混合图像python旗舰版
仔细看这个图像。然后后退几米再看。你看到了什么?
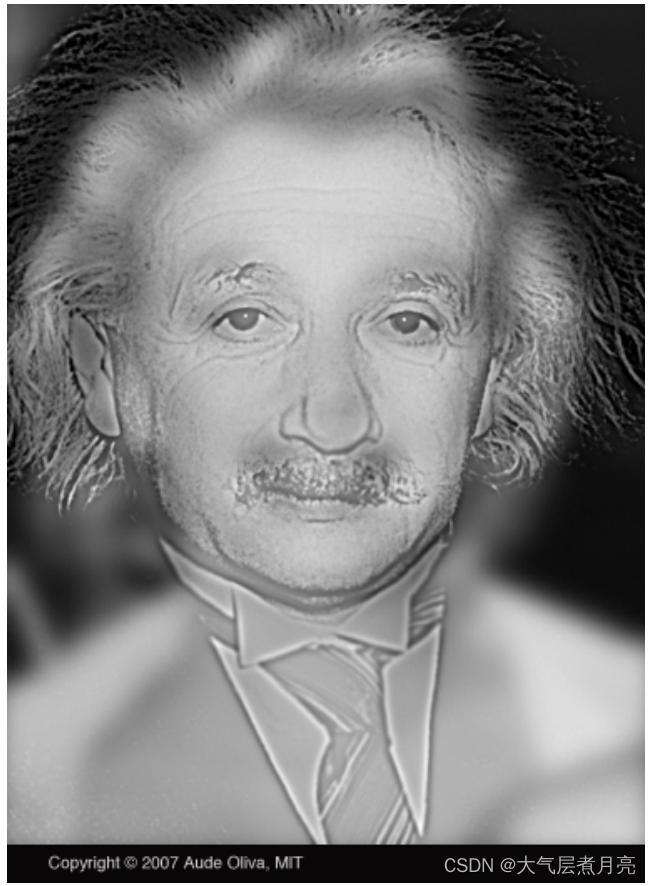
混合图像是指将一张图片的低频与另一张图片的高频相结合的图片。根据观看距离的不同,所得到的图像有两种解释。在上面的图片中,你可以看到阿尔伯特·爱因斯坦,一旦你离开屏幕或缩小观众的图像大小,他就变成了玛丽莲·梦露。这个概念是在2006年的论文中提出的。为了实现这一效果,您必须实现低通和高通滤波操作来应用于您选择的两幅图像,并线性组合过滤后的图像,得到具有所需的两种解释的混合图像,即最后将只有低频信息的图片和只有高频信息的图像叠加在一起。
对于图像的低频部分:可以理解为图像的“轮廓”,比如一幅画的线条等。
对于图像的高频部分:可以理解为图像的“细节”,比如一幅画的颜色搭配,颜色深度等。
值得一提的是:对图像做模糊处理后得到了图像的低频部分,对图像做锐化处理会让图像的高频信息更多。
实现过滤功能
步骤
您的目标是在hybrid.py中实现以下函数:
cross_correlation_2d:实现了你的过滤功能的核心;
convolve_2d:必须使用cross_correlation_2d功能;
gaussian_blur_kernel_2d:你在这里创建的高斯核,与convolve_2d配对,创建一个高斯模糊滤波器;
low_pass:从图像中删除细节,你的实现必须使用高斯模糊;
high_pass:保留很细的细节和删除低频,您的实现必须使用高斯模糊作为一个子例程。
注意,您必须从头开始实现所有的函数,只使用基本的矩阵操作,而任何来自NumPy、OpenCV、Scipy或类似包的任何过滤函数都是禁止的。功能,如填充,创建网格网格,等。被认为是基本的操作,如果您想快速编写代码并避免多个嵌套的Python循环,则是允许的。
生成混合图像
一旦在hybrid.py中实现了函数,使用提供的创建混合图像。然而,创建一个被我们的大脑很好地解释的混合图像的一个重要因素是对齐两个图像的显著特征。注意:如果您使用多个嵌套的Python循环来实现过滤操作,那么您的函数可能会非常慢。在更改参数后,您必须保持耐心,或者使用基本的矩阵功能来更加努力地优化代码。
最终,你应该得到一张像下面这样的图片:
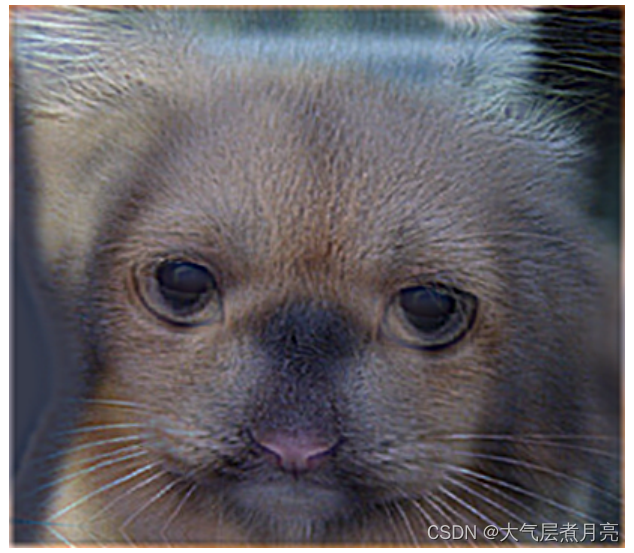
import cv2
import numpy as npdef cross_correlation_2d(img, kernel):'''Given a kernel of arbitrary m x n dimensions, with both m and n beingodd, compute the cross correlation of the given image with the givenkernel, such that the output is of the same dimensions as the image and thatyou assume the pixels out of the bounds of the image to be zero. Note thatyou need to apply the kernel to each channel separately, if the given imageis an RGB image.Inputs:img: Either an RGB image (height x width x 3) or a grayscale image(height x width) as a numpy array.kernel: A 2D numpy array (m x n), with m and n both odd (but may not beequal).Output:Return an image of the same dimensions as the input image (same width,height and the number of color channels)'''# TODO-BLOCK-BEGIN# rotating kernel with 180 degreeskernel = np.rot90(kernel, 2)kernel_heigh = int(np.array(kernel).shape[0])kernel_width = int(np.array(kernel).shape[1])# set kernel matrix to random int matrixif ((kernel_heigh % 2 != 0) & (kernel_width % 2 != 0)): # make sure that the scale of kernel is odd# the scale of resultconv_heigh = img.shape[0] - kernel.shape[0] + 1conv_width = img.shape[1] - kernel.shape[1] + 1conv = np.zeros((conv_heigh, conv_width))# convolvefor i in range(int(conv_heigh)):for j in range(int(conv_width )):result = (img[i:i + kernel_heigh, j:j + kernel_width] * kernel).sum()if(result<0):result = 0elif(result>255):result = 255conv[i][j] = resultreturn convelse: raise Exception('make sure that the scale of kernel is odd')# raise Exception("TODO in hybrid.py not implemented")# TODO-BLOCK-ENDdef convolve_2d(img, kernel):'''Use cross_correlation_2d() to carry out a 2D convolution.Inputs:img: Either an RGB image (height x width x 3) or a grayscale image(height x width) as a numpy array.kernel: A 2D numpy array (m x n), with m and n both odd (but may not beequal).Output:Return an image of the same dimensions as the input image (same width,height and the number of color channels)'''# TODO-BLOCK-BEGIN# zero paddingkernel_half_row = int((kernel.shape[0]-1)/2)kernel_half_col = int((kernel.shape[1]-1)/2)# judge how many channelsif len(img.shape) == 3:img = np.pad(img, ((kernel_half_row, kernel_half_row), (kernel_half_col, kernel_half_col),(0, 0)), 'constant', constant_values=0)# if image.shape[2] == 3 or image.shape[2] == 4:# if style is png, there will be four channels, but we just need to use the first three# if the style is bmp or jpg, there will be three channelsimage_r = img[:, :, 0]image_g = img[:, :, 1]image_b = img[:, :, 2]result_r = cross_correlation_2d(image_r, kernel)result_g = cross_correlation_2d(image_g, kernel)result_b = cross_correlation_2d(image_b, kernel)result_picture = np.dstack([result_r, result_g, result_b])# if the picture is black and whiteelif len(img.shape) == 2:img = np.pad(img, ((kernel_half_row, kernel_half_row), (kernel_half_col, kernel_half_col)), 'constant', constant_values=0)result_picture = cross_correlation_2d(img, kernel)# returns the convolved image (of the same shape as the input image)return result_picture# raise Exception("TODO in hybrid.py not implemented")# TODO-BLOCK-ENDdef gaussian_blur_kernel_2d(sigma, height, width):'''Return a Gaussian blur kernel of the given dimensions and with the givensigma. Note that width and height are different.Input:sigma: The parameter that controls the radius of the Gaussian blur.Note that, in our case, it is a circular Gaussian (symmetricacross height and width).width: The width of the kernel.height: The height of the kernel.Output:Return a kernel of dimensions height x width such that convolving itwith an image results in a Gaussian-blurred image.'''# TODO-BLOCK-BEGINm,n = [(ss-1.)/2. for ss in (height, width)]y, x = np.ogrid[-m:m+1, -n:n+1]h = np.exp( - (x*x + y*y) / (2.*sigma*sigma))h[ h < np.finfo(h.dtype).eps*h.max()] = 0sumh = h.sum()if sumh != 0:h /= sumhreturn h# raise Exception("TODO in hybrid.py not implemented")# TODO-BLOCK-ENDdef low_pass(img, sigma, size):'''Filter the image as if its filtered with a low pass filter of the givensigma and a square kernel of the given size. A low pass filter supressesthe higher frequency components (finer details) of the image.Output:Return an image of the same dimensions as the input image (same width,height and the number of color channels)'''# TODO-BLOCK-BEGIN# make kernellow_kernel = gaussian_blur_kernel_2d(sigma, size, size)# convolve low-pass pictureslow_image = convolve_2d(img, low_kernel)return low_image# raise Exception("TODO in hybrid.py not implemented")# TODO-BLOCK-ENDdef high_pass(img, sigma, size):'''Filter the image as if its filtered with a high pass filter of the givensigma and a square kernel of the given size. A high pass filter suppressesthe lower frequency components (coarse details) of the image.Output:Return an image of the same dimensions as the input image (same width,height and the number of color channels)'''# TODO-BLOCK-BEGIN# make kernelhigh_kernel = gaussian_blur_kernel_2d(sigma, size, size)# make high-pass picturehigh_image = (img - convolve_2d(img, high_kernel))return high_image# raise Exception("TODO in hybrid.py not implemented")# TODO-BLOCK-ENDdef create_hybrid_image(img1, img2, sigma1, size1, high_low1, sigma2, size2,high_low2, mixin_ratio, scale_factor):'''This function adds two images to create a hybrid image, based onparameters specified by the user.'''high_low1 = high_low1.lower()high_low2 = high_low2.lower()if img1.dtype == np.uint8:img1 = img1.astype(np.float32) / 255.0img2 = img2.astype(np.float32) / 255.0if high_low1 == 'low':img1 = low_pass(img1, sigma1, size1)else:img1 = high_pass(img1, sigma1, size1)if high_low2 == 'low':img2 = low_pass(img2, sigma2, size2)else:img2 = high_pass(img2, sigma2, size2)img1 *= (1 - mixin_ratio)img2 *= mixin_ratiocv2.imshow('img1', img1)cv2.imshow('img2', img2)cv2.imwrite('high_left.png', img1)cv2.imwrite('low_right.png', img2)hybrid_img = (img1 + img2) * scale_factorreturn (hybrid_img * 255).clip(0, 255).astype(np.uint8)if __name__ == "__main__":hybrid_image = create_hybrid_image(img1=cv2.imread(r'resources\cat.jpg'),img2=cv2.imread(r'resources\dog.jpg'),sigma1=7,size1=29,high_low1='high',sigma2=7.0,size2=29,high_low2='low',mixin_ratio=0.5,scale_factor=1)cv2.imshow('hybrid_image', hybrid_image)cv2.waitKey(0)cv2.imwrite('hybrid_image.png', hybrid_image)
相关文章:
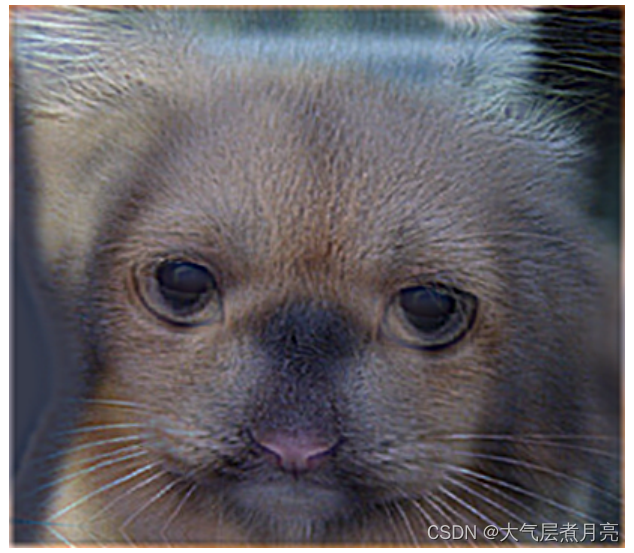
混合图像python旗舰版
仔细看这个图像。然后后退几米再看。你看到了什么?混合图像是指将一张图片的低频与另一张图片的高频相结合的图片。根据观看距离的不同,所得到的图像有两种解释。在上面的图片中,你可以看到阿尔伯特爱因斯坦,一旦你离开屏幕或缩小…...
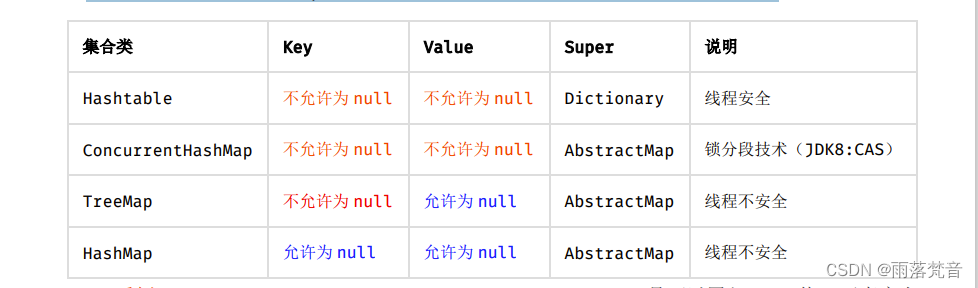
开发手册——一、编程规约_5.集合处理
这篇文章主要梳理了在java的实际开发过程中的编程规范问题。本篇文章主要借鉴于《阿里巴巴java开发手册终极版》 下面我们一起来看一下吧。 1. 【强制】关于 hashCode 和 equals 的处理,遵循如下规则: 只要重写 equals,就必须重写 hashCod…...
【elastic】elastic高可用集群部署
文章目录前言一、资源分享1、包含源码包、配置文件二、部署过程三、报错锦集四、es的部分相关命令前言 本博客内容仅为记录博主思路,仅供参考,一切以自己实践结果为准。 一、资源分享 1、包含源码包、配置文件 链接:https://pan.baidu.com…...
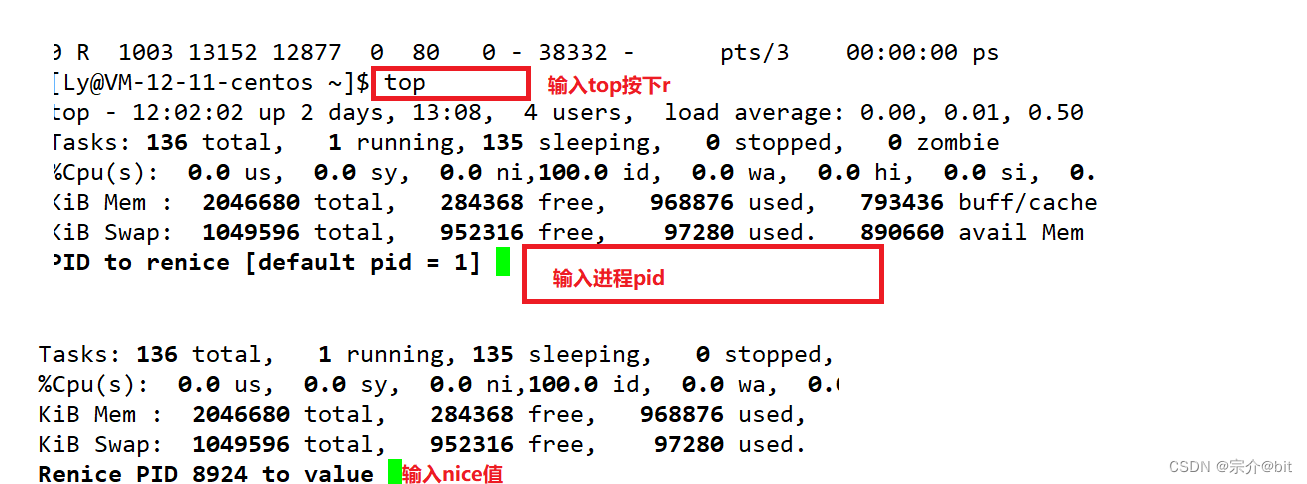
初识Liunx下的进程状态和环境变量以及进程优先级
文章目录前言1.进程状态1.阻塞与挂起2.Linux下的进程状态1.概念知识2.R状态2.休眠状态(S/D)3.T状态4.Z状态(僵尸进程)和X状态5.孤儿进程3.环境变量1.概念2.获取环境变量1.环境变量表2.函数获取环境变量3.关于环境变量的理解和main函数中的两个参数1.环境变量的理解2…...
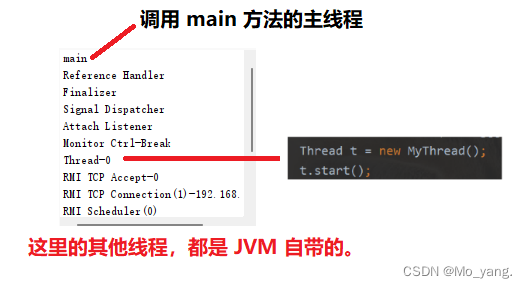
JavaEE——何为线程及创建线程
文章目录一、认识线程1. 线程的概念2. 出现多线程的原因3. 进程与线程4. 对多线程的详细解释二、初次实现多线程代码1. 初步了解2. 使用 Java 中的工具查看当前的所有线程3. Java 中创建线程的多种方式一、认识线程 1. 线程的概念 所谓线程,就是指在一个 ‘执行流…...
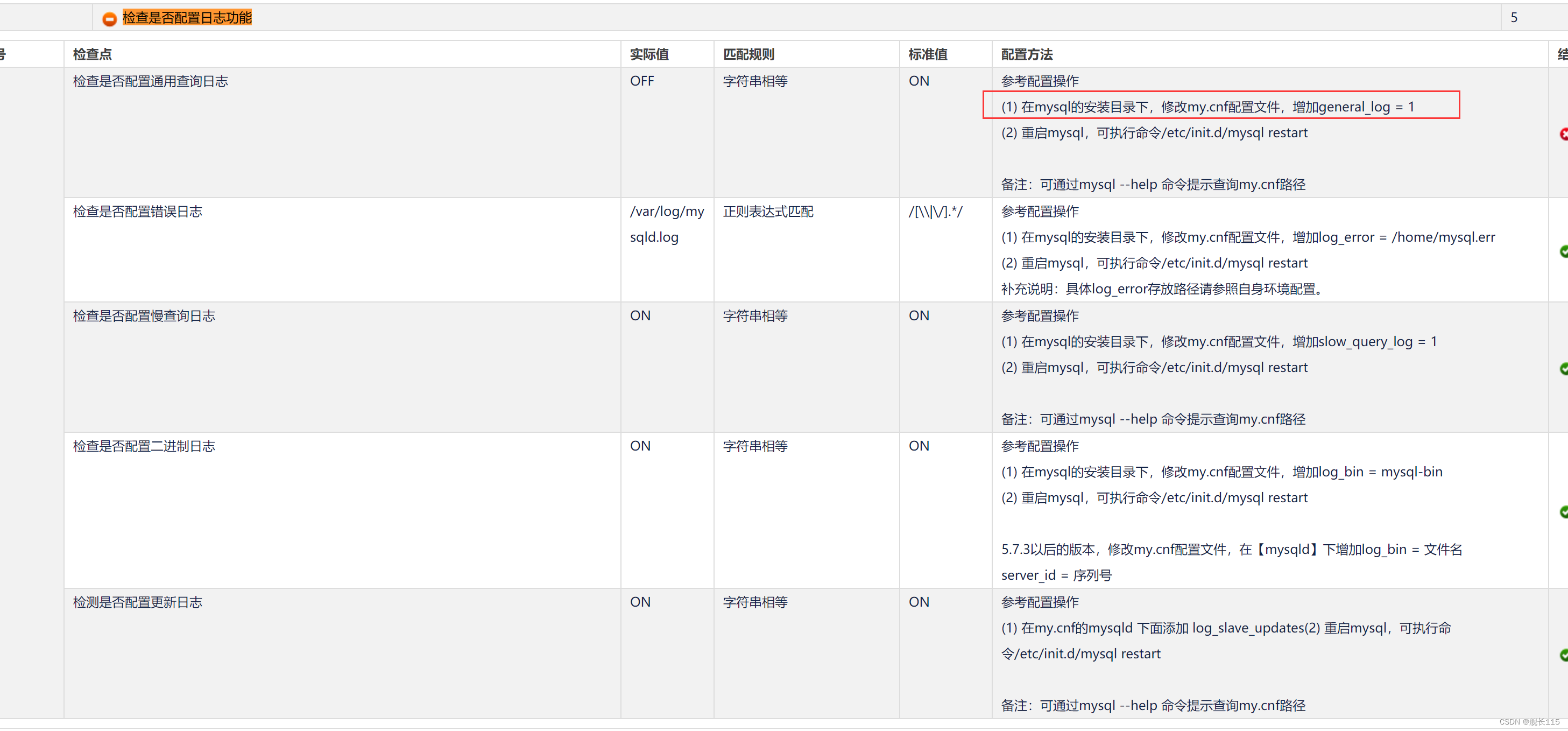
linux配置核查MySQL 配置规范 (Linux)_S3A3G3
linux的配置核查问题: 解决: 1.检查是否禁止mysql对本地文件存取 方法一:在my.cnf的mysql字段下加local-infile0 方法二:启动mysql时加参数local-infile0 /etc/init.d/mysql start --local-infile0 假如需要获取本地文件…...
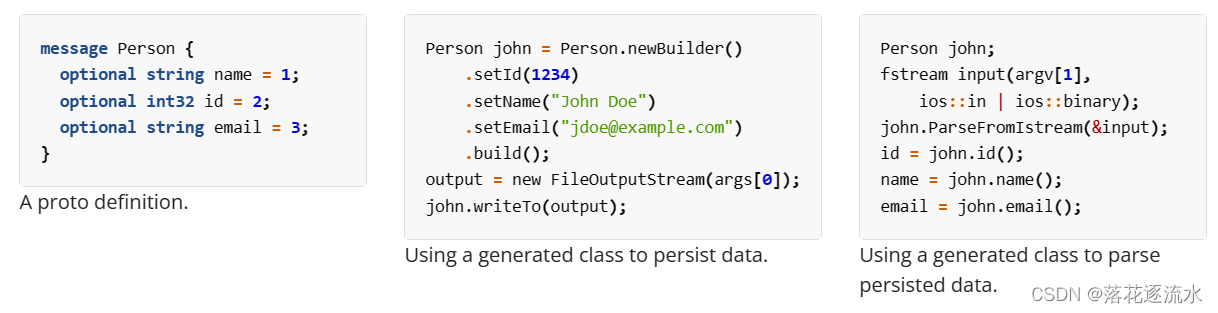
Protobuf简介
Protobuf简介 1. Protocol Buffers1.1. 什么是Protocol Buffers?1.2. 选择你最喜欢的语言1.3. 如何开始2. Protocol Buffer Basics: C++2.1. 问题领域2.2. 在哪里找到示例代码2.3. 定义协议格式(Defining Your Protocol Format)1. Protocol Buffers Protocol Buffers(协议缓冲…...
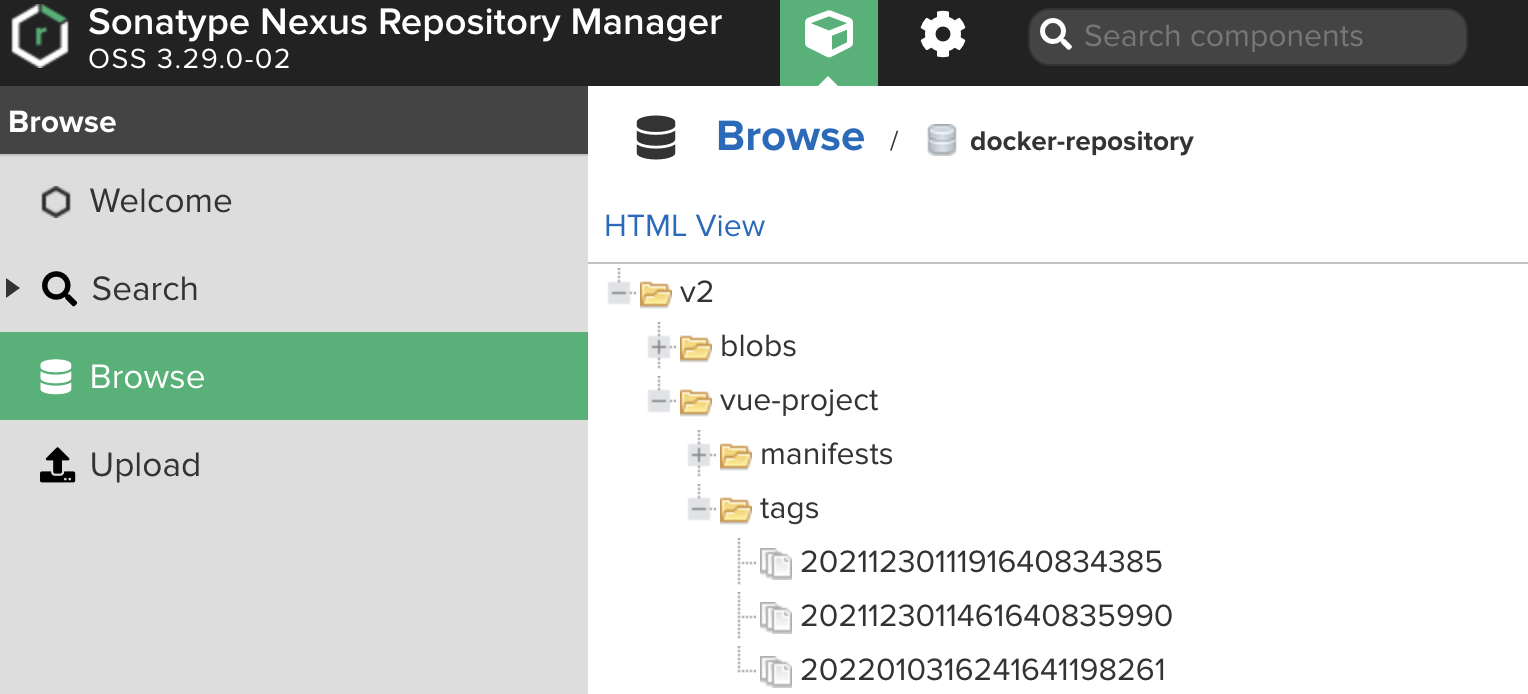
【Kubernetes】第十七篇 - ECS 服务停机和环境修复
一,前言 上一篇,介绍了 Secret 镜像的使用; 三台服务每天大概 15 块钱的支出,用一个月也是不少钱; 闲时可以停掉,这样每天只有 4 块钱支出,剩下一大笔; ECS 服务停机后公网 IP 会…...
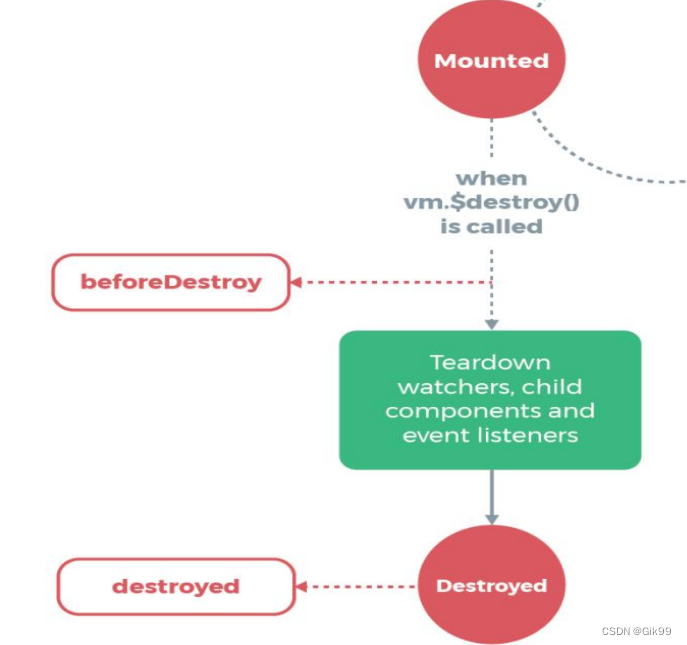
Vue2的生命周期(详解)
Vue的生命周期一、生命周期的概念二、钩子函数三、Vue2的生命周期3.1 初始化阶段3.2 挂载阶段3.3 更新阶段3.4 销毁阶段一、生命周期的概念 Vue实例的生命周期: 从创建到销毁的整个过程 二、钩子函数 Vue框架内置函数,随着组件的生命周期阶段,自动执行 作用:特定的时间点,执行特…...
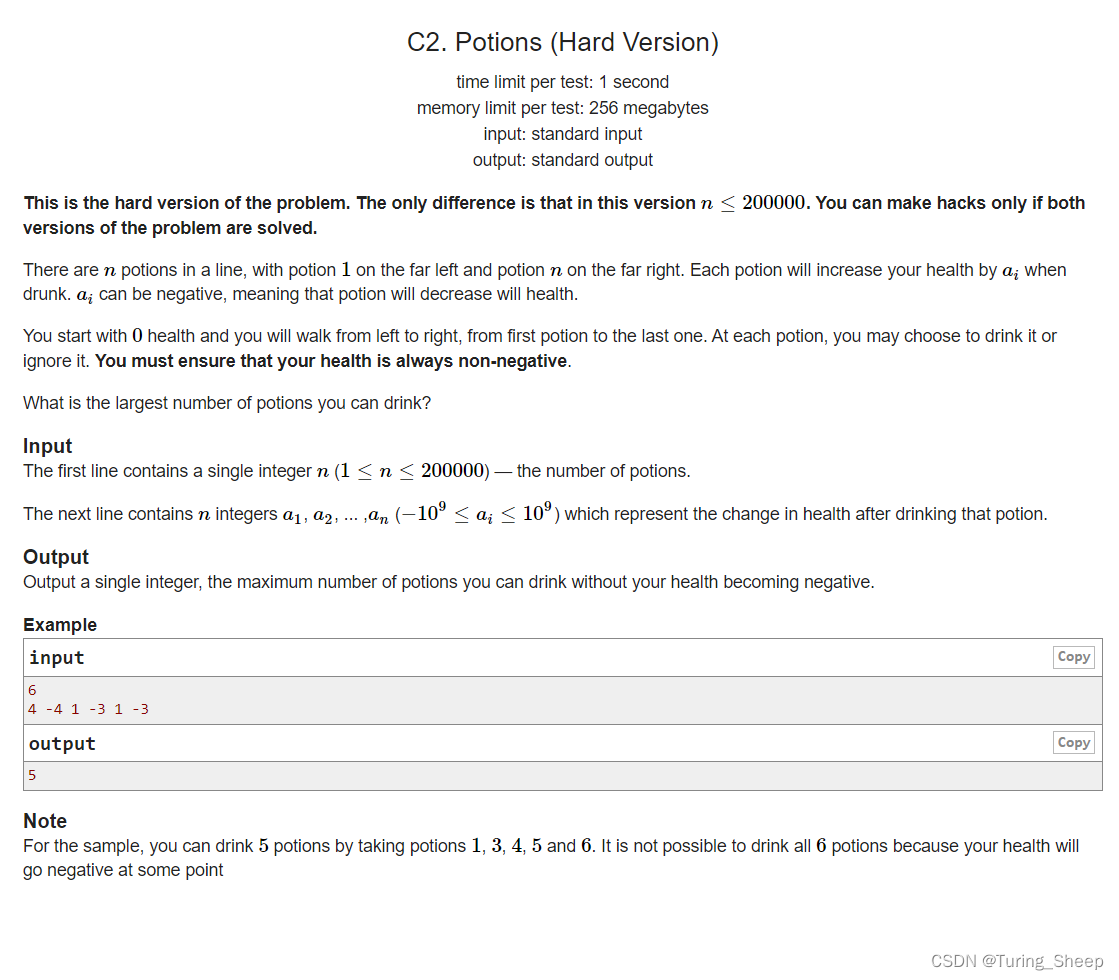
Potions (Hard Version) and (Easy Version)(背包DP + 反悔贪心)
[TOC](Potions (Hard Version) and (Easy Version)) 一、Potions(Easy Version) 1、问题 2、分析(背包DP 贪心) 简而言之就是我们需要从左到右开始选数字,选的过程中我们需要保证我们选的数字的和始终是大于等于0的,在满足这个…...
剑指 Offer II 017. 含有所有字符的最短字符串
题目链接 剑指 Offer II 017. 含有所有字符的最短字符串 hard 题目描述 给定两个字符串 s和 t。返回 s中包含 t的所有字符的最短子字符串。如果 s中不存在符合条件的子字符串,则返回空字符串 ""。 如果 s中存在多个符合条件的子字符串,返回任…...
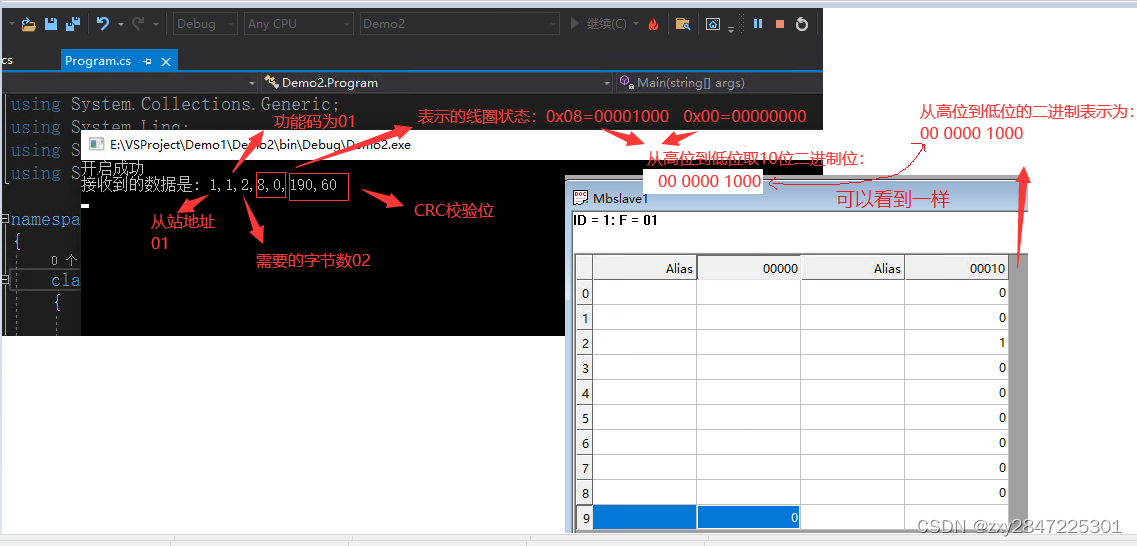
Modbus协议初探(C#实现)
由于作者水平有限,如有写得不对得地方请指正 趁着今天休息,就折腾一下Modbus协议,之前零零散散的看过几篇博客,听说搞上位机开发的要会这个协议,虽然我不是搞上位机开发的,但个人对这个比较感兴趣。按照我个…...
【华为OD机试2023】静态扫描 C++ Java Python
【华为OD机试2023】静态扫描 C++ Java Python 前言 如果您在准备华为的面试,期间有想了解的可以私信我,我会尽可能帮您解答,也可以给您一些建议! 本文解法非最优解(即非性能最优),不能保证通过率。 Tips1:机试为ACM 模式 你的代码需要处理输入输出,input/cin接收输入、…...
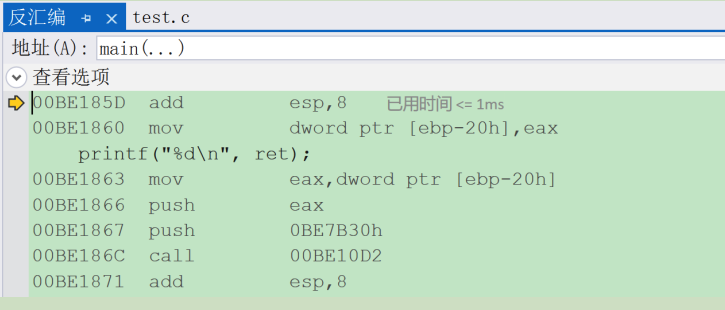
函数栈帧的创建和销毁(详解)
函数栈帧的创建和销毁🦖函数栈帧是什么?🦖函数栈帧的创建和销毁解析🐋栈是什么?🐋认识相关寄存器和汇编指令🐋解析函数栈帧的创建和销毁🐳预备知识🐳函数的调用堆栈&…...
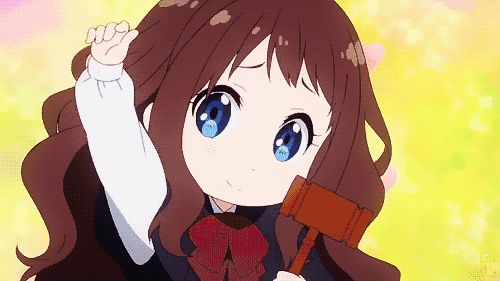
【100个 Unity实用技能】 | 脚本无需挂载到游戏对象上也可执行的方法
Unity 小科普 老规矩,先介绍一下 Unity 的科普小知识: Unity是 实时3D互动内容创作和运营平台 。包括游戏开发、美术、建筑、汽车设计、影视在内的所有创作者,借助 Unity 将创意变成现实。Unity 平台提供一整套完善的软件解决方案ÿ…...
条件期望5
条件期望例题 随机图 从节点1开始, N为一个随机变量, 表示整个过程第一次出现"贪吃蛇"情形时, 所进行的步数.即Nk⇒Xk(1)∈{1,X(1),X2(1),...Xk−1(1)}其中1,X(1),X2(1),...Xk−1(1)各不相同N k \Rightarrow X^k(1) \in \{1,X(1), X^2(1),...X^{k-1}(1)\} \\ 其中1…...
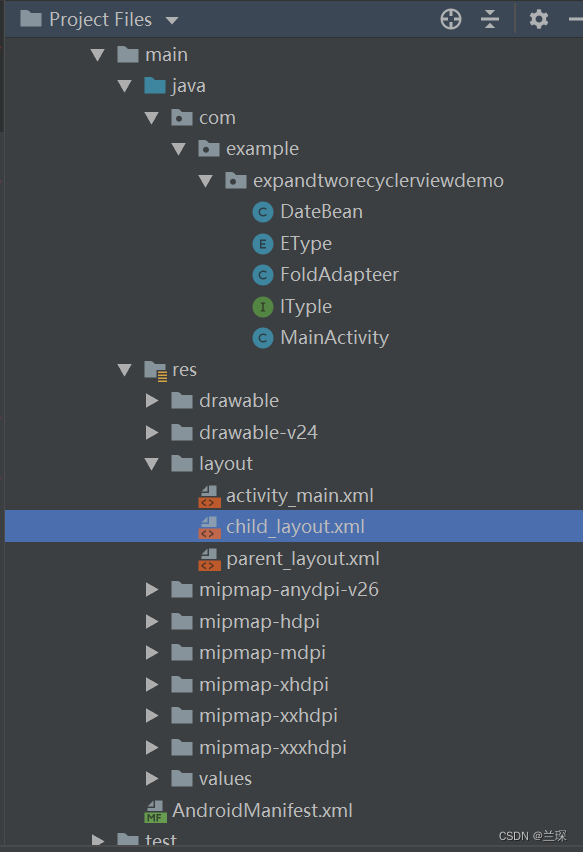
RecyclerView ViewType二级
实现效果描述: 1、点击recyclerview中item,列表下方出现其他样式的item,作为子item,如下所示 所需要的java文件和xml文件有: 1、创建FoldAdapteradapter, 在FoldAdapter中,定义两种不同的类型ÿ…...

将对象或数组存在 dom元素的属性上,最后取不到完整数据,只取到 [{
目录 一、问题 二、问题及解决方法 三、总结 一、问题 1.我需要在dom元素里面添加了一个属性test存一个对象数组temp,以便我下一次找到这个dom元素时可以直接拿到属性里面的数据来渲染页面。 2.dom 属性上存 对象和数组,必须先JSON.stringify(arr),转…...
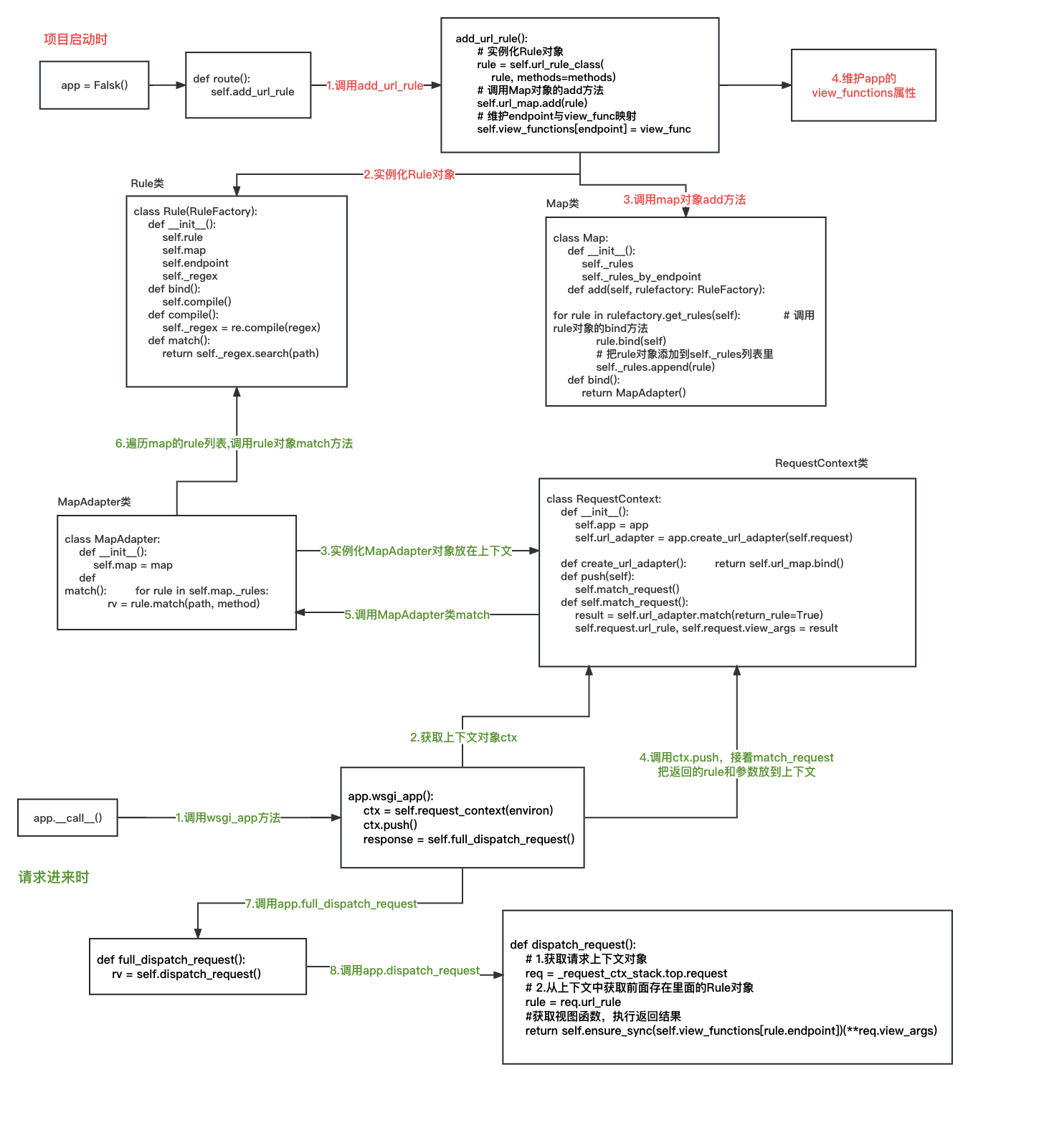
Flask源码篇:Flask路由规则与请求匹配过程(超详细,易懂)
目录1 启动时路由相关操作(1)分析app.route()(2)分析add_url_rule()(3)分析Rule类(4)分析Map类(5)分析MapAdapter类(6)分析 url_rule_…...

Jmeter接口测试教程之【参数化技巧总结】,总有一个是你不知道的
目录:导读 一、随机值 二、随机字符串 三、时间戳 四、唯一字符串UUID 说起接口测试,相信大家在工作中用的最多的还是Jmeter。 大家看这个目录就知道jmeter的应用有多广泛了:https://www.bilibili.com/video/BV1e44y1X78S/? JMeter是一个…...
RestClient
什么是RestClient RestClient 是 Elasticsearch 官方提供的 Java 低级 REST 客户端,它允许HTTP与Elasticsearch 集群通信,而无需处理 JSON 序列化/反序列化等底层细节。它是 Elasticsearch Java API 客户端的基础。 RestClient 主要特点 轻量级ÿ…...
ES6从入门到精通:前言
ES6简介 ES6(ECMAScript 2015)是JavaScript语言的重大更新,引入了许多新特性,包括语法糖、新数据类型、模块化支持等,显著提升了开发效率和代码可维护性。 核心知识点概览 变量声明 let 和 const 取代 var…...
React Native 导航系统实战(React Navigation)
导航系统实战(React Navigation) React Navigation 是 React Native 应用中最常用的导航库之一,它提供了多种导航模式,如堆栈导航(Stack Navigator)、标签导航(Tab Navigator)和抽屉…...
CRMEB 框架中 PHP 上传扩展开发:涵盖本地上传及阿里云 OSS、腾讯云 COS、七牛云
目前已有本地上传、阿里云OSS上传、腾讯云COS上传、七牛云上传扩展 扩展入口文件 文件目录 crmeb\services\upload\Upload.php namespace crmeb\services\upload;use crmeb\basic\BaseManager; use think\facade\Config;/*** Class Upload* package crmeb\services\upload* …...
【碎碎念】宝可梦 Mesh GO : 基于MESH网络的口袋妖怪 宝可梦GO游戏自组网系统
目录 游戏说明《宝可梦 Mesh GO》 —— 局域宝可梦探索Pokmon GO 类游戏核心理念应用场景Mesh 特性 宝可梦玩法融合设计游戏构想要素1. 地图探索(基于物理空间 广播范围)2. 野生宝可梦生成与广播3. 对战系统4. 道具与通信5. 延伸玩法 安全性设计 技术选…...
Pinocchio 库详解及其在足式机器人上的应用
Pinocchio 库详解及其在足式机器人上的应用 Pinocchio (Pinocchio is not only a nose) 是一个开源的 C 库,专门用于快速计算机器人模型的正向运动学、逆向运动学、雅可比矩阵、动力学和动力学导数。它主要关注效率和准确性,并提供了一个通用的框架&…...
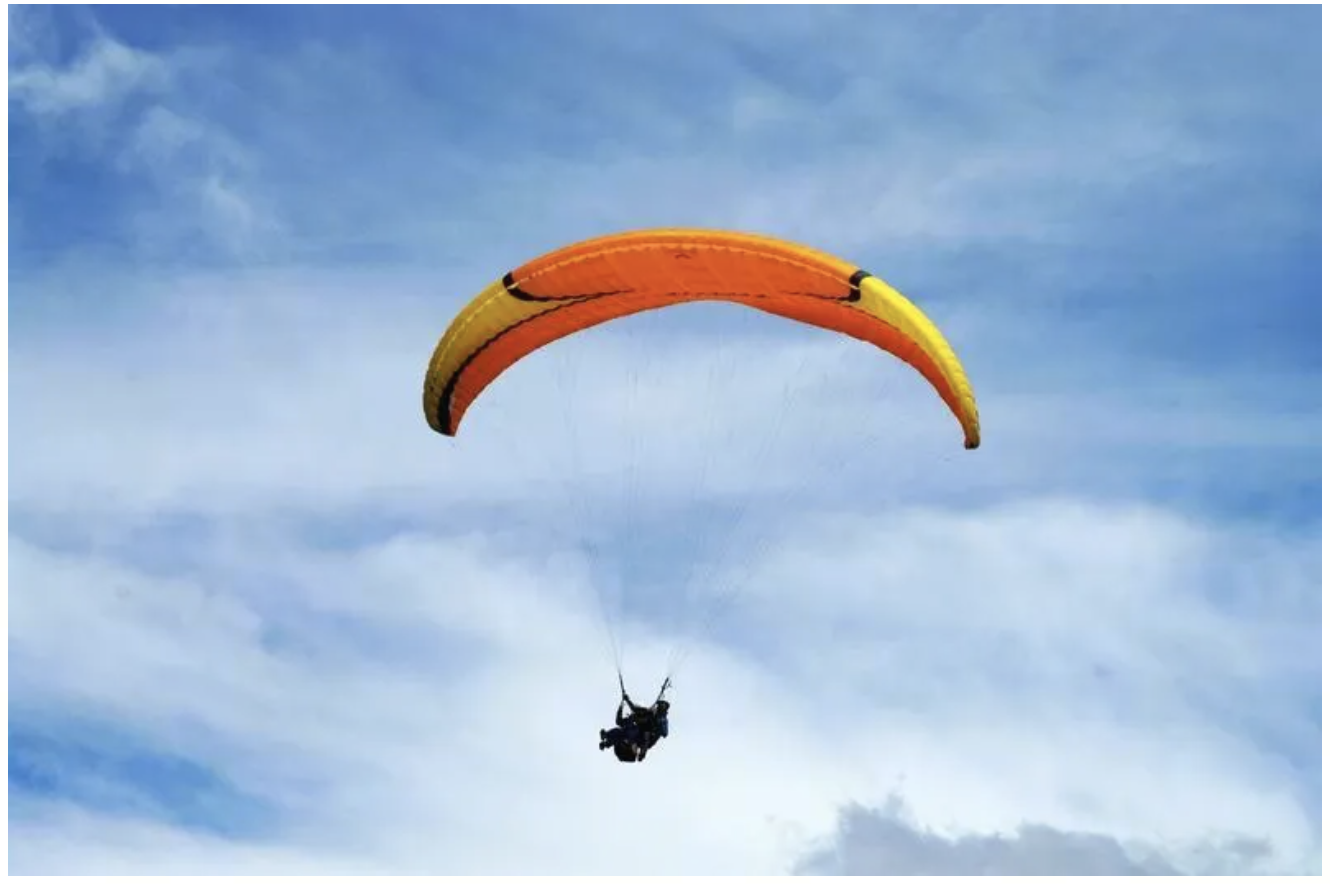
数学建模-滑翔伞伞翼面积的设计,运动状态计算和优化 !
我们考虑滑翔伞的伞翼面积设计问题以及运动状态描述。滑翔伞的性能主要取决于伞翼面积、气动特性以及飞行员的重量。我们的目标是建立数学模型来描述滑翔伞的运动状态,并优化伞翼面积的设计。 一、问题分析 滑翔伞在飞行过程中受到重力、升力和阻力的作用。升力和阻力与伞翼面…...
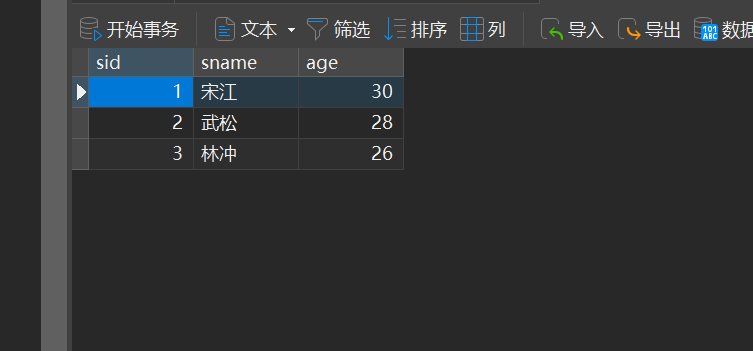
MySQL的pymysql操作
本章是MySQL的最后一章,MySQL到此完结,下一站Hadoop!!! 这章很简单,完整代码在最后,详细讲解之前python课程里面也有,感兴趣的可以往前找一下 一、查询操作 我们需要打开pycharm …...
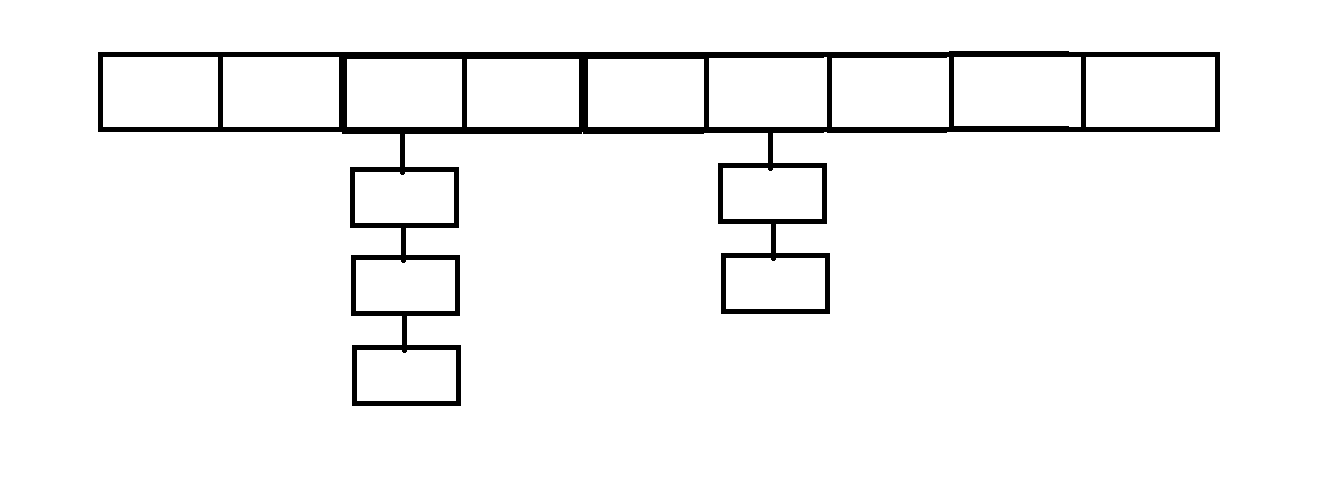
C++_哈希表
本篇文章是对C学习的哈希表部分的学习分享 相信一定会对你有所帮助~ 那咱们废话不多说,直接开始吧! 一、基础概念 1. 哈希核心思想: 哈希函数的作用:通过此函数建立一个Key与存储位置之间的映射关系。理想目标:实现…...
前端调试HTTP状态码
1xx(信息类状态码) 这类状态码表示临时响应,需要客户端继续处理请求。 100 Continue 服务器已收到请求的初始部分,客户端应继续发送剩余部分。 2xx(成功类状态码) 表示请求已成功被服务器接收、理解并处…...