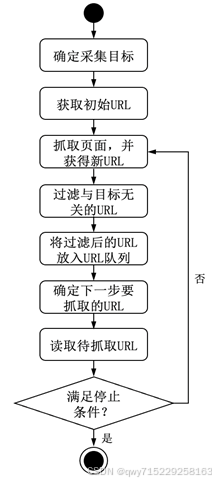
1-认识网络爬虫
1.什么是网络爬虫 网络爬虫(Web Crawler)又称网络蜘蛛、网络机器人,它是一种按照一定规则,自动浏览万维网的程序或脚本。通俗地讲,网络爬虫就是一个模拟真人浏览万维网行为的程序,这个程序可以代替真人…...
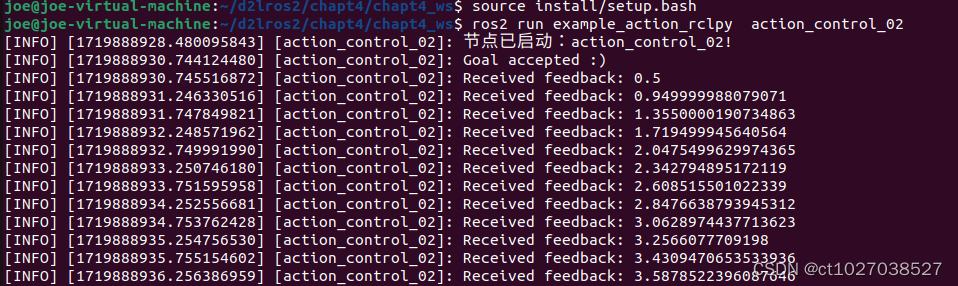
ROS2使用Python开发动作通信
1.创建接口节点 cd chapt4_ws/ ros2 pkg create robot_control_interfaces --build-type ament_cmake --destination-directory src --maintainer-name "joe" --maintainer-email "1027038527qq.com" mkdir -p src/robot_control_interfaces/action touch…...
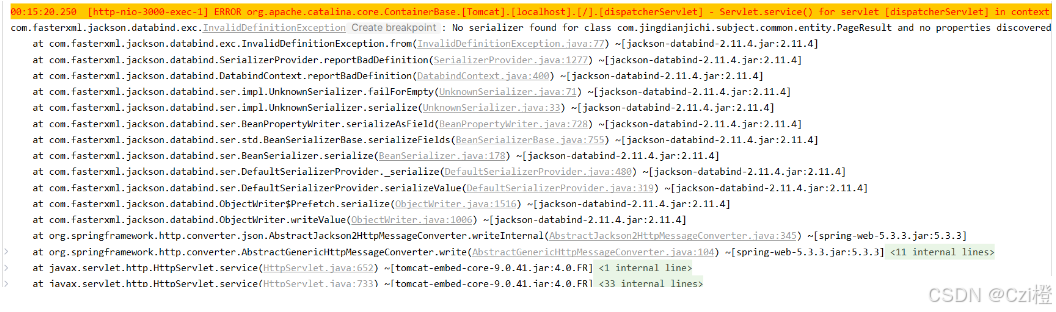
Bug记录:【com.fasterxml.jackson.databind.exc.InvalidDefinitionException】
bug记录 序列化错误 异常com.fasterxml.jackson.databind.exc.InvalidDefinitionException: 完整错误(主要是FAIL_ON_EMPTY_BEANS) 00:15:20.250 [http-nio-3000-exec-1] ERROR org.apache.catalina.core.ContainerBase.[Tomcat].[localhost].[/].[dispatcherServlet] - S…...

Mongodb索引的删除
学习mongodb,体会mongodb的每一个使用细节,欢迎阅读威赞的文章。这是威赞发布的第87篇mongodb技术文章,欢迎浏览本专栏威赞发布的其他文章。如果您认为我的文章对您有帮助或者解决您的问题,欢迎在文章下面点个赞,或者关…...
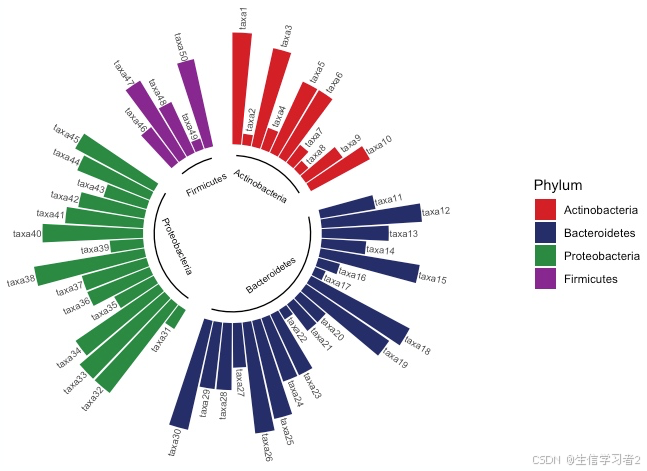
科研绘图系列:R语言径向柱状图(Radial Bar Chart)
介绍 径向柱状图(Radial Bar Chart),又称为雷达图或蜘蛛网图(Spider Chart),是一种在极坐标系中绘制的柱状图。这种图表的特点是将数据点沿着一个或多个从中心向外延伸的轴来展示,这些轴通常围绕着一个中心点均匀分布。 特点: 极坐标系统:数据点不是在直角坐标系中展…...
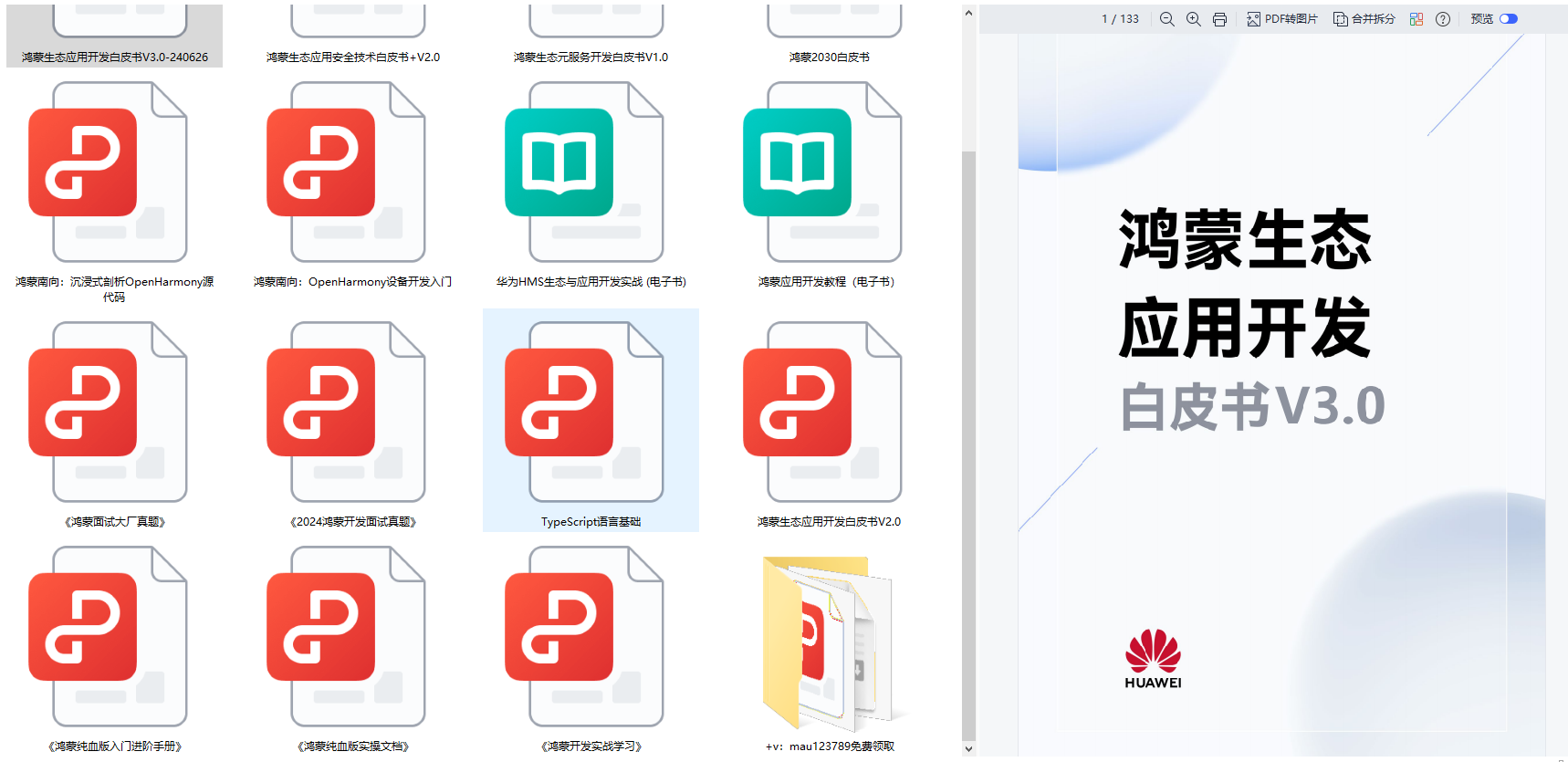
鸿蒙开发管理:【@ohos.account.distributedAccount (分布式帐号管理)】
分布式帐号管理 本模块提供管理分布式帐号的一些基础功能,主要包括查询和更新帐号登录状态。 说明: 本模块首批接口从API version 7开始支持。后续版本的新增接口,采用上角标单独标记接口的起始版本。开发前请熟悉鸿蒙开发指导文档ÿ…...
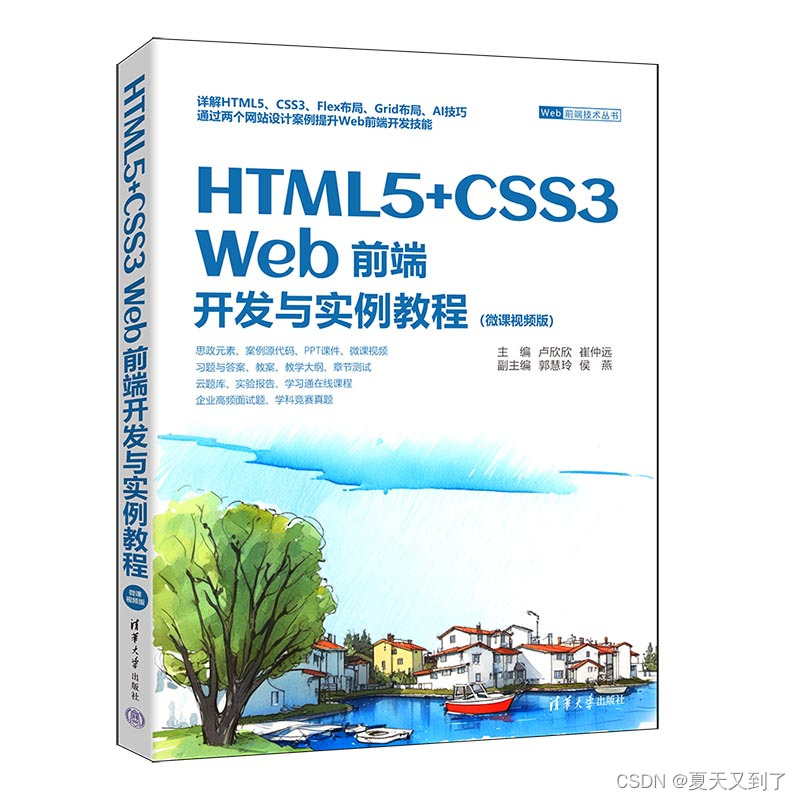
【图书推荐】《HTML5+CSS3 Web前端开发与实例教程(微课视频版)》
本书用来干什么 详解HTML5、CSS3、Flex布局、Grid布局、AI技巧,通过两个网站设计案例提升Web前端开发技能,为读者深入学习Web前端开发打下牢固的基础。 配套资源非常齐全,可以当Web前端基础课的教材。 内容简介 本书秉承“思政引领&#…...
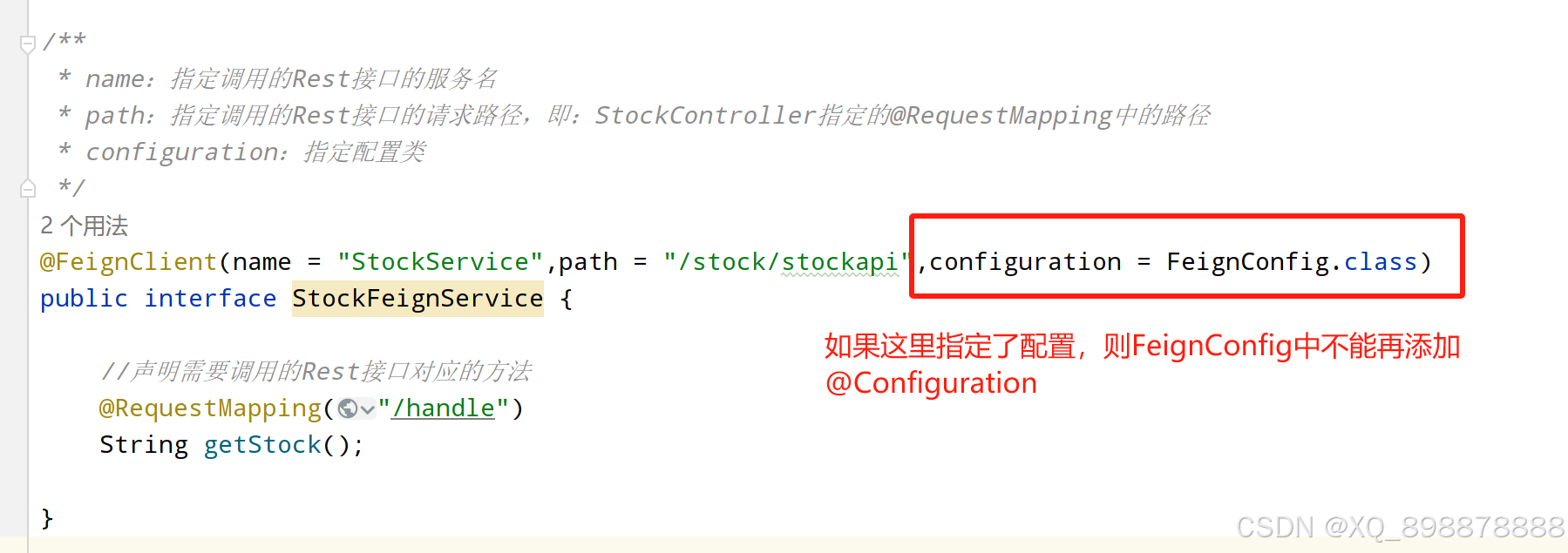
【04】微服务通信组件Feign
1、项目中接口的调用方式 1.1 HttpClient HttpClient 是 Apache Jakarta Common 下的子项目,用来提供高效的、最新的、功能丰富的支持 Http 协议的客户端编程工具包,并且它支持 HTTP 协议最新版本和建议。HttpClient 相比传统 JDK 自带的 URLConnectio…...
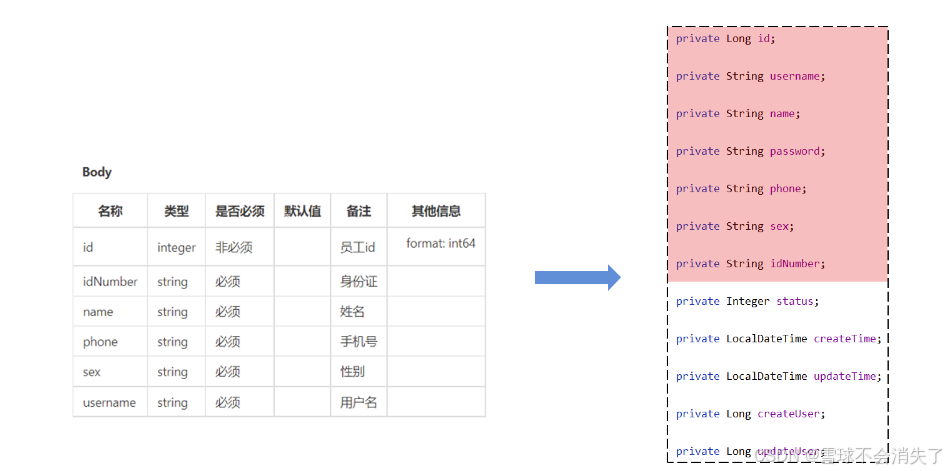
为什么要设计DTO类
为什么要使用DTO类,下面以新增员工接口为例来介绍。 新增员工 1.1 需求分析和设计 1.1.1 产品原型 一般在做需求分析时,往往都是对照着产品原型进行分析,因为产品原型比较直观,便于我们理解业务。 后台系统中可以管理员工信息…...

流批一体计算引擎-11-[Flink]实战使用DataStream对接kafka
1 消费kafka[DataStreamAPI] 参考官网DataStream API 教程 参考官网DataStream中的Apache Kafka 连接器 flink 1.14版本及以前,不支持python flink 1.15版本为FlinkKafkaConsumer和FlinkKafkaProducer flink 1.16版本及以后为KafkaSource和KafkaSink pip install apache-flin…...

数据仓库面试题
一、ODS、DWD、DWS、ADS划分与职责 数据仓库中的ODS、DWD、DWS、ADS分别代表以下层次,并各自承担不同的职责:--ODS(Operational Data Store): 名称:贴源层 主要职责:作为数据仓库的第一层&…...
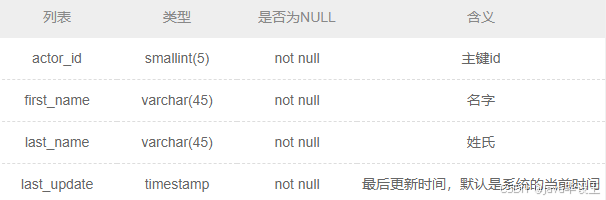
SQL 创建一个actor表,包含如下列信息
系列文章目录 文章目录 系列文章目录前言 前言 前些天发现了一个巨牛的人工智能学习网站,通俗易懂,风趣幽默,忍不住分享一下给大家。点击跳转到网站,这篇文章男女通用,看懂了就去分享给你的码吧。 描述 创建一个acto…...

STM32+ESP8266连接阿里云
完整工程文件(百度网盘免费下载,提取码:0625)在文章末尾,需要请移步至文章末尾。 目录 宏定义配置 串口通信配置 消息解析及数据发送 ESP8266初始化 注意事项 完整工程文件 经过基础教程使用AT指令连接阿里云后…...

shark云原生-日志体系-ECK
文章目录 0. ECK 介绍1. 部署 CRDS & Opereator2. 部署 Elasticsearch 集群3. 配置存储4. 部署示例 0. ECK 介绍 ECK(Elastic Cloud on Kubernetes)是Elasticsearch官方提供的一种方式,用于在Kubernetes上部署、管理和扩展Elasticsearch…...
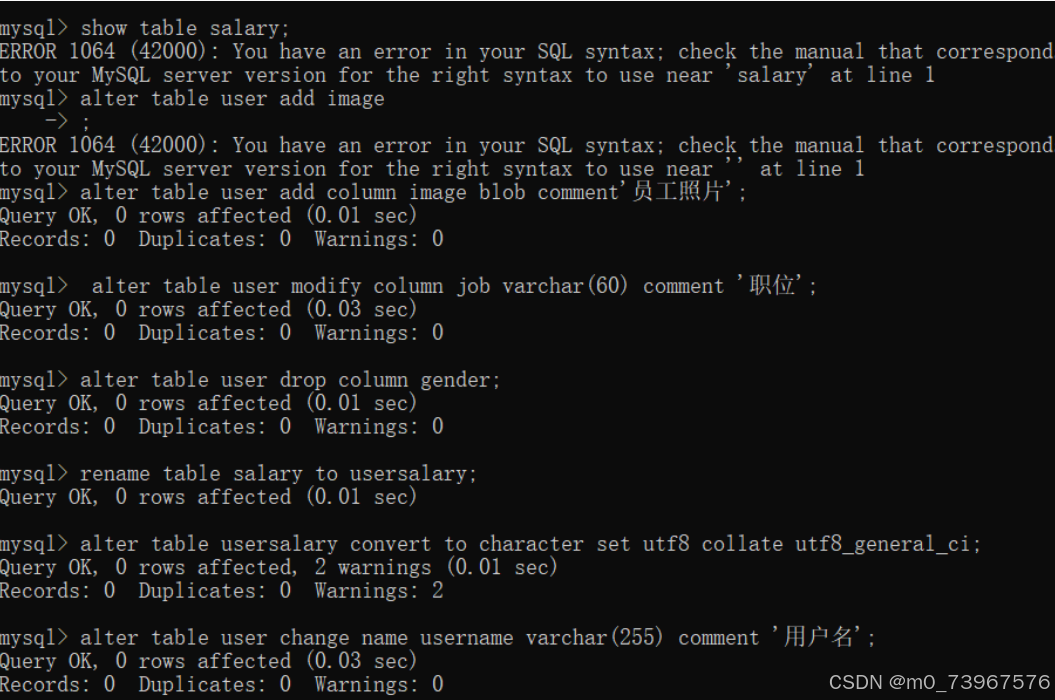
第二次作业
一、数据库 1、登陆数据库 2、创建数据库zoo 3、修改数据库zoo字符集为gbk 4、选择当前数据库为zoo 5、查看创建数据库zoo信息 6、删除数据库zoo 一、数据库(步骤) 1、登陆数据库 mysql -hlocalhost -uadmin -p123456 2、创建…...

Java8 新特性stream、forEach常用方法总结
1、去重 List<Long> list new ArrayList<>();list.add(1L);list.add(2L);list.add(3L);list.add(3L);list.stream().distinct().collect(Collectors.toList()); 2、筛选出符合条件的数据 1)单条件筛选 筛选出性别为男的学生: List<…...

C语言4 运算符
目录 1. 算术运算符 2. 关系运算符 3. 逻辑运算符 4. 位运算符 5. 赋值运算符 6. 自增和自减运算符 7. 条件运算符(三元运算符) 8. 逗号运算符 9. sizeof 运算符 10. 取地址和解引用运算符 11.运算符的优先级 1. 算术运算符 (加法)࿱…...
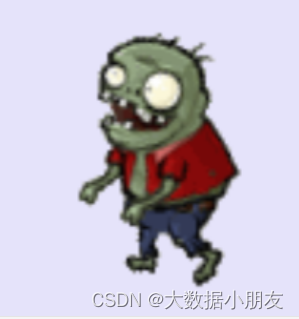
【数据分析】Pandas_DataFrame读写详解:案例解析(第24天)
系列文章目录 一、 读写文件数据 二、df查询数据操作 三、df增加列操作 四、df删除行列操作 五、df数据去重操作 六、df数据修改操作 文章目录 系列文章目录前言一、 读写文件数据1.1 读写excel文件1.2 读写csv文件1.3 读写mysql数据库 二、df查询数据操作2.1 查询df子集基本方…...

quill编辑器使用总结
一、vue-quill-editor 与 quill 若使用版本1.0,这两个组件使用哪个都是一样的,无非代码有点偏差;若需要使用表格功能,必须使用 quill2.0 版本,因为 vue-quill-editor 不支持table功能。 二、webpack版本问题 在使用 q…...
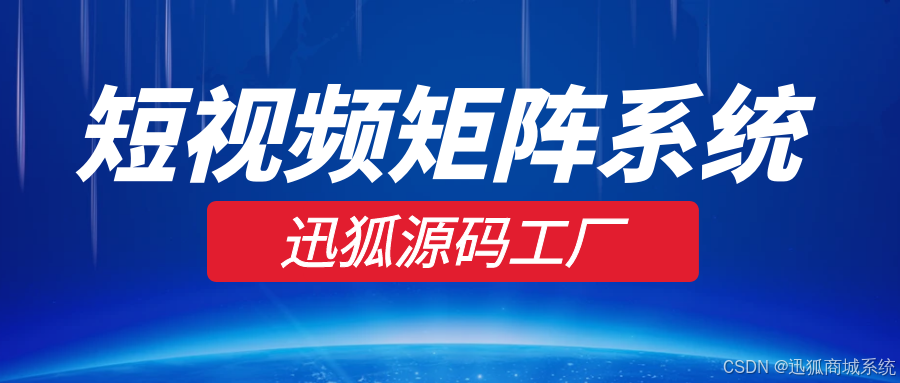
快手矩阵管理系统:引领短视频运营新潮流
在短视频行业蓬勃发展的今天,如何高效运营和优化内容创作已成为企业和创作者关注的焦点。快手矩阵管理系统以其强大的核心功能,为短视频内容的创作、发布和管理提供了一站式解决方案。 智能创作:AI自动生成文案 快手矩阵管理系统的智能创作…...

文心一言:探索AI写作的新境界
在人工智能飞速发展的今天,AI写作助手已经成为许多写作者、内容创作者和营销专家的重要工具。"文心一言"作为一个先进的AI写作平台,以其强大的语言理解和生成能力,为用户提供了从文本生成到编辑、优化等一系列服务。本文将介绍如何…...
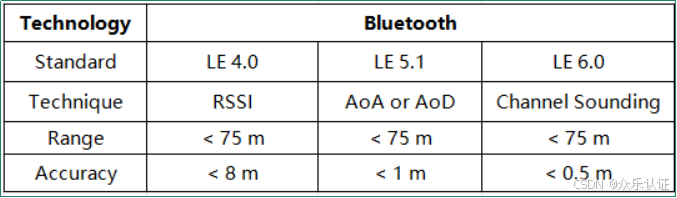
认证资讯|Bluetooth SIG认证
在当今高度互联的世界中,无线技术的普及已经成为我们生活和工作中不可或缺的一部分。作为领先的无线通信技术之一,Bluetooth技术以其稳定性、便捷性和广泛的应用场景而备受青睐。然而,要想在激烈的市场竞争中脱颖而出,获得Bluetoo…...
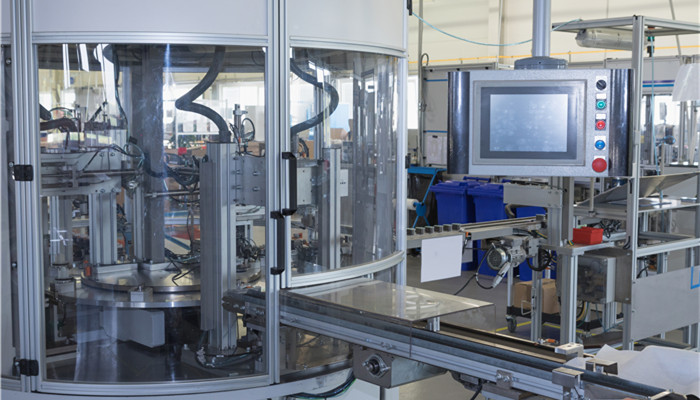
我国静止无功发生器(SVG)市场规模逐渐扩大 高压SVG为主流产品
我国静止无功发生器(SVG)市场规模逐渐扩大 高压SVG为主流产品 静止无功发生器(SVG)又称为静止同步补偿器、先进静止补偿器、静止调相机等,是利用全控型功率器件组成的桥式变流器来实现动态无功调节的一种先进无功自动补…...
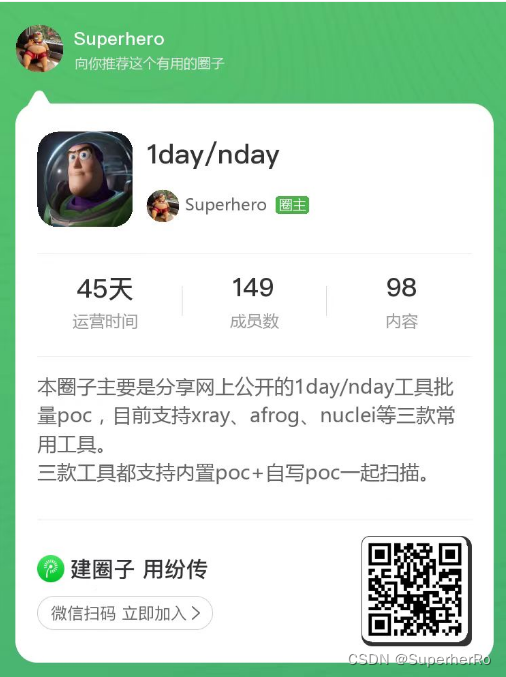
【漏洞复现】用友U8 CRM downloadfile 任意文件读取漏洞
0x01 产品简介 用友U8 CRM客户关系管理系统是一款专业的企业级CRM软件,旨在帮助企业高效管理客户关系、提升销售业绩和提供优质的客户服务。 0x02 漏洞概述 用友 U8 CRM客户关系管理系统 /pub/downloadfile.php接囗处存在任意文件读取漏洞,未经身份验证的远程攻击…...
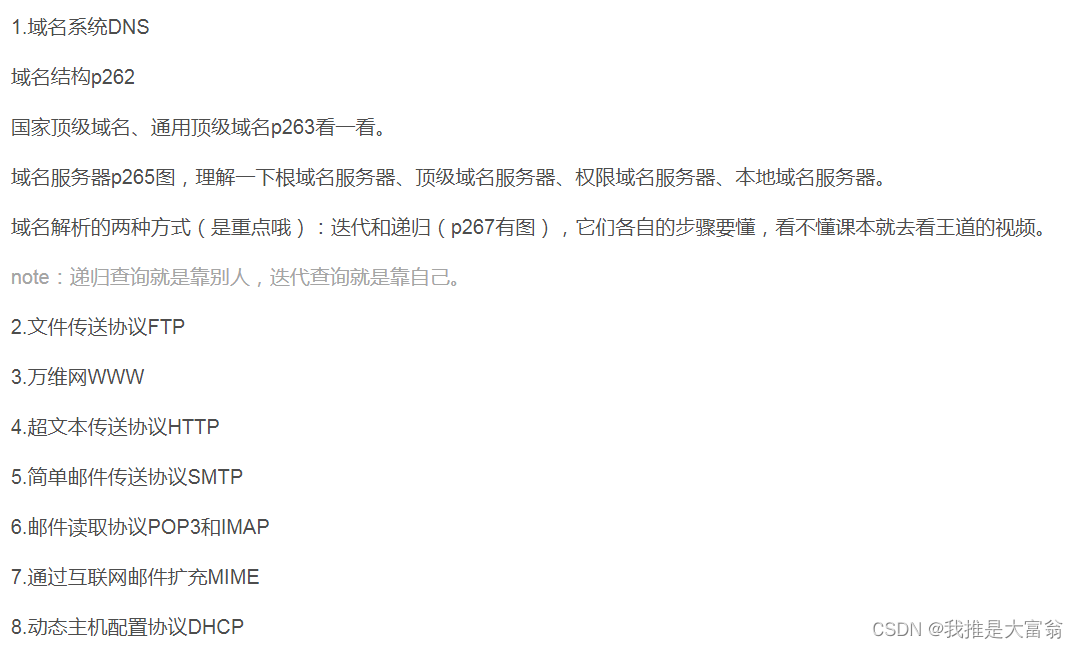
计算机网络 | 期末复习
物理层: 奈氏准则:带宽(w Hz),在不考虑噪音的情况下,最大速率(2W)码元/秒 信噪比S/N:以分贝(dB)为度量单位。信噪比(dB)…...