3.langchain中的prompt模板 (few shot examples in chat models)
本教程将介绍如何使用LangChain库和智谱清言的 GLM-4-Plus 模型来理解和推理一个自定义的运算符(例如使用鹦鹉表情符号🦜)。我们将通过一系列示例来训练模型,使其能够理解和推断该运算符的含义。
环境准备
首先,确保你已经安装了以下库:
langchain_openai
langchain_core
langchain_chroma
langchain_community
你可以使用以下命令进行安装:
pip install langchain_openai langchain_core langchain_chroma langchain_community
第一步:初始化模型
首先,初始化OpenAI的Chat模型。
from langchain_openai import ChatOpenAImodel = ChatOpenAI(temperature=0,model="GLM-4-Plus",openai_api_key="your api key",openai_api_base="https://open.bigmodel.cn/api/paas/v4/"
)
第二步:初步测试模型
测试一下模型对自定义运算符的理解。
response = model.invoke("What is 2 🦜 9?")
print(response.content)
输出结果:
The notation "2 🦜 9" is not a standard mathematical or widely recognized symbol. It appears to be using an emoji (a parrot) in place of a traditional operator. Without additional context or clarification, it's difficult to determine the intended meaning.However, if we consider the parrot emoji as a playful or whimsical substitute for a standard mathematical operation, we might speculate on a few possibilities:1. **Repetition or Iteration**: Parrots are known for repeating sounds. If the parrot emoji is meant to imply repetition, "2 🦜 9" could be interpreted as repeating the number 2 nine times, resulting in "222222222".2. **Multiplication**: If the parrot is whimsically standing in for a multiplication sign, "2 🦜 9" could mean \(2 \times 9 = 18\).3. **Concatenation**: If the parrot is meant to indicate combining the numbers, "2 🦜 9" could simply mean concatenating 2 and 9 to form the number 29.Without further context, these are just educated guesses. If you have more information about the context or the specific rules governing the use of the parrot emoji in this notation, please provide it for a more accurate interpretation.
第三步:构建示例提示
为了让模型更好地理解自定义运算符,提供一些示例。
from langchain_core.prompts import ChatPromptTemplate, FewShotChatMessagePromptTemplateexamples = [{"input": "2 🦜 2", "output": "4"},{"input": "2 🦜 3", "output": "5"},
]example_prompt = ChatPromptTemplate.from_messages([("human", "{input}"),("ai", "{output}"),]
)
few_shot_prompt = FewShotChatMessagePromptTemplate(example_prompt=example_prompt,examples=examples,
)print(few_shot_prompt.invoke({}).to_messages())
输出结果:
[HumanMessage(content='2 🦜 2', additional_kwargs={}, response_metadata={}), AIMessage(content='4', additional_kwargs={}, response_metadata={}), HumanMessage(content='2 🦜 3', additional_kwargs={}, response_metadata={}), AIMessage(content='5', additional_kwargs={}, response_metadata={})]
第四步:构建最终提示
将示例提示与系统提示结合起来,构建最终的提示。
final_prompt = ChatPromptTemplate.from_messages([("system", "You are a wondrous wizard of math."),few_shot_prompt,("human", "{input}"),]
)
第五步:链式调用模型
我们将最终提示与模型结合起来,进行链式调用。
chain = final_prompt | modelresponse = chain.invoke({"input": "What is 2 🦜 9?"})
print(response.content)
输出结果:
The symbol "🦜" seems to represent an operation, and based on the previous examples:- 2 🦜 2 = 4
- 2 🦜 3 = 5It appears that the operation "🦜" adds the second number to the first number. So, following this pattern:2 🦜 9 = 2 + 9 = 11Therefore, 2 🦜 9 is 11.
第六步:使用语义相似性选择示例
为了进一步提高模型的推理能力,我们可以使用语义相似性来选择最相关的示例。
from langchain_chroma import Chroma
from langchain_core.example_selectors import SemanticSimilarityExampleSelectorexamples = [{"input": "2 🦜 2", "output": "4"},{"input": "2 🦜 3", "output": "5"},{"input": "2 🦜 4", "output": "6"},{"input": "What did the cow say to the moon?", "output": "nothing at all"},{"input": "Write me a poem about the moon","output": "One for the moon, and one for me, who are we to talk about the moon?",},
]from langchain_community.embeddings import ZhipuAIEmbeddingsto_vectorize = [" ".join(example.values()) for example in examples]
embeddings = ZhipuAIEmbeddings(model="embedding-3",api_key="your api key",
)
vectorstore = Chroma.from_texts(to_vectorize, embeddings, metadatas=examples)example_selector = SemanticSimilarityExampleSelector(vectorstore=vectorstore,k=2,
)selected_examples = example_selector.select_examples({"input": "What's 3 🦜 3?"})
print(selected_examples)
输出结果:
[{'input': '2 🦜 3', 'output': '5'}, {'input': '2 🦜 4', 'output': '6'}]
第七步:构建新的提示并调用模型
使用选定的示例构建新的提示,并调用模型。
from langchain_core.prompts import ChatPromptTemplate, FewShotChatMessagePromptTemplate# Define the few-shot prompt.
few_shot_prompt = FewShotChatMessagePromptTemplate(# The input variables select the values to pass to the example_selectorinput_variables=["input"],example_selector=example_selector,example_prompt=ChatPromptTemplate.from_messages([("human", "{input}"), ("ai", "{output}")]),
)print(few_shot_prompt.invoke(input="What's 3 🦜 3?").to_messages())
输出结果:
[HumanMessage(content='2 🦜 3', additional_kwargs={}, response_metadata={}), AIMessage(content='5', additional_kwargs={}, response_metadata={}), HumanMessage(content='2 🦜 4', additional_kwargs={}, response_metadata={}), AIMessage(content='6', additional_kwargs={}, response_metadata={})]
final_prompt = ChatPromptTemplate.from_messages([("system", "You are a wondrous wizard of math."),few_shot_prompt,("human", "{input}"),]
)print(final_prompt.invoke(input="What's 3 🦜 3?").to_messages())
输出结果:
[SystemMessage(content='You are a wondrous wizard of math.', additional_kwargs={}, response_metadata={}), HumanMessage(content='2 🦜 3', additional_kwargs={}, response_metadata={}), AIMessage(content='5', additional_kwargs={}, response_metadata={}), HumanMessage(content='2 🦜 4', additional_kwargs={}, response_metadata={}), AIMessage(content='6', additional_kwargs={}, response_metadata={}), HumanMessage(content="What's 3 🦜 3?", additional_kwargs={}, response_metadata={})]
chain = final_prompt | modelprint(chain.invoke({"input": "What's 3 🦜 3?"}).content)
输出结果:
It seems like the "🦜" symbol is being used as an operation, but its specific rules aren't standard in mathematics. Based on the previous examples:- 2 🦜 3 = 5
- 2 🦜 4 = 6One possible interpretation is that "🦜" might represent an operation where you add the two numbers together. Following this pattern:- 3 🦜 3 would be 3 + 3 = 6So, 3 🦜 3 = 6. However, if there's a different rule or context for this operation, please provide more details for a precise answer!
参考链接:https://python.langchain.com/docs/how_to/few_shot_examples_chat/
希望这个教程对你有所帮助!如果有任何问题,欢迎随时提问。
相关文章:

3.langchain中的prompt模板 (few shot examples in chat models)
本教程将介绍如何使用LangChain库和智谱清言的 GLM-4-Plus 模型来理解和推理一个自定义的运算符(例如使用鹦鹉表情符号🦜)。我们将通过一系列示例来训练模型,使其能够理解和推断该运算符的含义。 环境准备 首先,确保…...
量子感知机
神经网络类似于人类大脑,是模拟生物神经网络进行信息处理的一种数学模型。它能解决分类、回归等问题,是机器学习的重要组成部分。量子神经网络是将量子理论与神经网络相结合而产生的一种新型计算模式。1995年美国路易斯安那州立大学KAK教授首次提出了量子…...
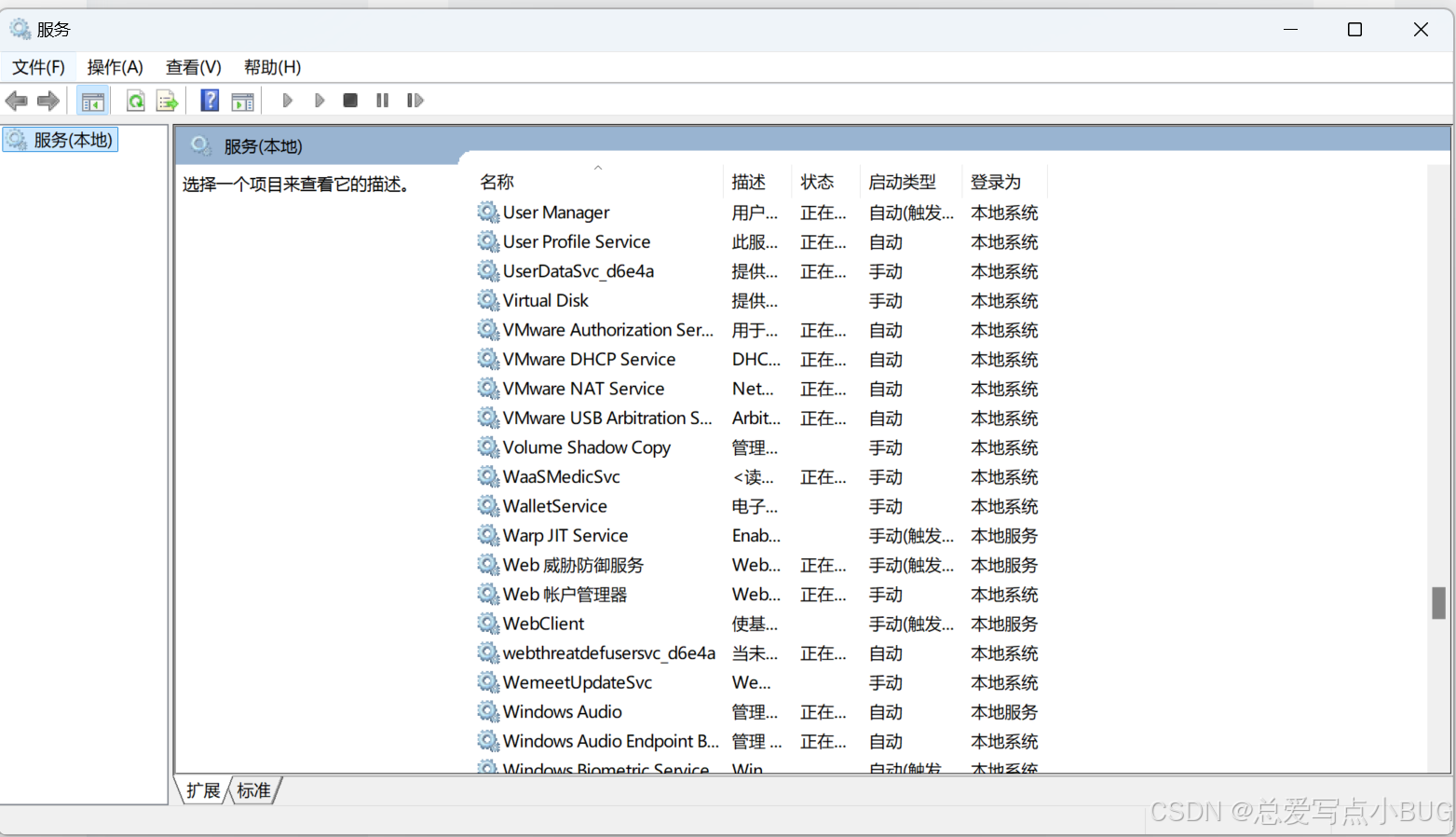
VM虚拟机装MAC后无法联网,如何解决?
✨在vm虚拟机上,给虚拟机MacOS设置网络适配器。选择NAT模式用于共享主机的IP地址 ✨在MacOS设置中设置网络 以太网 使用DHCP ✨回到本地电脑上,打开 服务,找到VMware DHCP和VMware NAT,把这两个服务打开,专一般问题就…...
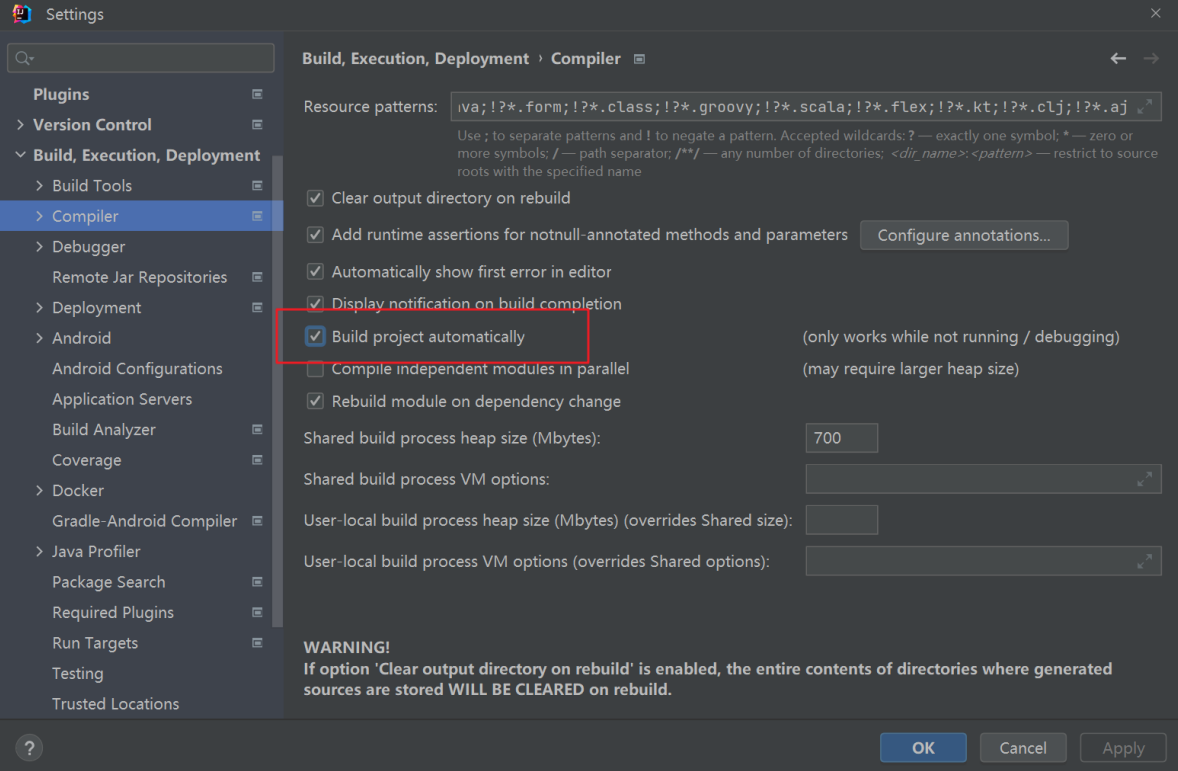
IDEA 基本设置
设置主题 设置字体 设置编码格式 改变字体大小 开启 按住 ctrl 滚轮 改变字体大小。 开启自动编译...
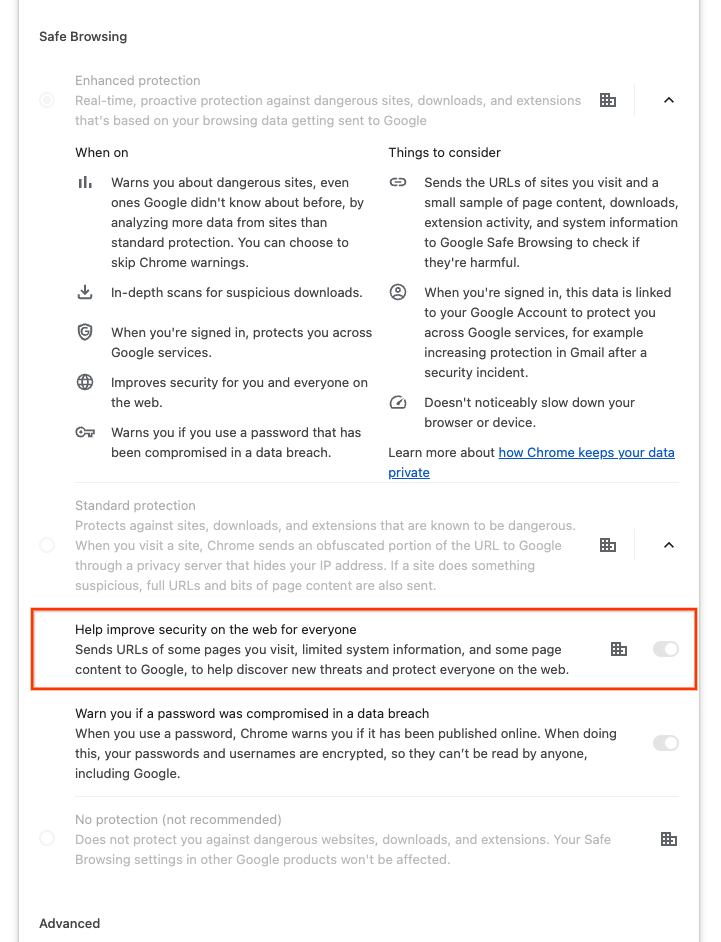
Chrome 浏览器 131 版本新特性
Chrome 浏览器 131 版本新特性 一、Chrome 浏览器 131 版本更新 1. 在 iOS 上使用 Google Lens 搜索 自 Chrome 126 版本以来,用户可以通过 Google Lens 搜索屏幕上看到的任何图片或文字。 要使用此功能,请访问网站,并点击聚焦时出现在地…...

使用php和Xunsearch提升音乐网站的歌曲搜索效果
文章精选推荐 1 JetBrains Ai assistant 编程工具让你的工作效率翻倍 2 Extra Icons:JetBrains IDE的图标增强神器 3 IDEA插件推荐-SequenceDiagram,自动生成时序图 4 BashSupport Pro 这个ides插件主要是用来干嘛的 ? 5 IDEA必装的插件&…...
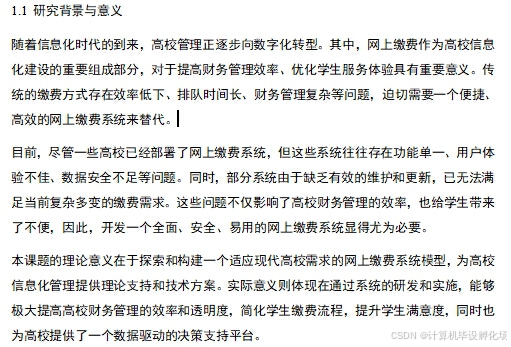
计算机毕设-基于springboot的高校网上缴费综合务系统视频的设计与实现(附源码+lw+ppt+开题报告)
博主介绍:✌多个项目实战经验、多个大型网购商城开发经验、在某机构指导学员上千名、专注于本行业领域✌ 技术范围:Java实战项目、Python实战项目、微信小程序/安卓实战项目、爬虫大数据实战项目、Nodejs实战项目、PHP实战项目、.NET实战项目、Golang实战…...
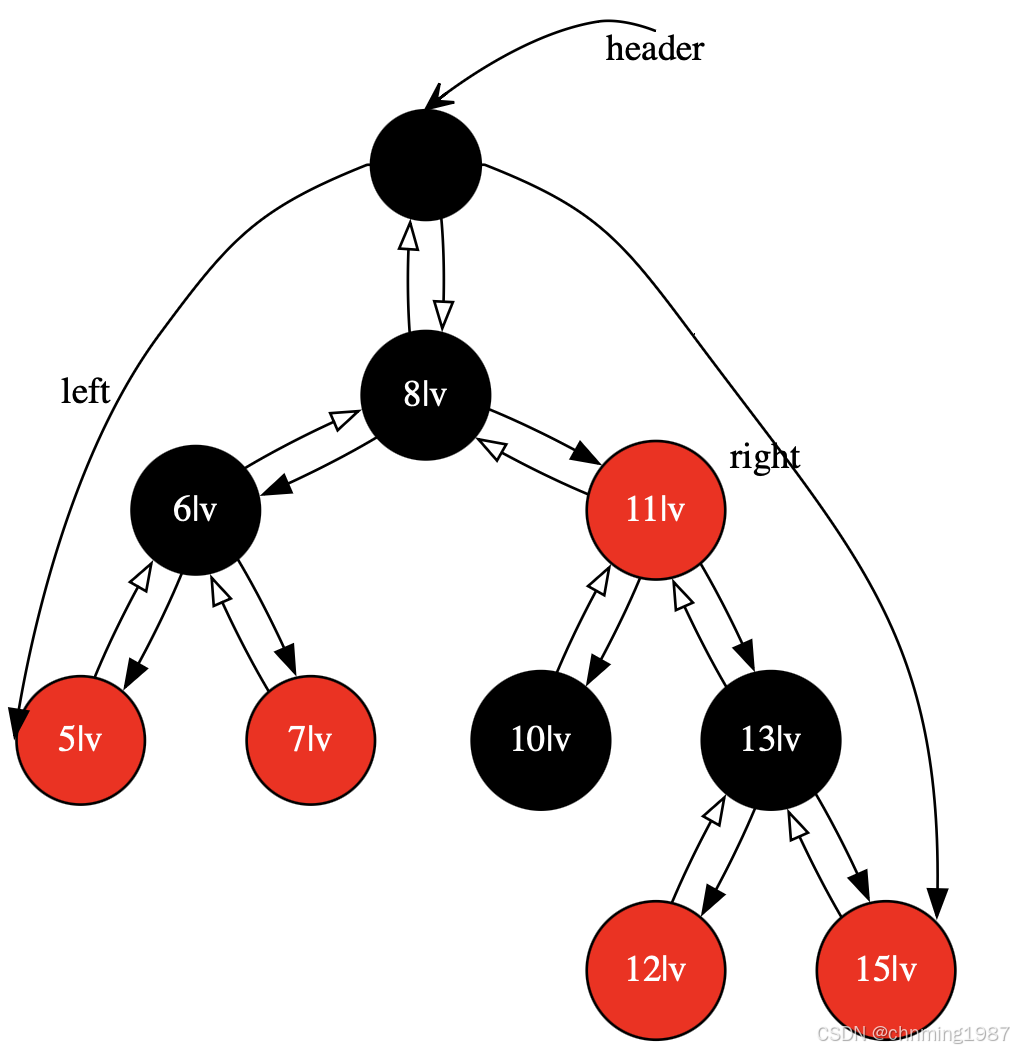
STL关联式容器之map
map的特性是,所有元素都会根据元素的键值自动被排序。map的所有元素都是pair,同时拥有实值(value)和键值(key)。pair的第一元素被视为键值,第二元素被视为实值。map不允许两个元素拥有相同的键值。下面是<stl_pair.h>中pair的定义 tem…...
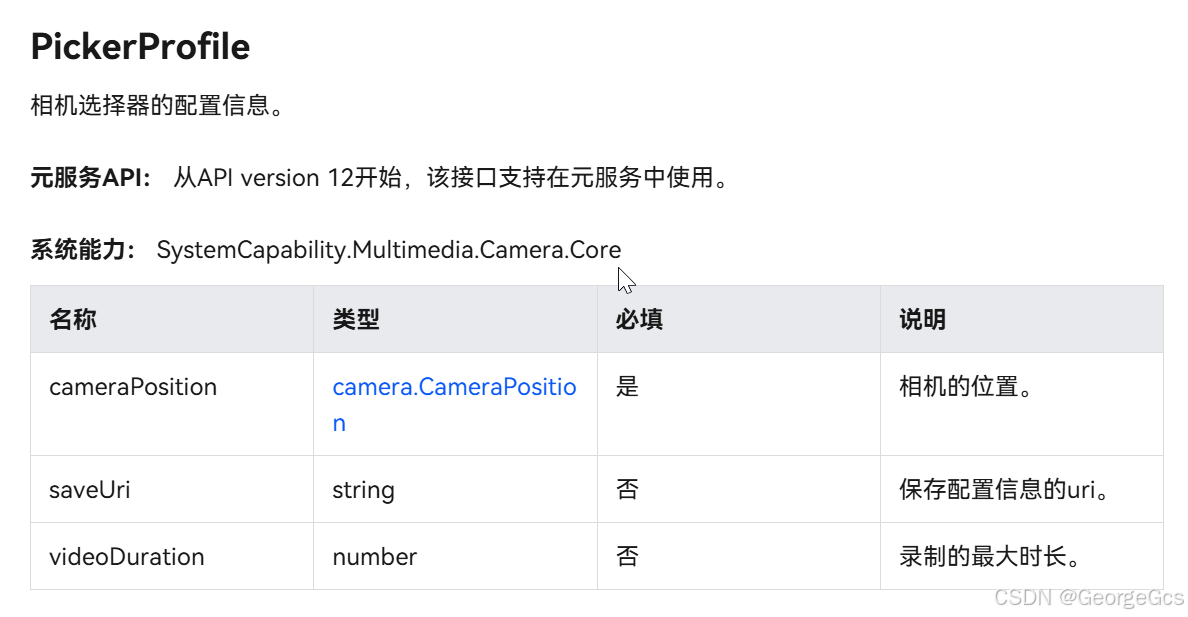
【HarmonyOS】鸿蒙应用唤起系统相机拍照
【HarmonyOS】鸿蒙应用唤起系统相机拍照 方案一: 官方推荐的方式,使用CameraPicker来调用安全相机进行拍照。 let pathDir getContext().filesDir;let fileName ${new Date().getTime()}let filePath pathDir /${fileName}.tmpfileIo.createRandomA…...

Linux系统使用valgrind分析C++程序内存资源使用情况
内存占用是我们开发的时候需要重点关注的一个问题,我们可以人工根据代码推理出一个消耗内存较大的函数,也可以推理出大概会消耗多少内存,但是这种方法不仅麻烦,而且得到的只是推理的数据,而不是实际的数据。 我们可以…...

Java基础夯实——2.7 线程上下文切换
线程上下文切换(Thread Context Switching)是操作系统在多线程环境中,切换CPU从执行一个线程的上下文到另一个线程的上下文的过程。这种切换是实现多线程并发执行的核心机制之一。 1 上下文: 线程的上下文指线程在某一时刻的执行状态,如&am…...

死锁相关习题 10道 附详解
2022 设系统中有三种类型的资源(A,B,C)和五个进程(P1,P2,P3,P4,P5),A资源的数量是17,B资源的数量是6,C资源的数量是19。在T0时刻系统的状态: 最大资源需求量已分配资源量A,B,CA,B,…...
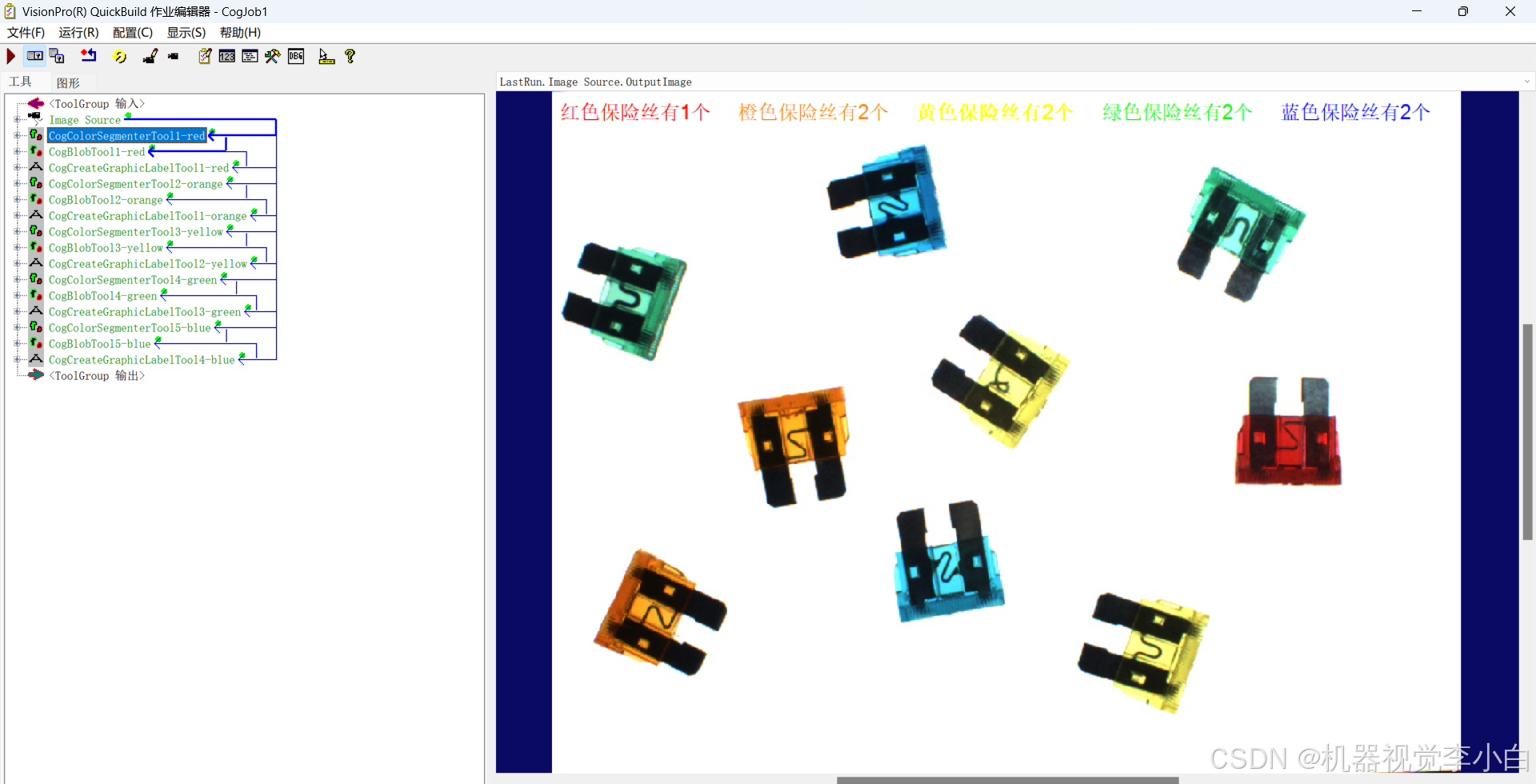
VisionPro 机器视觉案例 之 彩色保险丝个数统计
第十四篇 机器视觉案例 之 彩色保险丝颜色识别个数统计 文章目录 第十四篇 机器视觉案例 之 彩色保险丝颜色识别个数统计1.案例要求2.实现思路2.1 方法一 颜色分离工具CogColorSegmenterTool将每一种颜色分离出来,得到对应的单独图像,使用斑点工具CogBlo…...
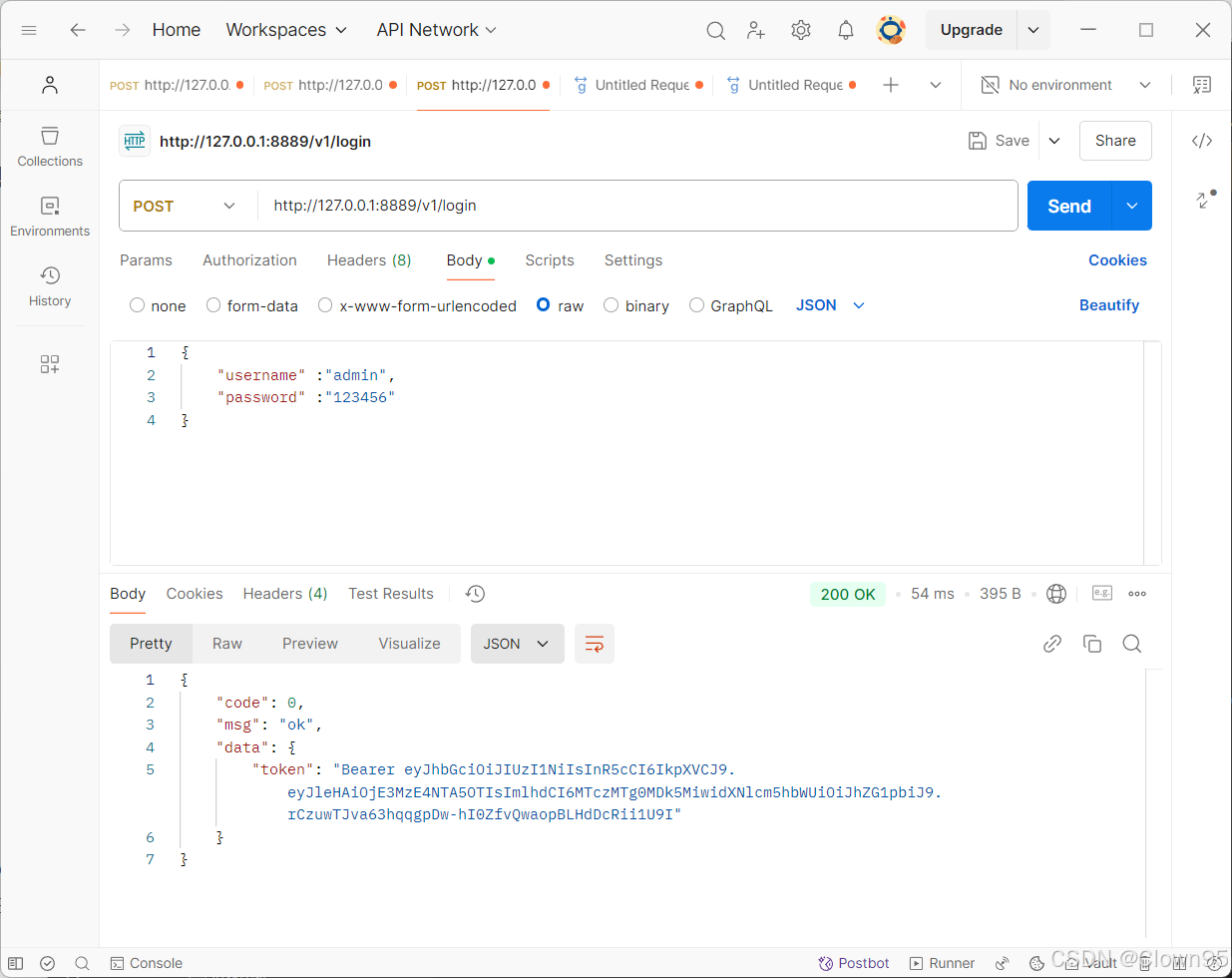
go-zero(七) RPC服务和ETCD
go-zero 实现 RPC 服务 在实际的开发中,我们是通过RPC来传递数据的,下面我将通过一个简单的示例,说明如何使用go-zero框架和 Protocol Buffers 定义 RPC 服务。 一、生成 RPC项目 在这个教程中,我们根据user.api文件࿰…...
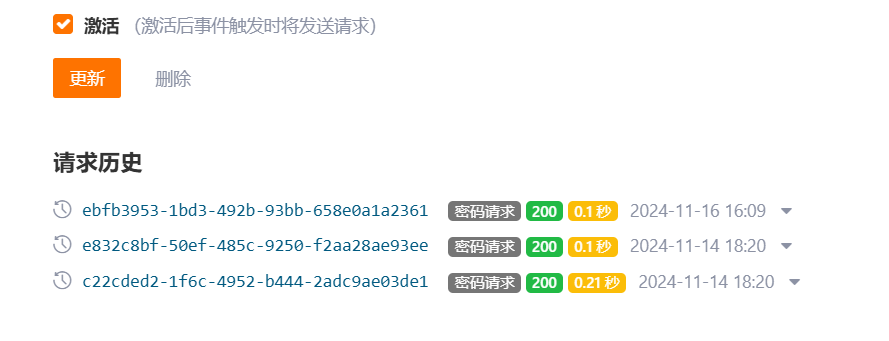
Jenkins + gitee 自动触发项目拉取部署(Webhook配置)
目录 前言 Generic Webhook Trigger 插件 下载插件 编辑 配置WebHook 生成tocken 总结 前言 前文简单介绍了Jenkins环境搭建,本文主要来介绍一下如何使用 WebHook 触发自动拉取构建项目; Generic Webhook Trigger 插件 实现代码推送后,触…...
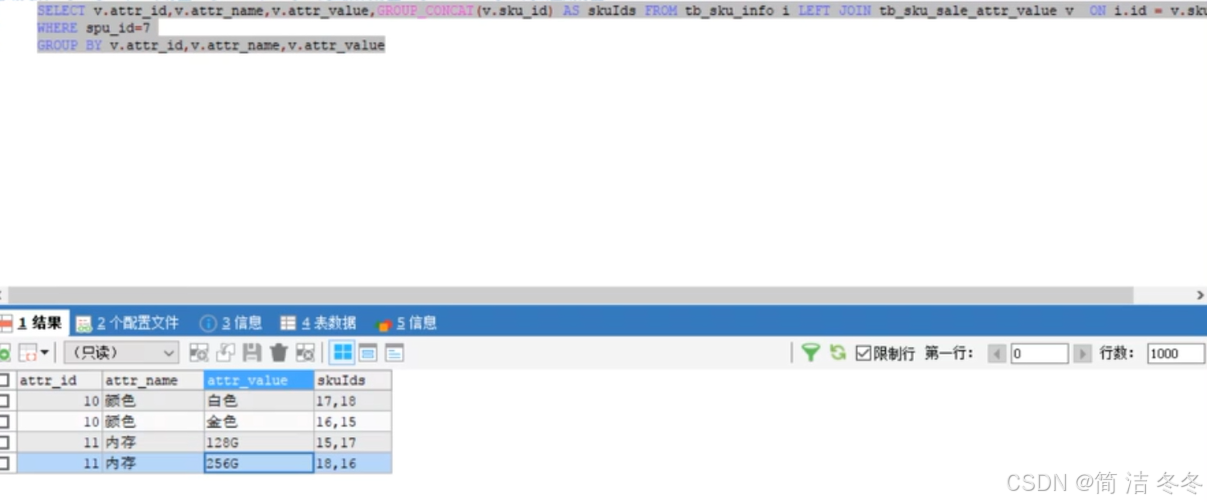
043 商品详情
文章目录 详情页数据表结构voSkuItemVo.javaSkuItemSaleAttrVo.javaAttrValueAndSkuIdVo.javaSpuAttrGroupVo.javaGroupAttrParamVo.java pom.xmlSkuSaleAttrValueDao.xmlSkuSaleAttrValueDao.javaAttrGroupDao.xmlAttrGroupServiceImpl.javaSkuInfoServiceImpl.javaSkuSaleAtt…...

【人工智能】Python与Scikit-learn的模型选择与调参:用GridSearchCV和RandomizedSearchCV提升模型性能
解锁Python编程的无限可能:《奇妙的Python》带你漫游代码世界 在机器学习建模过程中,模型的表现往往取决于参数的选择与优化。Scikit-learn提供了便捷的工具GridSearchCV和RandomizedSearchCV,帮助我们在参数空间中搜索最佳组合以提升模型表现。本文将从理论和实践两个角度…...
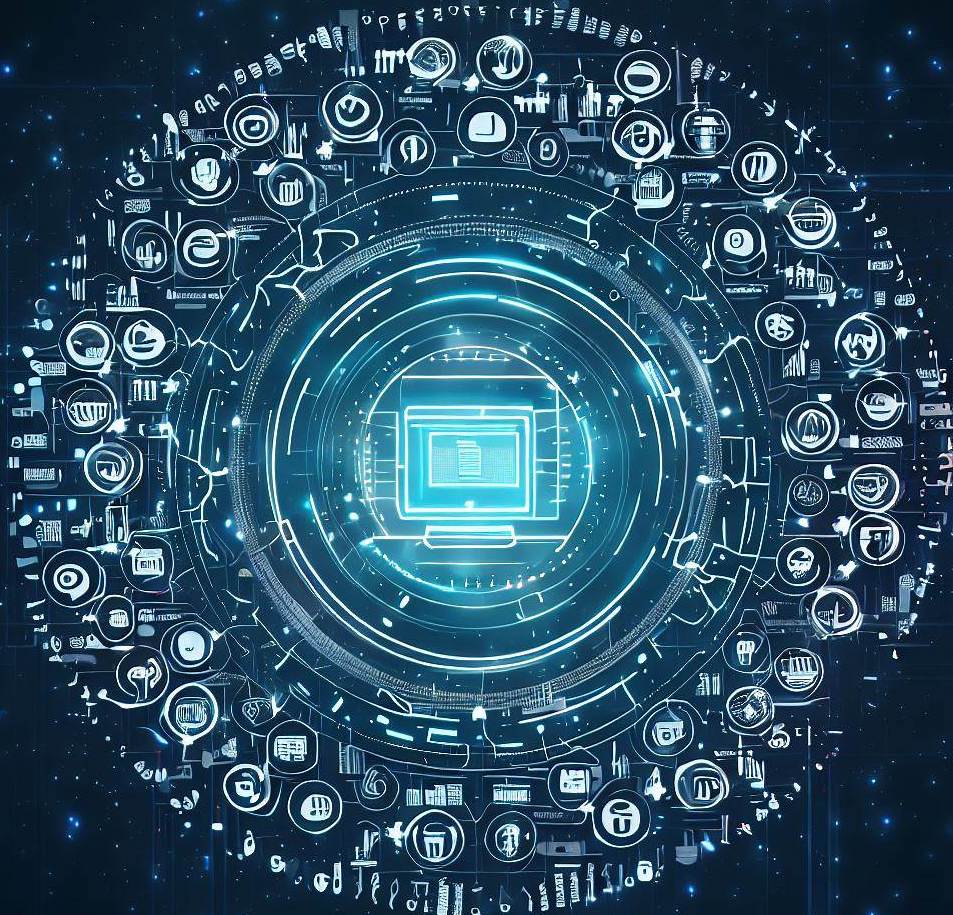
深入探讨 Puppeteer 如何使用 X 和 Y 坐标实现鼠标移动
背景介绍 现代爬虫技术中,模拟人类行为已成为绕过反爬虫系统的关键策略之一。无论是模拟用户点击、滚动,还是鼠标的轨迹移动,都可以为爬虫脚本带来更高的“伪装性”。在众多的自动化工具中,Puppeteer作为一个无头浏览器控制库&am…...
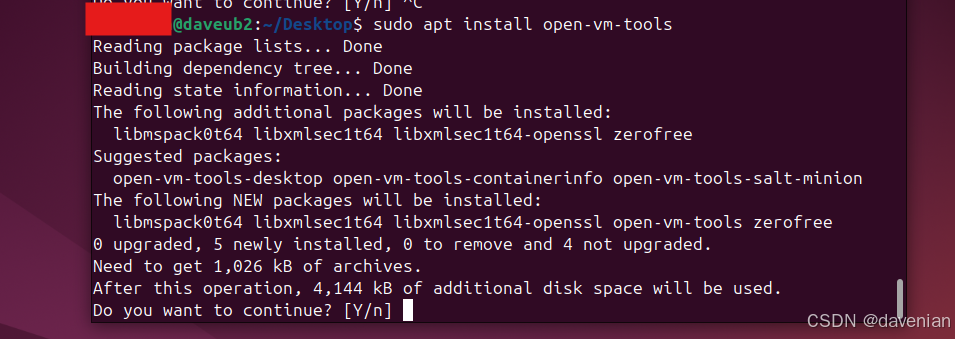
<OS 有关> ubuntu 24 不同版本介绍 安装 Vmware tools
原因 想用 apt-get download 存到本地 / NAS上,减少网络流浪。 看到 VMware 上的确实有 ubuntu,只是版本是16。 ubuntu 版本比较:LTS vs RR LTS: Long-Term Support 长周期支持, 一般每 2 年更新,会更可靠与更稳定…...

C#调用JAVA
参考教程:使用IKVMC转换Jar为dll动态库(含idea打包jar方法)-CSDN博客 已经实践过,好使。...

JavaEE-多线程基础知识
文章目录 前言与回顾创建一个多线程线程的创建以及运行机制简述step1: 继承Thread类step2: 实现Runable接口step3: 基于step1使用匿名内部类step4: 基于step2使用匿名内部类step5: 基于step4使用lambda表达式(推荐) Thread的常见方法关于jconsole监视线程的工具构造方法解析获取…...
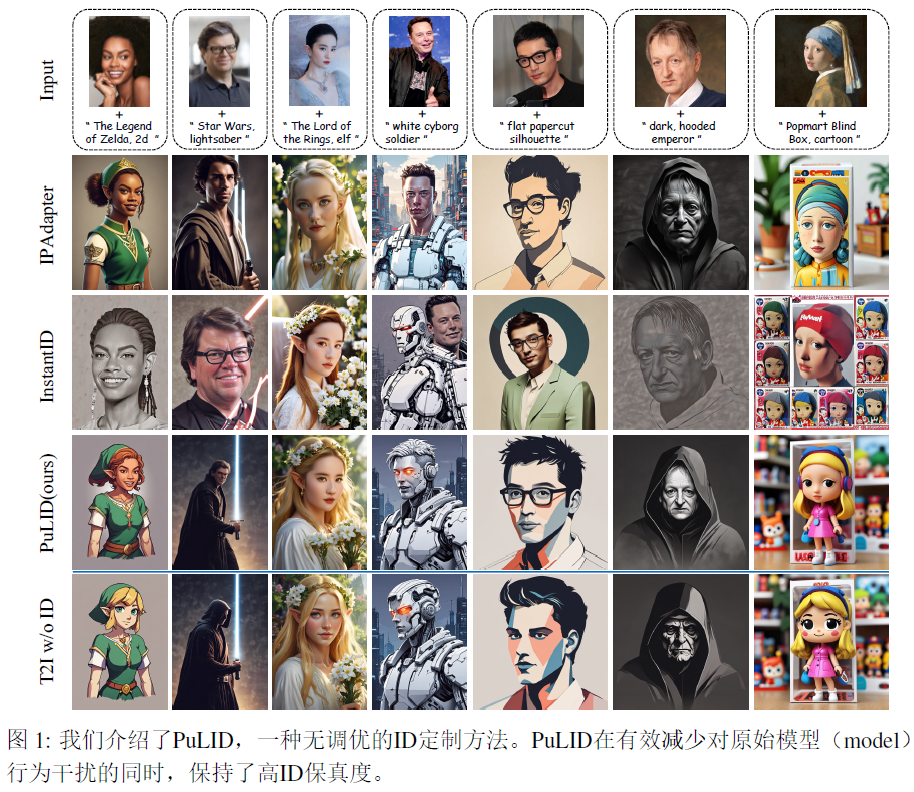
Pulid:pure and lightning id customization via contrastive alignment
1.introduction 基于微调的方案,对每个id进行定制需要花费数十分钟。另一项研究则放弃了对每个id进行微调,而是选择在一个庞大的肖像数据集上预训练一个id适配器。这些方法通常利用编码器例如clip来提取id特征,提取的特征随后以特定方式例如嵌入到cross attention集成到基础…...

什么是GraphQL,有什么特点
什么是GraphQL? GraphQL 是一种用于 API(应用程序编程接口)的查询语言,由 Facebook 在 2012 年开发,并于 2015 年开源。它提供了一种更高效、强大的方式来获取和操作数据,与传统的 RESTful API 相比&#…...
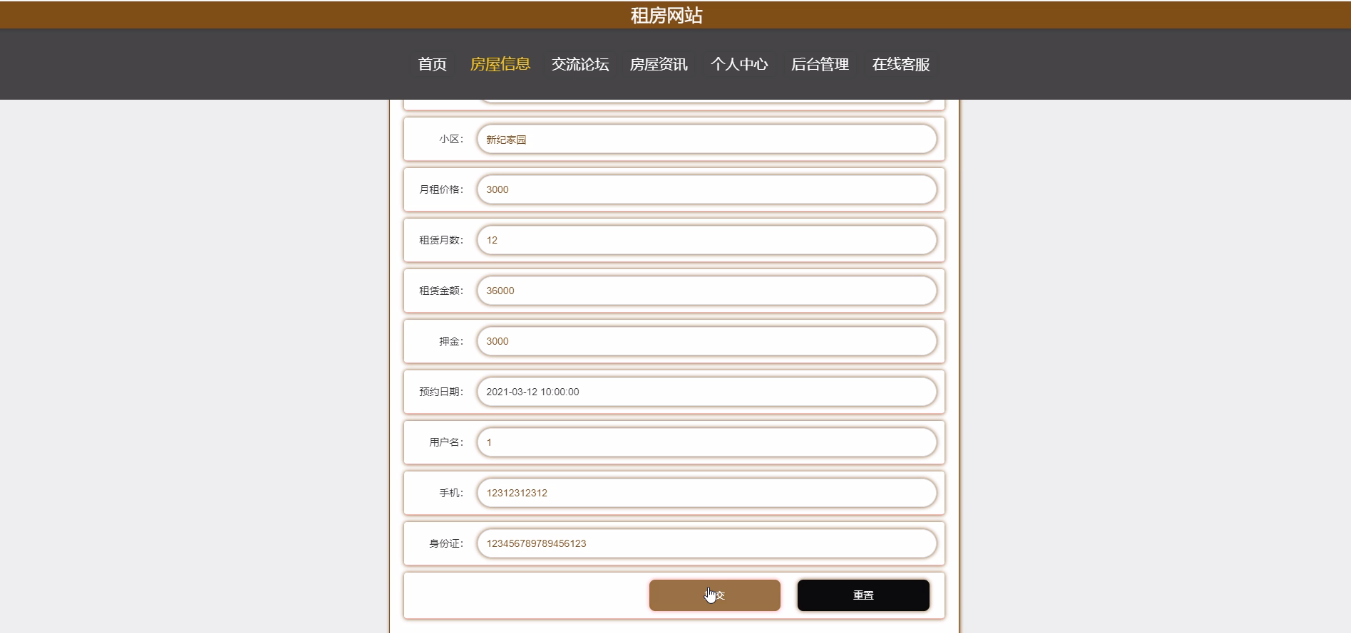
Java项目-基于SpringBoot+vue的租房网站设计与实现
博主介绍:✌程序员徐师兄、7年大厂程序员经历。全网粉丝12w、csdn博客专家、掘金/华为云/阿里云/InfoQ等平台优质作者、专注于Java技术领域和毕业项目实战✌ 🍅文末获取源码联系🍅 👇🏻 精彩专栏推荐订阅👇…...
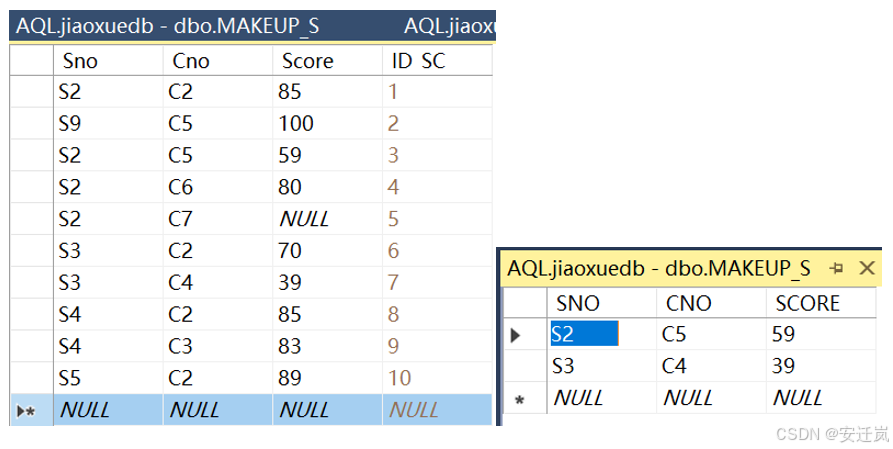
【SQL Server】华中农业大学空间数据库实验报告 实验三 数据操作
1.实验目的 熟悉了解掌握SQL Server软件的基本操作与使用方法,以及通过理论课学习与实验参考书的帮助,熟练掌握使用T-SQL语句和交互式方法对数据表进行插入数据、修改数据、删除数据等等的操作;作为后续实验的基础,根据实验要求重…...
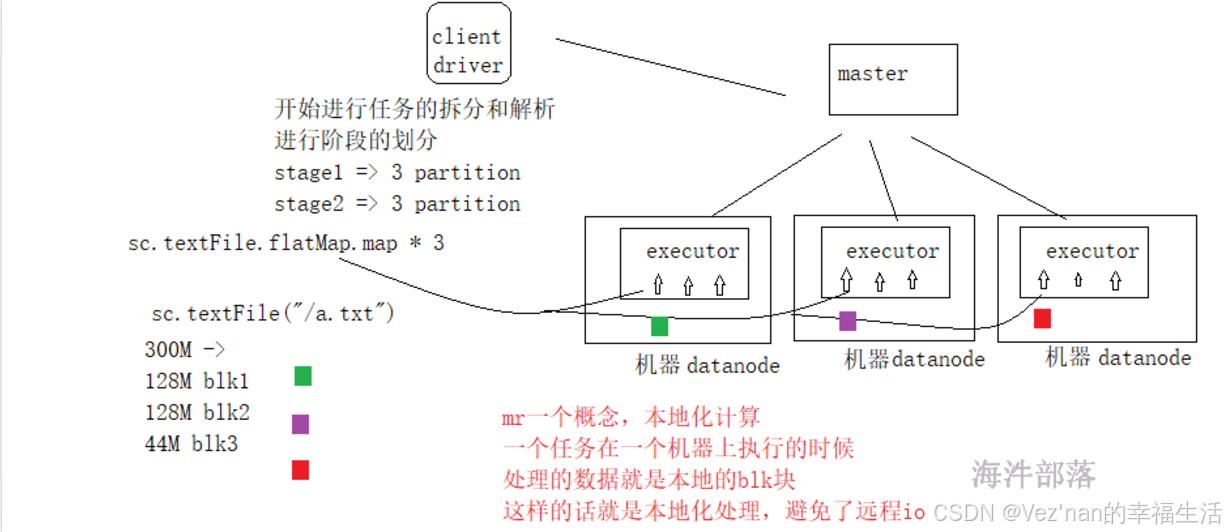
【大数据学习 | Spark】RDD的概念与Spark任务的执行流程
1. RDD的设计背景 在实际应用中,存在许多迭代式计算,这些应用场景的共同之处是,不同计算阶段之间会重用中间结果,即一个阶段的输出结果会作为下一个阶段的输入。但是,目前的MapReduce框架都是把中间结果写入到HDFS中&…...

ruoyi框架完成分库分表,按月自动建表功能
前提 这个分库分表功能,按月自动建表,做的比较久了,还没上线,是在ruoyi框架内做的,踩了不少坑,但是已经实现了,就分享一下代码吧 参考 先分享一些参考文章 【若依系列】集成ShardingSphere S…...
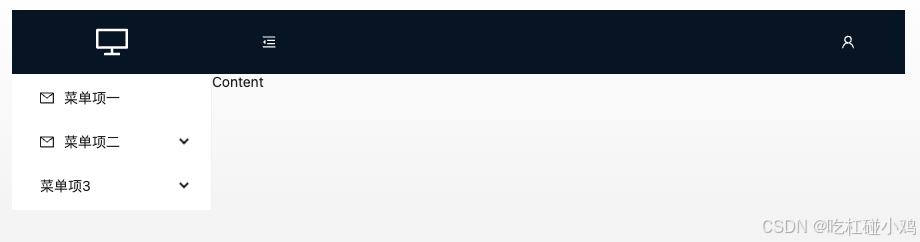
Antd中的布局组件
文章目录 一、Layout二、Menu三、Grid栅格 布局组件涉及项目框架的搭建,往往被忽略和低关注,毕竟不是经常用到,但是在调整项目结构的时候往往又需要重新设计布局,所以有必要提前归纳分析; 一、Layout Layout导出Sider,…...
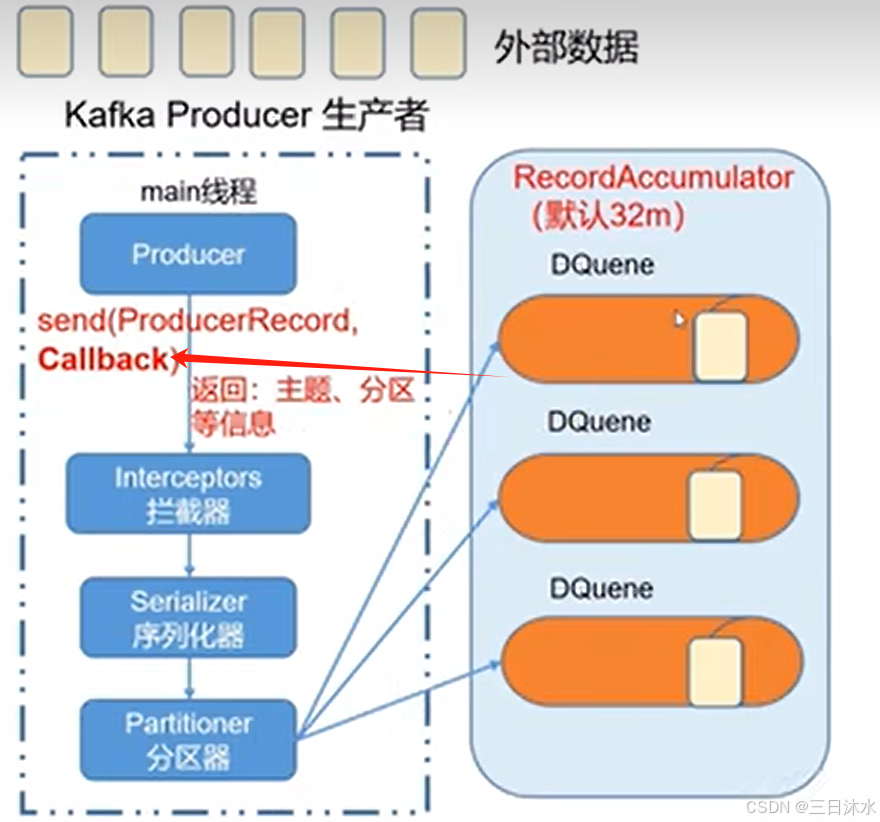
一文详解kafka知识点
目录 1、kafka定义 2、消息队列 2.1、产品选择 2.2、应用场景 2.3、消息队列的两种模式 3、kafka架构 4、kafka生产者 4.1、kafka生产者原理 4.2、kafka生产者异步发送 4.3、同步发送 4.4、分区 4.4.1、kafka分区好处 4.4.2、分区策略 4.4.3、自定义分区 4.5、生成吞…...
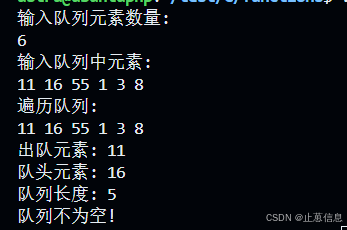
C语言基础学习:抽象数据类型(ADT)
基础概念 抽象数据类型(ADT)是一种数据类型,它定义了一组数据以及可以在这组数据上执行的操作,但隐藏了数据的具体存储方式和实现细节。在C语言中,抽象数据类型(ADT)是一种非常重要的概念&…...