Linux——多线程(五)
1.线程池
1.1初期框架
thread.hpp
#include<iostream>
#include <string>
#include <unistd.h>
#include <functional>
#include <pthread.h>namespace ThreadModule
{using func_t = std::function<void()>;class Thread{public:void Excute(){_func();}public:Thread(func_t func, std::string name="none-name"): _func(func), _threadname(name), _stop(true){}static void *threadroutine(void *args) //注意:类成员函数,形参是有this指针的{Thread *self = static_cast<Thread *>(args);self->Excute();return nullptr;}bool Start(){int n = pthread_create(&_tid, nullptr, threadroutine, this);if(!n){_stop = false;return true;}else{return false;}}void Detach(){if(!_stop){pthread_detach(_tid);}}void Join(){if(!_stop){pthread_join(_tid, nullptr);}}std::string name(){return _threadname;}void Stop(){_stop = true;}~Thread() {}private:pthread_t _tid;//线程tidstd::string _threadname;//线程名字func_t _func;//线程所要执行的函数bool _stop;//判断线程是否停止};
}
ThreadPool.hpp
#include<vector>
#include<unistd.h>
#include<string>
#include<queue>
#include"Thread.hpp"using namespace ThreadModule;
const int g_thread_num = 3;//默认线程数
// 线程池->一批线程,一批任务,有任务push、有任务pop,本质是: 生产消费模型
template <typename T>
class ThreadPool
{
public:ThreadPool(int threadnum=g_thread_num)//构造函数:_threadnum(threadnum), _waitnum(0), _isrunning(false){pthread_mutex_init(&_mutex,nullptr);//初始化锁pthread_cond_init(&_cond,nullptr);//初始化条件变量}void Print(){while(true){std::cout<<"我是一个线程"<<std::endl;sleep(1);}}void InitThreadPool(){// 指向构建出所有的线程,并不启动for (int num = 0; num < _threadnum; num++){std::string name = "thread-" + std::to_string(num + 1);_threads.emplace_back(Print,name);//线程处理函数是Print,注意这里有问题}_isrunning = true;}void Start()//启动线程池{for(auto &thread:_threads){thread.Start();std::cout<<thread.name()<<"线程:启动成功"<<std::endl;}}void Wait(){for(auto &thread:_threads){thread.Join();}}// bool Enqueue(const T &t)// {// }~ThreadPool()//析构{pthread_mutex_destroy(&_mutex);pthread_cond_destroy(&_cond);}
private:int _threadnum;//线程的数量std::vector<Thread> _threads;//用vector来存线程std::queue<T> _task_queue;//任务队列pthread_mutex_t _mutex;//锁pthread_cond_t _cond;//条件变量int _waitnum;//有几个线程阻塞bool _isrunning;//判断线程池是否在运行
};
main.cc
#include <iostream>
#include <string>
#include <memory>
#include "threadpool.hpp" int main()
{std::unique_ptr<ThreadPool<int>> tp(new ThreadPool<int>()); tp->InitThreadPool();tp->Start();sleep(5);tp->Wait();return 0;
}
此时会报错:无效使用非静态成员函数...
主要原因是成员函数包含this指针而thread.hpp中线程所要执行函数的参数为空:using func_t = std::function<void()>;,导致参数类型不匹配
有两种解决方法
方法一:在Print函数前面加上static
static void Print(){while(true){std::cout<<"我是一个线程"<<std::endl;sleep(1);}}
方法二:在初始化线程池时用bind绑定ThreadPool内部的Print方法,缺省地设置参数this,就是将this参数默认的绑定到Print方法上,这样一来就和thread.hpp中的参数匹配上了
void InitThreadPool(){// 指向构建出所有的线程,并不启动for (int num = 0; num < _threadnum; num++){std::string name = "thread-" + std::to_string(num + 1);//_threads.emplace_back(Print,name);//线程处理函数是Print_threads.emplace_back(std::bind(&ThreadPool::Print,this),name);}_isrunning = true;}
也是成功运行
就算后面我们需要更改线程的参数 那么也可以在初始化函数那里固定式的绑定参数了
不需要再去单独给线程设计参数对象了
一个类的成员方法设计成另一个类的回调方法,常见的实现就是这种
类的成员方法也可以成为另一个类的回调方法,方便我们继续类级别的互相调用
1.2代码完善
接下来就是如何入队列以及我们的新线程应该做什么任务...
处理任务:每一个线程进来的时候都需要去任务队列中获取任务,所以我们首当其冲的就要对任务队列给它锁住
任务队列的加锁、解锁以及线程的等待与唤醒(条件变量)
private:void LockQueue(){pthread_mutex_lock(&_mutex);}void UnlockQueue(){pthread_mutex_unlock(&_mutex);}void ThreadSleep(){pthread_cond_wait(&_cond, &_mutex);}void ThreadWakeup(){pthread_cond_signal(&_cond);}void ThreadWakeupAll(){pthread_cond_broadcast(&_cond);}
处理任务
void HandlerTask(std::string name)//线程处理任务{while (true){//加锁LockQueue();//任务队列中不一定有数据,如果任务队列为空且线程池在跑,那么就阻塞住while(_task_queue.empty()&&_isrunning){_waitnum++;ThreadSleep();_waitnum--;}//如果任务队列是空的,然后线程池又退出了,那么就没必要运行了if(_task_queue.empty() && !_isrunning){UnlockQueue();std::cout<<name<<"quit..."<<std::endl;sleep(1);break;}//不论线程池有没有退出,走到这说明一定有任务 ->处理任务T t = _task_queue.front();_task_queue.pop();UnlockQueue();//解锁t();}}
注意:这个任务是属于线程独占的任务,不能再任务队列的加锁、解锁之间处理
入任务队列
如果线程阻塞等待的数量大于0,就唤醒一个线程
bool Enqueue(const T &t){bool ret = false;LockQueue();if(_isrunning){_task_queue.push(t);if(_waitnum>0){ThreadWakeup();}ret = true;}UnlockQueue();return ret;}
threadpool.hpp
任务还没写,所以t()先注释掉
#include<iostream>
#include<vector>
#include<unistd.h>
#include<string>
#include<queue>
#include"LockGuard.hpp"
#include"Thread.hpp"using namespace ThreadModule;
const int g_thread_num = 3;//默认线程数
// 线程池->一批线程,一批任务,有任务push、有任务pop,本质是: 生产消费模型
template <typename T>
class ThreadPool
{
private:void LockQueue(){pthread_mutex_lock(&_mutex);}void UnlockQueue(){pthread_mutex_unlock(&_mutex);}void ThreadSleep(){pthread_cond_wait(&_cond, &_mutex);}void ThreadWakeup(){pthread_cond_signal(&_cond);}void ThreadWakeupAll(){pthread_cond_broadcast(&_cond);}
public:ThreadPool(int threadnum=g_thread_num)//构造函数:_threadnum(threadnum), _waitnum(0), _isrunning(false){pthread_mutex_init(&_mutex,nullptr);//初始化锁pthread_cond_init(&_cond,nullptr);//初始化条件变量}// static void Print()// {// while(true)// {// std::cout<<"我是一个线程"<<std::endl;// sleep(1);// }// }// void Print(std::string name)// {// while(true)// {// std::cout<<"我是一个线程,线程名是"<<name<<std::endl;// sleep(1);// }// }void InitThreadPool(){// 指向构建出所有的线程,并不启动for (int num = 0; num < _threadnum; num++){std::string name = "thread-" + std::to_string(num + 1);//_threads.emplace_back(Print,name);//线程处理函数是Print//_threads.emplace_back(std::bind(&ThreadPool::Print,this,std::placeholders::_1),name);_threads.emplace_back(std::bind(&ThreadPool::HandlerTask,this,std::placeholders::_1),name);}_isrunning = true;}void Start()//启动线程池{for(auto &thread:_threads){thread.Start();std::cout<<thread.name()<<"线程:启动成功"<<std::endl;}}void HandlerTask(std::string name)//线程处理任务{while (true){//加锁LockQueue();//任务队列中不一定有数据,如果任务队列为空且线程池在跑,那么就阻塞住while(_task_queue.empty()&&_isrunning){_waitnum++;std::cout<<name<<"线程阻塞中..."<<std::endl;ThreadSleep();_waitnum--;}//如果任务队列是空的,然后线程池又退出了,那么就没必要运行了if(_task_queue.empty() && !_isrunning){UnlockQueue();std::cout<<name<<"quit..."<<std::endl;sleep(1);break;}//不论线程池有没有退出,走到这说明一定有任务 ->处理任务T t = _task_queue.front();_task_queue.pop();UnlockQueue();//解锁//t();}}void Stop(){LockQueue();_isrunning = false;ThreadWakeupAll();UnlockQueue(); }void Wait(){for(auto &thread:_threads){thread.Join();}}bool Enqueue(const T &t){bool ret = false;LockQueue();if(_isrunning){_task_queue.push(t);if(_waitnum>0){ThreadWakeup();}ret = true;}UnlockQueue();return ret;}~ThreadPool()//析构{pthread_mutex_destroy(&_mutex);pthread_cond_destroy(&_cond);}
private:int _threadnum;//线程的数量std::vector<Thread> _threads;//用vector来存线程std::queue<T> _task_queue;//任务队列pthread_mutex_t _mutex;//锁pthread_cond_t _cond;//条件变量int _waitnum;bool _isrunning;//判断线程池是否在运行
};
main.cc
#include <iostream>
#include <string>
#include <memory>
#include "Task.hpp"
#include "threadpool.hpp" int main()
{std::unique_ptr<ThreadPool<int>> tp(new ThreadPool<int>()); tp->InitThreadPool();tp->Start();sleep(2);tp->Stop();tp->Wait();return 0;
}
2.加上日志与任务
LOG.hpp(日志)
#pragma once
#include <iostream>
#include <fstream>
#include <cstdio>
#include <string>
#include <ctime>
#include <cstdarg>
#include <sys/types.h>
#include <unistd.h>
#include <pthread.h>
#include"LockGuard.hpp"
bool gIsSave = false;
const std::string logname = "log.txt";// 1. 日志是有等级的
enum Level
{DEBUG = 0,INFO,WARNING,ERROR,FATAL
};
void SaveFile(const std::string &filename, const std::string &message)
{std::ofstream out(filename, std::ios::app);if (!out.is_open()){return;}out << message;out.close();
}
std::string LevelToString(int level)
{switch (level){case DEBUG:return "Debug";case INFO:return "Info";case WARNING:return "Warning";case ERROR:return "Error";case FATAL:return "Fatal";default:return "Unknown";}
}std::string GetTimeString()
{time_t curr_time = time(nullptr);struct tm *format_time = localtime(&curr_time);if (format_time == nullptr)return "None";char time_buffer[1024];snprintf(time_buffer, sizeof(time_buffer), "%d-%d-%d %d:%d:%d",format_time->tm_year + 1900,format_time->tm_mon + 1,format_time->tm_mday,format_time->tm_hour,format_time->tm_min,format_time->tm_sec);return time_buffer;
}pthread_mutex_t lock = PTHREAD_MUTEX_INITIALIZER;
// 日志是有格式的
// 日志等级 时间 代码所在的文件名/行数 日志的内容
void LogMessage(std::string filename, int line,bool issave,int level, const char *format, ...)
{std::string levelstr = LevelToString(level);std::string timestr = GetTimeString();pid_t selfid = getpid();char buffer[1024];va_list arg;va_start(arg, format);vsnprintf(buffer, sizeof(buffer), format, arg);va_end(arg);std::string message = "[" + timestr + "]" + "[" + levelstr + "]" +"[" + std::to_string(selfid) + "]" +"[" + filename + "]" + "[" + std::to_string(line) + "] " + buffer + "\n";LockGuard lockguard(&lock);if (!issave){std::cout << message;}else{SaveFile(logname, message);}
}
#define LOG(level, format, ...) \do \{ \LogMessage(__FILE__, __LINE__, gIsSave, level, format, ##__VA_ARGS__); \} while (0)
LockGuard.hpp
#ifndef __LOCK_GUARD_HPP__
#define __LOCK_GUARD_HPP__#include <iostream>
#include <pthread.h>class LockGuard
{
public:LockGuard(pthread_mutex_t *mutex):_mutex(mutex){pthread_mutex_lock(_mutex); // 构造加锁}~LockGuard(){pthread_mutex_unlock(_mutex);}
private:pthread_mutex_t *_mutex;
};#endif
threadpool.hpp
#include<iostream>
#include<vector>
#include<unistd.h>
#include<string>
#include<queue>
#include"LOG.hpp"
#include"LockGuard.hpp"
#include"Thread.hpp"using namespace ThreadModule;
const int g_thread_num = 3;//默认线程数
// 线程池->一批线程,一批任务,有任务push、有任务pop,本质是: 生产消费模型
template <typename T>
class ThreadPool
{
private:void LockQueue(){pthread_mutex_lock(&_mutex);}void UnlockQueue(){pthread_mutex_unlock(&_mutex);}void ThreadSleep(){pthread_cond_wait(&_cond, &_mutex);}void ThreadWakeup(){pthread_cond_signal(&_cond);}void ThreadWakeupAll(){pthread_cond_broadcast(&_cond);}
public:ThreadPool(int threadnum=g_thread_num)//构造函数:_threadnum(threadnum), _waitnum(0), _isrunning(false){pthread_mutex_init(&_mutex,nullptr);//初始化锁pthread_cond_init(&_cond,nullptr);//初始化条件变量LOG(INFO, "线程池构造成功");}// static void Print()// {// while(true)// {// std::cout<<"我是一个线程"<<std::endl;// sleep(1);// }// }// void Print(std::string name)// {// while(true)// {// std::cout<<"我是一个线程,线程名是"<<name<<std::endl;// sleep(1);// }// }void InitThreadPool(){// 指向构建出所有的线程,并不启动for (int num = 0; num < _threadnum; num++){std::string name = "thread-" + std::to_string(num + 1);//_threads.emplace_back(Print,name);//线程处理函数是Print//_threads.emplace_back(std::bind(&ThreadPool::Print,this,std::placeholders::_1),name);_threads.emplace_back(std::bind(&ThreadPool::HandlerTask,this,std::placeholders::_1),name);LOG(INFO, "线程 %s 初始化成功", name.c_str());}_isrunning = true;}void Start()//启动线程池{for(auto &thread:_threads){thread.Start();std::cout<<thread.name()<<"线程:启动成功"<<std::endl;}}void HandlerTask(std::string name)//线程处理任务{LOG(INFO, "%s 正在运行...", name.c_str());while (true){//加锁LockQueue();//任务队列中不一定有数据,如果任务队列为空且线程池在跑,那么就阻塞住while(_task_queue.empty()&&_isrunning){_waitnum++;ThreadSleep();_waitnum--;}//如果任务队列是空的,然后线程池又退出了,那么就没必要运行了if(_task_queue.empty() && !_isrunning){UnlockQueue();//std::cout<<name<<"quit..."<<std::endl;sleep(1);break;}//不论线程池有没有退出,走到这说明一定有任务 ->处理任务T t = _task_queue.front();_task_queue.pop();UnlockQueue();//解锁LOG(DEBUG, "%s 获得任务", name.c_str());t();LOG(DEBUG,"%s 处理任务中,结果是%s",name.c_str(), t.ResultToString().c_str());}}void Stop(){LockQueue();_isrunning = false;ThreadWakeupAll();UnlockQueue(); }void Wait(){for(auto &thread:_threads){thread.Join();LOG(INFO, "%s 线程退出...", thread.name().c_str());}}bool Enqueue(const T &t){bool ret = false;LockQueue();if(_isrunning){_task_queue.push(t);if(_waitnum>0){ThreadWakeup();}LOG(DEBUG, "任务入队列成功");ret = true;}UnlockQueue();return ret;}~ThreadPool()//析构{pthread_mutex_destroy(&_mutex);pthread_cond_destroy(&_cond);}
private:int _threadnum;//线程的数量std::vector<Thread> _threads;//用vector来存线程std::queue<T> _task_queue;//任务队列pthread_mutex_t _mutex;//锁pthread_cond_t _cond;//条件变量int _waitnum;bool _isrunning;//判断线程池是否在运行
};
thread.hpp
#include<iostream>
#include <string>
#include <unistd.h>
#include <functional>
#include <pthread.h>namespace ThreadModule
{using func_t = std::function<void(std::string)>;class Thread{public:void Excute(){_func(_threadname);}public:Thread(func_t func, std::string name="none-name"): _func(func), _threadname(name), _stop(true){}static void *threadroutine(void *args) // 类成员函数,形参是有this指针的!!{Thread *self = static_cast<Thread *>(args);self->Excute();return nullptr;}bool Start(){int n = pthread_create(&_tid, nullptr, threadroutine, this);if(!n){_stop = false;return true;}else{return false;}}void Detach(){if(!_stop){pthread_detach(_tid);}}void Join(){if(!_stop){pthread_join(_tid, nullptr);}}std::string name(){return _threadname;}void Stop(){_stop = true;}~Thread() {}private:pthread_t _tid;//线程tidstd::string _threadname;//线程名字func_t _func;//线程所要执行的函数bool _stop;//判断线程是否停止};
}
main.cc
#include <iostream>
#include <string>
#include <memory>
#include "LOG.hpp"
#include "threadpool.hpp"
#include "Task.hpp"
#include<ctime>int main()
{srand(time(nullptr) ^ getpid() ^ pthread_self());std::unique_ptr<ThreadPool<Task>> tp(new ThreadPool<Task>(5)); tp->InitThreadPool();tp->Start();int tasknum=3;while(tasknum){int a = rand() % 12 + 1;usleep(1000);int b = rand() % 4 + 1;Task t(a, b);LOG(INFO, "主线程推送任务: %s", t.DebugToString().c_str());tp->Enqueue(t);sleep(1);tasknum--;}tp->Stop();tp->Wait();return 0;
}
相关文章:
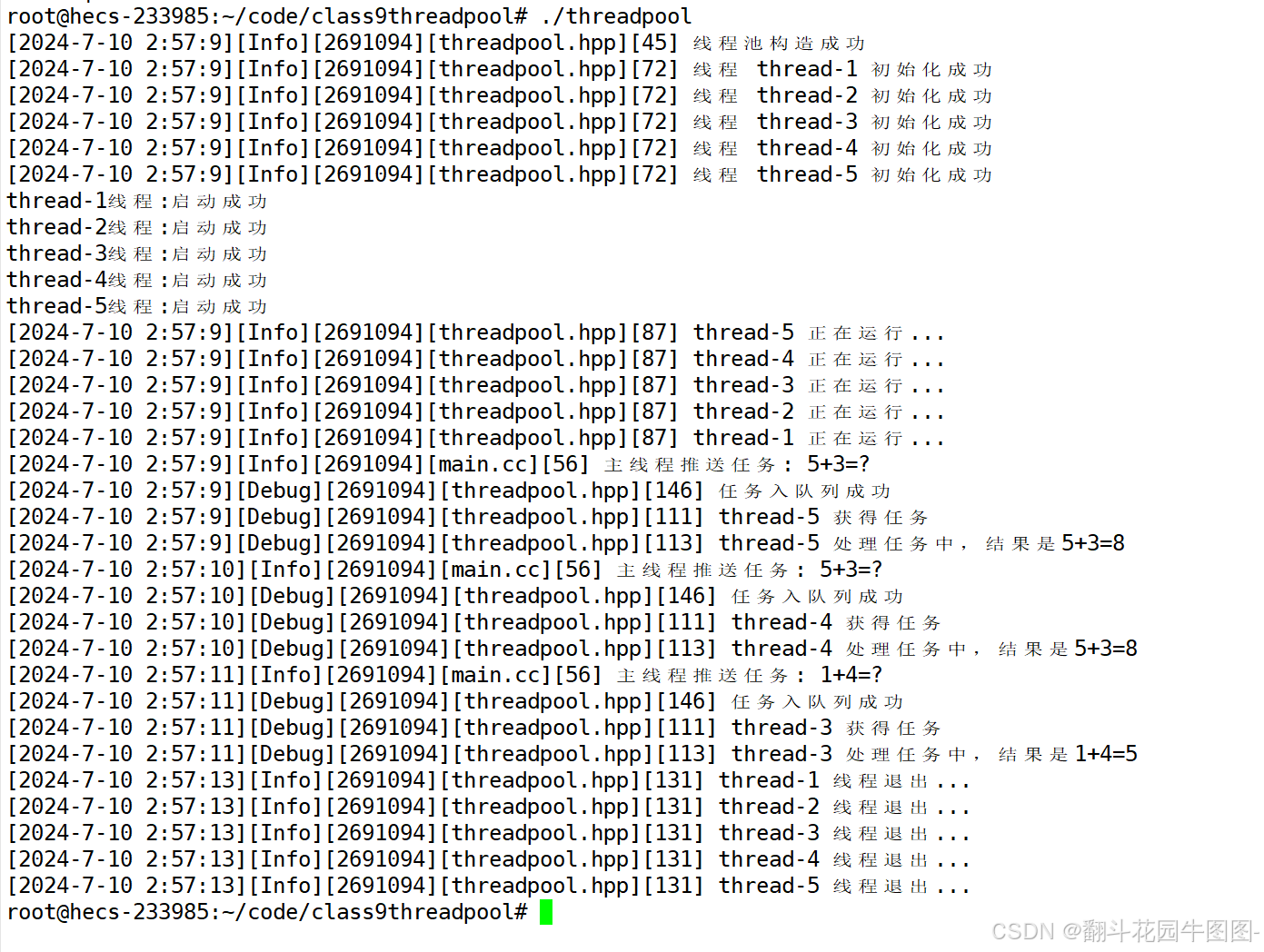
Linux——多线程(五)
1.线程池 1.1初期框架 thread.hpp #include<iostream> #include <string> #include <unistd.h> #include <functional> #include <pthread.h>namespace ThreadModule {using func_t std::function<void()>;class Thread{public:void E…...
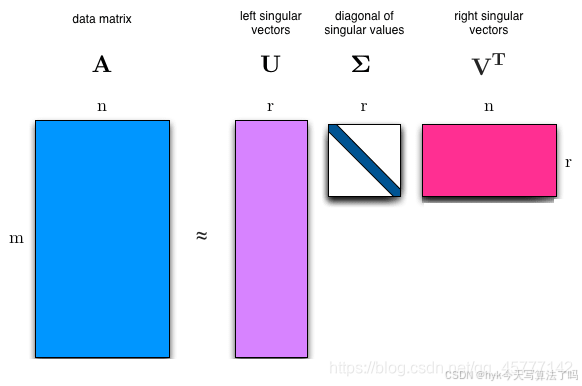
张量分解(4)——SVD奇异值分解
🍅 写在前面 👨🎓 博主介绍:大家好,这里是hyk写算法了吗,一枚致力于学习算法和人工智能领域的小菜鸟。 🔎个人主页:主页链接(欢迎各位大佬光临指导) ⭐️近…...
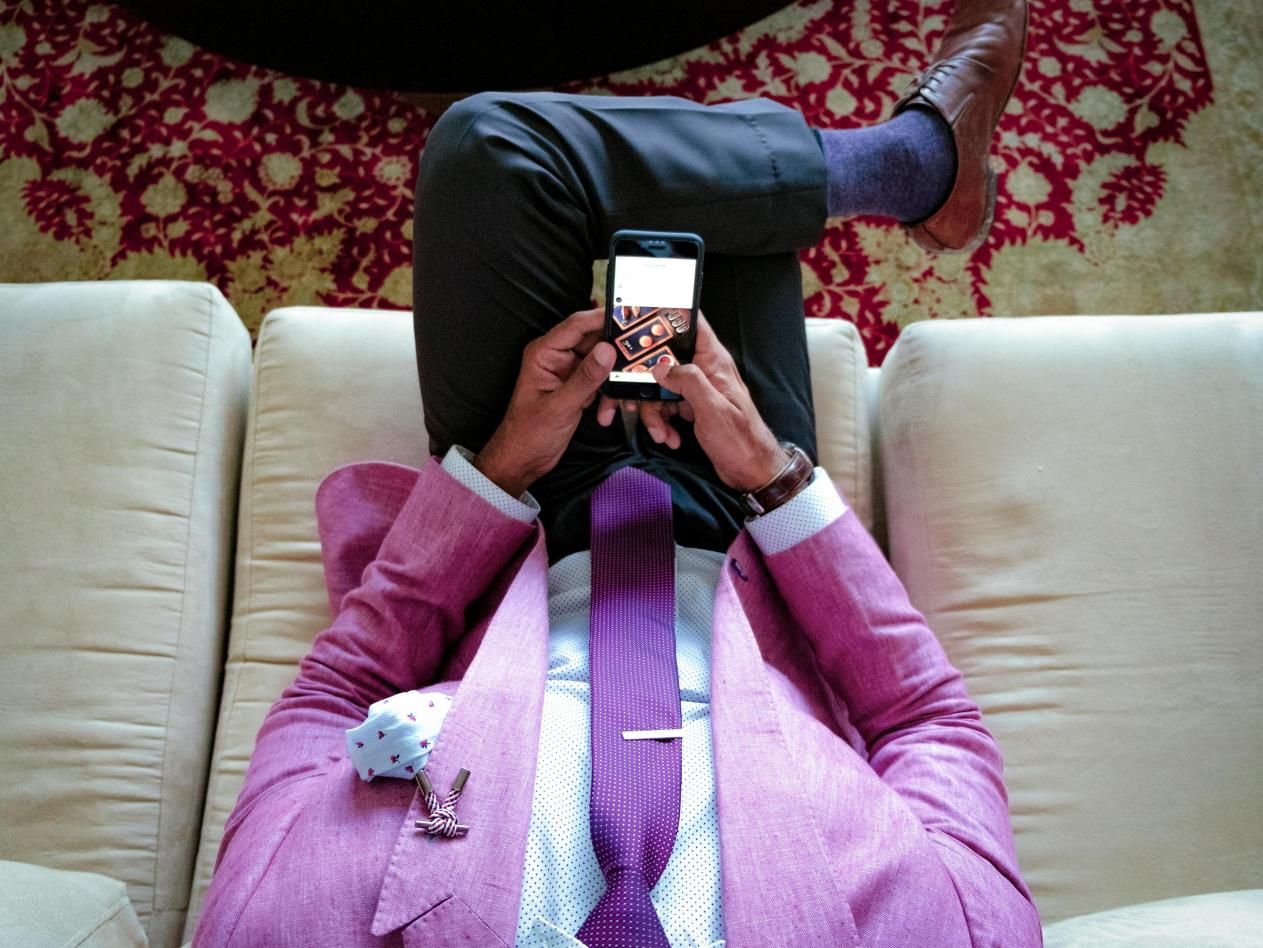
第三方配件也能适配苹果了,iOS 18与iPadOS 18将支持快速配对
苹果公司以其对用户体验的不懈追求和对创新技术的不断探索而闻名。随着iOS 18和iPadOS 18的发布,苹果再次证明了其在移动操作系统领域的领先地位。 最新系统版本中的一项引人注目的功能,便是对蓝牙和Wi-Fi配件的配对方式进行了重大改进,不仅…...

Docker 部署 Nginx 并在容器内配置申请免费 SSL 证书
文章目录 dockerdocker-compose.yml申请免费 SSL 证书请求头参数带下划线 docker https://hub.docker.com/_/nginx docker pull nginx:1.27注: 国内网络原因无法下载镜像,nginx 镜像文件下载链接 https://pan.baidu.com/s/1O35cPbx6AHWUJL1v5-REzA?pw…...
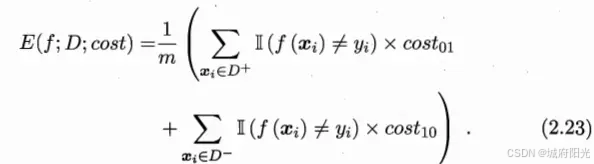
模型评估与选择
2.1 经验误差与过拟合 错误率(error rate): 分类错误的样本数占样本总数的比例 精度(accuracy):1- 错误率 训练误差 / 经验误差:在训练集上的误差 泛化误差:在新样本上的误差 过…...
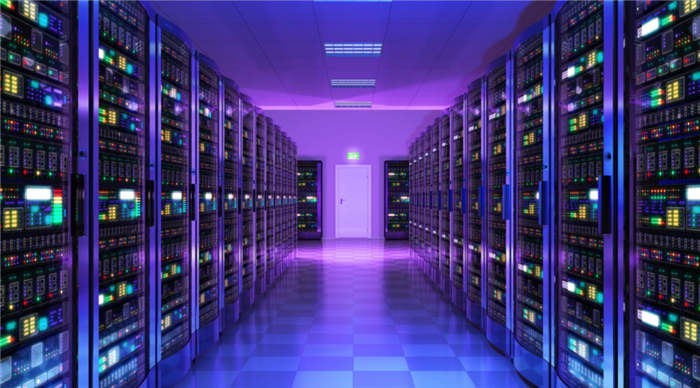
有必要把共享服务器升级到VPS吗?
根据自己的需求来选择是否升级,虚拟专用服务器 (VPS) 是一种托管解决方案,它以低得多的成本提供专用服务器的大部分功能。使用 VPS,您的虚拟服务器将与在其上运行的其他虚拟服务器共享硬件服务器的资源。但是,与传统的共享托管&am…...
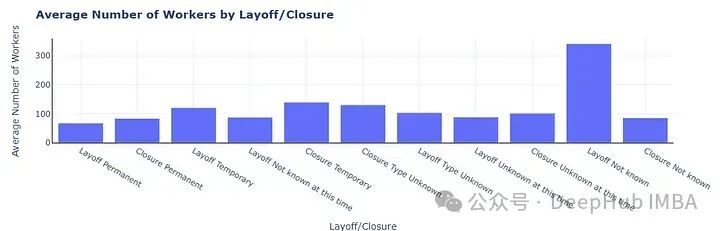
LLM代理应用实战:构建Plotly数据可视化代理
如果你尝试过像ChatGPT这样的LLM,就会知道它们几乎可以为任何语言或包生成代码。但是仅仅依靠LLM是有局限的。对于数据可视化的问题我们需要提供一下的内容 描述数据:模型本身并不知道数据集的细节,比如列名和行细节。手动提供这些信息可能很麻烦&#…...

大模型系列3--pytorch dataloader的原理
pytorch dataloader运行原理 1. 背景2. 环境搭建2.1. 安装WSL & vscode2.2. 安装conda & pytorch_gpu环境 & pytorch 2.112.3 命令行验证python环境2.4. vscode启用pytorch_cpu虚拟环境 3. 调试工具3.1. vscode 断点调试3.2. py-spy代码栈探测3.3. gdb attach3.4. …...

SQLServer 如何设置端口
在SQL Server中,可以通过以下步骤设置端口: 打开SQL Server配置管理器。可以在开始菜单中搜索“SQL Server配置管理器”来找到它。 在左侧导航窗格中,展开“SQL Server网络配置”节点。 选择你要配置的实例,如“SQL Server Netw…...
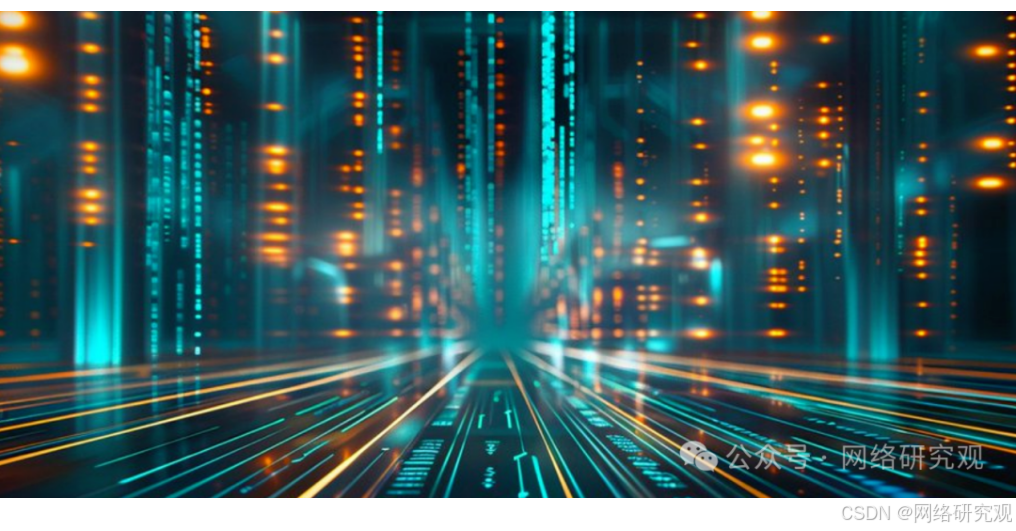
调整网络安全策略以适应不断升级的威胁形势
关键网络安全统计数据和趋势 当今数字时代网络安全的重要性...

(leetcode学习)9. 回文数
给你一个整数 x ,如果 x 是一个回文整数,返回 true ;否则,返回 false 。 回文数 是指正序(从左向右)和倒序(从右向左)读都是一样的整数。 例如,121 是回文,而…...
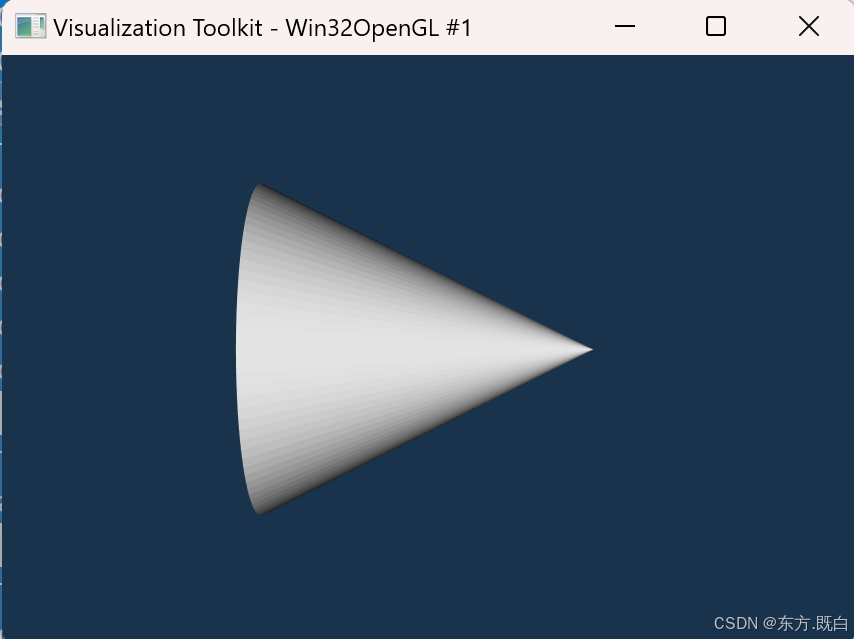
QT VTK 简单测试工程
目录 1 目录结构 2 文件源码 3 运行结果 4 报错及处理 使用编译好的VTK库进行测试 1 目录结构 2 文件源码 Pro文件 QT core guigreaterThan(QT_MAJOR_VERSION, 4): QT widgetsCONFIG c17# You can make your code fail to compile if it uses deprecated APIs. #…...

ES6 Generator函数的异步应用 (八)
ES6 Generator 函数的异步应用主要通过与 Promise 配合使用来实现。这种模式被称为 “thunk” 模式,它允许你编写看起来是同步的异步代码。 特性: 暂停执行:当 Generator 函数遇到 yield 表达式时,它会暂停执行,等待 …...
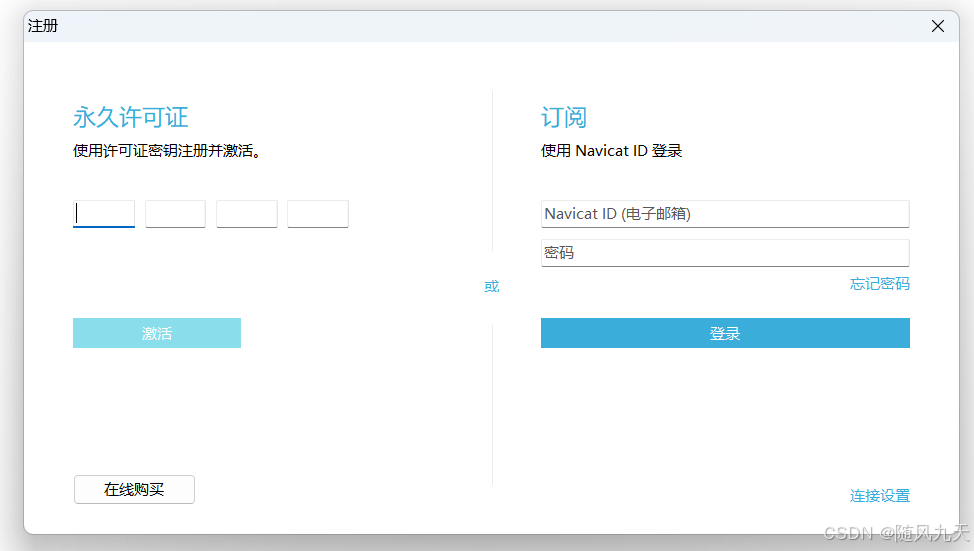
Navicat:打造高效数据库管理之道
1. 导言 1.1 介绍Navicat Navicat是一款功能强大的数据库管理工具,旨在帮助用户高效地管理多种类型的数据库,包括MySQL、PostgreSQL、Oracle、SQL Server等。通过Navicat,用户可以轻松地进行数据库的创建、编辑、备份、同步和调试等操作,极大地简化了数据库管理的复杂性。…...
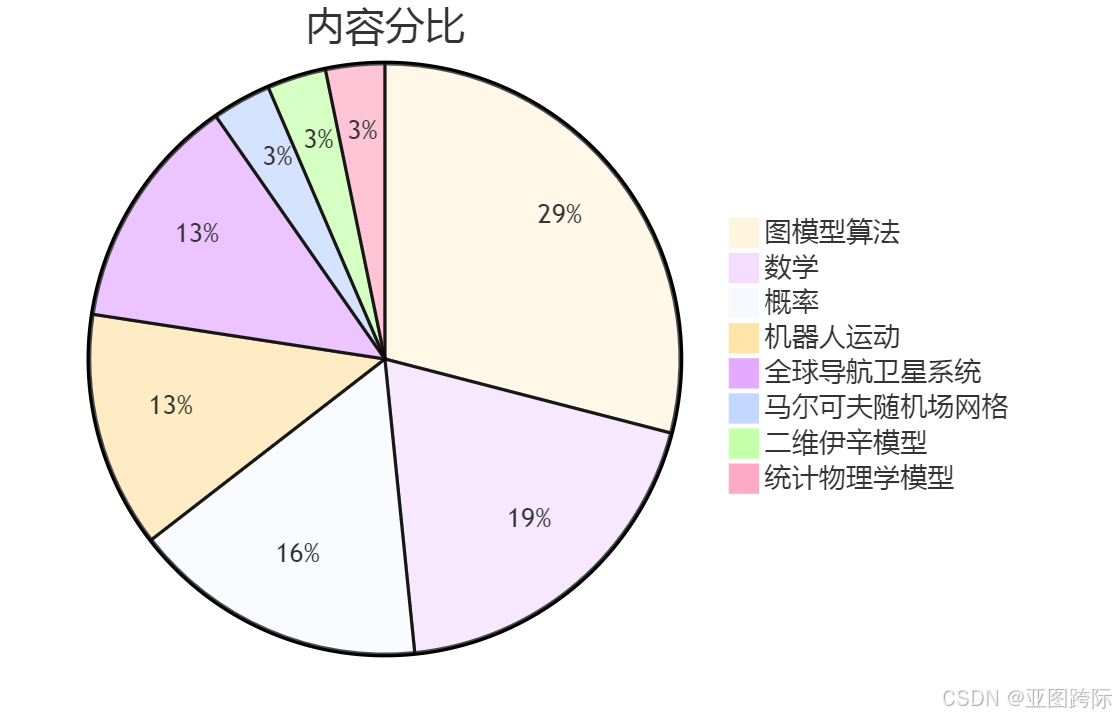
Python和C++全球导航卫星系统和机器人姿态触觉感知二分图算法
🎯要点 🎯马尔可夫随机场网格推理学习 | 🎯二维伊辛模型四连网格模型推理 | 🎯统计物理学模型扰动与最大乘积二值反卷积 | 🎯受限玻尔兹曼机扰动和最大乘积采样 | 🎯视觉概率生成模型测试图像 dz…...
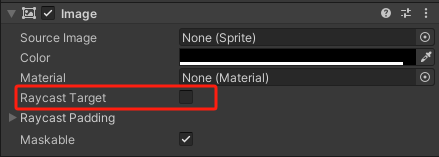
Unity 优化合集
1️⃣ 贴图优化 1. Read/Write Enable 这个属性勾选后允许你在运行时读取和写入纹理数据,这对于需要实时生成内容或者需要动态修改纹理的场合非常有用但在大部分情况下这是不必要的。如果打开这个属性,会使运行时贴图大小翻倍,内存中会额外…...

第九届MathorCup高校数学建模挑战赛-A题:基于数据驱动的城市轨道交通网络优化研究
目录 摘 要 一、 问题的提出 二、 基本假设 三、 符号说明 四、 问题分析 4.1 问题 1 的分析 4.2 问题 2 的分析 4.3 问题 3 的分析 4.4 问题 4 的分析 五、 问题 1 的模型建立与求解 5.1 问题分析 5.2 数据处理 5.2.1 数据统计 5.2.2 异常数据处理方法 5.2.3 剔除异常数据值 5…...
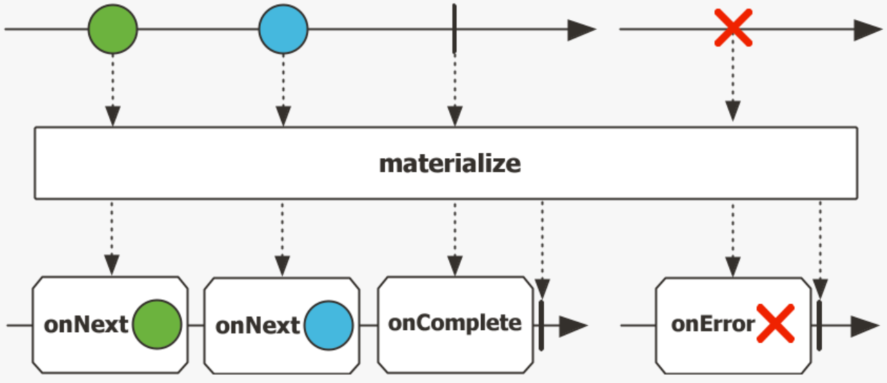
Spring webflux基础核心技术
一、 用操作符转换响应式流 1 、 映射响应式流元素 转换序列的最自然方式是将每个元素映射到一个新值。 Flux 和 Mono 给出了 map 操作符,具有 map(Function<T,R>) 签名的方法可用于逐个处理元素。 当操作符将元素的类型从 T 转变为 R 时…...
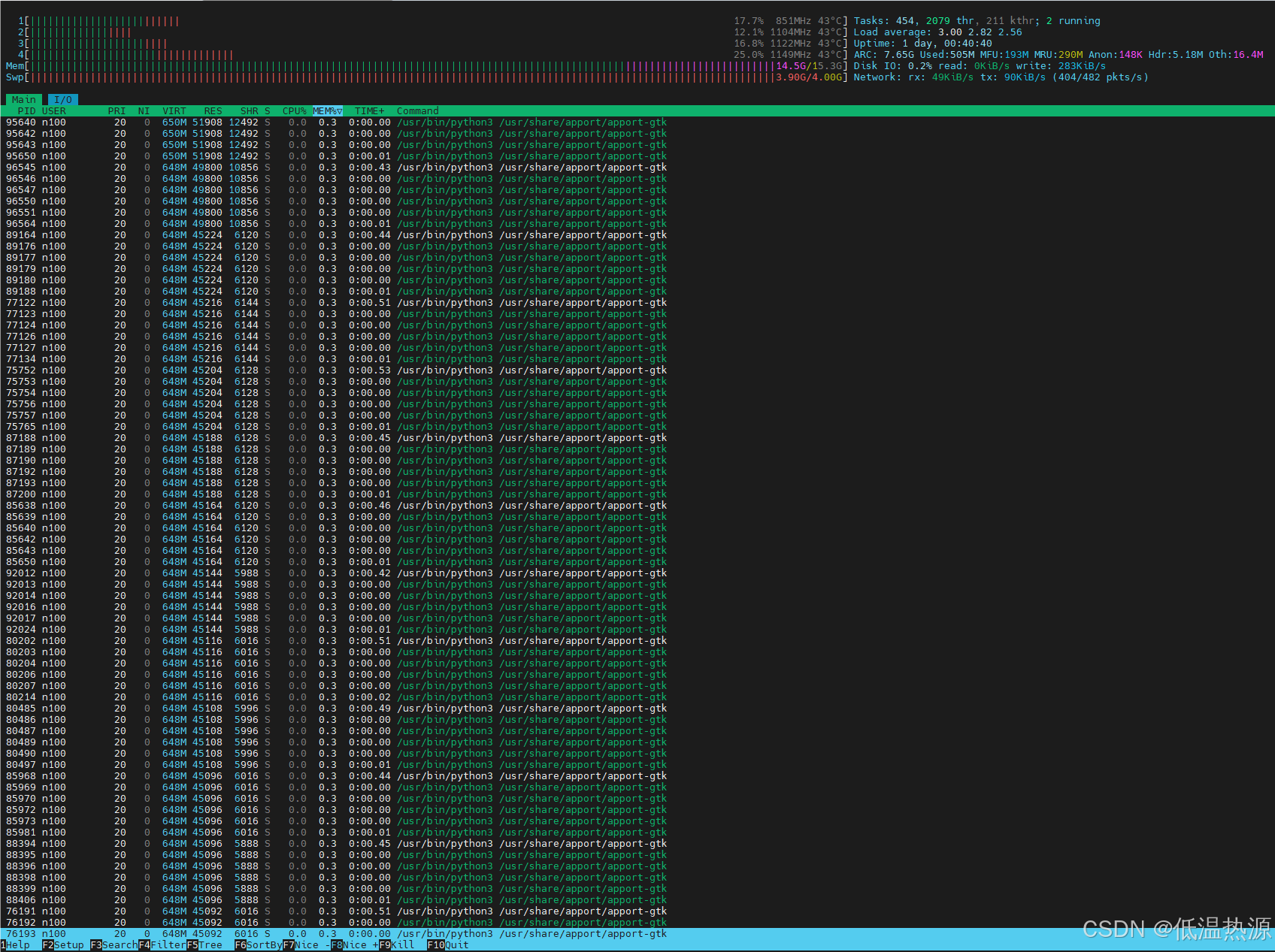
关闭Ubuntu烦人的apport
先来看让人绷不住的(恼) 我查半天apport是啥玩意发现就一错误报告弹窗,十秒钟给我弹一次一天给我内存弹爆了 就算我程序就算真的不停崩溃,也没你这傻比apport杀伤性强啊??? 原则上是不建议关闭…...
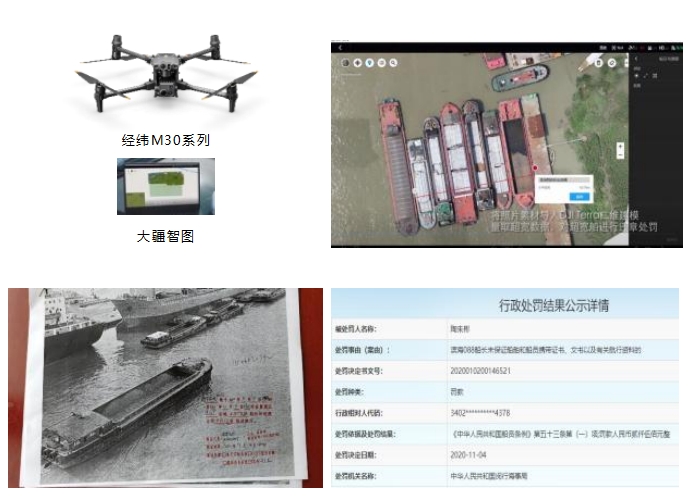
海事无人机解决方案
海事巡察 海事巡察现状 巡查效率低下,存在视野盲区,耗时长,人力成本高。 海事的职能 统一管理水上交通安全和防治船舶污染。 管理通航秩序、通航环境。负责水域的划定和监督管理,维护水 上交通秩序;核定船舶靠泊安…...

Docker--在linux安装软件
Docker 引用Docker原因是在linux中安装软件 以前在linux中安装软件,是直接安装在linux操作系统上,软件和操作系统耦合度很高,不方便管理,因为linux版本不同,环境也就改变了 docker是一种容器技术,提供标…...
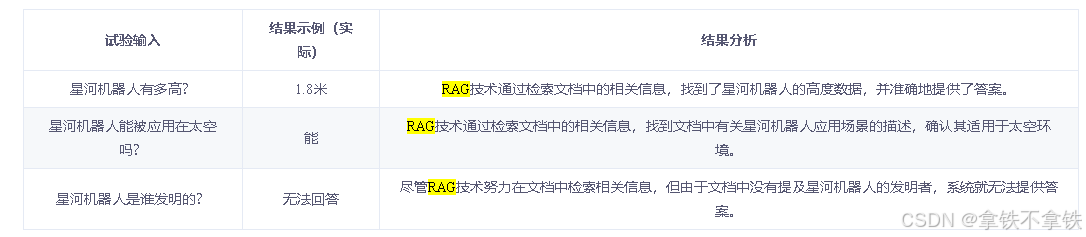
知识库与RAG
认识知识库的技术原理 第一步:📖➡️📈将文档的文本转换为向量,向量存储到向量数据库。第二步:🗨️➡️🔍将用户的提问内容转换成向量,在向量数据库中检索相似的文本内容࿰…...

【2024最新】C++扫描线算法介绍+实战例题
扫描线介绍:OI-Wiki 【简单】一维扫描线(差分优化) 网上一维扫描线很少有人讲,可能认为它太简单了吧,也可能认为这应该算在差分里(事实上讲差分的文章里也几乎没有扫描线的影子)。但我认为&am…...
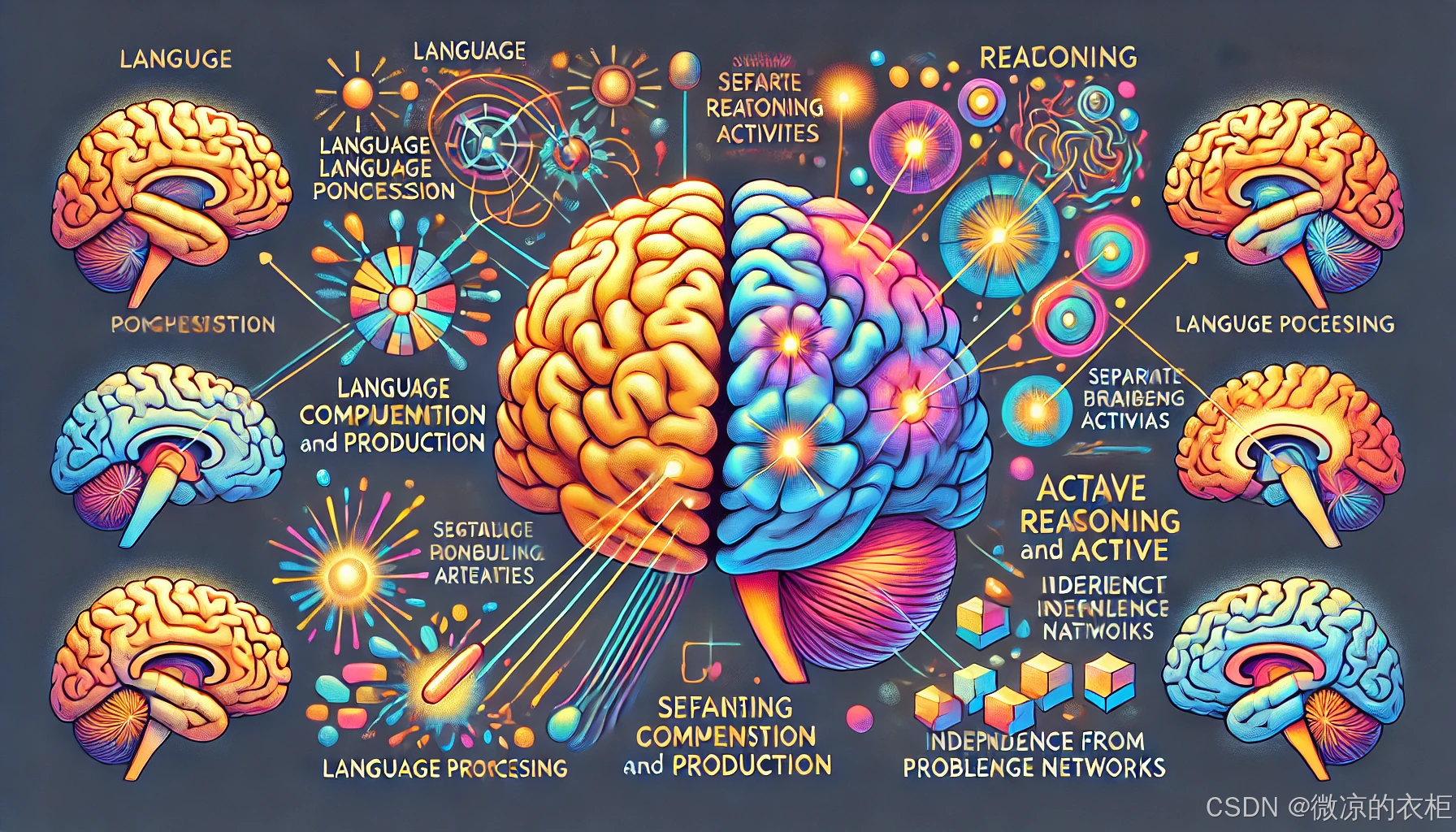
语言主要是一种交流工具,而不是思维工具?GPT5何去何从?
引言 在人工智能领域,特别是大语言模型(LLM)的发展中,语言和思维的关系一直是一个备受关注的话题。近期,麻省理工学院(MIT)在《Nature》杂志上发表了一篇题为《Language is primarily a tool f…...
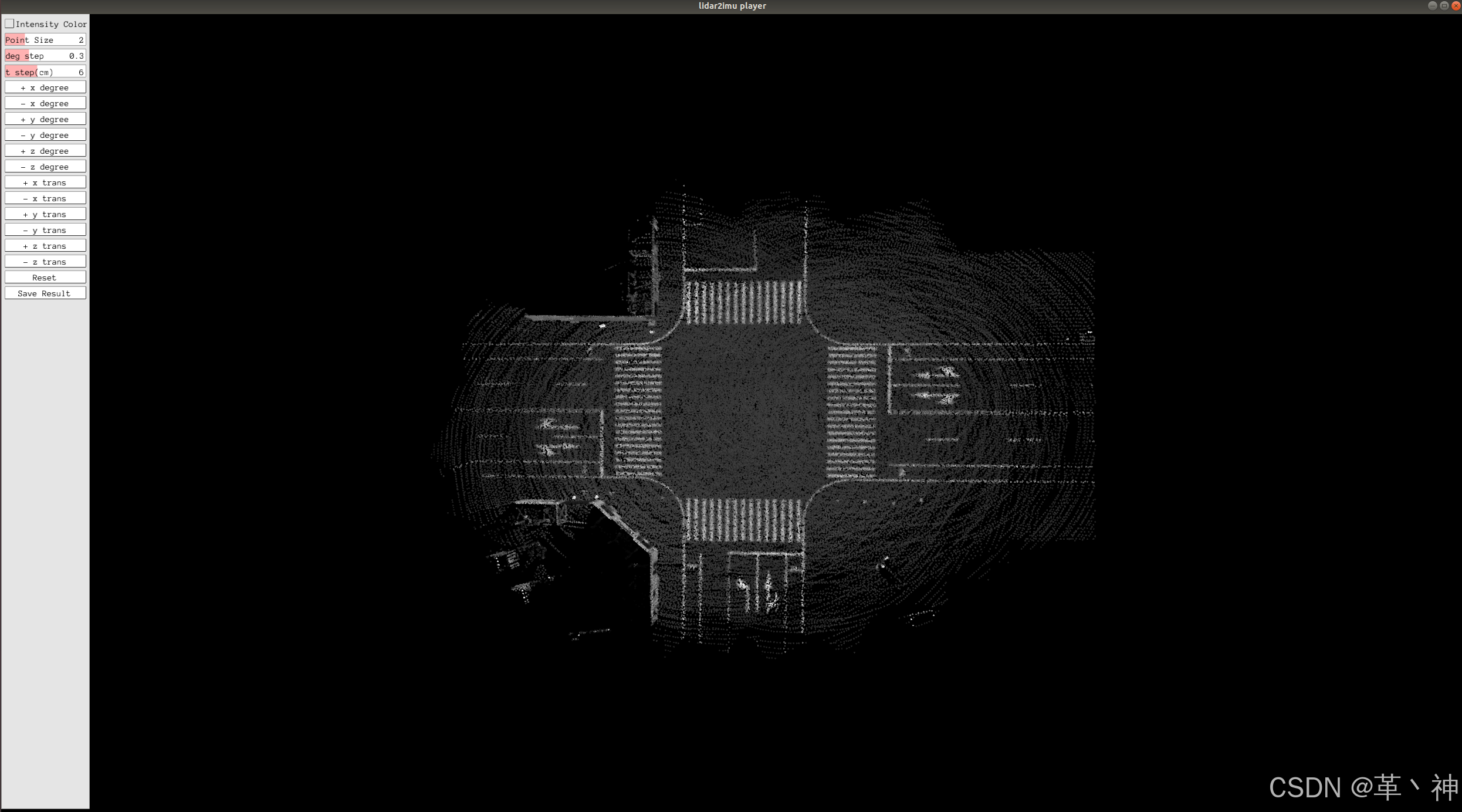
传感器标定(三)激光雷达外参标定(lidar2ins)
一、数据采集 1、LiDAR 传感器的 LiDAR PCD 数据 2、来自 IMU 传感器的姿势文件 3、手动测量传感器之间外部参数初始值并写入的 JSON 文件 二、下载标定工具 //总的git地址: https://github.com/PJLab-ADG/SensorsCalibration git地址: https://githu…...
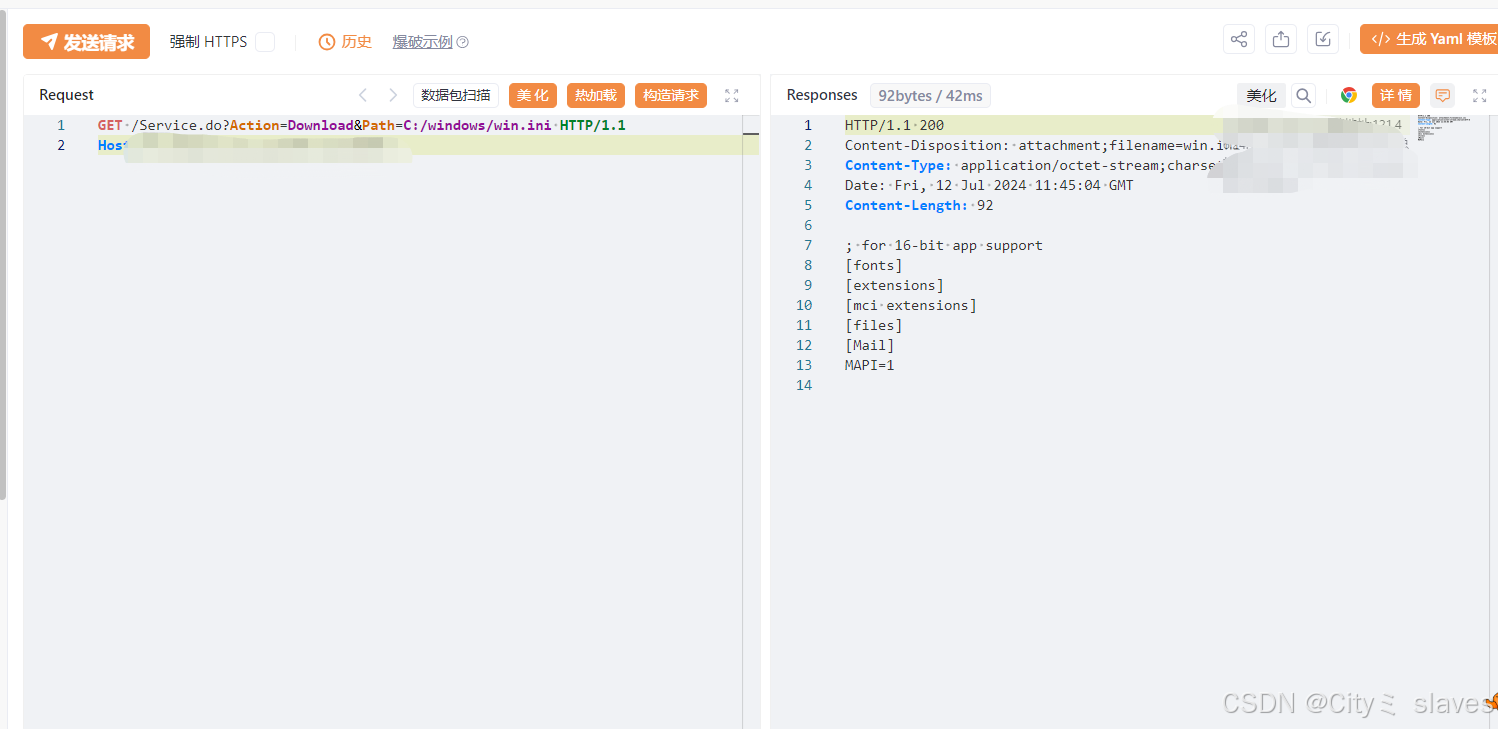
【漏洞复现】Crocus系统—Download 文件读取
声明:本文档或演示材料仅用于教育和教学目的。如果任何个人或组织利用本文档中的信息进行非法活动,将与本文档的作者或发布者无关。 一、漏洞描述 Crocus系统中的Download文件读取漏洞允许未经身份验证的攻击者通过特定请求读取系统上的任意文件。Crocu…...

游戏开发面试题1
说说对单例模式的了解 单例模式(Singleton Pattern)是一种设计模式,其目的是确保一个类只有一个实例,并提供一个全局访问点来访问该实例。这在某些情况下非常有用,比如需要一个唯一的配置管理器、日志记录器、或资源管…...
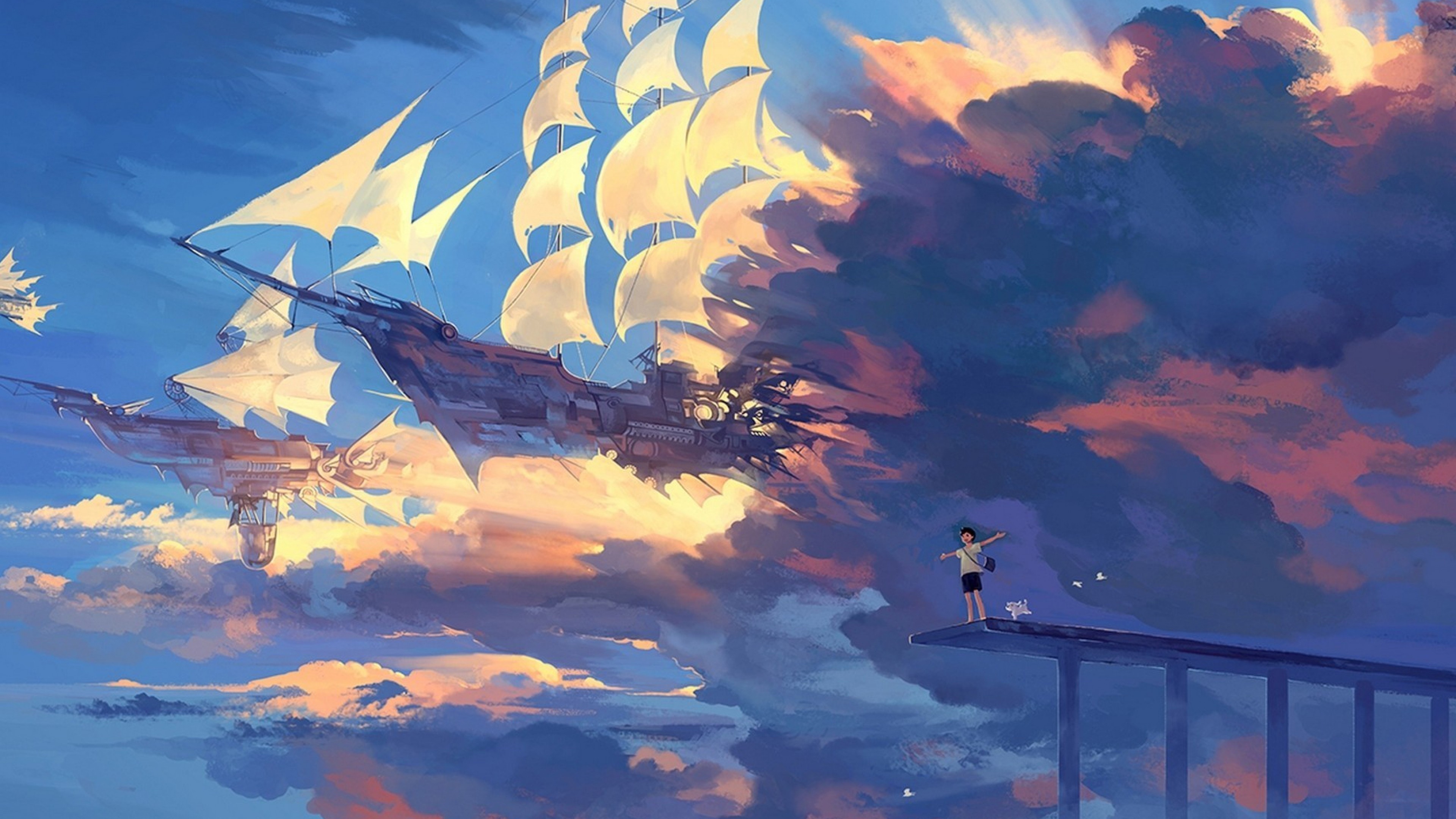
线程池笔记
笔记梳理 前言.PHONYC标准库头文件C/C通用或C特有头文件mkdirc_str()snprintfvsnprintfumaskopen函数可变参数列表va_startva_endfunctionalstatic_castpthread_cond_init_threads.emplace_backstd::bindstd::placeholdersThreadPool(const ThreadPool<T> &tp) dele…...

Go语言基础数据类型、变量及自增语法
本文内容为Go语言的基础数据类型、变量定义和赋值及自增语法介绍。 目录 基础数据类型 变量 先定义后赋值 定义时直接赋值 自动推导定义赋值 平行赋值 自增语法 总结 基础数据类型 int,int8 intl6, int32, int64 uint8... uint64 float32,float64 true/false 变量 …...

ES6-ES13符号:单双引号、变量的解构赋值、占位符 、字符串模版`${} `、扩展运算符...、?,??,_,||=,=,in
原型、this、闭包,for四类循环,ES6-14(2023)_es6-es14-CSDN博客 目录 查看ES版本 单双引号:无区别 变量的解构赋值:声明变量被数组/对象中的元素赋值 推荐用const,因为是从其他地方获取值 …...