成为Python砖家(2): str 最常用的8大方法
str 类最常用的8个方法
str.lower()
str.upper()
str.split(sep=None, maxsplit=-1)
str.count(sub[, start[, end]])
str.replace(old, new[, count])
str.center(width[, fillchar])
str.strip([chars])
str.join(iterable)
查询方法的文档
根据 成为Python砖家(1): 在本地查询Python HTML文档 提供的方法,在本地查询文档,得到对应函数的说明。
8大方法使用举例
# Python3 str 最常用的8大方法
# author: https://github.com/zchrissirhcz
# ref: https://www.bilibili.com/video/BV1JL4y1x7xC/?p=15# h1/8
# str.lower() 返回字符串的副本,全部字符为小写
# file:///Users/zz/Documents/python-3.12.5-docs-html/library/stdtypes.html#str.lower
# Return a copy of the string with all the cased characters [4] converted to lowercase.
s = "HELLO WORLD"
t = s.lower()
print(t)# h2/8
# str.uppper() 返回字符串的副本,全部字符为大写
# file:///Users/zz/Documents/python-3.12.5-docs-html/library/stdtypes.html#str.upper
# Return a copy of the string with all the cased characters [4] converted to uppercase. Note that s.upper().isupper() might be False if s contains uncased characters or if the Unicode category of the resulting character(s) is not “Lu” (Letter, uppercase), but e.g. “Lt” (Letter, titlecase).
u = "hello world"
v = u.upper()
print(v)
print(v.isupper())# h3/8
# str.split(sep=None, maxsplit=-1) 返回一个列表,由 str 根据 sep 被分割的部分组成
# sep 可以是多个字符, 它们的整体被当作分隔符
# maxsplit表示分隔的数量,默认值-1表示分隔所有的;如果是大于0的取值,那么最多可以得到 maxsplit+1 个元素的 list,最后一个是没有被分割的字符串
# file:///Users/zz/Documents/python-3.12.5-docs-html/library/stdtypes.html#str.split
# Return a list of the words in the string, using sep as the delimiter string. If maxsplit is given, at most maxsplit splits are done (thus, the list will have at most maxsplit+1 elements). If maxsplit is not specified or -1, then there is no limit on the number of splits (all possible splits are made).
#
# If sep is given, consecutive delimiters are not grouped together and are deemed to delimit empty strings (for example, '1,,2'.split(',') returns ['1', '', '2']). The sep argument may consist of multiple characters as a single delimiter (to split with multiple delimiters, use re.split()). Splitting an empty string with a specified separator returns [''].
s1 = "The cat sat on the mat, watching the birds through the window while the sun set slowly beyond the horizon."
t1 = s1.split("the")
t2 = s1.split("the", 2)
print("t1", t1)
print("t2", t2)# h4/8
# str.count(sub[, start[, end]]) 返回字串 sub 在 str 中出现的次数
# file:///Users/zz/Documents/python-3.12.5-docs-html/library/stdtypes.html#str.count
# 可选参数 start 和 end 表示范围,相当于 str[start:end]. 注意,这并不会包含 str[end] 字符
# Return the number of non-overlapping occurrences of substring sub in the range [start, end]. Optional arguments start and end are interpreted as in slice notation.
#
# If sub is empty, returns the number of empty strings between characters which is the length of the string plus one.
s1 = "hello world"
cnt = s1.count('o')
print(f"number of 'o' in '{s1}' = {cnt}")
cnt2 = s1.count('') # 12
print(f"number of '' in '{s1}' = {cnt2}")
cnt3 = s1.count('o', 0, 4)
print(cnt3) # 0
cnt4 = s1.count('o', 0, 5)
print(cnt4) # 1s2 = "ababab"
cnt5 = s2.count("ab")
print(cnt5) # 3# h5/8
# str.replace(old, new[, count])
# file:///Users/zz/Documents/python-3.12.5-docs-html/library/stdtypes.html#str.replace
# Return a copy of the string with all occurrences of substring old replaced by new. If the optional argument count is given, only the first count occurrences are replaced.
# 返回字符串 str 的副本, 所有 old 被替换为 new。 如果给了 count 参数,那么只替换前 count 次
s1 = "hello world"
s2 = s1.replace("world", "python")
print(f"s2: {s2}") # hello python# h6/8
# str.center(width[, fillchar])
# file:///Users/zz/Documents/python-3.12.5-docs-html/library/stdtypes.html#str.center
# Return centered in a string of length width. Padding is done using the specified fillchar (default is an ASCII space). The original string is returned if width is less than or equal to len(s).
# 返回字符串 str 根据宽度 width 在左右两侧均等填充 fillchar 后的字符串
# 如果没指定填充字符, 则使用空格填充
# 如果原始字符串长度大于指定的参数 width,那么不填充, 直接返回原始字符串
s1 = "python"
s2 = s1.center(20, "=")
print(s2) # =======python=======# h7/8
# str.strip([chars])
# file:///Users/zz/Documents/python-3.12.5-docs-html/library/stdtypes.html#str.strip
# Return a copy of the string with the leading and trailing characters removed. The chars argument is a string specifying the set of characters to be removed. If omitted or None, the chars argument defaults to removing whitespace. The chars argument is not a prefix or suffix; rather, all combinations of its values are stripped.
# 从字符串左右两侧,删除 chars 里出现的字符
# 参数 chars 类型是字符串,但它里面每个字符都被均等对待,任何在 chars 里的字符,都将被剔除
# 如果 chars 参数没指定,则空白字符会被剔除
s1 = "www.example.com"
s2 = s1.strip('comw')
print(s2) # .example.s3 = "= python= "
s4 = s3.strip(" =np")
print(s4) # "ytho"# h8/8
# str.join(iterable)
# file:///Users/zz/Documents/python-3.12.5-docs-html/library/stdtypes.html#str.join
# str.join(iterable)
# Return a string which is the concatenation of the strings in iterable. A TypeError will be raised if there are any non-string values in iterable, including bytes objects. The separator between elements is the string providing this method.
# 返回一个字符串,是 iterable 参数中的元素的相互拼接,拼接的粘连符号是 str 本身
s1 = ["1", "2", "3"]
s2 = ','.join(s1)
print(s2) # 1,2,3
相关文章:

成为Python砖家(2): str 最常用的8大方法
str 类最常用的8个方法 str.lower()str.upper()str.split(sepNone, maxsplit-1)str.count(sub[, start[, end]])str.replace(old, new[, count])str.center(width[, fillchar])str.strip([chars])str.join(iterable) 查询方法的文档 根据 成为Python砖家(1): 在本地查询Pyth…...
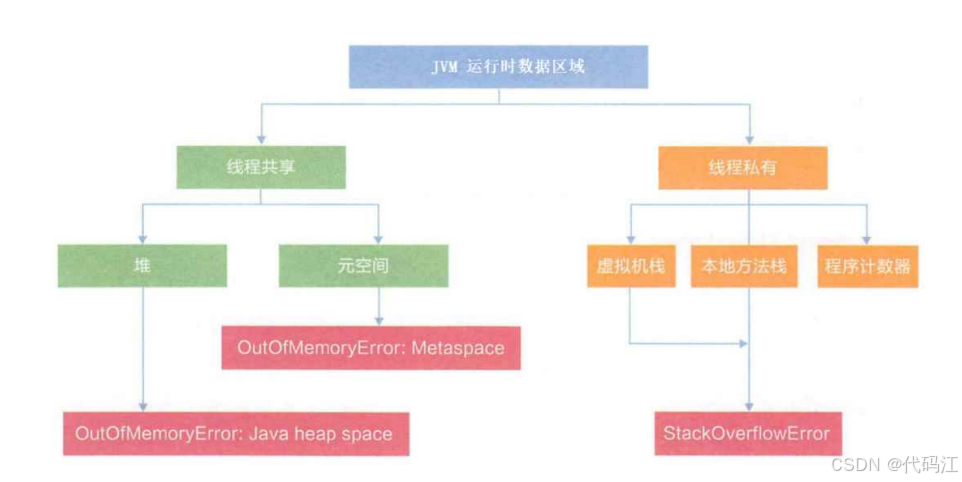
深入理解JVM运行时数据区(内存布局 )5大部分 | 异常讨论
前言: JVM运行时数据区(内存布局)是Java程序执行时用于存储各种数据的内存区域。这些区域在JVM启动时被创建,并在JVM关闭时销毁。它们的布局和管理方式对Java程序的性能和稳定性有着重要影响。 目录 一、由以下5大部分组成 1.…...

JAVA根据表名获取Oracle表结构信息
响应实体封装 import lombok.AllArgsConstructor; import lombok.Builder; import lombok.Data; import lombok.NoArgsConstructor;/*** author CQY* version 1.0* date 2024/8/15 16:33**/ Data NoArgsConstructor AllArgsConstructor Builder public class OracleTableInfo …...

网络性能优化
网络性能优化是确保网络稳定性、速度和可靠性的关键步骤。优化过程通常包括诊断问题、识别瓶颈以及实施具体的解决方案。以下是关于如何进行网络性能优化的详细指南: 一、问题诊断 网络性能监控 网络流量分析工具:使用Wireshark、NetFlow、Ntop等工具监…...
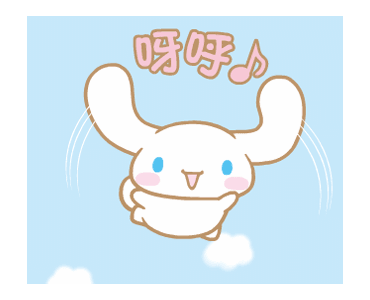
[C++String]接口解读,深拷贝和浅拷贝,string的模拟实现
💖💖💖欢迎来到我的博客,我是anmory💖💖💖 又和大家见面了 欢迎来到C探索系列 作为一个程序员你不能不掌握的知识 先来自我推荐一波 个人网站欢迎访问以及捐款 推荐阅读 如何低成本搭建个人网站…...
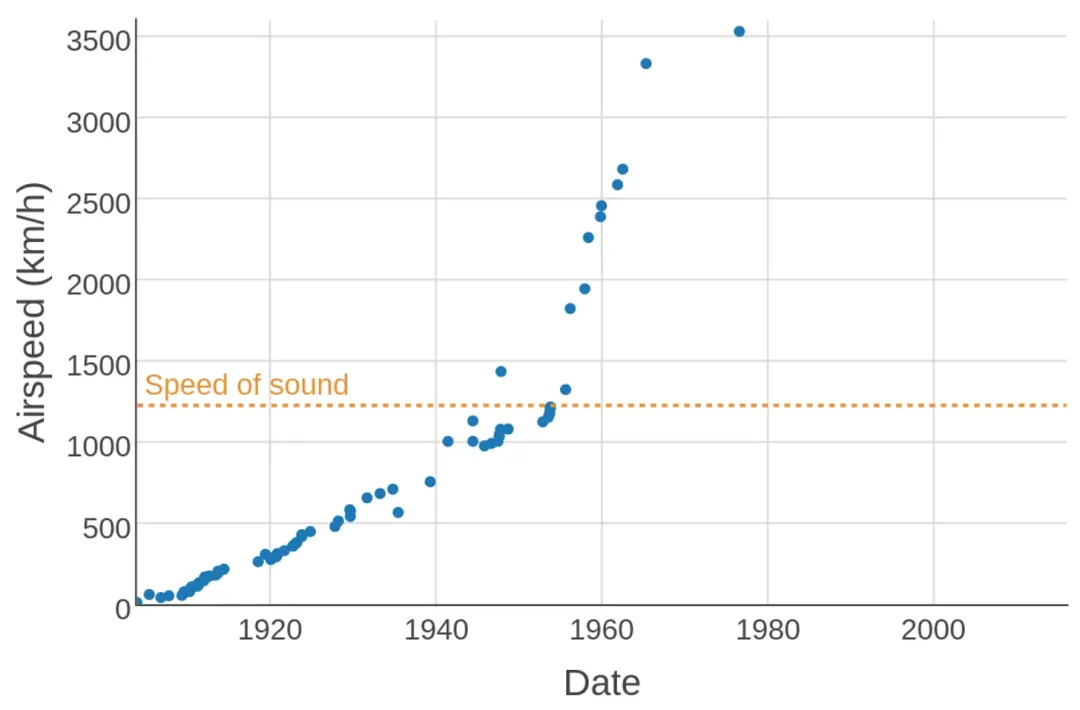
理性看待、正确理解 AI 中的 Scaling “laws”
编者按:LLMs 规模和性能的不断提升,让人们不禁产生疑问:这种趋势是否能一直持续下去?我们是否能通过不断扩大模型规模最终实现通用人工智能(AGI)?回答这些问题对于理解 AI 的未来发展轨迹至关重…...
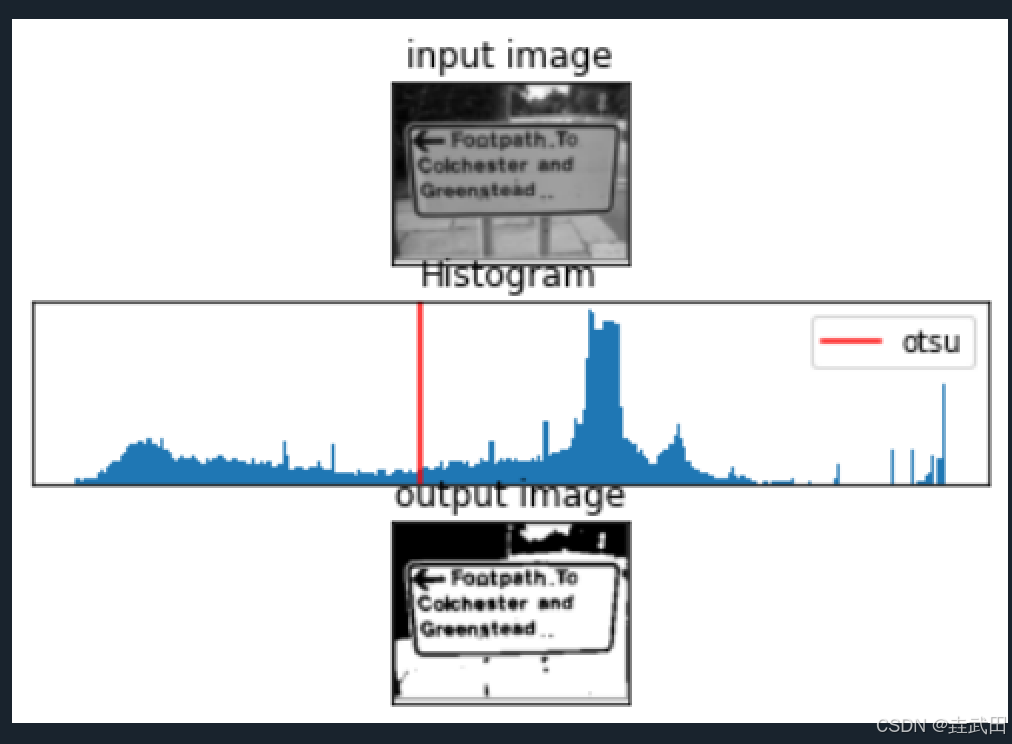
【OCR 学习笔记】二值化——全局阈值方法
二值化——全局阈值方法 固定阈值方法Otsu算法在OpenCV中的实现固定阈值Otsu算法 图像二值化(Image Binarization)是指将像素点的灰度值设为0或255,使图像呈现明显的黑白效果。二值化一方面减少了数据维度,另一方面通过排除原图中…...
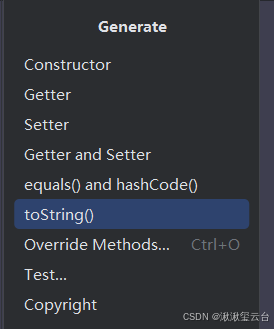
Java - IDEA开发
使用IDEA开发Java程序步骤: 创建工程 Project;创建模块 Module;创建包 Package;创建类;编写代码; 如何查看JDK版本 Package介绍: package是将项目中的各种文件,比如源代码、编译生成的字节码、配置文件、…...

Oracle(62)什么是内存优化表(In-Memory Table)?
内存优化表(In-Memory Table)是指将表的数据存储在内存中,以提高数据访问和查询性能的一种技术。内存优化表通过利用内存的高速访问特性,显著减少I/O操作的延迟,提升数据处理的速度。这种技术在需要高性能数据处理的应…...
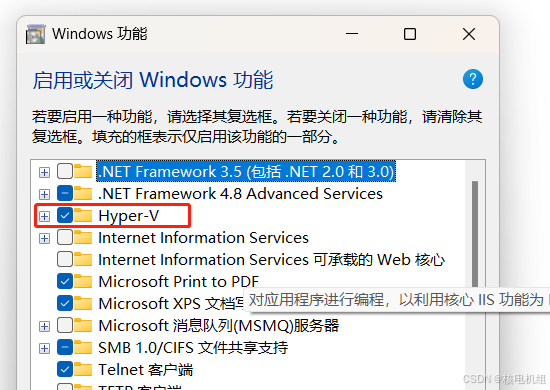
#window家庭版安装hyper-v#
由于window 11 家庭版没有hyper-v虚拟机服务,则需要安装一下,使用如下操作 1:新建一个txt文件,拷贝如下脚本到里面 pushd "%\~dp0" dir /b %SystemRoot%\servicing\Packages\*Hyper-V*.mum >hyper-v.txt for /f %%i in (findst…...
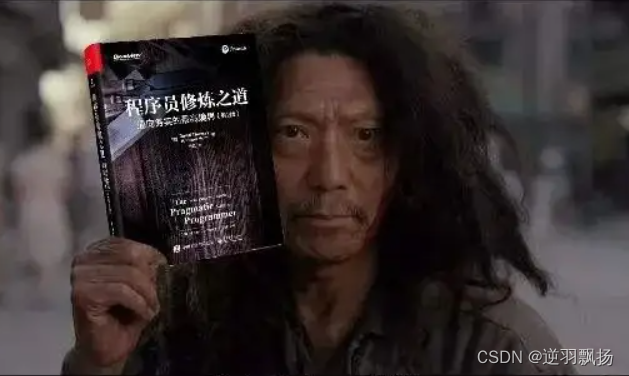
【云原生】Pass容器研发基础——汇总篇
云原生基础汇总 系列综述: 💞目的:本系列是个人整理为了云计算学习的,整理期间苛求每个知识点,平衡理解简易度与深入程度。 🥰来源:每个知识点的修正和深入主要参考各平台大佬的文章,…...
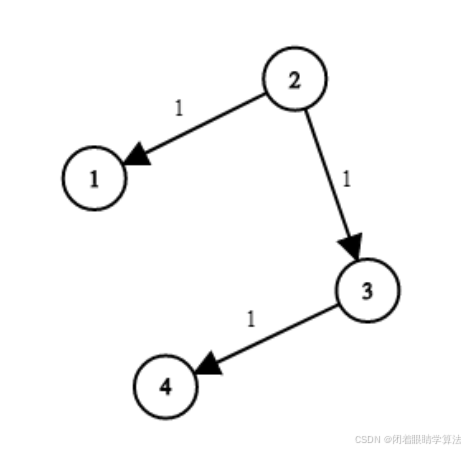
【Py/Java/C++三种语言详解】LeetCode743、网络延迟时间【单源最短路问题Djikstra算法】
可上 欧弟OJ系统 练习华子OD、大厂真题 绿色聊天软件戳 od1441了解算法冲刺训练(备注【CSDN】否则不通过) 文章目录 相关推荐阅读一、题目描述二、题目解析三、参考代码PythonJavaC 时空复杂度 华为OD算法/大厂面试高频题算法练习冲刺训练 相关推荐阅读 …...

交替输出
交替输出 题目:线程 1 输出 a 5 次,线程 2 输出 b 5 次,线程 3 输出 c 5 次。现在要求输出 abcabcabcabcabc wait notify 版 public class SyncWaitNotify {private volatile int flag;private volatile int loopNumber;public SyncWaitNo…...

JS(三)——更改html内数据
获取 DOM 元素,然后修改其属性或内容。使用 getElementById 方法获取特定 ID 的元素: <p id"myParagraph">这是初始的文本</p> const paragraph document.getElementById(myParagraph); paragraph.innerHTML 这是修改后的文本…...
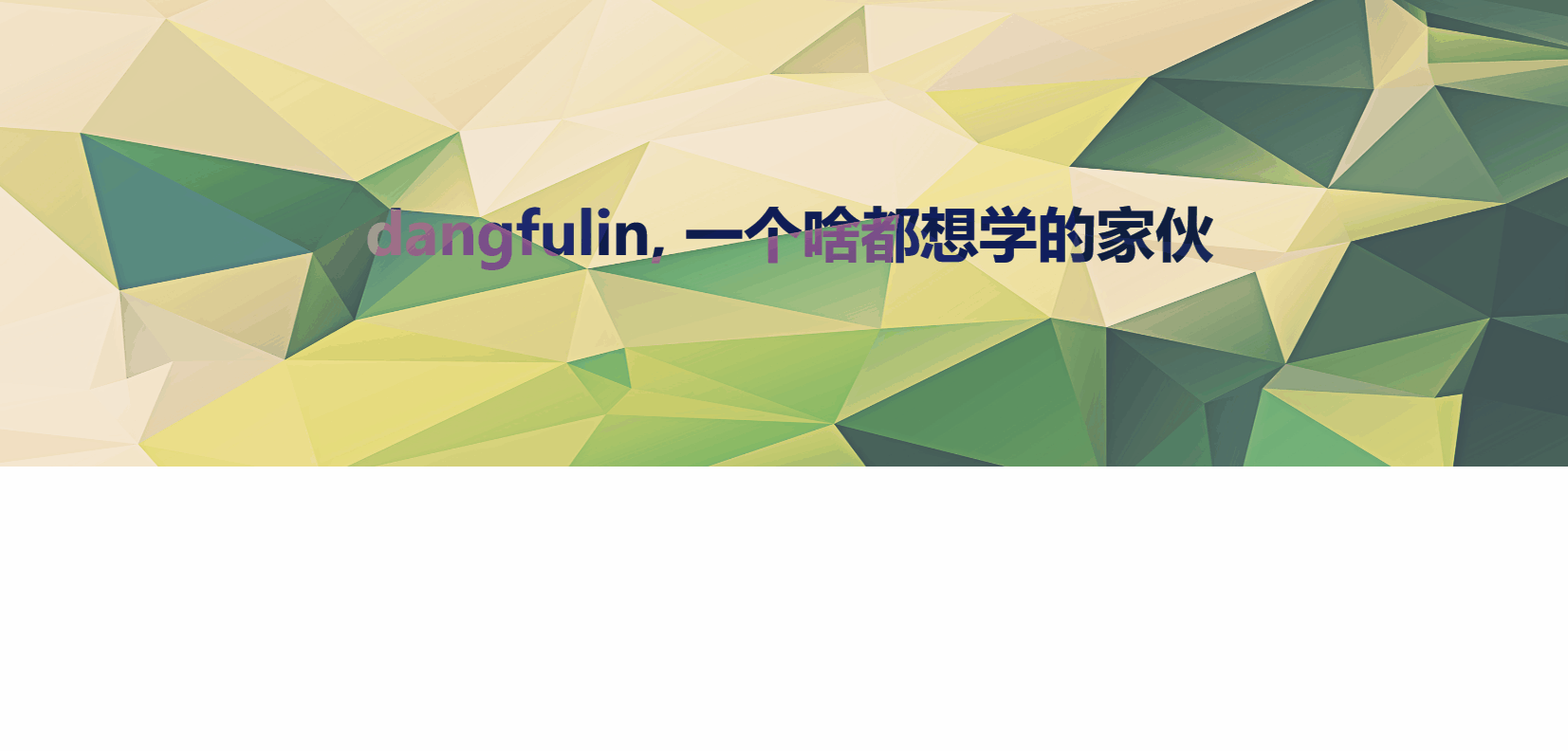
CSS小玩意儿:文字适配背景
一,效果 二,代码 1,搭个框架 添加一张背景图片,在图片中显示一行文字。 <!DOCTYPE html> <html lang"en"> <head><meta charset"UTF-8"><meta name"viewport" conte…...
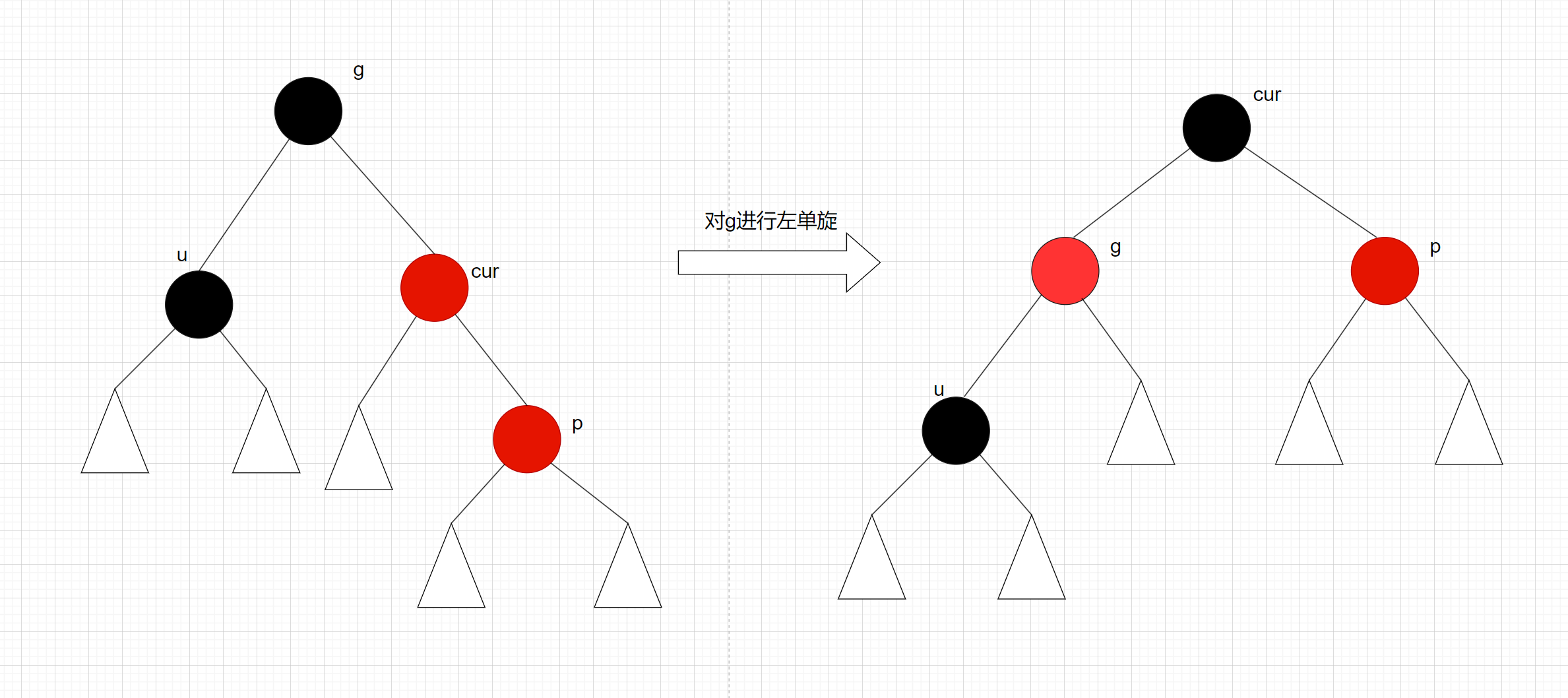
C++:平衡二叉搜索树之红黑树
一、红黑树的概念 红黑树, 和AVL都是二叉搜索树, 红黑树通过在每个节点上增加一个储存位表示节点的颜色, 可以是RED或者BLACK, 通过任何一条从根到叶子的路径上各个节点着色方式的限制,红黑树能够确保没有一条路径会比…...

CentOS 7 系统优化
CentOS 7 系统优化 1、配置YUM源 阿里云的YUM源配置: CentOS 7使用以下命令: sudo wget -O /etc/yum.repos.d/CentOS-Base.repo http://mirrors.aliyun.com/repo/Centos-7.repoCentOS 8使用以下命令: sudo wget -O /etc/yum.repos.d/CentOS…...

扫雷游戏——附源代码
扫雷游戏的源代码比较简单,不设计比较复杂的代码,主要是多个函数的组合,每个函数执行自己的功能,最终支持游戏的完成。 1.菜单 我们需要一个提醒信息来让用户进行选择。 void menu() {printf("***********************\n&…...
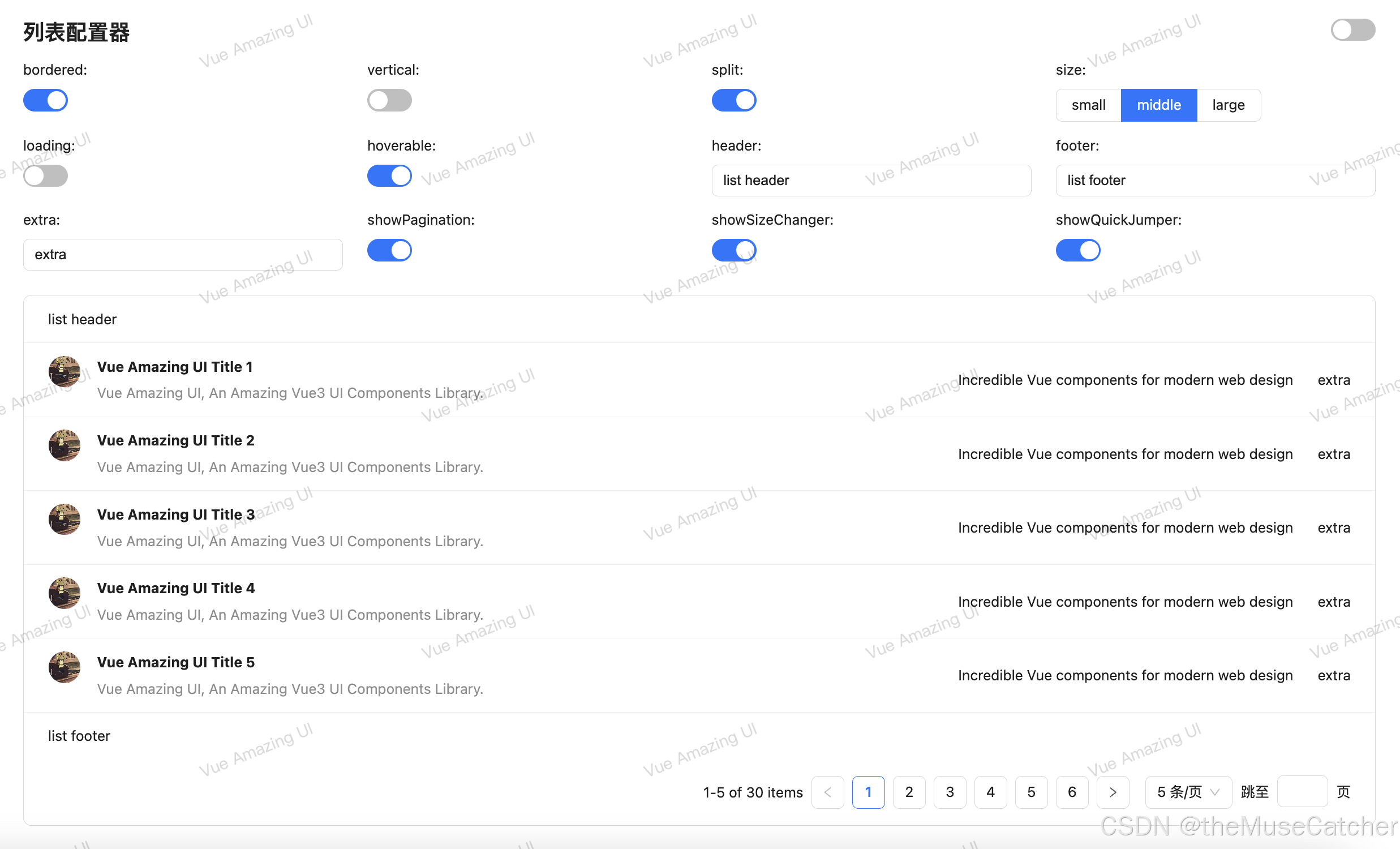
Vue3列表(List)
效果如下图:在线预览 APIs List 参数说明类型默认值bordered是否展示边框booleanfalsevertical是否使用竖直样式booleanfalsesplit是否展示分割线booleantruesize列表尺寸‘small’ | ‘middle’ | ‘large’‘middle’loading是否加载中booleanfalsehoverable是否…...
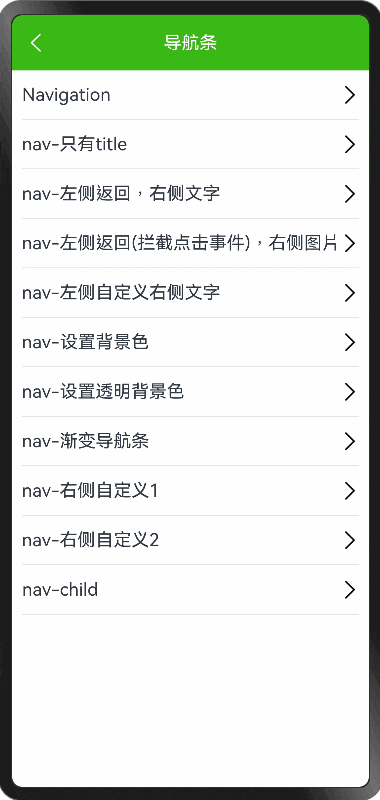
HarmonyOS NEXT - Navigation组件封装BaseNavigation
demo 地址: https://github.com/iotjin/JhHarmonyDemo 代码不定时更新,请前往github查看最新代码 在demo中这些组件和工具类都通过module实现了,具体可以参考HarmonyOS NEXT - 通过 module 模块化引用公共组件和utils 官方介绍 组件导航 (Navigation)(推…...
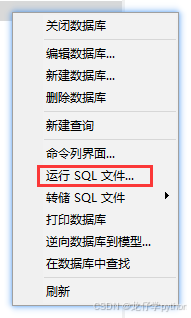
浅看MySQL数据库
有这么一句话:“一个不会数据库的程序员不是合格的程序员”。有点夸张,但是确是如此。透彻学习数据库是要学习好多知识,需要学的东西也是偏难的。我们今天来看数据库MySQL的一些简单基础东西,跟着小编一起来看一下吧。 什么是数据…...

Pytorch常用训练套路框架(CPU)
文章目录 1. 数据准备示例:加载 CIFAR-10 数据集 2. 模型定义示例:定义一个简单的卷积神经网络 3. 损失函数和优化器示例:定义损失函数和优化器 4. 训练循环示例:训练循环 5. 评估和测试示例:评估模型 6. 保存和加载模…...
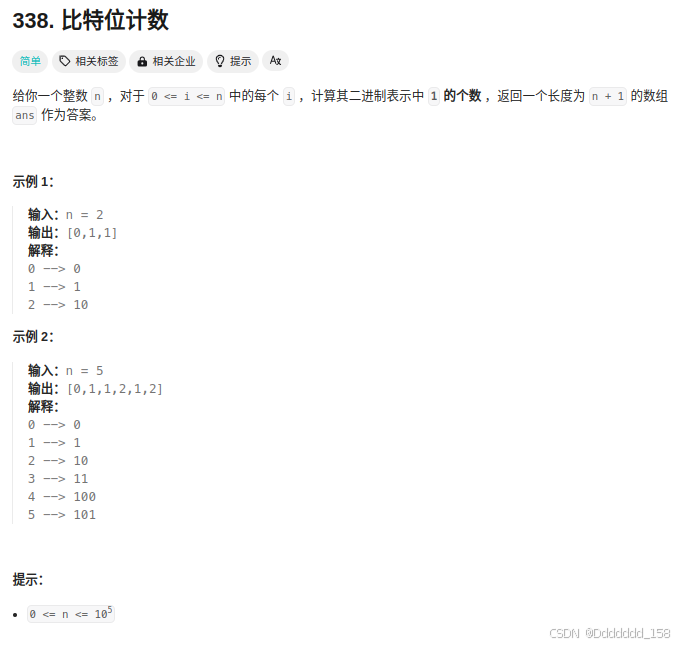
C++ | Leetcode C++题解之第338题比特位计数
题目: 题解: class Solution { public:vector<int> countBits(int n) {vector<int> bits(n 1);for (int i 1; i < n; i) {bits[i] bits[i & (i - 1)] 1;}return bits;} };...
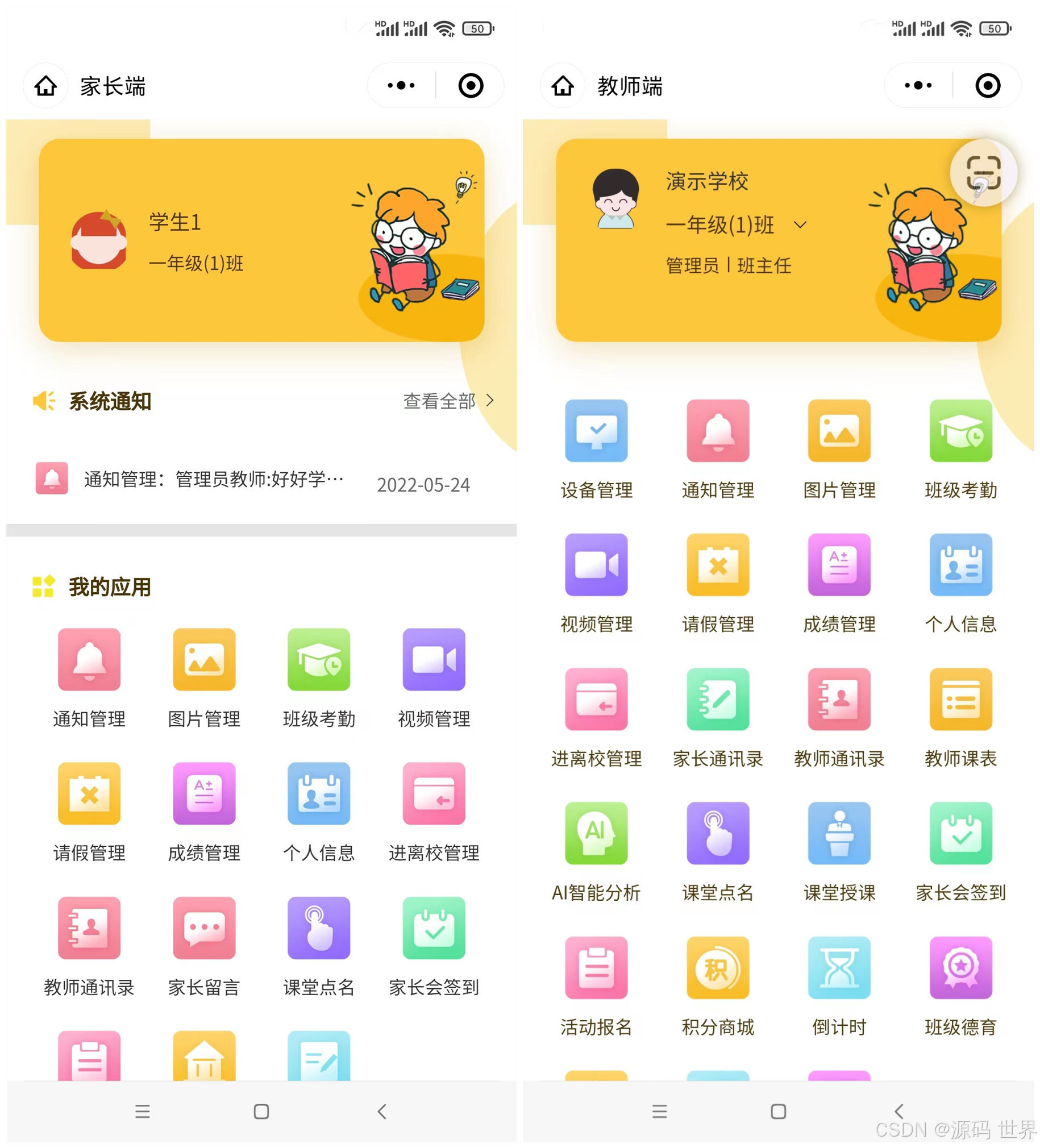
智慧校园云平台电子班牌系统源码,智慧教育一体化云解决方案
智慧校园云平台电子班牌系统,利用先进的云计算技术,将教育信息化资源和教学管理系统进行有效整合,实现生态基础数据共享、应用生态统一管理,为智慧教育建设的统一性,稳定性,可扩展性,互通性提供…...

数据库系统 第17节 数据仓库 案例赏析
下面我将通过几个具体的案例来说明数据仓库如何在不同的行业中发挥作用,并解决实际业务问题。 案例 1: 零售业 背景: 一家大型零售商希望改进其库存管理和市场营销策略,以提高销售额和顾客满意度。 解决方案: 数据仓库: 构建一个数据仓库࿰…...
硬件面试经典 100 题(71~90 题)
71、请问下图电路的作用是什么? 该电路实现 IIC 信号的电平转换(3.3V 和 5V 电平转换),并且是双向通信的。 上下两路是一样的,只分析 SDA 一路: 1) 从左到右通信(SDA2 为输入状态&…...

【git】代理相关
问题: 开启了翻墙代理工具,拉取代码时报错:fatal: 无法访问 xxxx : Failed to connect to github.com port 443: 连接超时 解决: 0,取消代理仍然无法拉取 1,查看控制面板-网络与Internet-代理ÿ…...

golang gin框架中创建自定义中间件的2种方式总结 - func(*gin.Context)方式和闭包函数方式定义gin中间件
在gin框架中,我们可以通过2种方式创建自定义中间件: 1. 直接定义一个类型为 func(*gin.Context)的函数或者方法 这种方式是我们常用的方式,也就是定义一个参数为*gin.Context的函数或者方法。定义的方法就是创建一个 参数类型为 gin.Handler…...

Linux高级编程 8.13 文件IO
一、文件IO 操作系统为了方便用户使用系统功能而对外提供的一组系统函数。称之为 系统调用(unistd.h) 其中有个 文件IO,一般都是对设备文件操作,当然也可以对普通文件进行操作。 这是一个基于Linux内核的没有缓存的IO机制 文件IO特性&…...

【k8s】ubuntu18.04 containerd 手动从1.7.15 换为1.7.20
ubutnu18.04之前手动安装了1.7.15现在下载1.7.20containerd-1.7.20-linux-amd64.tar.gz root@k8s-worker-i58265u:/home/zhangbin# root@k8s-worker-i58265u:/home/zhangbin# https://github.com/containerd/containerd/releases/download/v1.7.20/containerd-1.7.20-linux-am…...