List(CS61B学习记录)
- 问题引入
上图中,赋给b海象的weight会改变a海象的weight,但x的赋值又不会改变y的赋值
Bits
要解释上图的问题,我们应该从Java的底层入手
相同的二进制编码,却因为数据类型不同,输出不同的值
变量的声明
基本类型
Java does not write anything into the reserved box when a variable is declared. In other words, there are no default values. As a result, the Java compiler prevents you from using a variable until after the box has been filled with bits using the = operator. For this reason, I have avoided showing any bits in the boxes in the figure above.
引用类型
When we declare a variable of any reference type (Walrus, Dog, Planet, array, etc.), Java allocates a box of 64 bits, no matter what type of object.
96位大于64位,这似乎有些矛盾:
在Java中:the 64 bit box contains not the data about the walrus, but instead the address of the Walrus in memory.
Gloden rule
练习
答案:B
解析:
- 在调用方法(函数)时,doStuff方法中的int x与main方法中的int x 实际上处于两个不同的scope(调用方法时会new 一个scope,并将main方法中的x变量的位都传递给doStuff方法中的x变量,即值传递),所以x = x - 5实际上只作用于doStuff方法中的x,而不是main方法中的x。
- 但对于引用类型来说,引用类型储存的是引用的地址,所以在进行值传递时传递的是对象的地址,所以doStuff方法中的int x与main方法中的walrus实际上指向相同的一个对象,这使得doStuff中执行的语句会作用于main方法中walrus指向的对象,所以反作用于main方法中的walrus
IntLists
使用递归求链表中元素的个数
/** Return the size of the list using... recursion! */
public int size() {if (rest == null) {return 1;}return 1 + this.rest.size();
}
Exercise: You might wonder why we don’t do something like if (this == null) return 0;. Why wouldn’t this work?
Answer: Think about what happens when you call size. You are calling it on an object, for example L.size(). If L were null, then you would get a NullPointer error!
不使用递归求元素个数
/** Return the size of the list using no recursion! */
public int iterativeSize() {IntList p = this;int totalSize = 0;while (p != null) {totalSize += 1;p = p.rest;}return totalSize;
}
求第n个元素
SLList
接下来我们对IntList进行改进,使链表看上去不那么naked
有了节点类,现在我们补充上链表类
public class SLList {public IntNode first;public SLList(int x) {first = new IntNode(x, null);}
}
对比SLList和IntList,我们发现,在使用SLList时无需强调null
IntList L1 = new IntList(5, null);
SLList L2 = new SLList(5);
addFirst() and getFirst()
/** Adds an item to the front of the list. */public void addFirst(int x) {first = new IntNode(x, first);}/** Retrieves the front item from the list. */public int getFirst() {return first.item;
}
private and nested class
public class SLList {public class IntNode {public int item;public IntNode next;public IntNode(int i, IntNode n) {item = i;next = n;}}private IntNode first;public SLList(int x) {first = new IntNode(x, null);}/** Adds an item to the front of the list. */public void addFirst(int x) {first = new IntNode(x,first);}/** Retrieves the front item from the list. */public int getFirst() {return first.item;}
}
addLast() and Size()
/** Adds an item to the end of the list. */
public void addLast(int x) {IntNode p = first;/* Advance p to the end of the list. */while (p.next != null) {p = p.next;}p.next = new IntNode(x, null);
}
/** Returns the size of the list starting at IntNode p. */
private static int size(IntNode p) {if (p.next == null) {return 1;}return 1 + size(p.next);
}
优化低效的Size()方法
在改变链表的Size时直接记录下,就可以不需要在Size()方法中遍历链表了
public class SLList {... /* IntNode declaration omitted. */private IntNode first;private int size;public SLList(int x) {first = new IntNode(x, null);size = 1;}public void addFirst(int x) {first = new IntNode(x, first);size += 1;}public int size() {return size;}...
}
空链表( The Empty List)
创建空链表很简单(对于SLList),但会导致addLast()方法报错
public SLList() {first = null;size = 0;
}
public void addLast(int x) {size += 1;IntNode p = first;while (p.next != null) {p = p.next;}p.next = new IntNode(x, null);
}
p指向null,故p.next会出现空指针异常
addLast()改进(使用分支)
public void addLast(int x) {size += 1;if (first == null) {first = new IntNode(x, null);return;}IntNode p = first;while (p.next != null) {p = p.next;}p.next = new IntNode(x, null);
}
addLast()改进(更robust的做法)
对于存在很多特殊情况需要讨论的数据结构,上面的方法就显得十分低效。
故我们需要考虑将其改进为一个具有普适性的方法:将first改为sentinal.next
sentinal将会永远存在于链表的上位
package lists.sslist;public class SLList {public class IntNode {public int item;public IntNode next;public IntNode(int i, IntNode n) {item = i;next = n;}}private IntNode sentinel;private int size;public SLList() {sentinel = new IntNode(63,null);size = 0;}public SLList(int x) {sentinel = new IntNode(63,null);sentinel.next = new IntNode(x, null);size = 1;}/** Adds an item to the front of the list. */public void addFirst(int x) {sentinel.next = new IntNode(x, sentinel.next);size += 1;}/** Retrieves the front item from the list. */public int getFirst() {return sentinel.next.item;}/** Returns the number of items in the list. */public int size() {return size;}/** Adds an item to the end of the list. */public void addLast(int x) {IntNode p = sentinel;/* Advance p to the end of the list. */while (p.next != null) {p = p.next;}p.next = new IntNode(x, null);}/** Crashes when you call addLast on an empty SLList. Fix it. */public static void main(String[] args) {SLList x = new SLList();x.addLast(5);}
}
相关文章:
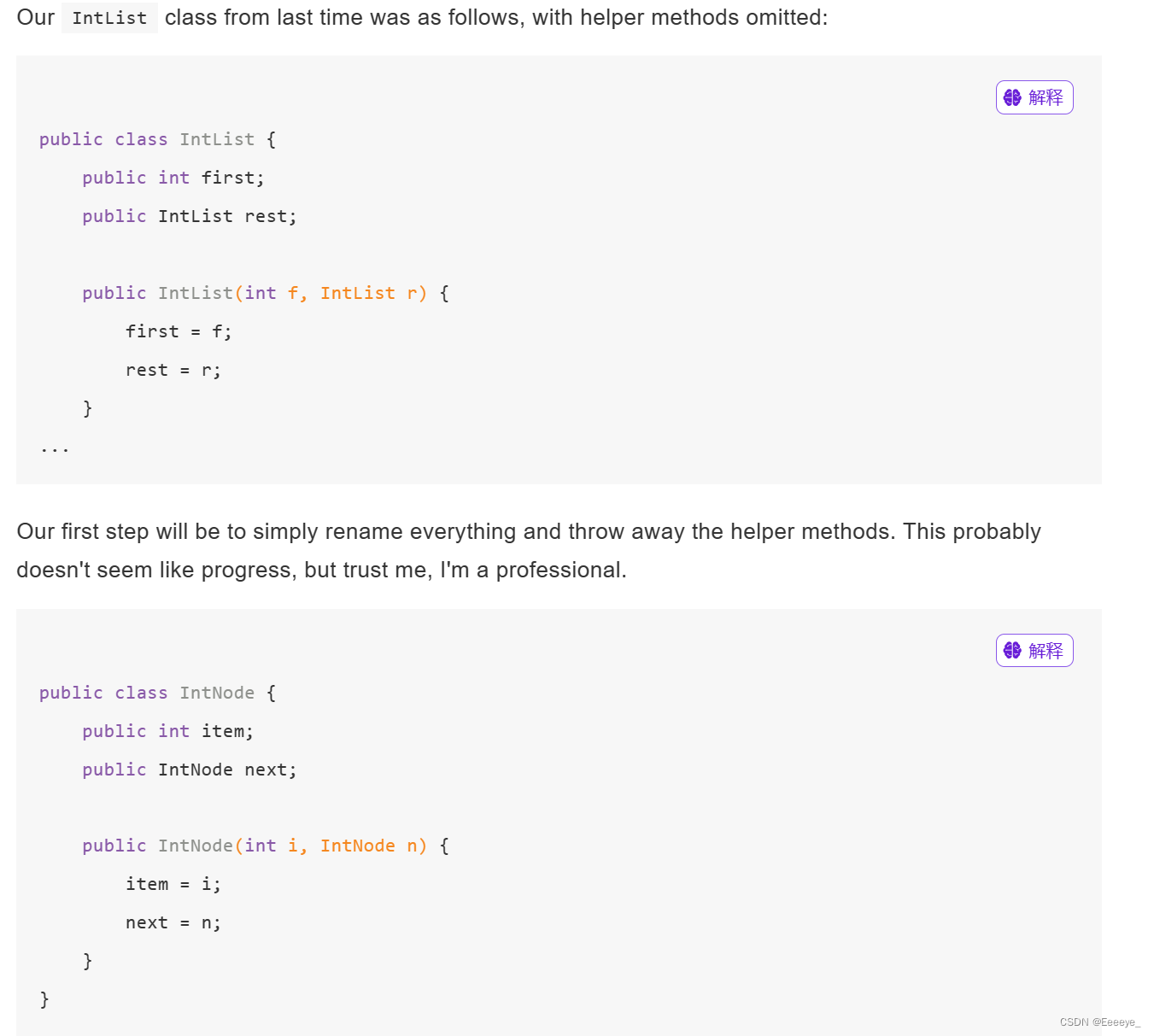
List(CS61B学习记录)
问题引入 上图中,赋给b海象的weight会改变a海象的weight,但x的赋值又不会改变y的赋值 Bits 要解释上图的问题,我们应该从Java的底层入手 相同的二进制编码,却因为数据类型不同,输出不同的值 变量的声明 基本类型…...
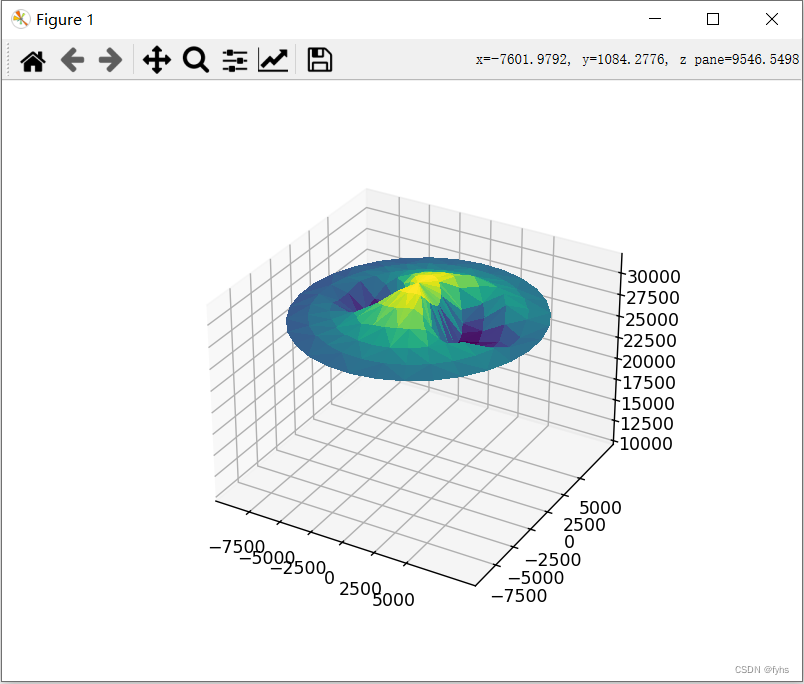
Python 导入Excel三维坐标数据 生成三维曲面地形图(面) 1、线条折线曲面
环境和包: 环境 python:python-3.12.0-amd64包: matplotlib 3.8.2 pandas 2.1.4 openpyxl 3.1.2 代码: import pandas as pd import matplotlib.pyplot as plt import numpy as np from mpl_toolkits.mplot3d import Axes3D from matplotlib.colors import ListedColor…...
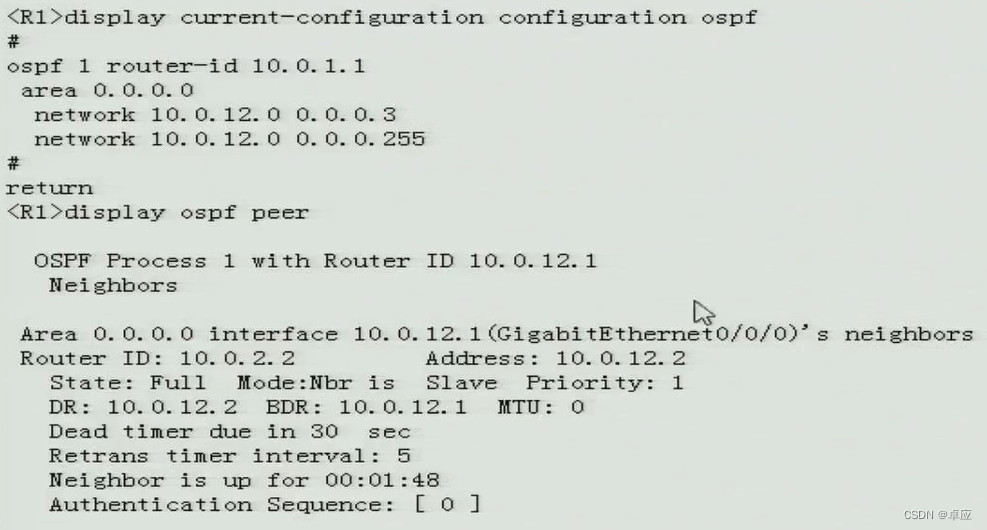
2024年华为HCIA-DATACOM新增题库(H12-811)
801、[单选题]178/832、在系统视图下键入什么命令可以切换到用户视图? A quit B souter C system-view D user-view 试题答案:A 试题解析:在系统视图下键入quit命令退出到用户视图。因此答案选A。 802、[单选题]“网络管理员在三层交换机上创建了V…...

离线安装数据库 mysql 5.7 linux
离线安装数据库 mysql 5.7 linux 方法一 参考链接Linux(Debian10.2)安装MySQL5.7.24环境 赋予文件执行权限chmod x 文件名 使用root用户sudo su解压文件tar xvf mysql-5.7.42-linux-glibc2.12-x86_64.tar.gz重命名mv mysql-5.7.42-linux-glibc2.12-x86_64 mysql将桌面的mys…...

2024-03-14学习笔记(YoloV9)
1.认知越高的人,越敬畏因果 摘要:本文讲述了认知越高的人越敬畏因果的道理。通过故事和名人案例,阐述了敬畏因果对于个人成长和成功的重要性。文章强调了遵循规律、不走捷径、正向思维的重要性,以及思维、行动、习惯、性格和命运…...
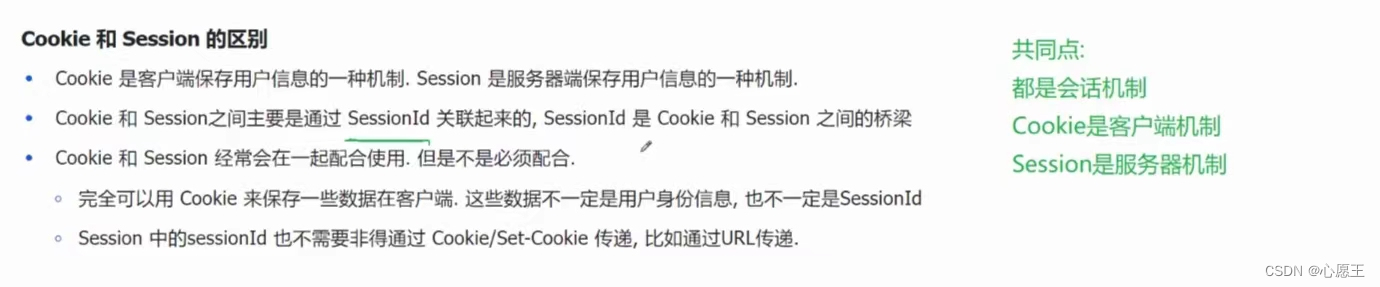
Cookie和Session介绍
1、Cookie的一些重要结论(令牌,类似就诊卡记住我们的信息): (1)Cookie从哪里来:服务器返回给浏览器的,通常是首次访问/登录成功之后(cookie是在header中传输)…...
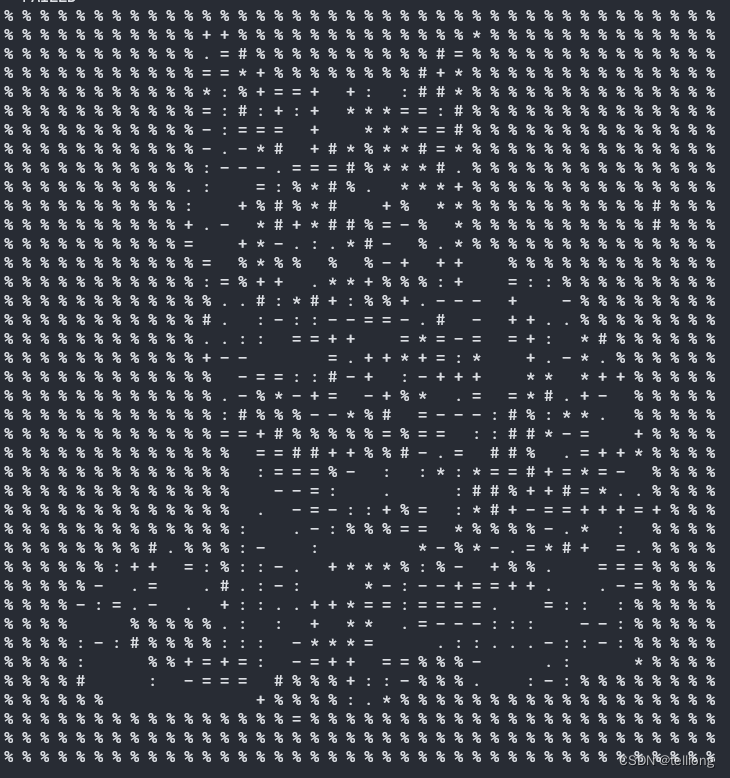
OpenCV 将rgb图像转化成字符图像
将RGB图像转换成字符图像(ASCII art)通常涉及到灰度化、降采样、映射字符等一系列步骤。以下是一个简化的OpenCVC实现示例: #include <opencv2/opencv.hpp> #include <iostream> #include <string>// 字符映射表ÿ…...
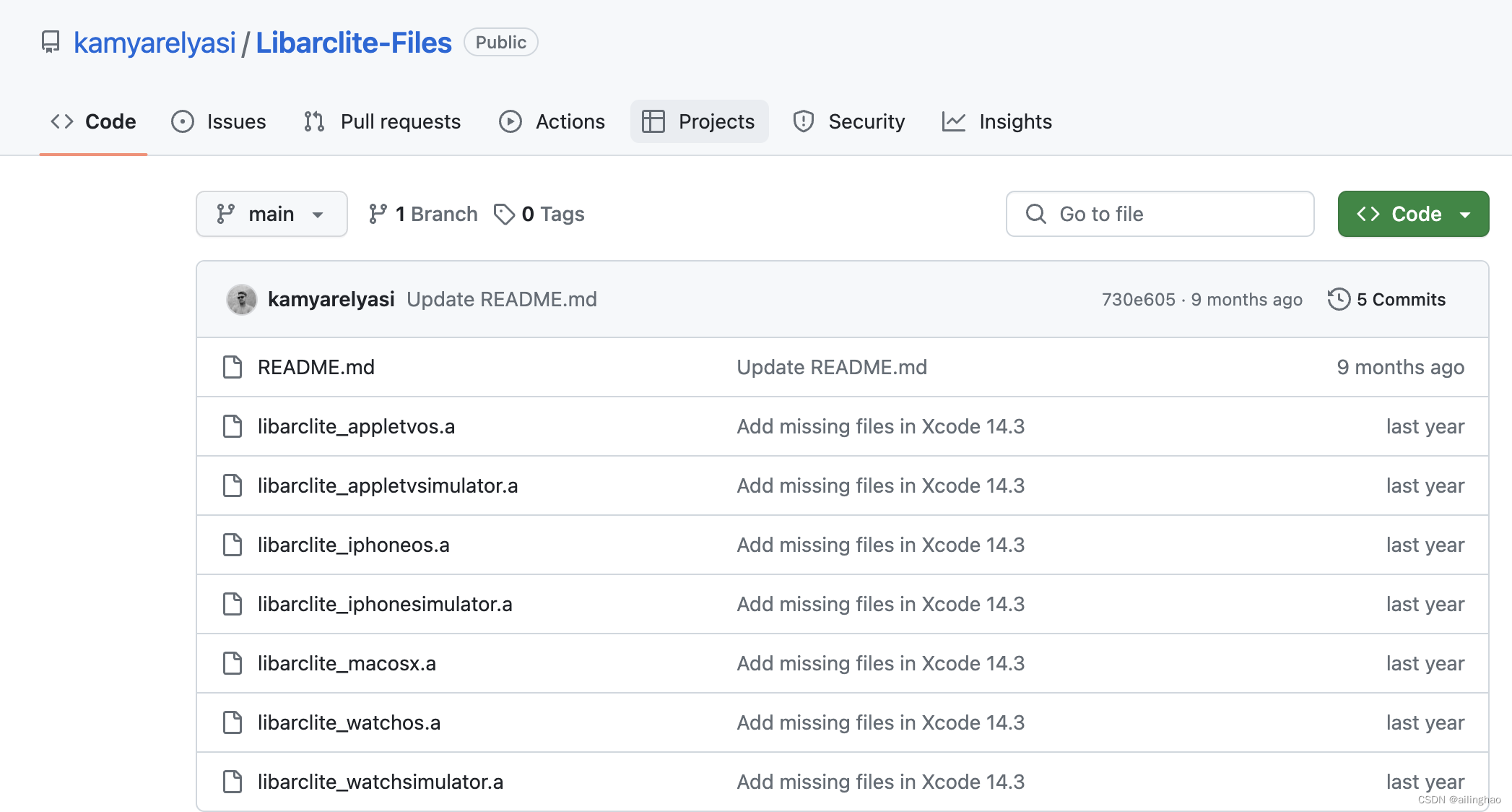
ios开发错误积累
1.xcode 下载模拟器报错 Could not download iOS 报错: 解决: 1、去官网下载自己需要 地址(https://developer.apple.com/download/all) 2、下载完成后,执行以下命令添加:xcrun simctl runtime add /路径…...
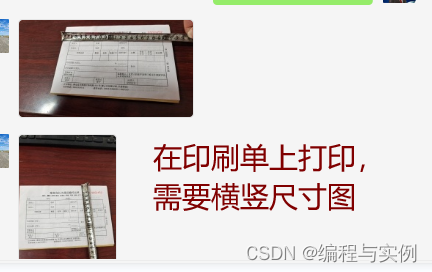
软件实际应用实例,物流打印用什么软件,佳易王物流货运快运单打印查询管理系统软件,可以在已经印刷好的单子上打印,也可直接打印
软件实际应用实例,物流打印用什么软件,佳易王物流货运快运单打印查询管理系统软件,可以在已经印刷好的单子上打印,也可直接打印 一、前言 以下软件教程以 佳易王物流单打印查询管理系统软件V17.0为例说明 软件文件下载可以点击…...
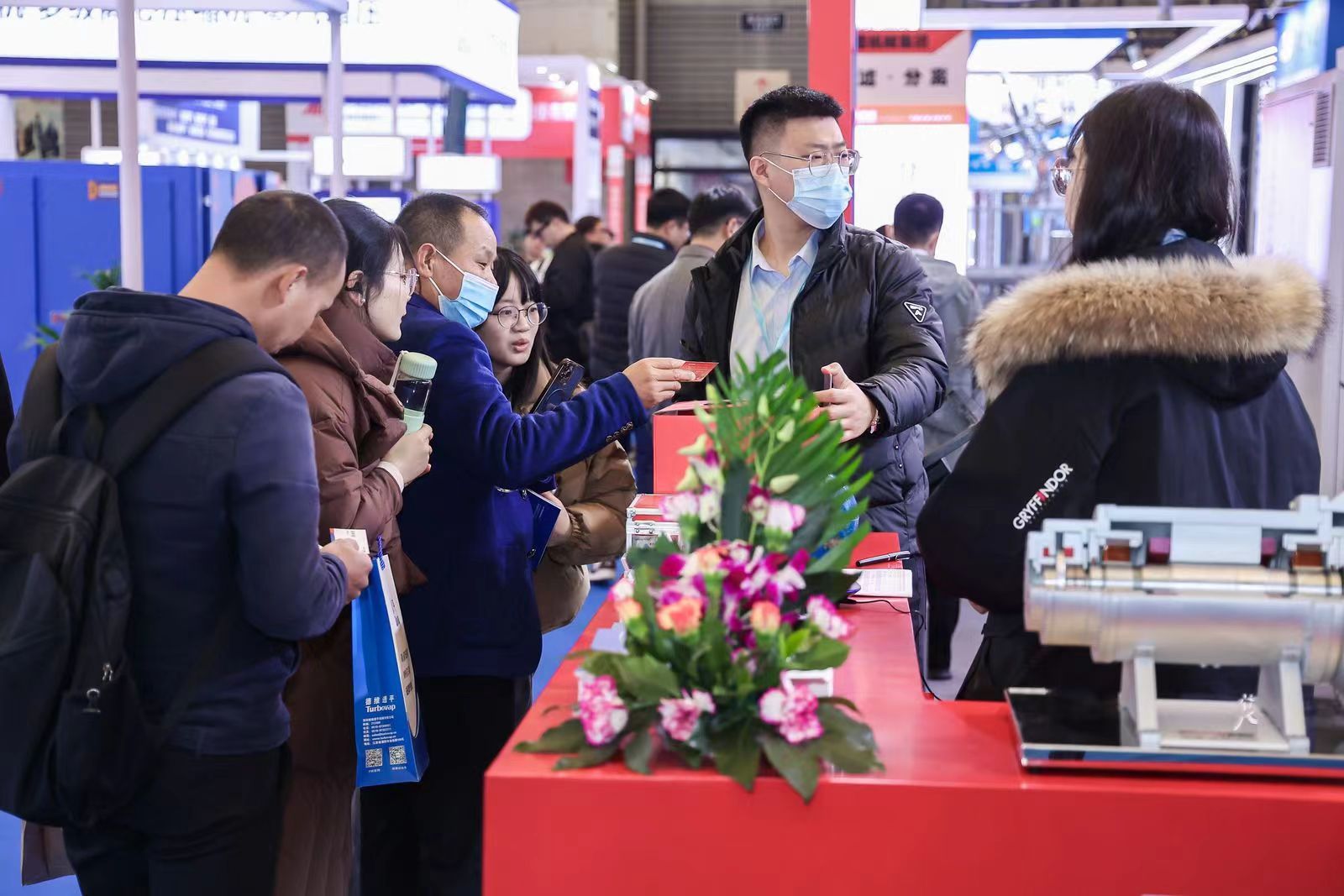
第六届上海国际垃圾焚烧发电展将于12月11-13日上海举办
第六届上海国际垃圾焚烧发电暨固废处理技术展览会 2024年12月11-13日 上海新国际博览中心 主办单位:中华环保联合会 废弃物发电专委会 支持单位:垃圾焚烧技术与装备国家工程实验室 承办单位:上海怡涵展览服务有限公司 展会介绍:…...
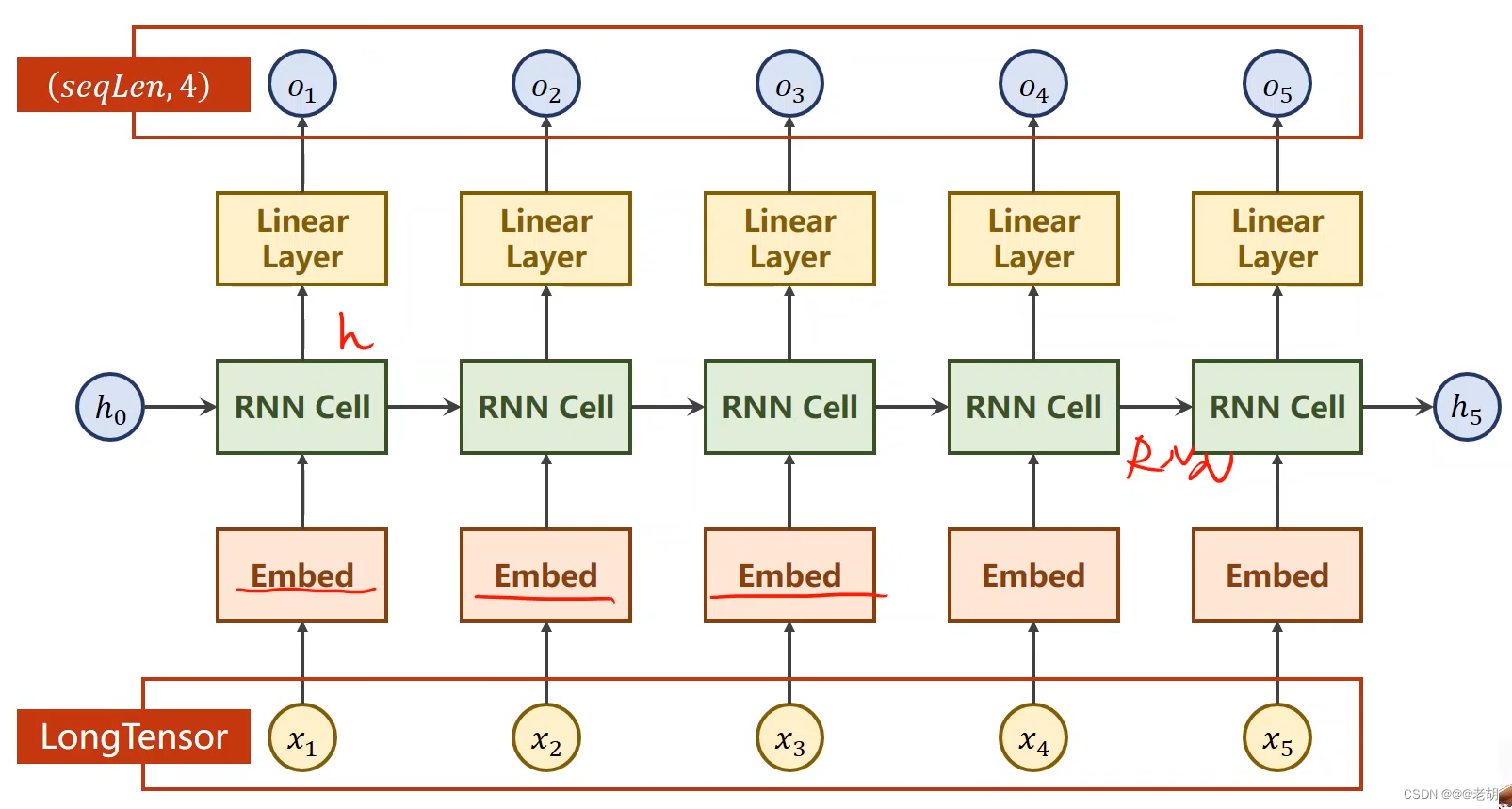
pytorch(十)循环神经网络
文章目录 卷积神经网络与循环神经网络的区别RNN cell结构构造RNN例子 seq2seq 卷积神经网络与循环神经网络的区别 卷积神经网络:在卷积神经网络中,全连接层的参数占比是最多的。 卷积神经网络主要用语处理图像、语音等空间数据,它的特点是局部…...
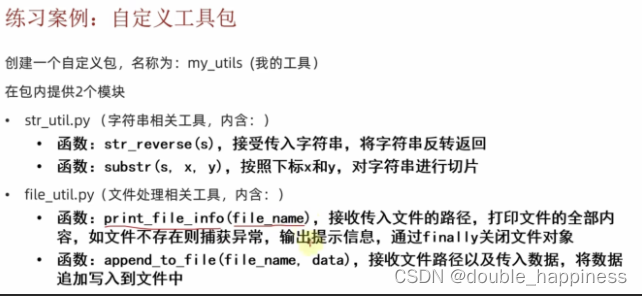
【黑马程序员】Python文件、异常、模块、包
文章目录 文件操作文件编码什么是编码为什么要使用编码 文件的读取openmodel常用的三种基础访问模式读操作相关方法 文件的写入注意代码示例 异常定义异常捕获捕获指定异常捕获多个异常捕获所有异常异常else异常finally 异常的传递 python 模块定义模块的导入import模块名from …...
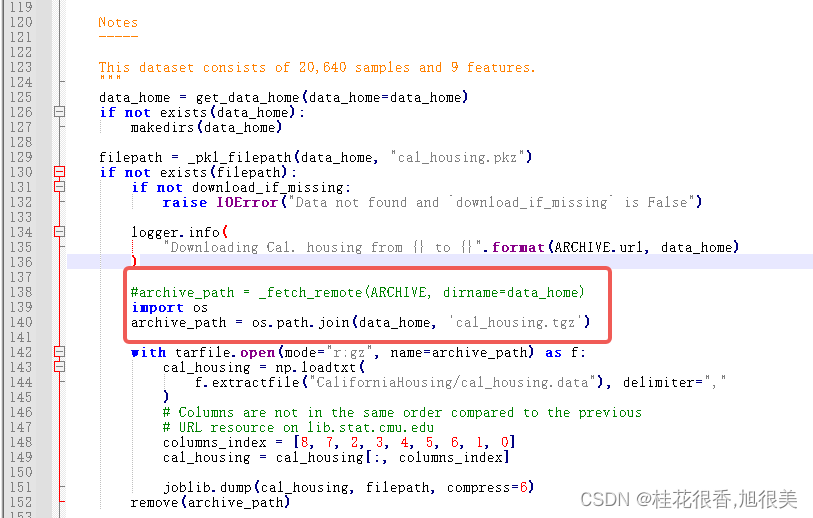
导入fetch_california_housing 加州房价数据集报错解决(HTTPError: HTTP Error 403: Forbidden)
报错 HTTPError Traceback (most recent call last) Cell In[3], line 52 from sklearn.datasets import fetch_california_housing3 from sklearn.model_selection import train_test_split ----> 5 X, Y fetch_california_housing(retu…...
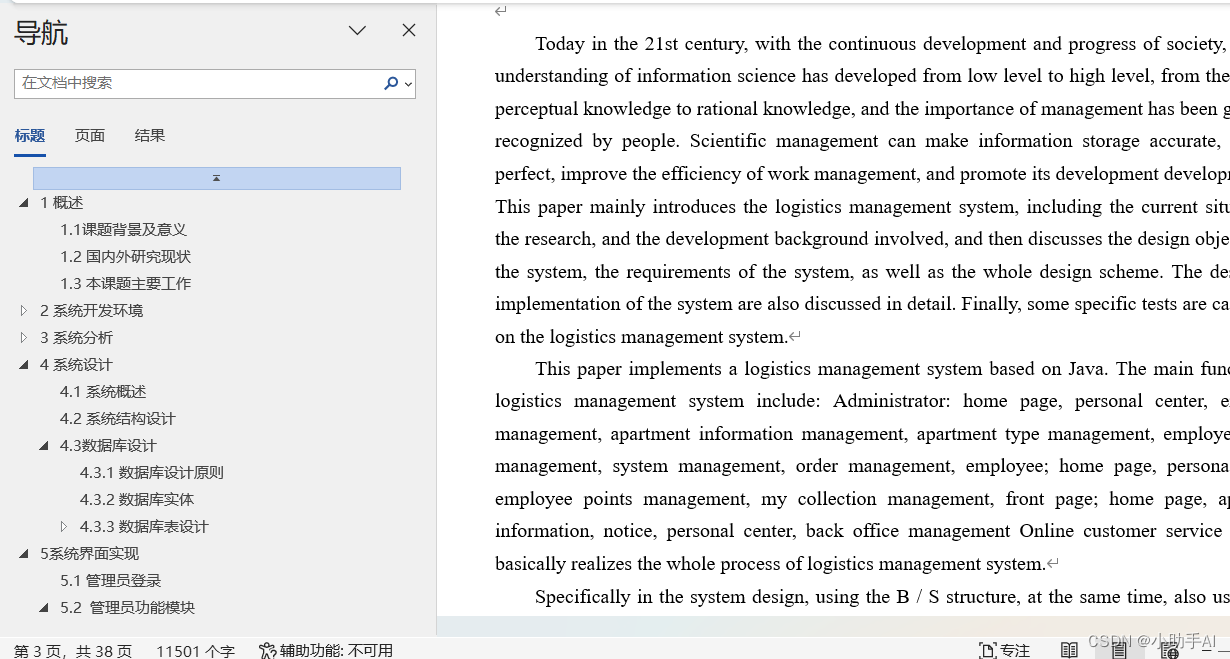
后勤管理系统|基于SSM 框架+vue+ Mysql+Java+B/S架构技术的后勤管理系统设计与实现(可运行源码+数据库+设计文档+部署说明+视频演示)
目录 文末获取源码 前台首页功能 员工注册、员工登录 个人中心 公寓信息 员工功能模块 员工积分管理 管理员登录 编辑管理员功能模块 个人信息 编辑员工管理 公寓户型管理 编辑公寓信息管理 系统结构设计 数据库设计 luwen参考 概述 源码获取 文末获取源…...
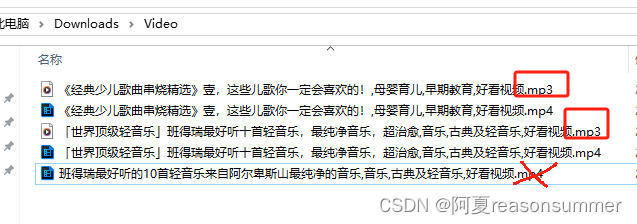
【办公类-40-01】20240311 用Python将MP4转MP3提取音频 (家长会系列一)
作品展示: 背景需求: 马上就要家长会,我负责做会议前的照片滚动PPT,除了大量照片视频,还需要一个时间很长的背景音乐MP3 一、下载“歌曲串烧” 装一个IDM 下载三个“串烧音乐MP4”。 代码展示 家长会背景音乐: 歌曲串…...

人类的谋算与量子计算
量子计算并不等价于并行计算。量子计算和并行计算是两种不同的计算模型。 在经典计算中,通过增加计算机的处理器核心和内存等资源,可以实现并行计算,即多个任务同时进行。并行计算可以显著提高计算速度,尤其是对于可以被细分为多个…...
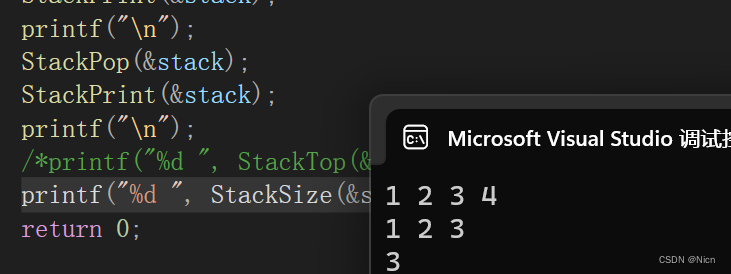
【数据结构和算法初阶(C语言)】栈的概念和实现(后进先出---后来者居上的神奇线性结构带来的惊喜体验)
目录 1.栈 1.1栈的概念及结构 2.栈的实现 3.栈结构对数据的处理方式 3.1对栈进行初始化 3.2 从栈顶添加元素 3.3 打印栈元素 3.4移除栈顶元素 3.5获取栈顶元素 3.6获取栈中的有效个数 3.7 判断链表是否为空 3.9 销毁栈空间 4.结语及整个源码 1.栈 1.1栈的概念及结构 栈&am…...
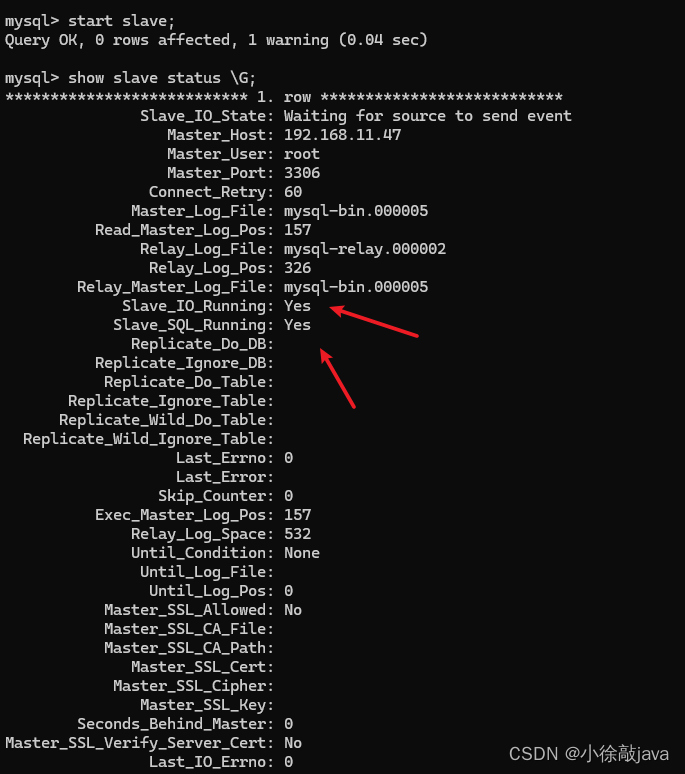
搭建mysql主从复制(主主复制)
1:设主库允许远程连接(注意:设置账号密码必须使用的插件是mysql_native_password,其他的会连接失败) #切换到mysql这个数据库,修改user表中的host,使其可以实现远程连接 mysql>use mysql; mysql>update user se…...
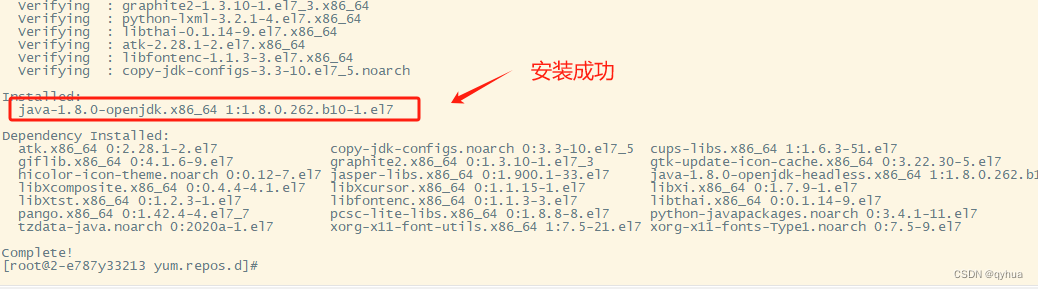
centos 系统 yum 无法安装(换国内镜像地下)
centos 系统 yum 因为无法连接到国外的官网而无法安装,问题如下图: 更换阿里镜像,配置文件路径:/etc/yum.repos.d/CentOS-Base.repo(如果目录有多余的文件可以移动到子目录,以免造成影响) bas…...
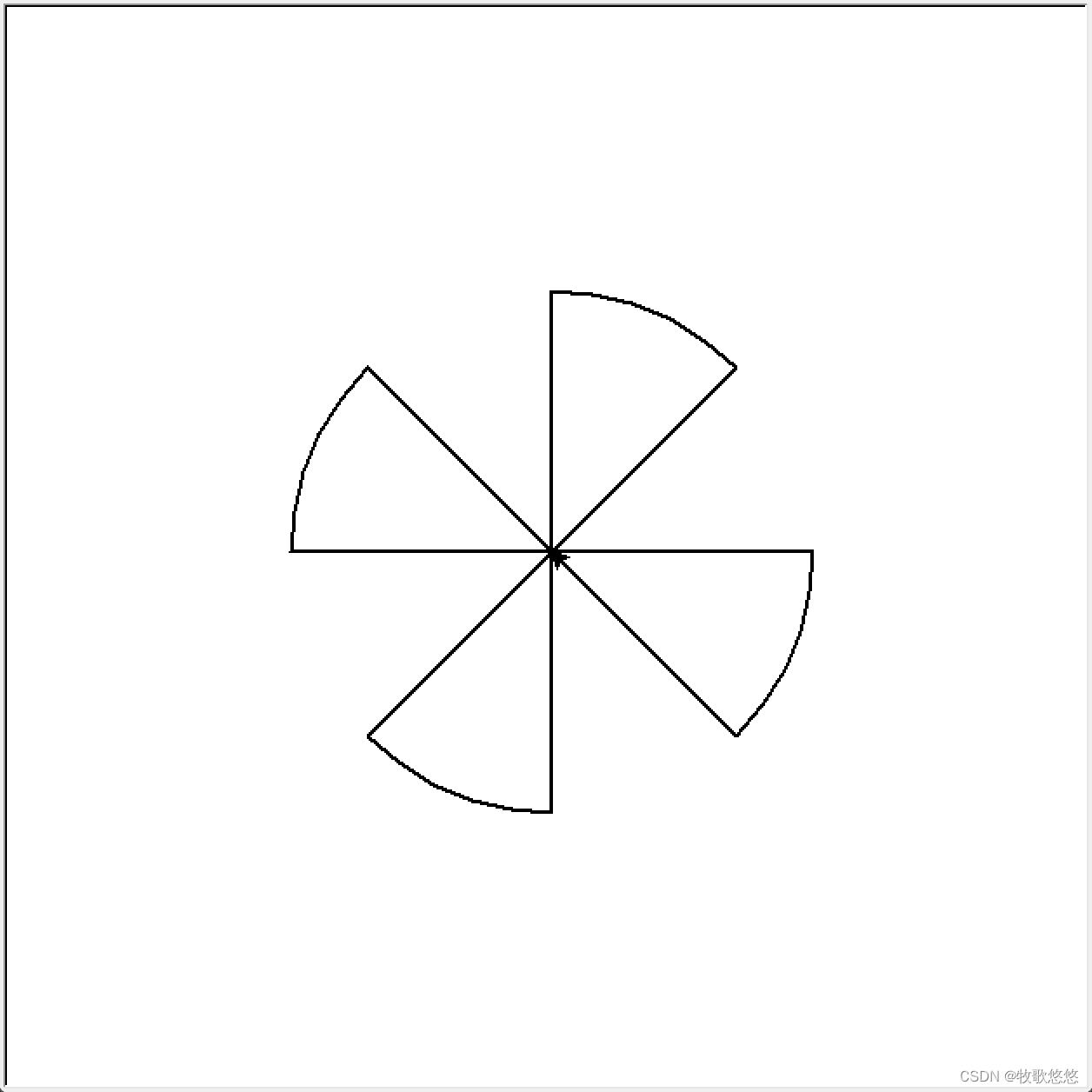
【python绘图】turle 绘图基本案例
文章目录 0. 基础知识1. 蟒蛇绘制2. 正方形绘制3. 六边形绘制4. 叠边形绘制5. 风轮绘制 0. 基础知识 资料来自中国mooc北京理工大学python课程 1. 蟒蛇绘制 import turtle turtle.setup(650, 350, 200, 200) turtle.penup() turtle.fd(-250) turtle.pendown() turtle.pen…...
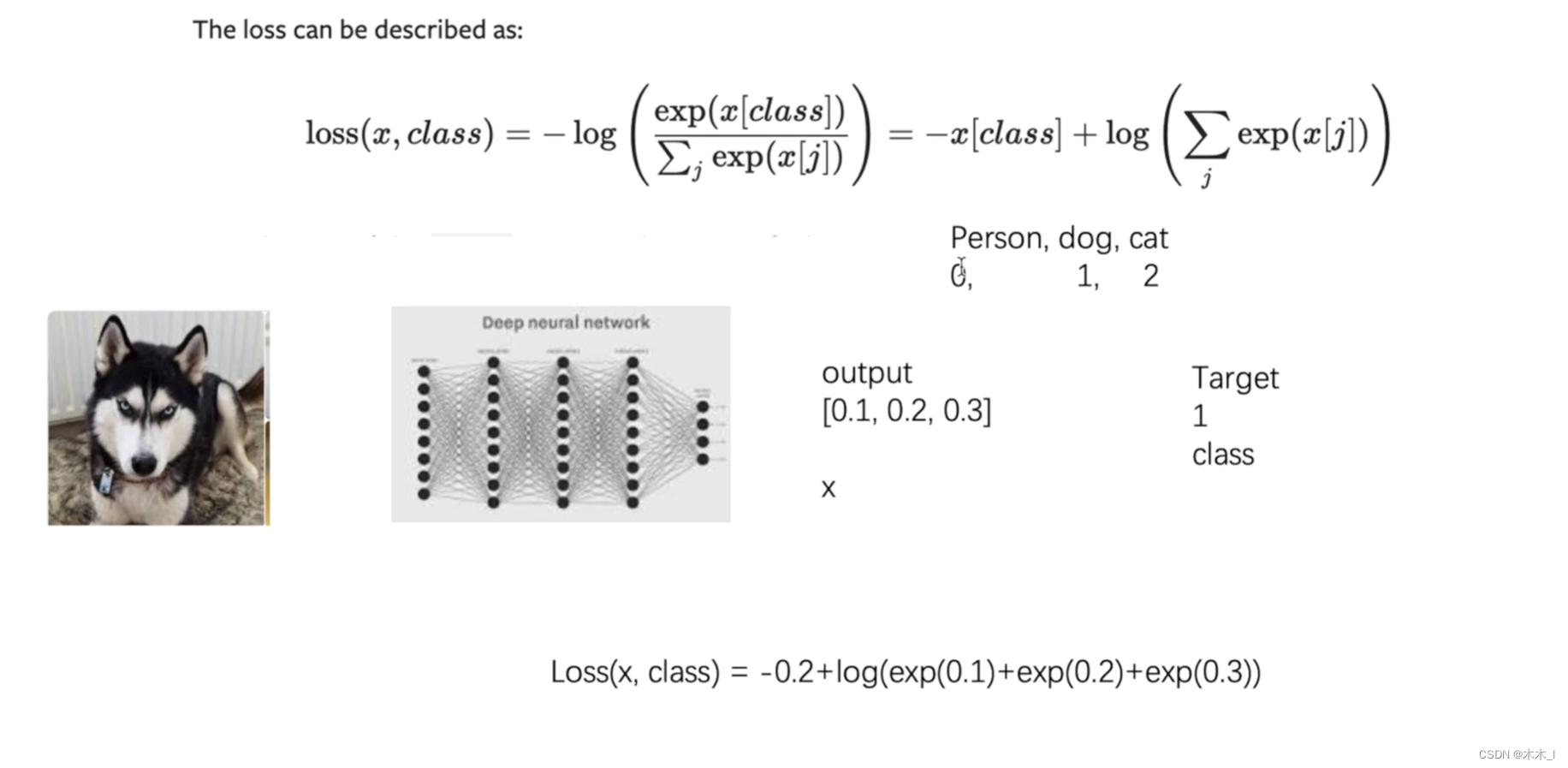
损失函数和反向传播
1. 损失函数的基础 import torch from torch.nn import L1Loss from torch import nninputs torch.tensor([1, 2, 3], dtypetorch.float32) targets torch.tensor([1, 2, 5], dtypetorch.float32)inputs torch.reshape(inputs, (1, 1, 1, 3)) targets torch.reshape(targe…...

Nginx:配置拦截/禁用ip地址
分析nginx日志 1、分析截止目前为止访问量最高的ip排行 awk {print $1} access.log |sort |uniq -c|sort -nr |head -20过滤出access.log日志文件中访问量前20的ip sort :将文件进行排序,并将排序结果标准输出uniq -nr : 去重并在右边显示…...
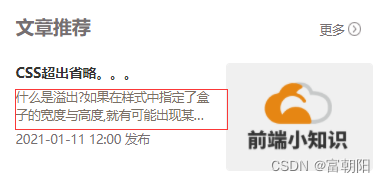
css超出部分显示省略号
目录 前言 一、CSS单行实现 二、CSS多行实现(CSS3出的,兼容性需要注意) 三、微信小程序超过2行出现省略号实现 四、JavaScript脚本实现 前言 CSS文本溢出就显示省略号,就是在样式中指定了盒子的宽度与高度,有可能出现某些内…...

python-0001-安装虚拟环境
版本 软件版本python3.9.10django2.2.5sqlite33.45.1pycharm2023.3.4 安装python3.9.10 升级sqlite3 下载地址:https://download.csdn.net/download/qq_41833259/88944701 升级命令: tar -zxvf sqlite-autoconf-3399999.tar.gz cd sqlite-autoconf-…...
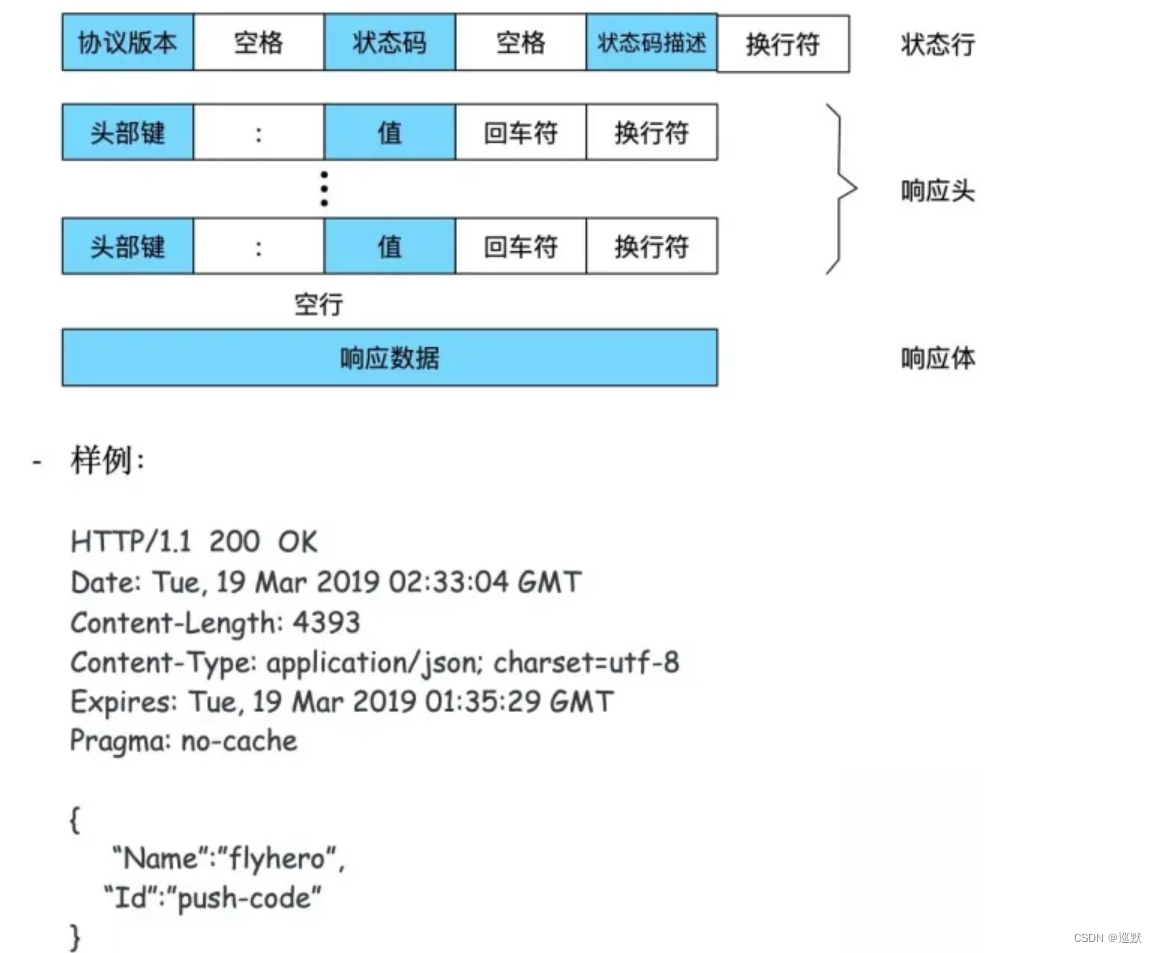
Python爬虫:原理与实战
引言 在当今的信息时代,互联网上的数据如同浩瀚的海洋,充满了无尽的宝藏。Python爬虫作为一种高效的数据抓取工具,能够帮助我们轻松地获取这些数据,并进行后续的分析和处理。本文将深入探讨Python爬虫的原理,并结合实战…...
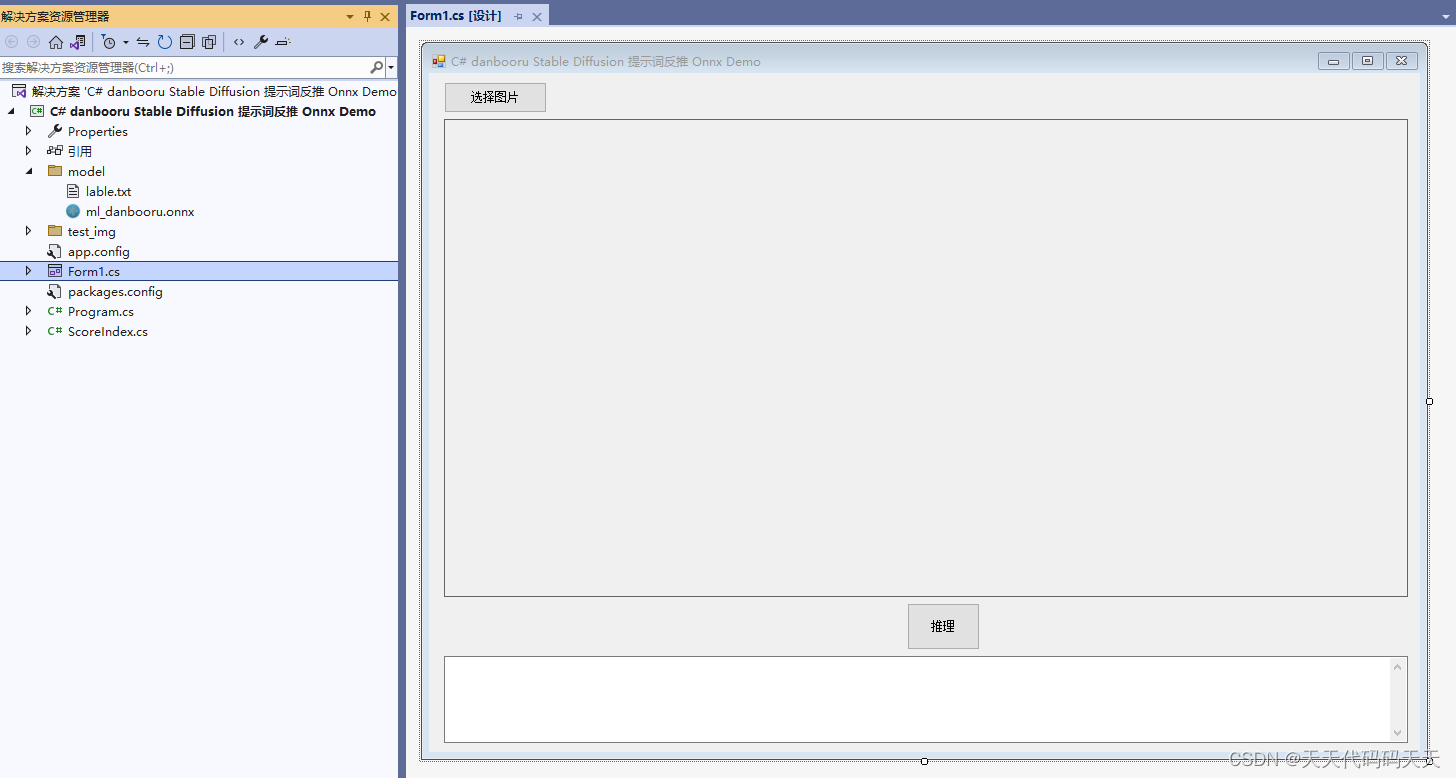
C# danbooru Stable Diffusion 提示词反推 Onnx Demo
目录 说明 效果 模型信息 项目 代码 下载 C# danbooru Stable Diffusion 提示词反推 Onnx Demo 说明 模型下载地址:https://huggingface.co/deepghs/ml-danbooru-onnx 效果 模型信息 Model Properties ------------------------- ----------------------…...
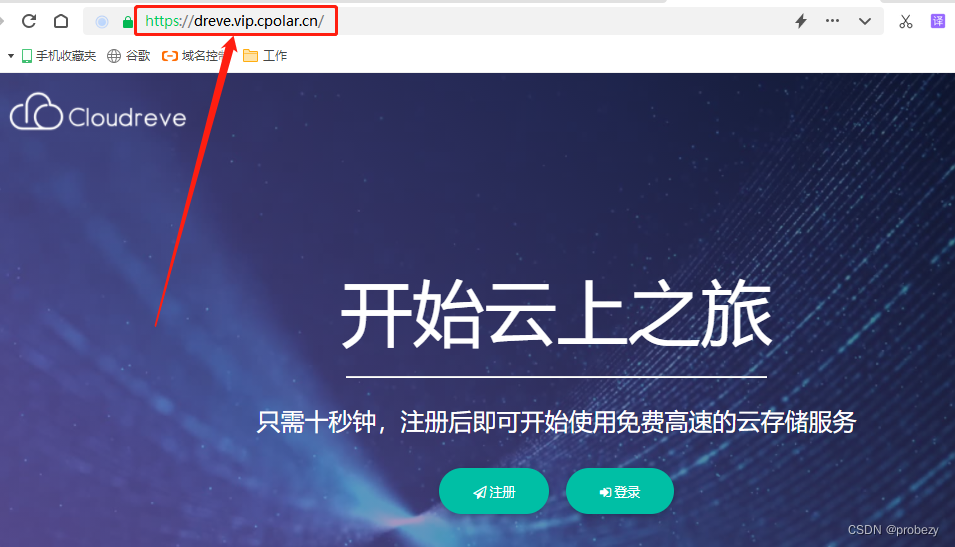
Windows系统搭建Cloudreve结合内网穿透打造可公网访问的私有云盘
目录 ⛳️推荐 1、前言 2、本地网站搭建 2.1 环境使用 2.2 支持组件选择 2.3 网页安装 2.4 测试和使用 2.5 问题解决 3、本地网页发布 3.1 cpolar云端设置 3.2 cpolar本地设置 4、公网访问测试 5、结语 ⛳️推荐 前些天发现了一个巨牛的人工智能学习网站ÿ…...
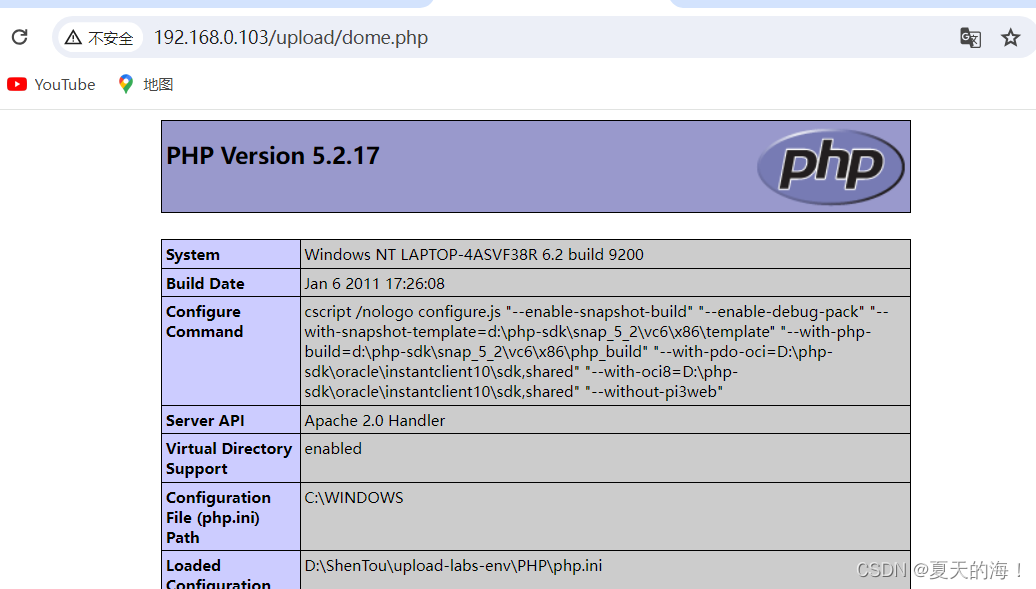
upload-labs 0.1 靶机详解
下载地址https://github.com/c0ny1/upload-labs/releases Pass-01 他让我们上传一张图片,我们先尝试上传一个php文件 发现他只允许上传图片格式的文件,我们来看看源码 我们可以看到它使用js来限制我们可以上传的内容 但是我们的浏览器是可以关闭js功能的…...
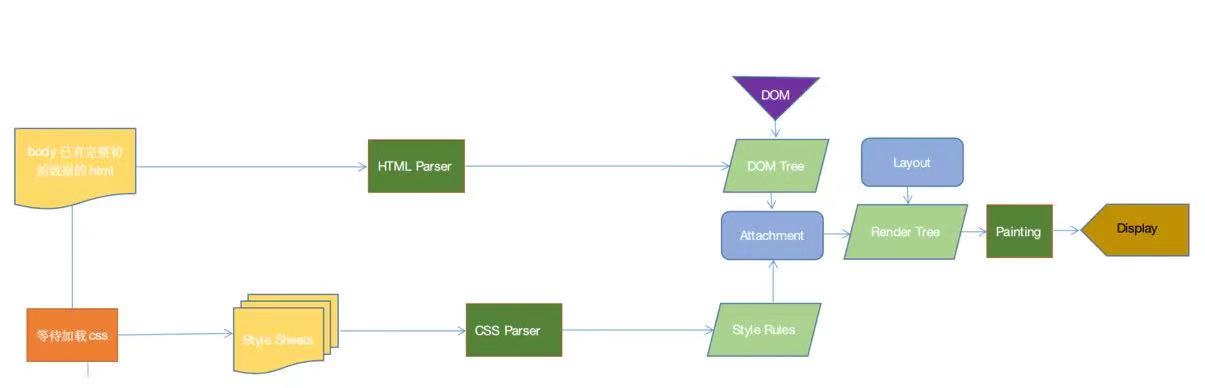
react 综合题-旧版
一、组件基础 1. React 事件机制 javascript 复制代码<div onClick{this.handleClick.bind(this)}>点我</div> React并不是将click事件绑定到了div的真实DOM上,而是在document处监听了所有的事件,当事件发生并且冒泡到document处的时候&a…...
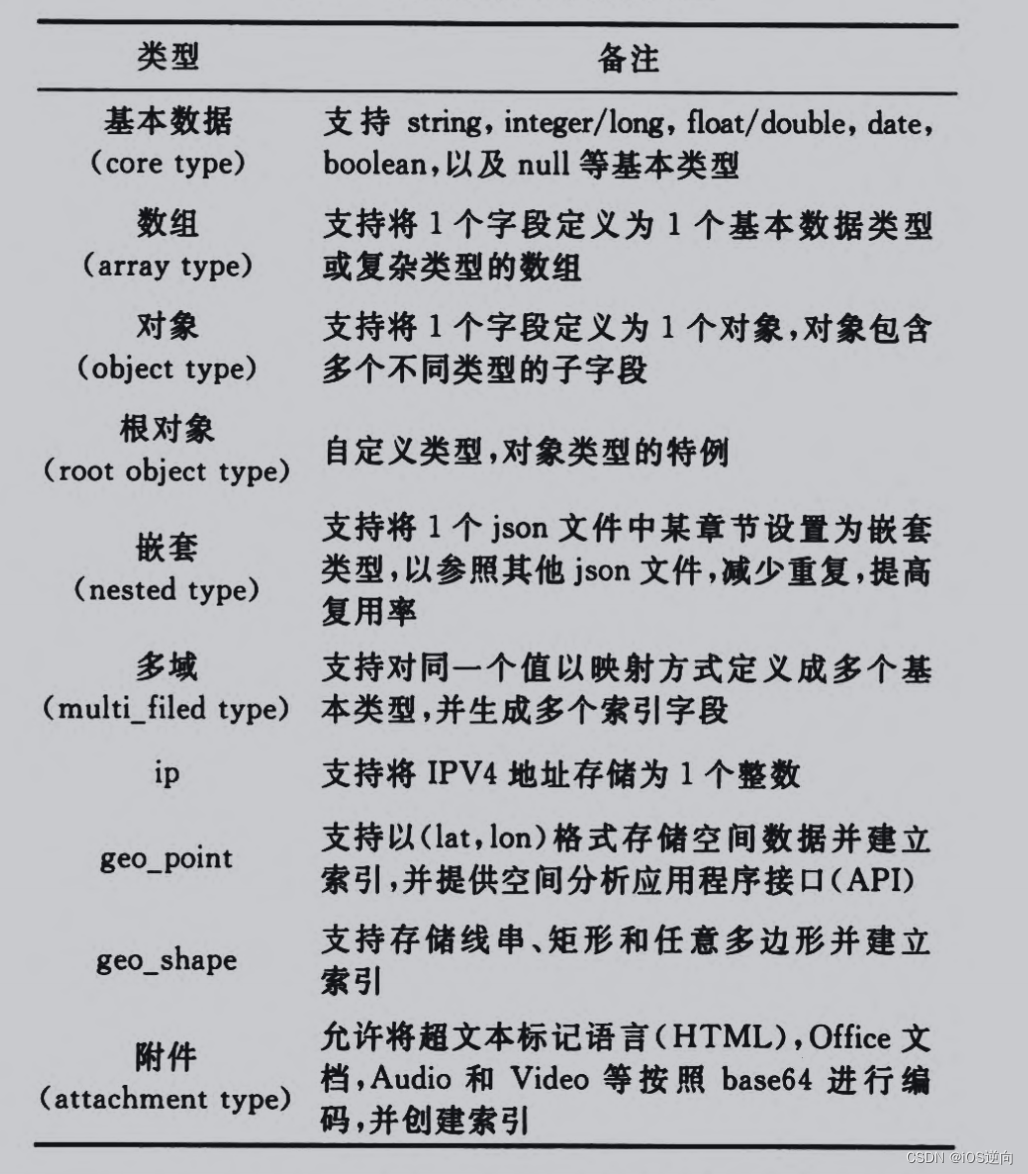
基于ElasticSearch存储海量AIS数据:AIS数据索引机制篇
文章目录 引言I 预备知识1.1 索引结构1.2 AIS信息项II AIS数据索引2.1 AIS数据静态数据索引2.2 AIS数据动态信息索引2.3 引入静态信息的AIS数据轨迹信息索引引言 AIS数据信息根据其不同更新频率可分为静态和动态信息。索引结构设计包含了静态、动态和轨迹信息索引。同时,为了…...