How to run Flutter on an Embedded Device
basysKom GmbH | How to run Flutter on an Embedded Device
https://github.com/sony/flutter-embedded-linux/wiki/Building-Flutter-Engine-from-source
flutter源码下载(最新)-CSDN博客
flutter_engine 交叉编译【自定义编译器(最新)】_flutter。engine 修改-CSDN博客
flutter移植arm32位板子_flutter框架 移植-CSDN博客
Essential Summary
In this blog we will cross compile Flutter for a generic ARMv7 Embedded Linux target. We will prepare the sdk, compile flutter and in the end run some demos.
Implementing cross platform HMIs is something we at basysKom do on a daily basis. Usually we use either Qt/QML or Web Technologies, but we are always looking at a broader spectrum of options to provide our customers the best possible solution.
Flutter is one of those other options. It is an UI Framework based on Dart, made by Google. While the primary focus of Flutter are mobile platforms like iOS and Android with a growing support for the Web, Flutter is also heading towards Linux and Windows. Interesting to know is that Google uses Flutter to provide the HMI for their own embedded devices and that it will become even more important once Fuchsia does succeed Android, since it will be the primary Ui Framework of Fuchsia.
So I wondered if it is possible to run Flutter on an UX-Gruppe MACH platform (which is an iMX.6 SOC). The short answer is: Yes it is possible.
In this blog I will explain how you can setup everything to compile and run Flutter for and on a generic ARMv7 Embedded Linux target. Since I do not know which hardware you may have at hand, I need to assume that you will be able to fill the gaps. An i.MX6 with a recent Buildroot or Yocto BSP is a good starting point.
I will show you the basic dependencies and command lines you need in order to cross-compile Flutter for ARMv7. What you can also expect is a general instruction of what needs to be done in order to finally run a Flutter App on your platform (after you compiled the engine).
Hardware Requirements
The mentioned specs are simply the spec of the hardware I used, they are not necessarily the minimum hardware requirements of Flutter on Embedded.
As mentioned, I use our own UX-Gruppe Hardware, an Ultratronic Mach Platform evaluation board with a single core iMX.6 and 1 GB of Memory. The ARMv7 iMX.6 comes with an integrated graphic chip. The touch-display draws in 1280x800px with 60hz.
SDK Requirements
All my work is done on a Thinkpad X1, running Windows 10 with an Ubuntu 18.04 executed within the Windows Linux Subsystem Version 1.0. You can of course simply run Linux natively, it’s your choice.
The UX-Gruppe hardware comes with a Buildroot Embedded Linux and an SDK/cross-toolchain for ARMv7 containing Clang/LLVM.
Make sure you have an SDK in place that is, in general, able to cross-compile to ARMv7. Ideally your SDK already supports Clang/LLVM, if not, you can of course try to build it on your own, including the required build-tools. Though this is not a trivial task and it may take you a moment or two.
Preparing Clang/LLVM Toolchain for your SDK
This is a little sidetrack since I didn’t actually needed to do it. This is something that you may only need to follow in case your SDK does either not come with any, or a not a recent enough Clang/LLVM support. Expect some stormy weather here, it all depends on the state of the SDK you are using to do this. You should know what you do since I can only give you orientation but not the path.
Get and build Clang/LLVM
To be sure you use the latest instructions, you can find them here. Ensure your host fulfills the general dependencies. Of course you need cmake, git, ssh,… .
#Setup working directory
cd ~
mkdir flutter-exp
cd flutter-exp#Clone llvm
git clone https://github.com/llvm/llvm-project.git
cd llvm-project#create build directory
mkdir build
cd build#Build the TOOLCHAIN
cmake ../llvm \-DLLVM_TARGETS_TO_BUILD=ARM \-DLLVM_DEFAULT_TARGET_TRIPLE=arm-linux-gnueabihf \-DCMAKE_BUILD_TYPE=Release \-DCMAKE_INSTALL_PREFIX=/PATH/TO/YOUR/SDKmake # consider some -j
make install
Get and compile Bintools
#Setup working directory
cd ~
#mkdir flutter-exp
cd flutter-expgit clone git://sourceware.org/git/binutils-gdb.gitcd binutils-gdb./configure --prefix="/PATH/TO/YOUR/SDK" \--enable-ld \--target=arm-linux-gnueabihf
make
make install
Get and compile libcxx and libcxxabi
This might be the most troublesome part. In order to avoid issues you want to be sure that the libc you are using to build the engine is new enough to support the requirements of the flutter engine.
In order to avoid breaking your embedded system by introducing a new libc I recommend to compile and link and provide them as static lib.
You already have checked out sources for both together with llvm-project.
Build libcxxabi
cd ~
cd flutter-exp
cd llvm-project
cd build cmake ../llvm/projects/libcxxabi \-DCMAKE_CROSSCOMPILING=True \-DLLVM_TARGETS_TO_BUILD=ARM \-DCMAKE_SYSROOT=/PATH/TO/YOUR/SDK/sysroot #NOTICE SYSROOT HERE! \-DCMAKE_INSTALL_PREFIX=/PATH/TO/YOUR/SDK \-DCMAKE_BUILD_TYPE=Release \-DCMAKE_SYSTEM_NAME=Linux \-DCMAKE_SYSTEM_PROCESSOR=ARM \-DCMAKE_C_COMPILER=/PATH/TO/YOUR/SDK/bin/clang \-DCMAKE_CXX_COMPILER=/PATH/TO/YOUR/SDK/bin/clang++ \-DLIBCXX_ENABLE_SHARED=False \-DLIBCXXABI_ENABLE_EXCEPTIONS=False
make # consider some -j
make check-cxx # Test
make install-cxxabi
Build libcxx
cd ~
cd flutter-exp
cd llvm-project
cd build # you may have trouble here because __cxxabi_config.h
# and cxxabi.h are not placed in /PATH/TO/YOUR/SDK/include/c++/v1
# find them and copy them there.cmake ../llvm/projects/libcxx \-DCMAKE_CROSSCOMPILING=True \-DLLVM_TARGETS_TO_BUILD=ARM \-DCMAKE_SYSROOT=/PATH/TO/YOUR/SDK/sysroot #NOTICE SYSROOT HERE! \-DCMAKE_INSTALL_PREFIX=/PATH/TO/YOUR/SDK \-DCMAKE_BUILD_TYPE=Release \-DCMAKE_SYSTEM_NAME=Linux \-DCMAKE_SYSTEM_PROCESSOR=ARM \-DCMAKE_C_COMPILER=/PATH/TO/YOUR/SDK/bin/clang \-DCMAKE_CXX_COMPILER=/PATH/TO/YOUR/SDK/bin/clang++ -DLIBCXX_ENABLE_SHARED=False \-DLIBCXX_ENABLE_EXCEPTIONS=False \-DLIBCXX_CXX_ABI=libcxxabi \-DLIBCXX_CXX_ABI_INCLUDE_PATHS=/PATH/TO/YOUR/SDK/include/c++/v1 \-DLIBCXX_CXX_ABI_LIBRARY_PATH=/PATH/TO/YOUR/SDK/lib \-DLIBCXX_ENABLE_STATIC_ABI_LIBRARY=True
make # consider some -j
make check-cxx # Test
make install-cxx
Congrats! If you managed to get here with(out) trouble, you are all set to build your own Flutter-Engine.
Cross Compiling Flutter for ARM
Setup your Environment
First, get the Chromium depot tools.
cd ~
mkdir flutter-expgit clone https://chromium.googlesource.com/chromium/tools/depot_tools.git#consider this to be added to your ~/.bashrc
export PATH=~/flutter-exp/depot_tools:$PATH
Then, follow the instructions of the setup development environment page of Flutter.
You have to follow Step 1 up to Step 6. Ignore Step 7, 8, 9. The last step you should do is to add a remote for the upstream repository.
Compile the Engine
Following along the instructions for desktop Linux we will now compile the engine.
cd engine
cd src./flutter/tools/gn \ --target-toolchain /PATH/TO/YOUR/SDK \--target-sysroot /PATH/TO/YOUR/SDK/sysroot \--target-triple arm-linux-gnueabihf \--arm-float-abi hard \--linux-cpu arm \--runtime-mode debug \--embedder-for-target \--no-lto \ --target-os linuxninja -C out/linux_debug_arm
If you run into issue like missing *.o
files during linking you do experience an issue most likely caused by libgcc not being installed correctly within the sysroot of the sdk.
You may want to make a coffee (or two) while it compiles…
Once you are done and everything is built, create a good and save location on your disk and copy
libflutter_engine.so
,icudtl.dat
andflutter_embedder.h
from out/linux_debug_arm
to your target disk.
You can check with file libflutter_engine.so
that you have created an ARM ELF file.
The Embedder
The application which provides the flutter engine an opengl context and access to system resources is called “the embedder”.
A very light-weight starting point can be found here. The instructions are made for a raspberry pi + compiling on the pi. I will point out what we need to cross-compile in our more general case.
Make sure the compiler and linker can find libflutter_engine.so
and flutter_embedded.h
.
git clone https://github.com/andyjjones28/flutter-pi.git
cd flutter-pi
mkdir out/PATH/TO/YOUR/SDK/bin/arm-linux-gnueabihf-cc -D_GNU_SOURCE \
-lEGL \
-ldrm \
-lgbm \
-lGLESv2 \
-lrt \
-lflutter_engine \
-lpthread \
-ldl \
-I./include \
-I/usr/include \
-I/usr/include/libdrm \
./src/flutter-pi.c \
./src/platformchannel.c \
./src/pluginregistry.c \
./src/plugins/services-plugin.c \
-o out/flutter-pi
How to run your Flutter App
Now that you have built it, you sure want to verify its working, right?
Prepare your device
First you have to copy the libflutter_engine.so
and the icudtl.dat
along with the flutter-pi
to your target hardware.
Prepare the app code
For some samples, take a look here in the sample repository. Please note that many of them require deeper support, support that the sample embedder does not provide to them. You may also (depending on your system) experience some ssl certificate issues on some demos.
What should work with the embedder is the nice background particle demo.
Once you got the sources you will have to patch the main function in ./lib/main.dart
. By adding debugDefaultTargetPlatformOverride = TargetPlatform.fuchsia;
before the runApp
call.
// ADD THIS IMPORT
import 'package:flutter/foundation.dart';// ...... void main() {debugDefaultTargetPlatformOverride = TargetPlatform.fuchsia;runApp(MyApp());
}
Bundle the app
For this step you have to install flutter on your host system (if you have not done this already). It’s a really awesome experience, don’t worry.
If you have a flutter dev setup on your host. Go to your sample directory and call:
# cd flutter-exampleflutter build bundle
And now copy it to your target, next to your embedder.
Run the app
The time has come to flutter on your target. For more detailed instructions just take a look in the README of the runner repo. You can also pass flutter engine flags along by adding them after the bundle directory.
/path/to/assets/bundle/directory
is the path of the flutter asset bundle directory (i.e. the directory containing the kernel_blob.bin) of the flutter app you’re trying to run.
./flutter-pi -t /dev/input0 /path/to/assets/bundle/directory
And now you should see your Flutter App on your target device display, along with many additional information on your terminal.
Live capture
Add Your Heading Text Here
Conclusion
After testing and experimenting with Flutter on the iMX.6, I was really surprised by the performance you get with an unoptimized embedder within a debug build. I did not spent much time on profiling, so I can not provide you hard numbers, but it really feels like a very good start.
On the negative side, creating and maintaining an embedder with the required features is hard groundwork. This is definitely not something for juniors, since you have to get down into some dirt where even experienced developers will need to take a sip of coffee first.
On the plus side, you will get a very light-weight, fast and flexible environment to run your app in. This app can run on iOS, Android, Desktop, the Web (Javascript!) and of course on your device.
Together with the ability to use the SKIA backend and render in software Flutter should be able to run on very low end devices and render even there some basic HMI’s.
Flutter is definitely an option to take into consideration if you plan to build a multi platform HMI with a relative small dependency footprint and a very friendly BSD-3 open source license.
I hope you enjoyed this adventure into the world of Flutter like I did. If you have any suggestion or question just drop a comment down below.
Further reads
There are some great resources found in the internet that helped me to put down this article.
- Flutter on RaspberryPi
- Flutter on Toradex
- Flutter Pi
相关文章:
How to run Flutter on an Embedded Device
basysKom GmbH | How to run Flutter on an Embedded Device https://github.com/sony/flutter-embedded-linux/wiki/Building-Flutter-Engine-from-source flutter源码下载(最新)-CSDN博客 flutter_engine 交叉编译【自定义编译器(最新)】_flutter。engine 修改-CSDN博客 …...
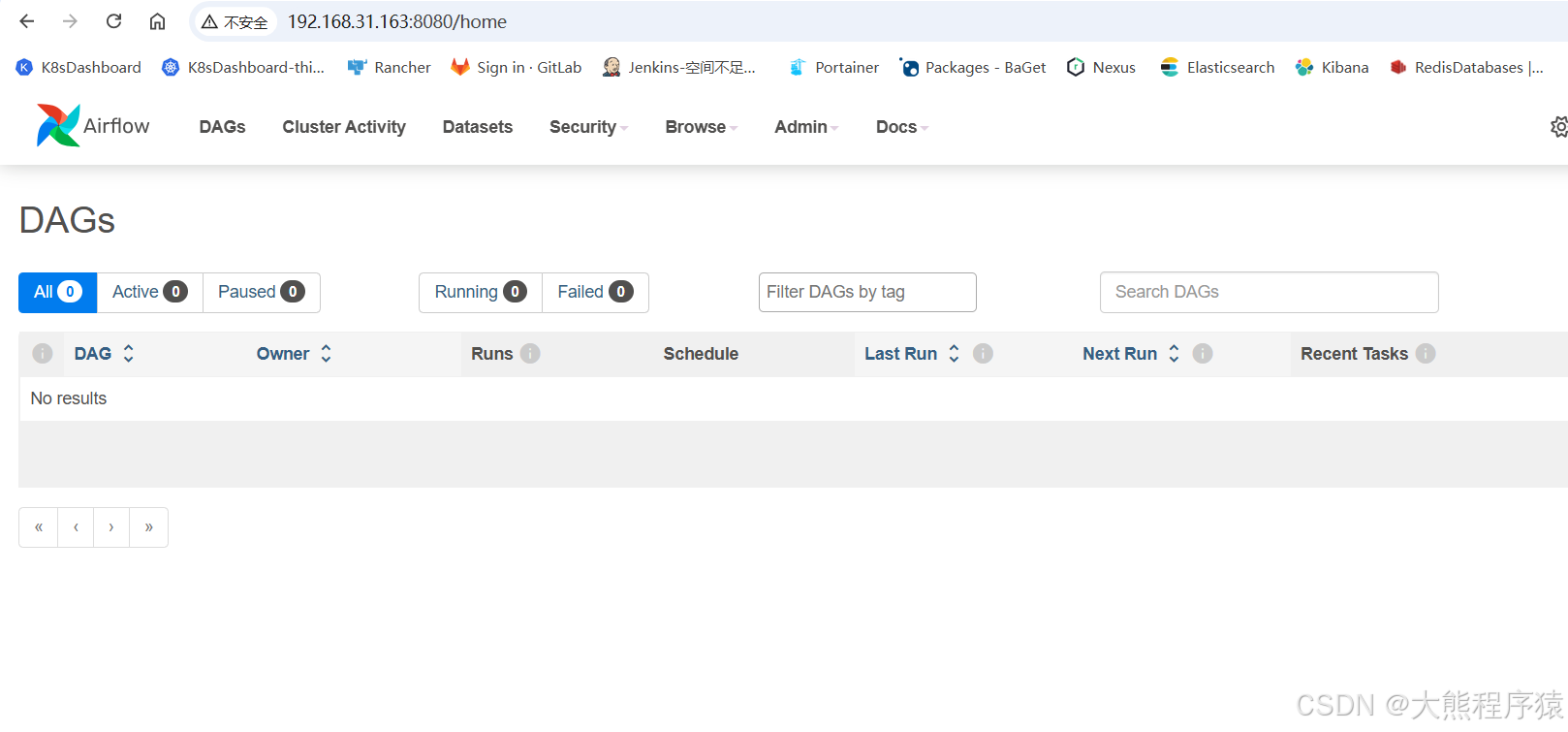
airflow docker 安装
mkdir -p /root/airflow cd /root/airflow && mkdir -p ./dags ./logs ./plugins ./configcd /root/airflow/ wget https://airflow.apache.org/docs/apache-airflow/2.10.4/docker-compose.yaml nano docker-compose.yamlAIRFLOW__CORE__LOAD_EXAMPLES: false #初始化…...
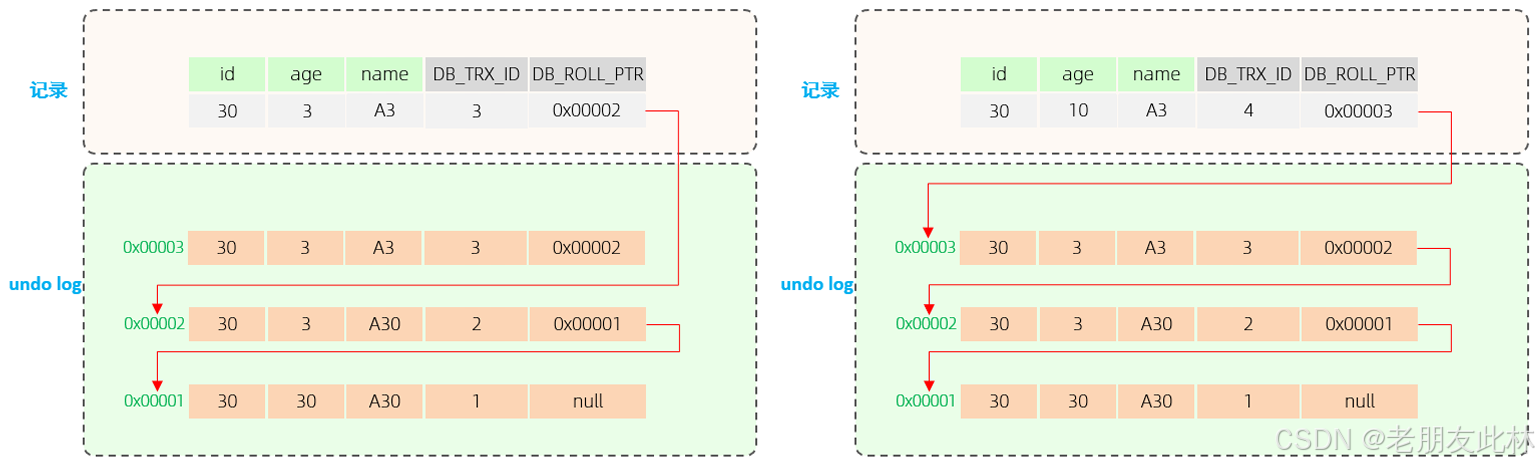
浅析InnoDB引擎架构(已完结)
大家好,我是此林。 今天来介绍下InnoDB底层架构。 1. 磁盘架构 我们所有的数据库文件都保存在 /var/lib/mysql目录下。 由于我这边是docker部署的mysql,用如下命令查看mysql数据挂载。 docker inspect mysql-master 如下图,目前只有一个数…...
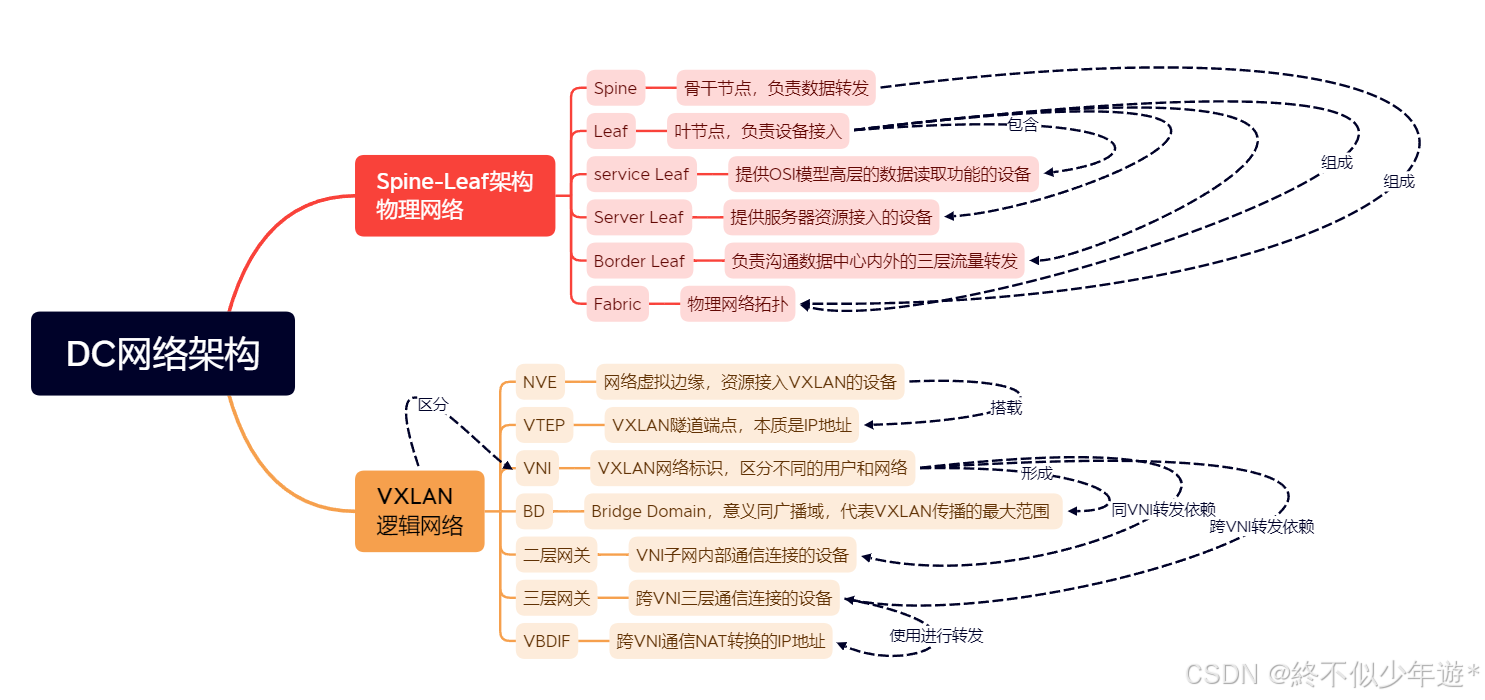
华为云计算HCIE笔记02
第二章:华为云Stack规划设计 交付总流程 准备工作:了解客户的基本现场,并且对客户的需求有基本的认知。 HLD方案BOQ报价设备采购和设备上架 2.安装部署流程 硬件架构设计 硬件设备选配 设备上架与初始化配置 准备相关资料(自动下载…...
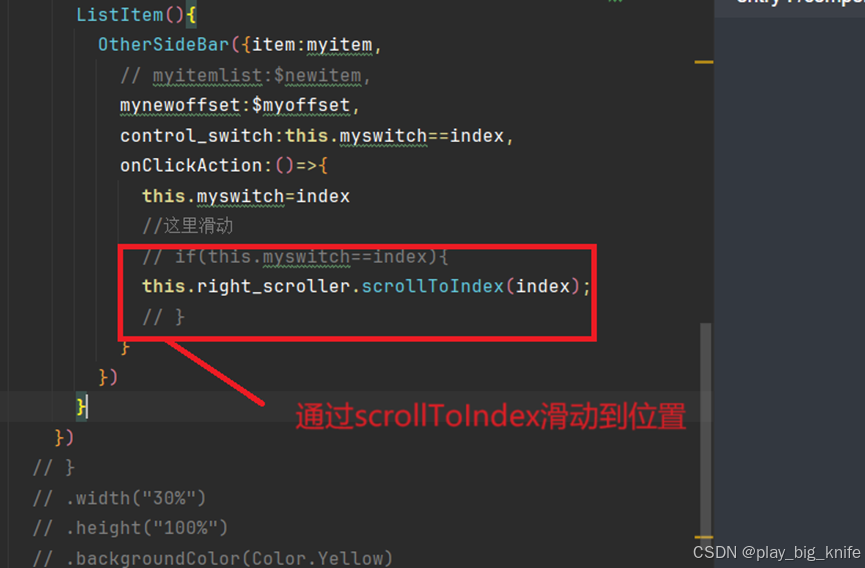
鸿蒙项目云捐助第十讲鸿蒙App应用分类页面二级联动功能实现
鸿蒙项目云捐助第十讲鸿蒙App应用分类页面二级联动功能实现 在之前的教程中完成了分类页面的左右两侧的列表结构,如下图所示。 接下来需要实现左侧分类导航项的点击操作,可以友好的提示用户选择了哪一个文字分类导航项。 一、左侧文字分类导航的处理 …...
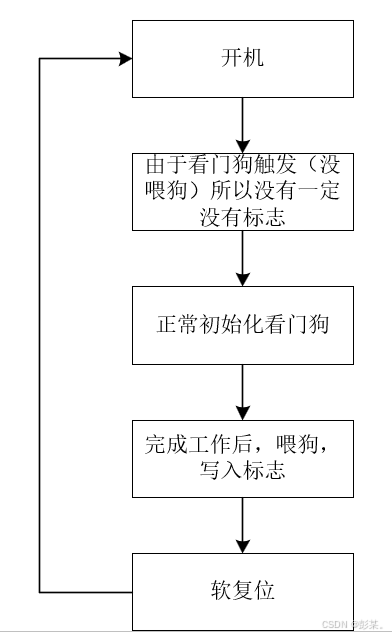
STM32低功耗模式结合看门狗
STM32低功耗模式结合看门狗 前言 最近做到一个需求要使用STM32的低功耗模式进行长时间待机应用,每隔十分钟发送一次数据到服务器上,当不发送的时候就处于低功耗模式。在经过一段时间的测试以后发现板子过三四天左右就没有数据上传服务器了,…...
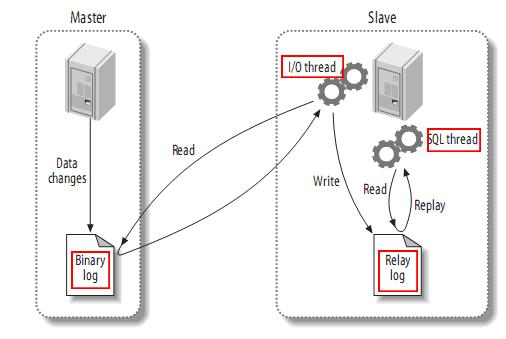
数据迁移工具,用这8种!
前言 最近有些小伙伴问我,ETL数据迁移工具该用哪些。 ETL(是Extract-Transform-Load的缩写,即数据抽取、转换、装载的过程),对于企业应用来说,我们经常会遇到各种数据的处理、转换、迁移的场景。 今天特地给大家汇总了一些目前…...
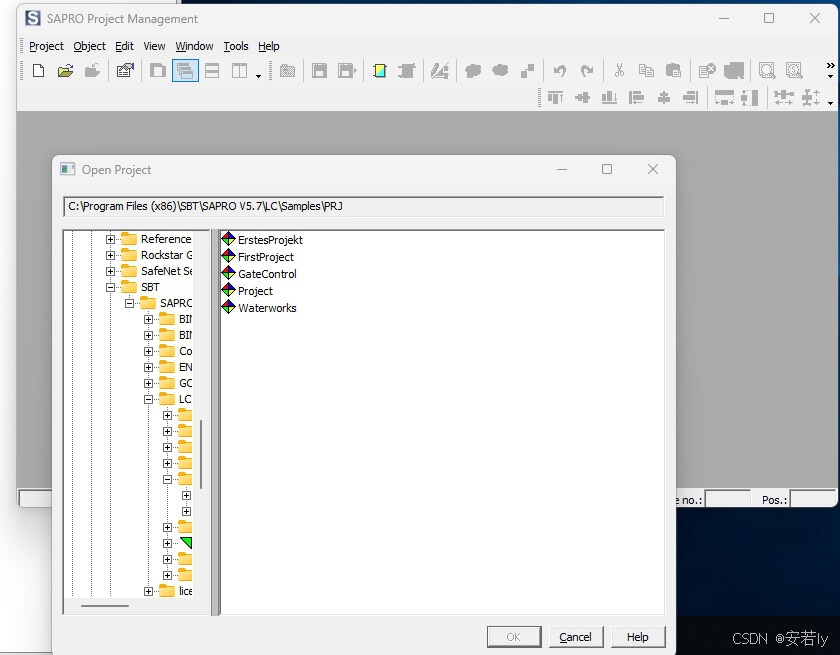
Sapro编程软件
Sapro软件是由西门子建筑科技公司开发的一款编程软件,主要用于Climatix控制器的编程、调试及相关功能实现.以下是其具体介绍: • 功能强大:可进行HVAC控制编程,实现设备控制、HMI用户访问和设备集成等功能,满足复杂的…...

Python图注意力神经网络GAT与蛋白质相互作用数据模型构建、可视化及熵直方图分析...
全文链接:https://tecdat.cn/?p38617 本文聚焦于图注意力网络GAT在蛋白质 - 蛋白质相互作用数据集中的应用。首先介绍了研究背景与目的,阐述了相关概念如归纳设置与转导设置的差异。接着详细描述了数据加载与可视化的过程,包括代码实现与分析…...
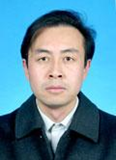
2024年图像处理、多媒体技术与机器学习
重要信息 官网:www.ipmml.org 时间:2024年12月27-29日 地点:中国-大理 简介 2024年图像处理、多媒体技术与机器学习(CIPMT 2024)将于2024年12月27-29日于中国大理召开。将围绕图像处理与多媒体技术、机器学习等在…...
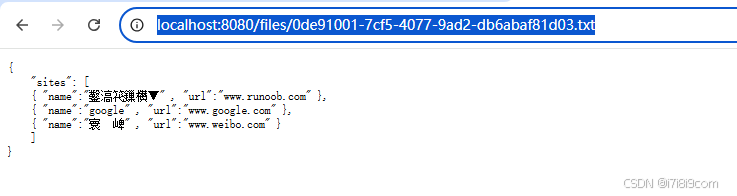
java 1.8+springboot文件上传+vue3+ts+antdv
1.参考 使用SpringBoot优雅的实现文件上传_51CTO博客_springboot 上传文件 2.postman测试 报错 :postman调用时body参数中没有file单词 Resolved [org.springframework.web.multipart.support.MissingServletRequestPartException: Required request part file is…...
【机器人】机械臂轨迹和转矩控制对比
动力学控制和轨迹跟踪控制是机器人控制中的两个概念,它们在目标、方法和应用上有所不同,但也有一定关联。以下是它们的区别和联系: 1. 动力学控制 动力学控制是基于机器人动力学模型的控制方法,目标是控制机器人关节力矩或力&…...
如何利用矩阵化简平面上的二次型曲线
二次型曲线的定义 在二维欧式平面上,一个二次型曲线都可以写成一个关于 x , y x,y x,y的二元二次多项式: F ( x , y ) a 11 x 2 2 a 12 x y a 22 y 2 2 a 1 x 2 a 2 y a 0 0 \begin{equation} F(x,y)a_{11}x^22a_{12}xya_{22}y^22a_1x2a_2ya_00…...
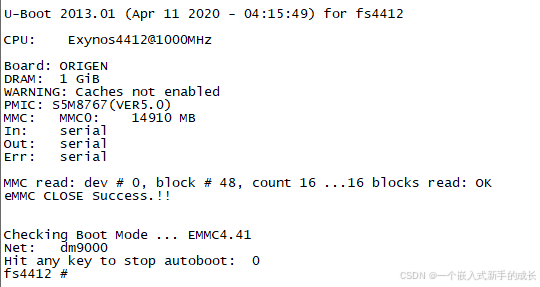
【系统移植】制作SD卡启动——将uboot烧写到SD卡
在开发板上启动Linux内核,一般有两种方法,一种是从EMMC启动,还有一种就是从SD卡启动,不断哪种启动方法,当开发板上电之后,首先运行的是uboot。 制作SD卡启动,首先要将uboot烧写到SD卡ÿ…...
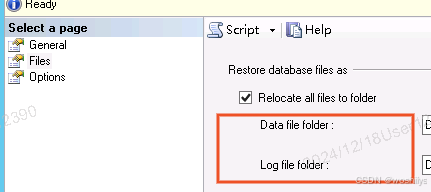
sql server 数据库还原,和数据检查
右键数据库选择还原, 还原的备份文件必须选择在本地的文件(远程文件没有试过)还原数据库名字可以修改,然后file选择中有个2个目录data file 的目录 ,和log data 的目录都可以重新选择还原到的新的目录,不要…...
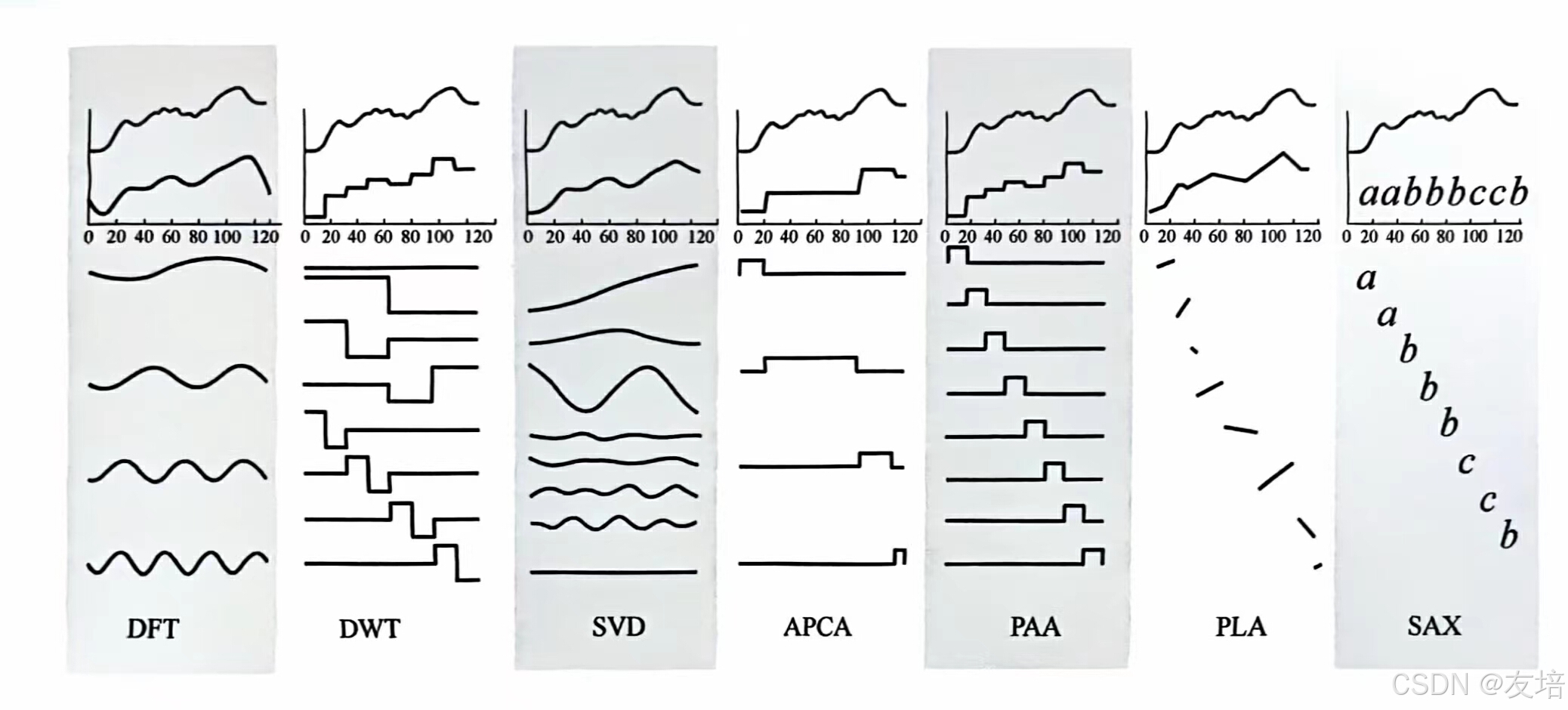
工业大数据分析算法实战-day12
文章目录 day12时序分解STL(季节性趋势分解法)奇异谱分析(SSA)经验模态分解(EMD) 时序分割ChangpointTreeSplitAutoplait有价值的辅助 时序再表征 day12 今天是第12天,昨天主要是针对信号处理算…...
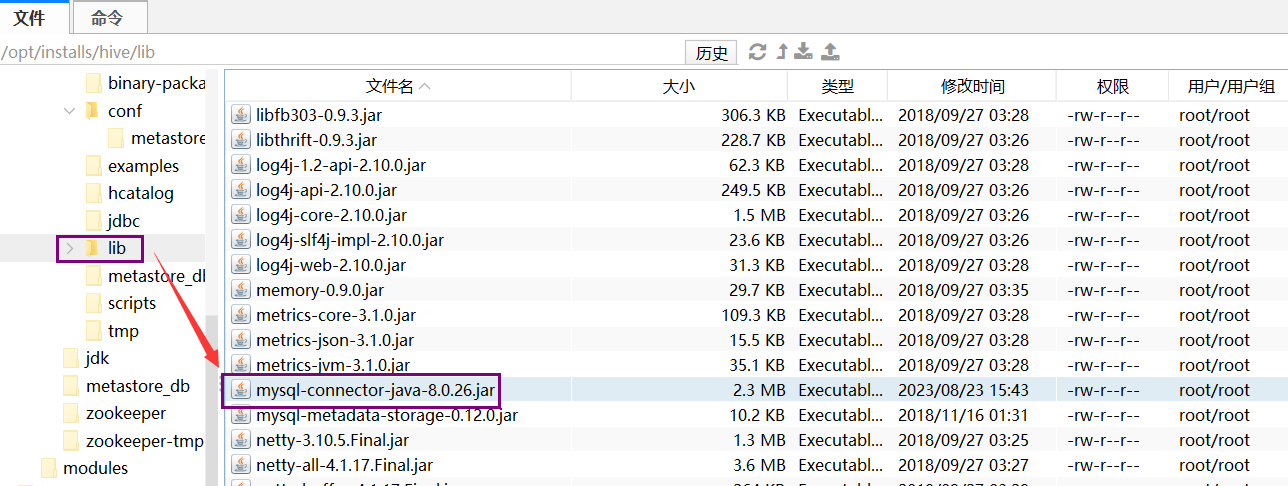
Hive其一,简介、体系结构和内嵌模式、本地模式的安装
目录 一、Hive简介 二、体系结构 三、安装 1、内嵌模式 2、测试内嵌模式 3、本地模式--最常使用的模式 一、Hive简介 Hive 是一个框架,可以通过编写sql的方式,自动的编译为MR任务的一个工具。 在这个世界上,会写SQL的人远远大于会写ja…...
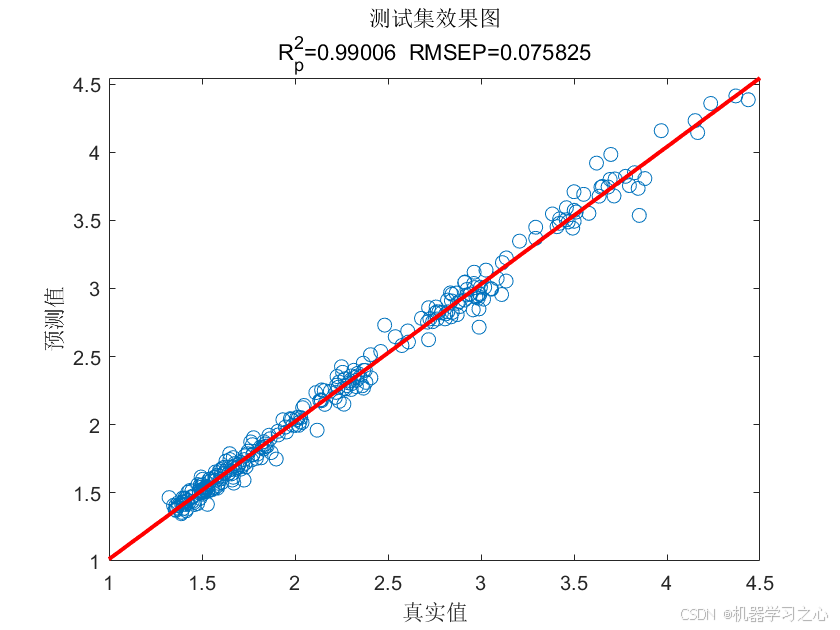
LSTM-SVM时序预测 | Matlab基于LSTM-SVM基于长短期记忆神经网络-支持向量机时间序列预测
LSTM-SVM时序预测 | Matlab基于LSTM-SVM基于长短期记忆神经网络-支持向量机时间序列预测 目录 LSTM-SVM时序预测 | Matlab基于LSTM-SVM基于长短期记忆神经网络-支持向量机时间序列预测预测效果基本介绍程序设计参考资料 预测效果 基本介绍 1.LSTM-SVM时序预测 | Matlab基于LSTM…...
MacPorts 中安装高/低版本软件方式,以 RabbitMQ 为例
查询信息 这里以 RabbitMQ 为例,通过搜索得到默认安装版本信息: port search rabbitmq-server结果 ~/Downloads> port search rabbitmq-server rabbitmq-server 3.11.15 (net)The RabbitMQ AMQP Server ~/Downloads>获取二进制文件 但当前官网…...
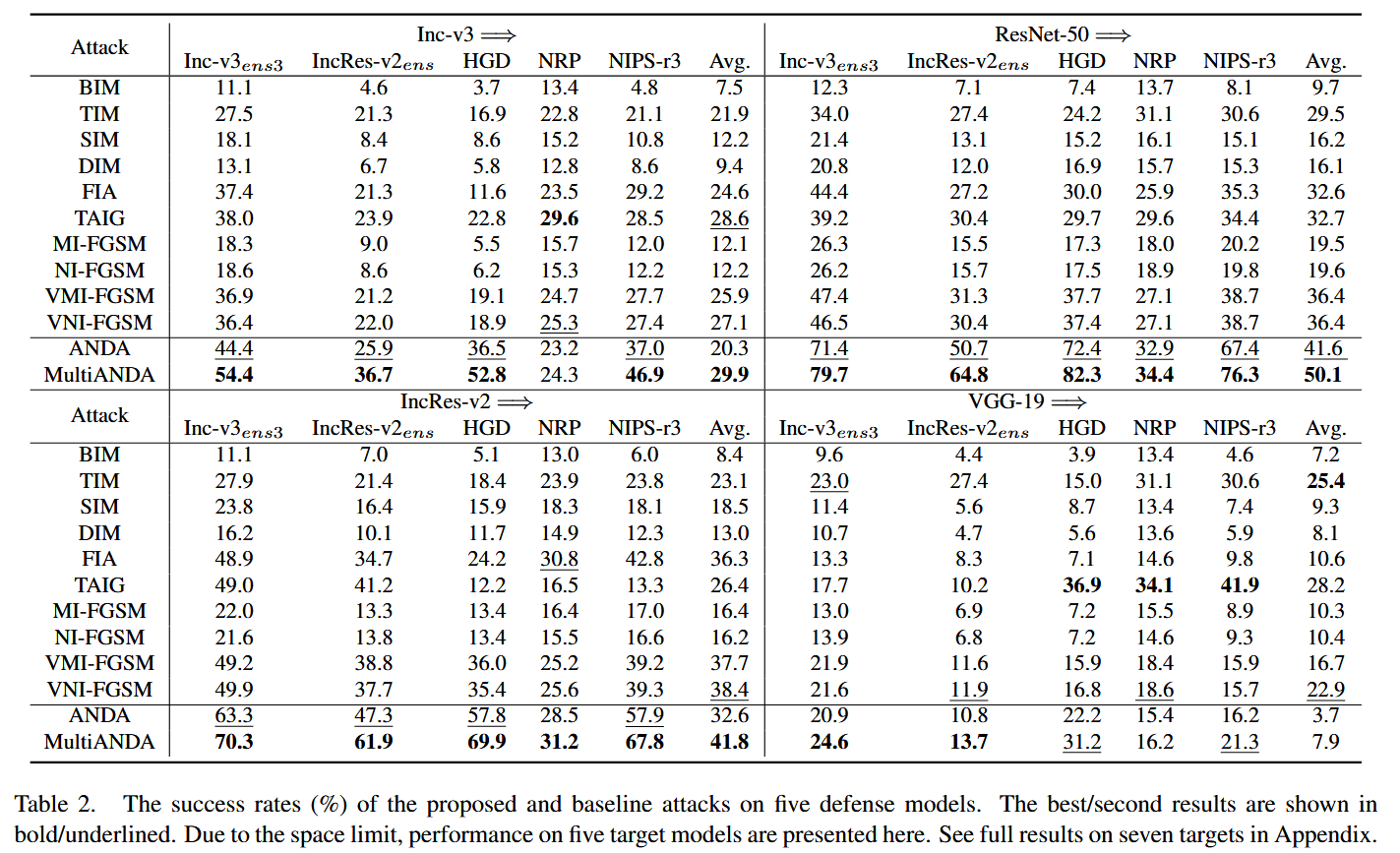
CVPR2024 | 通过集成渐近正态分布学习实现强可迁移对抗攻击
Strong Transferable Adversarial Attacks via Ensembled Asymptotically Normal Distribution Learning 摘要-Abstract引言-Introduction相关工作及前期准备-Related Work and Preliminaries1. 黑盒对抗攻击2. SGD的渐近正态性 提出的方法-Proposed Method随机 BIM 的渐近正态…...
基于算法竞赛的c++编程(28)结构体的进阶应用
结构体的嵌套与复杂数据组织 在C中,结构体可以嵌套使用,形成更复杂的数据结构。例如,可以通过嵌套结构体描述多层级数据关系: struct Address {string city;string street;int zipCode; };struct Employee {string name;int id;…...
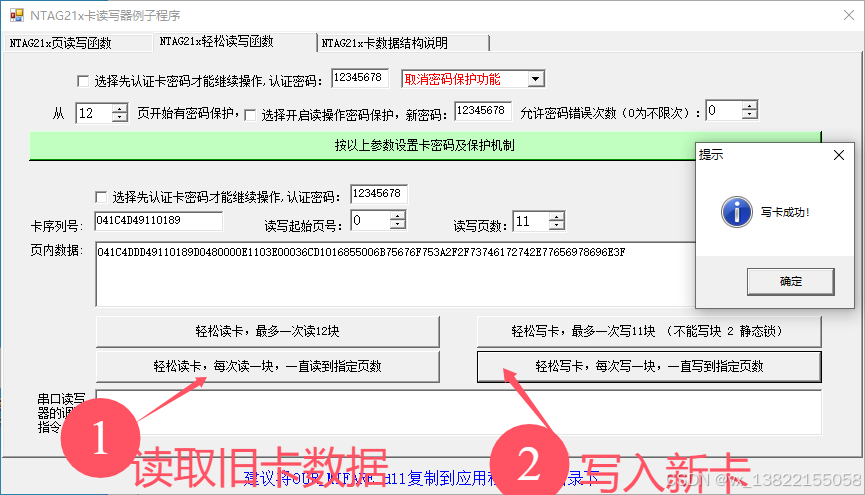
VB.net复制Ntag213卡写入UID
本示例使用的发卡器:https://item.taobao.com/item.htm?ftt&id615391857885 一、读取旧Ntag卡的UID和数据 Private Sub Button15_Click(sender As Object, e As EventArgs) Handles Button15.Click轻松读卡技术支持:网站:Dim i, j As IntegerDim cardidhex, …...
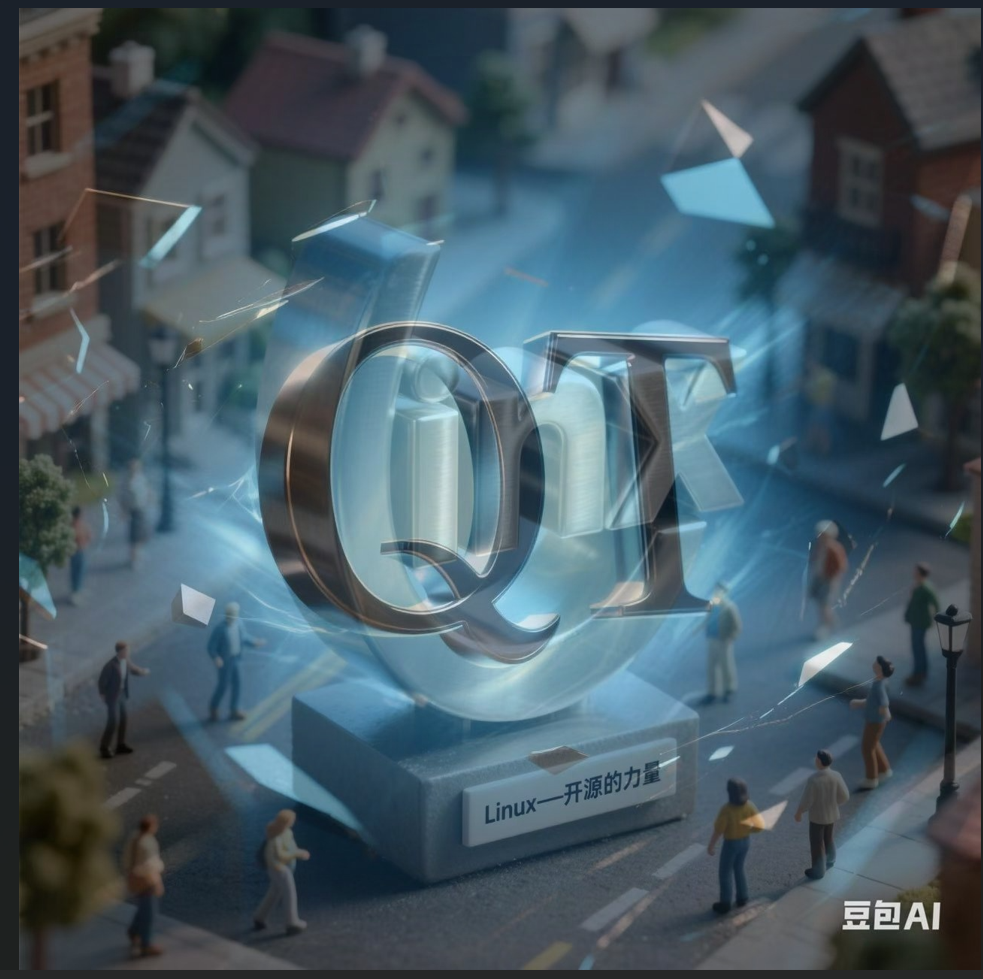
Opencv中的addweighted函数
一.addweighted函数作用 addweighted()是OpenCV库中用于图像处理的函数,主要功能是将两个输入图像(尺寸和类型相同)按照指定的权重进行加权叠加(图像融合),并添加一个标量值&#x…...
Java编程之桥接模式
定义 桥接模式(Bridge Pattern)属于结构型设计模式,它的核心意图是将抽象部分与实现部分分离,使它们可以独立地变化。这种模式通过组合关系来替代继承关系,从而降低了抽象和实现这两个可变维度之间的耦合度。 用例子…...
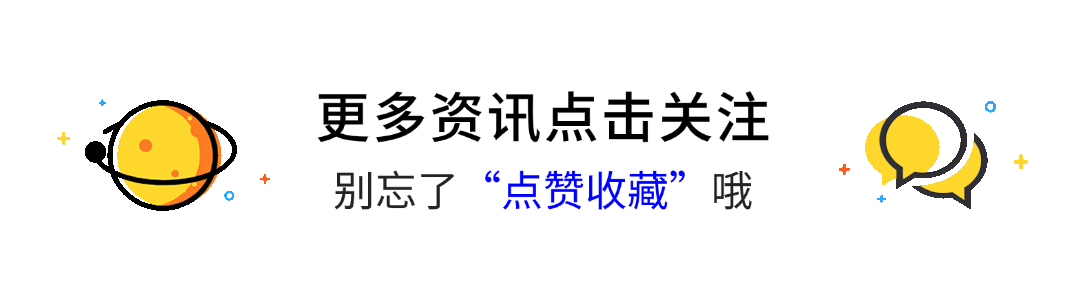
AI+无人机如何守护濒危物种?YOLOv8实现95%精准识别
【导读】 野生动物监测在理解和保护生态系统中发挥着至关重要的作用。然而,传统的野生动物观察方法往往耗时耗力、成本高昂且范围有限。无人机的出现为野生动物监测提供了有前景的替代方案,能够实现大范围覆盖并远程采集数据。尽管具备这些优势…...
CSS | transition 和 transform的用处和区别
省流总结: transform用于变换/变形,transition是动画控制器 transform 用来对元素进行变形,常见的操作如下,它是立即生效的样式变形属性。 旋转 rotate(角度deg)、平移 translateX(像素px)、缩放 scale(倍数)、倾斜 skewX(角度…...
适应性Java用于现代 API:REST、GraphQL 和事件驱动
在快速发展的软件开发领域,REST、GraphQL 和事件驱动架构等新的 API 标准对于构建可扩展、高效的系统至关重要。Java 在现代 API 方面以其在企业应用中的稳定性而闻名,不断适应这些现代范式的需求。随着不断发展的生态系统,Java 在现代 API 方…...
LangFlow技术架构分析
🔧 LangFlow 的可视化技术栈 前端节点编辑器 底层框架:基于 (一个现代化的 React 节点绘图库) 功能: 拖拽式构建 LangGraph 状态机 实时连线定义节点依赖关系 可视化调试循环和分支逻辑 与 LangGraph 的深…...
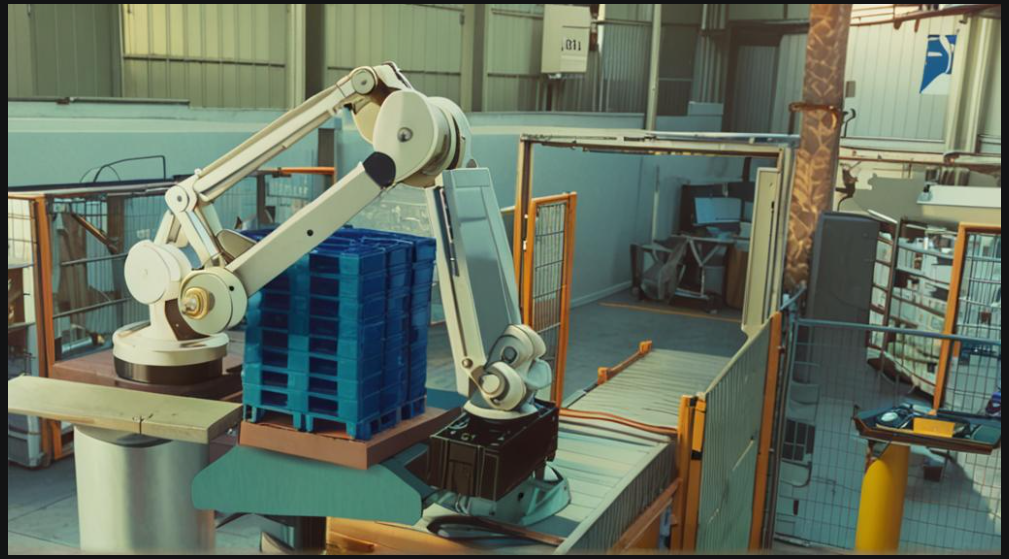
【堆垛策略】设计方法
堆垛策略的设计是积木堆叠系统的核心,直接影响堆叠的稳定性、效率和容错能力。以下是分层次的堆垛策略设计方法,涵盖基础规则、优化算法和容错机制: 1. 基础堆垛规则 (1) 物理稳定性优先 重心原则: 大尺寸/重量积木在下…...
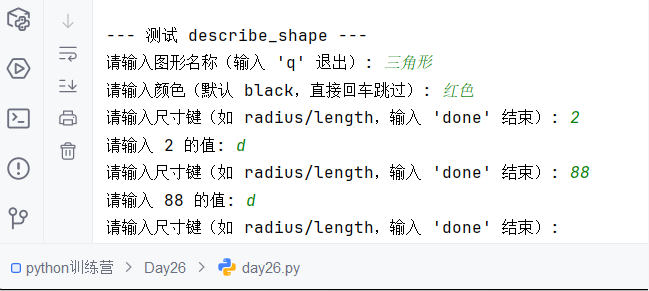
Python训练营-Day26-函数专题1:函数定义与参数
题目1:计算圆的面积 任务: 编写一个名为 calculate_circle_area 的函数,该函数接收圆的半径 radius 作为参数,并返回圆的面积。圆的面积 π * radius (可以使用 math.pi 作为 π 的值)要求:函数接收一个位置参数 radi…...