Java web与Java中的Servlet
一。前言
Java语言大多用于开发web系统的后端,也就是我们是的B/S架构。通过浏览器一个URL去访问系统的后端资源和逻辑。 当我在代码里看到这个类HttpServletRequest 时 让我想到了Servlet,Servlet看上去多么像是Java的一个普通类,但是它确实不是一个类,它是通过Java实现的一项功能,就是用来连接URL和服务端程序的中间件。除了HttpServletRequest 对应的还有HttpServletResponse
二。介绍Servlet
什么事Servlet
Servlet(Server Applet)是JavaServlet的简称,称为小服务程序或服务连接器,用Java编写的服务器端程序,具有独立于平台和协议的特性,主要功能在于交互式地浏览和生成数据,生成动态Web内容是
JavaWeb中,我们将会接触到三大组件(Servlet、Filter、Listener),Servlet由服务器调用,处理服务器接收到的请求,即完成,接受请求数据 --> 处理请求 --> 完成响应,其本质就是一个实现了Servlet接口的java类
实现Servlet的方式
实现Servlet有三种方式:
- 实现 javax.servlet.Servlet 接口;
- 继承 javax.servlet.GenericServlet类;
- 继承 javax.servlet.http.HttpServlet类;
实际开发中,我们通常会选择继承HttpServlet类来完成我们的Servlet,但认识Servlet接口这种方式也是很重要的,是我们入门知识中不可或缺的部分
使用Servlet
/** Licensed to the Apache Software Foundation (ASF) under one or more* contributor license agreements. See the NOTICE file distributed with* this work for additional information regarding copyright ownership.* The ASF licenses this file to You under the Apache License, Version 2.0* (the "License"); you may not use this file except in compliance with* the License. You may obtain a copy of the License at** http://www.apache.org/licenses/LICENSE-2.0** Unless required by applicable law or agreed to in writing, software* distributed under the License is distributed on an "AS IS" BASIS,* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.* See the License for the specific language governing permissions and* limitations under the License.*/package javax.servlet.http;import java.io.IOException;
import java.util.Collection;
import java.util.Enumeration;import javax.servlet.ServletException;
import javax.servlet.ServletRequest;/*** Extends the {@link javax.servlet.ServletRequest} interface to provide request* information for HTTP servlets.* <p>* The servlet container creates an <code>HttpServletRequest</code> object and* passes it as an argument to the servlet's service methods* (<code>doGet</code>, <code>doPost</code>, etc).*/
public interface HttpServletRequest extends ServletRequest {/*** String identifier for Basic authentication. Value "BASIC"*/public static final String BASIC_AUTH = "BASIC";/*** String identifier for Form authentication. Value "FORM"*/public static final String FORM_AUTH = "FORM";/*** String identifier for Client Certificate authentication. Value* "CLIENT_CERT"*/public static final String CLIENT_CERT_AUTH = "CLIENT_CERT";/*** String identifier for Digest authentication. Value "DIGEST"*/public static final String DIGEST_AUTH = "DIGEST";/*** Returns the name of the authentication scheme used to protect the* servlet. All servlet containers support basic, form and client* certificate authentication, and may additionally support digest* authentication. If the servlet is not authenticated <code>null</code> is* returned.* <p>* Same as the value of the CGI variable AUTH_TYPE.** @return one of the static members BASIC_AUTH, FORM_AUTH, CLIENT_CERT_AUTH,* DIGEST_AUTH (suitable for == comparison) or the* container-specific string indicating the authentication scheme,* or <code>null</code> if the request was not authenticated.*/public String getAuthType();/*** Returns an array containing all of the <code>Cookie</code> objects the* client sent with this request. This method returns <code>null</code> if* no cookies were sent.** @return an array of all the <code>Cookies</code> included with this* request, or <code>null</code> if the request has no cookies*/public Cookie[] getCookies();/*** Returns the value of the specified request header as a <code>long</code>* value that represents a <code>Date</code> object. Use this method with* headers that contain dates, such as <code>If-Modified-Since</code>.* <p>* The date is returned as the number of milliseconds since January 1, 1970* GMT. The header name is case insensitive.* <p>* If the request did not have a header of the specified name, this method* returns -1. If the header can't be converted to a date, the method throws* an <code>IllegalArgumentException</code>.** @param name* a <code>String</code> specifying the name of the header* @return a <code>long</code> value representing the date specified in the* header expressed as the number of milliseconds since January 1,* 1970 GMT, or -1 if the named header was not included with the* request* @exception IllegalArgumentException* If the header value can't be converted to a date*/public long getDateHeader(String name);/*** Returns the value of the specified request header as a* <code>String</code>. If the request did not include a header of the* specified name, this method returns <code>null</code>. If there are* multiple headers with the same name, this method returns the first head* in the request. The header name is case insensitive. You can use this* method with any request header.** @param name* a <code>String</code> specifying the header name* @return a <code>String</code> containing the value of the requested* header, or <code>null</code> if the request does not have a* header of that name*/public String getHeader(String name);/*** Returns all the values of the specified request header as an* <code>Enumeration</code> of <code>String</code> objects.* <p>* Some headers, such as <code>Accept-Language</code> can be sent by clients* as several headers each with a different value rather than sending the* header as a comma separated list.* <p>* If the request did not include any headers of the specified name, this* method returns an empty <code>Enumeration</code>. The header name is case* insensitive. You can use this method with any request header.** @param name* a <code>String</code> specifying the header name* @return an <code>Enumeration</code> containing the values of the requested* header. If the request does not have any headers of that name* return an empty enumeration. If the container does not allow* access to header information, return null*/public Enumeration<String> getHeaders(String name);/*** Returns an enumeration of all the header names this request contains. If* the request has no headers, this method returns an empty enumeration.* <p>* Some servlet containers do not allow servlets to access headers using* this method, in which case this method returns <code>null</code>** @return an enumeration of all the header names sent with this request; if* the request has no headers, an empty enumeration; if the servlet* container does not allow servlets to use this method,* <code>null</code>*/public Enumeration<String> getHeaderNames();/*** Returns the value of the specified request header as an <code>int</code>.* If the request does not have a header of the specified name, this method* returns -1. If the header cannot be converted to an integer, this method* throws a <code>NumberFormatException</code>.* <p>* The header name is case insensitive.** @param name* a <code>String</code> specifying the name of a request header* @return an integer expressing the value of the request header or -1 if the* request doesn't have a header of this name* @exception NumberFormatException* If the header value can't be converted to an* <code>int</code>*/public int getIntHeader(String name);/*** Returns the name of the HTTP method with which this request was made, for* example, GET, POST, or PUT. Same as the value of the CGI variable* REQUEST_METHOD.** @return a <code>String</code> specifying the name of the method with* which this request was made*/public String getMethod();/*** Returns any extra path information associated with the URL the client* sent when it made this request. The extra path information follows the* servlet path but precedes the query string and will start with a "/"* character.* <p>* This method returns <code>null</code> if there was no extra path* information.* <p>* Same as the value of the CGI variable PATH_INFO.** @return a <code>String</code>, decoded by the web container, specifying* extra path information that comes after the servlet path but* before the query string in the request URL; or <code>null</code>* if the URL does not have any extra path information*/public String getPathInfo();/*** Returns any extra path information after the servlet name but before the* query string, and translates it to a real path. Same as the value of the* CGI variable PATH_TRANSLATED.* <p>* If the URL does not have any extra path information, this method returns* <code>null</code> or the servlet container cannot translate the virtual* path to a real path for any reason (such as when the web application is* executed from an archive). The web container does not decode this string.** @return a <code>String</code> specifying the real path, or* <code>null</code> if the URL does not have any extra path* information*/public String getPathTranslated();/*** Returns the portion of the request URI that indicates the context of the* request. The context path always comes first in a request URI. The path* starts with a "/" character but does not end with a "/" character. For* servlets in the default (root) context, this method returns "". The* container does not decode this string.** @return a <code>String</code> specifying the portion of the request URI* that indicates the context of the request*/public String getContextPath();/*** Returns the query string that is contained in the request URL after the* path. This method returns <code>null</code> if the URL does not have a* query string. Same as the value of the CGI variable QUERY_STRING.** @return a <code>String</code> containing the query string or* <code>null</code> if the URL contains no query string. The value* is not decoded by the container.*/public String getQueryString();/*** Returns the login of the user making this request, if the user has been* authenticated, or <code>null</code> if the user has not been* authenticated. Whether the user name is sent with each subsequent request* depends on the browser and type of authentication. Same as the value of* the CGI variable REMOTE_USER.** @return a <code>String</code> specifying the login of the user making* this request, or <code>null</code> if the user login is not known*/public String getRemoteUser();/*** Returns a boolean indicating whether the authenticated user is included* in the specified logical "role". Roles and role membership can be defined* using deployment descriptors. If the user has not been authenticated, the* method returns <code>false</code>.** @param role* a <code>String</code> specifying the name of the role* @return a <code>boolean</code> indicating whether the user making this* request belongs to a given role; <code>false</code> if the user* has not been authenticated*/public boolean isUserInRole(String role);/*** Returns a <code>java.security.Principal</code> object containing the name* of the current authenticated user. If the user has not been* authenticated, the method returns <code>null</code>.** @return a <code>java.security.Principal</code> containing the name of the* user making this request; <code>null</code> if the user has not* been authenticated*/public java.security.Principal getUserPrincipal();/*** Returns the session ID specified by the client. This may not be the same* as the ID of the current valid session for this request. If the client* did not specify a session ID, this method returns <code>null</code>.** @return a <code>String</code> specifying the session ID, or* <code>null</code> if the request did not specify a session ID* @see #isRequestedSessionIdValid*/public String getRequestedSessionId();/*** Returns the part of this request's URL from the protocol name up to the* query string in the first line of the HTTP request. The web container* does not decode this String. For example:* <table summary="Examples of Returned Values">* <tr align=left>* <th>First line of HTTP request</th>* <th>Returned Value</th>* <tr>* <td>POST /some/path.html HTTP/1.1* <td>* <td>/some/path.html* <tr>* <td>GET http://foo.bar/a.html HTTP/1.0* <td>* <td>/a.html* <tr>* <td>HEAD /xyz?a=b HTTP/1.1* <td>* <td>/xyz* </table>* <p>* To reconstruct an URL with a scheme and host, use* {@link #getRequestURL}.** @return a <code>String</code> containing the part of the URL from the* protocol name up to the query string* @see #getRequestURL*/public String getRequestURI();/*** Reconstructs the URL the client used to make the request. The returned* URL contains a protocol, server name, port number, and server path, but* it does not include query string parameters.* <p>* Because this method returns a <code>StringBuffer</code>, not a string,* you can modify the URL easily, for example, to append query parameters.* <p>* This method is useful for creating redirect messages and for reporting* errors.** @return a <code>StringBuffer</code> object containing the reconstructed* URL*/public StringBuffer getRequestURL();/*** Returns the part of this request's URL that calls the servlet. This path* starts with a "/" character and includes either the servlet name or a* path to the servlet, but does not include any extra path information or a* query string. Same as the value of the CGI variable SCRIPT_NAME.* <p>* This method will return an empty string ("") if the servlet used to* process this request was matched using the "/*" pattern.** @return a <code>String</code> containing the name or path of the servlet* being called, as specified in the request URL, decoded, or an* empty string if the servlet used to process the request is* matched using the "/*" pattern.*/public String getServletPath();/*** Returns the current <code>HttpSession</code> associated with this request* or, if there is no current session and <code>create</code> is true,* returns a new session.* <p>* If <code>create</code> is <code>false</code> and the request has no valid* <code>HttpSession</code>, this method returns <code>null</code>.* <p>* To make sure the session is properly maintained, you must call this* method before the response is committed. If the container is using* cookies to maintain session integrity and is asked to create a new* session when the response is committed, an IllegalStateException is* thrown.** @param create* <code>true</code> to create a new session for this request if* necessary; <code>false</code> to return <code>null</code> if* there's no current session* @return the <code>HttpSession</code> associated with this request or* <code>null</code> if <code>create</code> is <code>false</code>* and the request has no valid session* @see #getSession()*/public HttpSession getSession(boolean create);/*** Returns the current session associated with this request, or if the* request does not have a session, creates one.** @return the <code>HttpSession</code> associated with this request* @see #getSession(boolean)*/public HttpSession getSession();/*** Changes the session ID of the session associated with this request. This* method does not create a new session object it only changes the ID of the* current session.** @return the new session ID allocated to the session* @see HttpSessionIdListener* @since Servlet 3.1*/public String changeSessionId();/*** Checks whether the requested session ID is still valid.** @return <code>true</code> if this request has an id for a valid session* in the current session context; <code>false</code> otherwise* @see #getRequestedSessionId* @see #getSession*/public boolean isRequestedSessionIdValid();/*** Checks whether the requested session ID came in as a cookie.** @return <code>true</code> if the session ID came in as a cookie;* otherwise, <code>false</code>* @see #getSession*/public boolean isRequestedSessionIdFromCookie();/*** Checks whether the requested session ID came in as part of the request* URL.** @return <code>true</code> if the session ID came in as part of a URL;* otherwise, <code>false</code>* @see #getSession*/public boolean isRequestedSessionIdFromURL();/*** @return {@link #isRequestedSessionIdFromURL()}* @deprecated As of Version 2.1 of the Java Servlet API, use* {@link #isRequestedSessionIdFromURL} instead.*/@SuppressWarnings("dep-ann")// Spec API does not use @Deprecatedpublic boolean isRequestedSessionIdFromUrl();/*** Triggers the same authentication process as would be triggered if the* request is for a resource that is protected by a security constraint.** @param response The response to use to return any authentication* challenge* @return <code>true</code> if the user is successfully authenticated and* <code>false</code> if not** @throws IOException if the authentication process attempted to read from* the request or write to the response and an I/O error occurred* @throws IllegalStateException if the authentication process attempted to* write to the response after it had been committed* @throws ServletException if the authentication failed and the caller is* expected to handle the failure* @since Servlet 3.0*/public boolean authenticate(HttpServletResponse response)throws IOException, ServletException;/*** Authenticate the provided user name and password and then associated the* authenticated user with the request.** @param username The user name to authenticate* @param password The password to use to authenticate the user** @throws ServletException* If any of {@link #getRemoteUser()},* {@link #getUserPrincipal()} or {@link #getAuthType()} are* non-null, if the configured authenticator does not support* user name and password authentication or if the* authentication fails* @since Servlet 3.0*/public void login(String username, String password) throws ServletException;/*** Removes any authenticated user from the request.** @throws ServletException* If the logout fails* @since Servlet 3.0*/public void logout() throws ServletException;/*** Return a collection of all uploaded Parts.** @return A collection of all uploaded Parts.* @throws IOException* if an I/O error occurs* @throws IllegalStateException* if size limits are exceeded or no multipart configuration is* provided* @throws ServletException* if the request is not multipart/form-data* @since Servlet 3.0*/public Collection<Part> getParts() throws IOException,ServletException;/*** Gets the named Part or null if the Part does not exist. Triggers upload* of all Parts.** @param name The name of the Part to obtain** @return The named Part or null if the Part does not exist* @throws IOException* if an I/O error occurs* @throws IllegalStateException* if size limits are exceeded* @throws ServletException* if the request is not multipart/form-data* @since Servlet 3.0*/public Part getPart(String name) throws IOException,ServletException;/*** Start the HTTP upgrade process and pass the connection to the provided* protocol handler once the current request/response pair has completed* processing. Calling this method sets the response status to {@link* HttpServletResponse#SC_SWITCHING_PROTOCOLS} and flushes the response.* Protocol specific headers must have already been set before this method* is called.** @param <T> The type of the upgrade handler* @param httpUpgradeHandlerClass The class that implements the upgrade* handler** @return A newly created instance of the specified upgrade handler type** @throws IOException* if an I/O error occurred during the upgrade* @throws ServletException* if the given httpUpgradeHandlerClass fails to be instantiated* @since Servlet 3.1*/public <T extends HttpUpgradeHandler> T upgrade(Class<T> httpUpgradeHandlerClass) throws java.io.IOException, ServletException;
}
/** Licensed to the Apache Software Foundation (ASF) under one or more* contributor license agreements. See the NOTICE file distributed with* this work for additional information regarding copyright ownership.* The ASF licenses this file to You under the Apache License, Version 2.0* (the "License"); you may not use this file except in compliance with* the License. You may obtain a copy of the License at** http://www.apache.org/licenses/LICENSE-2.0** Unless required by applicable law or agreed to in writing, software* distributed under the License is distributed on an "AS IS" BASIS,* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.* See the License for the specific language governing permissions and* limitations under the License.*/
package javax.servlet.http;import java.io.IOException;
import java.util.Collection;import javax.servlet.ServletResponse;/*** Extends the {@link ServletResponse} interface to provide HTTP-specific* functionality in sending a response. For example, it has methods to access* HTTP headers and cookies.* <p>* The servlet container creates an <code>HttpServletResponse</code> object and* passes it as an argument to the servlet's service methods (<code>doGet</code>, <code>doPost</code>, etc).** @see javax.servlet.ServletResponse*/
public interface HttpServletResponse extends ServletResponse {/*** Adds the specified cookie to the response. This method can be called* multiple times to set more than one cookie.** @param cookie* the Cookie to return to the client*/public void addCookie(Cookie cookie);/*** Returns a boolean indicating whether the named response header has* already been set.** @param name* the header name* @return <code>true</code> if the named response header has already been* set; <code>false</code> otherwise*/public boolean containsHeader(String name);/*** Encodes the specified URL by including the session ID in it, or, if* encoding is not needed, returns the URL unchanged. The implementation of* this method includes the logic to determine whether the session ID needs* to be encoded in the URL. For example, if the browser supports cookies,* or session tracking is turned off, URL encoding is unnecessary.* <p>* For robust session tracking, all URLs emitted by a servlet should be run* through this method. Otherwise, URL rewriting cannot be used with* browsers which do not support cookies.** @param url* the url to be encoded.* @return the encoded URL if encoding is needed; the unchanged URL* otherwise.*/public String encodeURL(String url);/*** Encodes the specified URL for use in the <code>sendRedirect</code> method* or, if encoding is not needed, returns the URL unchanged. The* implementation of this method includes the logic to determine whether the* session ID needs to be encoded in the URL. Because the rules for making* this determination can differ from those used to decide whether to encode* a normal link, this method is separated from the <code>encodeURL</code>* method.* <p>* All URLs sent to the <code>HttpServletResponse.sendRedirect</code> method* should be run through this method. Otherwise, URL rewriting cannot be* used with browsers which do not support cookies.** @param url* the url to be encoded.* @return the encoded URL if encoding is needed; the unchanged URL* otherwise.* @see #sendRedirect* @see #encodeUrl*/public String encodeRedirectURL(String url);/*** @param url* the url to be encoded.* @return the encoded URL if encoding is needed; the unchanged URL* otherwise.* @deprecated As of version 2.1, use encodeURL(String url) instead*/@SuppressWarnings("dep-ann")// Spec API does not use @Deprecatedpublic String encodeUrl(String url);/*** @param url* the url to be encoded.* @return the encoded URL if encoding is needed; the unchanged URL* otherwise.* @deprecated As of version 2.1, use encodeRedirectURL(String url) instead*/@SuppressWarnings("dep-ann")// Spec API does not use @Deprecatedpublic String encodeRedirectUrl(String url);/*** Sends an error response to the client using the specified status code and* clears the output buffer. The server defaults to creating the response to* look like an HTML-formatted server error page containing the specified* message, setting the content type to "text/html", leaving cookies and* other headers unmodified. If an error-page declaration has been made for* the web application corresponding to the status code passed in, it will* be served back in preference to the suggested msg parameter.* <p>* If the response has already been committed, this method throws an* IllegalStateException. After using this method, the response should be* considered to be committed and should not be written to.** @param sc* the error status code* @param msg* the descriptive message* @exception IOException* If an input or output exception occurs* @exception IllegalStateException* If the response was committed*/public void sendError(int sc, String msg) throws IOException;/*** Sends an error response to the client using the specified status code and* clears the buffer. This is equivalent to calling {@link #sendError(int,* String)} with the same status code and <code>null</code> for the message.** @param sc* the error status code* @exception IOException* If an input or output exception occurs* @exception IllegalStateException* If the response was committed before this method call*/public void sendError(int sc) throws IOException;/*** Sends a temporary redirect response to the client using the specified* redirect location URL. This method can accept relative URLs; the servlet* container must convert the relative URL to an absolute URL before sending* the response to the client. If the location is relative without a leading* '/' the container interprets it as relative to the current request URI.* If the location is relative with a leading '/' the container interprets* it as relative to the servlet container root.* <p>* If the response has already been committed, this method throws an* IllegalStateException. After using this method, the response should be* considered to be committed and should not be written to.** @param location* the redirect location URL* @exception IOException* If an input or output exception occurs* @exception IllegalStateException* If the response was committed or if a partial URL is given* and cannot be converted into a valid URL*/public void sendRedirect(String location) throws IOException;/*** Sets a response header with the given name and date-value. The date is* specified in terms of milliseconds since the epoch. If the header had* already been set, the new value overwrites the previous one. The* <code>containsHeader</code> method can be used to test for the presence* of a header before setting its value.** @param name* the name of the header to set* @param date* the assigned date value* @see #containsHeader* @see #addDateHeader*/public void setDateHeader(String name, long date);/*** Adds a response header with the given name and date-value. The date is* specified in terms of milliseconds since the epoch. This method allows* response headers to have multiple values.** @param name* the name of the header to set* @param date* the additional date value* @see #setDateHeader*/public void addDateHeader(String name, long date);/*** Sets a response header with the given name and value. If the header had* already been set, the new value overwrites the previous one. The* <code>containsHeader</code> method can be used to test for the presence* of a header before setting its value.** @param name* the name of the header* @param value* the header value If it contains octet string, it should be* encoded according to RFC 2047* (http://www.ietf.org/rfc/rfc2047.txt)* @see #containsHeader* @see #addHeader*/public void setHeader(String name, String value);/*** Adds a response header with the given name and value. This method allows* response headers to have multiple values.** @param name* the name of the header* @param value* the additional header value If it contains octet string, it* should be encoded according to RFC 2047* (http://www.ietf.org/rfc/rfc2047.txt)* @see #setHeader*/public void addHeader(String name, String value);/*** Sets a response header with the given name and integer value. If the* header had already been set, the new value overwrites the previous one.* The <code>containsHeader</code> method can be used to test for the* presence of a header before setting its value.** @param name* the name of the header* @param value* the assigned integer value* @see #containsHeader* @see #addIntHeader*/public void setIntHeader(String name, int value);/*** Adds a response header with the given name and integer value. This method* allows response headers to have multiple values.** @param name* the name of the header* @param value* the assigned integer value* @see #setIntHeader*/public void addIntHeader(String name, int value);/*** Sets the status code for this response. This method is used to set the* return status code when there is no error (for example, for the status* codes SC_OK or SC_MOVED_TEMPORARILY). If there is an error, and the* caller wishes to invoke an error-page defined in the web application, the* <code>sendError</code> method should be used instead.* <p>* The container clears the buffer and sets the Location header, preserving* cookies and other headers.** @param sc* the status code* @see #sendError*/public void setStatus(int sc);/*** Sets the status code and message for this response.** @param sc* the status code* @param sm* the status message* @deprecated As of version 2.1, due to ambiguous meaning of the message* parameter. To set a status code use* <code>setStatus(int)</code>, to send an error with a* description use <code>sendError(int, String)</code>.*/@SuppressWarnings("dep-ann")// Spec API does not use @Deprecatedpublic void setStatus(int sc, String sm);/*** Get the HTTP status code for this Response.** @return The HTTP status code for this Response** @since Servlet 3.0*/public int getStatus();/*** Return the value for the specified header, or <code>null</code> if this* header has not been set. If more than one value was added for this* name, only the first is returned; use {@link #getHeaders(String)} to* retrieve all of them.** @param name Header name to look up** @return The first value for the specified header. This is the raw value* so if multiple values are specified in the first header then they* will be returned as a single header value .** @since Servlet 3.0*/public String getHeader(String name);/*** Return a Collection of all the header values associated with the* specified header name.** @param name Header name to look up** @return The values for the specified header. These are the raw values so* if multiple values are specified in a single header that will be* returned as a single header value.** @since Servlet 3.0*/public Collection<String> getHeaders(String name);/*** Get the header names set for this HTTP response.** @return The header names set for this HTTP response.** @since Servlet 3.0*/public Collection<String> getHeaderNames();/** Server status codes; see RFC 2068.*//*** Status code (100) indicating the client can continue.*/public static final int SC_CONTINUE = 100;/*** Status code (101) indicating the server is switching protocols according* to Upgrade header.*/public static final int SC_SWITCHING_PROTOCOLS = 101;/*** Status code (200) indicating the request succeeded normally.*/public static final int SC_OK = 200;/*** Status code (201) indicating the request succeeded and created a new* resource on the server.*/public static final int SC_CREATED = 201;/*** Status code (202) indicating that a request was accepted for processing,* but was not completed.*/public static final int SC_ACCEPTED = 202;/*** Status code (203) indicating that the meta information presented by the* client did not originate from the server.*/public static final int SC_NON_AUTHORITATIVE_INFORMATION = 203;/*** Status code (204) indicating that the request succeeded but that there* was no new information to return.*/public static final int SC_NO_CONTENT = 204;/*** Status code (205) indicating that the agent <em>SHOULD</em> reset the* document view which caused the request to be sent.*/public static final int SC_RESET_CONTENT = 205;/*** Status code (206) indicating that the server has fulfilled the partial* GET request for the resource.*/public static final int SC_PARTIAL_CONTENT = 206;/*** Status code (300) indicating that the requested resource corresponds to* any one of a set of representations, each with its own specific location.*/public static final int SC_MULTIPLE_CHOICES = 300;/*** Status code (301) indicating that the resource has permanently moved to a* new location, and that future references should use a new URI with their* requests.*/public static final int SC_MOVED_PERMANENTLY = 301;/*** Status code (302) indicating that the resource has temporarily moved to* another location, but that future references should still use the* original URI to access the resource. This definition is being retained* for backwards compatibility. SC_FOUND is now the preferred definition.*/public static final int SC_MOVED_TEMPORARILY = 302;/*** Status code (302) indicating that the resource reside temporarily under a* different URI. Since the redirection might be altered on occasion, the* client should continue to use the Request-URI for future* requests.(HTTP/1.1) To represent the status code (302), it is recommended* to use this variable.*/public static final int SC_FOUND = 302;/*** Status code (303) indicating that the response to the request can be* found under a different URI.*/public static final int SC_SEE_OTHER = 303;/*** Status code (304) indicating that a conditional GET operation found that* the resource was available and not modified.*/public static final int SC_NOT_MODIFIED = 304;/*** Status code (305) indicating that the requested resource <em>MUST</em> be* accessed through the proxy given by the <code><em>Location</em></code>* field.*/public static final int SC_USE_PROXY = 305;/*** Status code (307) indicating that the requested resource resides* temporarily under a different URI. The temporary URI <em>SHOULD</em> be* given by the <code><em>Location</em></code> field in the response.*/public static final int SC_TEMPORARY_REDIRECT = 307;/*** Status code (400) indicating the request sent by the client was* syntactically incorrect.*/public static final int SC_BAD_REQUEST = 400;/*** Status code (401) indicating that the request requires HTTP* authentication.*/public static final int SC_UNAUTHORIZED = 401;/*** Status code (402) reserved for future use.*/public static final int SC_PAYMENT_REQUIRED = 402;/*** Status code (403) indicating the server understood the request but* refused to fulfill it.*/public static final int SC_FORBIDDEN = 403;/*** Status code (404) indicating that the requested resource is not* available.*/public static final int SC_NOT_FOUND = 404;/*** Status code (405) indicating that the method specified in the* <code><em>Request-Line</em></code> is not allowed for the resource* identified by the <code><em>Request-URI</em></code>.*/public static final int SC_METHOD_NOT_ALLOWED = 405;/*** Status code (406) indicating that the resource identified by the request* is only capable of generating response entities which have content* characteristics not acceptable according to the accept headers sent in* the request.*/public static final int SC_NOT_ACCEPTABLE = 406;/*** Status code (407) indicating that the client <em>MUST</em> first* authenticate itself with the proxy.*/public static final int SC_PROXY_AUTHENTICATION_REQUIRED = 407;/*** Status code (408) indicating that the client did not produce a request* within the time that the server was prepared to wait.*/public static final int SC_REQUEST_TIMEOUT = 408;/*** Status code (409) indicating that the request could not be completed due* to a conflict with the current state of the resource.*/public static final int SC_CONFLICT = 409;/*** Status code (410) indicating that the resource is no longer available at* the server and no forwarding address is known. This condition* <em>SHOULD</em> be considered permanent.*/public static final int SC_GONE = 410;/*** Status code (411) indicating that the request cannot be handled without a* defined <code><em>Content-Length</em></code>.*/public static final int SC_LENGTH_REQUIRED = 411;/*** Status code (412) indicating that the precondition given in one or more* of the request-header fields evaluated to false when it was tested on the* server.*/public static final int SC_PRECONDITION_FAILED = 412;/*** Status code (413) indicating that the server is refusing to process the* request because the request entity is larger than the server is willing* or able to process.*/public static final int SC_REQUEST_ENTITY_TOO_LARGE = 413;/*** Status code (414) indicating that the server is refusing to service the* request because the <code><em>Request-URI</em></code> is longer than the* server is willing to interpret.*/public static final int SC_REQUEST_URI_TOO_LONG = 414;/*** Status code (415) indicating that the server is refusing to service the* request because the entity of the request is in a format not supported by* the requested resource for the requested method.*/public static final int SC_UNSUPPORTED_MEDIA_TYPE = 415;/*** Status code (416) indicating that the server cannot serve the requested* byte range.*/public static final int SC_REQUESTED_RANGE_NOT_SATISFIABLE = 416;/*** Status code (417) indicating that the server could not meet the* expectation given in the Expect request header.*/public static final int SC_EXPECTATION_FAILED = 417;/*** Status code (500) indicating an error inside the HTTP server which* prevented it from fulfilling the request.*/public static final int SC_INTERNAL_SERVER_ERROR = 500;/*** Status code (501) indicating the HTTP server does not support the* functionality needed to fulfill the request.*/public static final int SC_NOT_IMPLEMENTED = 501;/*** Status code (502) indicating that the HTTP server received an invalid* response from a server it consulted when acting as a proxy or gateway.*/public static final int SC_BAD_GATEWAY = 502;/*** Status code (503) indicating that the HTTP server is temporarily* overloaded, and unable to handle the request.*/public static final int SC_SERVICE_UNAVAILABLE = 503;/*** Status code (504) indicating that the server did not receive a timely* response from the upstream server while acting as a gateway or proxy.*/public static final int SC_GATEWAY_TIMEOUT = 504;/*** Status code (505) indicating that the server does not support or refuses* to support the HTTP protocol version that was used in the request* message.*/public static final int SC_HTTP_VERSION_NOT_SUPPORTED = 505;
}
Servlet生命周期
-
客户端请求该 Servlet;
- 2.
加载 Servlet 类到内存;
- 3.
实例化并调用init()方法初始化该 Servlet;
- 4.
service()(根据请求方法不同调用doGet() 或者 doPost(),此外还有doHead()、doPut()、doTrace()、doDelete()、doOptions()、destroy())。
- 5.
加载和实例化 Servlet。这项操作一般是动态执行的。然而,Server 通常会提供一个管理的选项,用于在 Server 启动时强制装载和初始化特定的 Servlet。
Server 创建一个 Servlet的实例
第一个客户端的请求到达 Server
Server 调用 Servlet 的 init() 方法(可配置为 Server 创建 Servlet 实例时调用,在 web.xml 中 标签下配置 标签,配置的值为整型,值越小 Servlet 的启动优先级越高)
一个客户端的请求到达 Server
Server 创建一个请求对象,处理客户端请求
Server 创建一个响应对象,响应客户端请求
Server 激活 Servlet 的 service() 方法,传递请求和响应对象作为参数
service() 方法获得关于请求对象的信息,处理请求,访问其他资源,获得需要的信息
service() 方法使用响应对象的方法,将响应传回Server,最终到达客户端。service()方法可能激活其它方法以处理请求,如 doGet() 或 doPost() 或程序员自己开发的新的方法。
对于更多的客户端请求,Server 创建新的请求和响应对象,仍然激活此 Servlet 的 service() 方法,将这两个对象作为参数传递给它。如此重复以上的循环,但无需再次调用 init() 方法。一般 Servlet 只初始化一次(只有一个对象),当 Server 不再需要 Servlet 时(一般当 Server 关闭时),Server 调用 Servlet 的 destroy() 方法。
相关文章:

Java web与Java中的Servlet
一。前言 Java语言大多用于开发web系统的后端,也就是我们是的B/S架构。通过浏览器一个URL去访问系统的后端资源和逻辑。 当我在代码里看到这个类HttpServletRequest 时 让我想到了Servlet,Servlet看上去多么像是Java的一个普通类,但是它确实…...

kafka常用目录文件解析
文章目录 1、消息日志文件(.log)2、消费者偏移量文件(__consumer_offsets)3、偏移量索引文件(.index)4、时间索引文件( .timeindex)5、检查点引文件( .checkpoint&#x…...

RV1126+FFMPEG推流项目源码
源码在我的gitee上面,感兴趣的可以自行了解 nullhttps://gitee.com/x-lan/rv126-ffmpeg-streaming-projecthttps://gitee.com/x-lan/rv126-ffmpeg-streaming-project...

ANSYS SimAI
ANSYS SimAI 是 ANSYS 公司推出的一款基于人工智能(AI)的仿真解决方案,旨在通过机器学习技术加速仿真流程,降低计算资源需求,并为用户提供更高效的工程决策支持。其核心目标是简化复杂仿真过程,帮助工程师快…...
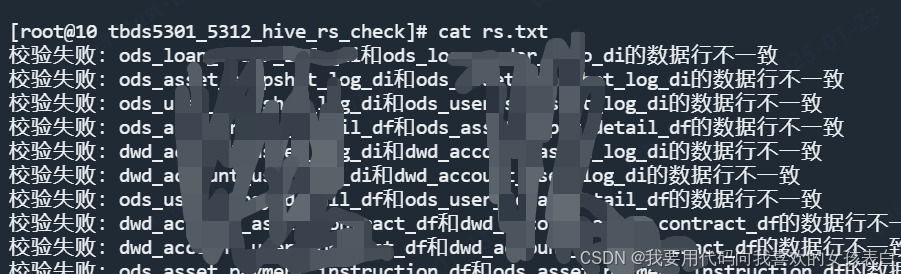
hedfs和hive数据迁移后校验脚本
先谈论校验方法,本人腾讯云大数据工程师。 1、hdfs的校验 这个通常就是distcp校验,hdfs通过distcp迁移到另一个集群,怎么校验你的对不对。 有人会说,默认会有校验CRC校验。我们关闭了,为什么关闭?全量迁…...
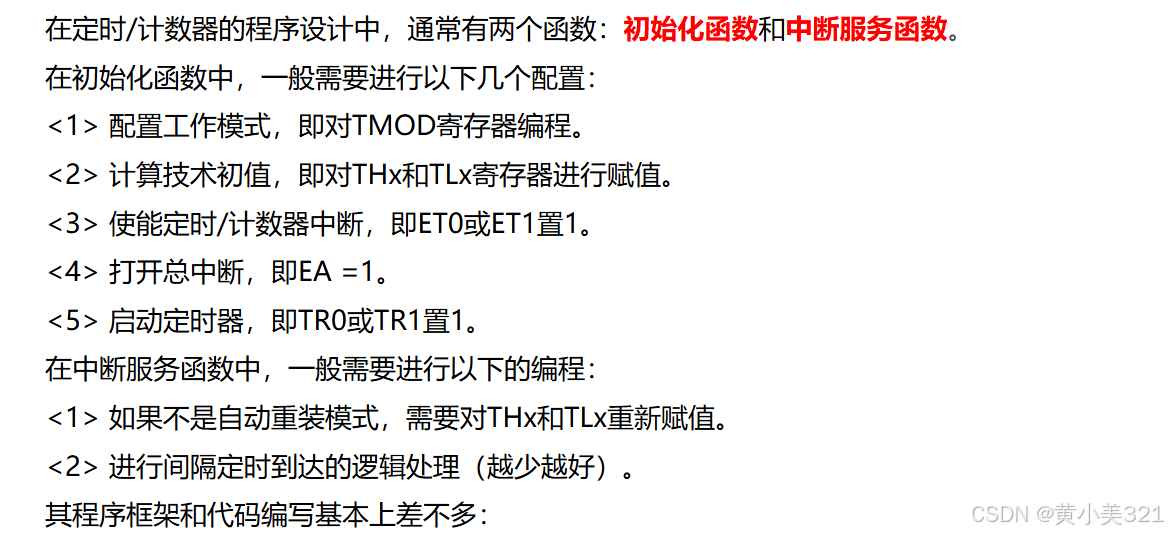
蓝桥杯单片机(八)定时器的基本原理与应用
模块训练: 当有长定时情况时,也就是定时长度超过65.5ms时,采用多次定时累加 一、定时器介绍 1.单片机的定时/计数器 2.定时器工作原理 3.定时器相关寄存器 二、定时器使用程序设计 1.程序设计思路 与写中断函数一样,先写一个初…...
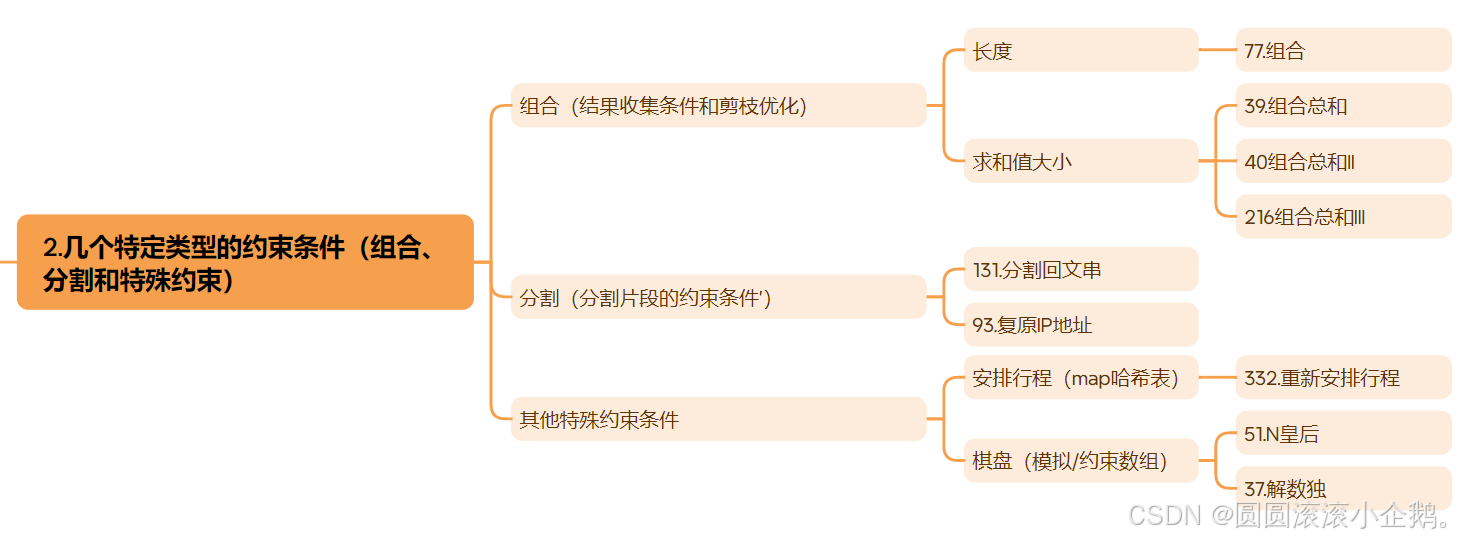
刷题总结 回溯算法
为了方便复习并且在把算法忘掉的时候能尽量快速的捡起来 刷完回溯算法这里需要做个总结 回溯算法的适用范围 回溯算法是深度优先搜索(DFS)的一种特定应用,在DFS的基础上引入了约束检查和回退机制。 相比于普通的DFS,回溯法的优…...

C++ 静态变量static的使用方法
static概述: static关键字有三种使用方式,其中前两种只指在C语言中使用,第三种在C中使用。 静态局部变量(C) 静态全局变量/函数(C) 静态数据成员/成员函数(C) 静态局部变量 静态局部变量&…...
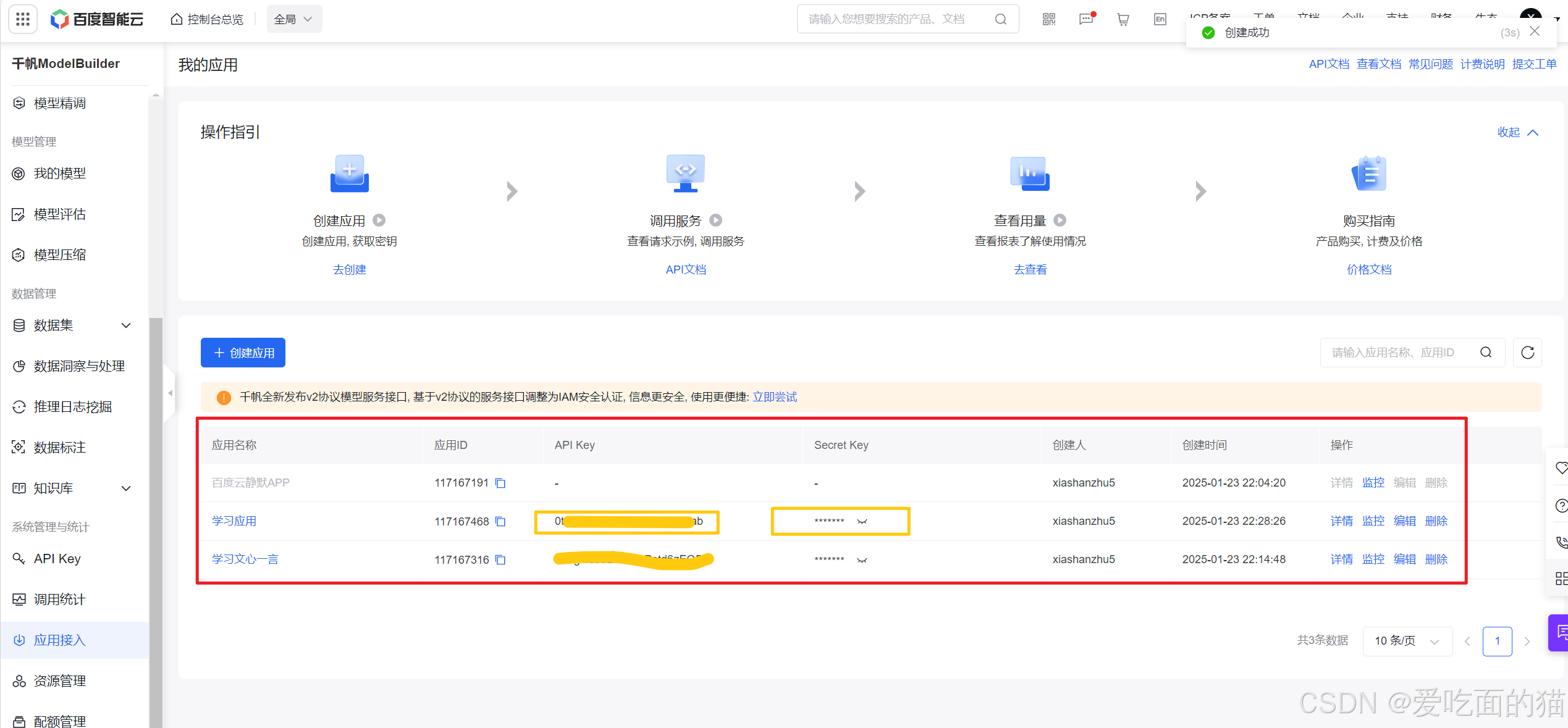
Langchain+文心一言调用
import osfrom langchain_community.llms import QianfanLLMEndpointos.environ["QIANFAN_AK"] "" os.environ["QIANFAN_SK"] ""llm_wenxin QianfanLLMEndpoint()res llm_wenxin.invoke("中国国庆日是哪一天?") print(…...

20250124 Flink中 窗口开始时间和結束時間
增量聚合的 ProcessWindowFunction # ProcessWindowFunction 可以与 ReduceFunction 或 AggregateFunction 搭配使用, 使其能够在数据到达窗口的时候进行增量聚合。当窗口关闭时,ProcessWindowFunction 将会得到聚合的结果。 这样它就可以增量聚合窗口的…...
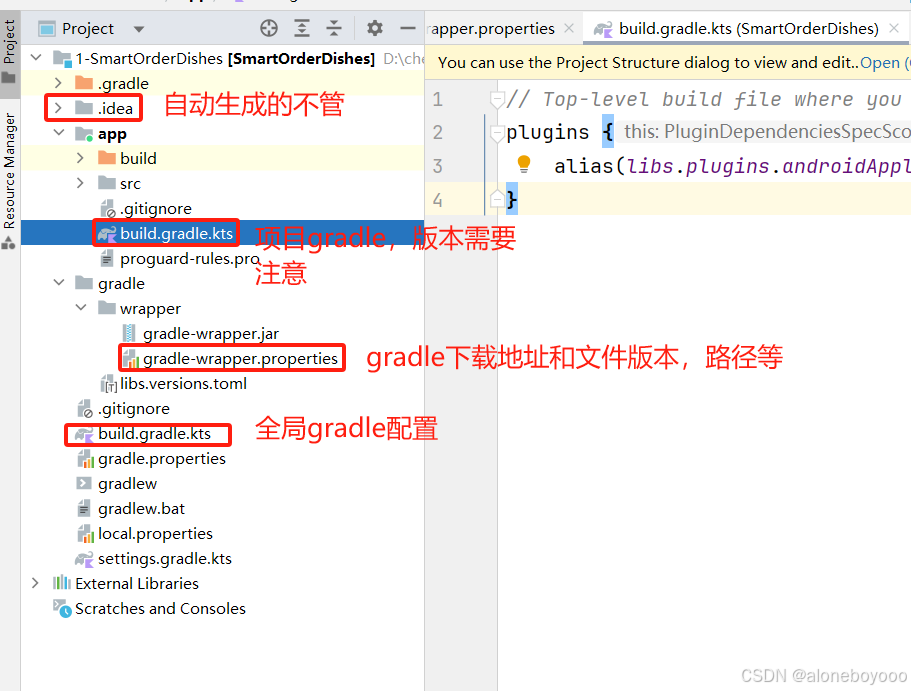
Android Studio安装配置
一、注意事项 想做安卓app和开发板通信,踩了大坑,Android 开发不是下载了就能直接开发的,对于新手需要注意的如下: 1、Android Studio版本,根据自己的Android Studio版本对应决定了你所兼容的AGP(Android…...
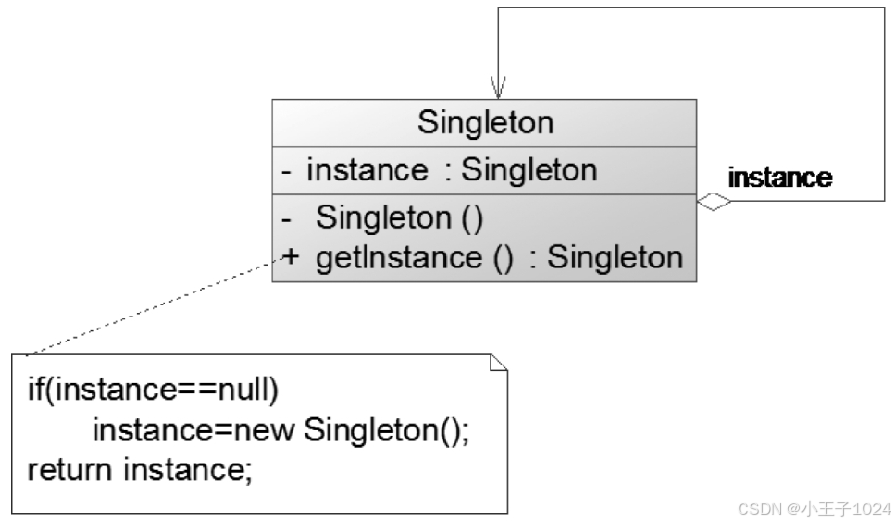
设计模式Python版 单例模式
文章目录 前言一、单例模式二、单例模式实现方式三、单例模式示例四、单例模式在Django框架的应用 前言 GOF设计模式分三大类: 创建型模式:关注对象的创建过程,包括单例模式、简单工厂模式、工厂方法模式、抽象工厂模式、原型模式和建造者模…...
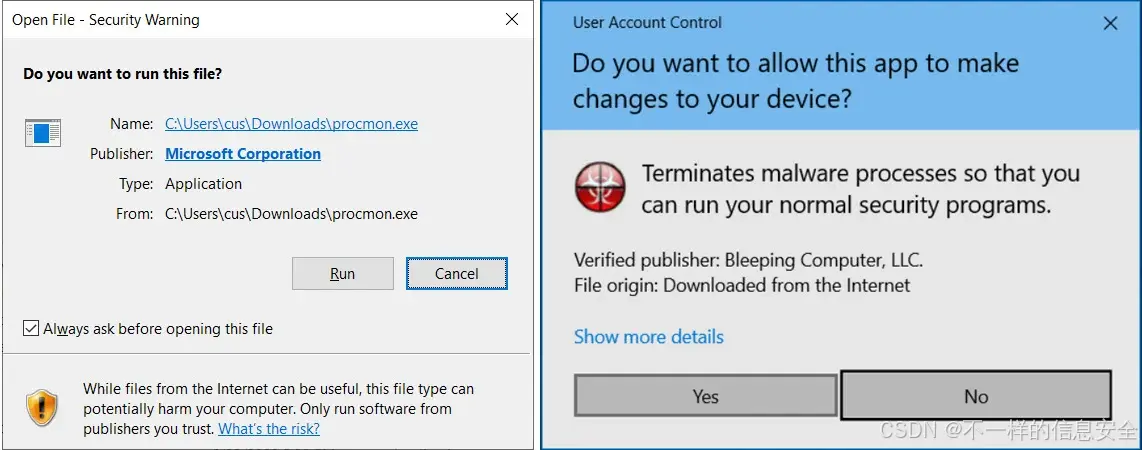
7-Zip高危漏洞CVE-2025-0411:解析与修复
7-Zip高危漏洞CVE-2025-0411:解析与修复 免责声明 本系列工具仅供安全专业人员进行已授权环境使用,此工具所提供的功能只为网络安全人员对自己所负责的网站、服务器等(包括但不限于)进行检测或维护参考,未经授权请勿利…...
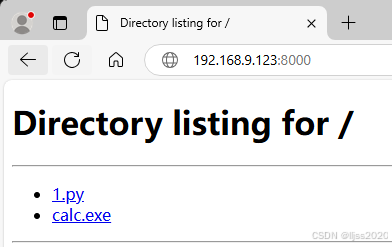
python实现http文件服务器访问下载
//1.py import http.server import socketserver import os import threading import sys# 获取当前脚本所在的目录 DIRECTORY os.path.dirname(os.path.abspath(__file__))# 设置服务器的端口 PORT 8000# 自定义Handler,将根目录设置为脚本所在目录 class MyHTT…...

《一文讲透》第4期:KWDB 数据库运维(6)—— 容灾与备份
一、KWDB 容灾 WAL 概述 KWDB 采用预写式日志(Write-Ahead Logging,WAL),记录每个时序表的模式变更和数据变更,以实现时序数据库的数据灾难恢复、时序数据的一致性和原子性。 KWDB 默认会将保存在 WAL 日志缓存中的…...
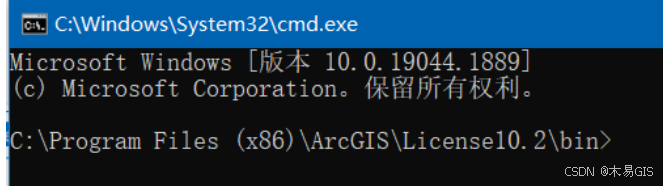
ArcGIS10.2 许可License点击始终启动无响应的解决办法及正常启动的前提
1、问题描述 在ArcGIS License Administrator中,手动点击“启动”无响应;且在计算机管理-服务中,无ArcGIS License 或者License的启动、停止、禁止等均为灰色,无法操作。 2、解决方法 ①通过cmd对service.txt进行手动服务的启动…...

Level2逐笔成交逐笔委托毫秒记录:今日分享优质股票数据20250124
逐笔成交逐笔委托下载 链接: https://pan.baidu.com/s/1UWVY11Q1IOfME9itDN5aZA?pwdhgeg 提取码: hgeg Level2逐笔成交逐笔委托数据分享下载 通过Level2逐笔成交与逐笔委托的详细数据,这种以毫秒为单位的信息能揭示许多关键点,如庄家意图、误导性行为…...
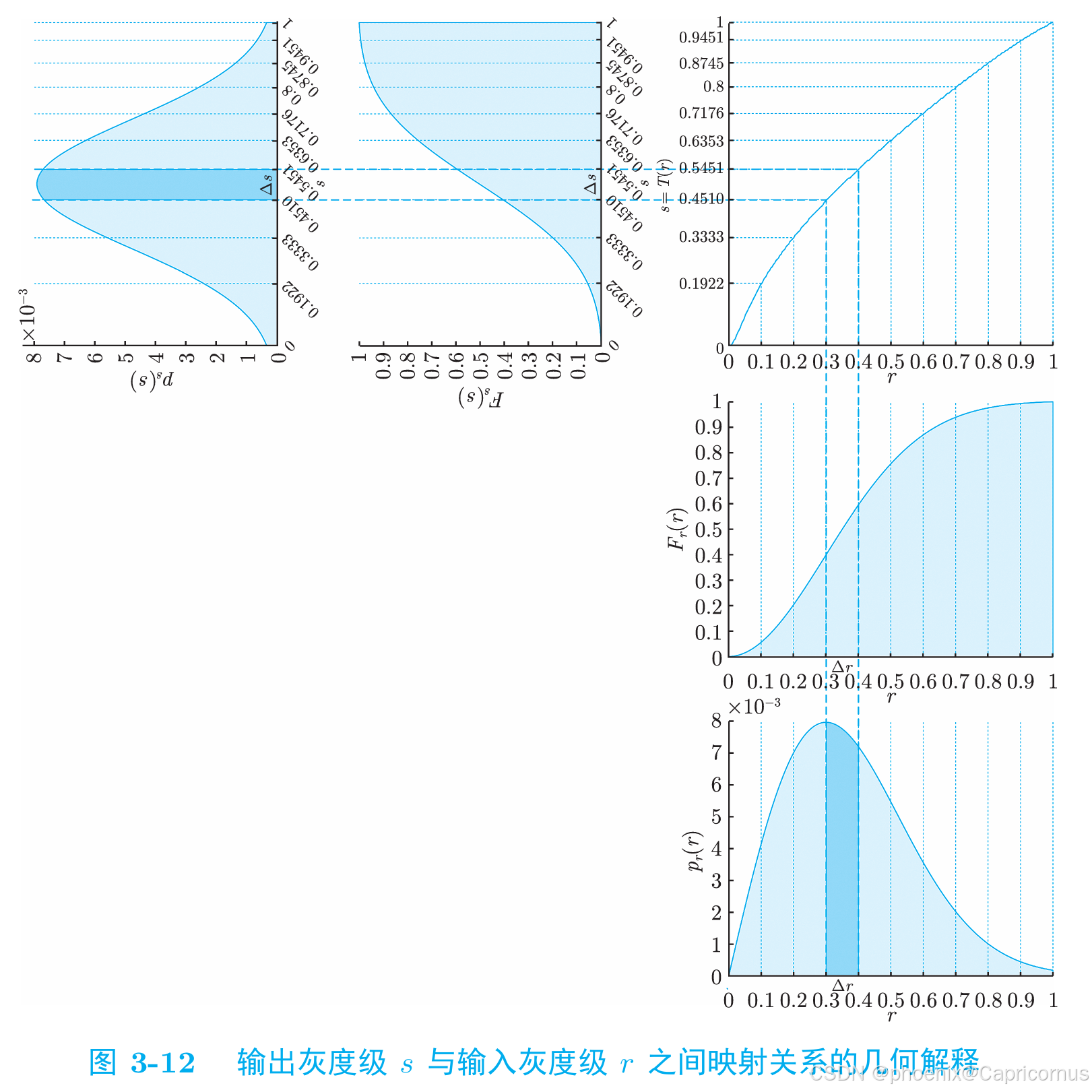
概率密度函数(PDF)分布函数(CDF)——直方图累积直方图——直方图规定化的数学基础
对于连续型随机变量,分布函数(Cumulative Distribution Function, CDF)是概率密度函数(Probability Density Function, PDF)的变上限积分,概率密度函数是分布函数的导函数。 如果我们有一个连续型随机变量…...
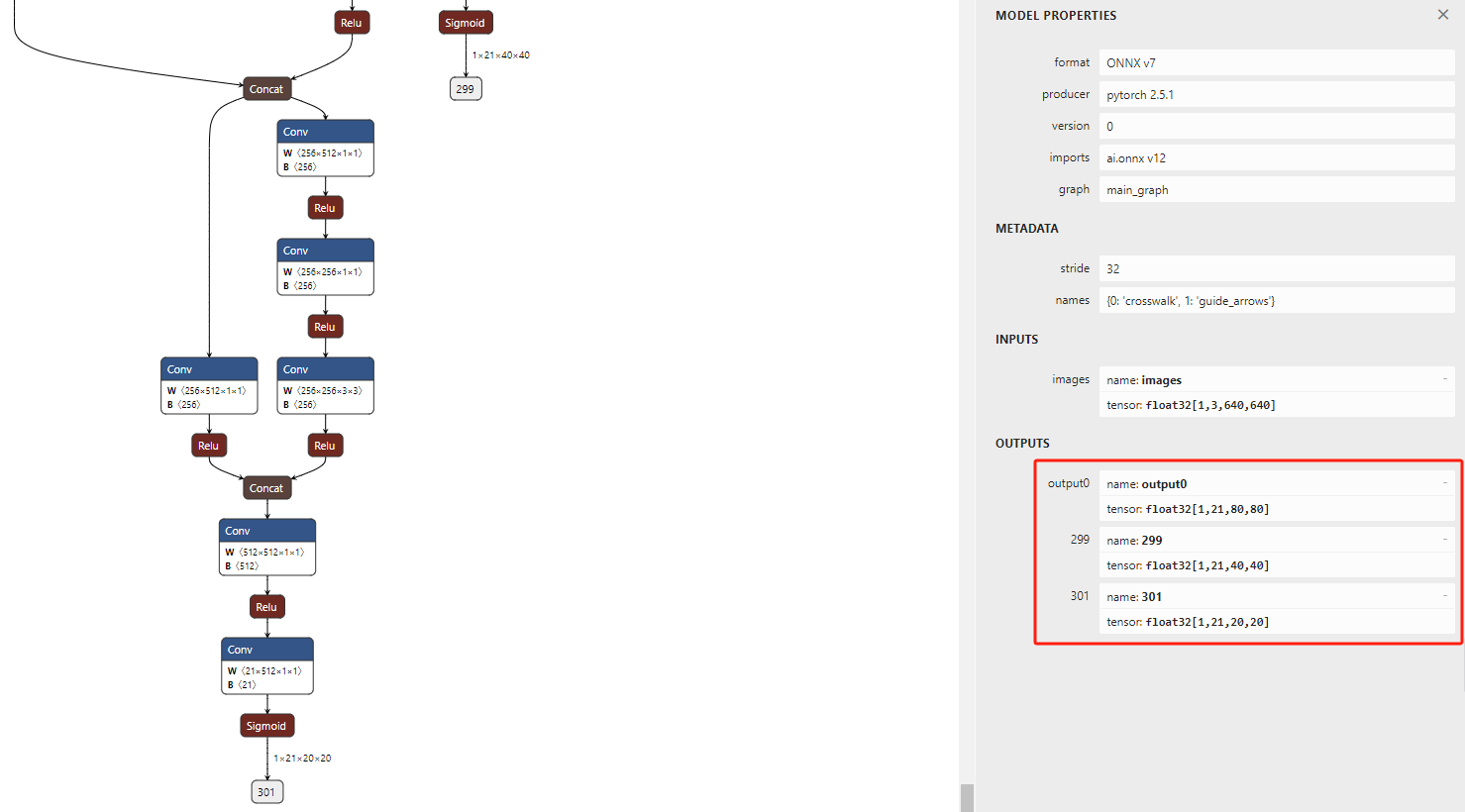
YOLOv5训练自己的数据及rknn部署
YOLOv5训练自己的数据及rknn部署 一、下载源码二、准备自己的数据集2.1 标注图像2.2 数据集结构 三、配置YOLOv5训练3.1 修改配置文件3.2 模型选择 四、训练五、测试六、部署6.1 pt转onnx6.2 onnx转rknn 七、常见错误7.1 训练过程中的错误7.1.1 cuda: out of memory7.1.2 train…...
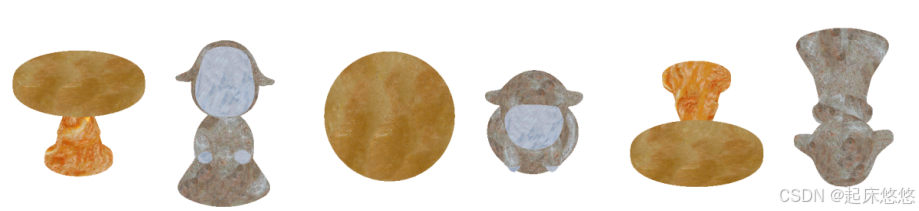
计算机图形学:实验四 带纹理的OBJ文件读取和显示
一、程序功能设计 在程序中读取带纹理的obj文件,载入相应的纹理图片文件,将带纹理的模型显示在程序窗口中。实现带纹理的OBJ文件读取与显示功能,具体设计如下: OBJ文件解析与数据存储 通过实现TriMesh类中的readObj函数&#x…...

SQL Server 使用SELECT INTO实现表备份
在数据库管理过程中,有时我们需要对表进行备份,以防数据丢失或修改错误。在 SQL Server 中,可以使用 SELECT INTO 语句将数据从一个表备份到另一个表。 备份表的 SQL 语法: SELECT * INTO 【备份表名】 FROM 【要备份的表】 SEL…...
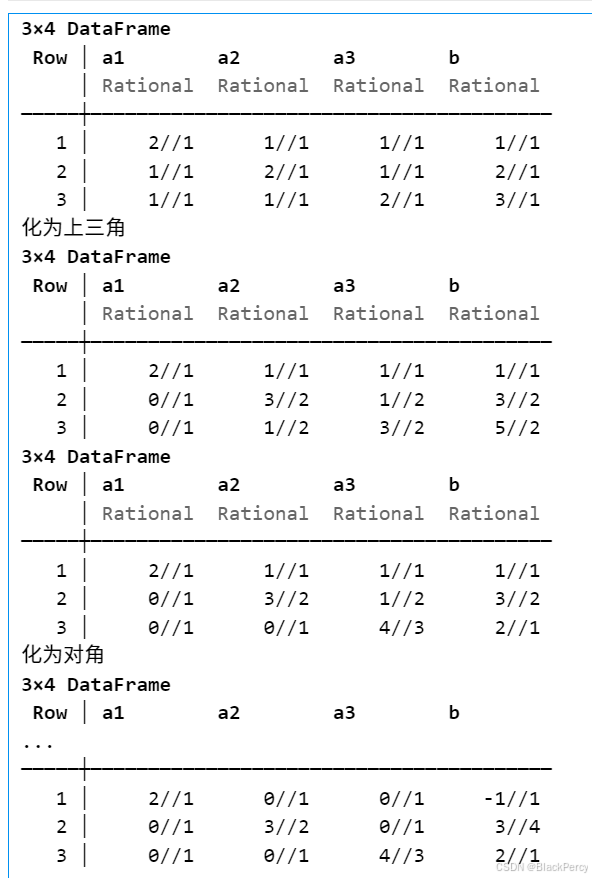
【线性代数】基础版本的高斯消元法
[精确算法] 高斯消元法求线性方程组 线性方程组 考虑线性方程组, 已知 A ∈ R n , n , b ∈ R n A\in \mathbb{R}^{n,n},b\in \mathbb{R}^n A∈Rn,n,b∈Rn, 求未知 x ∈ R n x\in \mathbb{R}^n x∈Rn A 1 , 1 x 1 A 1 , 2 x 2 ⋯ A 1 , n x n b 1…...

Python标准库 threading 的 start 和 join 的使用
python 的多线程机制可以的适用场景不适合与计算密集型的,因为 GIL 的存在,多线程在处理计算密集型时,实际上也是串行的,因为每个时刻只有一个线程可以获得 GIL,但是对于 IO 处理来说,不管是网络IO还是文件…...
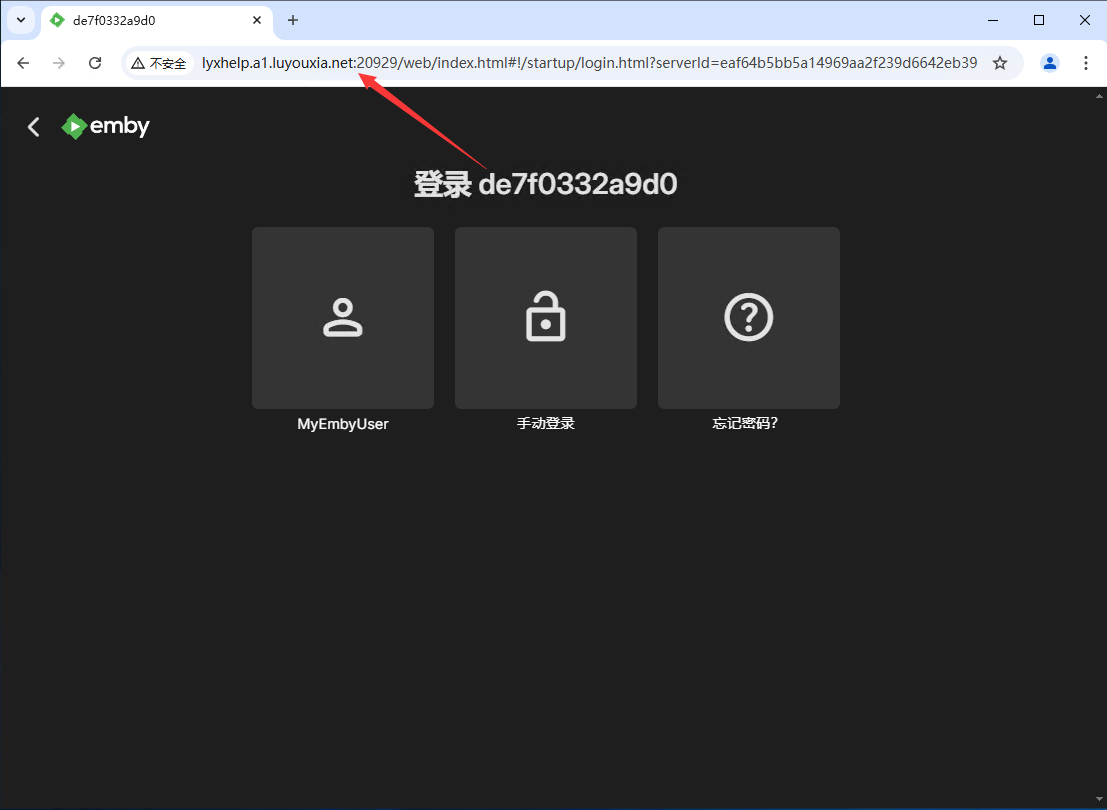
无公网IP 外网访问媒体服务器 Emby
Emby 是一款多媒体服务器软件,用户可以在 Emby 创建自己的个人多媒体娱乐中心,并且可以跨多个设备访问自己的媒体库。它允许用户管理传输自己的媒体内容,比如电影、电视节目、音乐和照片等。 本文将详细的介绍如何利用 Docker 在本地部署 Emb…...
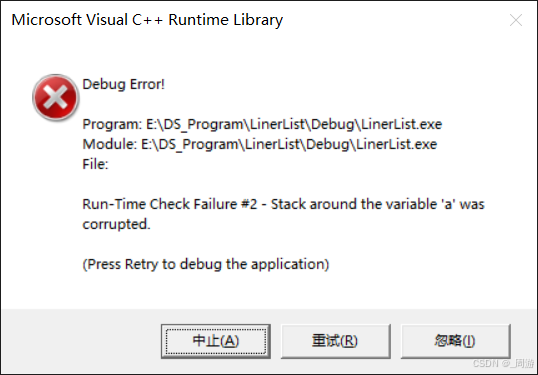
【数据结构】_顺序表
目录 1. 概念与结构 1.1 静态顺序表 1.2 动态顺序表 2. 动态顺序表实现 2.1 SeqList.h 2.2 SeqList.c 2.3 Test_SeqList.c 3. 顺序表性能分析 线性表是n个具有相同特性的数据元素的有限序列。 常见的线性表有:顺序表、链表、栈、队列、字符串等;…...

[MySQL]数据库表内容的增删查改操作大全
目录 一、增加表数据 1.全列插入与指定列插入 2.多行数据插入 3.更新与替换插入 二、查看表数据 1.全列查询与指定列查询 2.查询表达式字段 3.为查询结果起别名 4.结果去重 5.WHERE条件 6.结果排序 7.筛选分页结果 8.插入查询的结果 9.group by子句 三、修改表数…...

解决双系统引导问题:Ubuntu 启动时不显示 Windows 选项的处理方法
方法 1:检查 GRUB 引导菜单是否隐藏 启动进入 Ubuntu 系统。打开终端,输入以下命令编辑 GRUB 配置文件:sudo nano /etc/default/grub检查以下配置项: GRUB_TIMEOUT0:如果是 0,将其改为一个较大的值&#x…...
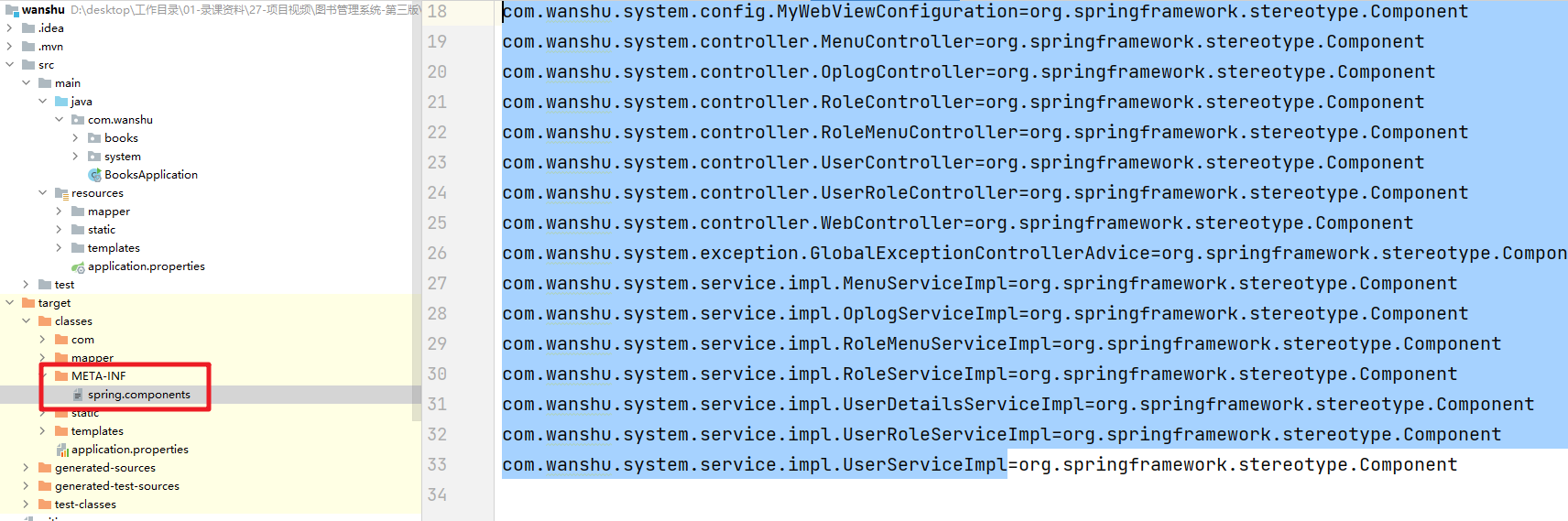
Java面试题2025-Spring
讲师:邓澎波 Spring面试专题 1.Spring应该很熟悉吧?来介绍下你的Spring的理解 1.1 Spring的发展历程 先介绍Spring是怎么来的,发展中有哪些核心的节点,当前的最新版本是什么等 通过上图可以比较清晰的看到Spring的各个时间版本对…...

CentOS7安装使用containerd
一,安装 1.1、安装containerd 下载 https://github.com/containerd/containerd/releases/download/v1.7.24/cri-containerd-cni-1.7.24-linux-amd64.tar.gz wget https://github.com/containerd/containerd/releases/download/v1.7.24/cri-containerd-cni-1.7.24-…...

Redis 集群模式入门
Redis 集群模式入门 一、简介 Redis 有三种集群模式:主从模式、Sentinel 哨兵模式、cluster 分片模式 主从复制(Master-Slave Replication): 在这种模式下,数据可以从一个 Redis 实例(主节点 Master)复…...