flutter日期选择器仅选择年、月
引入包:flutter_datetime_picker: 1.5.0
封装
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter_datetime_picker/flutter_datetime_picker.dart';class ATuiDateTimePicker {static Future<DateTime> showDatePicker(BuildContext context, {bool showTitleActions: true,bool isShowDay: true,DateTime minTime,DateTime maxTime,DateChangedCallback onChanged,DateChangedCallback onConfirm,DateCancelledCallback onCancel,locale: LocaleType.en,DateTime currentTime,DatePickerTheme theme,}) async {return await Navigator.push(context,_DatePickerRoute(showTitleActions: showTitleActions,onChanged: onChanged,onConfirm: onConfirm,onCancel: onCancel,locale: locale,theme: theme,isShowDay: isShowDay,barrierLabel:MaterialLocalizations.of(context).modalBarrierDismissLabel,pickerModel: DatePickerModel(currentTime: currentTime,maxTime: maxTime,minTime: minTime,locale: locale,),),);}
}class _DatePickerRoute<T> extends PopupRoute<T> {_DatePickerRoute({this.showTitleActions,this.onChanged,this.onConfirm,this.onCancel,theme,this.barrierLabel,this.locale,this.isShowDay,RouteSettings settings,pickerModel,}) : this.pickerModel = pickerModel ?? DatePickerModel(),this.theme = theme ?? DatePickerTheme(),super(settings: settings);final bool showTitleActions;final DateChangedCallback onChanged;final DateChangedCallback onConfirm;final DateCancelledCallback onCancel;final DatePickerTheme theme;final LocaleType locale;final BasePickerModel pickerModel;final bool isShowDay;Duration get transitionDuration => const Duration(milliseconds: 200); bool get barrierDismissible => true;final String barrierLabel;Color get barrierColor => Colors.black54;AnimationController _animationController;AnimationController createAnimationController() {assert(_animationController == null);_animationController =BottomSheet.createAnimationController(navigator.overlay);return _animationController;}Widget buildPage(BuildContext context, Animation<double> animation,Animation<double> secondaryAnimation) {Widget bottomSheet = MediaQuery.removePadding(context: context,removeTop: true,child: _DatePickerComponent(onChanged: onChanged,locale: this.locale,route: this,pickerModel: pickerModel,isShowDay: isShowDay,),);return InheritedTheme.captureAll(context, bottomSheet);}
}class _DatePickerComponent extends StatefulWidget {_DatePickerComponent({Key key, this.route,this.onChanged,this.locale,this.pickerModel,this.isShowDay}): super(key: key);final DateChangedCallback onChanged;final _DatePickerRoute route;final LocaleType locale;final BasePickerModel pickerModel;final bool isShowDay;State<StatefulWidget> createState() {return _DatePickerState();}
}class _DatePickerState extends State<_DatePickerComponent> {FixedExtentScrollController leftScrollCtrl, middleScrollCtrl, rightScrollCtrl;void initState() {super.initState();refreshScrollOffset();}void refreshScrollOffset() {
// print('refreshScrollOffset ${widget.pickerModel.currentRightIndex()}');leftScrollCtrl = FixedExtentScrollController(initialItem: widget.pickerModel.currentLeftIndex());middleScrollCtrl = FixedExtentScrollController(initialItem: widget.pickerModel.currentMiddleIndex());rightScrollCtrl = FixedExtentScrollController(initialItem: widget.pickerModel.currentRightIndex());}Widget build(BuildContext context) {DatePickerTheme theme = widget.route.theme;return GestureDetector(child: AnimatedBuilder(animation: widget.route.animation,builder: (BuildContext context, Widget child) {final double bottomPadding = MediaQuery.of(context).padding.bottom;return ClipRect(child: CustomSingleChildLayout(delegate: _BottomPickerLayout(widget.route.animation.value,theme,showTitleActions: widget.route.showTitleActions,bottomPadding: bottomPadding,),child: GestureDetector(child: Material(color: theme.backgroundColor ?? Colors.white,child: _renderPickerView(theme),),),),);},),);}void _notifyDateChanged() {if (widget.onChanged != null) {widget.onChanged(widget.pickerModel.finalTime());}}Widget _renderPickerView(DatePickerTheme theme) {Widget itemView = _renderItemView(theme);if (widget.route.showTitleActions) {return Column(children: <Widget>[_renderTitleActionsView(theme),itemView,],);}return itemView;}Widget _renderColumnView(ValueKey key,DatePickerTheme theme,StringAtIndexCallBack stringAtIndexCB,ScrollController scrollController,int layoutProportion,ValueChanged<int> selectedChangedWhenScrolling,ValueChanged<int> selectedChangedWhenScrollEnd,) {return Expanded(flex: layoutProportion,child: Container(padding: EdgeInsets.all(8.0),height: theme.containerHeight,decoration: BoxDecoration(color: theme.backgroundColor ?? Colors.white),child: NotificationListener(onNotification: (ScrollNotification notification) {if (notification.depth == 0 &&selectedChangedWhenScrollEnd != null &¬ification is ScrollEndNotification &¬ification.metrics is FixedExtentMetrics) {final FixedExtentMetrics metrics = notification.metrics;final int currentItemIndex = metrics.itemIndex;selectedChangedWhenScrollEnd(currentItemIndex);}return false;},child: CupertinoPicker.builder(key: key,backgroundColor: theme.backgroundColor ?? Colors.white,scrollController: scrollController,itemExtent: theme.itemHeight,onSelectedItemChanged: (int index) {selectedChangedWhenScrolling(index);},useMagnifier: true,itemBuilder: (BuildContext context, int index) {final content = stringAtIndexCB(index);if (content == null) {return null;}return Container(height: theme.itemHeight,alignment: Alignment.center,child: Text(content,style: theme.itemStyle,textAlign: TextAlign.start,),);},),),),);}Widget _renderItemView(DatePickerTheme theme) {return Container(color: theme.backgroundColor ?? Colors.white,child: Row(mainAxisAlignment: MainAxisAlignment.spaceBetween,children: <Widget>[Container(child: widget.pickerModel.layoutProportions()[0] > 0? _renderColumnView(ValueKey(widget.pickerModel.currentLeftIndex()),theme,widget.pickerModel.leftStringAtIndex,leftScrollCtrl,widget.pickerModel.layoutProportions()[0], (index) {widget.pickerModel.setLeftIndex(index);}, (index) {setState(() {refreshScrollOffset();_notifyDateChanged();});}): null,),Text(widget.pickerModel.leftDivider(),style: theme.itemStyle,),Container(child: widget.pickerModel.layoutProportions()[1] > 0? _renderColumnView(ValueKey(widget.pickerModel.currentLeftIndex()),theme,widget.pickerModel.middleStringAtIndex,middleScrollCtrl,widget.pickerModel.layoutProportions()[1], (index) {widget.pickerModel.setMiddleIndex(index);}, (index) {setState(() {refreshScrollOffset();_notifyDateChanged();});}): null,),Text(widget.pickerModel.rightDivider(),style: theme.itemStyle,),widget.isShowDay ?? true? Container(child: widget.pickerModel.layoutProportions()[2] > 0? _renderColumnView(ValueKey(widget.pickerModel.currentMiddleIndex() * 100 +widget.pickerModel.currentLeftIndex()),theme,widget.pickerModel.rightStringAtIndex,rightScrollCtrl,widget.pickerModel.layoutProportions()[2], (index) {widget.pickerModel.setRightIndex(index);}, (index) {setState(() {refreshScrollOffset();_notifyDateChanged();});}): null,): Container(),],),);}// Title ViewWidget _renderTitleActionsView(DatePickerTheme theme) {final done = _localeDone();final cancel = _localeCancel();return Container(height: theme.titleHeight,decoration: BoxDecoration(color: theme.headerColor ?? theme.backgroundColor ?? Colors.white,),child: Row(mainAxisAlignment: MainAxisAlignment.spaceBetween,children: <Widget>[Container(height: theme.titleHeight,child: CupertinoButton(pressedOpacity: 0.3,padding: EdgeInsets.only(left: 16, top: 0),child: Text('$cancel',style: theme.cancelStyle,),onPressed: () {Navigator.pop(context);if (widget.route.onCancel != null) {widget.route.onCancel();}},),),Container(height: theme.titleHeight,child: CupertinoButton(pressedOpacity: 0.3,padding: EdgeInsets.only(right: 16, top: 0),child: Text('$done',style: theme.doneStyle,),onPressed: () {Navigator.pop(context, widget.pickerModel.finalTime());if (widget.route.onConfirm != null) {widget.route.onConfirm(widget.pickerModel.finalTime());}},),),],),);}String _localeDone() {return i18nObjInLocale(widget.locale)['done'];}String _localeCancel() {return i18nObjInLocale(widget.locale)['cancel'];}
}class _BottomPickerLayout extends SingleChildLayoutDelegate {_BottomPickerLayout(this.progress,this.theme, {this.itemCount,this.showTitleActions,this.bottomPadding = 0,});final double progress;final int itemCount;final bool showTitleActions;final DatePickerTheme theme;final double bottomPadding;BoxConstraints getConstraintsForChild(BoxConstraints constraints) {double maxHeight = theme.containerHeight;if (showTitleActions) {maxHeight += theme.titleHeight;}return BoxConstraints(minWidth: constraints.maxWidth,maxWidth: constraints.maxWidth,minHeight: 0.0,maxHeight: maxHeight + bottomPadding,);}Offset getPositionForChild(Size size, Size childSize) {final height = size.height - childSize.height * progress;return Offset(0.0, height);} bool shouldRelayout(_BottomPickerLayout oldDelegate) {return progress != oldDelegate.progress;}
}
引用
GestureDetector(
child: Container(width:100,height:45,color:Colors.red)
onTap: (){LocaleType localeType;await ATuiSharedPreferences.getStorage("localType").then((value) {if (value == "zh") {localeType = LocaleType.zh;} else if (value == "en") {localeType = LocaleType.en;}});ATuiDateTimePicker.showDatePicker(context,isShowDay: false,locale: localeType ?? LocaleType.zh,theme: DatePickerTheme(cancelStyle: TextStyle(fontSize: 30.sp,color: Color(0xff5dc8b6),fontWeight: FontWeight.w600),doneStyle: TextStyle(fontSize: 30.sp,color: Color(0xff5dc8b6),fontWeight: FontWeight.w600)),onConfirm: (datetime) async {String newDate = '${datetime.year}-';newDate += datetime.month < 10? '0${datetime.month}': datetime.month.toString();walletListModel.changeDate(newDate);walletListModel.changeShowDate('${datetime.year}年${datetime.month}月');walletListModel.refreshData();},onChanged: (datetime) {print('dateTime: ${datetime}');},);},
)
相关文章:
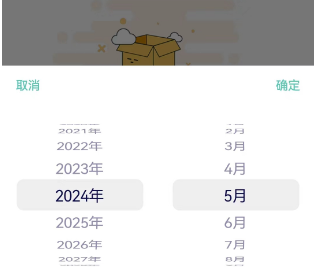
flutter日期选择器仅选择年、月
引入包:flutter_datetime_picker: 1.5.0 封装 import package:flutter/cupertino.dart; import package:flutter/material.dart; import package:flutter_datetime_picker/flutter_datetime_picker.dart;class ATuiDateTimePicker {static Future<DateTime> …...
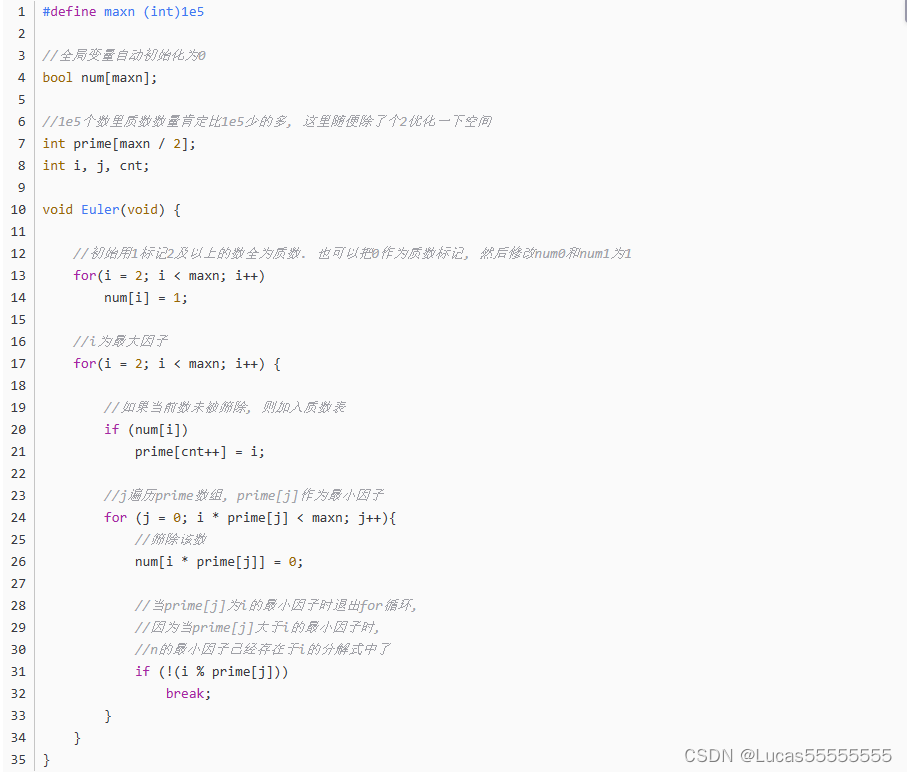
素数筛详解c++
一、埃式筛法 代码 二、线性筛法(欧拉筛法) 主要的思想就是一个质数的倍数(倍数为1除外)肯定是合数,那么我们利用这个质数算出合数,然后划掉这个合数,下次就可以不用判断它是不是质数,节省了大量的时间。 …...
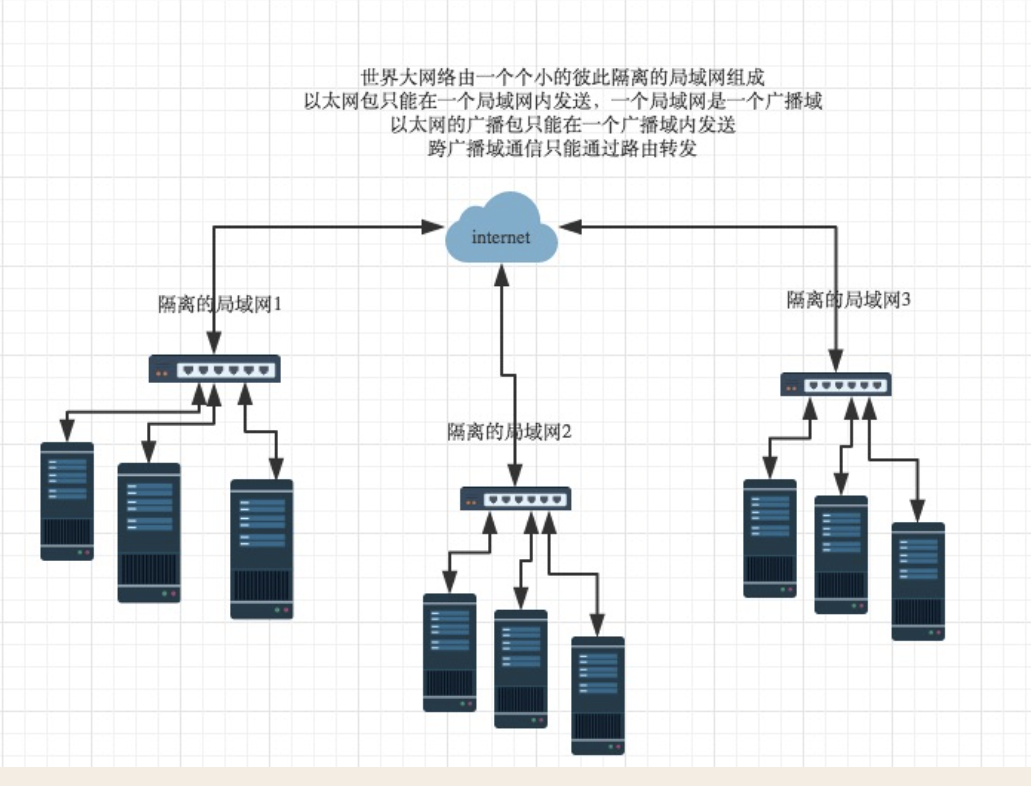
【Python超详细的学习笔记】Python超详细的学习笔记,涉及多个领域,是个很不错的笔记
获取笔记链接 Python超详细的学习笔记 一,逆向加密模块 1,Python中运行JS代码 1.1 解决中文乱码或者报错问题 import subprocess from functools import partial subprocess.Popen partial(subprocess.Popen, encodingutf-8) import execjs1.2 常用…...
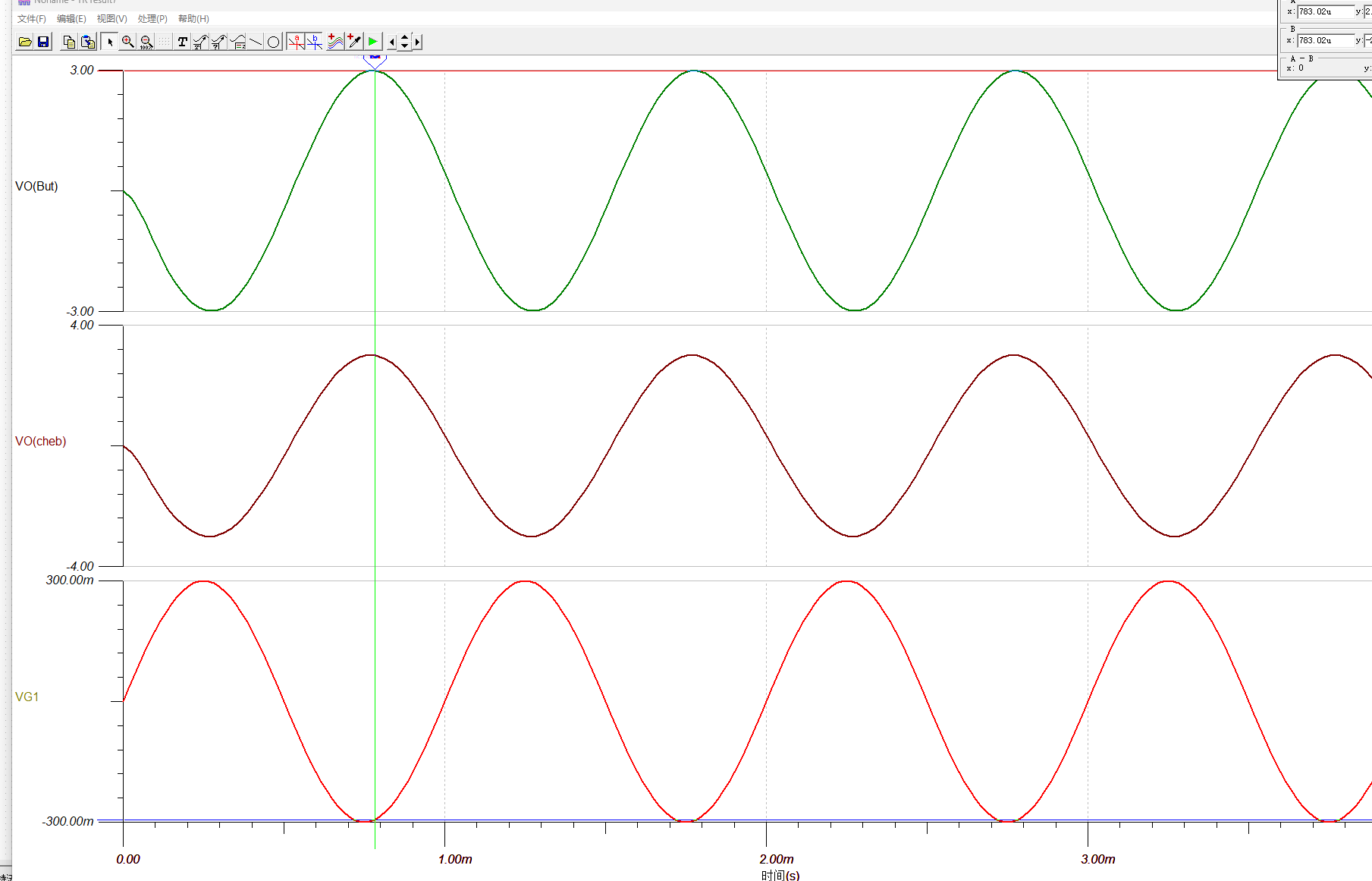
TINA 使用教程
常用功能 分析-电气规则检查:短路,断路等分析- 直流分析 交流分析 瞬态分析 视图-分离曲线 由于输出的容性负载导致的振荡 增加5欧电阻后OK 横扫参数 添加横扫曲线的电阻,选择R3:8K-20K PWL和WAV文件的支持 示例一:…...
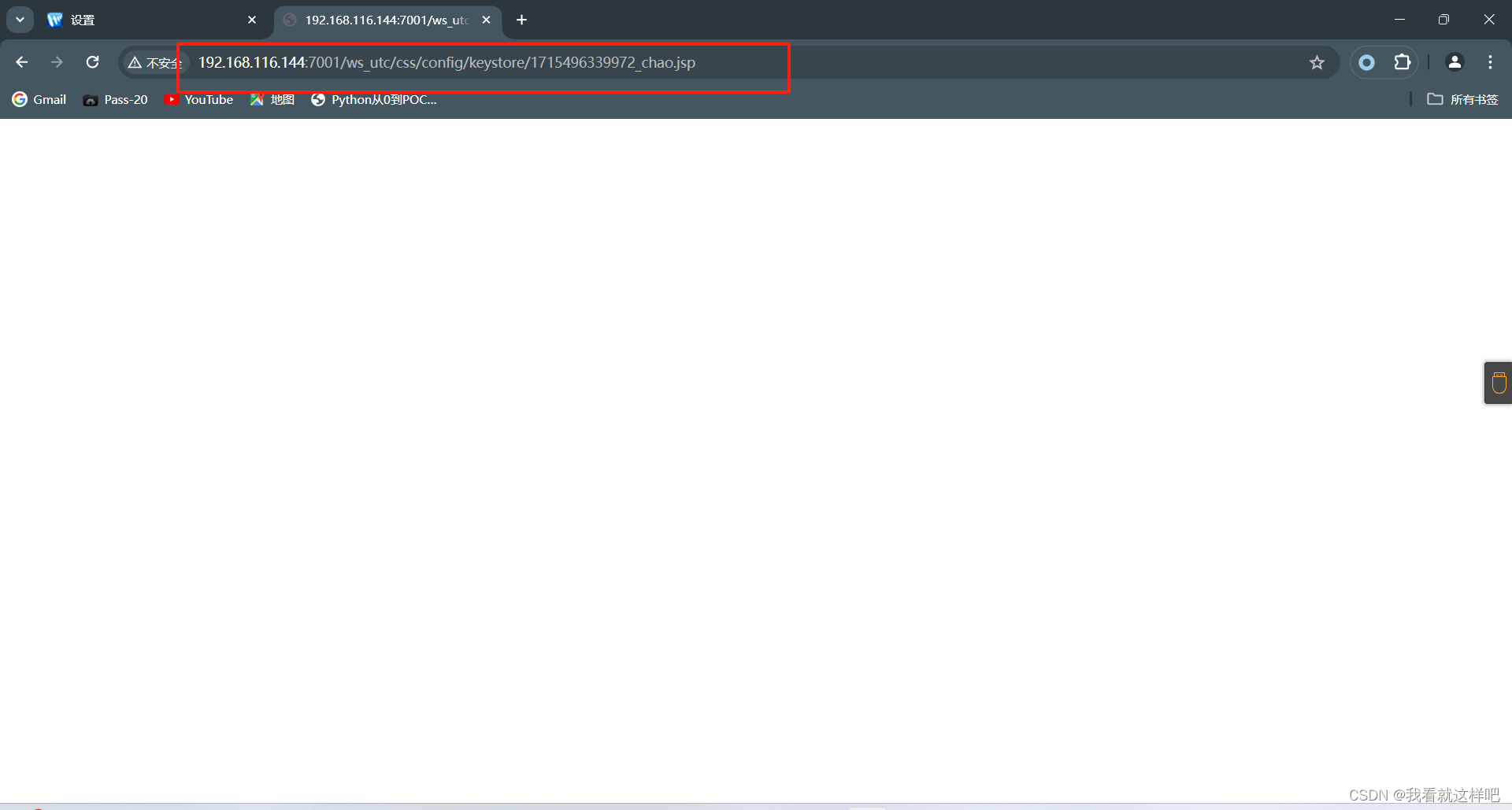
weblogic 任意文件上传 CVE-2018-2894
一、漏洞简介 在 Weblogic Web Service Test Page 中存在一处任意文件上传漏洞, Web Service Test Page 在"生产模式"下默认不开启,所以该漏洞有一定限制。利用该 漏洞,可以上传任意 jsp 文件,进而获取服务器权限。 二…...

我的第一个网页:武理天协
1. html代码 1.1 首页.html <!DOCTYPE html> <html lang"zh"> <head><meta charset"UTF-8"><title>武理天协</title><link rel"stylesheet" href"./style.css"><link rel"stylesh…...
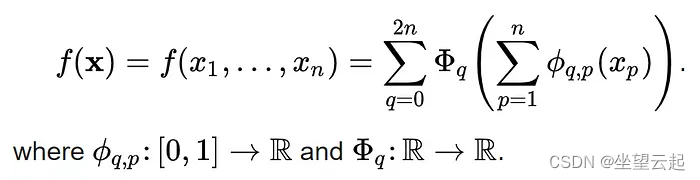
机器学习笔记 KAN网络架构简述(Kolmogorov-Arnold Networks)
一、简述 在最近的研究中,出现了号称传统多层感知器 (MLP) 的突破性替代方案,重塑了人工神经网络 (ANN) 的格局。这种创新架构被称为柯尔莫哥洛夫-阿诺德网络 (KAN),它提出了一种受柯尔莫哥洛夫-阿诺德表示定理启发的函数逼近的方法。 与 MLP 不同,MLP 依赖于各个节…...
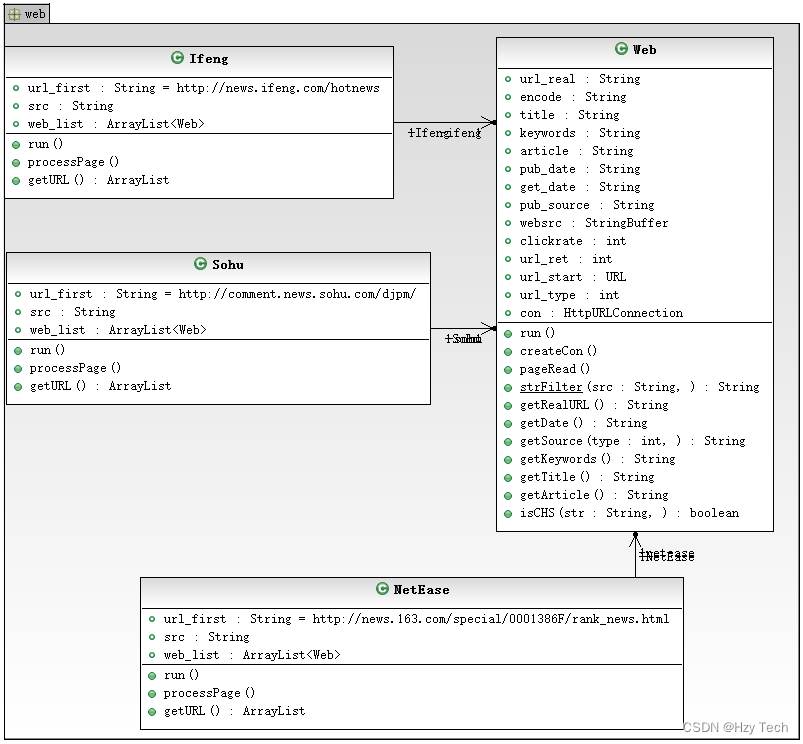
基于网络爬虫技术的网络新闻分析(二)
目录 2 系统需求分析 2.1 系统需求概述 2.2 系统需求分析 2.2.1 系统功能要求 2.2.2 系统IPO图 2.2 系统非功能性需求分析 3 系统概要设计 3.1 设计约束 3.1.1 需求约束 3.1.2 设计策略 3.1.3 技术实现 3.3 模块结构 3.3.1 模块结构图 3.3.2 系统层次图 3.3.3…...
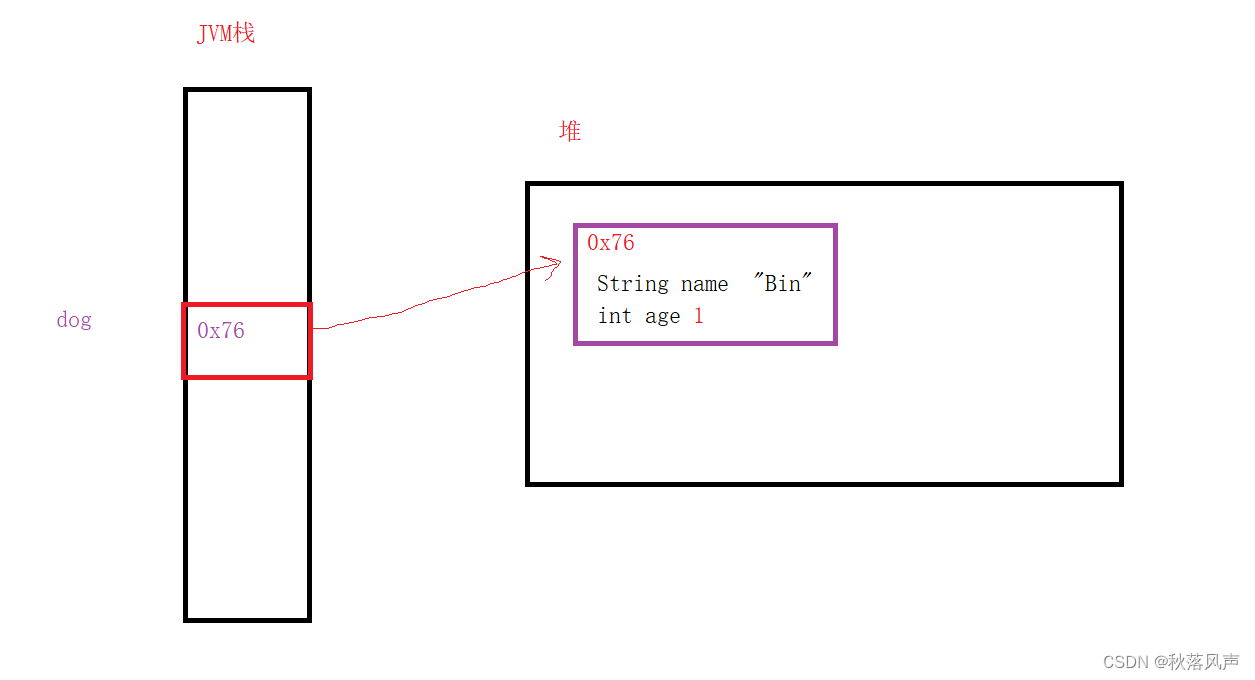
Java--初识类和对象
前言 本篇讲解Java类和对象的入门版本。 学习目的: 1.理解什么是类和对象。 2.引入面向对象程序设计的概念 3.学会如何定义类和创建对象。 4.理解this引用。 5.了解构造方法的概念并学会使用 考虑到篇幅过长问题,作者决定分多次发布。 面向对象的引入 J…...

SpringBoot如何实现动态数据源?
在Spring Boot中实现动态数据源主要涉及到创建和管理不同的数据源,并在运行时根据需要切换。这可以通过编程方式配置Spring的AbstractRoutingDataSource来完成。下面我会逐步介绍如何实现动态数据源,并给出代码示例。 第1步:添加依赖 首先&…...
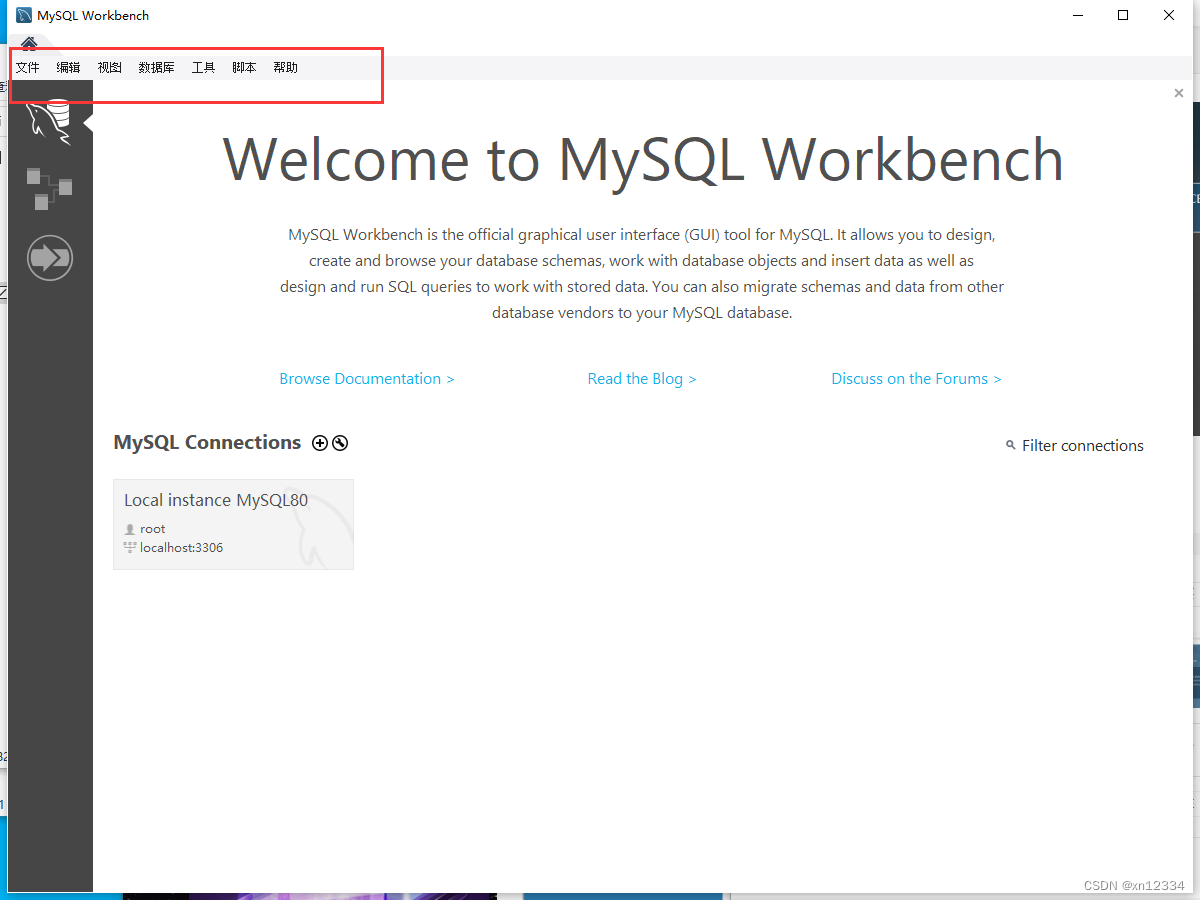
win10安装mysql8.0+汉化
一、官网安装 MySQL 1. 在mysql官网进行下载页面 2. 下滑页面,选择 MySQL community download 3.下载windows版本 4.选择第二个download 5.不用登陆,no thanks,just start my download. 6.下载 二、安装 1. 双击安装 2. 选 Full->next 3…...
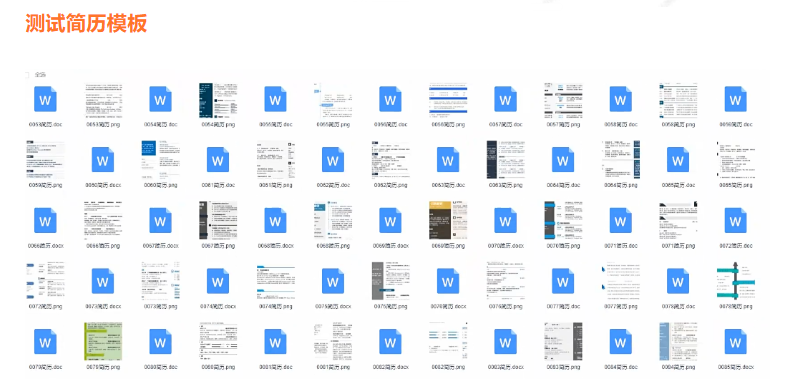
全网最全的Postman接口自动化测试!
该篇文章针对已经掌握 Postman 基本用法的读者,即对接口相关概念有一定了解、已经会使用 Postman 进行模拟请求的操作。 当前环境: Window 7 - 64 Postman 版本(免费版):Chrome App v5.5.3 不同版本页面 UI 和部分…...
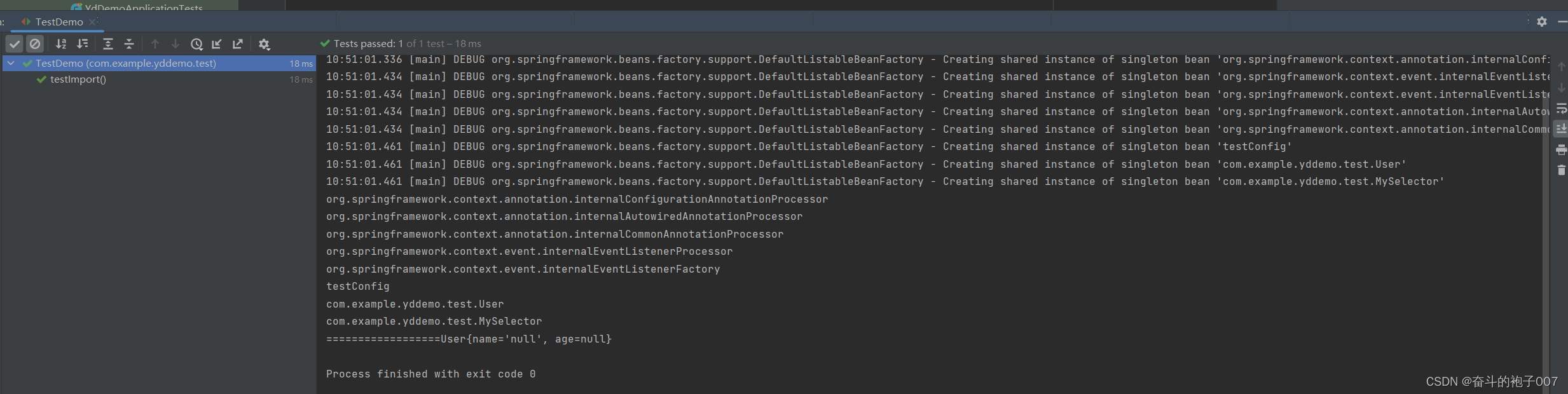
Spring:了解@Import注解的三种用法
一、前言 在 Spring 框架中,Import 注解用于导入配置类,使得你可以在一个配置类中引入另一个或多个配置类,从而实现配置的模块化。这对于组织大型应用程序的配置非常有用,因为它允许你将配置分散到多个类中,然后再将它…...
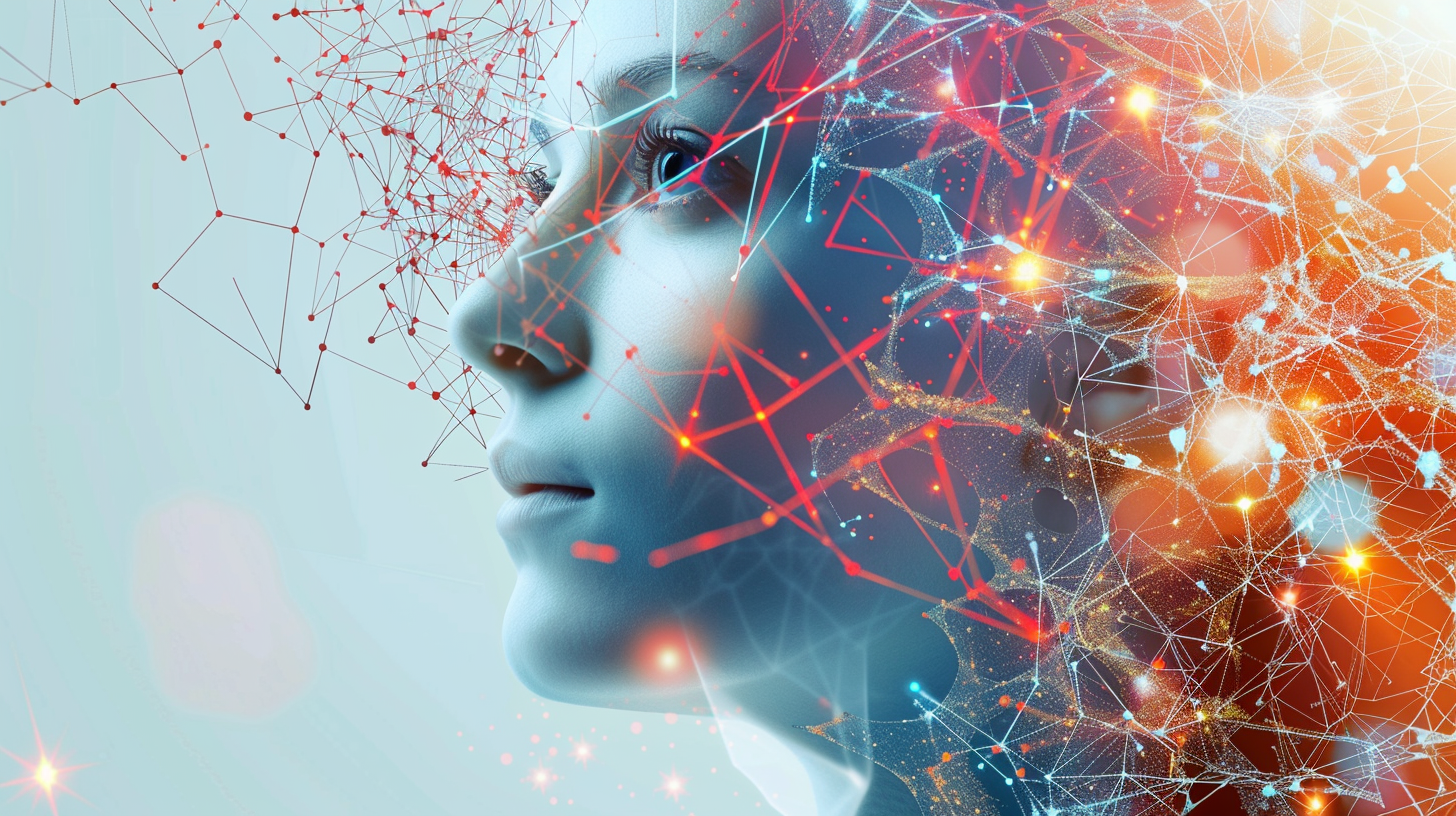
简要介绍三大脚本语言 Shell、Python 和 Lua
🍉 CSDN 叶庭云:https://yetingyun.blog.csdn.net/ 脚本语言是一种用于自动化操作系统任务和应用程序功能的编程语言。它们通常用于编写小到中等规模的程序,以提高任务执行的速度和效率。在众多脚本语言中,Shell、Python 和 Lua 是…...
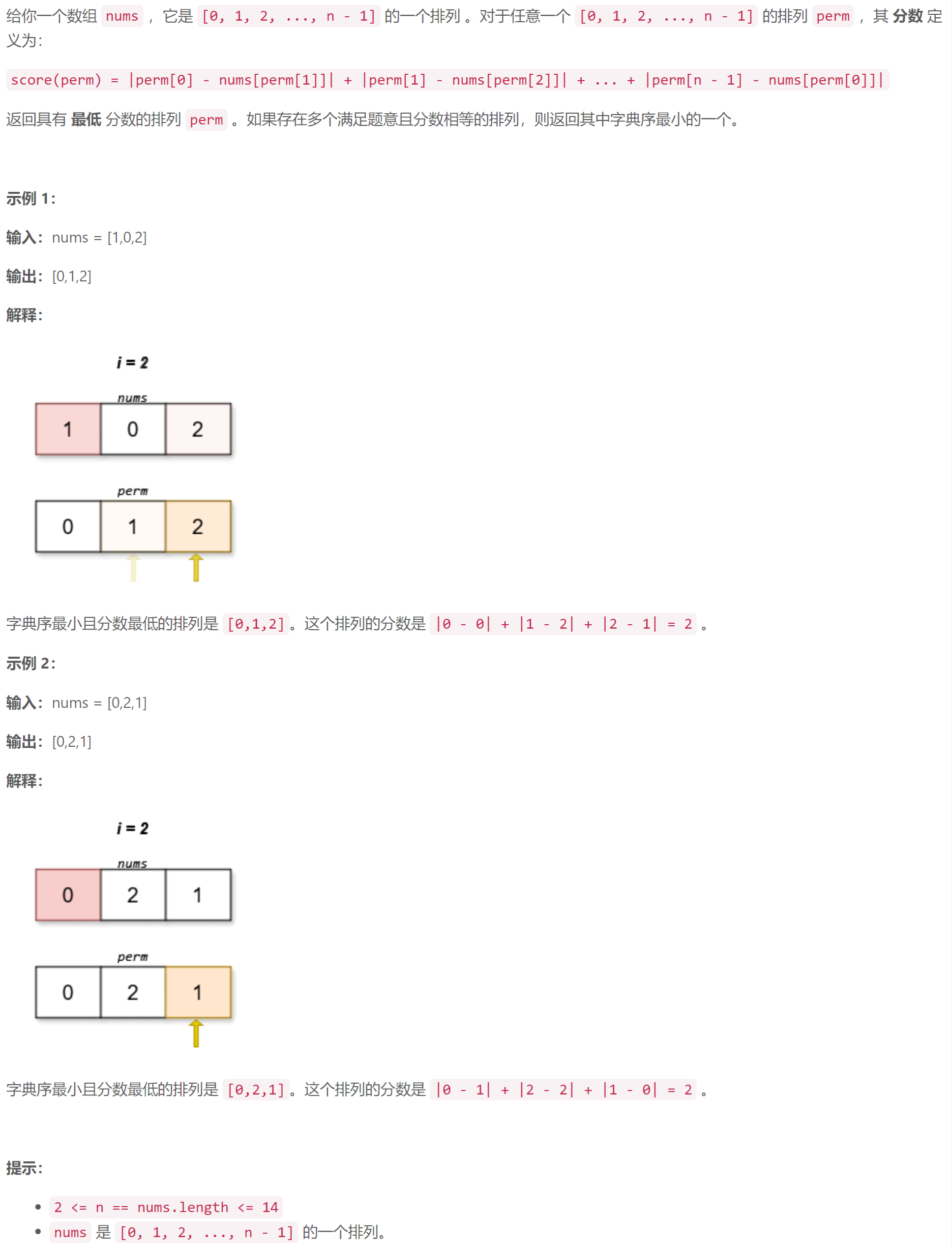
第 397 场 LeetCode 周赛题解
A 两个字符串的排列差 模拟:遍历 s s s 记录各字符出现的位置,然后遍历 t t t 计算排列差 class Solution {public:int findPermutationDifference(string s, string t) {int n s.size();vector<int> loc(26);for (int i 0; i < n; i)loc[s…...
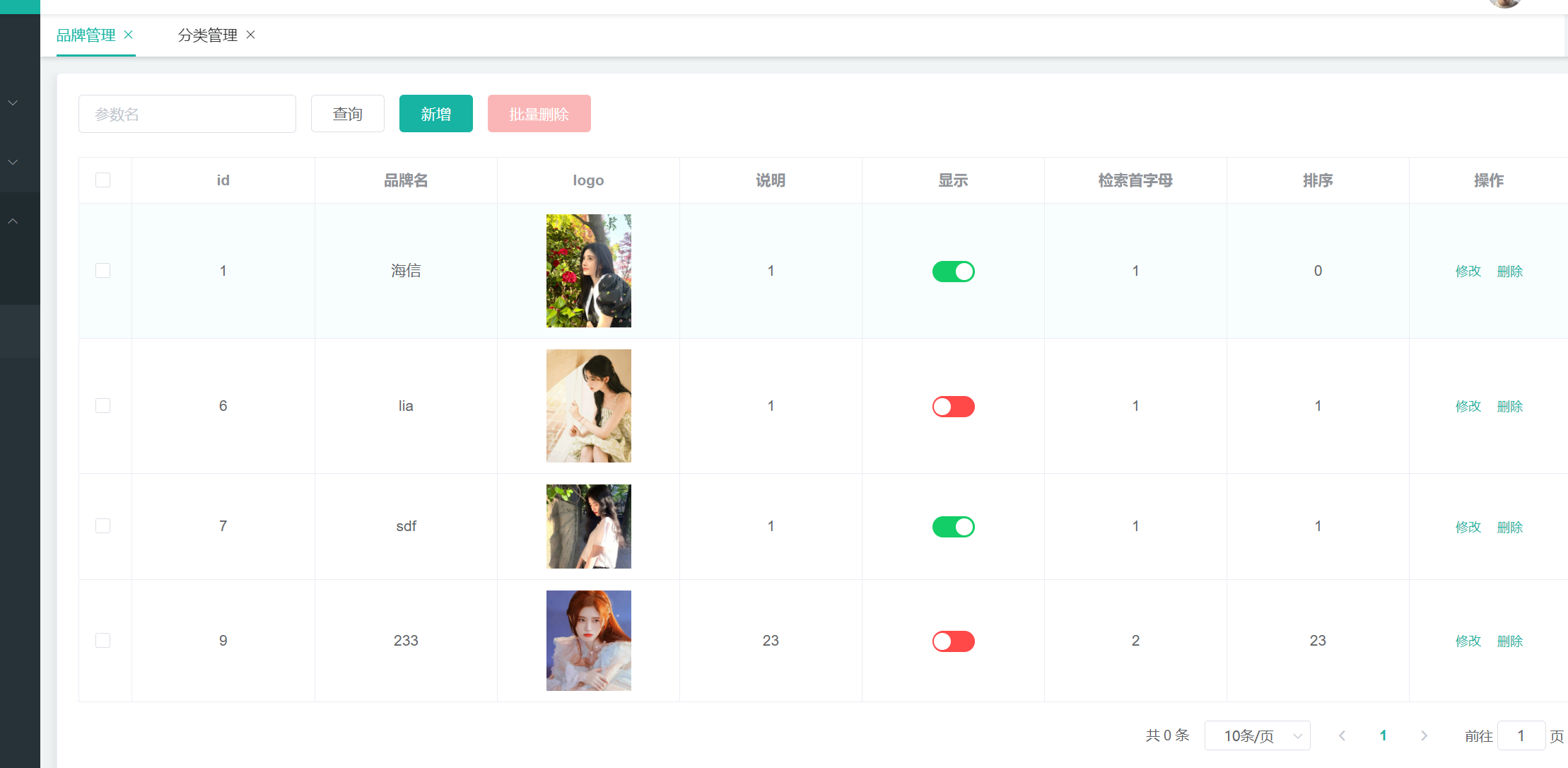
文件存储解决方案-阿里云OSS
文章目录 1.菜单分级显示问题1.问题引出1.苹果灯,放到节能灯下面也就是id大于1272.查看菜单,并没有出现苹果灯3.放到灯具下面id42,就可以显示 2.问题分析和解决1.判断可能出现问题的位置2.找到递归返回树形菜单数据的位置3.这里出现问题的原因…...
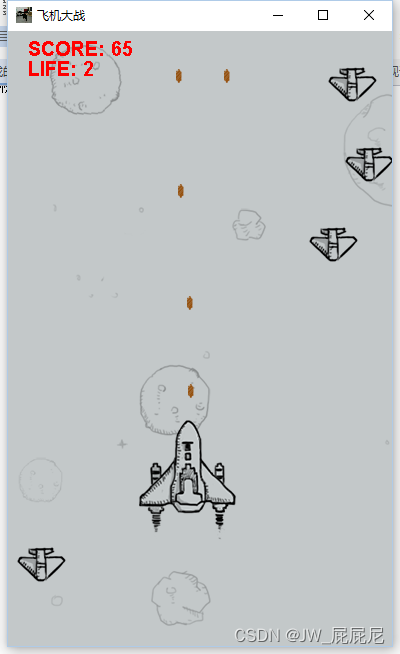
基于Java的飞机大战游戏的设计与实现(论文 + 源码)
关于基于Java的飞机大战游戏.zip资源-CSDN文库https://download.csdn.net/download/JW_559/89313362 基于Java的飞机大战游戏的设计与实现 摘 要 现如今,随着智能手机的兴起与普及,加上4G(the 4th Generation mobile communication &#x…...
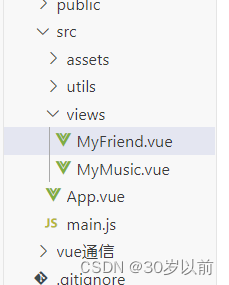
Vue路由开启步骤
1.在控制台输入命令 //控制台下载安装npm add vue-router3.6.5 2.在main.js下导入并注册组件 import Vue from vue import App from ./App.vue//控制台下载安装npm add vue-router3.6.5 //导入 import VueRouter from "vue-router";//注册 Vue.use(VueRouter) con…...

【碎片知识】2024_05_15
char int long float double运算的时候是从低转到高的,表达式的类型会自动提升或者转 换为参与表达式求值的最上级类型. 关于代码的说法正确的是( ) #include <stdio.h> int main() {int x -1;unsigned int y 2;if (x > y){printf…...
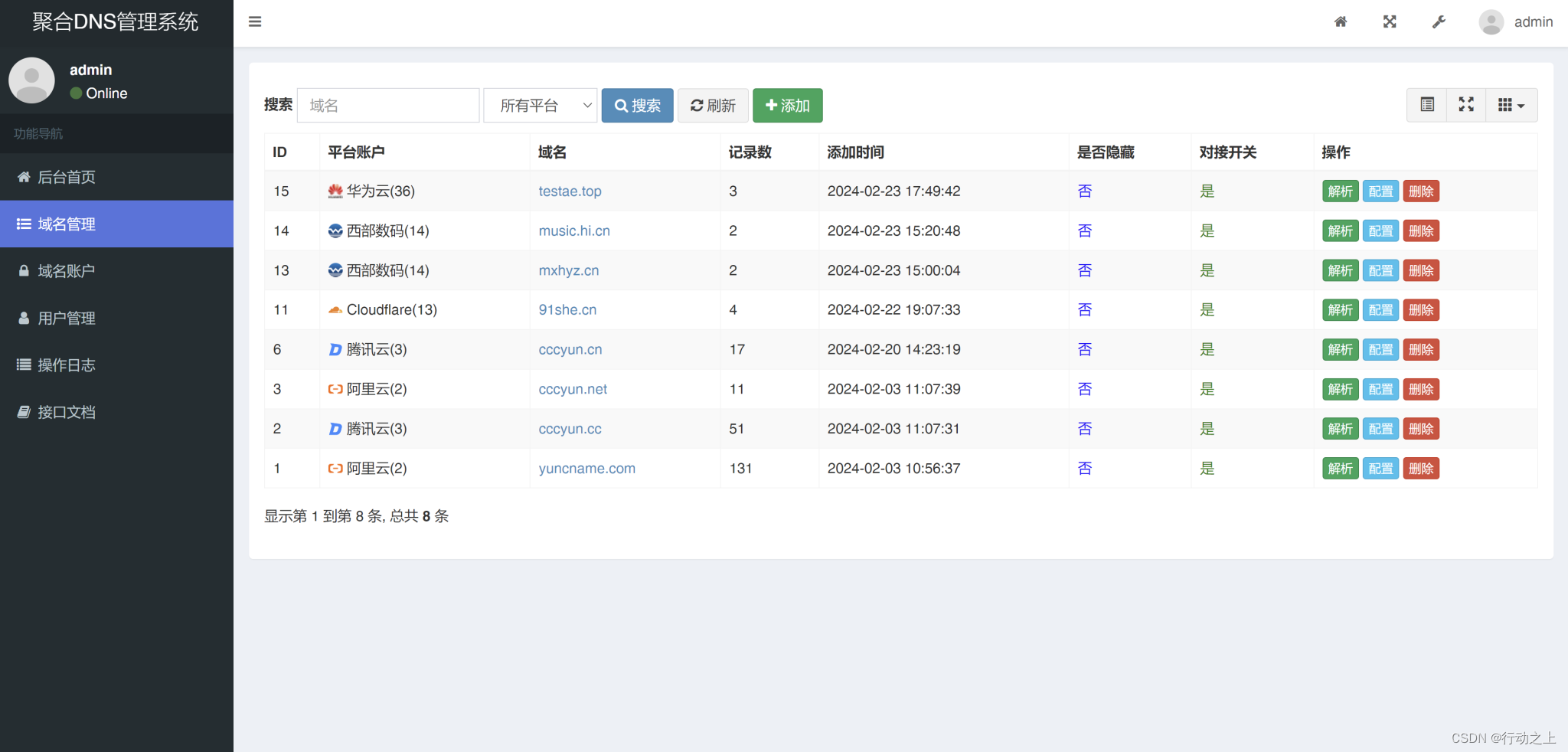
彩虹聚合DNS管理系统
聚合DNS管理系统可以实现在一个网站内管理多个平台的域名解析,目前已支持的域名平台有:阿里云、腾讯云、华为云、西部数码、CloudFlare。本系统支持多用户,每个用户可分配不同的域名解析权限;支持API接口,支持获取域名…...
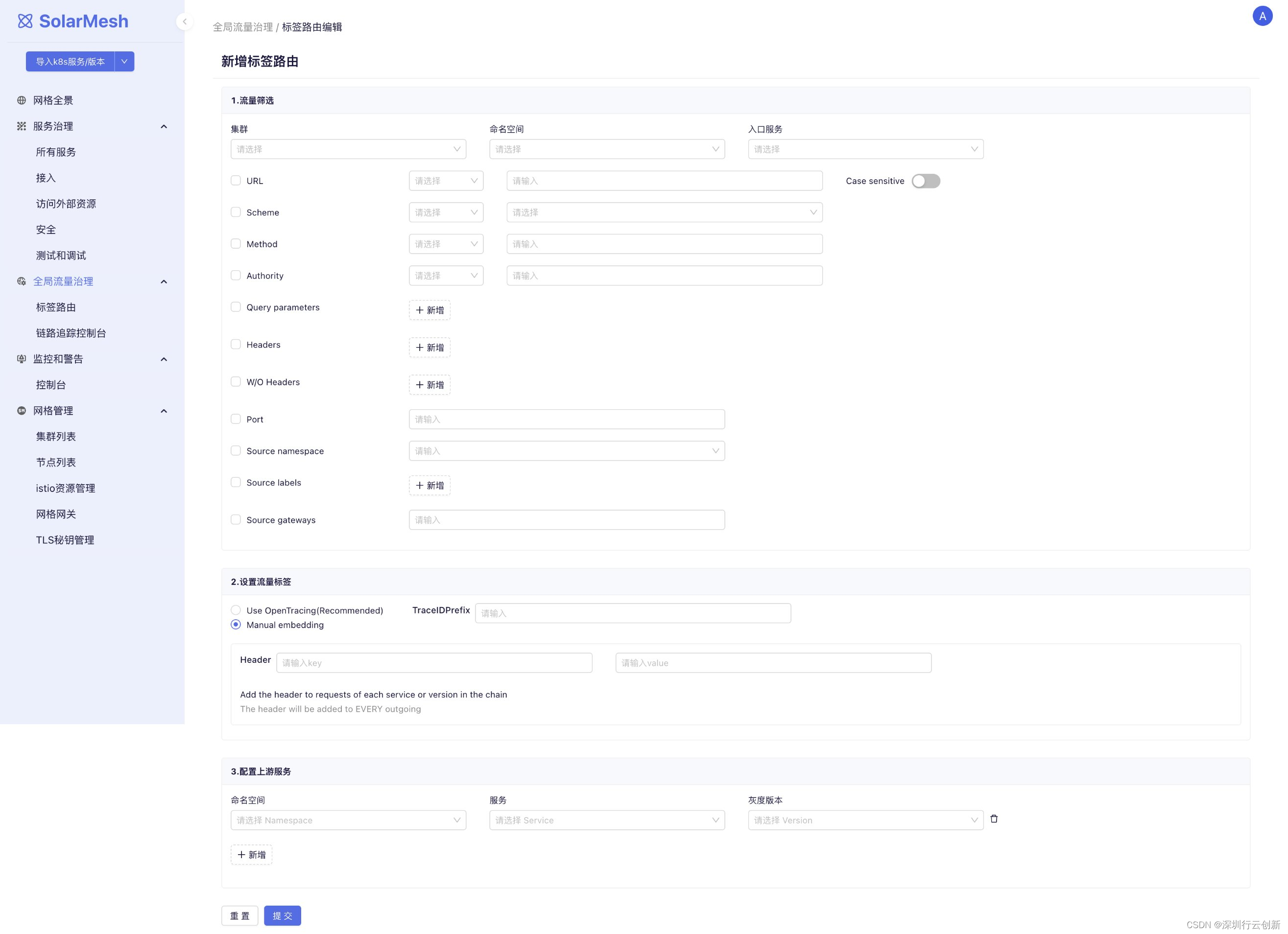
服务网格 SolarMesh v1.13 重磅发布
SolarMesh是行云创新推出的流量治理平台,它基于Istio,为部署在K8s集群上的应用提供全面的流量治理能力。 在之前的版本中,SolarMesh提供的能力有:流量视图,流量控制策略批量配置,API级别的流量数据采集和展…...
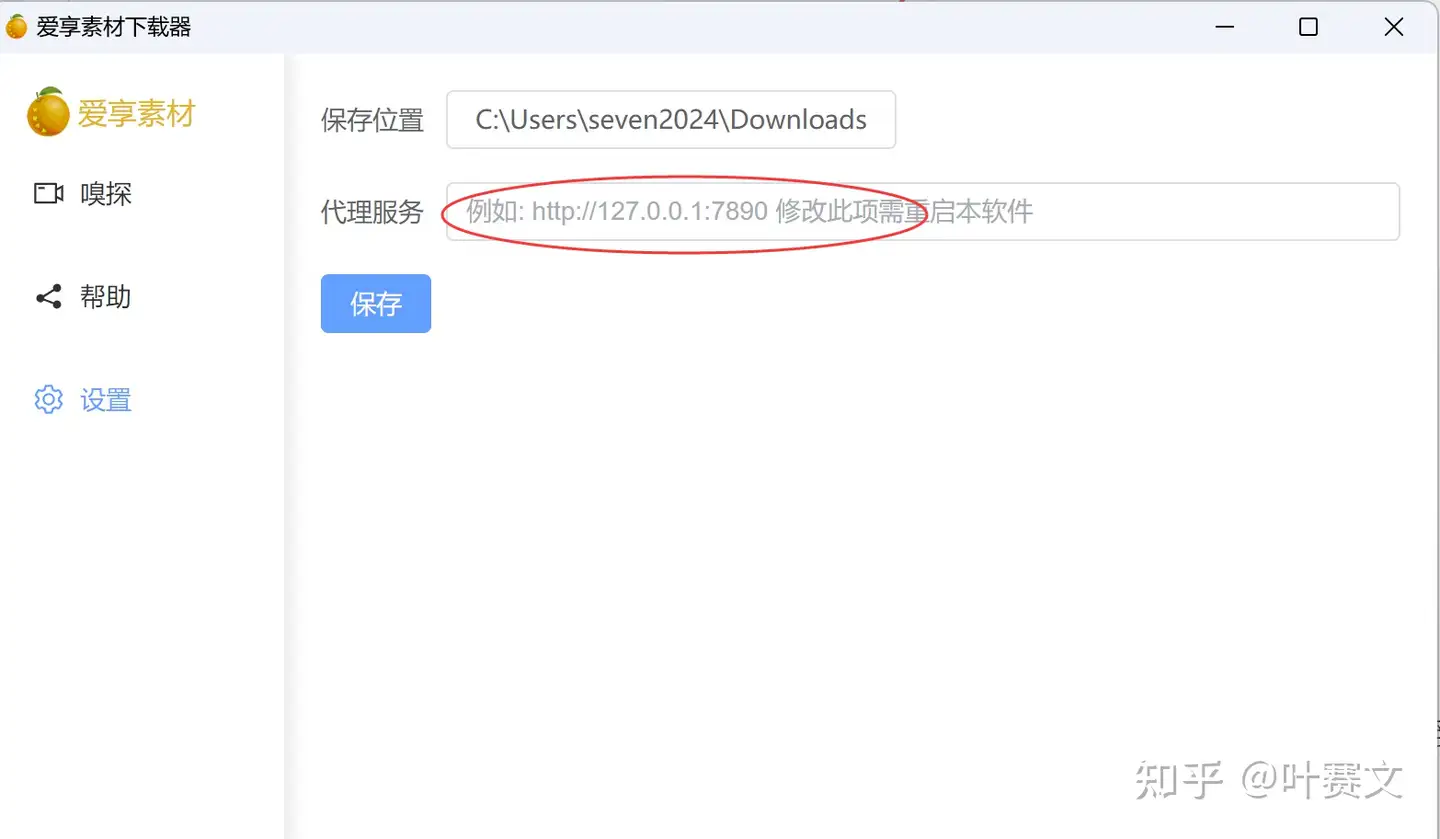
三大平台直播视频下载保存方法
终于解决了视频号下载的问题,2024年5月15日亲测可用。 而且免费。 教程第二部分,有本地电脑无法下载的解决方案。 第一部分:使用教程(正常) 第1步:下载安装包 下载迅雷网盘搜索:大海福利合集…...
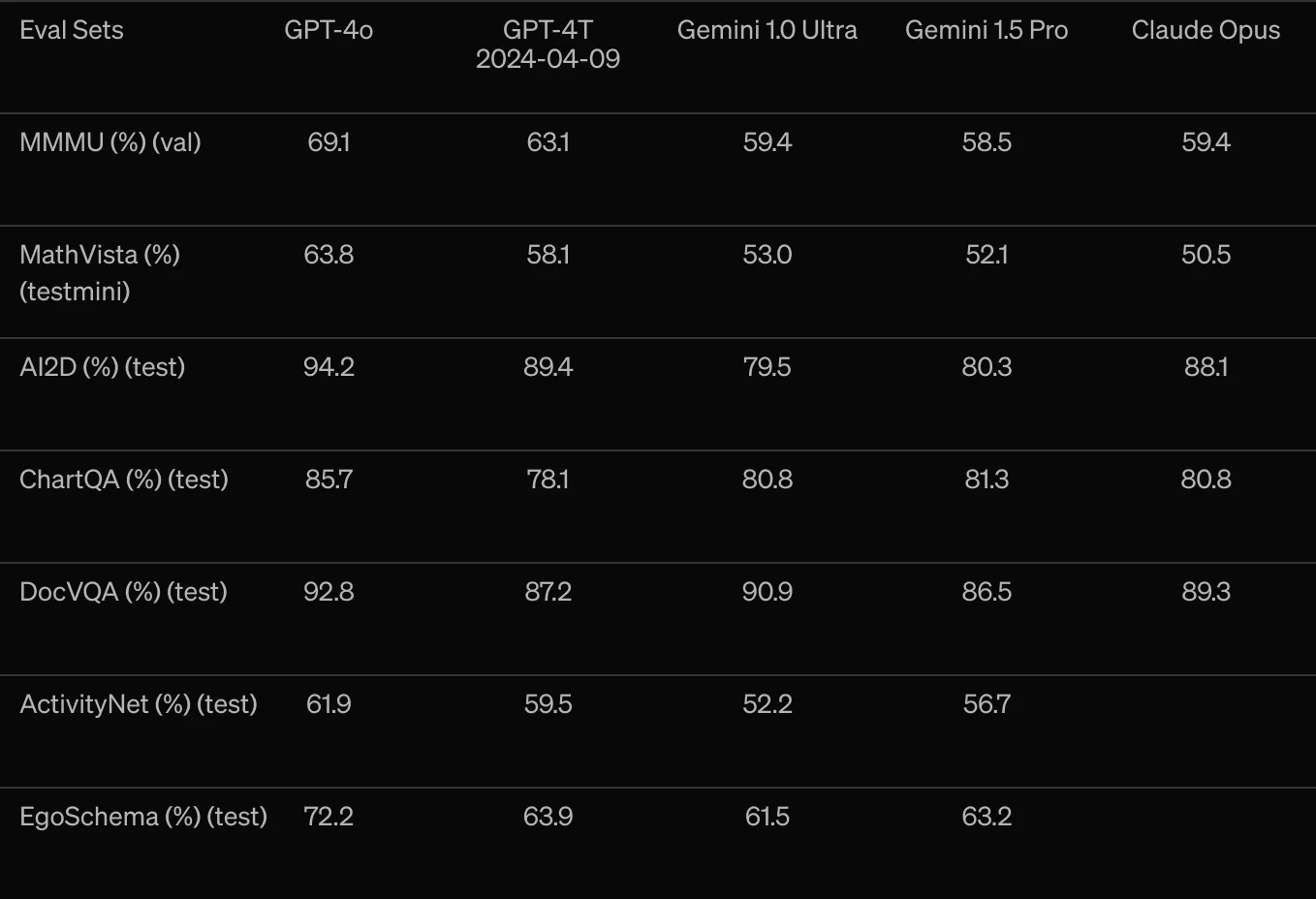
OpenAI GPT-4o - 介绍
本文翻译整理自: Hello GPT-4o https://openai.com/index/hello-gpt-4o/ 文章目录 一、关于 GPT-4o二、模型能力三、能力探索四、模型评估1、文本评价2、音频 ASR 性能3、音频翻译性能4、M3Exam 零样本结果5、视觉理解评估6、语言 tokenization 六、模型安全性和局限…...

QTreeView学习 branch 虚线设置
1、方法一: #include <QStyleFactory> ui.treeView->setStyle(QStyleFactory::create("windows")); 2、方法二: QString strtyle2 R"( QTreeView::branch:has-siblings:!adjoins-item { border-image: url(:/TreeViewDe…...
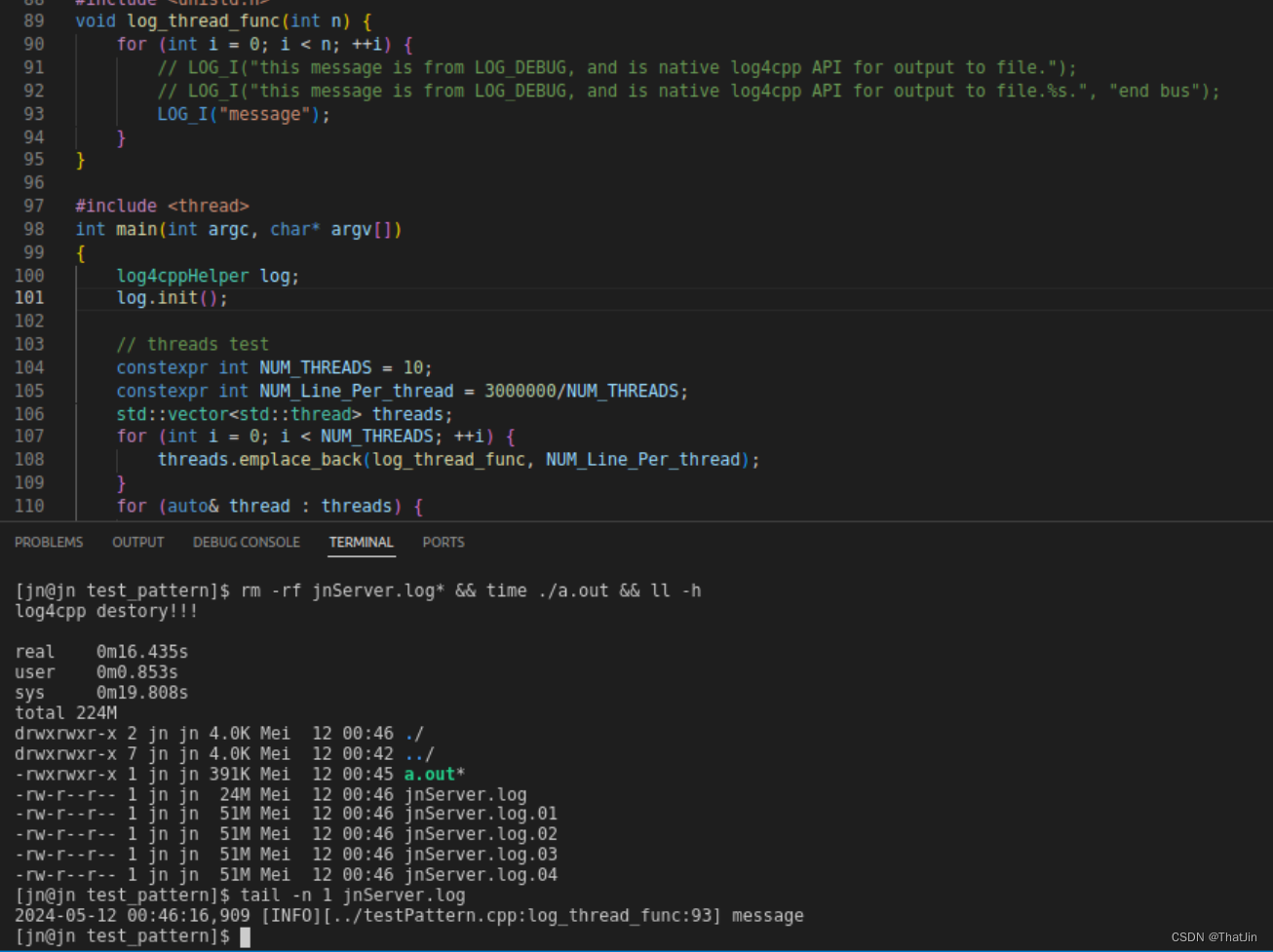
C++ 日志库 log4cpp 编译、压测及其范例代码 [全流程手工实践]
文章目录 一、 log4cpp官网二、下载三、编译1.目录结构如下2.configure 编译3.cmake 编译 四、测试五、压测源码及结果1.运行环境信息2.压测源码3.压测结果 文章内容:包含了对其linux上的完整使用流程,下载、编译、安装、测试用例尝试、以及一份自己写好…...

python数据处理与分析入门-pandas使用(4)
往期文章: pandas使用1pandas使用2pandas使用3 pandas使用技巧 创建一个DF对象 # 首先创建一个时间序列 dates pd.date_range(20180101, periods6) print(dates)# 创建DataFrame对象,指定index和columns标签 df pd.DataFrame(np.random.randn(6,4), …...

操作系统-单片机进程状态问题(三态模型问题)
例题:在单处理机计算机系统中有1台打印机、1台扫描仪,系统采用先来先服务调度算法。假设系统中有进程P1、P2、P3、P4,其中P1为运行状态,P2为就绪状态,P3等待打印机,P4等待扫描仪。此时,若P1释放…...
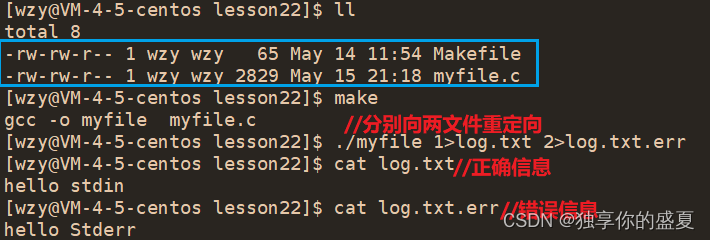
Linux文件:重定向底层实现原理(输入重定向、输出重定向、追加重定向)
Linux文件:重定向底层实现原理(输入重定向、输出重定向、追加重定向) 前言一、文件描述符fd的分配规则二、输出重定向(>)三、输出重定向底层实现原理四、追加重定向(>>)五、输入重定向…...
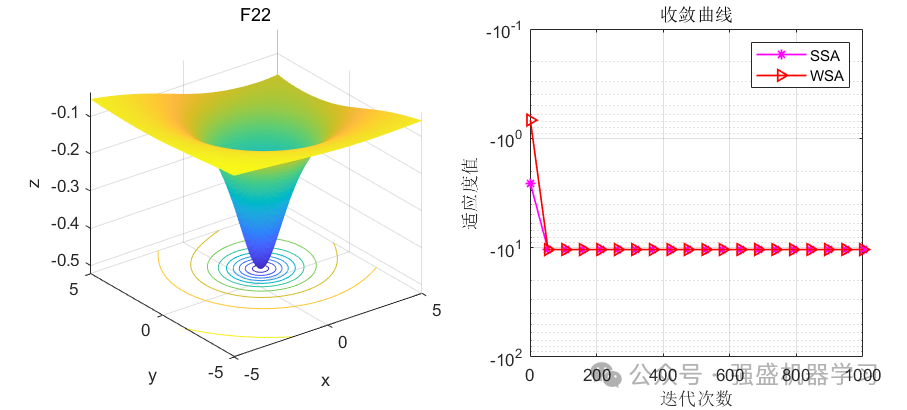
波搜索算法(WSA)-2024年SCI新算法-公式原理详解与性能测评 Matlab代码免费获取
声明:文章是从本人公众号中复制而来,因此,想最新最快了解各类智能优化算法及其改进的朋友,可关注我的公众号:强盛机器学习,不定期会有很多免费代码分享~ 目录 原理简介 一、初始化阶段 二、全…...
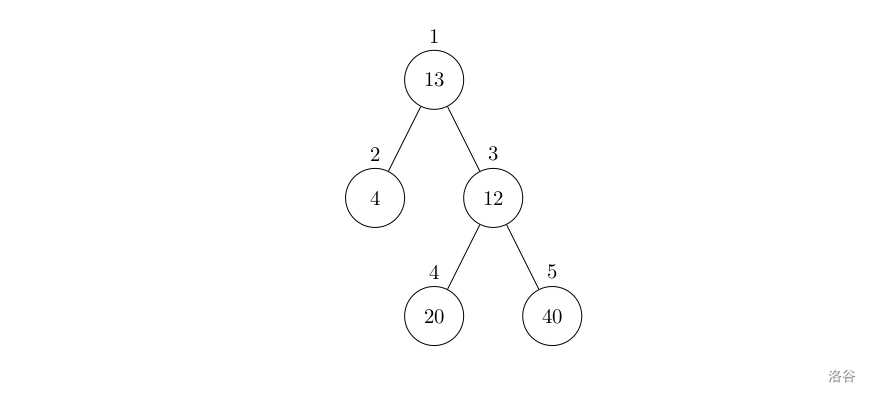
洛谷P1364 医院设置
P1364 医院设置 题目描述 设有一棵二叉树,如图: 其中,圈中的数字表示结点中居民的人口。圈边上数字表示结点编号,现在要求在某个结点上建立一个医院,使所有居民所走的路程之和为最小,同时约定,…...