Flutter第三弹:常用的Widget
目标:
1)常用的Widget有哪些?有什么特征?
2)开发一个简单的登录页面。
一、Flutter常用Widget
对于Flutter来说,一切皆Widget.
常用的Widget,包括一些基础功能的Widget.
控件名称 | 功能 | 备注 |
Text | 文本小组件 | |
Icon/IconButton | ||
Row | 水平布局组件 | |
Column | 竖直布局组件 | |
Stack | ||
Container | 容器小组件 | 类似层叠式布局 |
EdgeInsets | 边框小组件 | 用于设置padding |
Padding | 内边距 | 用于设置容器内边距 |
Scaffold | 脚手架小组件 | 规划页面主要布局位置,包括AppBar,底部导航栏按钮,页面Body等 |
MaterialApp | Material风格UI入口 | |
AppBar | Material风格AppBar |
Flutter Widget列表
https://flutter.cn/docs/reference/widgets
1.1 输入框 TextField
Flutter的输入框是常见的用户界面组件之一,用于接收用户的文本输入。对应的组件源码
text_field.dart
controller
:控制输入框的文本内容,可以通过TextEditingController
进行管理。decoration
:输入框的装饰,可以定义输入框的边框、背景、提示文本等样式。obscureText
:是否将输入内容隐藏,常用于密码输入框。enabled
:输入框是否可编辑。maxLength
:允许输入的最大字符数。textInputAction
:键盘操作按钮的类型,例如完成、继续等。keyboardType
:键盘的类型,如文本、数字、URL等。onChanged
:文本变化时的回调函数。onSubmitted
:用户提交输入时的回调函数。focusNode
:用于控制输入框的焦点获取和失去。autofocus
:是否自动获取焦点。style
:输入框文本的样式,如字体大小、颜色等。
1. controller
属性
controller
属性用于控制输入框的文本内容,可以通过TextEditingController
进行管理。使用TextEditingController
可以获取、设置和监听输入框的文本内容。
TextEditingController _textController = TextEditingController();TextField(controller: _textController,
);
通过_textController.text
来获取或设置输入框中的文本内容。
2. decoration属性
decoration
属性用于定义输入框的装饰,包括边框、背景、提示文本等样式。它使用InputDecoration
类来配置输入框的外观。
TextField(decoration: InputDecoration(border: OutlineInputBorder(),filled: true,fillColor: Colors.grey[200],hintText: 'Enter your name',hintStyle: TextStyle(color: Colors.grey),prefixIcon: Icon(Icons.person),),
);
3. obscureText属性
obscureText
属性用于指定输入框的文本是否应该被隐藏,常用于密码输入框。当设置为true
时,输入的文本将以圆点或其他隐藏字符显示。掩码显示。
TextField(obscureText: true,
);
4. enabled属性-是否可编辑
enabled
属性用于指定输入框是否可编辑。当设置为false
时,输入框将变为只读状态,用户无法编辑其中的文本。
5. maxLength属性
maxLength
属性用于限制输入框可输入的最大字符数。设置此属性后,用户将无法输入超过指定字符数的文本。
TextField(maxLength: 10,
);
6. textInputAction属性
textInputAction
属性用于指定键盘操作按钮的类型,例如完成、继续等。它们用于控制键盘上右下角按钮的标签和功能。
TextField(textInputAction: TextInputAction.done,
);
7. keyboardType属性
keyboardType
属性用于指定键盘的类型,例如文本、数字、URL等。根据需要选择适合的键盘类型,以提供更好的用户体验。
TextField(keyboardType: TextInputType.emailAddress,
);
8. onChanged
和onSubmitted属性
onChanged
和onSubmitted
属性用于定义输入框文本变化和提交输入的回调函数。onChanged
在输入框文本发生变化时被调用,而onSubmitted
在用户提交输入时被调用。
TextField(onChanged: (value) {// 处理文本变化},onSubmitted: (value) {// 处理提交输入},
);
9. style属性
style
属性用于定义输入框文本的样式,如字体大小、颜色等。
TextField(style: TextStyle(fontSize: 16.0,color: Colors.black,),
);
1.2 文本框 Text
文本框是Flutter用于显示文本的小组件。
创建函数如下:
const Text(String this.data, {Key? key,this.style,this.strutStyle,this.textAlign,this.textDirection,this.locale,this.softWrap,this.overflow,this.textScaleFactor,this.maxLines,this.semanticsLabel,this.textWidthBasis,this.textHeightBehavior,}) : assert(data != null,'A non-null String must be provided to a Text widget.',),textSpan = null,super(key: key);
创建函数存在一个非空必选参数String data,存储文本内容。其他是一些可选参数。
1. text属性
存储文本
2. key属性 用于识别组件的唯一键。
3. style属性
TextStyle对象,用于指定文本的样式,例如字体、颜色、大小等。常用的属性配置:
- color: 文本颜色。
- fontSize: 字体大小。
- fontWeight: 字体粗细。
- fontStyle: 字体样式,如斜体。
- letterSpacing: 字母间距。
- wordSpacing: 单词间距。
- background: 文本背景颜色。
- decoration: 文本修饰,如下划线、删除线等。
- decorationColor: 文本修饰的颜色。
- decorationStyle: 文本修饰的样式。
- height: 行高。
- textBaseline: 基线对齐方式。
- shadows: 文本阴影效果。
4. textAlign属性
文本对齐方式,可以是左对齐(left)、右对齐(right)、居中对齐(center)或两端对齐(justify)。
5. textDirection属性
文本方向,可以是从左到右(ltr)或从右到左(rtl)。
6. softWrap属性
softWrap是否自动换行,默认为true。
7. overflow属性
文本溢出处理方式,可以是省略号(ellipsis)、截断(clip)或折叠(fade)
8. maxLines属性
最大显示行数,超过部分将根据overflow属性进行处理。
9. textScaleFactor属性
文本缩放因子,用于调整文本大小的比例。
10. locale属性
文本的本地化配置,用于支持多语言显示。
11. strutStyle属性
文本行高样式。
12. textWidthBasis属性
文本宽度计算基准,可以是parent(父容器宽度)或 longestLine(最长行宽度)。
13. textHeightBehavior属性
文本高度行为配置。
1.3 按钮 Button
常用的按钮分类
- ElevatedButton: 凸起按钮,具有立体效果。
- TextButton: 文本按钮,通常用于文字链接或简单的按钮。
- OutlinedButton: 带边框的按钮,边框颜色可自定义。
- IconButton: 图标按钮,使用图标作为按钮的内容。
1. onPressed属性
点击按钮时触发的回调函数
2. onLongPress属性
长按按钮时触发的回调函数
3. style属性
ButtonStyle对象,用于设置按钮的样式。常用属性如下:
- backgroundColor:按钮的背景颜色。【常用】
- foregroundColor:按钮的前景颜色,如文字颜色。【常用】
- elevation:按钮的海拔高度,用于创建立体效果。
- padding:按钮的内边距。【常用】
- side:按钮的边框样式。
- shape:按钮的形状。【常用】
- minimumSize:按钮的最小尺寸。
- alignment:按钮内容的对齐方式。
ElevatedButton(onPressed: () {// 点击了按钮print("ElevatedButton pressed");},style: ButtonStyle(backgroundColor: MaterialStateProperty.all(Colors.blue),foregroundColor: MaterialStateProperty.all(Colors.white),padding: MaterialStateProperty.all(EdgeInsets.all(12.0)),),child: Text("登录",style: TextStyle(fontSize: 26.0,color: Colors.black,)),),
4. child属性
按钮的子组件,通常是一个Text
或Icon
等用于显示按钮内容的组件
5. onHighlightChanged属性
当按钮被按下或释放时触发的回调函数
6. focusNode属性
控制按钮的焦点状态
7. autofocus属性
是否自动获取焦点
8. clipBehavior属性
内容溢出时的裁剪行为
9. enableFeedback属性
是否为按钮点击提供音频和触觉反馈
二、开发简单的登录页面
登录页比较简单,用户名+密码+登录按钮。采用Material风格脚手架。
import 'package:flutter/material.dart';void main() {runApp(const MyApp());
}class MyApp extends StatelessWidget {const MyApp({Key? key}) : super(key: key);// This widget is the root of your application.@overrideWidget build(BuildContext context) {return MaterialApp(title: 'Flutter Demo', // 标题theme: ThemeData(// This is the theme of your application.//// Try running your application with "flutter run". You'll see the// application has a blue toolbar. Then, without quitting the app, try// changing the primarySwatch below to Colors.green and then invoke// "hot reload" (press "r" in the console where you ran "flutter run",// or simply save your changes to "hot reload" in a Flutter IDE).// Notice that the counter didn't reset back to zero; the application// is not restarted.primarySwatch: Colors.blue,),home: const MyHomePage(title: 'Flutter Demo Home Page'),);}
}class MyHomePage extends StatefulWidget {const MyHomePage({Key? key, required this.title}) : super(key: key);// This widget is the home page of your application. It is stateful, meaning// that it has a State object (defined below) that contains fields that affect// how it looks.// This class is the configuration for the state. It holds the values (in this// case the title) provided by the parent (in this case the App widget) and// used by the build method of the State. Fields in a Widget subclass are// always marked "final".final String title;@overrideState<MyHomePage> createState() => _MyHomePageState();
}class _MyHomePageState extends State<MyHomePage> with TickerProviderStateMixin {int _counter = 0;// 文本内容String textToShow = 'I love Flutter!';// 创建焦点节点对象FocusNode _nameFocusNode = FocusNode();FocusNode _passwordFocusNode = FocusNode();// 记录焦点状态bool _nameHasFocus = false;bool _passwordHasFocus = false;/*** 动画控制器*/late AnimationController controller;/*** 角度动画*/late CurvedAnimation curve;void _incrementCounter() {setState(() {// This call to setState tells the Flutter framework that something has// changed in this State, which causes it to rerun the build method below// so that the display can reflect the updated values. If we changed// _counter without calling setState(), then the build method would not be// called again, and so nothing would appear to happen._counter++;// 更新文本内容if (_counter % 4 == 0) {textToShow = 'I Study Flutter now';} else if (_counter % 4 == 1) {textToShow = 'Flutter is so interesting';} else if (_counter % 4 == 2) {textToShow = 'I love it more';} else {textToShow = 'I learn it more funny';}});// 播放动画controller.forward();}/*** 监听输入框焦点变化,焦点变化时保存焦点状态*/void _nameFocusChange() {// 存储状态变化setState(() {_nameHasFocus = _nameFocusNode.hasFocus;});}/*** 监听输入框焦点变化,焦点变化时保存焦点状态*/void _passwdFocusChange() {// 存储状态变化setState(() {_passwordHasFocus = _passwordFocusNode.hasFocus;});}@overridevoid initState() {super.initState();controller = AnimationController(duration: const Duration(milliseconds: 2000),vsync: this,);curve = CurvedAnimation(parent: controller,curve: Curves.easeIn,);// 监听输入框焦点变化_nameFocusNode.addListener(_nameFocusChange);_passwordFocusNode.addListener(_passwdFocusChange);}@overridevoid dispose() {_nameFocusNode.removeListener(_nameFocusChange);_passwordFocusNode.removeListener(_passwdFocusChange);super.dispose();}@overrideWidget build(BuildContext context) {// This method is rerun every time setState is called, for instance as done// by the _incrementCounter method above.//// The Flutter framework has been optimized to make rerunning build methods// fast, so that you can just rebuild anything that needs updating rather// than having to individually change instances of widgets.return Scaffold(appBar: AppBar(// Here we take the value from the MyHomePage object that was created by// the App.build method, and use it to set our appbar title.title: Text(widget.title),),body: Center(child: Padding(padding: EdgeInsets.all(12.0),child: Column(// Column is also a layout widget. It takes a list of children and// arranges them vertically. By default, it sizes itself to fit its// children horizontally, and tries to be as tall as its parent.//// Invoke "debug painting" (press "p" in the console, choose the// "Toggle Debug Paint" action from the Flutter Inspector in Android// Studio, or the "Toggle Debug Paint" command in Visual Studio Code)// to see the wireframe for each widget.//// Column has various properties to control how it sizes itself and// how it positions its children. Here we use mainAxisAlignment to// center the children vertically; the main axis here is the vertical// axis because Columns are vertical (the cross axis would be// horizontal).mainAxisAlignment: MainAxisAlignment.center,children: <Widget>[const Text('用户登录',style: TextStyle(fontSize: 25.0,color: Colors.black,fontWeight: FontWeight.w200),),SizedBox(height: 12.0),TextField(focusNode: _nameFocusNode,decoration: InputDecoration(filled: true,fillColor:_nameHasFocus ? Colors.lightBlue[100] : Colors.grey[200],border: OutlineInputBorder(),hintText: '请输入姓名',hintStyle: TextStyle(color: Colors.grey),prefixIcon: Icon(Icons.person),),),SizedBox(height: 12.0),TextField(focusNode: _passwordFocusNode,obscureText: true, // 定输入框的文本是否应该被隐藏,常用于密码输入框decoration: InputDecoration(filled: true,fillColor:_nameHasFocus ? Colors.lightBlue[100] : Colors.grey[200],border: OutlineInputBorder(),hintText: '请输入密码',hintStyle: TextStyle(color: Colors.grey),prefixIcon: Icon(Icons.lock),),),TextField(enabled: true,style: TextStyle(fontSize: 20.0, color: Colors.black,backgroundColor: Colors.cyan, // 文本输入颜色),),Text('You have pushed the button this many times:',),Text('$_counter',style: Theme.of(context).textTheme.headline4,),// 新增一个自定义的Text(在State中存储)Text(textToShow),Center(child: FadeTransition(opacity: curve,child: const FlutterLogo(size: 100,),),),],),),),floatingActionButton: FloatingActionButton(onPressed: _incrementCounter,tooltip: 'Increment',child: const Icon(Icons.add),), // This trailing comma makes auto-formatting nicer for build methods.);}
}
参考文献:
Flutter速来系列6: Flutter同学 输入框TextField就算了,还整个表单? - 掘金
相关文章:

Flutter第三弹:常用的Widget
目标: 1)常用的Widget有哪些?有什么特征? 2)开发一个简单的登录页面。 一、Flutter常用Widget 对于Flutter来说,一切皆Widget. 常用的Widget,包括一些基础功能的Widget. 控件名称功能备注…...
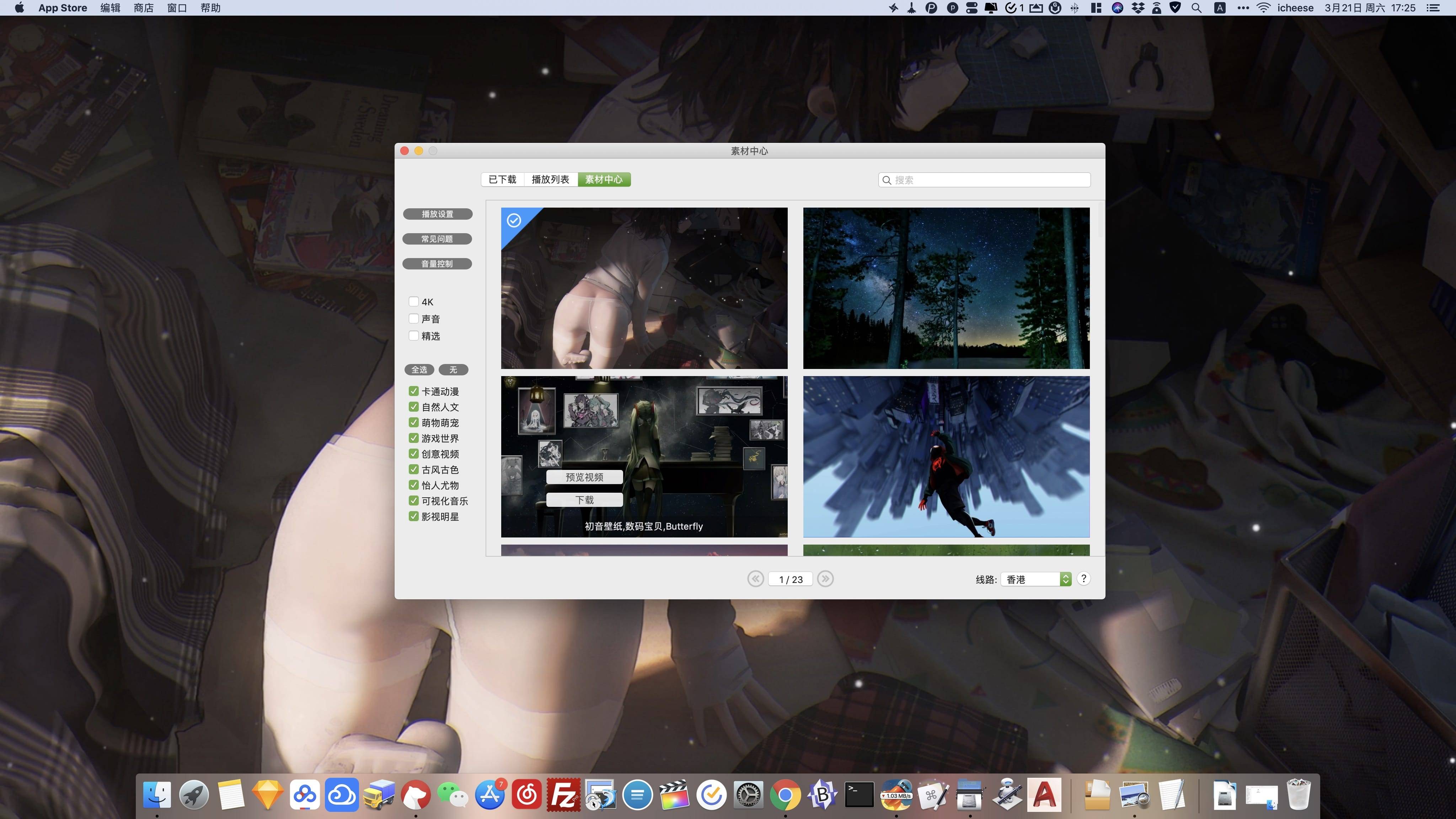
Dynamic Wallpaper v17.4 mac版 动态视频壁纸 兼容 M1/M2
Dynamic Wallpaper Engine 是一款适用于 Mac 电脑的视频动态壁纸, 告别单调的静态壁纸,拥抱活泼的动态壁纸。内置在线视频素材库,一键下载应用,也可导入本地视频,同时可以将视频设置为您的电脑屏保。 应用介绍 Dynam…...

Windows / Mac应用程序在Linux系统中的兼容性问题 解决方案
Linux系统可以通过多种方式提高与Windows或Mac应用程序的兼容性。这里有一些解决方案 Windows应用程序兼容性解决方案: Wine Wine是一个允许Linux和Unix系统上运行Windows应用程序的兼容层。 它不是模拟器,而是实现了Windows API的开源实现。 许多W…...
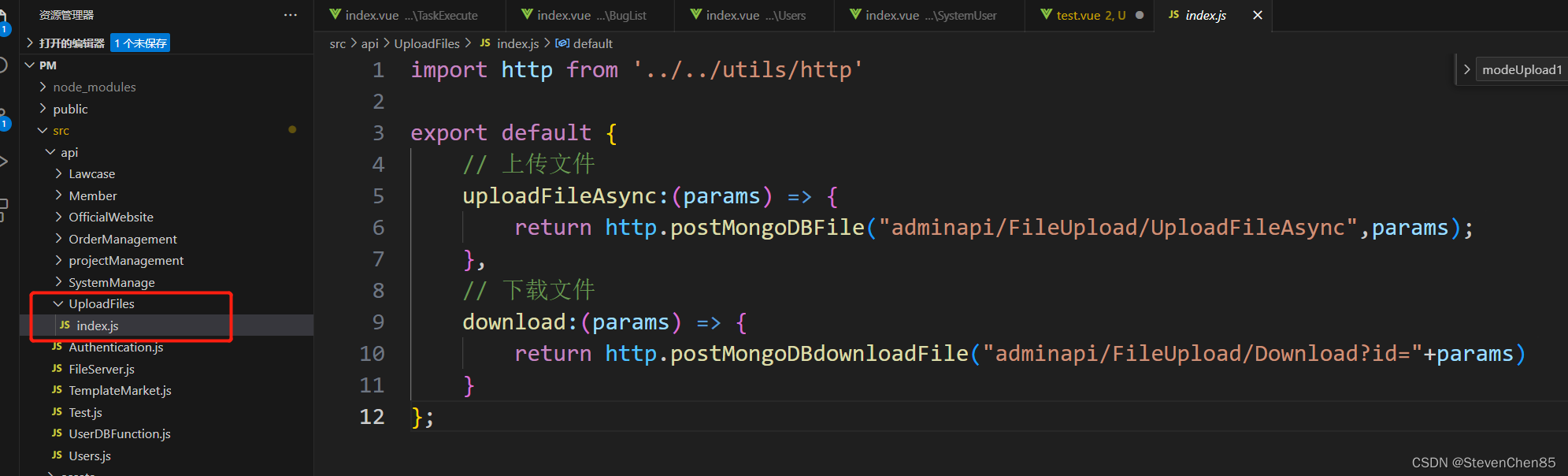
Net Core 使用Mongodb操作文件(上传,下载)
Net Core 使用Mongodb操作文件(上传,下载) 1.Mongodb GridFS 文件操作帮助类。 GridFS 介绍 https://baike.baidu.com/item/GridFS/6342715?fraladdin DLL源码:https://gitee.com/chenjianhua1985/mongodb-client-encapsulati…...
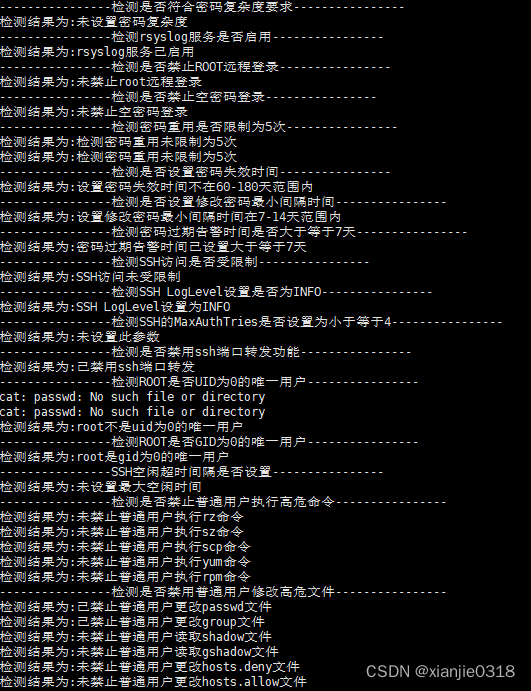
适用于系统版本:CentOS 6/7/8的基线安全检测脚本
#!/bin/bash #适用于系统版本:CentOS 6/7/8 echo "----------------检测是否符合密码复杂度要求----------------" #把minlen(密码最小长度)设置为8-32位,把minclass(至少包含小写字母、大写字母、数字、特殊…...
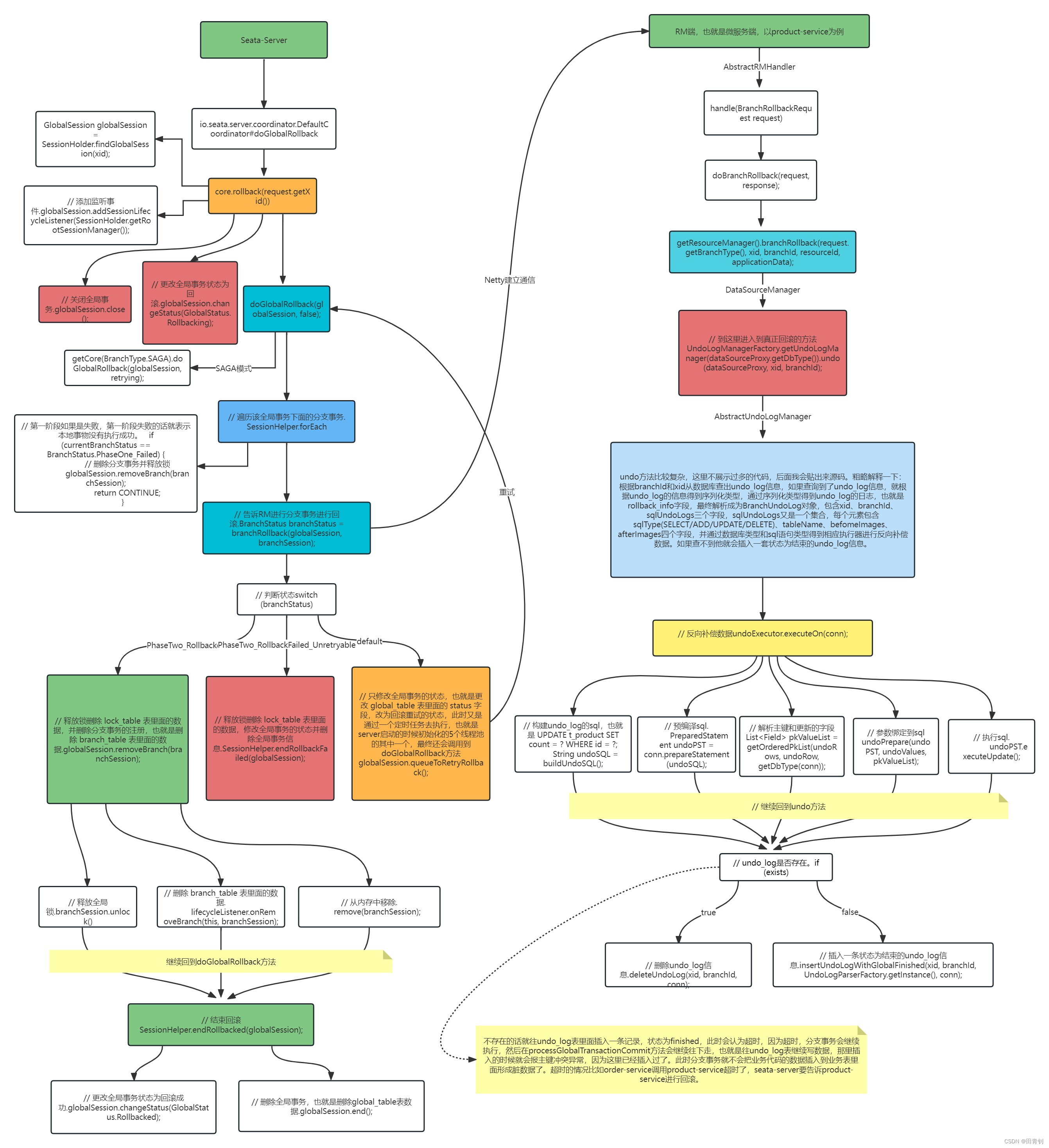
Seata源码流程图
1.第一阶段分支事务的注册 流程图地址:https://www.processon.com/view/link/6108de4be401fd6714ba761d 2.第一阶段开启全局事务 流程图地址:https://www.processon.com/view/link/6108de13e0b34d3e35b8e4ef 3.第二阶段全局事务的提交 流程图地址…...
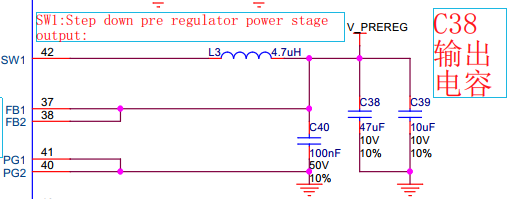
英飞凌电源管理PMIC的安全应用
摘要 本篇文档主要用来介绍英飞凌电源管理芯片TLF35584的使用,基于电动助力转向应用来介绍。包含一些安全机制的执行。 TLF35584介绍 TLF35584是英飞凌推出的针对车辆安全应用的电源管理芯片,符合ASIL D安全等级要求,具有高效多电源输出通道&…...

快速在Linux系统安装MySQL
虚拟机使用docker安装MySQL 使用docker拉去镜像 查看mysql的镜像 docker search mysql拉去mysql镜像 docker pull mysql查看下载的镜像 docker images启动容器 docker start mysql进入MySQL容器 docker exec -it mysql /bin/bash登录mysql mysql -u root -p检查是否进入…...

数据库相关理论知识(有目录便于直接锁定相关知识点+期末复习)
一,数据模型,关系型数据模型,网状模型,层次模型 1.数据库模型是用来描述和表示现实世界中的事物、概念以及它们之间的关系的工具,但是并不是越专业越好,还要平衡它的模型的复杂性、通用性和成本效益等因素…...

NCC环境配置
一、后端配置 1.安装eclipse汉化插件 2.安装svn插件...

用python实现Dubins曲线生成
Dubins曲线是连接两个具有指定方向和位置的点的最短路径,其中路径受到固定曲率约束(如车辆的转向限制)。Dubins曲线常用于机器人路径规划、车辆轨迹规划等领域。 Dubins曲线可以分为三种类型:CCC (Curve-Curve-Curve), CCL (Curv…...

智能技术上的“是”并不代表具体领域的“应该”
技术上的“是”并不代表具体领域的“应该” 。技术上的“是”仅仅是指某种方法或技术在实践中是否可行或有效,而不涉及是否该采取这种方法或技术。决定是否采取某种方法或技术还需要考虑伦理、法律、可行性等其他方面的因素。技术的发展可能会有各种可能性ÿ…...
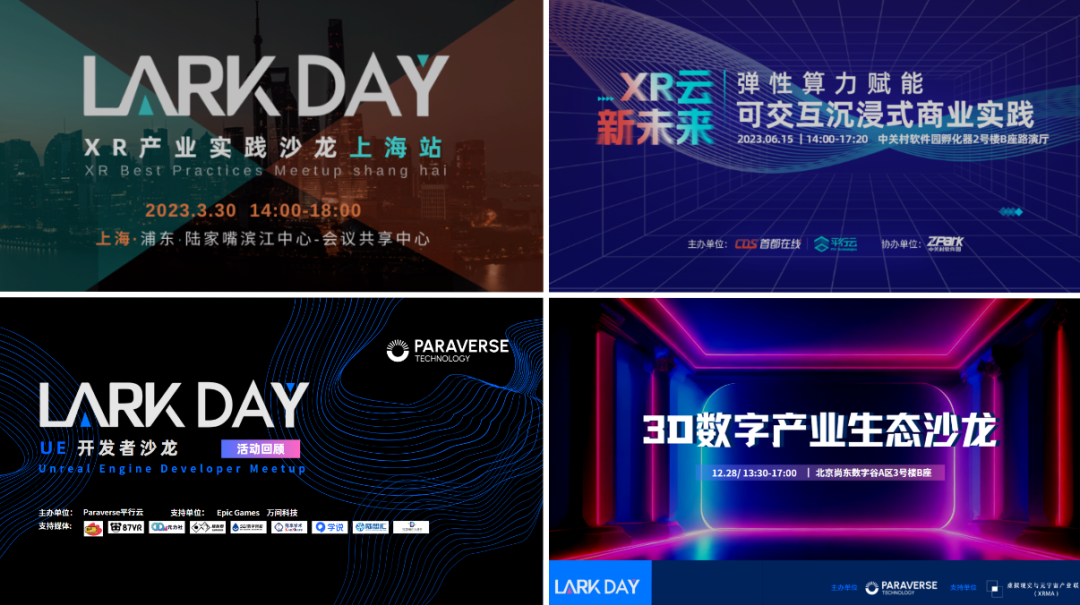
永热爱 敢向前 | Paraverse平行云的2023 年终总结
永热爱,敢向前 值此新年,回顾2023,仅以此句,献给所有XR产业信仰者 2023 年,是XR产业技术和场景承上启下的关键之年 在这场波澜壮阔的技术潮中 「Paraverse平行云」踏浪前行 已是第八个年头,让我们一起…...

c/c++的内存分配,详细说一下栈、堆和静态存储区
栈区(Stack):由编译器自动分配和回收,栈中存放函数调用的相关信息,栈帧(记录函数的栈帧开始的位置),参数,局部变量,返回地址。其操作方法类似于数据结构中的栈…...

每日构造题训练——C. Divan and bitwise operations
每日构造题训练 题目链接: 题目传送门 前置知识: 按位或运算 一、题意: 1 1 1、 有一个长度为 n n n的但是元素未知的数组 a a a, 给定 m m m个约束,每个约束都有 l , r , x l, r, x l,r,x, 并且满足 1 ≤ l ≤ r ≤ n , 1 ≤ x < 2 30 , a [ l ] ∣ a [ l 1 …...
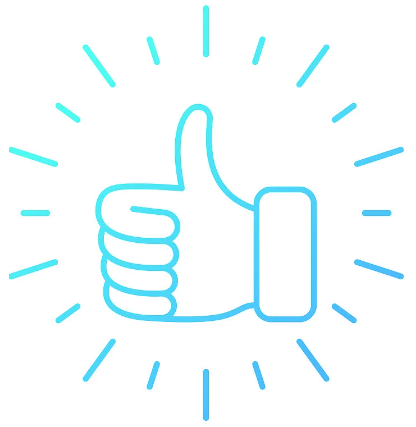
【C++练级之路】【Lv.13】多态(你真的了解虚函数和虚函数表吗?)
快乐的流畅:个人主页 个人专栏:《C语言》《数据结构世界》《进击的C》 远方有一堆篝火,在为久候之人燃烧! 文章目录 一、虚函数与重写1.1 虚函数1.2 虚函数的重写1.3 重写的特例1.4 final和override(C11)1.…...
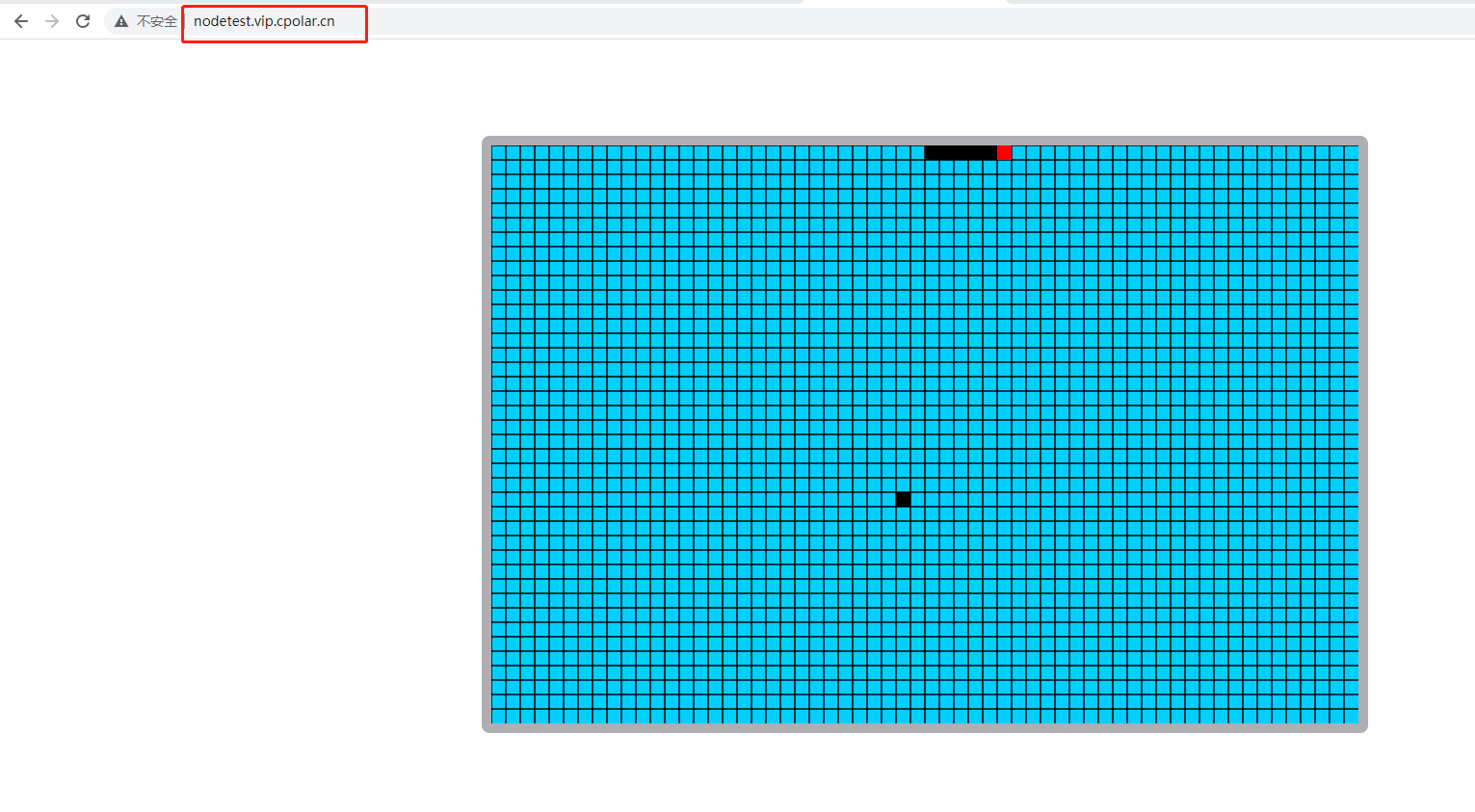
如何在Windows系统安装Node.js环境并制作html页面发布公网远程访问?
文章目录 前言1.安装Node.js环境2.创建node.js服务3. 访问node.js 服务4.内网穿透4.1 安装配置cpolar内网穿透4.2 创建隧道映射本地端口 5.固定公网地址 前言 Node.js 是能够在服务器端运行 JavaScript 的开放源代码、跨平台运行环境。Node.js 由 OpenJS Foundation࿰…...
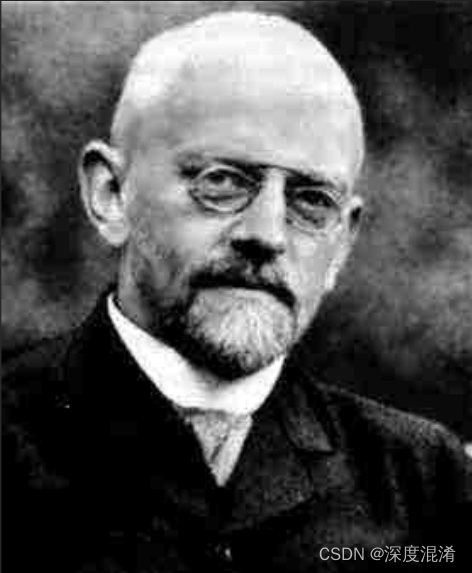
C#,数值计算,希尔伯特矩阵(Hilbert Matrix)的算法与源代码
Hilbert, David (1862-1943) 1 希尔伯特(Hilbert) 德国数学家,在《几何学基础》中提出了第一套严格的几何公理(1899年)。他还证明了自己的系统是自洽的。他发明了一条简单的空间填充曲线,即埃里克魏斯汀的数学世界,即希尔伯特曲线,埃里克魏斯汀的数学世界,并证明了不…...
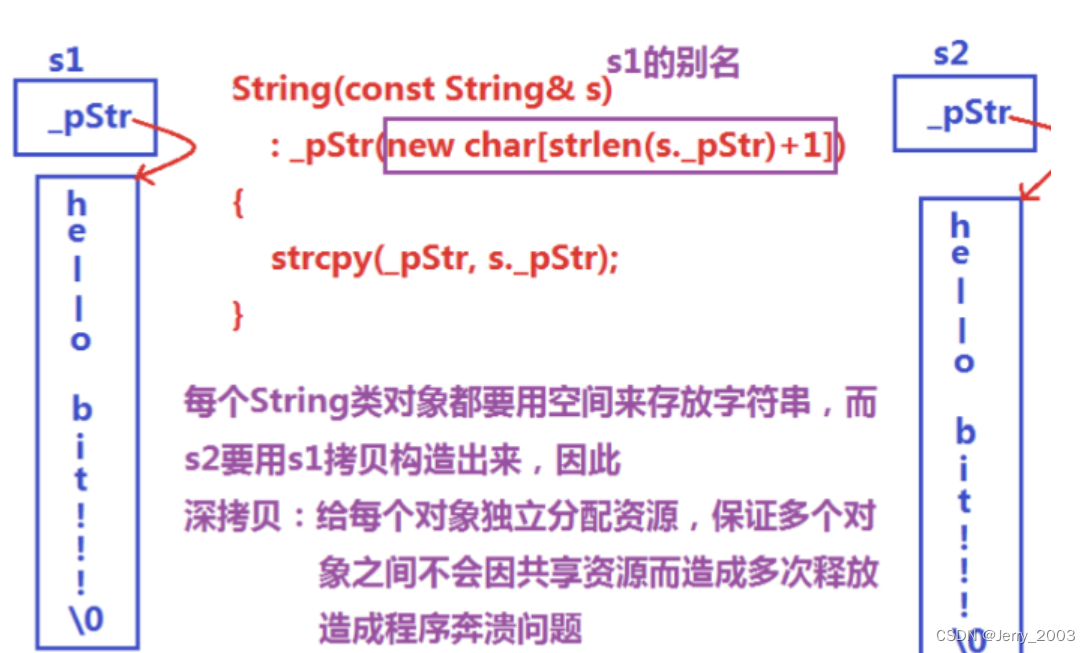
【C++教程从0到1入门编程】第八篇:STL中string类的模拟实现
一、 string类的模拟实现 下面是一个列子 #include <iostream> namespace y {class string{public: //string() //无参构造函数// :_str(nullptr)//{}//string(char* str) //有参构造函数// :_str(str)//{}string():_str(new char[1]){_str[0] \0;}string(c…...
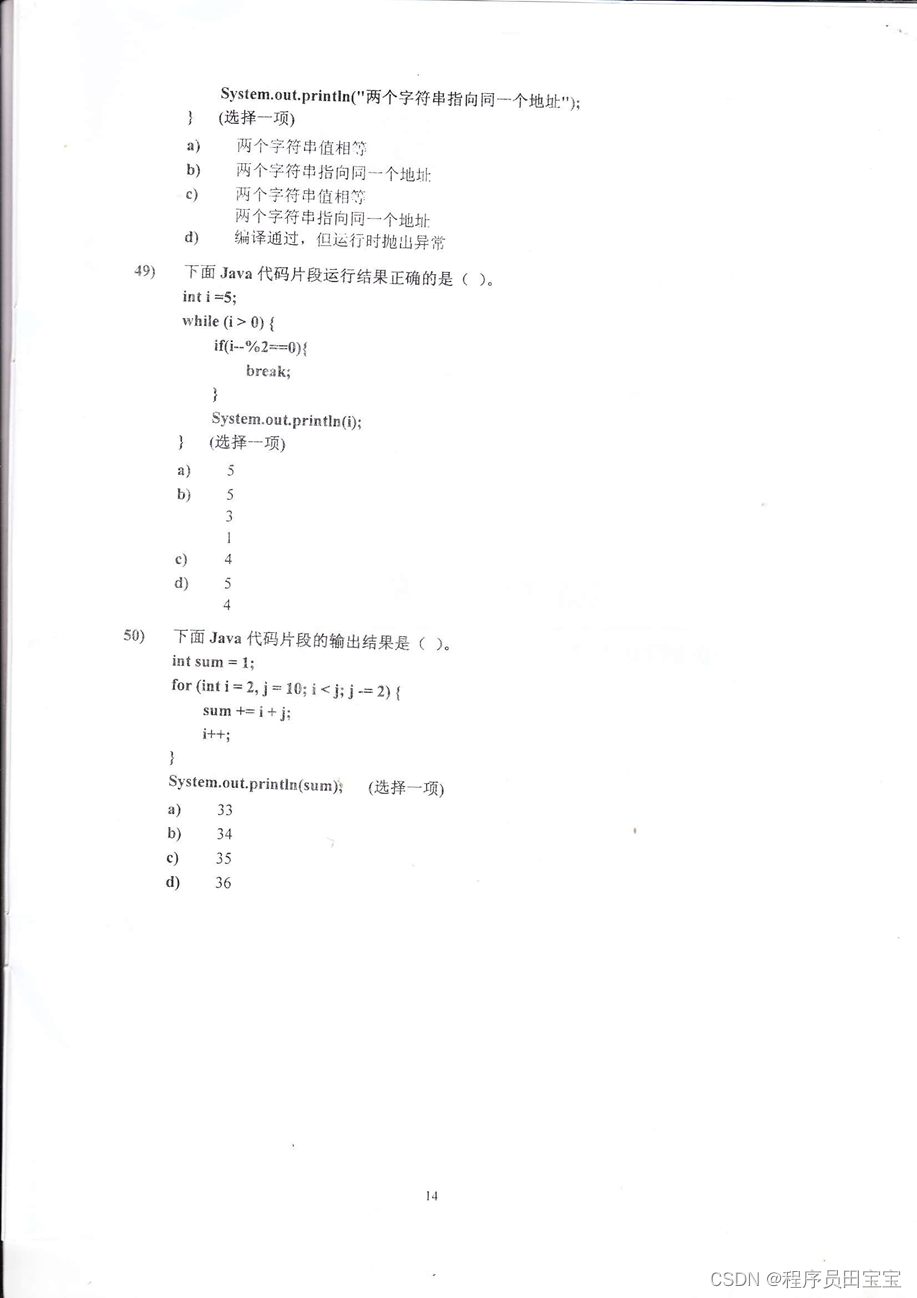
学生时期学习资源同步-1 第一学期结业考试题6
原创作者:田超凡(程序员田宝宝) 版权所有,引用请注明原作者,严禁复制转载...

迁移学习怎么用
如果想实现一个计算机视觉应用,而不想从零开始训练权重,比方从随机初始化开始训练,更快的方式是下载已经训练好权重的网络结构,把这个作为预训练,迁移到你感兴趣的新任务上。ImageNet、PASCAL等等数据库已经公开在线。…...
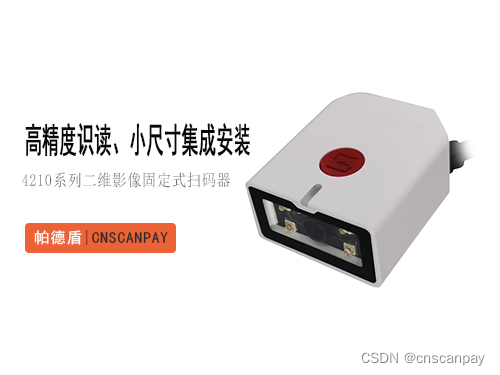
医疗手持智能终端读取条码二维码的难点有哪些?
在医疗科技行业信息化的大潮中,医疗手持式智能终端的应用越发普及,医疗手持式智能终端对条码二维码技术应用显得尤为关键,作为信息朔源载体的条码二维码读取方面,在实际应用中却面临着诸多问题,我们该如何应对…...
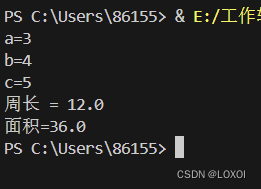
Python小设计
1. 五个PPT上的界面打印【print、input函数】 (1)英雄商城登陆界面 print(英雄联盟商城登录界面 ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~1. 用户登录2. 新用户注册3. 退出系统 ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~…...

今日讲讲父子传值~
今天来讲讲父子传值中的几种方法~ 项目中往往会把一些常用的公共代码抽离出来,写成一个子组件。或者在一个页面中的代码太多,可以根据功能的不同抽离出相关代码写成子组件,这样代码结构会更加简洁明了,后续维护更加方便。…...
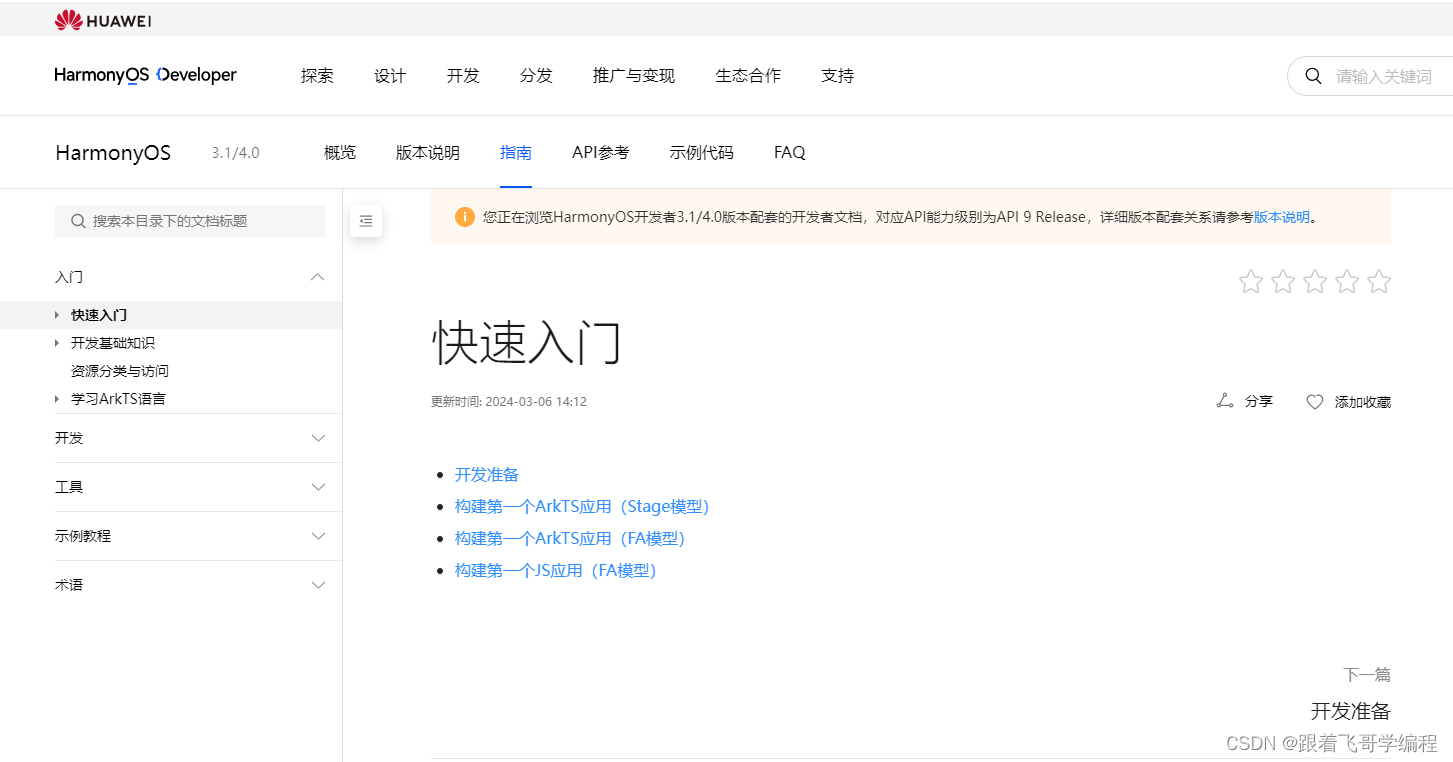
三、HarmonyOS 应用开发入门之运行Hello World
目录 1、课程对象 1.1、有移动端开发经验 1.2、无移动端开发经验 1.3、对 HarmonyOS 感兴趣 2、DevEco Studio 的使用 2.1、DevEco Studio 的关键特性 智能代码编辑 低代码开发 多段双向实时预览 多端模拟仿真 2.2、安装配置 DevEco Studio 2.2.1、官网开发工具下载地…...
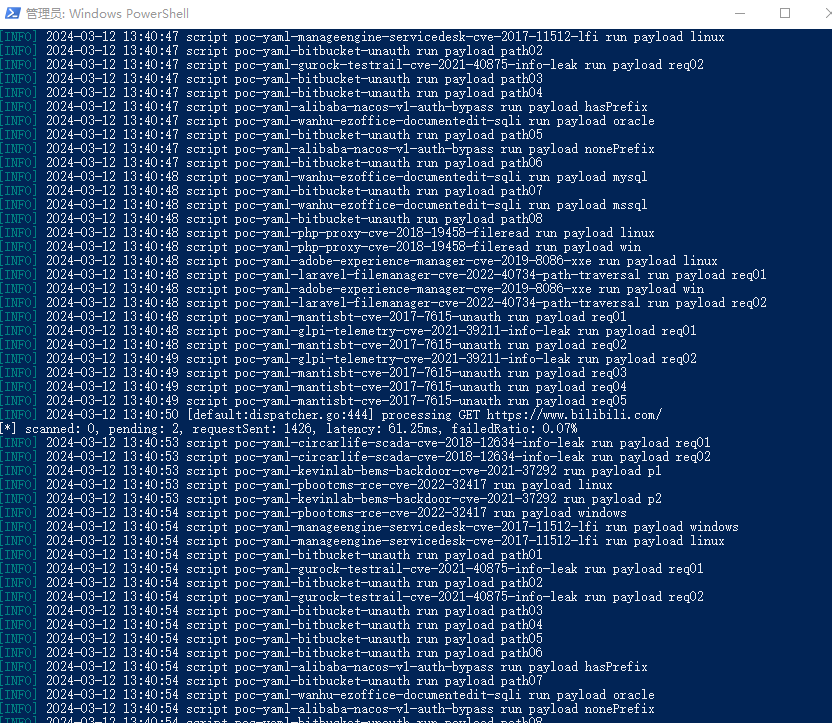
国科大网络行为学导论代码作业--更新中
一、Xray安装 参考自:Xray的安装与使用(超详细)_xray使用教程-CSDN博客 下载网址:Releases chaitin/xray GitHub 解压 双击安装 生成证书 cd到xray目录,生成证书 复制链接 然后cd到xray目录 .\xray_windows_amd6…...

JAVA后端开发面试基础知识(九)——SpringBoot
启动原理 SpringBoot启动非常简单,因其内置了Tomcat,所以只需要通过下面几种方式启动即可: @SpringBootApplication(scanBasePackages = {"cn.dark"}) public class SpringbootDemo {public static void main(String[] args) {// 第一种SpringApplication.run(S…...
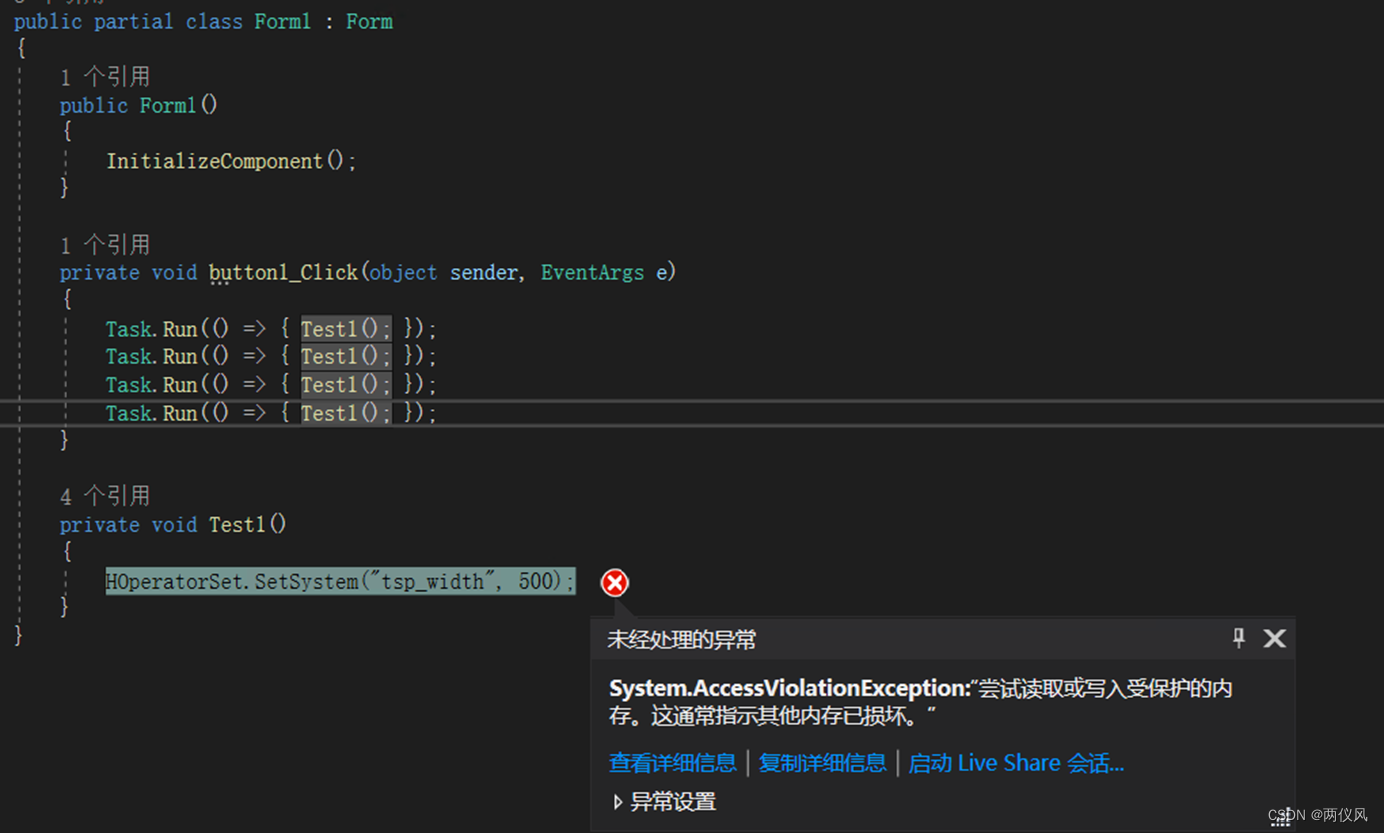
C#调用Halcon出现尝试读取或写入受保护的内存,这通常指示其他内存已损坏。System.AccessViolationException
一、现象 在C#中调用Halcon,出现异常提示:尝试读取或写入受保护的内存,这通常指示其他内存已损坏。System.AccessViolationException 二、原因 多个线程同时访问Halcon中的某个公共变量,导致程序报错 三、测试 3.1 Halcon代码 其中tsp_width…...

ts基础知识
1. any 类型,unknown 类型,never 类型 TypeScript 有两个“顶层类型”(any和unknown),但是“底层类型”只有never唯一一个 1、any 1.1 基本含义 any 类型表示没有任何限制,该类型的变量可以赋予任意类型的…...

KLayout Python Script ------ 绘制1个 Box 物体
KLayout Python Script ------ 绘制两个 Box 物体 引言正文引言 本人通常使用 IPKISS 进行版图绘制,然而很多时候,IPKISS 的功能十分鸡肋。因此,萌生了一种自己写绘制软件的想法,因为 IPKISS 绘制的版图最终也是使用 KLayout 来呈现的,因此,再研究了 KLayout 提供的 API…...