Chromium 中JavaScript Fetch API接口c++代码实现(一)
Fetch API
主要暴露了三个接口一个方法。
- 三个接口
Request
(资源请求)Response
(请求的响应)Headers
(Request/Response
头部信息)
- 一个方法
fetch()
(获取资源调用的方法- 更多介绍参考 Fetch API - Web API | MDN (mozilla.org)
一、 来看一段前端代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Test fetch</title>
<script>
function Test() {fetch("http://192.168.8.1/chfs/shared/test/format.1728106021784.json").then((response) => response.json()).then((data) => console.log(data));}
</script>
</head>
<body><button onclick="Test()">Test fetch</button></body>
</html>
二、看c++代码对fetch response具体实现
1、services\network\public\mojom\fetch_api.mojom
// Copyright 2017 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.module network.mojom;// Corresponds to Fetch request's "mode" and "use-CORS-preflight flag":
// https://fetch.spec.whatwg.org/#concept-request-mode
//
// These values are persisted to logs. Entries should not be renumbered and
// numeric values should never be reused.
enum RequestMode {kSameOrigin = 0,kNoCors = 1,kCors = 2,kCorsWithForcedPreflight = 3,kNavigate = 4,// Add a new type here, then update "FetchRequestMode" in enums.xml.
};// Corresponds to Fetch request's "destination":
// https://fetch.spec.whatwg.org/#concept-request-destination
//
// These values are persisted to logs. Entries should not be renumbered and
// numeric values should never be reused.
enum RequestDestination {kEmpty = 0,kAudio = 1,kAudioWorklet = 2,// kDocument is for a main resource request in a main frame, or a Portal.kDocument = 3,kEmbed = 4,kFont = 5,kFrame = 6,kIframe = 7,kImage = 8,kManifest = 9,kObject = 10,kPaintWorklet = 11,kReport = 12,kScript = 13,kServiceWorker = 14,kSharedWorker = 15,kStyle = 16,kTrack = 17,kVideo = 18,// kWebBundle represents a request for a WebBundle. A <script> element whose// type is "webbundle" uses this destination.//// e.g. <script type=webbundle> { "source": "foo.wbn", ... } </script>//// Fetch specifiction does not define this destination yet.// Tracking issue: https://github.com/whatwg/fetch/issues/1120kWebBundle = 19,kWorker = 20,kXslt = 21,// kFencedframe represents a main resource request in a fenced frame. A// <fencedframe> element uses this destination.//// e.g. <fencedframe src="example.com"></fencedframe>//// Fenced Frame is not standardized yet. See// https://github.com/shivanigithub/fenced-frame for the explainer and// crbug.com/1123606 for the implementation.kFencedframe = 22,// Requests from the federated credential management API,// https://fedidcg.github.io/FedCM/kWebIdentity = 23,// Requests for compression dictionarykDictionary = 24,// Requests for speculation rules.// https://wicg.github.io/nav-speculation/speculation-rules.htmlkSpeculationRules = 25,
};// Corresponds to Fetch request's "redirect mode":
// https://fetch.spec.whatwg.org/#concept-request-redirect-mode
enum RedirectMode {kFollow,kError,kManual,
};// Corresponds to Fetch request's "credentials mode":
// https://fetch.spec.whatwg.org/#concept-request-credentials-mode
enum CredentialsMode {// TODO(https://crbug.com/775438): Due to a bug, this does not properly// correspond to Fetch's "credentials mode", in that client certificates will// be sent is available, or the handshake will be aborted in order to allow// selecting a client cert. The correct behavior is to omit all client certs// and continue the handshake without sending one if requested.kOmit,kSameOrigin,kInclude,// TODO(https://crbug.com/775438): This works around kOmit not doing the// spec-defined behavior. This is a temporary workaround that explicitly// indicates the caller wants the spec-defined behavior. It's named as such// because this should be only temporary, until kOmit is fixed.kOmitBug_775438_Workaround
};// Corresponds to response types from the Fetch spec:
// https://fetch.spec.whatwg.org/#concept-response-type
//
// These values are persisted to logs. Entries should not be renumbered and
// numeric values should never be reused.
enum FetchResponseType {kBasic = 0,kCors = 1,kDefault = 2,kError = 3,kOpaque = 4,kOpaqueRedirect = 5,// Add a new type here, then update "FetchResponseType" in enums.xml.
};// Indicates the source of a response.
// This represents the source of the outmost response of a request.
// This is used only for histograms and isn't web-exposed.
enum FetchResponseSource {// The source is unspecified: e.g. "new Response('hi')" or a response from// a service worker.kUnspecified,// The response came from network: e.g. "fetch(req)".kNetwork,// The response came from HttpCache: e.g. "fetch(req)" and there is an entry// in HttpCache.kHttpCache,// The response came from CacheStorage: e.g. "cache.match(req)" in a fetch// event handler.kCacheStorage,
};
2、在third_party\blink\renderer\core\fetch\global_fetch.h 定义了Fetch函数具体实现
// Copyright 2014 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.#ifndef THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_GLOBAL_FETCH_H_
#define THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_GLOBAL_FETCH_H_#include "third_party/blink/renderer/bindings/core/v8/script_promise.h"
#include "third_party/blink/renderer/bindings/core/v8/v8_typedefs.h"
#include "third_party/blink/renderer/core/core_export.h"
#include "third_party/blink/renderer/core/fetch/request.h"namespace blink {class ExceptionState;
class LocalDOMWindow;
class NavigatorBase;
class RequestInit;
class DeferredRequestInit;
class ScriptState;
class WorkerGlobalScope;
class FetchLaterResult;class CORE_EXPORT GlobalFetch {STATIC_ONLY(GlobalFetch);public:class CORE_EXPORT ScopedFetcher : public GarbageCollectedMixin {public:virtual ~ScopedFetcher();virtual ScriptPromise Fetch(ScriptState*,const V8RequestInfo*,const RequestInit*,ExceptionState&) = 0;virtual FetchLaterResult* FetchLater(ScriptState*,const V8RequestInfo*,const DeferredRequestInit*,ExceptionState&);// Returns the number of fetch() method calls in the associated execution// context. This is used for metrics.virtual uint32_t FetchCount() const = 0;static ScopedFetcher* From(LocalDOMWindow&);static ScopedFetcher* From(WorkerGlobalScope&);static ScopedFetcher* From(NavigatorBase& navigator);void Trace(Visitor*) const override;};static ScriptPromise fetch(ScriptState* script_state,LocalDOMWindow& window,const V8RequestInfo* input,const RequestInit* init,ExceptionState& exception_state);static ScriptPromise fetch(ScriptState* script_state,WorkerGlobalScope& worker,const V8RequestInfo* input,const RequestInit* init,ExceptionState& exception_state);static FetchLaterResult* fetchLater(ScriptState* script_state,LocalDOMWindow& window,const V8RequestInfo* input,const DeferredRequestInit* init,ExceptionState& exception_state);
};} // namespace blink#endif // THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_GLOBAL_FETCH_H_
3、Fetch 管理类 third_party\blink\renderer\core\fetch\fetch_manager.h
4、前端接口 Request
(资源请求) third_party\blink\renderer\core\fetch\headers.idl
// Copyright 2014 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.// https://fetch.spec.whatwg.org/#typedefdef-headersinittypedef (sequence<sequence<ByteString>> or record<ByteString, ByteString>) HeadersInit;// https://fetch.spec.whatwg.org/#headers-class[Exposed=(Window,Worker)
] interface Headers {[CallWith=ScriptState, RaisesException] constructor(optional HeadersInit init);[CallWith=ScriptState, RaisesException] void append(ByteString name, ByteString value);[CallWith=ScriptState, ImplementedAs=remove, RaisesException] void delete(ByteString key);[RaisesException] ByteString? get(ByteString key);sequence<ByteString> getSetCookie();[RaisesException] boolean has(ByteString key);[CallWith=ScriptState, RaisesException] void set(ByteString key, ByteString value);iterable<ByteString, ByteString>;
};
对应实现c++类:
third_party\blink\renderer\core\fetch\headers.h
third_party\blink\renderer\core\fetch\headers.cc
// Copyright 2014 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.#ifndef THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_HEADERS_H_
#define THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_HEADERS_H_#include "third_party/blink/renderer/bindings/core/v8/iterable.h"
#include "third_party/blink/renderer/bindings/core/v8/v8_sync_iterator_headers.h"
#include "third_party/blink/renderer/bindings/core/v8/v8_typedefs.h"
#include "third_party/blink/renderer/core/core_export.h"
#include "third_party/blink/renderer/core/fetch/fetch_header_list.h"
#include "third_party/blink/renderer/platform/bindings/script_wrappable.h"
#include "third_party/blink/renderer/platform/heap/collection_support/heap_hash_set.h"
#include "third_party/blink/renderer/platform/wtf/forward.h"namespace blink {class ExceptionState;
class ScriptState;// http://fetch.spec.whatwg.org/#headers-class
class CORE_EXPORT Headers final : public ScriptWrappable,public PairSyncIterable<Headers> {DEFINE_WRAPPERTYPEINFO();public:enum Guard {kImmutableGuard,kRequestGuard,kRequestNoCorsGuard,kResponseGuard,kNoneGuard};static Headers* Create(ScriptState* script_state,ExceptionState& exception_state);static Headers* Create(ScriptState* script_state,const V8HeadersInit* init,ExceptionState& exception_state);// Shares the FetchHeaderList. Called when creating a Request or Response.static Headers* Create(FetchHeaderList*);Headers();// Shares the FetchHeaderList. Called when creating a Request or Response.explicit Headers(FetchHeaderList*);Headers* Clone() const;// Headers.idl implementation.void append(ScriptState* script_state,const String& name,const String& value,ExceptionState&);void remove(ScriptState* script_state, const String& key, ExceptionState&);String get(const String& key, ExceptionState&);Vector<String> getSetCookie();bool has(const String& key, ExceptionState&);void set(ScriptState* script_state,const String& key,const String& value,ExceptionState&);void SetGuard(Guard guard) { guard_ = guard; }Guard GetGuard() const { return guard_; }// These methods should only be called when size() would return 0.void FillWith(ScriptState* script_state, const Headers*, ExceptionState&);void FillWith(ScriptState* script_state,const V8HeadersInit* init,ExceptionState& exception_state);// https://fetch.spec.whatwg.org/#concept-headers-remove-privileged-no-cors-request-headersvoid RemovePrivilegedNoCorsRequestHeaders();FetchHeaderList* HeaderList() const { return header_list_.Get(); }void Trace(Visitor*) const override;private:class HeadersIterationSource final: public PairSyncIterable<Headers>::IterationSource {public:explicit HeadersIterationSource(Headers* headers);~HeadersIterationSource() override;bool FetchNextItem(ScriptState* script_state,String& key,String& value,ExceptionState& exception) override;void Trace(Visitor*) const override;void ResetHeaderList();private:// https://webidl.spec.whatwg.org/#dfn-value-pairs-to-iterate-overVector<std::pair<String, String>> headers_list_;// https://webidl.spec.whatwg.org/#default-iterator-object-indexwtf_size_t current_ = 0;Member<Headers> headers_;};// These methods should only be called when size() would return 0.void FillWith(ScriptState* script_state,const Vector<Vector<String>>&,ExceptionState&);void FillWith(ScriptState* script_state,const Vector<std::pair<String, String>>&,ExceptionState&);Member<FetchHeaderList> header_list_;Guard guard_;IterationSource* CreateIterationSource(ScriptState*,ExceptionState&) override;HeapHashSet<WeakMember<HeadersIterationSource>> iterators_;
};} // namespace blink#endif // THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_HEADERS_H_
5、Response
(请求的响应) third_party\blink\renderer\core\fetch\response.idl
// Copyright 2014 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.// https://fetch.spec.whatwg.org/#response-classenum ResponseType { "basic", "cors", "default", "error", "opaque", "opaqueredirect" };[Exposed=(Window,Worker)
] interface Response {// TODO(yhirano): We use "any" for body because the IDL processor doesn't// recognize ReadableStream implemented with V8 extras. Fix it.[CallWith=ScriptState, RaisesException] constructor(optional any body, optional ResponseInit init = {});[CallWith=ScriptState, NewObject] static Response error();[CallWith=ScriptState, NewObject, RaisesException] static Response redirect(USVString url, optional unsigned short status = 302);[CallWith=ScriptState, NewObject, RaisesException, ImplementedAs=staticJson] static Response json(any data, optional ResponseInit init = {});readonly attribute ResponseType type;readonly attribute USVString url;readonly attribute boolean redirected;readonly attribute unsigned short status;readonly attribute boolean ok;readonly attribute ByteString statusText;[SameObject] readonly attribute Headers headers;[RaisesException, CallWith=ScriptState, NewObject] Response clone();[Affects=Everything, MeasureAs=FetchBodyStream] readonly attribute ReadableStream? body;
};Response includes Body;
对应response接口c++实现类:
third_party\blink\renderer\core\fetch\response.h
third_party\blink\renderer\core\fetch\response.cc
// Copyright 2014 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.#ifndef THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_RESPONSE_H_
#define THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_RESPONSE_H_#include "services/network/public/mojom/fetch_api.mojom-blink.h"
#include "third_party/blink/public/mojom/fetch/fetch_api_response.mojom-blink-forward.h"
#include "third_party/blink/renderer/bindings/core/v8/dictionary.h"
#include "third_party/blink/renderer/bindings/core/v8/script_value.h"
#include "third_party/blink/renderer/core/core_export.h"
#include "third_party/blink/renderer/core/fetch/body.h"
#include "third_party/blink/renderer/core/fetch/body_stream_buffer.h"
#include "third_party/blink/renderer/core/fetch/fetch_response_data.h"
#include "third_party/blink/renderer/core/fetch/headers.h"
#include "third_party/blink/renderer/platform/bindings/script_wrappable.h"
#include "third_party/blink/renderer/platform/blob/blob_data.h"
#include "third_party/blink/renderer/platform/heap/garbage_collected.h"
#include "third_party/blink/renderer/platform/wtf/text/wtf_string.h"
#include "third_party/blink/renderer/platform/wtf/vector.h"namespace blink {class ExceptionState;
class ResponseInit;
class ScriptState;class CORE_EXPORT Response final : public ScriptWrappable, public Body {DEFINE_WRAPPERTYPEINFO();public:// These "create" function which takes a ScriptState* must be called with// entering an appropriate V8 context.// From Response.idl:static Response* Create(ScriptState*, ExceptionState&);static Response* Create(ScriptState*,ScriptValue body,const ResponseInit*,ExceptionState&);static Response* Create(ScriptState*,BodyStreamBuffer*,const String& content_type,const ResponseInit*,ExceptionState&);static Response* Create(ExecutionContext*, FetchResponseData*);static Response* Create(ScriptState*, mojom::blink::FetchAPIResponse&);static Response* CreateClone(const Response&);static Response* error(ScriptState*);static Response* redirect(ScriptState*,const String& url,uint16_t status,ExceptionState&);static Response* staticJson(ScriptState*,ScriptValue data,const ResponseInit*,ExceptionState&);static FetchResponseData* CreateUnfilteredFetchResponseDataWithoutBody(ScriptState*,mojom::blink::FetchAPIResponse&);static FetchResponseData* FilterResponseData(network::mojom::FetchResponseType response_type,FetchResponseData* response,WTF::Vector<WTF::String>& headers);explicit Response(ExecutionContext*);Response(ExecutionContext*, FetchResponseData*);Response(ExecutionContext*, FetchResponseData*, Headers*);Response(const Response&) = delete;Response& operator=(const Response&) = delete;const FetchResponseData* GetResponse() const { return response_.Get(); }// From Response.idl:String type() const;String url() const;bool redirected() const;uint16_t status() const;bool ok() const;String statusText() const;Headers* headers() const;// From Response.idl:// This function must be called with entering an appropriate V8 context.Response* clone(ScriptState*, ExceptionState&);// Does not contain the blob response body or any side data blob.// |request_url| is the current request URL that resulted in the response. It// is needed to process some response headers (e.g. CSP).// TODO(lfg, kinuko): The FetchResponseData::url_list_ should include the// request URL per step 9 in Main Fetch// https://fetch.spec.whatwg.org/#main-fetch. Just fixing it might break the// logic in ResourceMultiBufferDataProvider, please see// https://chromium-review.googlesource.com/c/1366464 for more details.mojom::blink::FetchAPIResponsePtr PopulateFetchAPIResponse(const KURL& request_url);bool HasBody() const;BodyStreamBuffer* BodyBuffer() override { return response_->Buffer(); }// Returns the BodyStreamBuffer of |m_response|. This method doesn't check// the internal response of |m_response| even if |m_response| has it.const BodyStreamBuffer* BodyBuffer() const override {return response_->Buffer();}// Returns the BodyStreamBuffer of the internal response of |m_response| if// any. Otherwise, returns one of |m_response|.BodyStreamBuffer* InternalBodyBuffer() { return response_->InternalBuffer(); }const BodyStreamBuffer* InternalBodyBuffer() const {return response_->InternalBuffer();}bool IsBodyUsed() const override;String ContentType() const override;String MimeType() const override;String InternalMIMEType() const;const Vector<KURL>& InternalURLList() const;FetchHeaderList* InternalHeaderList() const;void Trace(Visitor*) const override;private:const Member<FetchResponseData> response_;const Member<Headers> headers_;
};} // namespace blink#endif // THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_RESPONSE_H_
1)、 Response body对应实现类:third_party\blink\renderer\core\fetch\body.idl
// Copyright 2014 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.// https://fetch.spec.whatwg.org/#body[ActiveScriptWrappable
] interface mixin Body {readonly attribute boolean bodyUsed;[CallWith=ScriptState, NewObject, RaisesException] Promise<ArrayBuffer> arrayBuffer();[CallWith=ScriptState, NewObject, RaisesException] Promise<Blob> blob();[CallWith=ScriptState, NewObject, RaisesException] Promise<FormData> formData();[CallWith=ScriptState, NewObject, RaisesException] Promise<any> json();[CallWith=ScriptState, NewObject, RaisesException] Promise<USVString> text();// body attribute is defined in sub-interfaces, because the IDL processor// cannot deal with attribute inheritance with runtime enabled flag.// [RuntimeEnabled=ExperimentalStream] readonly attribute ReadableByteStream body;
};
third_party\blink\renderer\core\fetch\body.h
third_party\blink\renderer\core\fetch\body.cc
// Copyright 2014 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.#ifndef THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_BODY_H_
#define THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_BODY_H_#include "third_party/blink/renderer/bindings/core/v8/script_promise.h"
#include "third_party/blink/renderer/bindings/core/v8/script_value.h"
#include "third_party/blink/renderer/core/core_export.h"
#include "third_party/blink/renderer/core/execution_context/execution_context_lifecycle_observer.h"
#include "third_party/blink/renderer/platform/bindings/script_wrappable.h"
#include "third_party/blink/renderer/platform/wtf/text/wtf_string.h"namespace blink {class BodyStreamBuffer;
class ExceptionState;
class ExecutionContext;
class ReadableStream;
class ScriptPromiseResolver;
class ScriptState;// This class represents Body mix-in defined in the fetch spec
// https://fetch.spec.whatwg.org/#body-mixin.
//
// Note: This class has body stream and its predicate whereas in the current
// spec only Response has it and Request has a byte stream defined in the
// Encoding spec. The spec should be fixed shortly to be aligned with this
// implementation.
class CORE_EXPORT Body : public ExecutionContextClient {public:explicit Body(ExecutionContext*);Body(const Body&) = delete;Body& operator=(const Body&) = delete;ScriptPromise arrayBuffer(ScriptState*, ExceptionState&);ScriptPromise blob(ScriptState*, ExceptionState&);ScriptPromise formData(ScriptState*, ExceptionState&);ScriptPromise json(ScriptState*, ExceptionState&);ScriptPromise text(ScriptState*, ExceptionState&);ReadableStream* body();virtual BodyStreamBuffer* BodyBuffer() = 0;virtual const BodyStreamBuffer* BodyBuffer() const = 0;// This should only be called from the generated bindings. All other code// should use IsBodyUsed() instead.bool bodyUsed() const { return IsBodyUsed(); }// True if the body has been read from.virtual bool IsBodyUsed() const;// True if the body is locked.bool IsBodyLocked() const;private:// TODO(e_hakkinen): Fix |MimeType()| to always contain parameters and// remove |ContentType()|.virtual String ContentType() const = 0;virtual String MimeType() const = 0;// Body consumption algorithms will reject with a TypeError in a number of// error conditions. This method wraps those up into one call which throws// an exception if consumption cannot proceed. The caller must check// |exception_state| on return.void RejectInvalidConsumption(ExceptionState& exception_state) const;// The parts of LoadAndConvertBody() that do not depend on the template// parameters are split into this method to reduce binary size. Returns a// freshly-created ScriptPromiseResolver* on success, or nullptr on error. On// error, LoadAndConvertBody() must not continue.ScriptPromiseResolver* PrepareToLoadBody(ScriptState*, ExceptionState&);// Common implementation for body-reading accessors. To maximise performance// at the cost of code size, this is templated on the types of the lambdas// that are passed in.template <class Consumer,typename CreateLoaderFunction,typename OnNoBodyFunction>ScriptPromise LoadAndConvertBody(ScriptState*,CreateLoaderFunction,OnNoBodyFunction,ExceptionState&);
};} // namespace blink#endif // THIRD_PARTY_BLINK_RENDERER_CORE_FETCH_BODY_H_
6、Headers
(Request/Response
头部信息) third_party\blink\renderer\core\fetch\headers.idl
// Copyright 2014 The Chromium Authors
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.// https://fetch.spec.whatwg.org/#typedefdef-headersinittypedef (sequence<sequence<ByteString>> or record<ByteString, ByteString>) HeadersInit;// https://fetch.spec.whatwg.org/#headers-class[Exposed=(Window,Worker)
] interface Headers {[CallWith=ScriptState, RaisesException] constructor(optional HeadersInit init);[CallWith=ScriptState, RaisesException] void append(ByteString name, ByteString value);[CallWith=ScriptState, ImplementedAs=remove, RaisesException] void delete(ByteString key);[RaisesException] ByteString? get(ByteString key);sequence<ByteString> getSetCookie();[RaisesException] boolean has(ByteString key);[CallWith=ScriptState, RaisesException] void set(ByteString key, ByteString value);iterable<ByteString, ByteString>;
};
至此定义实现已经介绍完毕,在下一篇看下调用堆栈:
Chromium 中JavaScript Fetch API接口c++代码实现(二)-CSDN博客
相关文章:

Chromium 中JavaScript Fetch API接口c++代码实现(一)
Fetch API主要暴露了三个接口一个方法。 三个接口 Request(资源请求)Response(请求的响应)Headers(Request/Response头部信息)一个方法 fetch()(获取资源调用的方法更多介绍参考 Fetch API - Web API | MDN (mozilla.org) 一、 来看一段前端代码 <!DOCTYPE html> <h…...
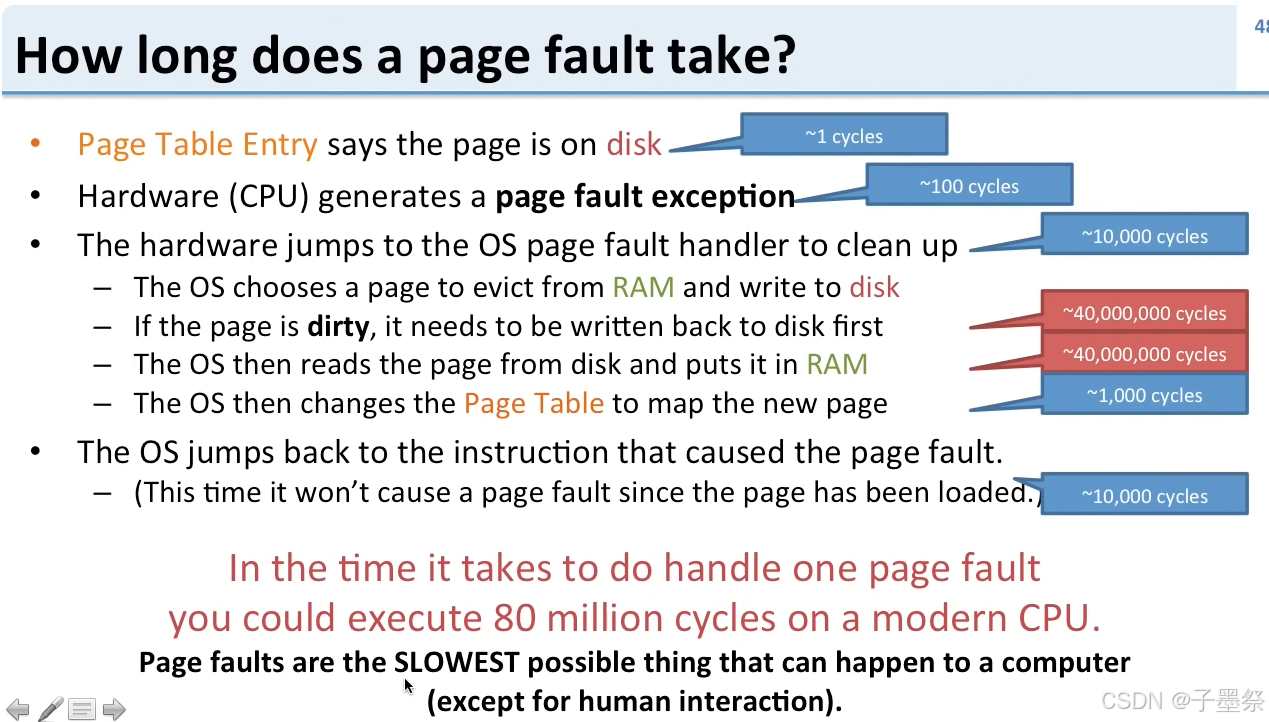
ARM(5)内存管理单元MMU
一、虚拟地址和物理地址 首先,计算机系统的内存被组成一个由M个连续的字节大小组成的数组。每字节都会有一个唯一的物理地址。CPU访问内存最简单的方式就是使用物理地址。如下图: 图 1 物理地址,物理寻址 而现在都是采用的都是虚拟寻址的方法。CPU生成一…...

文件上传漏洞原理
原理:\n应用中存在上传功能,但是上传的文件没有经过严格的合法性检验或者检验函数存在缺陷,导致可以上传木马文件到服务器,并且能够执行其中的恶意代码。\n\n危害:\n服务器的网页篡改,网站被挂马࿰…...
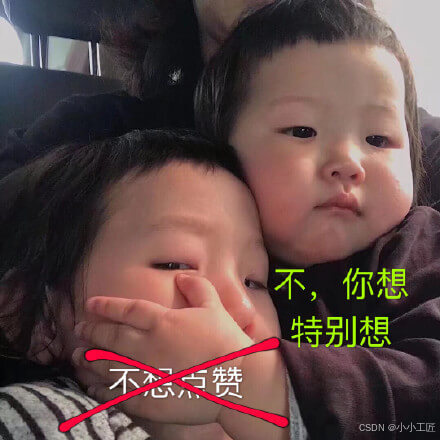
Web安全 - 安全防御工具和体系构建
文章目录 安全标准和框架1. 国内安全标准:等级保护制度(等保)2. 国际安全标准:ISO27000系列3. NIST安全框架:IDPRR方法4. COBIT与ITIL框架 防火墙防火墙的基本作用防火墙的三种主要类型防火墙的防护能力防火墙的盲区 W…...

服务器数据恢复—raid磁盘故障导致数据库文件损坏的数据恢复案例
服务器存储数据恢复环境&故障: 存储中有一组由3块SAS硬盘组建的raid。上层win server操作系统层面划分了3个分区,数据库存放在D分区,备份存放在E分区。 RAID中一块硬盘的指示灯亮红色,D分区无法识别;E分区可识别&a…...

requests 中data=xxx、json=xxx、params=xxx 分别什么时候用
如果是要做爬虫模拟一个页面提交,看原页面是post还是get,以及Content-Type是什么。 GET 请求 使用 paramsxxx,查询参数会被编码到 URL 中。POST 请求,Content-Type为 application/x-www-form-urlencoded的,使用 dataxx…...
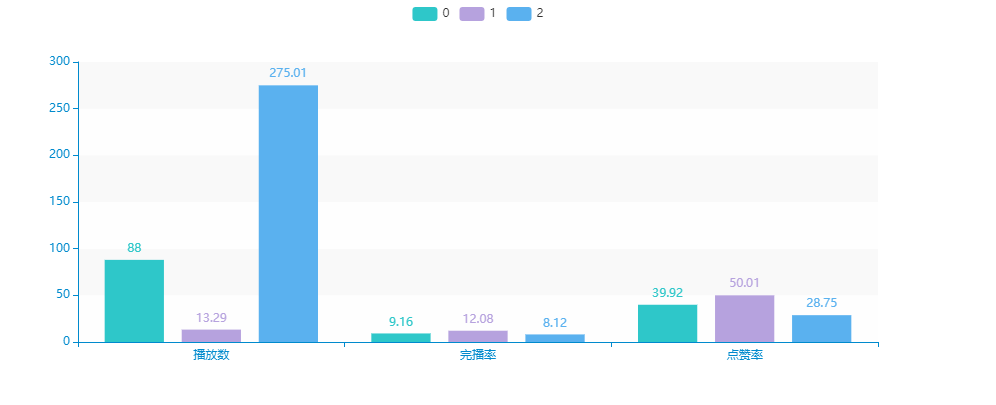
毕设 大数据抖音短视频数据分析与可视化(源码)
文章目录 0 前言1 课题背景2 数据清洗3 数据可视化地区-用户观看时间分界线每周观看观看路径发布地点视频时长整体点赞、完播 4 进阶分析相关性分析留存率 5 深度分析客户价值判断 0 前言 🔥 这两年开始毕业设计和毕业答辩的要求和难度不断提升,传统的毕…...

【SQL】深入理解SQL:从基础概念到常用命令
目录 1. SQL基础概念1.1 数据库与表1.2 行与列1.3 数据库与表结构示意图 2. 常用SQL命令3. DML 命令3.1 SELECT语句3.2 INSERT语句3.3 UPDATE语句3.4 DELETE语句 4. DDL 命令3.4.1 CREATE 命令3.4.2 ALTER 命令3.4.3 DROP 命令 5. DCL 命令3.6.1 GRANT 命令3.6.2 REVOKE 命令 学…...
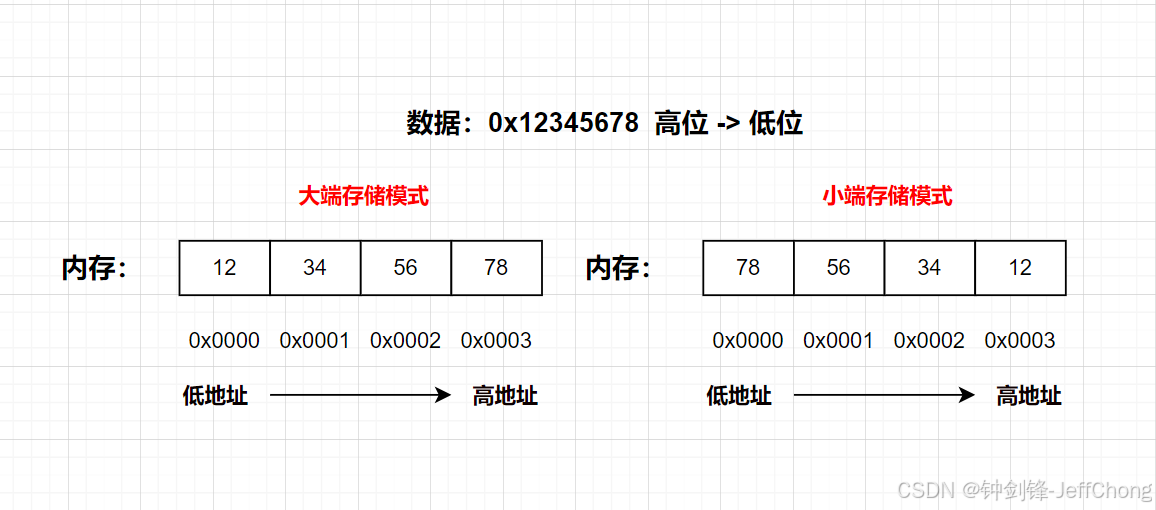
一文看懂计算机中的大小端(Endianess)
文章目录 前言一、什么是大小端二、如何判断大小端三、大小端的转换3.1 使用标准库函数3.2 手动实现大小端转换 前言 本文主要探讨计算机中大小端的相关概念以及如何进行大小端的判断和转换等。 一、什么是大小端 大小端(Endianess)是指计算机系统在存…...

如何给父母安排体检?
总结:给父母安排体检,常规项目针对项目。 其中针对项目是根据父母自身的病史来设计。 如何快速了解这些体检项目?我自己认为最快的方式,自己去医院体检两次,这样对体检的项目有一定的了解,比如这个项目怎么…...
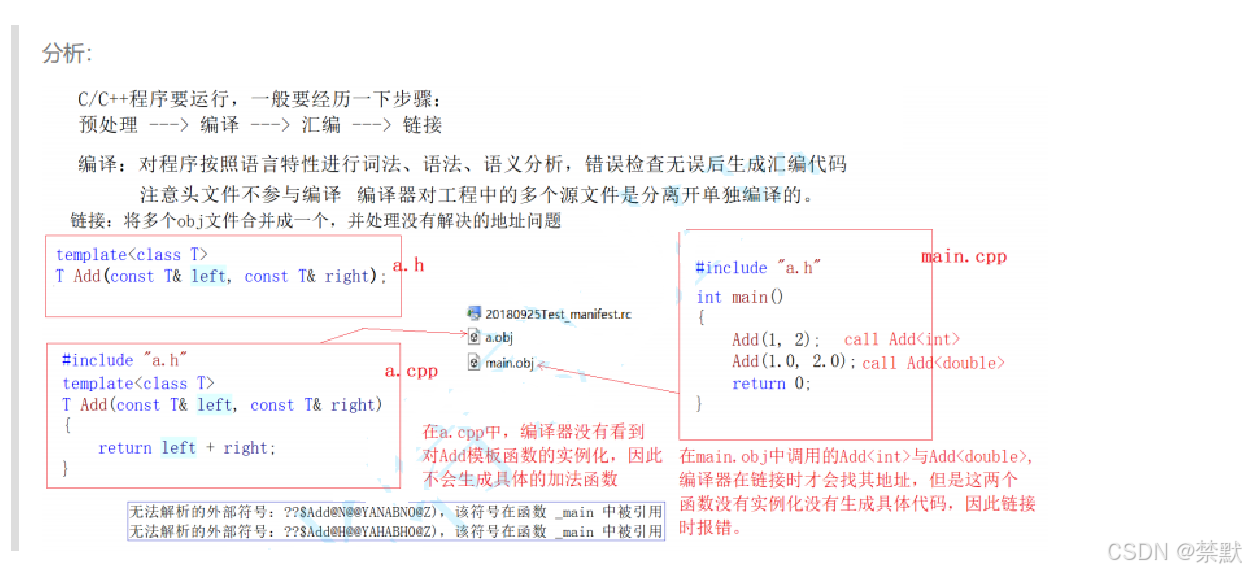
C++之模版进阶篇
目录 前言 1.非类型模版参数 2.模版的特化 2.1概念 2.2函数模版特化 2.3 类模板特化 2.3.1 全特化和偏特化 2.3.2类模版特化应用实例 3.模版分离编译 3.1 什么是分离编译 3.2 模板的分离编译 3.3 解决方法 4. 模板总结 结束语 前言 在模版初阶我们学习了函数模版和类…...

Vue3 中的 `replace` 属性:优化路由导航的利器
嘿,小伙伴们!今天给大家带来一个Vue3中非常实用的小技巧——replace属性的使用方法。在Vue Router中,replace属性可以帮助我们在导航时不留下历史记录,这对于一些特定的应用场景非常有用。话不多说,让我们直接进入实战…...
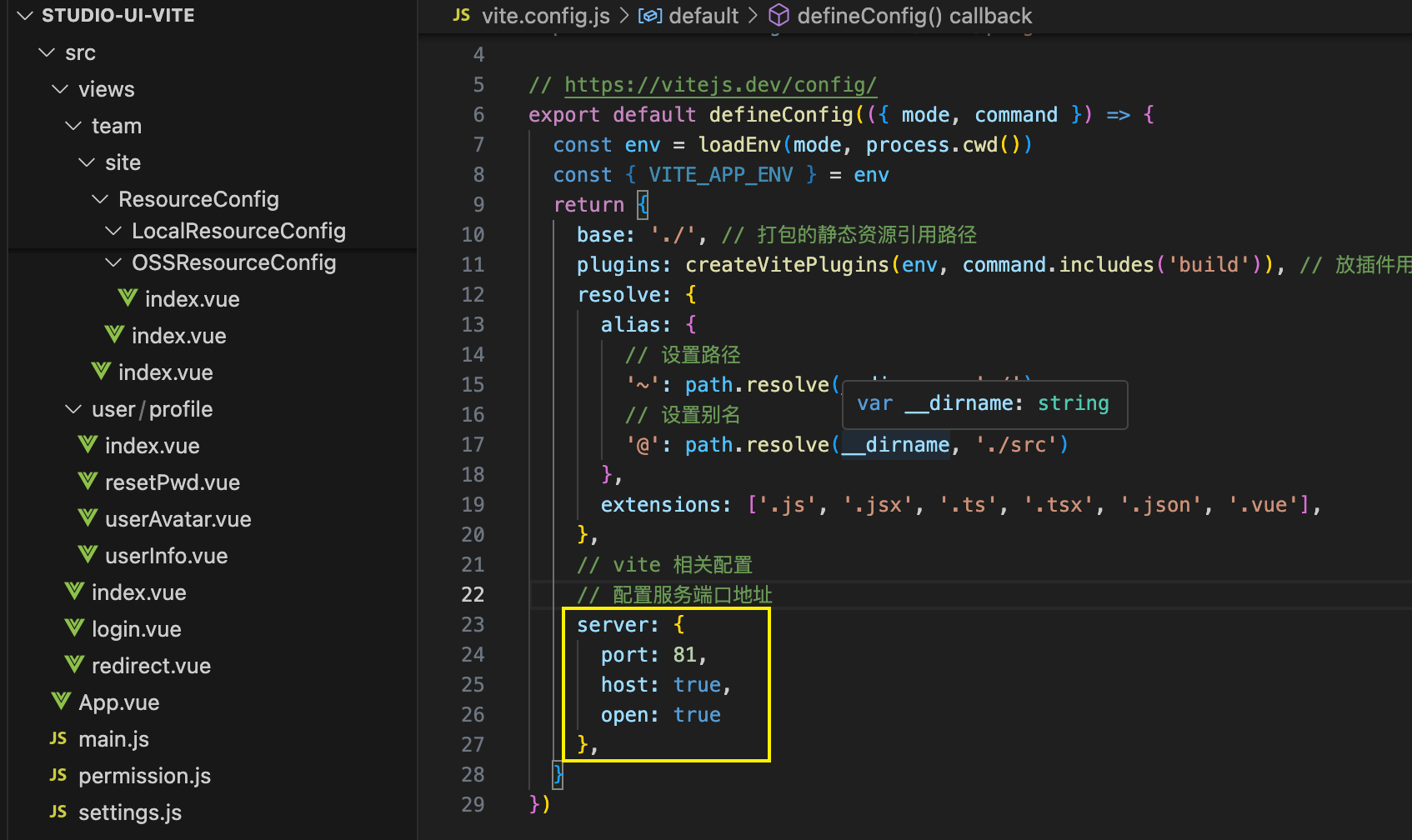
vite学习教程06、vite.config.js配置
前言 博主介绍:✌目前全网粉丝3W,csdn博客专家、Java领域优质创作者,博客之星、阿里云平台优质作者、专注于Java后端技术领域。 涵盖技术内容:Java后端、大数据、算法、分布式微服务、中间件、前端、运维等。 博主所有博客文件…...
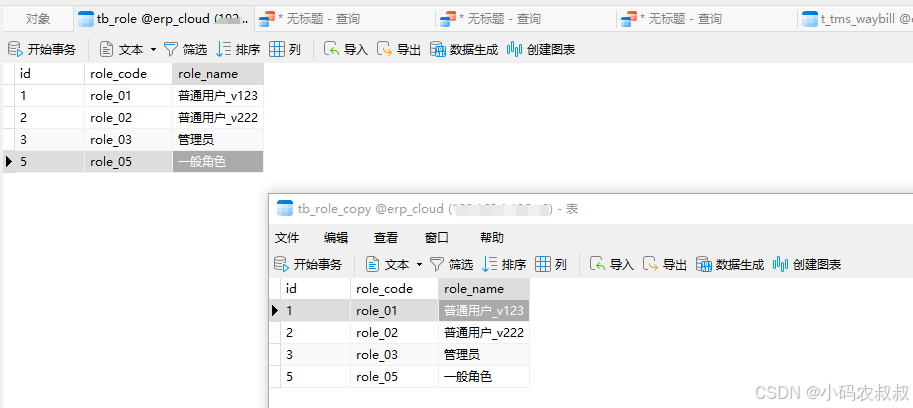
【大数据】Flink CDC 实时同步mysql数据
目录 一、前言 二、Flink CDC介绍 2.1 什么是Flink CDC 2.2 Flink CDC 特点 2.3 Flink CDC 核心工作原理 2.4 Flink CDC 使用场景 三、常用的数据同步方案对比 3.1 数据同步概述 3.1.1 数据同步来源 3.2 常用的数据同步方案汇总 3.3 为什么推荐Flink CDC 3.4 Flink …...
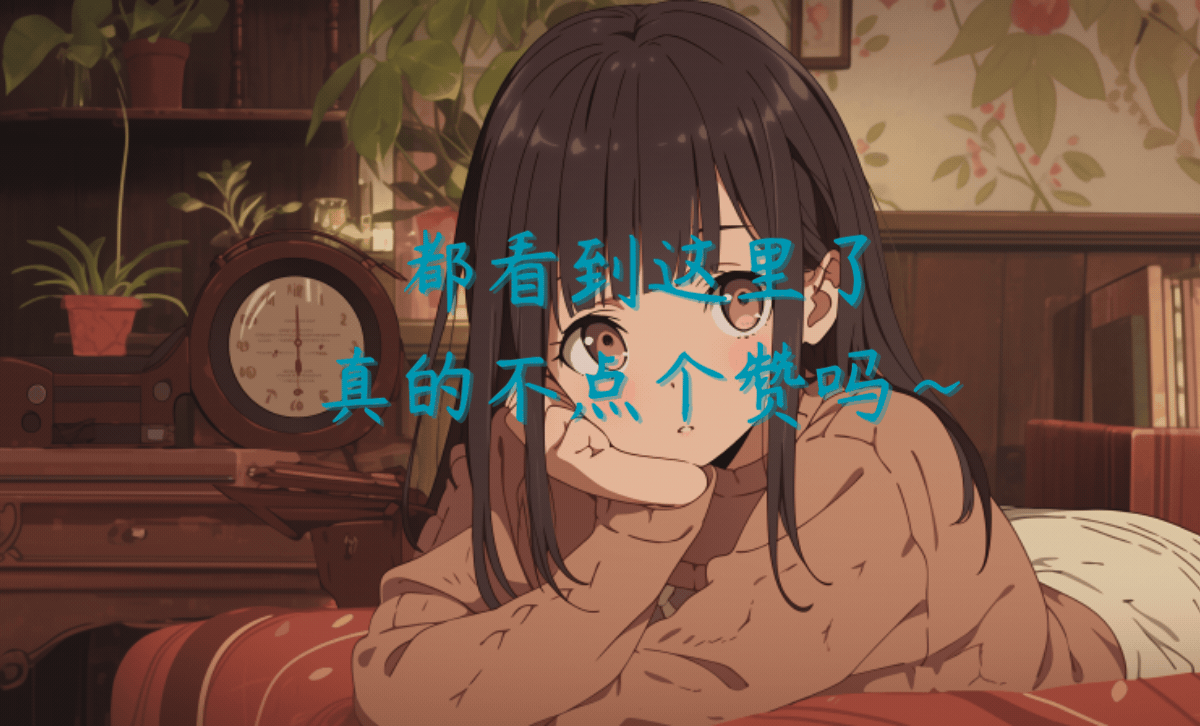
JavaEE: 深入解析HTTP协议的奥秘(1)
文章目录 HTTPHTTP 是什么HTTP 协议抓包fiddle 用法 HTTP 请求响应基本格式 HTTP HTTP 是什么 HTTP 全称为"超文本传输协议". HTTP不仅仅能传输文本,还能传输图片,传输音频文件,传输其他的各种数据. 因此它广泛应用在日常开发的各种场景中. HTTP 往往是基于传输层的…...

OpenStack Yoga版安装笔记(十六)Openstack网络理解
0、前言 本文将以Openstack在Linux Bridge环境下的应用为例进行阐述。 1、Openstack抽象网络 OpenStack的抽象网络主要包括网络(network)、子网(subnet)、端口(port),路由器(rout…...

PEFT库和transformers库在NLP大模型中的使用和常用方法详解
PEFT(Parameter-Efficient Fine-Tuning)库是一个用于有效微调大型预训练语言模型的工具,尤其是在计算资源有限的情况下。它提供了一系列技术,旨在提高微调过程的效率和灵活性。以下是PEFT库的详细解读以及一些常用方法的总结&…...
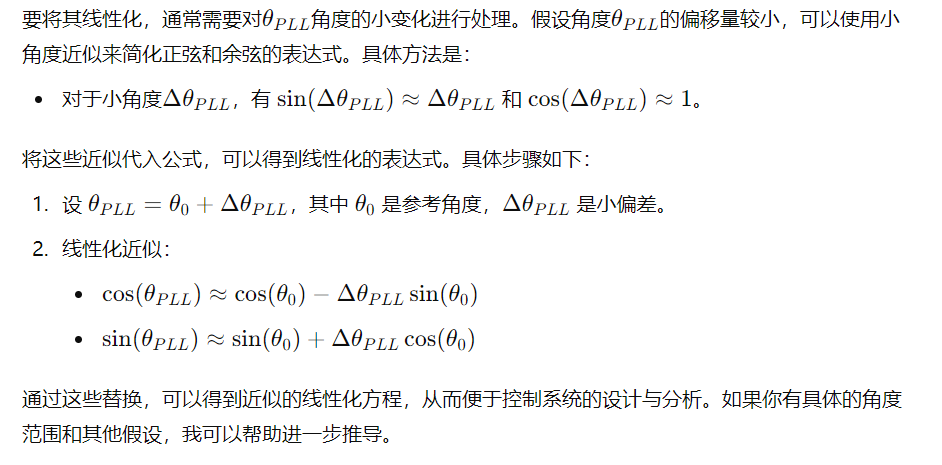
静止坐标系和旋转坐标系变换的线性化,锁相环线性化通用推导
将笛卡尔坐标系的电压 [ U x , U y ] [U_x, U_y] [Ux,Uy] 通过旋转变换(由锁相环角度 θ P L L \theta_{PLL} θPLL 控制)转换为 dq 坐标系下的电压 [ U d , U q ] [U_d, U_q] [Ud,Uq]。这个公式是非线性的,因为它涉及到正弦和余弦函数。 图片中的推导过程主要…...

AI学习指南深度学习篇-学习率衰减的变体及扩展应用
AI学习指南深度学习篇 - 学习率衰减的变体及扩展应用 在深度学习的训练过程中,学习率的选择对模型的收敛速度和最终效果有重要影响。为了提升模型性能,学习率衰减(Learning Rate Decay)作为一种优化技术被广泛应用。本文将探讨多…...
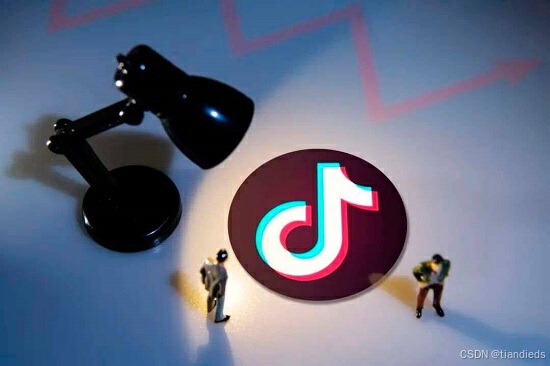
成都睿明智科技有限公司真实可靠吗?
在这个日新月异的电商时代,抖音作为短视频与直播电商的佼佼者,正以前所未有的速度重塑着消费者的购物习惯。而在这片充满机遇与挑战的蓝海中,成都睿明智科技有限公司以其独到的眼光和专业的服务,成为了众多商家信赖的合作伙伴。今…...
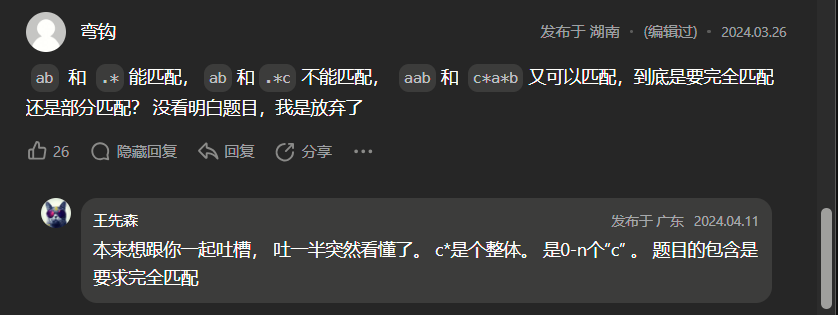
力扣6~10题
题6(中等): 思路: 这个相较于前面只能是简单,个人认为,会print打印菱形都能搞这个,直接设置一个2阶数组就好了,只要注意位置变化就好了 python代码: def convert(self,…...
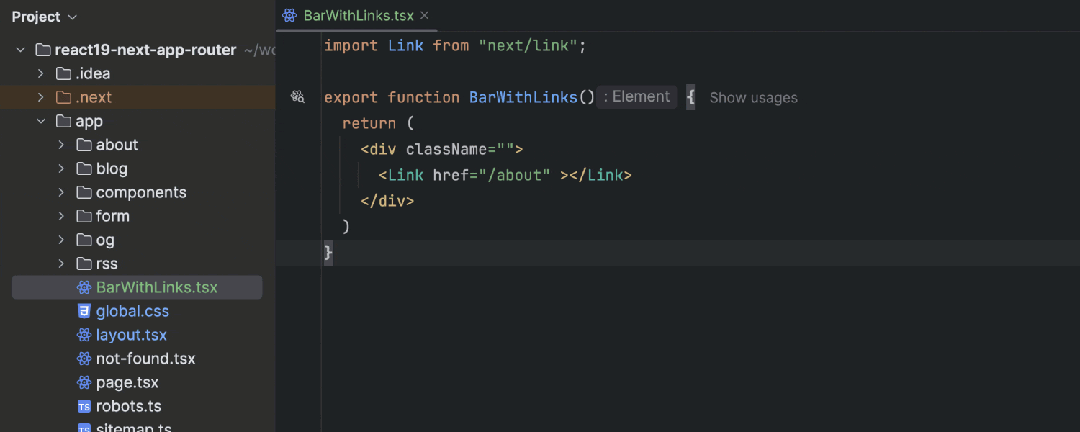
IntelliJ IDEA 2024.2 新特性概览
文章目录 1、重点特性:1.1 改进的 Spring Data JPA 支持1.2 改进的 cron 表达式支持1.3 使用 GraalJS 作为 HTTP 客户端的执行引擎1.4 更快的编码时间1.5 K2 模式下的 Kotlin 性能和稳定性改进 2、用户体验2.1 改进的全行代码补全2.2 新 UI 成为所有用户的默认界面2.3 Search E…...

C++基础(12)——初识list
目录 1.list的简介(引用自cplusplus官网) 2.list的相关使用 2.1有关list的定义 2.1.1方式一(构造某类型的空容器) 2.1.2方式二(构造n个val的容器) 2.1.3方式三(拷贝构造) 2.1.4…...

系统架构设计师论文《论NoSQL数据库技术及其应用》精选试读
论文真题 随着互联网web2.0网站的兴起,传统关系数据库在应对web2.0 网站,特别是超大规模和高并发的web2.0纯动态SNS网站上已经显得力不从心,暴露了很多难以克服的问题,而非关系型的数据库则由于其本身的特点得到了非常迅速的发展…...

产品经理产出的原型设计 - 需求文档应该怎么制作?
需求文档,产品经理最终产出的文档,也是产品设计最终的表述形式。本次分享呢,就是介绍如何写好一份需求文档。 所有元件均可复用,可作为管理端原型设计模板,按照实际项目需求进行功能拓展。有需要的话可分享源文件。 …...
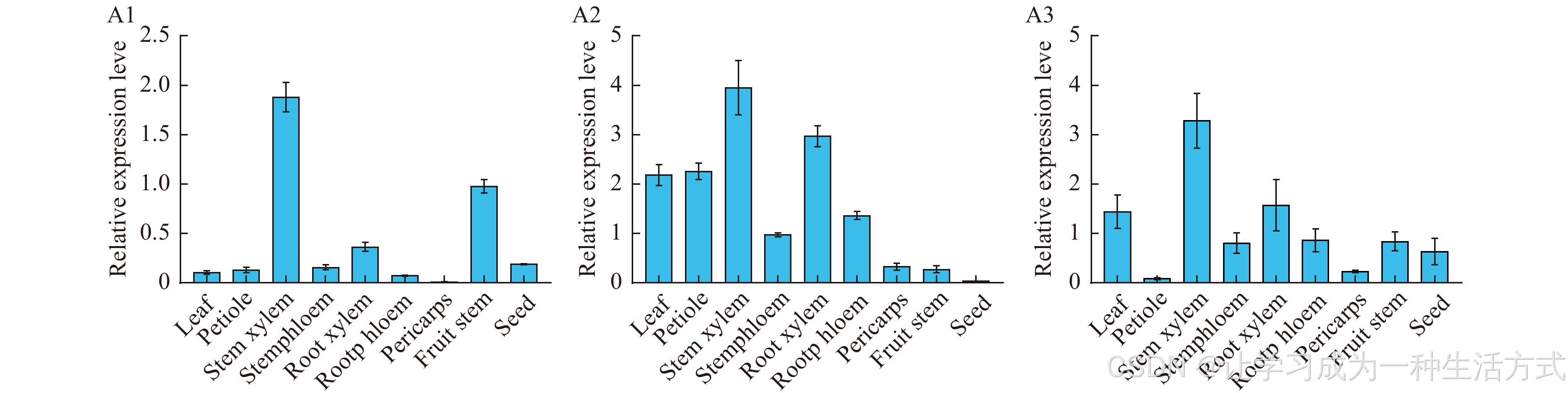
phenylalanine ammonia-lyase苯丙氨酸解氨酶PAL功能验证-文献精读61
Molecular cloning and characterization of three phenylalanine ammonia-lyase genes from Schisandra chinensis 五味子中三种苯丙氨酸解氨酶基因的分子克隆及特性分析 摘要 苯丙氨酸解氨酶(PAL)催化L-苯丙氨酸向反式肉桂酸的转化,是植物…...
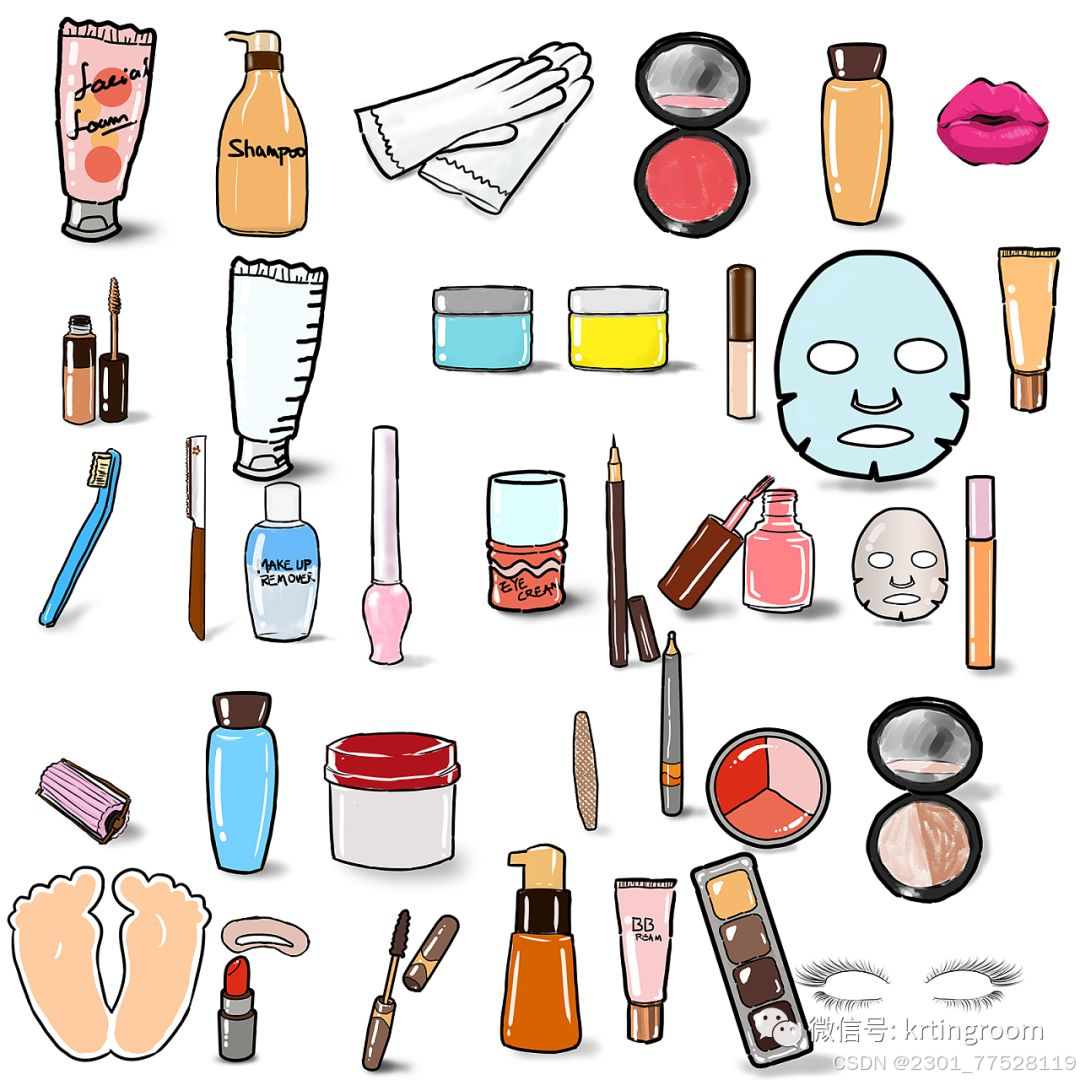
柯桥生活口语学习之在化妆品店可以用到的韩语句子
화장품을 사고 싶어요. 我想买化妆品。 어떤 화장품을 원하세요? 您想买什么化妆品。 스킨로션을 찾고 있어요. 我想买化妆水,乳液。 피부 타입은 어떠세요? 您是什么皮肤类型? 민감성 피부예요. 我是敏感性皮肤。 평소에 쓰시는 제품은 뭐예…...

Ubuntu 安装 Docker Compose
安装Docker Compose # 删除现有的 docker-compose(如果存在) sudo rm -f /usr/local/bin/docker-compose # 下载最新的 docker-compose 二进制文件 sudo curl -L "https://github.com/docker/compose/releases/latest/download/docker-compose-…...
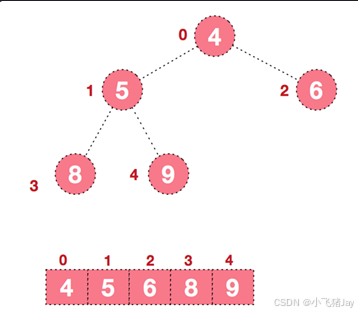
C++面试速通宝典——7
150. 数据库连接池的作用 数据库连接池的作用包括以下几个方面: 资源重用:连接池允许多个客户端共享有限的数据库连接,减少频繁创建和销毁连接的开销,从而提高资源的利用率。 统一的连接管理:连接池集中管理数据库连…...
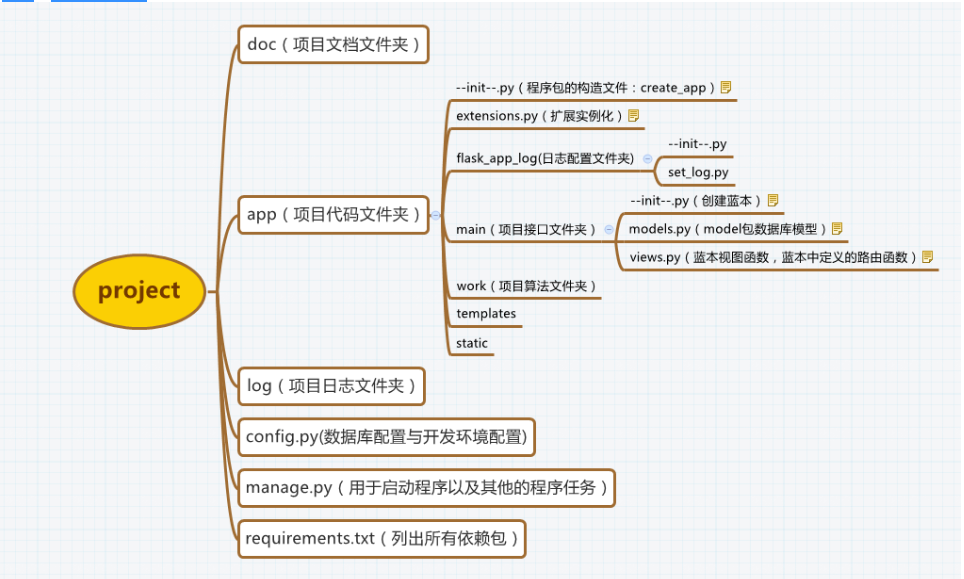
毕业设计 大数据电影数据分析与可视化系统
文章目录 0 简介1 课题背景2 效果实现3 爬虫及实现4 Flask框架5 Ajax技术6 Echarts7 最后 0 简介 今天学长向大家介绍一个机器视觉的毕设项目 🚩基于大数据的电影数据分析与可视化系统 项目运行效果(视频): 毕业设计 大数据电影评论情感分析 …...